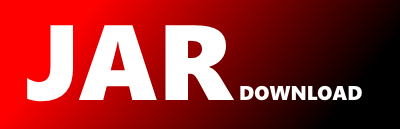
com.oracle.graal.python.lib.PyIterCheckNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.PythonAbstractObject;
import com.oracle.graal.python.builtins.objects.iterator.PBuiltinIterator;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.attributes.LookupCallableSlotInMRONode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyIterCheckNode#doIterator}
* Activation probability: 0.21250
* With/without class size: 6/0 bytes
* Specialization {@link PyIterCheckNode#doPythonObject}
* Activation probability: 0.18750
* With/without class size: 7/0 bytes
* Specialization {@link PyIterCheckNode#doInt}
* Activation probability: 0.16250
* With/without class size: 5/0 bytes
* Specialization {@link PyIterCheckNode#doLong}
* Activation probability: 0.13750
* With/without class size: 5/0 bytes
* Specialization {@link PyIterCheckNode#doBoolean}
* Activation probability: 0.11250
* With/without class size: 5/0 bytes
* Specialization {@link PyIterCheckNode#doDouble}
* Activation probability: 0.08750
* With/without class size: 5/0 bytes
* Specialization {@link PyIterCheckNode#doPBCT}
* Activation probability: 0.06250
* With/without class size: 4/0 bytes
* Specialization {@link PyIterCheckNode#doGeneric}
* Activation probability: 0.03750
* With/without class size: 4/4 bytes
*
*/
@GeneratedBy(PyIterCheckNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyIterCheckNodeGen extends PyIterCheckNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link PyIterCheckNode#doPythonObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_CLASS_NODE = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_0_UPDATER.subUpdater(8, 17), ReferenceField.create(MethodHandles.lookup(), "getClassNode_field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link PyIterCheckNode#doIterator}
* 1: SpecializationActive {@link PyIterCheckNode#doPythonObject}
* 2: SpecializationActive {@link PyIterCheckNode#doGeneric}
* 3: SpecializationActive {@link PyIterCheckNode#doInt}
* 4: SpecializationActive {@link PyIterCheckNode#doLong}
* 5: SpecializationActive {@link PyIterCheckNode#doBoolean}
* 6: SpecializationActive {@link PyIterCheckNode#doDouble}
* 7: SpecializationActive {@link PyIterCheckNode#doPBCT}
* 8-24: InlinedCache
* Specialization: {@link PyIterCheckNode#doPythonObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link PyIterCheckNode#doPythonObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getClassNode_field1_;
/**
* Source Info:
* Specialization: {@link PyIterCheckNode#doPythonObject}
* Parameter: {@link LookupCallableSlotInMRONode} lookupNext
*/
@Child private LookupCallableSlotInMRONode lookupNext;
/**
* Source Info:
* Specialization: {@link PyIterCheckNode#doGeneric}
* Parameter: {@link InteropLibrary} interopLibrary
*/
@Child private InteropLibrary generic_interopLibrary_;
private PyIterCheckNodeGen() {
}
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111) != 0 /* is SpecializationActive[PyIterCheckNode.doIterator(PBuiltinIterator)] || SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] || SpecializationActive[PyIterCheckNode.doInt(Integer)] || SpecializationActive[PyIterCheckNode.doLong(Long)] || SpecializationActive[PyIterCheckNode.doBoolean(Boolean)] || SpecializationActive[PyIterCheckNode.doDouble(Double)] || SpecializationActive[PyIterCheckNode.doPBCT(PythonBuiltinClassType)] || SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyIterCheckNode.doIterator(PBuiltinIterator)] */ && arg1Value instanceof PBuiltinIterator) {
PBuiltinIterator arg1Value_ = (PBuiltinIterator) arg1Value;
return PyIterCheckNode.doIterator(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] */ && arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
{
LookupCallableSlotInMRONode lookupNext_ = this.lookupNext;
if (lookupNext_ != null) {
return PyIterCheckNode.doPythonObject(this, arg1Value_, INLINED_GET_CLASS_NODE, lookupNext_);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyIterCheckNode.doInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyIterCheckNode.doInt(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyIterCheckNode.doLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyIterCheckNode.doLong(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[PyIterCheckNode.doBoolean(Boolean)] */ && arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return PyIterCheckNode.doBoolean(arg1Value_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[PyIterCheckNode.doDouble(Double)] */ && arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return PyIterCheckNode.doDouble(arg1Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[PyIterCheckNode.doPBCT(PythonBuiltinClassType)] */ && arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return PyIterCheckNode.doPBCT(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */) {
{
InteropLibrary interopLibrary__ = this.generic_interopLibrary_;
if (interopLibrary__ != null) {
LookupCallableSlotInMRONode lookupNext_1 = this.lookupNext;
if (lookupNext_1 != null) {
return PyIterCheckNode.doGeneric(this, arg1Value, interopLibrary__, INLINED_GET_CLASS_NODE, lookupNext_1);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof PBuiltinIterator) {
PBuiltinIterator arg1Value_ = (PBuiltinIterator) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyIterCheckNode.doIterator(PBuiltinIterator)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doIterator(arg1Value_);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */ && arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
LookupCallableSlotInMRONode lookupNext_;
LookupCallableSlotInMRONode lookupNext__shared = this.lookupNext;
if (lookupNext__shared != null) {
lookupNext_ = lookupNext__shared;
} else {
lookupNext_ = this.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Next)));
if (lookupNext_ == null) {
throw new IllegalStateException("Specialization 'doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)' contains a shared cache with name 'lookupNext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupNext == null) {
VarHandle.storeStoreFence();
this.lookupNext = lookupNext_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doPythonObject(this, arg1Value_, INLINED_GET_CLASS_NODE, lookupNext_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyIterCheckNode.doInt(Integer)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyIterCheckNode.doLong(Long)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[PyIterCheckNode.doBoolean(Boolean)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[PyIterCheckNode.doDouble(Double)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doDouble(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[PyIterCheckNode.doPBCT(PythonBuiltinClassType)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doPBCT(arg1Value_);
}
InteropLibrary interopLibrary__ = this.insert((INTEROP_LIBRARY_.createDispatched(3)));
Objects.requireNonNull(interopLibrary__, "Specialization 'doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)' cache 'interopLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.generic_interopLibrary_ = interopLibrary__;
LookupCallableSlotInMRONode lookupNext_1;
LookupCallableSlotInMRONode lookupNext_1_shared = this.lookupNext;
if (lookupNext_1_shared != null) {
lookupNext_1 = lookupNext_1_shared;
} else {
lookupNext_1 = this.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Next)));
if (lookupNext_1 == null) {
throw new IllegalStateException("Specialization 'doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)' contains a shared cache with name 'lookupNext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupNext == null) {
VarHandle.storeStoreFence();
this.lookupNext = lookupNext_1;
}
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */;
this.state_0_ = state_0;
return PyIterCheckNode.doGeneric(this, arg1Value, interopLibrary__, INLINED_GET_CLASS_NODE, lookupNext_1);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11111111) & ((state_0 & 0b11111111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyIterCheckNode create() {
return new PyIterCheckNodeGen();
}
@NeverDefault
public static PyIterCheckNode getUncached() {
return PyIterCheckNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#getClassNode_field1_}
*
- {@link Inlined#lookupNext}
*
- {@link Inlined#generic_interopLibrary_}
*
*/
@NeverDefault
public static PyIterCheckNode inline(@RequiredField(bits = 25, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyIterCheckNodeGen.Inlined(target);
}
@GeneratedBy(PyIterCheckNode.class)
@DenyReplace
private static final class Inlined extends PyIterCheckNode {
/**
* State Info:
* 0: SpecializationActive {@link PyIterCheckNode#doIterator}
* 1: SpecializationActive {@link PyIterCheckNode#doPythonObject}
* 2: SpecializationActive {@link PyIterCheckNode#doGeneric}
* 3: SpecializationActive {@link PyIterCheckNode#doInt}
* 4: SpecializationActive {@link PyIterCheckNode#doLong}
* 5: SpecializationActive {@link PyIterCheckNode#doBoolean}
* 6: SpecializationActive {@link PyIterCheckNode#doDouble}
* 7: SpecializationActive {@link PyIterCheckNode#doPBCT}
* 8-24: InlinedCache
* Specialization: {@link PyIterCheckNode#doPythonObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField getClassNode_field1_;
private final ReferenceField lookupNext;
private final ReferenceField generic_interopLibrary_;
/**
* Source Info:
* Specialization: {@link PyIterCheckNode#doPythonObject}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getClassNode;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyIterCheckNode.class);
this.state_0_ = target.getState(0, 25);
this.getClassNode_field1_ = target.getReference(1, Node.class);
this.lookupNext = target.getReference(2, LookupCallableSlotInMRONode.class);
this.generic_interopLibrary_ = target.getReference(3, InteropLibrary.class);
this.getClassNode = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, state_0_.subUpdater(8, 17), getClassNode_field1_));
}
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b11111111) != 0 /* is SpecializationActive[PyIterCheckNode.doIterator(PBuiltinIterator)] || SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] || SpecializationActive[PyIterCheckNode.doInt(Integer)] || SpecializationActive[PyIterCheckNode.doLong(Long)] || SpecializationActive[PyIterCheckNode.doBoolean(Boolean)] || SpecializationActive[PyIterCheckNode.doDouble(Double)] || SpecializationActive[PyIterCheckNode.doPBCT(PythonBuiltinClassType)] || SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyIterCheckNode.doIterator(PBuiltinIterator)] */ && arg1Value instanceof PBuiltinIterator) {
PBuiltinIterator arg1Value_ = (PBuiltinIterator) arg1Value;
return PyIterCheckNode.doIterator(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] */ && arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
{
LookupCallableSlotInMRONode lookupNext_ = this.lookupNext.get(arg0Value);
if (lookupNext_ != null) {
return PyIterCheckNode.doPythonObject(arg0Value, arg1Value_, this.getClassNode, lookupNext_);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyIterCheckNode.doInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyIterCheckNode.doInt(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyIterCheckNode.doLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyIterCheckNode.doLong(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[PyIterCheckNode.doBoolean(Boolean)] */ && arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return PyIterCheckNode.doBoolean(arg1Value_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[PyIterCheckNode.doDouble(Double)] */ && arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return PyIterCheckNode.doDouble(arg1Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[PyIterCheckNode.doPBCT(PythonBuiltinClassType)] */ && arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return PyIterCheckNode.doPBCT(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */) {
{
InteropLibrary interopLibrary__ = this.generic_interopLibrary_.get(arg0Value);
if (interopLibrary__ != null) {
LookupCallableSlotInMRONode lookupNext_1 = this.lookupNext.get(arg0Value);
if (lookupNext_1 != null) {
return PyIterCheckNode.doGeneric(arg0Value, arg1Value, interopLibrary__, this.getClassNode, lookupNext_1);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof PBuiltinIterator) {
PBuiltinIterator arg1Value_ = (PBuiltinIterator) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyIterCheckNode.doIterator(PBuiltinIterator)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doIterator(arg1Value_);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */ && arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
LookupCallableSlotInMRONode lookupNext_;
LookupCallableSlotInMRONode lookupNext__shared = this.lookupNext.get(arg0Value);
if (lookupNext__shared != null) {
lookupNext_ = lookupNext__shared;
} else {
lookupNext_ = arg0Value.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Next)));
if (lookupNext_ == null) {
throw new IllegalStateException("Specialization 'doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)' contains a shared cache with name 'lookupNext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupNext.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.lookupNext.set(arg0Value, lookupNext_);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doPythonObject(arg0Value, arg1Value_, this.getClassNode, lookupNext_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyIterCheckNode.doInt(Integer)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyIterCheckNode.doLong(Long)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[PyIterCheckNode.doBoolean(Boolean)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[PyIterCheckNode.doDouble(Double)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doDouble(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[PyIterCheckNode.doPBCT(PythonBuiltinClassType)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doPBCT(arg1Value_);
}
InteropLibrary interopLibrary__ = arg0Value.insert((INTEROP_LIBRARY_.createDispatched(3)));
Objects.requireNonNull(interopLibrary__, "Specialization 'doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)' cache 'interopLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.generic_interopLibrary_.set(arg0Value, interopLibrary__);
LookupCallableSlotInMRONode lookupNext_1;
LookupCallableSlotInMRONode lookupNext_1_shared = this.lookupNext.get(arg0Value);
if (lookupNext_1_shared != null) {
lookupNext_1 = lookupNext_1_shared;
} else {
lookupNext_1 = arg0Value.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Next)));
if (lookupNext_1 == null) {
throw new IllegalStateException("Specialization 'doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)' contains a shared cache with name 'lookupNext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupNext.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.lookupNext.set(arg0Value, lookupNext_1);
}
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[PyIterCheckNode.doPythonObject(Node, PythonAbstractObject, GetClassNode, LookupCallableSlotInMRONode)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyIterCheckNode.doGeneric(Node, Object, InteropLibrary, GetClassNode, LookupCallableSlotInMRONode)] */;
this.state_0_.set(arg0Value, state_0);
return PyIterCheckNode.doGeneric(arg0Value, arg1Value, interopLibrary__, this.getClassNode, lookupNext_1);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyIterCheckNode.class)
@DenyReplace
private static final class Uncached extends PyIterCheckNode {
@TruffleBoundary
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof PBuiltinIterator) {
PBuiltinIterator arg1Value_ = (PBuiltinIterator) arg1Value;
return PyIterCheckNode.doIterator(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyIterCheckNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyIterCheckNode.doLong(arg1Value_);
}
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return PyIterCheckNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return PyIterCheckNode.doDouble(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return PyIterCheckNode.doPBCT(arg1Value_);
}
return PyIterCheckNode.doGeneric(arg0Value, arg1Value, (INTEROP_LIBRARY_.getUncached()), (GetClassNode.getUncached()), (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.Next)));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy