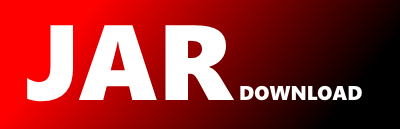
com.oracle.graal.python.lib.PyNumberIndexNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.modules.WarningsModuleBuiltins.WarnNode;
import com.oracle.graal.python.builtins.objects.ints.PInt;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyNumberIndexNode#doInt}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link PyNumberIndexNode#doLong}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link PyNumberIndexNode#doPInt}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link PyNumberIndexNode#doCallIndexInt}
* Activation probability: 0.14000
* With/without class size: 7/11 bytes
* Specialization {@link PyNumberIndexNode#doCallIndex}
* Activation probability: 0.08000
* With/without class size: 7/30 bytes
*
*/
@GeneratedBy(PyNumberIndexNode.class)
@SuppressWarnings("javadoc")
public final class PyNumberIndexNodeGen {
private static final StateField CALL_INDEX_INT__PY_NUMBER_INDEX_NODE_CALL_INDEX_INT_STATE_0_UPDATER = StateField.create(CallIndexIntData.lookup_(), "callIndexInt_state_0_");
private static final StateField CALL_INDEX__PY_NUMBER_INDEX_NODE_CALL_INDEX_STATE_0_UPDATER = StateField.create(CallIndexData.lookup_(), "callIndex_state_0_");
private static final StateField CALL_INDEX__PY_NUMBER_INDEX_NODE_CALL_INDEX_STATE_1_UPDATER = StateField.create(CallIndexData.lookup_(), "callIndex_state_1_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyNumberIndexNode getUncached() {
return PyNumberIndexNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#lookupIndex}
*
- {@link Inlined#callIndex}
*
- {@link Inlined#isSubtype}
*
- {@link Inlined#callIndexInt_cache}
*
- {@link Inlined#callIndex_cache}
*
*/
@NeverDefault
public static PyNumberIndexNode inline(@RequiredField(bits = 6, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyNumberIndexNodeGen.Inlined(target);
}
@GeneratedBy(PyNumberIndexNode.class)
@DenyReplace
private static final class Inlined extends PyNumberIndexNode {
/**
* State Info:
* 0: SpecializationActive {@link PyNumberIndexNode#doInt}
* 1: SpecializationActive {@link PyNumberIndexNode#doLong}
* 2: SpecializationActive {@link PyNumberIndexNode#doPInt}
* 3: SpecializationActive {@link PyNumberIndexNode#doCallIndexInt}
* 4: SpecializationExcluded {@link PyNumberIndexNode#doCallIndexInt}
* 5: SpecializationActive {@link PyNumberIndexNode#doCallIndex}
*
*/
private final StateField state_0_;
private final ReferenceField lookupIndex;
private final ReferenceField callIndex;
private final ReferenceField isSubtype;
private final ReferenceField callIndexInt_cache;
private final ReferenceField callIndex_cache;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndexInt}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode callIndexInt_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndexInt}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy callIndexInt_raiseNode_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode callIndex_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy callIndex_raiseNode_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link GetClassNode} resultClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode callIndex_resultClassNode_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link PyLongCheckExactNode} isInt
* Inline method: {@link PyLongCheckExactNodeGen#inline}
*/
private final PyLongCheckExactNode callIndex_isInt_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyNumberIndexNode.class);
this.state_0_ = target.getState(0, 6);
this.lookupIndex = target.getReference(1, LookupSpecialMethodSlotNode.class);
this.callIndex = target.getReference(2, CallUnaryMethodNode.class);
this.isSubtype = target.getReference(3, IsSubtypeNode.class);
this.callIndexInt_cache = target.getReference(4, CallIndexIntData.class);
this.callIndex_cache = target.getReference(5, CallIndexData.class);
this.callIndexInt_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, CALL_INDEX_INT__PY_NUMBER_INDEX_NODE_CALL_INDEX_INT_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(CallIndexIntData.lookup_(), "callIndexInt_getClassNode__field1_", Node.class)));
this.callIndexInt_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, CALL_INDEX_INT__PY_NUMBER_INDEX_NODE_CALL_INDEX_INT_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(CallIndexIntData.lookup_(), "callIndexInt_raiseNode__field1_", Node.class)));
this.callIndex_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, CALL_INDEX__PY_NUMBER_INDEX_NODE_CALL_INDEX_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(CallIndexData.lookup_(), "callIndex_getClassNode__field1_", Node.class)));
this.callIndex_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, CALL_INDEX__PY_NUMBER_INDEX_NODE_CALL_INDEX_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(CallIndexData.lookup_(), "callIndex_raiseNode__field1_", Node.class)));
this.callIndex_resultClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, CALL_INDEX__PY_NUMBER_INDEX_NODE_CALL_INDEX_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(CallIndexData.lookup_(), "callIndex_resultClassNode__field1_", Node.class)));
this.callIndex_isInt_ = PyLongCheckExactNodeGen.inline(InlineTarget.create(PyLongCheckExactNode.class, CALL_INDEX__PY_NUMBER_INDEX_NODE_CALL_INDEX_STATE_0_UPDATER.subUpdater(18, 6)));
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b101111) != 0 /* is SpecializationActive[PyNumberIndexNode.doInt(int)] || SpecializationActive[PyNumberIndexNode.doLong(long)] || SpecializationActive[PyNumberIndexNode.doPInt(PInt)] || SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] || SpecializationActive[PyNumberIndexNode.doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyNumberIndexNode.doInt(int)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
return PyNumberIndexNode.doInt(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyNumberIndexNode.doLong(long)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
return PyNumberIndexNode.doLong(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyNumberIndexNode.doPInt(PInt)] */ && arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
return PyNumberIndexNode.doPInt(arg1Value_);
}
if ((state_0 & 0b101000) != 0 /* is SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] || SpecializationActive[PyNumberIndexNode.doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] */) {
CallIndexIntData s3_ = this.callIndexInt_cache.get(arg0Value);
if (s3_ != null) {
{
LookupSpecialMethodSlotNode lookupIndex_ = this.lookupIndex.get(arg0Value);
if (lookupIndex_ != null) {
CallUnaryMethodNode callIndex_ = this.callIndex.get(arg0Value);
if (callIndex_ != null) {
IsSubtypeNode isSubtype_ = this.isSubtype.get(arg0Value);
if (isSubtype_ != null) {
try {
return PyNumberIndexNode.doCallIndexInt((VirtualFrame) frameValue, s3_, arg1Value, this.callIndexInt_getClassNode_, lookupIndex_, callIndex_, isSubtype_, this.callIndexInt_raiseNode_);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_.get(arg0Value);
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] */;
state_0 = state_0 | 0b10000 /* add SpecializationExcluded */;
this.state_0_.set(arg0Value, state_0);
this.callIndexInt_cache.set(arg0Value, null);
return ex.getResult();
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[PyNumberIndexNode.doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)] */) {
CallIndexData s4_ = this.callIndex_cache.get(arg0Value);
if (s4_ != null) {
{
LookupSpecialMethodSlotNode lookupIndex_1 = this.lookupIndex.get(arg0Value);
if (lookupIndex_1 != null) {
CallUnaryMethodNode callIndex_1 = this.callIndex.get(arg0Value);
if (callIndex_1 != null) {
IsSubtypeNode isSubtype_1 = this.isSubtype.get(arg0Value);
if (isSubtype_1 != null) {
return PyNumberIndexNode.doCallIndex((VirtualFrame) frameValue, s4_, arg1Value, this.callIndex_getClassNode_, lookupIndex_1, callIndex_1, isSubtype_1, this.callIndex_raiseNode_, this.callIndex_resultClassNode_, s4_.resultSubtype_, this.callIndex_isInt_, s4_.warnNode_, s4_.factory_);
}
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyNumberIndexNode.doInt(int)] */;
this.state_0_.set(arg0Value, state_0);
return PyNumberIndexNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyNumberIndexNode.doLong(long)] */;
this.state_0_.set(arg0Value, state_0);
return PyNumberIndexNode.doLong(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyNumberIndexNode.doPInt(PInt)] */;
this.state_0_.set(arg0Value, state_0);
return PyNumberIndexNode.doPInt(arg1Value_);
}
if (((state_0 & 0b100000)) == 0 /* is-not SpecializationActive[PyNumberIndexNode.doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)] */ && ((state_0 & 0b10000)) == 0 /* is-not SpecializationExcluded */) {
CallIndexIntData s3_ = arg0Value.insert(new CallIndexIntData());
LookupSpecialMethodSlotNode lookupIndex_;
LookupSpecialMethodSlotNode lookupIndex__shared = this.lookupIndex.get(arg0Value);
if (lookupIndex__shared != null) {
lookupIndex_ = lookupIndex__shared;
} else {
lookupIndex_ = s3_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Index)));
if (lookupIndex_ == null) {
throw new IllegalStateException("Specialization 'doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)' contains a shared cache with name 'lookupIndex' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupIndex.get(arg0Value) == null) {
this.lookupIndex.set(arg0Value, lookupIndex_);
}
CallUnaryMethodNode callIndex_;
CallUnaryMethodNode callIndex__shared = this.callIndex.get(arg0Value);
if (callIndex__shared != null) {
callIndex_ = callIndex__shared;
} else {
callIndex_ = s3_.insert((CallUnaryMethodNode.create()));
if (callIndex_ == null) {
throw new IllegalStateException("Specialization 'doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)' contains a shared cache with name 'callIndex' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callIndex.get(arg0Value) == null) {
this.callIndex.set(arg0Value, callIndex_);
}
IsSubtypeNode isSubtype_;
IsSubtypeNode isSubtype__shared = this.isSubtype.get(arg0Value);
if (isSubtype__shared != null) {
isSubtype_ = isSubtype__shared;
} else {
isSubtype_ = s3_.insert((IsSubtypeNode.create()));
if (isSubtype_ == null) {
throw new IllegalStateException("Specialization 'doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)' contains a shared cache with name 'isSubtype' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isSubtype.get(arg0Value) == null) {
this.isSubtype.set(arg0Value, isSubtype_);
}
VarHandle.storeStoreFence();
this.callIndexInt_cache.set(arg0Value, s3_);
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
try {
return PyNumberIndexNode.doCallIndexInt((VirtualFrame) frameValue, s3_, arg1Value, this.callIndexInt_getClassNode_, lookupIndex_, callIndex_, isSubtype_, this.callIndexInt_raiseNode_);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_.get(arg0Value);
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] */;
state_0 = state_0 | 0b10000 /* add SpecializationExcluded */;
this.state_0_.set(arg0Value, state_0);
this.callIndexInt_cache.set(arg0Value, null);
return ex.getResult();
}
}
CallIndexData s4_ = arg0Value.insert(new CallIndexData());
LookupSpecialMethodSlotNode lookupIndex_1;
LookupSpecialMethodSlotNode lookupIndex_1_shared = this.lookupIndex.get(arg0Value);
if (lookupIndex_1_shared != null) {
lookupIndex_1 = lookupIndex_1_shared;
} else {
lookupIndex_1 = s4_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Index)));
if (lookupIndex_1 == null) {
throw new IllegalStateException("Specialization 'doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)' contains a shared cache with name 'lookupIndex' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupIndex.get(arg0Value) == null) {
this.lookupIndex.set(arg0Value, lookupIndex_1);
}
CallUnaryMethodNode callIndex_1;
CallUnaryMethodNode callIndex_1_shared = this.callIndex.get(arg0Value);
if (callIndex_1_shared != null) {
callIndex_1 = callIndex_1_shared;
} else {
callIndex_1 = s4_.insert((CallUnaryMethodNode.create()));
if (callIndex_1 == null) {
throw new IllegalStateException("Specialization 'doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)' contains a shared cache with name 'callIndex' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callIndex.get(arg0Value) == null) {
this.callIndex.set(arg0Value, callIndex_1);
}
IsSubtypeNode isSubtype_1;
IsSubtypeNode isSubtype_1_shared = this.isSubtype.get(arg0Value);
if (isSubtype_1_shared != null) {
isSubtype_1 = isSubtype_1_shared;
} else {
isSubtype_1 = s4_.insert((IsSubtypeNode.create()));
if (isSubtype_1 == null) {
throw new IllegalStateException("Specialization 'doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)' contains a shared cache with name 'isSubtype' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isSubtype.get(arg0Value) == null) {
this.isSubtype.set(arg0Value, isSubtype_1);
}
IsSubtypeNode resultSubtype__ = s4_.insert((IsSubtypeNode.create()));
Objects.requireNonNull(resultSubtype__, "Specialization 'doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)' cache 'resultSubtype' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.resultSubtype_ = resultSubtype__;
WarnNode warnNode__ = s4_.insert((WarnNode.create()));
Objects.requireNonNull(warnNode__, "Specialization 'doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)' cache 'warnNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.warnNode_ = warnNode__;
PythonObjectFactory factory__ = s4_.insert((PythonObjectFactory.create()));
Objects.requireNonNull(factory__, "Specialization 'doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)' cache 'factory' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.factory_ = factory__;
VarHandle.storeStoreFence();
this.callIndex_cache.set(arg0Value, s4_);
this.callIndexInt_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyNumberIndexNode.doCallIndexInt(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy)] */;
state_0 = state_0 | 0b100000 /* add SpecializationActive[PyNumberIndexNode.doCallIndex(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, IsSubtypeNode, Lazy, GetClassNode, IsSubtypeNode, PyLongCheckExactNode, WarnNode, PythonObjectFactory)] */;
this.state_0_.set(arg0Value, state_0);
return PyNumberIndexNode.doCallIndex((VirtualFrame) frameValue, s4_, arg1Value, this.callIndex_getClassNode_, lookupIndex_1, callIndex_1, isSubtype_1, this.callIndex_raiseNode_, this.callIndex_resultClassNode_, resultSubtype__, this.callIndex_isInt_, warnNode__, factory__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyNumberIndexNode.class)
@DenyReplace
private static final class CallIndexIntData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyNumberIndexNode#doCallIndexInt}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link PyNumberIndexNode#doCallIndexInt}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int callIndexInt_state_0_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndexInt}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callIndexInt_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndexInt}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callIndexInt_raiseNode__field1_;
CallIndexIntData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyNumberIndexNode.class)
@DenyReplace
private static final class CallIndexData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* 18-23: InlinedCache
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link PyLongCheckExactNode} isInt
* Inline method: {@link PyLongCheckExactNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int callIndex_state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link GetClassNode} resultClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int callIndex_state_1_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callIndex_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callIndex_raiseNode__field1_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link GetClassNode} resultClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callIndex_resultClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link IsSubtypeNode} resultSubtype
*/
@Child IsSubtypeNode resultSubtype_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link WarnNode} warnNode
*/
@Child WarnNode warnNode_;
/**
* Source Info:
* Specialization: {@link PyNumberIndexNode#doCallIndex}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child PythonObjectFactory factory_;
CallIndexData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyNumberIndexNode.class)
@DenyReplace
private static final class Uncached extends PyNumberIndexNode {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
return PyNumberIndexNode.doInt(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
return PyNumberIndexNode.doLong(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
return PyNumberIndexNode.doPInt(arg1Value_);
}
return PyNumberIndexNode.doCallIndex((VirtualFrame) frameValue, arg0Value, arg1Value, (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.Index)), (CallUnaryMethodNode.getUncached()), (IsSubtypeNode.getUncached()), (Lazy.getUncached()), (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()), (PyLongCheckExactNode.getUncached()), (WarnNode.getUncached()), (PythonObjectFactory.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy