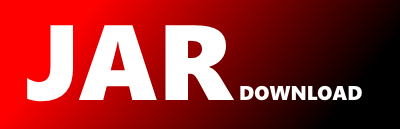
com.oracle.graal.python.lib.CanBeDoubleNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.attributes.LookupCallableSlotInMRONode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.nodes.util.LazyInteropLibrary;
import com.oracle.graal.python.nodes.util.LazyInteropLibraryNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link CanBeDoubleNode#doDouble}
* Activation probability: 0.23929
* With/without class size: 6/0 bytes
* Specialization {@link CanBeDoubleNode#doInt}
* Activation probability: 0.20714
* With/without class size: 6/0 bytes
* Specialization {@link CanBeDoubleNode#doLong}
* Activation probability: 0.17500
* With/without class size: 6/0 bytes
* Specialization {@link CanBeDoubleNode#doBoolean}
* Activation probability: 0.14286
* With/without class size: 5/0 bytes
* Specialization {@link CanBeDoubleNode#doString}
* Activation probability: 0.11071
* With/without class size: 5/0 bytes
* Specialization {@link CanBeDoubleNode#doPBCT}
* Activation probability: 0.07857
* With/without class size: 4/0 bytes
* Specialization {@link CanBeDoubleNode#doGeneric}
* Activation probability: 0.04643
* With/without class size: 5/19 bytes
*
*/
@GeneratedBy(CanBeDoubleNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class CanBeDoubleNodeGen {
private static final StateField FALLBACK__CAN_BE_DOUBLE_NODE_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static CanBeDoubleNode getUncached() {
return CanBeDoubleNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#fallback_cache}
*
*/
@NeverDefault
public static CanBeDoubleNode inline(@RequiredField(bits = 7, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new CanBeDoubleNodeGen.Inlined(target);
}
@GeneratedBy(CanBeDoubleNode.class)
@DenyReplace
private static final class Inlined extends CanBeDoubleNode {
/**
* State Info:
* 0: SpecializationActive {@link CanBeDoubleNode#doDouble}
* 1: SpecializationActive {@link CanBeDoubleNode#doInt}
* 2: SpecializationActive {@link CanBeDoubleNode#doLong}
* 3: SpecializationActive {@link CanBeDoubleNode#doBoolean}
* 4: SpecializationActive {@link CanBeDoubleNode#doString}
* 5: SpecializationActive {@link CanBeDoubleNode#doPBCT}
* 6: SpecializationActive {@link CanBeDoubleNode#doGeneric}
*
*/
private final StateField state_0_;
private final ReferenceField fallback_cache;
/**
* Source Info:
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link LazyInteropLibrary} lazyInteropLibrary
* Inline method: {@link LazyInteropLibraryNodeGen#inline}
*/
private final LazyInteropLibrary fallback_lazyInteropLibrary_;
/**
* Source Info:
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode fallback_getClassNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(CanBeDoubleNode.class);
this.state_0_ = target.getState(0, 7);
this.fallback_cache = target.getReference(1, FallbackData.class);
this.fallback_lazyInteropLibrary_ = LazyInteropLibraryNodeGen.inline(InlineTarget.create(LazyInteropLibrary.class, FALLBACK__CAN_BE_DOUBLE_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(0, 1), ReferenceField.create(FallbackData.lookup_(), "fallback_lazyInteropLibrary__field1_", Node.class)));
this.fallback_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, FALLBACK__CAN_BE_DOUBLE_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(1, 17), ReferenceField.create(FallbackData.lookup_(), "fallback_getClassNode__field1_", Node.class)));
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[CanBeDoubleNode.doDouble(Double)] */) && arg1Value instanceof Double) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[CanBeDoubleNode.doInt(Integer)] */) && arg1Value instanceof Integer) {
return false;
}
if (!((state_0 & 0b100) != 0 /* is SpecializationActive[CanBeDoubleNode.doLong(Long)] */) && arg1Value instanceof Long) {
return false;
}
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[CanBeDoubleNode.doBoolean(Boolean)] */) && arg1Value instanceof Boolean) {
return false;
}
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[CanBeDoubleNode.doString(TruffleString)] */) && arg1Value instanceof TruffleString) {
return false;
}
if (!((state_0 & 0b100000) != 0 /* is SpecializationActive[CanBeDoubleNode.doPBCT(PythonBuiltinClassType)] */) && arg1Value instanceof PythonBuiltinClassType) {
return false;
}
return true;
}
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[CanBeDoubleNode.doDouble(Double)] || SpecializationActive[CanBeDoubleNode.doInt(Integer)] || SpecializationActive[CanBeDoubleNode.doLong(Long)] || SpecializationActive[CanBeDoubleNode.doBoolean(Boolean)] || SpecializationActive[CanBeDoubleNode.doString(TruffleString)] || SpecializationActive[CanBeDoubleNode.doPBCT(PythonBuiltinClassType)] || SpecializationActive[CanBeDoubleNode.doGeneric(Node, Object, LazyInteropLibrary, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, GetClassNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[CanBeDoubleNode.doDouble(Double)] */ && arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return CanBeDoubleNode.doDouble(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CanBeDoubleNode.doInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return CanBeDoubleNode.doInt(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[CanBeDoubleNode.doLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return CanBeDoubleNode.doLong(arg1Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[CanBeDoubleNode.doBoolean(Boolean)] */ && arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return CanBeDoubleNode.doBoolean(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[CanBeDoubleNode.doString(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return CanBeDoubleNode.doString(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[CanBeDoubleNode.doPBCT(PythonBuiltinClassType)] */ && arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return CanBeDoubleNode.doPBCT(arg1Value_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[CanBeDoubleNode.doGeneric(Node, Object, LazyInteropLibrary, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, GetClassNode)] */) {
FallbackData s6_ = this.fallback_cache.get(arg0Value);
if (s6_ != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return CanBeDoubleNode.doGeneric(s6_, arg1Value, this.fallback_lazyInteropLibrary_, s6_.lookupFloat_, s6_.lookupIndex_, this.fallback_getClassNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[CanBeDoubleNode.doDouble(Double)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doDouble(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[CanBeDoubleNode.doInt(Integer)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[CanBeDoubleNode.doLong(Long)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[CanBeDoubleNode.doBoolean(Boolean)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[CanBeDoubleNode.doString(TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doString(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[CanBeDoubleNode.doPBCT(PythonBuiltinClassType)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doPBCT(arg1Value_);
}
FallbackData s6_ = arg0Value.insert(new FallbackData());
LookupCallableSlotInMRONode lookupFloat__ = s6_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Float)));
Objects.requireNonNull(lookupFloat__, "Specialization 'doGeneric(Node, Object, LazyInteropLibrary, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, GetClassNode)' cache 'lookupFloat' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.lookupFloat_ = lookupFloat__;
LookupCallableSlotInMRONode lookupIndex__ = s6_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Index)));
Objects.requireNonNull(lookupIndex__, "Specialization 'doGeneric(Node, Object, LazyInteropLibrary, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, GetClassNode)' cache 'lookupIndex' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.lookupIndex_ = lookupIndex__;
VarHandle.storeStoreFence();
this.fallback_cache.set(arg0Value, s6_);
state_0 = state_0 | 0b1000000 /* add SpecializationActive[CanBeDoubleNode.doGeneric(Node, Object, LazyInteropLibrary, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, GetClassNode)] */;
this.state_0_.set(arg0Value, state_0);
return CanBeDoubleNode.doGeneric(s6_, arg1Value, this.fallback_lazyInteropLibrary_, lookupFloat__, lookupIndex__, this.fallback_getClassNode_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(CanBeDoubleNode.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link LazyInteropLibrary} lazyInteropLibrary
* Inline method: {@link LazyInteropLibraryNodeGen#inline}
* 1-17: InlinedCache
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* Source Info:
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link LazyInteropLibrary} lazyInteropLibrary
* Inline method: {@link LazyInteropLibraryNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_lazyInteropLibrary__field1_;
/**
* Source Info:
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link LookupCallableSlotInMRONode} lookupFloat
*/
@Child LookupCallableSlotInMRONode lookupFloat_;
/**
* Source Info:
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link LookupCallableSlotInMRONode} lookupIndex
*/
@Child LookupCallableSlotInMRONode lookupIndex_;
/**
* Source Info:
* Specialization: {@link CanBeDoubleNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getClassNode__field1_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(CanBeDoubleNode.class)
@DenyReplace
private static final class Uncached extends CanBeDoubleNode {
@TruffleBoundary
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return CanBeDoubleNode.doDouble(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return CanBeDoubleNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return CanBeDoubleNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return CanBeDoubleNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return CanBeDoubleNode.doString(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return CanBeDoubleNode.doPBCT(arg1Value_);
}
return CanBeDoubleNode.doGeneric(arg0Value, arg1Value, (LazyInteropLibraryNodeGen.getUncached()), (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.Float)), (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.Index)), (GetClassNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy