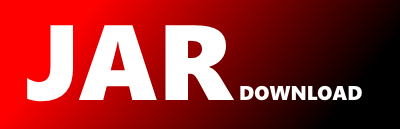
com.oracle.truffle.js.nodes.access.JSHasPropertyNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of js-language Show documentation
Show all versions of js-language Show documentation
Graal JavaScript implementation
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import com.oracle.truffle.js.nodes.IntToLongTypeSystemGen;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.array.TypedArrayLengthNode;
import com.oracle.truffle.js.nodes.array.TypedArrayLengthNodeGen;
import com.oracle.truffle.js.nodes.cast.JSToPropertyKeyNode;
import com.oracle.truffle.js.nodes.cast.JSToStringNode;
import com.oracle.truffle.js.nodes.interop.ForeignObjectPrototypeNode;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.JSRuntime;
import com.oracle.truffle.js.runtime.Strings;
import com.oracle.truffle.js.runtime.Symbol;
import com.oracle.truffle.js.runtime.array.ScriptArray;
import com.oracle.truffle.js.runtime.builtins.JSClass;
import com.oracle.truffle.js.runtime.builtins.JSTypedArrayObject;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSHasPropertyNode#arrayLongCached}
* Activation probability: 0.15909
* With/without class size: 7/4 bytes
* Specialization {@link JSHasPropertyNode#arrayLong}
* Activation probability: 0.14545
* With/without class size: 6/0 bytes
* Specialization {@link JSHasPropertyNode#typedArray}
* Activation probability: 0.13182
* With/without class size: 7/5 bytes
* Specialization {@link JSHasPropertyNode#objectStringCached}
* Activation probability: 0.11818
* With/without class size: 7/12 bytes
* Specialization {@link JSHasPropertyNode#arrayStringCached}
* Activation probability: 0.10455
* With/without class size: 6/8 bytes
* Specialization {@link JSHasPropertyNode#objectString}
* Activation probability: 0.09091
* With/without class size: 5/0 bytes
* Specialization {@link JSHasPropertyNode#objectSymbol}
* Activation probability: 0.07727
* With/without class size: 4/0 bytes
* Specialization {@link JSHasPropertyNode#objectLong}
* Activation probability: 0.06364
* With/without class size: 4/0 bytes
* Specialization {@link JSHasPropertyNode#foreignObject}
* Activation probability: 0.05000
* With/without class size: 5/16 bytes
* Specialization {@link JSHasPropertyNode#foreignObject}
* Activation probability: 0.03636
* With/without class size: 5/12 bytes
* Specialization {@link JSHasPropertyNode#objectObject}
* Activation probability: 0.02273
* With/without class size: 4/4 bytes
*
*/
@GeneratedBy(JSHasPropertyNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class JSHasPropertyNodeGen extends JSHasPropertyNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField STATE_0_JSHasPropertyNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField ARRAY_LONG_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "arrayLongCached_cache", ArrayLongCachedData.class);
static final ReferenceField OBJECT_STRING_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "objectStringCached_cache", ObjectStringCachedData.class);
static final ReferenceField ARRAY_STRING_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "arrayStringCached_cache", ArrayStringCachedData.class);
static final ReferenceField FOREIGN_OBJECT0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "foreignObject0_cache", ForeignObject0Data.class);
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#arrayLongCached}
* Parameter: {@link InlinedConditionProfile} hasElementProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_HAS_ELEMENT_PROFILE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_UPDATER.subUpdater(13, 2)));
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#typedArray}
* Parameter: {@link TypedArrayLengthNode} typedArrayLengthNode
* Inline method: {@link TypedArrayLengthNodeGen#inline}
*/
private static final TypedArrayLengthNode INLINED_TYPED_ARRAY_TYPED_ARRAY_LENGTH_NODE_ = TypedArrayLengthNodeGen.inline(InlineTarget.create(TypedArrayLengthNode.class, STATE_0_JSHasPropertyNode_UPDATER.subUpdater(15, 7), ReferenceField.create(MethodHandles.lookup(), "typedArray_typedArrayLengthNode__field1_", Node.class)));
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link JSHasPropertyNode#arrayLongCached}
* 1: SpecializationActive {@link JSHasPropertyNode#arrayLong}
* 2: SpecializationActive {@link JSHasPropertyNode#typedArray}
* 3: SpecializationActive {@link JSHasPropertyNode#objectStringCached}
* 4: SpecializationActive {@link JSHasPropertyNode#objectString}
* 5: SpecializationActive {@link JSHasPropertyNode#arrayStringCached}
* 6: SpecializationActive {@link JSHasPropertyNode#objectSymbol}
* 7: SpecializationActive {@link JSHasPropertyNode#objectLong}
* 8: SpecializationActive {@link JSHasPropertyNode#foreignObject}
* 9: SpecializationActive {@link JSHasPropertyNode#foreignObject}
* 10: SpecializationActive {@link JSHasPropertyNode#objectObject}
* 11-12: ImplicitCast[type=long, index=1]
* 13-14: InlinedCache
* Specialization: {@link JSHasPropertyNode#arrayLongCached}
* Parameter: {@link InlinedConditionProfile} hasElementProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 15-21: InlinedCache
* Specialization: {@link JSHasPropertyNode#typedArray}
* Parameter: {@link TypedArrayLengthNode} typedArrayLengthNode
* Inline method: {@link TypedArrayLengthNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#objectStringCached}
* Parameter: {@link EqualNode} equalNode
*/
@Child private EqualNode equalNode;
@UnsafeAccessedField @Child private ArrayLongCachedData arrayLongCached_cache;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#typedArray}
* Parameter: {@link TypedArrayLengthNode} typedArrayLengthNode
* Inline method: {@link TypedArrayLengthNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node typedArray_typedArrayLengthNode__field1_;
@UnsafeAccessedField @Child private ObjectStringCachedData objectStringCached_cache;
@UnsafeAccessedField @Child private ArrayStringCachedData arrayStringCached_cache;
@UnsafeAccessedField @Child private ForeignObject0Data foreignObject0_cache;
@Child private ForeignObject1Data foreignObject1_cache;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#objectObject}
* Parameter: {@link JSToPropertyKeyNode} toPropertyKeyNode
*/
@Child private JSToPropertyKeyNode objectObject_toPropertyKeyNode_;
private JSHasPropertyNodeGen(boolean hasOwnProperty) {
super(hasOwnProperty);
}
@ExplodeLoop
@Override
public boolean executeBoolean(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111111) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] || SpecializationActive[JSHasPropertyNode.objectStringCached(JSDynamicObject, TruffleString, JSClass, TruffleString, HasPropertyCacheNode, EqualNode)] || SpecializationActive[JSHasPropertyNode.arrayStringCached(JSDynamicObject, TruffleString, TruffleString, HasPropertyCacheNode, EqualNode)] || SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] || SpecializationActive[JSHasPropertyNode.objectSymbol(JSDynamicObject, Symbol)] || SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */) {
if ((state_0 & 0b111) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] */ && IntToLongTypeSystemGen.isImplicitLong((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=long, index=1] */, arg1Value)) {
long arg1Value_ = IntToLongTypeSystemGen.asImplicitLong((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=long, index=1] */, arg1Value);
if ((state_0 & 0b11) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] */ && (JSGuards.isJSFastArray(arg0Value_)) && (JSRuntime.isArrayIndex(arg1Value_))) {
ArrayLongCachedData s0_ = this.arrayLongCached_cache;
while (s0_ != null) {
if ((s0_.cachedArrayType_.isInstance(JSHasPropertyNode.getArrayType(arg0Value_)))) {
Node node__ = (this);
return arrayLongCached(arg0Value_, arg1Value_, node__, s0_.cachedArrayType_, INLINED_HAS_ELEMENT_PROFILE);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] */) {
if ((JSGuards.isJSFastArray(arg0Value_)) && (JSRuntime.isArrayIndex(arg1Value_))) {
return arrayLong(arg0Value_, arg1Value_, INLINED_HAS_ELEMENT_PROFILE);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] */ && arg0Value instanceof JSTypedArrayObject) {
JSTypedArrayObject arg0Value_ = (JSTypedArrayObject) arg0Value;
return typedArray(arg0Value_, arg1Value_, INLINED_TYPED_ARRAY_TYPED_ARRAY_LENGTH_NODE_);
}
}
if ((state_0 & 0b11111000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectStringCached(JSDynamicObject, TruffleString, JSClass, TruffleString, HasPropertyCacheNode, EqualNode)] || SpecializationActive[JSHasPropertyNode.arrayStringCached(JSDynamicObject, TruffleString, TruffleString, HasPropertyCacheNode, EqualNode)] || SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] || SpecializationActive[JSHasPropertyNode.objectSymbol(JSDynamicObject, Symbol)] || SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((state_0 & 0b111000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectStringCached(JSDynamicObject, TruffleString, JSClass, TruffleString, HasPropertyCacheNode, EqualNode)] || SpecializationActive[JSHasPropertyNode.arrayStringCached(JSDynamicObject, TruffleString, TruffleString, HasPropertyCacheNode, EqualNode)] || SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectStringCached(JSDynamicObject, TruffleString, JSClass, TruffleString, HasPropertyCacheNode, EqualNode)] */) {
ObjectStringCachedData s3_ = this.objectStringCached_cache;
if (s3_ != null) {
{
EqualNode equalNode_ = this.equalNode;
if (equalNode_ != null) {
assert DSLSupport.assertIdempotence((s3_.cachedObjectType_ != null));
if ((s3_.cachedObjectType_.isInstance(arg0Value_)) && (Strings.equals(equalNode_, s3_.cachedName_, arg1Value_))) {
return objectStringCached(arg0Value_, arg1Value_, s3_.cachedObjectType_, s3_.cachedName_, s3_.hasPropertyNode_, equalNode_);
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayStringCached(JSDynamicObject, TruffleString, TruffleString, HasPropertyCacheNode, EqualNode)] */) {
ArrayStringCachedData s4_ = this.arrayStringCached_cache;
if (s4_ != null) {
{
EqualNode equalNode_1 = this.equalNode;
if (equalNode_1 != null) {
if ((JSGuards.isJSArray(arg0Value_))) {
assert DSLSupport.assertIdempotence((!(JSRuntime.isArrayIndex(s4_.cachedName_))));
if ((Strings.equals(equalNode_1, s4_.cachedName_, arg1Value_))) {
return arrayStringCached(arg0Value_, arg1Value_, s4_.cachedName_, s4_.hasPropertyNode_, equalNode_1);
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] */) {
return objectString(arg0Value_, arg1Value_);
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectSymbol(JSDynamicObject, Symbol)] */ && arg1Value instanceof Symbol) {
Symbol arg1Value_ = (Symbol) arg1Value;
return objectSymbol(arg0Value_, arg1Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] */ && IntToLongTypeSystemGen.isImplicitLong((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=long, index=1] */, arg1Value)) {
long arg1Value_ = IntToLongTypeSystemGen.asImplicitLong((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=long, index=1] */, arg1Value);
if ((!(JSGuards.isJSFastArray(arg0Value_))) && (!(JSGuards.isJSArrayBufferView(arg0Value_)))) {
return objectLong(arg0Value_, arg1Value_);
}
}
}
if ((state_0 & 0b11100000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */) {
if ((state_0 & 0b1100000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
ForeignObject0Data s8_ = this.foreignObject0_cache;
while (s8_ != null) {
if ((s8_.interop_.accepts(arg0Value)) && (JSRuntime.isForeignObject(arg0Value))) {
return foreignObject(arg0Value, arg1Value, s8_.interop_, s8_.toStringNode_, s8_.foreignObjectPrototypeNode_, s8_.hasInPrototype_);
}
s8_ = s8_.next_;
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
ForeignObject1Data s9_ = this.foreignObject1_cache;
if (s9_ != null) {
if ((JSRuntime.isForeignObject(arg0Value))) {
return this.foreignObject1Boundary(state_0, s9_, arg0Value, arg1Value);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
{
JSToPropertyKeyNode toPropertyKeyNode__ = this.objectObject_toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
return objectObject(arg0Value_, arg1Value, toPropertyKeyNode__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private boolean foreignObject1Boundary(int state_0, ForeignObject1Data s9_, Object arg0Value, Object arg1Value) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arg0Value));
return foreignObject(arg0Value, arg1Value, interop__, s9_.toStringNode_, s9_.foreignObjectPrototypeNode_, s9_.hasInPrototype_);
}
} finally {
encapsulating_.set(prev_);
}
}
@ExplodeLoop
@Override
public boolean executeBoolean(Object arg0Value, long arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11110000111) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] || SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */) {
if ((state_0 & 0b10000111) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] || SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] */ && IntToLongTypeSystemGen.isImplicitLong((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=long, index=1] */, arg1Value)) {
long arg1Value_ = IntToLongTypeSystemGen.asImplicitLong((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=long, index=1] */, arg1Value);
if ((state_0 & 0b11) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] || SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] */ && (JSGuards.isJSFastArray(arg0Value_)) && (JSRuntime.isArrayIndex(arg1Value_))) {
ArrayLongCachedData s0_ = this.arrayLongCached_cache;
while (s0_ != null) {
if ((s0_.cachedArrayType_.isInstance(JSHasPropertyNode.getArrayType(arg0Value_)))) {
Node node__ = (this);
return arrayLongCached(arg0Value_, arg1Value_, node__, s0_.cachedArrayType_, INLINED_HAS_ELEMENT_PROFILE);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] */) {
if ((JSGuards.isJSFastArray(arg0Value_)) && (JSRuntime.isArrayIndex(arg1Value_))) {
return arrayLong(arg0Value_, arg1Value_, INLINED_HAS_ELEMENT_PROFILE);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] */ && arg0Value instanceof JSTypedArrayObject) {
JSTypedArrayObject arg0Value_ = (JSTypedArrayObject) arg0Value;
return typedArray(arg0Value_, arg1Value_, INLINED_TYPED_ARRAY_TYPED_ARRAY_LENGTH_NODE_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((!(JSGuards.isJSFastArray(arg0Value_))) && (!(JSGuards.isJSArrayBufferView(arg0Value_)))) {
return objectLong(arg0Value_, arg1Value_);
}
}
}
if ((state_0 & 0b11100000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */) {
if ((state_0 & 0b1100000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] || SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
ForeignObject0Data s8_ = this.foreignObject0_cache;
while (s8_ != null) {
if ((s8_.interop_.accepts(arg0Value)) && (JSRuntime.isForeignObject(arg0Value))) {
return foreignObject(arg0Value, arg1Value, s8_.interop_, s8_.toStringNode_, s8_.foreignObjectPrototypeNode_, s8_.hasInPrototype_);
}
s8_ = s8_.next_;
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
ForeignObject1Data s9_ = this.foreignObject1_cache;
if (s9_ != null) {
if ((JSRuntime.isForeignObject(arg0Value))) {
return this.foreignObject1Boundary0(state_0, s9_, arg0Value, arg1Value);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
{
JSToPropertyKeyNode toPropertyKeyNode__ = this.objectObject_toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
return objectObject(arg0Value_, arg1Value, toPropertyKeyNode__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private boolean foreignObject1Boundary0(int state_0, ForeignObject1Data s9_, Object arg0Value, long arg1Value) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arg0Value));
return foreignObject(arg0Value, arg1Value, interop__, s9_.toStringNode_, s9_.foreignObjectPrototypeNode_, s9_.hasInPrototype_);
}
} finally {
encapsulating_.set(prev_);
}
}
private boolean executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b11111111111);
try {
{
int longCast1;
if ((longCast1 = IntToLongTypeSystemGen.specializeImplicitLong(arg1Value)) != 0) {
long arg1Value_ = IntToLongTypeSystemGen.asImplicitLong(longCast1, arg1Value);
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
{
Node node__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] */ && (JSGuards.isJSFastArray(arg0Value_)) && (JSRuntime.isArrayIndex(arg1Value_))) {
while (true) {
int count0_ = 0;
ArrayLongCachedData s0_ = ARRAY_LONG_CACHED_CACHE_UPDATER.getVolatile(this);
ArrayLongCachedData s0_original = s0_;
while (s0_ != null) {
if ((s0_.cachedArrayType_.isInstance(JSHasPropertyNode.getArrayType(arg0Value_)))) {
node__ = (this);
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
ScriptArray cachedArrayType__ = (JSHasPropertyNode.getArrayType(arg0Value_));
if ((cachedArrayType__.isInstance(JSHasPropertyNode.getArrayType(arg0Value_))) && count0_ < (JSHasPropertyNode.MAX_ARRAY_TYPES)) {
s0_ = this.insert(new ArrayLongCachedData(s0_original));
node__ = (this);
s0_.cachedArrayType_ = cachedArrayType__;
if (!ARRAY_LONG_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = (state_0 | (longCast1 << 11) /* set-int ImplicitCast[type=long, index=1] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] */;
this.state_0_ = state_0;
}
}
} else {
state_0 = (state_0 | (longCast1 << 11) /* set-int ImplicitCast[type=long, index=1] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] */;
this.state_0_ = state_0;
}
if (s0_ != null) {
return arrayLongCached(arg0Value_, arg1Value_, node__, s0_.cachedArrayType_, INLINED_HAS_ELEMENT_PROFILE);
}
break;
}
}
}
if ((JSGuards.isJSFastArray(arg0Value_)) && (JSRuntime.isArrayIndex(arg1Value_))) {
this.arrayLongCached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[JSHasPropertyNode.arrayLongCached(JSDynamicObject, long, Node, ScriptArray, InlinedConditionProfile)] */;
state_0 = (state_0 | (longCast1 << 11) /* set-int ImplicitCast[type=long, index=1] */);
state_0 = state_0 | 0b10 /* add SpecializationActive[JSHasPropertyNode.arrayLong(JSDynamicObject, long, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return arrayLong(arg0Value_, arg1Value_, INLINED_HAS_ELEMENT_PROFILE);
}
}
if (arg0Value instanceof JSTypedArrayObject) {
JSTypedArrayObject arg0Value_ = (JSTypedArrayObject) arg0Value;
state_0 = (state_0 | (longCast1 << 11) /* set-int ImplicitCast[type=long, index=1] */);
state_0 = state_0 | 0b100 /* add SpecializationActive[JSHasPropertyNode.typedArray(JSTypedArrayObject, long, TypedArrayLengthNode)] */;
this.state_0_ = state_0;
return typedArray(arg0Value_, arg1Value_, INLINED_TYPED_ARRAY_TYPED_ARRAY_LENGTH_NODE_);
}
}
}
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] */) {
while (true) {
int count3_ = 0;
ObjectStringCachedData s3_ = OBJECT_STRING_CACHED_CACHE_UPDATER.getVolatile(this);
ObjectStringCachedData s3_original = s3_;
while (s3_ != null) {
{
EqualNode equalNode_ = this.equalNode;
if (equalNode_ != null) {
assert DSLSupport.assertIdempotence((s3_.cachedObjectType_ != null));
if ((s3_.cachedObjectType_.isInstance(arg0Value_)) && (Strings.equals(equalNode_, s3_.cachedName_, arg1Value_))) {
break;
}
}
}
count3_++;
s3_ = null;
break;
}
if (s3_ == null && count3_ < 1) {
{
JSClass cachedObjectType__ = (JSHasPropertyNode.getCacheableObjectType(arg0Value_));
if ((cachedObjectType__ != null) && (cachedObjectType__.isInstance(arg0Value_))) {
TruffleString cachedName__ = (arg1Value_);
EqualNode equalNode_;
EqualNode equalNode__shared = this.equalNode;
if (equalNode__shared != null) {
equalNode_ = equalNode__shared;
} else {
equalNode_ = this.insert((EqualNode.create()));
if (equalNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((Strings.equals(equalNode_, cachedName__, arg1Value_))) {
s3_ = this.insert(new ObjectStringCachedData());
s3_.cachedObjectType_ = cachedObjectType__;
s3_.cachedName_ = cachedName__;
s3_.hasPropertyNode_ = s3_.insert((getCachedPropertyGetter(arg0Value_, arg1Value_)));
if (this.equalNode == null) {
this.equalNode = equalNode_;
}
if (!OBJECT_STRING_CACHED_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSHasPropertyNode.objectStringCached(JSDynamicObject, TruffleString, JSClass, TruffleString, HasPropertyCacheNode, EqualNode)] */;
this.state_0_ = state_0;
}
}
}
}
if (s3_ != null) {
return objectStringCached(arg0Value_, arg1Value_, s3_.cachedObjectType_, s3_.cachedName_, s3_.hasPropertyNode_, this.equalNode);
}
break;
}
}
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] */) {
while (true) {
int count4_ = 0;
ArrayStringCachedData s4_ = ARRAY_STRING_CACHED_CACHE_UPDATER.getVolatile(this);
ArrayStringCachedData s4_original = s4_;
while (s4_ != null) {
{
EqualNode equalNode_1 = this.equalNode;
if (equalNode_1 != null) {
if ((JSGuards.isJSArray(arg0Value_))) {
assert DSLSupport.assertIdempotence((!(JSRuntime.isArrayIndex(s4_.cachedName_))));
if ((Strings.equals(equalNode_1, s4_.cachedName_, arg1Value_))) {
break;
}
}
}
}
count4_++;
s4_ = null;
break;
}
if (s4_ == null && count4_ < 1) {
if ((JSGuards.isJSArray(arg0Value_))) {
TruffleString cachedName__1 = (arg1Value_);
if ((!(JSRuntime.isArrayIndex(cachedName__1)))) {
EqualNode equalNode_1;
EqualNode equalNode_1_shared = this.equalNode;
if (equalNode_1_shared != null) {
equalNode_1 = equalNode_1_shared;
} else {
equalNode_1 = this.insert((EqualNode.create()));
if (equalNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((Strings.equals(equalNode_1, cachedName__1, arg1Value_))) {
s4_ = this.insert(new ArrayStringCachedData());
s4_.cachedName_ = cachedName__1;
s4_.hasPropertyNode_ = s4_.insert((getCachedPropertyGetter(arg0Value_, arg1Value_)));
if (this.equalNode == null) {
this.equalNode = equalNode_1;
}
if (!ARRAY_STRING_CACHED_CACHE_UPDATER.compareAndSet(this, s4_original, s4_)) {
continue;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSHasPropertyNode.arrayStringCached(JSDynamicObject, TruffleString, TruffleString, HasPropertyCacheNode, EqualNode)] */;
this.state_0_ = state_0;
}
}
}
}
if (s4_ != null) {
return arrayStringCached(arg0Value_, arg1Value_, s4_.cachedName_, s4_.hasPropertyNode_, this.equalNode);
}
break;
}
}
this.objectStringCached_cache = null;
this.arrayStringCached_cache = null;
state_0 = state_0 & 0xffffffd7 /* remove SpecializationActive[JSHasPropertyNode.objectStringCached(JSDynamicObject, TruffleString, JSClass, TruffleString, HasPropertyCacheNode, EqualNode)], SpecializationActive[JSHasPropertyNode.arrayStringCached(JSDynamicObject, TruffleString, TruffleString, HasPropertyCacheNode, EqualNode)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSHasPropertyNode.objectString(JSDynamicObject, TruffleString)] */;
this.state_0_ = state_0;
return objectString(arg0Value_, arg1Value_);
}
if (arg1Value instanceof Symbol) {
Symbol arg1Value_ = (Symbol) arg1Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSHasPropertyNode.objectSymbol(JSDynamicObject, Symbol)] */;
this.state_0_ = state_0;
return objectSymbol(arg0Value_, arg1Value_);
}
{
int longCast1;
if ((longCast1 = IntToLongTypeSystemGen.specializeImplicitLong(arg1Value)) != 0) {
long arg1Value_ = IntToLongTypeSystemGen.asImplicitLong(longCast1, arg1Value);
if ((!(JSGuards.isJSFastArray(arg0Value_))) && (!(JSGuards.isJSArrayBufferView(arg0Value_)))) {
state_0 = (state_0 | (longCast1 << 11) /* set-int ImplicitCast[type=long, index=1] */);
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSHasPropertyNode.objectLong(JSDynamicObject, long)] */;
this.state_0_ = state_0;
return objectLong(arg0Value_, arg1Value_);
}
}
}
}
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */) {
while (true) {
int count8_ = 0;
ForeignObject0Data s8_ = FOREIGN_OBJECT0_CACHE_UPDATER.getVolatile(this);
ForeignObject0Data s8_original = s8_;
while (s8_ != null) {
if ((s8_.interop_.accepts(arg0Value)) && (JSRuntime.isForeignObject(arg0Value))) {
break;
}
count8_++;
s8_ = s8_.next_;
}
if (s8_ == null) {
if ((JSRuntime.isForeignObject(arg0Value)) && count8_ < (JSConfig.InteropLibraryLimit)) {
// assert (s8_.interop_.accepts(arg0Value));
s8_ = this.insert(new ForeignObject0Data(s8_original));
InteropLibrary interop__ = s8_.insert((INTEROP_LIBRARY_.create(arg0Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s8_.interop_ = interop__;
JSToStringNode toStringNode__ = s8_.insert((JSToStringNode.create()));
Objects.requireNonNull(toStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s8_.toStringNode_ = toStringNode__;
ForeignObjectPrototypeNode foreignObjectPrototypeNode__ = s8_.insert((ForeignObjectPrototypeNode.create()));
Objects.requireNonNull(foreignObjectPrototypeNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s8_.foreignObjectPrototypeNode_ = foreignObjectPrototypeNode__;
JSHasPropertyNode hasInPrototype__ = s8_.insert((JSHasPropertyNode.create()));
Objects.requireNonNull(hasInPrototype__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s8_.hasInPrototype_ = hasInPrototype__;
if (!FOREIGN_OBJECT0_CACHE_UPDATER.compareAndSet(this, s8_original, s8_)) {
continue;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */;
this.state_0_ = state_0;
}
}
if (s8_ != null) {
return foreignObject(arg0Value, arg1Value, s8_.interop_, s8_.toStringNode_, s8_.foreignObjectPrototypeNode_, s8_.hasInPrototype_);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((JSRuntime.isForeignObject(arg0Value))) {
ForeignObject1Data s9_ = this.insert(new ForeignObject1Data());
interop__ = (INTEROP_LIBRARY_.getUncached(arg0Value));
JSToStringNode toStringNode__ = s9_.insert((JSToStringNode.create()));
Objects.requireNonNull(toStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s9_.toStringNode_ = toStringNode__;
ForeignObjectPrototypeNode foreignObjectPrototypeNode__ = s9_.insert((ForeignObjectPrototypeNode.create()));
Objects.requireNonNull(foreignObjectPrototypeNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s9_.foreignObjectPrototypeNode_ = foreignObjectPrototypeNode__;
JSHasPropertyNode hasInPrototype__ = s9_.insert((JSHasPropertyNode.create()));
Objects.requireNonNull(hasInPrototype__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s9_.hasInPrototype_ = hasInPrototype__;
VarHandle.storeStoreFence();
this.foreignObject1_cache = s9_;
this.foreignObject0_cache = null;
state_0 = state_0 & 0xfffffeff /* remove SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */;
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSHasPropertyNode.foreignObject(Object, Object, InteropLibrary, JSToStringNode, ForeignObjectPrototypeNode, JSHasPropertyNode)] */;
this.state_0_ = state_0;
return foreignObject(arg0Value, arg1Value, interop__, toStringNode__, foreignObjectPrototypeNode__, hasInPrototype__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
JSToPropertyKeyNode toPropertyKeyNode__ = this.insert((JSToPropertyKeyNode.create()));
Objects.requireNonNull(toPropertyKeyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.objectObject_toPropertyKeyNode_ = toPropertyKeyNode__;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[JSHasPropertyNode.objectObject(JSDynamicObject, Object, JSToPropertyKeyNode)] */;
this.state_0_ = state_0;
return objectObject(arg0Value_, arg1Value, toPropertyKeyNode__);
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
if (((oldState_0 & 0b10001010000) == 0 && (state_0_ & 0b10001010000) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@NeverDefault
public static JSHasPropertyNode create(boolean hasOwnProperty) {
return new JSHasPropertyNodeGen(hasOwnProperty);
}
@GeneratedBy(JSHasPropertyNode.class)
@DenyReplace
private static final class ArrayLongCachedData extends Node implements SpecializationDataNode {
@Child ArrayLongCachedData next_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#arrayLongCached}
* Parameter: {@link ScriptArray} cachedArrayType
*/
@CompilationFinal ScriptArray cachedArrayType_;
ArrayLongCachedData(ArrayLongCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(JSHasPropertyNode.class)
@DenyReplace
private static final class ObjectStringCachedData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#objectStringCached}
* Parameter: {@link JSClass} cachedObjectType
*/
@CompilationFinal JSClass cachedObjectType_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#objectStringCached}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#objectStringCached}
* Parameter: {@link HasPropertyCacheNode} hasPropertyNode
*/
@Child HasPropertyCacheNode hasPropertyNode_;
ObjectStringCachedData() {
}
}
@GeneratedBy(JSHasPropertyNode.class)
@DenyReplace
private static final class ArrayStringCachedData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#arrayStringCached}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#arrayStringCached}
* Parameter: {@link HasPropertyCacheNode} hasPropertyNode
*/
@Child HasPropertyCacheNode hasPropertyNode_;
ArrayStringCachedData() {
}
}
@GeneratedBy(JSHasPropertyNode.class)
@DenyReplace
private static final class ForeignObject0Data extends Node implements SpecializationDataNode {
@Child ForeignObject0Data next_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link JSToStringNode} toStringNode
*/
@Child JSToStringNode toStringNode_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link ForeignObjectPrototypeNode} foreignObjectPrototypeNode
*/
@Child ForeignObjectPrototypeNode foreignObjectPrototypeNode_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link JSHasPropertyNode} hasInPrototype
*/
@Child JSHasPropertyNode hasInPrototype_;
ForeignObject0Data(ForeignObject0Data next_) {
this.next_ = next_;
}
}
@GeneratedBy(JSHasPropertyNode.class)
@DenyReplace
private static final class ForeignObject1Data extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link JSToStringNode} toStringNode
*/
@Child JSToStringNode toStringNode_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link ForeignObjectPrototypeNode} foreignObjectPrototypeNode
*/
@Child ForeignObjectPrototypeNode foreignObjectPrototypeNode_;
/**
* Source Info:
* Specialization: {@link JSHasPropertyNode#foreignObject}
* Parameter: {@link JSHasPropertyNode} hasInPrototype
*/
@Child JSHasPropertyNode hasInPrototype_;
ForeignObject1Data() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy