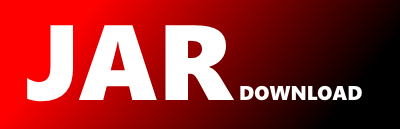
com.oracle.graal.python.lib.PyOSFSPathNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.bytes.PBytes;
import com.oracle.graal.python.builtins.objects.str.PString;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodNode.Dynamic;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodNodeGen.DynamicNodeGen;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyOSFSPathNode#doString}
* Activation probability: 0.38500
* With/without class size: 8/0 bytes
* Specialization {@link PyOSFSPathNode#doPString}
* Activation probability: 0.29500
* With/without class size: 7/0 bytes
* Specialization {@link PyOSFSPathNode#doBytes}
* Activation probability: 0.20500
* With/without class size: 6/0 bytes
* Specialization {@link PyOSFSPathNode#callFspath}
* Activation probability: 0.11500
* With/without class size: 8/24 bytes
*
*/
@GeneratedBy(PyOSFSPathNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyOSFSPathNodeGen {
private static final StateField FALLBACK__PY_O_S_F_S_PATH_NODE_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyOSFSPathNode getUncached() {
return PyOSFSPathNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#fallback_cache}
*
*/
@NeverDefault
public static PyOSFSPathNode inline(@RequiredField(bits = 4, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyOSFSPathNodeGen.Inlined(target);
}
@GeneratedBy(PyOSFSPathNode.class)
@DenyReplace
private static final class Inlined extends PyOSFSPathNode {
/**
* State Info:
* 0: SpecializationActive {@link PyOSFSPathNode#doString}
* 1: SpecializationActive {@link PyOSFSPathNode#doPString}
* 2: SpecializationActive {@link PyOSFSPathNode#doBytes}
* 3: SpecializationActive {@link PyOSFSPathNode#callFspath}
*
*/
private final StateField state_0_;
private final ReferenceField fallback_cache;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode fallback_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Dynamic} lookupFSPath
* Inline method: {@link DynamicNodeGen#inline}
*/
private final Dynamic fallback_lookupFSPath_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy fallback_raiseNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyOSFSPathNode.class);
this.state_0_ = target.getState(0, 4);
this.fallback_cache = target.getReference(1, FallbackData.class);
this.fallback_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, FALLBACK__PY_O_S_F_S_PATH_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(FallbackData.lookup_(), "fallback_getClassNode__field1_", Node.class)));
this.fallback_lookupFSPath_ = DynamicNodeGen.inline(InlineTarget.create(Dynamic.class, FALLBACK__PY_O_S_F_S_PATH_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(17, 7), ReferenceField.create(FallbackData.lookup_(), "fallback_lookupFSPath__field1_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_lookupFSPath__field2_", Node.class)));
this.fallback_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, FALLBACK__PY_O_S_F_S_PATH_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(24, 1), ReferenceField.create(FallbackData.lookup_(), "fallback_raiseNode__field1_", Node.class)));
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[PyOSFSPathNode.doString(TruffleString)] */) && arg1Value instanceof TruffleString) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[PyOSFSPathNode.doPString(PString)] */) && arg1Value instanceof PString) {
return false;
}
if (!((state_0 & 0b100) != 0 /* is SpecializationActive[PyOSFSPathNode.doBytes(PBytes)] */) && arg1Value instanceof PBytes) {
return false;
}
return true;
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyOSFSPathNode.doString(TruffleString)] || SpecializationActive[PyOSFSPathNode.doPString(PString)] || SpecializationActive[PyOSFSPathNode.doBytes(PBytes)] || SpecializationActive[PyOSFSPathNode.callFspath(VirtualFrame, Node, Object, GetClassNode, Dynamic, CallUnaryMethodNode, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyOSFSPathNode.doString(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return PyOSFSPathNode.doString(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyOSFSPathNode.doPString(PString)] */ && arg1Value instanceof PString) {
PString arg1Value_ = (PString) arg1Value;
return PyOSFSPathNode.doPString(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyOSFSPathNode.doBytes(PBytes)] */ && arg1Value instanceof PBytes) {
PBytes arg1Value_ = (PBytes) arg1Value;
return PyOSFSPathNode.doBytes(arg1Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyOSFSPathNode.callFspath(VirtualFrame, Node, Object, GetClassNode, Dynamic, CallUnaryMethodNode, Lazy)] */) {
FallbackData s3_ = this.fallback_cache.get(arg0Value);
if (s3_ != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return PyOSFSPathNode.callFspath((VirtualFrame) frameValue, s3_, arg1Value, this.fallback_getClassNode_, this.fallback_lookupFSPath_, s3_.callFSPath_, this.fallback_raiseNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyOSFSPathNode.doString(TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return PyOSFSPathNode.doString(arg1Value_);
}
if (arg1Value instanceof PString) {
PString arg1Value_ = (PString) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyOSFSPathNode.doPString(PString)] */;
this.state_0_.set(arg0Value, state_0);
return PyOSFSPathNode.doPString(arg1Value_);
}
if (arg1Value instanceof PBytes) {
PBytes arg1Value_ = (PBytes) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyOSFSPathNode.doBytes(PBytes)] */;
this.state_0_.set(arg0Value, state_0);
return PyOSFSPathNode.doBytes(arg1Value_);
}
FallbackData s3_ = arg0Value.insert(new FallbackData());
CallUnaryMethodNode callFSPath__ = s3_.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callFSPath__, "Specialization 'callFspath(VirtualFrame, Node, Object, GetClassNode, Dynamic, CallUnaryMethodNode, Lazy)' cache 'callFSPath' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.callFSPath_ = callFSPath__;
VarHandle.storeStoreFence();
this.fallback_cache.set(arg0Value, s3_);
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyOSFSPathNode.callFspath(VirtualFrame, Node, Object, GetClassNode, Dynamic, CallUnaryMethodNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
return PyOSFSPathNode.callFspath((VirtualFrame) frameValue, s3_, arg1Value, this.fallback_getClassNode_, this.fallback_lookupFSPath_, callFSPath__, this.fallback_raiseNode_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyOSFSPathNode.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17-23: InlinedCache
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Dynamic} lookupFSPath
* Inline method: {@link DynamicNodeGen#inline}
* 24: InlinedCache
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Dynamic} lookupFSPath
* Inline method: {@link DynamicNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_lookupFSPath__field1_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Dynamic} lookupFSPath
* Inline method: {@link DynamicNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_lookupFSPath__field2_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link CallUnaryMethodNode} callFSPath
*/
@Child CallUnaryMethodNode callFSPath_;
/**
* Source Info:
* Specialization: {@link PyOSFSPathNode#callFspath}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_raiseNode__field1_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyOSFSPathNode.class)
@DenyReplace
private static final class Uncached extends PyOSFSPathNode {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return PyOSFSPathNode.doString(arg1Value_);
}
if (arg1Value instanceof PString) {
PString arg1Value_ = (PString) arg1Value;
return PyOSFSPathNode.doPString(arg1Value_);
}
if (arg1Value instanceof PBytes) {
PBytes arg1Value_ = (PBytes) arg1Value;
return PyOSFSPathNode.doBytes(arg1Value_);
}
return PyOSFSPathNode.callFspath((VirtualFrame) frameValue, arg0Value, arg1Value, (GetClassNode.getUncached()), (DynamicNodeGen.getUncached()), (CallUnaryMethodNode.getUncached()), (Lazy.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy