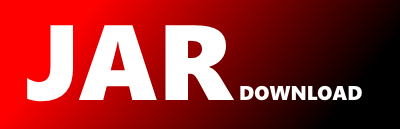
com.oracle.graal.python.lib.PyObjectCallMethodObjArgsNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.nodes.call.CallNode;
import com.oracle.graal.python.nodes.call.special.CallBinaryMethodNode;
import com.oracle.graal.python.nodes.call.special.CallQuaternaryMethodNode;
import com.oracle.graal.python.nodes.call.special.CallTernaryMethodNode;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectCallMethodObjArgs#callUnary}
* Activation probability: 0.32000
* With/without class size: 10/4 bytes
* Specialization {@link PyObjectCallMethodObjArgs#callBinary}
* Activation probability: 0.26000
* With/without class size: 9/4 bytes
* Specialization {@link PyObjectCallMethodObjArgs#callTernary}
* Activation probability: 0.20000
* With/without class size: 8/4 bytes
* Specialization {@link PyObjectCallMethodObjArgs#callQuad}
* Activation probability: 0.14000
* With/without class size: 6/4 bytes
* Specialization {@link PyObjectCallMethodObjArgs#call}
* Activation probability: 0.08000
* With/without class size: 8/41 bytes
*
*/
@GeneratedBy(PyObjectCallMethodObjArgs.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyObjectCallMethodObjArgsNodeGen extends PyObjectCallMethodObjArgs {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField CALL__PY_OBJECT_CALL_METHOD_OBJ_ARGS_CALL_STATE_0_UPDATER = StateField.create(CallData.lookup_(), "call_state_0_");
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
*/
private static final PyObjectGetMethod INLINED_GET_METHOD = PyObjectGetMethodNodeGen.inline(InlineTarget.create(PyObjectGetMethod.class, STATE_0_UPDATER.subUpdater(5, 5), ReferenceField.create(MethodHandles.lookup(), "getMethod_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field3_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field4_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field5_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field6_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field7_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field8_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getMethod_field9_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
*/
private static final PyObjectGetMethod INLINED_CALL_GET_METHOD_ = PyObjectGetMethodNodeGen.inline(InlineTarget.create(PyObjectGetMethod.class, CALL__PY_OBJECT_CALL_METHOD_OBJ_ARGS_CALL_STATE_0_UPDATER.subUpdater(0, 5), ReferenceField.create(CallData.lookup_(), "call_getMethod__field1_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field2_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field3_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field4_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field5_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field6_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field7_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field8_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field9_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link InlinedConditionProfile} isBoundProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_CALL_IS_BOUND_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, CALL__PY_OBJECT_CALL_METHOD_OBJ_ARGS_CALL_STATE_0_UPDATER.subUpdater(5, 2)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectCallMethodObjArgs#callUnary}
* 1: SpecializationActive {@link PyObjectCallMethodObjArgs#call}
* 2: SpecializationActive {@link PyObjectCallMethodObjArgs#callBinary}
* 3: SpecializationActive {@link PyObjectCallMethodObjArgs#callTernary}
* 4: SpecializationActive {@link PyObjectCallMethodObjArgs#callQuad}
* 5-9: InlinedCache
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field1_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field2_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field3_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field4_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field5_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field6
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field6_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field7
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field7_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field8
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field8_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field9
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getMethod_field9_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link CallUnaryMethodNode} callNode
*/
@Child private CallUnaryMethodNode callUnary_callNode_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callBinary}
* Parameter: {@link CallBinaryMethodNode} callNode
*/
@Child private CallBinaryMethodNode callBinary_callNode_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callTernary}
* Parameter: {@link CallTernaryMethodNode} callNode
*/
@Child private CallTernaryMethodNode callTernary_callNode_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callQuad}
* Parameter: {@link CallQuaternaryMethodNode} callNode
*/
@Child private CallQuaternaryMethodNode callQuad_callNode_;
@Child private CallData call_cache;
private PyObjectCallMethodObjArgsNodeGen() {
}
@Override
protected Object executeInternal(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)] */) {
{
CallUnaryMethodNode callNode__ = this.callUnary_callNode_;
if (callNode__ != null) {
if ((arg3Value.length == 0)) {
return PyObjectCallMethodObjArgs.callUnary(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)] */) {
{
CallBinaryMethodNode callNode__1 = this.callBinary_callNode_;
if (callNode__1 != null) {
if ((arg3Value.length == 1)) {
return PyObjectCallMethodObjArgs.callBinary(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__1);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)] */) {
{
CallTernaryMethodNode callNode__2 = this.callTernary_callNode_;
if (callNode__2 != null) {
if ((arg3Value.length == 2)) {
return PyObjectCallMethodObjArgs.callTernary(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__2);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] */) {
{
CallQuaternaryMethodNode callNode__3 = this.callQuad_callNode_;
if (callNode__3 != null) {
if ((arg3Value.length == 3)) {
return PyObjectCallMethodObjArgs.callQuad(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__3);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
CallData s4_ = this.call_cache;
if (s4_ != null) {
return PyObjectCallMethodObjArgs.call(frameValue, s4_, arg1Value, arg2Value, arg3Value, INLINED_CALL_GET_METHOD_, s4_.callNode_, INLINED_CALL_IS_BOUND_PROFILE_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 0)) {
CallUnaryMethodNode callNode__ = this.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callNode__, "Specialization 'callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callUnary_callNode_ = callNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)] */;
this.state_0_ = state_0;
return PyObjectCallMethodObjArgs.callUnary(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 1)) {
CallBinaryMethodNode callNode__1 = this.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callNode__1, "Specialization 'callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callBinary_callNode_ = callNode__1;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)] */;
this.state_0_ = state_0;
return PyObjectCallMethodObjArgs.callBinary(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__1);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 2)) {
CallTernaryMethodNode callNode__2 = this.insert((CallTernaryMethodNode.create()));
Objects.requireNonNull(callNode__2, "Specialization 'callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callTernary_callNode_ = callNode__2;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)] */;
this.state_0_ = state_0;
return PyObjectCallMethodObjArgs.callTernary(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__2);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 3)) {
CallQuaternaryMethodNode callNode__3 = this.insert((CallQuaternaryMethodNode.create()));
Objects.requireNonNull(callNode__3, "Specialization 'callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callQuad_callNode_ = callNode__3;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] */;
this.state_0_ = state_0;
return PyObjectCallMethodObjArgs.callQuad(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_METHOD, callNode__3);
}
}
CallData s4_ = this.insert(new CallData());
CallNode callNode__4 = s4_.insert((CallNode.create()));
Objects.requireNonNull(callNode__4, "Specialization 'call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callNode_ = callNode__4;
VarHandle.storeStoreFence();
this.call_cache = s4_;
this.callUnary_callNode_ = null;
this.callBinary_callNode_ = null;
this.callTernary_callNode_ = null;
this.callQuad_callNode_ = null;
state_0 = state_0 & 0xffffffe2 /* remove SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)], SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)], SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)], SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return PyObjectCallMethodObjArgs.call(frameValue, s4_, arg1Value, arg2Value, arg3Value, INLINED_CALL_GET_METHOD_, callNode__4, INLINED_CALL_IS_BOUND_PROFILE_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11111) & ((state_0 & 0b11111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyObjectCallMethodObjArgs create() {
return new PyObjectCallMethodObjArgsNodeGen();
}
@NeverDefault
public static PyObjectCallMethodObjArgs getUncached() {
return PyObjectCallMethodObjArgsNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#getMethod_field1_}
*
- {@link Inlined#getMethod_field2_}
*
- {@link Inlined#getMethod_field3_}
*
- {@link Inlined#getMethod_field4_}
*
- {@link Inlined#getMethod_field5_}
*
- {@link Inlined#getMethod_field6_}
*
- {@link Inlined#getMethod_field7_}
*
- {@link Inlined#getMethod_field8_}
*
- {@link Inlined#getMethod_field9_}
*
- {@link Inlined#callUnary_callNode_}
*
- {@link Inlined#callBinary_callNode_}
*
- {@link Inlined#callTernary_callNode_}
*
- {@link Inlined#callQuad_callNode_}
*
- {@link Inlined#call_cache}
*
*/
@NeverDefault
public static PyObjectCallMethodObjArgs inline(@RequiredField(bits = 10, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectCallMethodObjArgsNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectCallMethodObjArgs.class)
@DenyReplace
private static final class Inlined extends PyObjectCallMethodObjArgs {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectCallMethodObjArgs#callUnary}
* 1: SpecializationActive {@link PyObjectCallMethodObjArgs#call}
* 2: SpecializationActive {@link PyObjectCallMethodObjArgs#callBinary}
* 3: SpecializationActive {@link PyObjectCallMethodObjArgs#callTernary}
* 4: SpecializationActive {@link PyObjectCallMethodObjArgs#callQuad}
* 5-9: InlinedCache
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField getMethod_field1_;
private final ReferenceField getMethod_field2_;
private final ReferenceField getMethod_field3_;
private final ReferenceField getMethod_field4_;
private final ReferenceField getMethod_field5_;
private final ReferenceField getMethod_field6_;
private final ReferenceField getMethod_field7_;
private final ReferenceField getMethod_field8_;
private final ReferenceField getMethod_field9_;
private final ReferenceField callUnary_callNode_;
private final ReferenceField callBinary_callNode_;
private final ReferenceField callTernary_callNode_;
private final ReferenceField callQuad_callNode_;
private final ReferenceField call_cache;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#callUnary}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
*/
private final PyObjectGetMethod getMethod;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
*/
private final PyObjectGetMethod call_getMethod_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link InlinedConditionProfile} isBoundProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile call_isBoundProfile_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectCallMethodObjArgs.class);
this.state_0_ = target.getState(0, 10);
this.getMethod_field1_ = target.getReference(1, Node.class);
this.getMethod_field2_ = target.getReference(2, Node.class);
this.getMethod_field3_ = target.getReference(3, Node.class);
this.getMethod_field4_ = target.getReference(4, Node.class);
this.getMethod_field5_ = target.getReference(5, Node.class);
this.getMethod_field6_ = target.getReference(6, Node.class);
this.getMethod_field7_ = target.getReference(7, Node.class);
this.getMethod_field8_ = target.getReference(8, Node.class);
this.getMethod_field9_ = target.getReference(9, Node.class);
this.callUnary_callNode_ = target.getReference(10, CallUnaryMethodNode.class);
this.callBinary_callNode_ = target.getReference(11, CallBinaryMethodNode.class);
this.callTernary_callNode_ = target.getReference(12, CallTernaryMethodNode.class);
this.callQuad_callNode_ = target.getReference(13, CallQuaternaryMethodNode.class);
this.call_cache = target.getReference(14, CallData.class);
this.getMethod = PyObjectGetMethodNodeGen.inline(InlineTarget.create(PyObjectGetMethod.class, state_0_.subUpdater(5, 5), getMethod_field1_, getMethod_field2_, getMethod_field3_, getMethod_field4_, getMethod_field5_, getMethod_field6_, getMethod_field7_, getMethod_field8_, getMethod_field9_));
this.call_getMethod_ = PyObjectGetMethodNodeGen.inline(InlineTarget.create(PyObjectGetMethod.class, CALL__PY_OBJECT_CALL_METHOD_OBJ_ARGS_CALL_STATE_0_UPDATER.subUpdater(0, 5), ReferenceField.create(CallData.lookup_(), "call_getMethod__field1_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field2_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field3_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field4_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field5_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field6_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field7_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field8_", Node.class), ReferenceField.create(CallData.lookup_(), "call_getMethod__field9_", Node.class)));
this.call_isBoundProfile_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, CALL__PY_OBJECT_CALL_METHOD_OBJ_ARGS_CALL_STATE_0_UPDATER.subUpdater(5, 2)));
}
@Override
protected Object executeInternal(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] || SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)] */) {
{
CallUnaryMethodNode callNode__ = this.callUnary_callNode_.get(arg0Value);
if (callNode__ != null) {
if ((arg3Value.length == 0)) {
return PyObjectCallMethodObjArgs.callUnary(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)] */) {
{
CallBinaryMethodNode callNode__1 = this.callBinary_callNode_.get(arg0Value);
if (callNode__1 != null) {
if ((arg3Value.length == 1)) {
return PyObjectCallMethodObjArgs.callBinary(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__1);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)] */) {
{
CallTernaryMethodNode callNode__2 = this.callTernary_callNode_.get(arg0Value);
if (callNode__2 != null) {
if ((arg3Value.length == 2)) {
return PyObjectCallMethodObjArgs.callTernary(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__2);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] */) {
{
CallQuaternaryMethodNode callNode__3 = this.callQuad_callNode_.get(arg0Value);
if (callNode__3 != null) {
if ((arg3Value.length == 3)) {
return PyObjectCallMethodObjArgs.callQuad(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__3);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
CallData s4_ = this.call_cache.get(arg0Value);
if (s4_ != null) {
return PyObjectCallMethodObjArgs.call(frameValue, s4_, arg1Value, arg2Value, arg3Value, this.call_getMethod_, s4_.callNode_, this.call_isBoundProfile_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 0)) {
CallUnaryMethodNode callNode__ = arg0Value.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callNode__, "Specialization 'callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callUnary_callNode_.set(arg0Value, callNode__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectCallMethodObjArgs.callUnary(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 1)) {
CallBinaryMethodNode callNode__1 = arg0Value.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callNode__1, "Specialization 'callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callBinary_callNode_.set(arg0Value, callNode__1);
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectCallMethodObjArgs.callBinary(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__1);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 2)) {
CallTernaryMethodNode callNode__2 = arg0Value.insert((CallTernaryMethodNode.create()));
Objects.requireNonNull(callNode__2, "Specialization 'callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callTernary_callNode_.set(arg0Value, callNode__2);
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectCallMethodObjArgs.callTernary(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__2);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */) {
if ((arg3Value.length == 3)) {
CallQuaternaryMethodNode callNode__3 = arg0Value.insert((CallQuaternaryMethodNode.create()));
Objects.requireNonNull(callNode__3, "Specialization 'callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callQuad_callNode_.set(arg0Value, callNode__3);
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectCallMethodObjArgs.callQuad(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getMethod, callNode__3);
}
}
CallData s4_ = arg0Value.insert(new CallData());
CallNode callNode__4 = s4_.insert((CallNode.create()));
Objects.requireNonNull(callNode__4, "Specialization 'call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callNode_ = callNode__4;
VarHandle.storeStoreFence();
this.call_cache.set(arg0Value, s4_);
this.callUnary_callNode_.set(arg0Value, null);
this.callBinary_callNode_.set(arg0Value, null);
this.callTernary_callNode_.set(arg0Value, null);
this.callQuad_callNode_.set(arg0Value, null);
state_0 = state_0 & 0xffffffe2 /* remove SpecializationActive[PyObjectCallMethodObjArgs.callUnary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallUnaryMethodNode)], SpecializationActive[PyObjectCallMethodObjArgs.callBinary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallBinaryMethodNode)], SpecializationActive[PyObjectCallMethodObjArgs.callTernary(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallTernaryMethodNode)], SpecializationActive[PyObjectCallMethodObjArgs.callQuad(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallQuaternaryMethodNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectCallMethodObjArgs.call(Frame, Node, Object, TruffleString, Object[], PyObjectGetMethod, CallNode, InlinedConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectCallMethodObjArgs.call(frameValue, s4_, arg1Value, arg2Value, arg3Value, this.call_getMethod_, callNode__4, this.call_isBoundProfile_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectCallMethodObjArgs.class)
@DenyReplace
private static final class CallData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-4: InlinedCache
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* 5-6: InlinedCache
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link InlinedConditionProfile} isBoundProfile
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int call_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field2_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field3_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field4_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field5_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field6
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field6_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field7
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field7_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field8
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field8_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link PyObjectGetMethod} getMethod
* Inline method: {@link PyObjectGetMethodNodeGen#inline}
* Inline field: {@link Node} field9
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node call_getMethod__field9_;
/**
* Source Info:
* Specialization: {@link PyObjectCallMethodObjArgs#call}
* Parameter: {@link CallNode} callNode
*/
@Child CallNode callNode_;
CallData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectCallMethodObjArgs.class)
@DenyReplace
private static final class Uncached extends PyObjectCallMethodObjArgs {
@Override
protected Object executeInternal(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return PyObjectCallMethodObjArgs.call(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, (PyObjectGetMethodNodeGen.getUncached()), (CallNode.getUncached()), (InlinedConditionProfile.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy