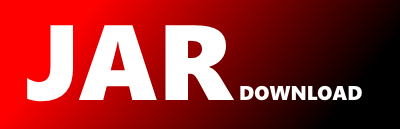
com.oracle.graal.python.lib.PyObjectAsFileDescriptorNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.CallNode;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectAsFileDescriptor#doInt}
* Activation probability: 0.48333
* With/without class size: 15/5 bytes
* Specialization {@link PyObjectAsFileDescriptor#doPyLong}
* Activation probability: 0.33333
* With/without class size: 16/18 bytes
* Specialization {@link PyObjectAsFileDescriptor#doNotLong}
* Activation probability: 0.18333
* With/without class size: 12/26 bytes
*
*/
@GeneratedBy(PyObjectAsFileDescriptor.class)
@SuppressWarnings("javadoc")
public final class PyObjectAsFileDescriptorNodeGen {
private static final StateField PY_LONG__PY_OBJECT_AS_FILE_DESCRIPTOR_PY_LONG_STATE_0_UPDATER = StateField.create(PyLongData.lookup_(), "pyLong_state_0_");
private static final StateField FALLBACK__PY_OBJECT_AS_FILE_DESCRIPTOR_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyObjectAsFileDescriptor getUncached() {
return PyObjectAsFileDescriptorNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#int_raise__field1_}
*
- {@link Inlined#pyLong_cache}
*
- {@link Inlined#fallback_cache}
*
*/
@NeverDefault
public static PyObjectAsFileDescriptor inline(@RequiredField(bits = 4, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectAsFileDescriptorNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectAsFileDescriptor.class)
@DenyReplace
private static final class Inlined extends PyObjectAsFileDescriptor {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectAsFileDescriptor#doInt}
* 1: SpecializationActive {@link PyObjectAsFileDescriptor#doPyLong}
* 2: SpecializationActive {@link PyObjectAsFileDescriptor#doNotLong}
* 3: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doInt}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField int_raise__field1_;
private final ReferenceField pyLong_cache;
private final ReferenceField fallback_cache;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doInt}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy int_raise_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongCheckNode} longCheckNode
* Inline method: {@link PyLongCheckNodeGen#inline}
*/
private final PyLongCheckNode pyLong_longCheckNode_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
*/
private final PyLongAsIntNode pyLong_asIntNode_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy pyLong_raise_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongCheckNode} checkResultNode
* Inline method: {@link PyLongCheckNodeGen#inline}
*/
private final PyLongCheckNode fallback_checkResultNode_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
*/
private final PyLongAsIntNode fallback_asIntNode_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy fallback_raise_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectAsFileDescriptor.class);
this.state_0_ = target.getState(0, 4);
this.int_raise__field1_ = target.getReference(1, Node.class);
this.pyLong_cache = target.getReference(2, PyLongData.class);
this.fallback_cache = target.getReference(3, FallbackData.class);
this.int_raise_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, state_0_.subUpdater(3, 1), int_raise__field1_));
this.pyLong_longCheckNode_ = PyLongCheckNodeGen.inline(InlineTarget.create(PyLongCheckNode.class, PY_LONG__PY_OBJECT_AS_FILE_DESCRIPTOR_PY_LONG_STATE_0_UPDATER.subUpdater(2, 5), ReferenceField.create(PyLongData.lookup_(), "pyLong_longCheckNode__field1_", Node.class)));
this.pyLong_asIntNode_ = PyLongAsIntNodeGen.inline(InlineTarget.create(PyLongAsIntNode.class, PY_LONG__PY_OBJECT_AS_FILE_DESCRIPTOR_PY_LONG_STATE_0_UPDATER.subUpdater(7, 9), ReferenceField.create(PyLongData.lookup_(), "pyLong_asIntNode__field1_", Node.class), ReferenceField.create(PyLongData.lookup_(), "pyLong_asIntNode__field2_", Node.class)));
this.pyLong_raise_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, PY_LONG__PY_OBJECT_AS_FILE_DESCRIPTOR_PY_LONG_STATE_0_UPDATER.subUpdater(16, 1), ReferenceField.create(PyLongData.lookup_(), "pyLong_raise__field1_", Node.class)));
this.fallback_checkResultNode_ = PyLongCheckNodeGen.inline(InlineTarget.create(PyLongCheckNode.class, FALLBACK__PY_OBJECT_AS_FILE_DESCRIPTOR_FALLBACK_STATE_0_UPDATER.subUpdater(0, 5), ReferenceField.create(FallbackData.lookup_(), "fallback_checkResultNode__field1_", Node.class)));
this.fallback_asIntNode_ = PyLongAsIntNodeGen.inline(InlineTarget.create(PyLongAsIntNode.class, FALLBACK__PY_OBJECT_AS_FILE_DESCRIPTOR_FALLBACK_STATE_0_UPDATER.subUpdater(5, 9), ReferenceField.create(FallbackData.lookup_(), "fallback_asIntNode__field1_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_asIntNode__field2_", Node.class)));
this.fallback_raise_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, FALLBACK__PY_OBJECT_AS_FILE_DESCRIPTOR_FALLBACK_STATE_0_UPDATER.subUpdater(14, 1), ReferenceField.create(FallbackData.lookup_(), "fallback_raise__field1_", Node.class)));
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectAsFileDescriptor.doInt(Node, int, Lazy)] */) && arg1Value instanceof Integer) {
return false;
}
{
PyLongData s1_ = this.pyLong_cache.get(arg0Value);
if ((s1_ == null || ((s1_.pyLong_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy), guardIndex=0] */ || (this.pyLong_longCheckNode_.execute(s1_, arg1Value)))) {
return false;
}
}
return true;
}
@Override
public int execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b111) != 0 /* is SpecializationActive[PyObjectAsFileDescriptor.doInt(Node, int, Lazy)] || SpecializationActive[PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy)] || SpecializationActive[PyObjectAsFileDescriptor.doNotLong(VirtualFrame, Node, Object, PyObjectLookupAttr, CallNode, PyLongCheckNode, PyLongAsIntNode, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectAsFileDescriptor.doInt(Node, int, Lazy)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
assert InlineSupport.validate(arg0Value, this.state_0_, this.int_raise__field1_);
return PyObjectAsFileDescriptor.doInt(arg0Value, arg1Value_, this.int_raise_);
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy)] || SpecializationActive[PyObjectAsFileDescriptor.doNotLong(VirtualFrame, Node, Object, PyObjectLookupAttr, CallNode, PyLongCheckNode, PyLongAsIntNode, Lazy)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy)] */) {
PyLongData s1_ = this.pyLong_cache.get(arg0Value);
if (s1_ != null) {
if ((s1_.pyLong_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */ && (this.pyLong_longCheckNode_.execute(s1_, arg1Value))) {
return PyObjectAsFileDescriptor.doPyLong((VirtualFrame) frameValue, s1_, arg1Value, this.pyLong_longCheckNode_, this.pyLong_asIntNode_, this.pyLong_raise_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectAsFileDescriptor.doNotLong(VirtualFrame, Node, Object, PyObjectLookupAttr, CallNode, PyLongCheckNode, PyLongAsIntNode, Lazy)] */) {
FallbackData s2_ = this.fallback_cache.get(arg0Value);
if (s2_ != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return PyObjectAsFileDescriptor.doNotLong((VirtualFrame) frameValue, s2_, arg1Value, s2_.lookupFileno_, s2_.callFileno_, this.fallback_checkResultNode_, this.fallback_asIntNode_, this.fallback_raise_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private int executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectAsFileDescriptor.doInt(Node, int, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.int_raise__field1_);
return PyObjectAsFileDescriptor.doInt(arg0Value, arg1Value_, this.int_raise_);
}
while (true) {
int count1_ = 0;
PyLongData s1_ = this.pyLong_cache.getVolatile(arg0Value);
PyLongData s1_original = s1_;
while (s1_ != null) {
if ((s1_.pyLong_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */ && (this.pyLong_longCheckNode_.execute(s1_, arg1Value))) {
break;
}
if ((s1_.pyLong_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count1_++;
}
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
{
s1_ = arg0Value.insert(new PyLongData());
if (((s1_.pyLong_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy), guardIndex=0] */) {
s1_.pyLong_state_0_ = s1_.pyLong_state_0_ | 0b1 /* add GuardActive[specialization=PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy), guardIndex=0] */;
if (!this.pyLong_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
s1_original = s1_;
s1_ = arg0Value.insert(new PyLongData(s1_));
}
if ((this.pyLong_longCheckNode_.execute(s1_, arg1Value))) {
s1_.pyLong_state_0_ = s1_.pyLong_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!this.pyLong_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectAsFileDescriptor.doPyLong(VirtualFrame, Node, Object, PyLongCheckNode, PyLongAsIntNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s1_ = null;
}
}
}
if (s1_ != null) {
return PyObjectAsFileDescriptor.doPyLong((VirtualFrame) frameValue, s1_, arg1Value, this.pyLong_longCheckNode_, this.pyLong_asIntNode_, this.pyLong_raise_);
}
break;
}
FallbackData s2_ = arg0Value.insert(new FallbackData());
PyObjectLookupAttr lookupFileno__ = s2_.insert((PyObjectLookupAttr.create()));
Objects.requireNonNull(lookupFileno__, "Specialization 'doNotLong(VirtualFrame, Node, Object, PyObjectLookupAttr, CallNode, PyLongCheckNode, PyLongAsIntNode, Lazy)' cache 'lookupFileno' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.lookupFileno_ = lookupFileno__;
CallNode callFileno__ = s2_.insert((CallNode.create()));
Objects.requireNonNull(callFileno__, "Specialization 'doNotLong(VirtualFrame, Node, Object, PyObjectLookupAttr, CallNode, PyLongCheckNode, PyLongAsIntNode, Lazy)' cache 'callFileno' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.callFileno_ = callFileno__;
VarHandle.storeStoreFence();
this.fallback_cache.set(arg0Value, s2_);
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectAsFileDescriptor.doNotLong(VirtualFrame, Node, Object, PyObjectLookupAttr, CallNode, PyLongCheckNode, PyLongAsIntNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectAsFileDescriptor.doNotLong((VirtualFrame) frameValue, s2_, arg1Value, lookupFileno__, callFileno__, this.fallback_checkResultNode_, this.fallback_asIntNode_, this.fallback_raise_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectAsFileDescriptor.class)
@DenyReplace
private static final class PyLongData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: GuardActive[guardIndex=0] {@link PyObjectAsFileDescriptor#doPyLong}
* 1: SpecializationCachesInitialized {@link PyObjectAsFileDescriptor#doPyLong}
* 2-6: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongCheckNode} longCheckNode
* Inline method: {@link PyLongCheckNodeGen#inline}
* 7-15: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
* 16: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int pyLong_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongCheckNode} longCheckNode
* Inline method: {@link PyLongCheckNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pyLong_longCheckNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pyLong_asIntNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pyLong_asIntNode__field2_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doPyLong}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pyLong_raise__field1_;
PyLongData() {
}
PyLongData(PyLongData delegate) {
this.pyLong_state_0_ = delegate.pyLong_state_0_;
this.pyLong_longCheckNode__field1_ = delegate.pyLong_longCheckNode__field1_;
this.pyLong_asIntNode__field1_ = delegate.pyLong_asIntNode__field1_;
this.pyLong_asIntNode__field2_ = delegate.pyLong_asIntNode__field2_;
this.pyLong_raise__field1_ = delegate.pyLong_raise__field1_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectAsFileDescriptor.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-4: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongCheckNode} checkResultNode
* Inline method: {@link PyLongCheckNodeGen#inline}
* 5-13: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
* 14: InlinedCache
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyObjectLookupAttr} lookupFileno
*/
@Child PyObjectLookupAttr lookupFileno_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link CallNode} callFileno
*/
@Child CallNode callFileno_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongCheckNode} checkResultNode
* Inline method: {@link PyLongCheckNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_checkResultNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_asIntNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link PyLongAsIntNode} asIntNode
* Inline method: {@link PyLongAsIntNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_asIntNode__field2_;
/**
* Source Info:
* Specialization: {@link PyObjectAsFileDescriptor#doNotLong}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_raise__field1_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectAsFileDescriptor.class)
@DenyReplace
private static final class Uncached extends PyObjectAsFileDescriptor {
@Override
public int execute(Frame frameValue, Node arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
return PyObjectAsFileDescriptor.doInt(arg0Value, arg1Value_, (Lazy.getUncached()));
}
if (((PyLongCheckNode.getUncached()).execute(arg0Value, arg1Value))) {
return PyObjectAsFileDescriptor.doPyLong((VirtualFrame) frameValue, arg0Value, arg1Value, (PyLongCheckNode.getUncached()), (PyLongAsIntNodeGen.getUncached()), (Lazy.getUncached()));
}
return PyObjectAsFileDescriptor.doNotLong((VirtualFrame) frameValue, arg0Value, arg1Value, (PyObjectLookupAttr.getUncached()), (CallNode.getUncached()), (PyLongCheckNode.getUncached()), (PyLongAsIntNodeGen.getUncached()), (Lazy.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy