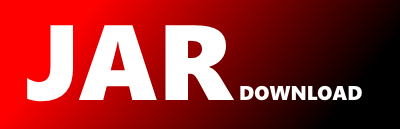
com.oracle.graal.python.lib.PyFloatAsDoubleNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.modules.WarningsModuleBuiltins.WarnNode;
import com.oracle.graal.python.builtins.objects.cext.PythonAbstractNativeObject;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccess.ReadDoubleNode;
import com.oracle.graal.python.builtins.objects.floats.PFloat;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.nodes.object.BuiltinClassProfiles.InlineIsBuiltinClassProfile;
import com.oracle.graal.python.nodes.object.BuiltinClassProfilesFactory.InlineIsBuiltinClassProfileNodeGen;
import com.oracle.graal.python.nodes.util.CastToJavaDoubleNode;
import com.oracle.graal.python.nodes.util.CastToJavaDoubleNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyFloatAsDoubleNode#doDouble}
* Activation probability: 0.23929
* With/without class size: 6/0 bytes
* Specialization {@link PyFloatAsDoubleNode#doPFloat}
* Activation probability: 0.20714
* With/without class size: 6/0 bytes
* Specialization {@link PyFloatAsDoubleNode#doInt}
* Activation probability: 0.17500
* With/without class size: 6/0 bytes
* Specialization {@link PyFloatAsDoubleNode#doLong}
* Activation probability: 0.14286
* With/without class size: 5/0 bytes
* Specialization {@link PyFloatAsDoubleNode#doBoolean}
* Activation probability: 0.11071
* With/without class size: 5/0 bytes
* Specialization {@link PyFloatAsDoubleNode#doNative}
* Activation probability: 0.07857
* With/without class size: 6/15 bytes
* Specialization {@link PyFloatAsDoubleNode#doObject}
* Activation probability: 0.04643
* With/without class size: 8/77 bytes
*
*/
@GeneratedBy(PyFloatAsDoubleNode.class)
@SuppressWarnings("javadoc")
public final class PyFloatAsDoubleNodeGen {
private static final StateField NATIVE__PY_FLOAT_AS_DOUBLE_NODE_NATIVE_STATE_0_UPDATER = StateField.create(NativeData.lookup_(), "native_state_0_");
private static final StateField OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_0_UPDATER = StateField.create(ObjectData.lookup_(), "object_state_0_");
private static final StateField OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_1_UPDATER = StateField.create(ObjectData.lookup_(), "object_state_1_");
private static final StateField OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_2_UPDATER = StateField.create(ObjectData.lookup_(), "object_state_2_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyFloatAsDoubleNode getUncached() {
return PyFloatAsDoubleNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#native_cache}
*
- {@link Inlined#object_cache}
*
*/
@NeverDefault
public static PyFloatAsDoubleNode inline(@RequiredField(bits = 7, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyFloatAsDoubleNodeGen.Inlined(target);
}
@GeneratedBy(PyFloatAsDoubleNode.class)
@DenyReplace
private static final class Inlined extends PyFloatAsDoubleNode {
/**
* State Info:
* 0: SpecializationActive {@link PyFloatAsDoubleNode#doDouble}
* 1: SpecializationActive {@link PyFloatAsDoubleNode#doPFloat}
* 2: SpecializationActive {@link PyFloatAsDoubleNode#doInt}
* 3: SpecializationActive {@link PyFloatAsDoubleNode#doLong}
* 4: SpecializationActive {@link PyFloatAsDoubleNode#doBoolean}
* 5: SpecializationActive {@link PyFloatAsDoubleNode#doNative}
* 6: SpecializationActive {@link PyFloatAsDoubleNode#doObject}
*
*/
private final StateField state_0_;
private final ReferenceField native_cache;
private final ReferenceField object_cache;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doNative}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode native_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode object_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link GetClassNode} resultClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode object_resultClassNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link InlineIsBuiltinClassProfile} resultProfile
* Inline method: {@link InlineIsBuiltinClassProfileNodeGen#inline}
*/
private final InlineIsBuiltinClassProfile object_resultProfile_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyIndexCheckNode} indexCheckNode
* Inline method: {@link PyIndexCheckNodeGen#inline}
*/
private final PyIndexCheckNode object_indexCheckNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
*/
private final PyNumberIndexNode object_indexNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link CastToJavaDoubleNode} cast
* Inline method: {@link CastToJavaDoubleNodeGen#inline}
*/
private final CastToJavaDoubleNode object_cast_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy object_raiseNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyFloatAsDoubleNode.class);
this.state_0_ = target.getState(0, 7);
this.native_cache = target.getReference(1, NativeData.class);
this.object_cache = target.getReference(2, ObjectData.class);
this.native_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, NATIVE__PY_FLOAT_AS_DOUBLE_NODE_NATIVE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(NativeData.lookup_(), "native_getClassNode__field1_", Node.class)));
this.object_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(ObjectData.lookup_(), "object_getClassNode__field1_", Node.class)));
this.object_resultClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(ObjectData.lookup_(), "object_resultClassNode__field1_", Node.class)));
this.object_resultProfile_ = InlineIsBuiltinClassProfileNodeGen.inline(InlineTarget.create(InlineIsBuiltinClassProfile.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_0_UPDATER.subUpdater(23, 3)));
this.object_indexCheckNode_ = PyIndexCheckNodeGen.inline(InlineTarget.create(PyIndexCheckNode.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_1_UPDATER.subUpdater(17, 7), ReferenceField.create(ObjectData.lookup_(), "object_indexCheckNode__field1_", Node.class)));
this.object_indexNode_ = PyNumberIndexNodeGen.inline(InlineTarget.create(PyNumberIndexNode.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_0_UPDATER.subUpdater(17, 6), ReferenceField.create(ObjectData.lookup_(), "object_indexNode__field1_", Node.class), ReferenceField.create(ObjectData.lookup_(), "object_indexNode__field2_", Node.class), ReferenceField.create(ObjectData.lookup_(), "object_indexNode__field3_", Node.class), ReferenceField.create(ObjectData.lookup_(), "object_indexNode__field4_", Node.class), ReferenceField.create(ObjectData.lookup_(), "object_indexNode__field5_", Node.class)));
this.object_cast_ = CastToJavaDoubleNodeGen.inline(InlineTarget.create(CastToJavaDoubleNode.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_2_UPDATER.subUpdater(0, 17), ReferenceField.create(ObjectData.lookup_(), "object_cast__field1_", Node.class), ReferenceField.create(ObjectData.lookup_(), "object_cast__field2_", Node.class), ReferenceField.create(ObjectData.lookup_(), "object_cast__field3_", Node.class)));
this.object_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, OBJECT__PY_FLOAT_AS_DOUBLE_NODE_OBJECT_STATE_0_UPDATER.subUpdater(26, 1), ReferenceField.create(ObjectData.lookup_(), "object_raiseNode__field1_", Node.class)));
}
@Override
public double execute(VirtualFrame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doDouble(double)] || SpecializationActive[PyFloatAsDoubleNode.doPFloat(PFloat)] || SpecializationActive[PyFloatAsDoubleNode.doInt(int)] || SpecializationActive[PyFloatAsDoubleNode.doLong(long)] || SpecializationActive[PyFloatAsDoubleNode.doBoolean(boolean)] || SpecializationActive[PyFloatAsDoubleNode.doNative(Node, PythonAbstractNativeObject, GetClassNode, IsSubtypeNode, ReadDoubleNode)] || SpecializationActive[PyFloatAsDoubleNode.doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doDouble(double)] */ && arg1Value instanceof Double) {
double arg1Value_ = (double) arg1Value;
return PyFloatAsDoubleNode.doDouble(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doPFloat(PFloat)] */ && arg1Value instanceof PFloat) {
PFloat arg1Value_ = (PFloat) arg1Value;
return PyFloatAsDoubleNode.doPFloat(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doInt(int)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
return PyFloatAsDoubleNode.doInt(arg1Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doLong(long)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
return PyFloatAsDoubleNode.doLong(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doBoolean(boolean)] */ && arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return PyFloatAsDoubleNode.doBoolean(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doNative(Node, PythonAbstractNativeObject, GetClassNode, IsSubtypeNode, ReadDoubleNode)] */ && arg1Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg1Value_ = (PythonAbstractNativeObject) arg1Value;
NativeData s5_ = this.native_cache.get(arg0Value);
if (s5_ != null) {
if ((PyFloatAsDoubleNode.isFloatSubtype(s5_, arg1Value_, this.native_getClassNode_, s5_.isSubtype_))) {
return PyFloatAsDoubleNode.doNative(s5_, arg1Value_, this.native_getClassNode_, s5_.isSubtype_, s5_.read_);
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[PyFloatAsDoubleNode.doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)] */) {
ObjectData s6_ = this.object_cache.get(arg0Value);
if (s6_ != null) {
if ((!(PGuards.isDouble(arg1Value))) && (!(PGuards.isInteger(arg1Value))) && (!(PGuards.isBoolean(arg1Value))) && (!(PGuards.isPFloat(arg1Value))) && (!(PyFloatAsDoubleNode.isFloatSubtype(s6_, arg1Value, this.object_getClassNode_, s6_.isSubtype_)))) {
return PyFloatAsDoubleNode.doObject(frameValue, s6_, arg1Value, this.object_getClassNode_, s6_.isSubtype_, s6_.lookup_, s6_.call_, this.object_resultClassNode_, this.object_resultProfile_, s6_.resultSubtypeNode_, this.object_indexCheckNode_, this.object_indexNode_, this.object_cast_, s6_.warnNode_, this.object_raiseNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
@SuppressWarnings("unused")
private double executeAndSpecialize(VirtualFrame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Double) {
double arg1Value_ = (double) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyFloatAsDoubleNode.doDouble(double)] */;
this.state_0_.set(arg0Value, state_0);
return PyFloatAsDoubleNode.doDouble(arg1Value_);
}
if (arg1Value instanceof PFloat) {
PFloat arg1Value_ = (PFloat) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyFloatAsDoubleNode.doPFloat(PFloat)] */;
this.state_0_.set(arg0Value, state_0);
return PyFloatAsDoubleNode.doPFloat(arg1Value_);
}
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyFloatAsDoubleNode.doInt(int)] */;
this.state_0_.set(arg0Value, state_0);
return PyFloatAsDoubleNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyFloatAsDoubleNode.doLong(long)] */;
this.state_0_.set(arg0Value, state_0);
return PyFloatAsDoubleNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyFloatAsDoubleNode.doBoolean(boolean)] */;
this.state_0_.set(arg0Value, state_0);
return PyFloatAsDoubleNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg1Value_ = (PythonAbstractNativeObject) arg1Value;
while (true) {
int count5_ = 0;
NativeData s5_ = this.native_cache.getVolatile(arg0Value);
NativeData s5_original = s5_;
while (s5_ != null) {
if ((PyFloatAsDoubleNode.isFloatSubtype(s5_, arg1Value_, this.native_getClassNode_, s5_.isSubtype_))) {
break;
}
count5_++;
s5_ = null;
break;
}
if (s5_ == null && count5_ < 1) {
{
IsSubtypeNode isSubtype__ = arg0Value.insert((IsSubtypeNode.create()));
s5_ = arg0Value.insert(new NativeData());
if ((PyFloatAsDoubleNode.isFloatSubtype(s5_, arg1Value_, this.native_getClassNode_, isSubtype__))) {
Objects.requireNonNull(s5_.insert(isSubtype__), "Specialization 'doNative(Node, PythonAbstractNativeObject, GetClassNode, IsSubtypeNode, ReadDoubleNode)' cache 'isSubtype' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s5_.isSubtype_ = isSubtype__;
ReadDoubleNode read__ = s5_.insert((ReadDoubleNode.create()));
Objects.requireNonNull(read__, "Specialization 'doNative(Node, PythonAbstractNativeObject, GetClassNode, IsSubtypeNode, ReadDoubleNode)' cache 'read' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s5_.read_ = read__;
if (!this.native_cache.compareAndSet(arg0Value, s5_original, s5_)) {
continue;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[PyFloatAsDoubleNode.doNative(Node, PythonAbstractNativeObject, GetClassNode, IsSubtypeNode, ReadDoubleNode)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s5_ = null;
}
}
}
if (s5_ != null) {
return PyFloatAsDoubleNode.doNative(s5_, arg1Value_, this.native_getClassNode_, s5_.isSubtype_, s5_.read_);
}
break;
}
}
while (true) {
int count6_ = 0;
ObjectData s6_ = this.object_cache.getVolatile(arg0Value);
ObjectData s6_original = s6_;
while (s6_ != null) {
if ((!(PGuards.isDouble(arg1Value))) && (!(PGuards.isInteger(arg1Value))) && (!(PGuards.isBoolean(arg1Value))) && (!(PGuards.isPFloat(arg1Value))) && (!(PyFloatAsDoubleNode.isFloatSubtype(s6_, arg1Value, this.object_getClassNode_, s6_.isSubtype_)))) {
break;
}
count6_++;
s6_ = null;
break;
}
if (s6_ == null && count6_ < 1) {
if ((!(PGuards.isDouble(arg1Value))) && (!(PGuards.isInteger(arg1Value))) && (!(PGuards.isBoolean(arg1Value))) && (!(PGuards.isPFloat(arg1Value)))) {
IsSubtypeNode isSubtype__1 = arg0Value.insert((IsSubtypeNode.create()));
s6_ = arg0Value.insert(new ObjectData());
if ((!(PyFloatAsDoubleNode.isFloatSubtype(s6_, arg1Value, this.object_getClassNode_, isSubtype__1)))) {
Objects.requireNonNull(s6_.insert(isSubtype__1), "Specialization 'doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)' cache 'isSubtype' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.isSubtype_ = isSubtype__1;
LookupSpecialMethodSlotNode lookup__ = s6_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Float)));
Objects.requireNonNull(lookup__, "Specialization 'doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)' cache 'lookup' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.lookup_ = lookup__;
CallUnaryMethodNode call__ = s6_.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(call__, "Specialization 'doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)' cache 'call' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.call_ = call__;
IsSubtypeNode resultSubtypeNode__ = s6_.insert((IsSubtypeNode.create()));
Objects.requireNonNull(resultSubtypeNode__, "Specialization 'doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)' cache 'resultSubtypeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.resultSubtypeNode_ = resultSubtypeNode__;
WarnNode warnNode__ = s6_.insert((WarnNode.create()));
Objects.requireNonNull(warnNode__, "Specialization 'doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)' cache 'warnNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.warnNode_ = warnNode__;
if (!this.object_cache.compareAndSet(arg0Value, s6_original, s6_)) {
continue;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[PyFloatAsDoubleNode.doObject(VirtualFrame, Node, Object, GetClassNode, IsSubtypeNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, InlineIsBuiltinClassProfile, IsSubtypeNode, PyIndexCheckNode, PyNumberIndexNode, CastToJavaDoubleNode, WarnNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s6_ = null;
}
} else {
s6_ = null;
}
}
if (s6_ != null) {
return PyFloatAsDoubleNode.doObject(frameValue, s6_, arg1Value, this.object_getClassNode_, s6_.isSubtype_, s6_.lookup_, s6_.call_, this.object_resultClassNode_, this.object_resultProfile_, s6_.resultSubtypeNode_, this.object_indexCheckNode_, this.object_indexNode_, this.object_cast_, s6_.warnNode_, this.object_raiseNode_);
}
break;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyFloatAsDoubleNode.class)
@DenyReplace
private static final class NativeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doNative}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int native_state_0_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doNative}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node native_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doNative}
* Parameter: {@link IsSubtypeNode} isSubtype
*/
@Child IsSubtypeNode isSubtype_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doNative}
* Parameter: {@link ReadDoubleNode} read
*/
@Child ReadDoubleNode read_;
NativeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyFloatAsDoubleNode.class)
@DenyReplace
private static final class ObjectData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17-22: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
* 23-25: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link InlineIsBuiltinClassProfile} resultProfile
* Inline method: {@link InlineIsBuiltinClassProfileNodeGen#inline}
* 26: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int object_state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link GetClassNode} resultClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17-23: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyIndexCheckNode} indexCheckNode
* Inline method: {@link PyIndexCheckNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int object_state_1_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link CastToJavaDoubleNode} cast
* Inline method: {@link CastToJavaDoubleNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int object_state_2_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link IsSubtypeNode} isSubtype
*/
@Child IsSubtypeNode isSubtype_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link LookupSpecialMethodSlotNode} lookup
*/
@Child LookupSpecialMethodSlotNode lookup_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link CallUnaryMethodNode} call
*/
@Child CallUnaryMethodNode call_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link GetClassNode} resultClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_resultClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link IsSubtypeNode} resultSubtypeNode
*/
@Child IsSubtypeNode resultSubtypeNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyIndexCheckNode} indexCheckNode
* Inline method: {@link PyIndexCheckNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_indexCheckNode__field1_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_indexNode__field1_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_indexNode__field2_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_indexNode__field3_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_indexNode__field4_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link PyNumberIndexNode} indexNode
* Inline method: {@link PyNumberIndexNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_indexNode__field5_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link CastToJavaDoubleNode} cast
* Inline method: {@link CastToJavaDoubleNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_cast__field1_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link CastToJavaDoubleNode} cast
* Inline method: {@link CastToJavaDoubleNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_cast__field2_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link CastToJavaDoubleNode} cast
* Inline method: {@link CastToJavaDoubleNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_cast__field3_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link WarnNode} warnNode
*/
@Child WarnNode warnNode_;
/**
* Source Info:
* Specialization: {@link PyFloatAsDoubleNode#doObject}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node object_raiseNode__field1_;
ObjectData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyFloatAsDoubleNode.class)
@DenyReplace
private static final class Uncached extends PyFloatAsDoubleNode {
@Override
public double execute(VirtualFrame frameValue, Node arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof Double) {
double arg1Value_ = (double) arg1Value;
return PyFloatAsDoubleNode.doDouble(arg1Value_);
}
if (arg1Value instanceof PFloat) {
PFloat arg1Value_ = (PFloat) arg1Value;
return PyFloatAsDoubleNode.doPFloat(arg1Value_);
}
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
return PyFloatAsDoubleNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
return PyFloatAsDoubleNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return PyFloatAsDoubleNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg1Value_ = (PythonAbstractNativeObject) arg1Value;
if ((PyFloatAsDoubleNode.isFloatSubtype(arg0Value, arg1Value_, (GetClassNode.getUncached()), (IsSubtypeNode.getUncached())))) {
return PyFloatAsDoubleNode.doNative(arg0Value, arg1Value_, (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()), (ReadDoubleNode.getUncached()));
}
}
if ((!(PGuards.isDouble(arg1Value))) && (!(PGuards.isInteger(arg1Value))) && (!(PGuards.isBoolean(arg1Value))) && (!(PGuards.isPFloat(arg1Value))) && (!(PyFloatAsDoubleNode.isFloatSubtype(arg0Value, arg1Value, (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()))))) {
return PyFloatAsDoubleNode.doObject(frameValue, arg0Value, arg1Value, (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.Float)), (CallUnaryMethodNode.getUncached()), (GetClassNode.getUncached()), (InlineIsBuiltinClassProfile.getUncached()), (IsSubtypeNode.getUncached()), (PyIndexCheckNodeGen.getUncached()), (PyNumberIndexNodeGen.getUncached()), (CastToJavaDoubleNodeGen.getUncached()), (WarnNode.getUncached()), (Lazy.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy