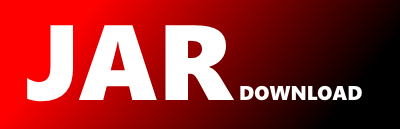
com.oracle.graal.python.lib.PyDictSetItemNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodes.HashingStorageSetItem;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodesFactory.HashingStorageSetItemNodeGen;
import com.oracle.graal.python.builtins.objects.dict.PDict;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.IntField;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedCountingConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link PyDictSetItem#setItemWithStringKey}
* Activation probability: 0.48333
* With/without class size: 11/0 bytes
* Specialization {@link PyDictSetItem#setItemCached}
* Activation probability: 0.33333
* With/without class size: 9/0 bytes
* Specialization {@link PyDictSetItem#setItem}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(PyDictSetItem.class)
@SuppressWarnings("javadoc")
public final class PyDictSetItemNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyDictSetItem getUncached() {
return PyDictSetItemNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#setItem}
*
- {@link Inlined#updateStorage_field0_}
*
- {@link Inlined#updateStorage_field1_}
*
*/
@NeverDefault
public static PyDictSetItem inline(@RequiredField(bits = 3, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(IntField.class)@RequiredField(IntField.class) InlineTarget target) {
return new PyDictSetItemNodeGen.Inlined(target);
}
@GeneratedBy(PyDictSetItem.class)
@DenyReplace
private static final class Inlined extends PyDictSetItem {
/**
* State Info:
* 0: SpecializationActive {@link PyDictSetItem#setItemWithStringKey}
* 1: SpecializationActive {@link PyDictSetItem#setItemCached}
* 2: SpecializationActive {@link PyDictSetItem#setItem}
*
*/
private final StateField state_0_;
private final ReferenceField setItem;
private final IntField updateStorage_field0_;
private final IntField updateStorage_field1_;
/**
* Source Info:
* Specialization: {@link PyDictSetItem#setItemWithStringKey}
* Parameter: {@link InlinedCountingConditionProfile} updateStorageProfile
* Inline method: {@link InlinedCountingConditionProfile#inline}
*/
private final InlinedCountingConditionProfile updateStorage;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyDictSetItem.class);
this.state_0_ = target.getState(0, 3);
this.setItem = target.getReference(1, HashingStorageSetItem.class);
this.updateStorage_field0_ = target.getPrimitive(2, IntField.class);
this.updateStorage_field1_ = target.getPrimitive(3, IntField.class);
this.updateStorage = InlinedCountingConditionProfile.inline(InlineTarget.create(InlinedCountingConditionProfile.class, updateStorage_field0_, updateStorage_field1_));
}
@Override
public void execute(Frame frameValue, Node arg0Value, PDict arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyDictSetItem.setItemWithStringKey(Node, PDict, TruffleString, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] || SpecializationActive[PyDictSetItem.setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] || SpecializationActive[PyDictSetItem.setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyDictSetItem.setItemWithStringKey(Node, PDict, TruffleString, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */ && arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
{
HashingStorageSetItem setItem_ = this.setItem.get(arg0Value);
if (setItem_ != null) {
PyDictSetItem.setItemWithStringKey(arg0Value, arg1Value, arg2Value_, arg3Value, setItem_, this.updateStorage);
return;
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[PyDictSetItem.setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] || SpecializationActive[PyDictSetItem.setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyDictSetItem.setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */) {
{
HashingStorageSetItem setItem_1 = this.setItem.get(arg0Value);
if (setItem_1 != null) {
PyDictSetItem.setItemCached((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, setItem_1, this.updateStorage);
return;
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyDictSetItem.setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */) {
{
HashingStorageSetItem setItem_2 = this.setItem.get(arg0Value);
if (setItem_2 != null) {
PyDictSetItem.setItem(arg0Value, arg1Value, arg2Value, arg3Value, setItem_2, this.updateStorage);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void executeAndSpecialize(Frame frameValue, Node arg0Value, PDict arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b110)) == 0 /* is-not SpecializationActive[PyDictSetItem.setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] && SpecializationActive[PyDictSetItem.setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */ && arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
HashingStorageSetItem setItem_;
HashingStorageSetItem setItem__shared = this.setItem.get(arg0Value);
if (setItem__shared != null) {
setItem_ = setItem__shared;
} else {
setItem_ = arg0Value.insert((HashingStorageSetItem.create()));
if (setItem_ == null) {
throw new IllegalStateException("Specialization 'setItemWithStringKey(Node, PDict, TruffleString, Object, HashingStorageSetItem, InlinedCountingConditionProfile)' contains a shared cache with name 'setItem' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.setItem.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.setItem.set(arg0Value, setItem_);
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyDictSetItem.setItemWithStringKey(Node, PDict, TruffleString, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
PyDictSetItem.setItemWithStringKey(arg0Value, arg1Value, arg2Value_, arg3Value, setItem_, this.updateStorage);
return;
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[PyDictSetItem.setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */) {
HashingStorageSetItem setItem_1;
HashingStorageSetItem setItem_1_shared = this.setItem.get(arg0Value);
if (setItem_1_shared != null) {
setItem_1 = setItem_1_shared;
} else {
setItem_1 = arg0Value.insert((HashingStorageSetItem.create()));
if (setItem_1 == null) {
throw new IllegalStateException("Specialization 'setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)' contains a shared cache with name 'setItem' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.setItem.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.setItem.set(arg0Value, setItem_1);
}
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PyDictSetItem.setItemWithStringKey(Node, PDict, TruffleString, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyDictSetItem.setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
PyDictSetItem.setItemCached((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, setItem_1, this.updateStorage);
return;
}
HashingStorageSetItem setItem_2;
HashingStorageSetItem setItem_2_shared = this.setItem.get(arg0Value);
if (setItem_2_shared != null) {
setItem_2 = setItem_2_shared;
} else {
setItem_2 = arg0Value.insert((HashingStorageSetItem.create()));
if (setItem_2 == null) {
throw new IllegalStateException("Specialization 'setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)' contains a shared cache with name 'setItem' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.setItem.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.setItem.set(arg0Value, setItem_2);
}
state_0 = state_0 & 0xfffffffc /* remove SpecializationActive[PyDictSetItem.setItemWithStringKey(Node, PDict, TruffleString, Object, HashingStorageSetItem, InlinedCountingConditionProfile)], SpecializationActive[PyDictSetItem.setItemCached(VirtualFrame, Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyDictSetItem.setItem(Node, PDict, Object, Object, HashingStorageSetItem, InlinedCountingConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
PyDictSetItem.setItem(arg0Value, arg1Value, arg2Value, arg3Value, setItem_2, this.updateStorage);
return;
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyDictSetItem.class)
@DenyReplace
private static final class Uncached extends PyDictSetItem {
@Override
public void execute(Frame frameValue, Node arg0Value, PDict arg1Value, Object arg2Value, Object arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
PyDictSetItem.setItem(arg0Value, arg1Value, arg2Value, arg3Value, (HashingStorageSetItemNodeGen.getUncached()), (InlinedCountingConditionProfile.getUncached()));
return;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy