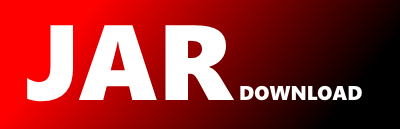
com.oracle.graal.python.lib.PyLongCheckNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.ints.PInt;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyLongCheckNode#doBool}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link PyLongCheckNode#doInt}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link PyLongCheckNode#doLong}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link PyLongCheckNode#doPInt}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link PyLongCheckNode#doGeneric}
* Activation probability: 0.08000
* With/without class size: 6/15 bytes
*
*/
@GeneratedBy(PyLongCheckNode.class)
@SuppressWarnings("javadoc")
public final class PyLongCheckNodeGen {
private static final StateField GENERIC__PY_LONG_CHECK_NODE_GENERIC_STATE_0_UPDATER = StateField.create(GenericData.lookup_(), "generic_state_0_");
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
@NeverDefault
public static PyLongCheckNode getUncached() {
return PyLongCheckNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#generic_cache}
*
*/
@NeverDefault
public static PyLongCheckNode inline(@RequiredField(bits = 5, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyLongCheckNodeGen.Inlined(target);
}
@GeneratedBy(PyLongCheckNode.class)
@DenyReplace
private static final class Inlined extends PyLongCheckNode {
/**
* State Info:
* 0: SpecializationActive {@link PyLongCheckNode#doBool}
* 1: SpecializationActive {@link PyLongCheckNode#doInt}
* 2: SpecializationActive {@link PyLongCheckNode#doLong}
* 3: SpecializationActive {@link PyLongCheckNode#doPInt}
* 4: SpecializationActive {@link PyLongCheckNode#doGeneric}
*
*/
private final StateField state_0_;
private final ReferenceField generic_cache;
/**
* Source Info:
* Specialization: {@link PyLongCheckNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode generic_getClassNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyLongCheckNode.class);
this.state_0_ = target.getState(0, 5);
this.generic_cache = target.getReference(1, GenericData.class);
this.generic_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GENERIC__PY_LONG_CHECK_NODE_GENERIC_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GenericData.lookup_(), "generic_getClassNode__field1_", Node.class)));
}
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyLongCheckNode.doBool(Boolean)] || SpecializationActive[PyLongCheckNode.doInt(Integer)] || SpecializationActive[PyLongCheckNode.doLong(Long)] || SpecializationActive[PyLongCheckNode.doPInt(PInt)] || SpecializationActive[PyLongCheckNode.doGeneric(Node, Object, GetClassNode, IsSubtypeNode, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyLongCheckNode.doBool(Boolean)] */ && arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return PyLongCheckNode.doBool(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyLongCheckNode.doInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyLongCheckNode.doInt(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyLongCheckNode.doLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyLongCheckNode.doLong(arg1Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyLongCheckNode.doPInt(PInt)] */ && arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
return PyLongCheckNode.doPInt(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyLongCheckNode.doGeneric(Node, Object, GetClassNode, IsSubtypeNode, InteropLibrary)] */) {
GenericData s4_ = this.generic_cache.get(arg0Value);
if (s4_ != null) {
return PyLongCheckNode.doGeneric(s4_, arg1Value, this.generic_getClassNode_, s4_.isSubtypeNode_, s4_.interopLibrary_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyLongCheckNode.doBool(Boolean)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckNode.doBool(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyLongCheckNode.doInt(Integer)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyLongCheckNode.doLong(Long)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckNode.doLong(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyLongCheckNode.doPInt(PInt)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckNode.doPInt(arg1Value_);
}
GenericData s4_ = arg0Value.insert(new GenericData());
IsSubtypeNode isSubtypeNode__ = s4_.insert((IsSubtypeNode.create()));
Objects.requireNonNull(isSubtypeNode__, "Specialization 'doGeneric(Node, Object, GetClassNode, IsSubtypeNode, InteropLibrary)' cache 'isSubtypeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.isSubtypeNode_ = isSubtypeNode__;
InteropLibrary interopLibrary__ = s4_.insert((INTEROP_LIBRARY_.createDispatched(3)));
Objects.requireNonNull(interopLibrary__, "Specialization 'doGeneric(Node, Object, GetClassNode, IsSubtypeNode, InteropLibrary)' cache 'interopLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.interopLibrary_ = interopLibrary__;
VarHandle.storeStoreFence();
this.generic_cache.set(arg0Value, s4_);
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyLongCheckNode.doGeneric(Node, Object, GetClassNode, IsSubtypeNode, InteropLibrary)] */;
this.state_0_.set(arg0Value, state_0);
return PyLongCheckNode.doGeneric(s4_, arg1Value, this.generic_getClassNode_, isSubtypeNode__, interopLibrary__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyLongCheckNode.class)
@DenyReplace
private static final class GenericData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyLongCheckNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int generic_state_0_;
/**
* Source Info:
* Specialization: {@link PyLongCheckNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node generic_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyLongCheckNode#doGeneric}
* Parameter: {@link IsSubtypeNode} isSubtypeNode
*/
@Child IsSubtypeNode isSubtypeNode_;
/**
* Source Info:
* Specialization: {@link PyLongCheckNode#doGeneric}
* Parameter: {@link InteropLibrary} interopLibrary
*/
@Child InteropLibrary interopLibrary_;
GenericData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyLongCheckNode.class)
@DenyReplace
private static final class Uncached extends PyLongCheckNode {
@TruffleBoundary
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return PyLongCheckNode.doBool(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return PyLongCheckNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return PyLongCheckNode.doLong(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
return PyLongCheckNode.doPInt(arg1Value_);
}
return PyLongCheckNode.doGeneric(arg0Value, arg1Value, (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()), (INTEROP_LIBRARY_.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy