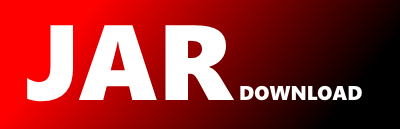
com.oracle.graal.python.lib.PyLongCheckExactNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.cext.PythonNativeVoidPtr;
import com.oracle.graal.python.builtins.objects.ints.PInt;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
/**
* Debug Info:
* Specialization {@link PyLongCheckExactNode#doInt}
* Activation probability: 0.27381
* With/without class size: 7/0 bytes
* Specialization {@link PyLongCheckExactNode#doLong}
* Activation probability: 0.23095
* With/without class size: 6/0 bytes
* Specialization {@link PyLongCheckExactNode#doBuiltinPInt}
* Activation probability: 0.18810
* With/without class size: 6/0 bytes
* Specialization {@link PyLongCheckExactNode#doOtherPInt}
* Activation probability: 0.14524
* With/without class size: 5/0 bytes
* Specialization {@link PyLongCheckExactNode#doOther}
* Activation probability: 0.10238
* With/without class size: 5/0 bytes
* Specialization {@link PyLongCheckExactNode#doNativePtr}
* Activation probability: 0.05952
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(PyLongCheckExactNode.class)
@SuppressWarnings("javadoc")
public final class PyLongCheckExactNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyLongCheckExactNode getUncached() {
return PyLongCheckExactNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static PyLongCheckExactNode inline(@RequiredField(bits = 6, value = StateField.class) InlineTarget target) {
return new PyLongCheckExactNodeGen.Inlined(target);
}
@GeneratedBy(PyLongCheckExactNode.class)
@DenyReplace
private static final class Inlined extends PyLongCheckExactNode {
/**
* State Info:
* 0: SpecializationActive {@link PyLongCheckExactNode#doInt}
* 1: SpecializationActive {@link PyLongCheckExactNode#doLong}
* 2: SpecializationActive {@link PyLongCheckExactNode#doBuiltinPInt}
* 3: SpecializationActive {@link PyLongCheckExactNode#doOtherPInt}
* 4: SpecializationActive {@link PyLongCheckExactNode#doOther}
* 5: SpecializationActive {@link PyLongCheckExactNode#doNativePtr}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyLongCheckExactNode.class);
this.state_0_ = target.getState(0, 6);
}
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyLongCheckExactNode.doInt(Integer)] || SpecializationActive[PyLongCheckExactNode.doLong(Long)] || SpecializationActive[PyLongCheckExactNode.doBuiltinPInt(PInt)] || SpecializationActive[PyLongCheckExactNode.doOtherPInt(PInt)] || SpecializationActive[PyLongCheckExactNode.doOther(Object)] || SpecializationActive[PyLongCheckExactNode.doNativePtr(PythonNativeVoidPtr)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyLongCheckExactNode.doInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyLongCheckExactNode.doInt(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyLongCheckExactNode.doLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyLongCheckExactNode.doLong(arg1Value_);
}
if ((state_0 & 0b1100) != 0 /* is SpecializationActive[PyLongCheckExactNode.doBuiltinPInt(PInt)] || SpecializationActive[PyLongCheckExactNode.doOtherPInt(PInt)] */ && arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyLongCheckExactNode.doBuiltinPInt(PInt)] */) {
if ((PGuards.isBuiltinPInt(arg1Value_))) {
return PyLongCheckExactNode.doBuiltinPInt(arg1Value_);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyLongCheckExactNode.doOtherPInt(PInt)] */) {
if ((!(PGuards.isBuiltinPInt(arg1Value_)))) {
return PyLongCheckExactNode.doOtherPInt(arg1Value_);
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyLongCheckExactNode.doOther(Object)] */) {
if ((!(PyLongCheckExactNode.canBeBuiltinInt(arg1Value)))) {
return PyLongCheckExactNode.doOther(arg1Value);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[PyLongCheckExactNode.doNativePtr(PythonNativeVoidPtr)] */ && arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
return PyLongCheckExactNode.doNativePtr(arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyLongCheckExactNode.doInt(Integer)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckExactNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyLongCheckExactNode.doLong(Long)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckExactNode.doLong(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
if ((PGuards.isBuiltinPInt(arg1Value_))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[PyLongCheckExactNode.doBuiltinPInt(PInt)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckExactNode.doBuiltinPInt(arg1Value_);
}
if ((!(PGuards.isBuiltinPInt(arg1Value_)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyLongCheckExactNode.doOtherPInt(PInt)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckExactNode.doOtherPInt(arg1Value_);
}
}
if ((!(PyLongCheckExactNode.canBeBuiltinInt(arg1Value)))) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyLongCheckExactNode.doOther(Object)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckExactNode.doOther(arg1Value);
}
if (arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[PyLongCheckExactNode.doNativePtr(PythonNativeVoidPtr)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckExactNode.doNativePtr(arg1Value_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyLongCheckExactNode.class)
@DenyReplace
private static final class Uncached extends PyLongCheckExactNode {
@TruffleBoundary
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyLongCheckExactNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyLongCheckExactNode.doLong(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
if ((PGuards.isBuiltinPInt(arg1Value_))) {
return PyLongCheckExactNode.doBuiltinPInt(arg1Value_);
}
if ((!(PGuards.isBuiltinPInt(arg1Value_)))) {
return PyLongCheckExactNode.doOtherPInt(arg1Value_);
}
}
if ((!(PyLongCheckExactNode.canBeBuiltinInt(arg1Value)))) {
return PyLongCheckExactNode.doOther(arg1Value);
}
if (arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
return PyLongCheckExactNode.doNativePtr(arg1Value_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy