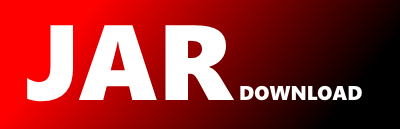
com.oracle.graal.python.lib.PyMemoryViewFromObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.buffer.PythonBufferAccessLibrary;
import com.oracle.graal.python.builtins.objects.buffer.PythonBufferAcquireLibrary;
import com.oracle.graal.python.builtins.objects.cext.PythonNativeObject;
import com.oracle.graal.python.builtins.objects.cext.capi.CExtNodes.CreateMemoryViewFromNativeNode;
import com.oracle.graal.python.builtins.objects.cext.capi.CExtNodesFactory.CreateMemoryViewFromNativeNodeGen;
import com.oracle.graal.python.builtins.objects.memoryview.PMemoryView;
import com.oracle.graal.python.builtins.objects.memoryview.MemoryViewNodes.InitFlagsNode;
import com.oracle.graal.python.builtins.objects.memoryview.MemoryViewNodesFactory.InitFlagsNodeGen;
import com.oracle.graal.python.builtins.objects.mmap.PMMap;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.attributes.ReadAttributeFromObjectNode;
import com.oracle.graal.python.nodes.call.CallNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString.CodePointAtIndexNode;
import com.oracle.truffle.api.strings.TruffleString.CodePointLengthNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyMemoryViewFromObject#fromMemoryView}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link PyMemoryViewFromObject#fromNative}
* Activation probability: 0.26000
* With/without class size: 9/4 bytes
* Specialization {@link PyMemoryViewFromObject#fromMMap}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link PyMemoryViewFromObject#fromManaged}
* Activation probability: 0.14000
* With/without class size: 10/27 bytes
* Specialization {@link PyMemoryViewFromObject#fromManaged}
* Activation probability: 0.08000
* With/without class size: 7/23 bytes
*
*/
@GeneratedBy(PyMemoryViewFromObject.class)
@SuppressWarnings("javadoc")
public final class PyMemoryViewFromObjectNodeGen extends PyMemoryViewFromObject {
private static final StateField FROM_MANAGED0__PY_MEMORY_VIEW_FROM_OBJECT_FROM_MANAGED0_STATE_0_UPDATER = StateField.create(FromManaged0Data.lookup_(), "fromManaged0_state_0_");
private static final StateField FROM_MANAGED1__PY_MEMORY_VIEW_FROM_OBJECT_FROM_MANAGED1_STATE_0_UPDATER = StateField.create(FromManaged1Data.lookup_(), "fromManaged1_state_0_");
static final ReferenceField FROM_MANAGED0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "fromManaged0_cache", FromManaged0Data.class);
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link InlinedConditionProfile} hasSlotProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_FROM_MANAGED0_HAS_SLOT_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, FROM_MANAGED0__PY_MEMORY_VIEW_FROM_OBJECT_FROM_MANAGED0_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_FROM_MANAGED0_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, FROM_MANAGED0__PY_MEMORY_VIEW_FROM_OBJECT_FROM_MANAGED0_STATE_0_UPDATER.subUpdater(2, 17), ReferenceField.create(FromManaged0Data.lookup_(), "fromManaged0_getClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link InitFlagsNode} initFlagsNode
* Inline method: {@link InitFlagsNodeGen#inline}
*/
private static final InitFlagsNode INLINED_FROM_MANAGED0_INIT_FLAGS_NODE_ = InitFlagsNodeGen.inline(InlineTarget.create(InitFlagsNode.class));
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link InlinedConditionProfile} hasSlotProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_FROM_MANAGED1_HAS_SLOT_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, FROM_MANAGED1__PY_MEMORY_VIEW_FROM_OBJECT_FROM_MANAGED1_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_FROM_MANAGED1_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, FROM_MANAGED1__PY_MEMORY_VIEW_FROM_OBJECT_FROM_MANAGED1_STATE_0_UPDATER.subUpdater(2, 17), ReferenceField.create(FromManaged1Data.lookup_(), "fromManaged1_getClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link InitFlagsNode} initFlagsNode
* Inline method: {@link InitFlagsNodeGen#inline}
*/
private static final InitFlagsNode INLINED_FROM_MANAGED1_INIT_FLAGS_NODE_ = InitFlagsNodeGen.inline(InlineTarget.create(InitFlagsNode.class));
private static final LibraryFactory PYTHON_BUFFER_ACQUIRE_LIBRARY_ = LibraryFactory.resolve(PythonBufferAcquireLibrary.class);
private static final LibraryFactory PYTHON_BUFFER_ACCESS_LIBRARY_ = LibraryFactory.resolve(PythonBufferAccessLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link PyMemoryViewFromObject#fromMemoryView}
* 1: SpecializationActive {@link PyMemoryViewFromObject#fromNative}
* 2: SpecializationActive {@link PyMemoryViewFromObject#fromMMap}
* 3: SpecializationActive {@link PyMemoryViewFromObject#fromManaged}
* 4: SpecializationActive {@link PyMemoryViewFromObject#fromManaged}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromMemoryView}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromMMap}
* Parameter: {@link CodePointLengthNode} lengthNode
*/
@Child private CodePointLengthNode lengthNode;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromMMap}
* Parameter: {@link CodePointAtIndexNode} atIndexNode
*/
@Child private CodePointAtIndexNode atIndexNode;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromNative}
* Parameter: {@link CreateMemoryViewFromNativeNode} fromNativeNode
*/
@Child private CreateMemoryViewFromNativeNode fromNative_fromNativeNode_;
@UnsafeAccessedField @Child private FromManaged0Data fromManaged0_cache;
@Child private FromManaged1Data fromManaged1_cache;
private PyMemoryViewFromObjectNodeGen() {
}
@ExplodeLoop
@Override
public PMemoryView execute(VirtualFrame frameValue, Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromMemoryView(PMemoryView, PythonObjectFactory)] || SpecializationActive[PyMemoryViewFromObject.fromNative(PythonNativeObject, CreateMemoryViewFromNativeNode)] || SpecializationActive[PyMemoryViewFromObject.fromMMap(PMMap, PythonObjectFactory, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromMemoryView(PMemoryView, PythonObjectFactory)] */ && arg0Value instanceof PMemoryView) {
PMemoryView arg0Value_ = (PMemoryView) arg0Value;
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
return fromMemoryView(arg0Value_, factory_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromNative(PythonNativeObject, CreateMemoryViewFromNativeNode)] */ && arg0Value instanceof PythonNativeObject) {
PythonNativeObject arg0Value_ = (PythonNativeObject) arg0Value;
{
CreateMemoryViewFromNativeNode fromNativeNode__ = this.fromNative_fromNativeNode_;
if (fromNativeNode__ != null) {
return fromNative(arg0Value_, fromNativeNode__);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromMMap(PMMap, PythonObjectFactory, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PMMap) {
PMMap arg0Value_ = (PMMap) arg0Value;
{
PythonObjectFactory factory_1 = this.factory;
if (factory_1 != null) {
CodePointLengthNode lengthNode_ = this.lengthNode;
if (lengthNode_ != null) {
CodePointAtIndexNode atIndexNode_ = this.atIndexNode;
if (atIndexNode_ != null) {
return fromMMap(arg0Value_, factory_1, lengthNode_, atIndexNode_);
}
}
}
}
}
if ((state_0 & 0b11000) != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */) {
FromManaged0Data s3_ = this.fromManaged0_cache;
while (s3_ != null) {
{
PythonObjectFactory factory_2 = this.factory;
if (factory_2 != null) {
CodePointLengthNode lengthNode_1 = this.lengthNode;
if (lengthNode_1 != null) {
CodePointAtIndexNode atIndexNode_1 = this.atIndexNode;
if (atIndexNode_1 != null) {
if ((s3_.bufferAcquireLib_.accepts(arg0Value)) && (!(PGuards.isMemoryView(arg0Value))) && (!(PGuards.isNativeObject(arg0Value))) && (!(PGuards.isMMap(arg0Value)))) {
Node inliningTarget__ = (s3_);
return fromManaged(frameValue, arg0Value, inliningTarget__, s3_.bufferAcquireLib_, s3_.bufferLib_, INLINED_FROM_MANAGED0_HAS_SLOT_PROFILE_, INLINED_FROM_MANAGED0_GET_CLASS_NODE_, s3_.readGetBufferNode_, s3_.readReleaseBufferNode_, s3_.callNode_, factory_2, INLINED_FROM_MANAGED0_INIT_FLAGS_NODE_, lengthNode_1, atIndexNode_1);
}
}
}
}
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */) {
FromManaged1Data s4_ = this.fromManaged1_cache;
if (s4_ != null) {
{
PythonObjectFactory factory_2 = this.factory;
if (factory_2 != null) {
CodePointLengthNode lengthNode_1 = this.lengthNode;
if (lengthNode_1 != null) {
CodePointAtIndexNode atIndexNode_1 = this.atIndexNode;
if (atIndexNode_1 != null) {
if ((!(PGuards.isMemoryView(arg0Value))) && (!(PGuards.isNativeObject(arg0Value))) && (!(PGuards.isMMap(arg0Value)))) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
Node inliningTarget__ = (s4_);
PythonBufferAcquireLibrary bufferAcquireLib__ = (PYTHON_BUFFER_ACQUIRE_LIBRARY_.getUncached());
return fromManaged(frameValue, arg0Value, inliningTarget__, bufferAcquireLib__, s4_.bufferLib_, INLINED_FROM_MANAGED1_HAS_SLOT_PROFILE_, INLINED_FROM_MANAGED1_GET_CLASS_NODE_, s4_.readGetBufferNode_, s4_.readReleaseBufferNode_, s4_.callNode_, factory_2, INLINED_FROM_MANAGED1_INIT_FLAGS_NODE_, lengthNode_1, atIndexNode_1);
}
} finally {
encapsulating_.set(prev_);
}
}
}
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value);
}
private PMemoryView executeAndSpecialize(VirtualFrame frameValue, Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof PMemoryView) {
PMemoryView arg0Value_ = (PMemoryView) arg0Value;
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory;
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = this.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'fromMemoryView(PMemoryView, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyMemoryViewFromObject.fromMemoryView(PMemoryView, PythonObjectFactory)] */;
this.state_0_ = state_0;
return fromMemoryView(arg0Value_, factory_);
}
if (arg0Value instanceof PythonNativeObject) {
PythonNativeObject arg0Value_ = (PythonNativeObject) arg0Value;
CreateMemoryViewFromNativeNode fromNativeNode__ = this.insert((CreateMemoryViewFromNativeNodeGen.create()));
Objects.requireNonNull(fromNativeNode__, "Specialization 'fromNative(PythonNativeObject, CreateMemoryViewFromNativeNode)' cache 'fromNativeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.fromNative_fromNativeNode_ = fromNativeNode__;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyMemoryViewFromObject.fromNative(PythonNativeObject, CreateMemoryViewFromNativeNode)] */;
this.state_0_ = state_0;
return fromNative(arg0Value_, fromNativeNode__);
}
if (arg0Value instanceof PMMap) {
PMMap arg0Value_ = (PMMap) arg0Value;
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory;
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = this.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'fromMMap(PMMap, PythonObjectFactory, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_1;
}
CodePointLengthNode lengthNode_;
CodePointLengthNode lengthNode__shared = this.lengthNode;
if (lengthNode__shared != null) {
lengthNode_ = lengthNode__shared;
} else {
lengthNode_ = this.insert((CodePointLengthNode.create()));
if (lengthNode_ == null) {
throw new IllegalStateException("Specialization 'fromMMap(PMMap, PythonObjectFactory, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'lengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lengthNode == null) {
VarHandle.storeStoreFence();
this.lengthNode = lengthNode_;
}
CodePointAtIndexNode atIndexNode_;
CodePointAtIndexNode atIndexNode__shared = this.atIndexNode;
if (atIndexNode__shared != null) {
atIndexNode_ = atIndexNode__shared;
} else {
atIndexNode_ = this.insert((CodePointAtIndexNode.create()));
if (atIndexNode_ == null) {
throw new IllegalStateException("Specialization 'fromMMap(PMMap, PythonObjectFactory, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'atIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.atIndexNode == null) {
VarHandle.storeStoreFence();
this.atIndexNode = atIndexNode_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyMemoryViewFromObject.fromMMap(PMMap, PythonObjectFactory, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return fromMMap(arg0Value_, factory_1, lengthNode_, atIndexNode_);
}
{
Node inliningTarget__ = null;
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count3_ = 0;
FromManaged0Data s3_ = FROM_MANAGED0_CACHE_UPDATER.getVolatile(this);
FromManaged0Data s3_original = s3_;
while (s3_ != null) {
{
PythonObjectFactory factory_2 = this.factory;
if (factory_2 != null) {
CodePointLengthNode lengthNode_1 = this.lengthNode;
if (lengthNode_1 != null) {
CodePointAtIndexNode atIndexNode_1 = this.atIndexNode;
if (atIndexNode_1 != null) {
if ((s3_.bufferAcquireLib_.accepts(arg0Value)) && (!(PGuards.isMemoryView(arg0Value))) && (!(PGuards.isNativeObject(arg0Value))) && (!(PGuards.isMMap(arg0Value)))) {
inliningTarget__ = (s3_);
break;
}
}
}
}
}
count3_++;
s3_ = s3_.next_;
}
if (s3_ == null) {
if ((!(PGuards.isMemoryView(arg0Value))) && (!(PGuards.isNativeObject(arg0Value))) && (!(PGuards.isMMap(arg0Value))) && count3_ < (3)) {
// assert (s3_.bufferAcquireLib_.accepts(arg0Value));
s3_ = this.insert(new FromManaged0Data(s3_original));
inliningTarget__ = (s3_);
PythonBufferAcquireLibrary bufferAcquireLib__ = s3_.insert((PYTHON_BUFFER_ACQUIRE_LIBRARY_.create(arg0Value)));
Objects.requireNonNull(bufferAcquireLib__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'bufferAcquireLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.bufferAcquireLib_ = bufferAcquireLib__;
PythonBufferAccessLibrary bufferLib__ = s3_.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(bufferLib__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.bufferLib_ = bufferLib__;
ReadAttributeFromObjectNode readGetBufferNode__ = s3_.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readGetBufferNode__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'readGetBufferNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.readGetBufferNode_ = readGetBufferNode__;
ReadAttributeFromObjectNode readReleaseBufferNode__ = s3_.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readReleaseBufferNode__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'readReleaseBufferNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.readReleaseBufferNode_ = readReleaseBufferNode__;
CallNode callNode__ = s3_.insert((CallNode.create()));
Objects.requireNonNull(callNode__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.callNode_ = callNode__;
PythonObjectFactory factory_2;
PythonObjectFactory factory_2_shared = this.factory;
if (factory_2_shared != null) {
factory_2 = factory_2_shared;
} else {
factory_2 = s3_.insert((PythonObjectFactory.create()));
if (factory_2 == null) {
throw new IllegalStateException("Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_2;
}
CodePointLengthNode lengthNode_1;
CodePointLengthNode lengthNode_1_shared = this.lengthNode;
if (lengthNode_1_shared != null) {
lengthNode_1 = lengthNode_1_shared;
} else {
lengthNode_1 = s3_.insert((CodePointLengthNode.create()));
if (lengthNode_1 == null) {
throw new IllegalStateException("Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'lengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lengthNode == null) {
this.lengthNode = lengthNode_1;
}
CodePointAtIndexNode atIndexNode_1;
CodePointAtIndexNode atIndexNode_1_shared = this.atIndexNode;
if (atIndexNode_1_shared != null) {
atIndexNode_1 = atIndexNode_1_shared;
} else {
atIndexNode_1 = s3_.insert((CodePointAtIndexNode.create()));
if (atIndexNode_1 == null) {
throw new IllegalStateException("Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'atIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.atIndexNode == null) {
this.atIndexNode = atIndexNode_1;
}
if (!FROM_MANAGED0_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
}
}
if (s3_ != null) {
return fromManaged(frameValue, arg0Value, inliningTarget__, s3_.bufferAcquireLib_, s3_.bufferLib_, INLINED_FROM_MANAGED0_HAS_SLOT_PROFILE_, INLINED_FROM_MANAGED0_GET_CLASS_NODE_, s3_.readGetBufferNode_, s3_.readReleaseBufferNode_, s3_.callNode_, this.factory, INLINED_FROM_MANAGED0_INIT_FLAGS_NODE_, this.lengthNode, this.atIndexNode);
}
break;
}
}
}
{
PythonBufferAcquireLibrary bufferAcquireLib__ = null;
Node inliningTarget__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((!(PGuards.isMemoryView(arg0Value))) && (!(PGuards.isNativeObject(arg0Value))) && (!(PGuards.isMMap(arg0Value)))) {
FromManaged1Data s4_ = this.insert(new FromManaged1Data());
inliningTarget__ = (s4_);
bufferAcquireLib__ = (PYTHON_BUFFER_ACQUIRE_LIBRARY_.getUncached());
PythonBufferAccessLibrary bufferLib__ = s4_.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(bufferLib__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.bufferLib_ = bufferLib__;
ReadAttributeFromObjectNode readGetBufferNode__ = s4_.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readGetBufferNode__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'readGetBufferNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.readGetBufferNode_ = readGetBufferNode__;
ReadAttributeFromObjectNode readReleaseBufferNode__ = s4_.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readReleaseBufferNode__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'readReleaseBufferNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.readReleaseBufferNode_ = readReleaseBufferNode__;
CallNode callNode__ = s4_.insert((CallNode.create()));
Objects.requireNonNull(callNode__, "Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callNode_ = callNode__;
PythonObjectFactory factory_2;
PythonObjectFactory factory_2_shared = this.factory;
if (factory_2_shared != null) {
factory_2 = factory_2_shared;
} else {
factory_2 = s4_.insert((PythonObjectFactory.create()));
if (factory_2 == null) {
throw new IllegalStateException("Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_2;
}
CodePointLengthNode lengthNode_1;
CodePointLengthNode lengthNode_1_shared = this.lengthNode;
if (lengthNode_1_shared != null) {
lengthNode_1 = lengthNode_1_shared;
} else {
lengthNode_1 = s4_.insert((CodePointLengthNode.create()));
if (lengthNode_1 == null) {
throw new IllegalStateException("Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'lengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lengthNode == null) {
this.lengthNode = lengthNode_1;
}
CodePointAtIndexNode atIndexNode_1;
CodePointAtIndexNode atIndexNode_1_shared = this.atIndexNode;
if (atIndexNode_1_shared != null) {
atIndexNode_1 = atIndexNode_1_shared;
} else {
atIndexNode_1 = s4_.insert((CodePointAtIndexNode.create()));
if (atIndexNode_1 == null) {
throw new IllegalStateException("Specialization 'fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'atIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.atIndexNode == null) {
this.atIndexNode = atIndexNode_1;
}
VarHandle.storeStoreFence();
this.fromManaged1_cache = s4_;
this.fromManaged0_cache = null;
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyMemoryViewFromObject.fromManaged(VirtualFrame, Object, Node, PythonBufferAcquireLibrary, PythonBufferAccessLibrary, InlinedConditionProfile, GetClassNode, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, CallNode, PythonObjectFactory, InitFlagsNode, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return fromManaged(frameValue, arg0Value, inliningTarget__, bufferAcquireLib__, bufferLib__, INLINED_FROM_MANAGED1_HAS_SLOT_PROFILE_, INLINED_FROM_MANAGED1_GET_CLASS_NODE_, readGetBufferNode__, readReleaseBufferNode__, callNode__, factory_2, INLINED_FROM_MANAGED1_INIT_FLAGS_NODE_, lengthNode_1, atIndexNode_1);
}
} finally {
encapsulating_.set(prev_);
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null}, arg0Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
FromManaged0Data s3_ = this.fromManaged0_cache;
if ((s3_ == null || s3_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyMemoryViewFromObject create() {
return new PyMemoryViewFromObjectNodeGen();
}
@GeneratedBy(PyMemoryViewFromObject.class)
@DenyReplace
private static final class FromManaged0Data extends Node implements SpecializationDataNode {
@Child FromManaged0Data next_;
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link InlinedConditionProfile} hasSlotProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 2-18: InlinedCache
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fromManaged0_state_0_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link PythonBufferAcquireLibrary} bufferAcquireLib
*/
@Child PythonBufferAcquireLibrary bufferAcquireLib_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child PythonBufferAccessLibrary bufferLib_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fromManaged0_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link ReadAttributeFromObjectNode} readGetBufferNode
*/
@Child ReadAttributeFromObjectNode readGetBufferNode_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link ReadAttributeFromObjectNode} readReleaseBufferNode
*/
@Child ReadAttributeFromObjectNode readReleaseBufferNode_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link CallNode} callNode
*/
@Child CallNode callNode_;
FromManaged0Data(FromManaged0Data next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyMemoryViewFromObject.class)
@DenyReplace
private static final class FromManaged1Data extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link InlinedConditionProfile} hasSlotProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 2-18: InlinedCache
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fromManaged1_state_0_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child PythonBufferAccessLibrary bufferLib_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fromManaged1_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link ReadAttributeFromObjectNode} readGetBufferNode
*/
@Child ReadAttributeFromObjectNode readGetBufferNode_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link ReadAttributeFromObjectNode} readReleaseBufferNode
*/
@Child ReadAttributeFromObjectNode readReleaseBufferNode_;
/**
* Source Info:
* Specialization: {@link PyMemoryViewFromObject#fromManaged}
* Parameter: {@link CallNode} callNode
*/
@Child CallNode callNode_;
FromManaged1Data() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy