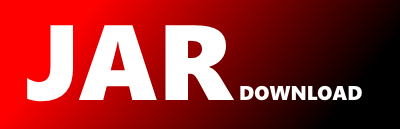
com.oracle.graal.python.lib.PyDictSetDefaultNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodes.HashingStorageGetItemWithHash;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodes.HashingStorageSetItemWithHash;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodesFactory.HashingStorageGetItemWithHashNodeGen;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodesFactory.HashingStorageSetItemWithHashNodeGen;
import com.oracle.graal.python.builtins.objects.dict.PDict;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
/**
* Debug Info:
* Specialization {@link PyDictSetDefault#doIt}
* Activation probability: 1.00000
* With/without class size: 76/55 bytes
*
*/
@GeneratedBy(PyDictSetDefault.class)
@SuppressWarnings("javadoc")
public final class PyDictSetDefaultNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyDictSetDefault getUncached() {
return PyDictSetDefaultNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#hashNode__field1_}
*
- {@link Inlined#hashNode__field2_}
*
- {@link Inlined#hashNode__field3_}
*
- {@link Inlined#getItem__field1_}
*
- {@link Inlined#getItem__field2_}
*
- {@link Inlined#getItem__field3_}
*
- {@link Inlined#getItem__field4_}
*
- {@link Inlined#getItem__field5_}
*
- {@link Inlined#setItem__field1_}
*
- {@link Inlined#setItem__field2_}
*
- {@link Inlined#setItem__field3_}
*
- {@link Inlined#setItem__field4_}
*
- {@link Inlined#setItem__field5_}
*
*/
@NeverDefault
public static PyDictSetDefault inline(@RequiredField(bits = 25, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyDictSetDefaultNodeGen.Inlined(target);
}
@GeneratedBy(PyDictSetDefault.class)
@DenyReplace
private static final class Inlined extends PyDictSetDefault {
/**
* State Info:
* 0: SpecializationActive {@link PyDictSetDefault#doIt}
* 1-7: InlinedCache
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link PyObjectHashNode} hashNode
* Inline method: {@link PyObjectHashNodeGen#inline}
* 8-16: InlinedCache
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link HashingStorageGetItemWithHash} getItem
* Inline method: {@link HashingStorageGetItemWithHashNodeGen#inline}
* 17-22: InlinedCache
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link HashingStorageSetItemWithHash} setItem
* Inline method: {@link HashingStorageSetItemWithHashNodeGen#inline}
* 23-24: InlinedCache
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link InlinedConditionProfile} hasValue
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
private final StateField state_0_;
private final ReferenceField hashNode__field1_;
private final ReferenceField hashNode__field2_;
private final ReferenceField hashNode__field3_;
private final ReferenceField getItem__field1_;
private final ReferenceField getItem__field2_;
private final ReferenceField getItem__field3_;
private final ReferenceField getItem__field4_;
private final ReferenceField getItem__field5_;
private final ReferenceField setItem__field1_;
private final ReferenceField setItem__field2_;
private final ReferenceField setItem__field3_;
private final ReferenceField setItem__field4_;
private final ReferenceField setItem__field5_;
/**
* Source Info:
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link PyObjectHashNode} hashNode
* Inline method: {@link PyObjectHashNodeGen#inline}
*/
private final PyObjectHashNode hashNode_;
/**
* Source Info:
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link HashingStorageGetItemWithHash} getItem
* Inline method: {@link HashingStorageGetItemWithHashNodeGen#inline}
*/
private final HashingStorageGetItemWithHash getItem_;
/**
* Source Info:
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link HashingStorageSetItemWithHash} setItem
* Inline method: {@link HashingStorageSetItemWithHashNodeGen#inline}
*/
private final HashingStorageSetItemWithHash setItem_;
/**
* Source Info:
* Specialization: {@link PyDictSetDefault#doIt}
* Parameter: {@link InlinedConditionProfile} hasValue
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile hasValue_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyDictSetDefault.class);
this.state_0_ = target.getState(0, 25);
this.hashNode__field1_ = target.getReference(1, Node.class);
this.hashNode__field2_ = target.getReference(2, Node.class);
this.hashNode__field3_ = target.getReference(3, Node.class);
this.getItem__field1_ = target.getReference(4, Node.class);
this.getItem__field2_ = target.getReference(5, Node.class);
this.getItem__field3_ = target.getReference(6, Node.class);
this.getItem__field4_ = target.getReference(7, Node.class);
this.getItem__field5_ = target.getReference(8, Node.class);
this.setItem__field1_ = target.getReference(9, Node.class);
this.setItem__field2_ = target.getReference(10, Node.class);
this.setItem__field3_ = target.getReference(11, Node.class);
this.setItem__field4_ = target.getReference(12, Node.class);
this.setItem__field5_ = target.getReference(13, Node.class);
this.hashNode_ = PyObjectHashNodeGen.inline(InlineTarget.create(PyObjectHashNode.class, state_0_.subUpdater(1, 7), hashNode__field1_, hashNode__field2_, hashNode__field3_));
this.getItem_ = HashingStorageGetItemWithHashNodeGen.inline(InlineTarget.create(HashingStorageGetItemWithHash.class, state_0_.subUpdater(8, 9), getItem__field1_, getItem__field2_, getItem__field3_, getItem__field4_, getItem__field5_));
this.setItem_ = HashingStorageSetItemWithHashNodeGen.inline(InlineTarget.create(HashingStorageSetItemWithHash.class, state_0_.subUpdater(17, 6), setItem__field1_, setItem__field2_, setItem__field3_, setItem__field4_, setItem__field5_));
this.hasValue_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, state_0_.subUpdater(23, 2)));
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyDictSetDefault.doIt(VirtualFrame, Node, PDict, Object, Object, PyObjectHashNode, HashingStorageGetItemWithHash, HashingStorageSetItemWithHash, InlinedConditionProfile)] */ && arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
assert InlineSupport.validate(arg0Value, this.state_0_, this.hashNode__field1_, this.hashNode__field2_, this.hashNode__field3_, this.state_0_, this.getItem__field1_, this.getItem__field2_, this.getItem__field3_, this.getItem__field4_, this.getItem__field5_, this.state_0_, this.setItem__field1_, this.setItem__field2_, this.setItem__field3_, this.setItem__field4_, this.setItem__field5_, this.state_0_);
return PyDictSetDefault.doIt((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, this.hashNode_, this.getItem_, this.setItem_, this.hasValue_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyDictSetDefault.doIt(VirtualFrame, Node, PDict, Object, Object, PyObjectHashNode, HashingStorageGetItemWithHash, HashingStorageSetItemWithHash, InlinedConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.hashNode__field1_, this.hashNode__field2_, this.hashNode__field3_, this.state_0_, this.getItem__field1_, this.getItem__field2_, this.getItem__field3_, this.getItem__field4_, this.getItem__field5_, this.state_0_, this.setItem__field1_, this.setItem__field2_, this.setItem__field3_, this.setItem__field4_, this.setItem__field5_, this.state_0_);
return PyDictSetDefault.doIt((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, this.hashNode_, this.getItem_, this.setItem_, this.hasValue_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyDictSetDefault.class)
@DenyReplace
private static final class Uncached extends PyDictSetDefault {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
return PyDictSetDefault.doIt((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, (PyObjectHashNode.getUncached()), (HashingStorageGetItemWithHashNodeGen.getUncached()), (HashingStorageSetItemWithHashNodeGen.getUncached()), (InlinedConditionProfile.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy