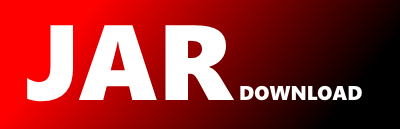
com.oracle.graal.python.lib.PyObjectGetItemNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.dict.PDict;
import com.oracle.graal.python.builtins.objects.list.PList;
import com.oracle.graal.python.builtins.objects.tuple.PTuple;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.IsTypeNode;
import com.oracle.graal.python.builtins.objects.type.TypeNodesFactory.IsTypeNodeGen;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.CallNode;
import com.oracle.graal.python.nodes.call.special.CallBinaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.nodes.object.BuiltinClassProfiles.InlineIsBuiltinClassProfile;
import com.oracle.graal.python.nodes.object.BuiltinClassProfilesFactory.InlineIsBuiltinClassProfileNodeGen;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectGetItem#doList}
* Activation probability: 0.38500
* With/without class size: 11/4 bytes
* Specialization {@link PyObjectGetItem#doTuple}
* Activation probability: 0.29500
* With/without class size: 9/4 bytes
* Specialization {@link PyObjectGetItem#doDict}
* Activation probability: 0.20500
* With/without class size: 8/4 bytes
* Specialization {@link PyObjectGetItem#doGeneric}
* Activation probability: 0.11500
* With/without class size: 8/23 bytes
*
*/
@GeneratedBy(PyObjectGetItem.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyObjectGetItemNodeGen extends PyObjectGetItem {
private static final StateField GENERIC__PY_OBJECT_GET_ITEM_GENERIC_STATE_0_UPDATER = StateField.create(GenericData.lookup_(), "generic_state_0_");
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GENERIC_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GENERIC__PY_OBJECT_GET_ITEM_GENERIC_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GenericData.lookup_(), "generic_getClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_GENERIC_RAISE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, GENERIC__PY_OBJECT_GET_ITEM_GENERIC_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(GenericData.lookup_(), "generic_raise__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectGetItem#doList}
* 1: SpecializationActive {@link PyObjectGetItem#doGeneric}
* 2: SpecializationActive {@link PyObjectGetItem#doTuple}
* 3: SpecializationActive {@link PyObjectGetItem#doDict}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doList}
* Parameter: {@link com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode} getItemNode
*/
@Child private com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode list_getItemNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doTuple}
* Parameter: {@link com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode} getItemNode
*/
@Child private com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode tuple_getItemNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doDict}
* Parameter: {@link com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode} getItemNode
*/
@Child private com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode dict_getItemNode_;
@Child private GenericData generic_cache;
private PyObjectGetItemNodeGen() {
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)] || SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)] || SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] || SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)] */ && arg1Value instanceof PList) {
PList arg1Value_ = (PList) arg1Value;
{
com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode getItemNode__ = this.list_getItemNode_;
if (getItemNode__ != null) {
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
return PyObjectGetItem.doList((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)] */ && arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
{
com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode getItemNode__1 = this.tuple_getItemNode_;
if (getItemNode__1 != null) {
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
return PyObjectGetItem.doTuple((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__1);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] */ && arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
{
com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode getItemNode__2 = this.dict_getItemNode_;
if (getItemNode__2 != null) {
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
return PyObjectGetItem.doDict((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__2);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */) {
GenericData s3_ = this.generic_cache;
if (s3_ != null) {
return PyObjectGetItem.doGeneric((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, INLINED_GENERIC_GET_CLASS_NODE_, s3_.lookupGetItem_, s3_.callGetItem_, s3_.getItemClass_, INLINED_GENERIC_RAISE_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */ && arg1Value instanceof PList) {
PList arg1Value_ = (PList) arg1Value;
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode getItemNode__ = this.insert((com.oracle.graal.python.builtins.objects.list.ListBuiltinsFactory.GetItemNodeFactory.create()));
Objects.requireNonNull(getItemNode__, "Specialization 'doList(VirtualFrame, PList, Object, GetItemNode)' cache 'getItemNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.list_getItemNode_ = getItemNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)] */;
this.state_0_ = state_0;
return PyObjectGetItem.doList((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */ && arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode getItemNode__1 = this.insert((com.oracle.graal.python.builtins.objects.tuple.TupleBuiltinsFactory.GetItemNodeFactory.create()));
Objects.requireNonNull(getItemNode__1, "Specialization 'doTuple(VirtualFrame, PTuple, Object, GetItemNode)' cache 'getItemNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.tuple_getItemNode_ = getItemNode__1;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)] */;
this.state_0_ = state_0;
return PyObjectGetItem.doTuple((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__1);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */ && arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode getItemNode__2 = this.insert((com.oracle.graal.python.builtins.objects.dict.DictBuiltinsFactory.GetItemNodeFactory.create()));
Objects.requireNonNull(getItemNode__2, "Specialization 'doDict(VirtualFrame, PDict, Object, GetItemNode)' cache 'getItemNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.dict_getItemNode_ = getItemNode__2;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] */;
this.state_0_ = state_0;
return PyObjectGetItem.doDict((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__2);
}
}
GenericData s3_ = this.insert(new GenericData());
LookupSpecialMethodSlotNode lookupGetItem__ = s3_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetItem)));
Objects.requireNonNull(lookupGetItem__, "Specialization 'doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)' cache 'lookupGetItem' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.lookupGetItem_ = lookupGetItem__;
CallBinaryMethodNode callGetItem__ = s3_.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callGetItem__, "Specialization 'doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)' cache 'callGetItem' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.callGetItem_ = callGetItem__;
LazyPyObjectGetItemClass getItemClass__ = s3_.insert((LazyPyObjectGetItemClassNodeGen.create()));
Objects.requireNonNull(getItemClass__, "Specialization 'doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)' cache 'getItemClass' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.getItemClass_ = getItemClass__;
VarHandle.storeStoreFence();
this.generic_cache = s3_;
this.list_getItemNode_ = null;
this.tuple_getItemNode_ = null;
this.dict_getItemNode_ = null;
state_0 = state_0 & 0xfffffff2 /* remove SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)], SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)], SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */;
this.state_0_ = state_0;
return PyObjectGetItem.doGeneric((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, INLINED_GENERIC_GET_CLASS_NODE_, lookupGetItem__, callGetItem__, getItemClass__, INLINED_GENERIC_RAISE_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyObjectGetItem create() {
return new PyObjectGetItemNodeGen();
}
@NeverDefault
public static PyObjectGetItem getUncached() {
return PyObjectGetItemNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#list_getItemNode_}
*
- {@link Inlined#tuple_getItemNode_}
*
- {@link Inlined#dict_getItemNode_}
*
- {@link Inlined#generic_cache}
*
*/
@NeverDefault
public static PyObjectGetItem inline(@RequiredField(bits = 4, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectGetItemNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectGetItem.class)
@DenyReplace
private static final class Inlined extends PyObjectGetItem {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectGetItem#doList}
* 1: SpecializationActive {@link PyObjectGetItem#doGeneric}
* 2: SpecializationActive {@link PyObjectGetItem#doTuple}
* 3: SpecializationActive {@link PyObjectGetItem#doDict}
*
*/
private final StateField state_0_;
private final ReferenceField list_getItemNode_;
private final ReferenceField tuple_getItemNode_;
private final ReferenceField dict_getItemNode_;
private final ReferenceField generic_cache;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode generic_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy generic_raise_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectGetItem.class);
this.state_0_ = target.getState(0, 4);
this.list_getItemNode_ = target.getReference(1, com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode.class);
this.tuple_getItemNode_ = target.getReference(2, com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode.class);
this.dict_getItemNode_ = target.getReference(3, com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode.class);
this.generic_cache = target.getReference(4, GenericData.class);
this.generic_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GENERIC__PY_OBJECT_GET_ITEM_GENERIC_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GenericData.lookup_(), "generic_getClassNode__field1_", Node.class)));
this.generic_raise_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, GENERIC__PY_OBJECT_GET_ITEM_GENERIC_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(GenericData.lookup_(), "generic_raise__field1_", Node.class)));
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)] || SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)] || SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] || SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)] */ && arg1Value instanceof PList) {
PList arg1Value_ = (PList) arg1Value;
{
com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode getItemNode__ = this.list_getItemNode_.get(arg0Value);
if (getItemNode__ != null) {
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
return PyObjectGetItem.doList((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)] */ && arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
{
com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode getItemNode__1 = this.tuple_getItemNode_.get(arg0Value);
if (getItemNode__1 != null) {
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
return PyObjectGetItem.doTuple((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__1);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] */ && arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
{
com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode getItemNode__2 = this.dict_getItemNode_.get(arg0Value);
if (getItemNode__2 != null) {
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
return PyObjectGetItem.doDict((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__2);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */) {
GenericData s3_ = this.generic_cache.get(arg0Value);
if (s3_ != null) {
return PyObjectGetItem.doGeneric((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, this.generic_getClassNode_, s3_.lookupGetItem_, s3_.callGetItem_, s3_.getItemClass_, this.generic_raise_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */ && arg1Value instanceof PList) {
PList arg1Value_ = (PList) arg1Value;
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
com.oracle.graal.python.builtins.objects.list.ListBuiltins.GetItemNode getItemNode__ = arg0Value.insert((com.oracle.graal.python.builtins.objects.list.ListBuiltinsFactory.GetItemNodeFactory.create()));
Objects.requireNonNull(getItemNode__, "Specialization 'doList(VirtualFrame, PList, Object, GetItemNode)' cache 'getItemNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.list_getItemNode_.set(arg0Value, getItemNode__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectGetItem.doList((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */ && arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
com.oracle.graal.python.builtins.objects.tuple.TupleBuiltins.GetItemNode getItemNode__1 = arg0Value.insert((com.oracle.graal.python.builtins.objects.tuple.TupleBuiltinsFactory.GetItemNodeFactory.create()));
Objects.requireNonNull(getItemNode__1, "Specialization 'doTuple(VirtualFrame, PTuple, Object, GetItemNode)' cache 'getItemNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.tuple_getItemNode_.set(arg0Value, getItemNode__1);
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectGetItem.doTuple((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__1);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */ && arg1Value instanceof PDict) {
PDict arg1Value_ = (PDict) arg1Value;
if ((PGuards.cannotBeOverriddenForImmutableType(arg1Value_))) {
com.oracle.graal.python.builtins.objects.dict.DictBuiltins.GetItemNode getItemNode__2 = arg0Value.insert((com.oracle.graal.python.builtins.objects.dict.DictBuiltinsFactory.GetItemNodeFactory.create()));
Objects.requireNonNull(getItemNode__2, "Specialization 'doDict(VirtualFrame, PDict, Object, GetItemNode)' cache 'getItemNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.dict_getItemNode_.set(arg0Value, getItemNode__2);
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectGetItem.doDict((VirtualFrame) frameValue, arg1Value_, arg2Value, getItemNode__2);
}
}
GenericData s3_ = arg0Value.insert(new GenericData());
LookupSpecialMethodSlotNode lookupGetItem__ = s3_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetItem)));
Objects.requireNonNull(lookupGetItem__, "Specialization 'doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)' cache 'lookupGetItem' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.lookupGetItem_ = lookupGetItem__;
CallBinaryMethodNode callGetItem__ = s3_.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callGetItem__, "Specialization 'doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)' cache 'callGetItem' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.callGetItem_ = callGetItem__;
LazyPyObjectGetItemClass getItemClass__ = s3_.insert((LazyPyObjectGetItemClassNodeGen.create()));
Objects.requireNonNull(getItemClass__, "Specialization 'doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)' cache 'getItemClass' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.getItemClass_ = getItemClass__;
VarHandle.storeStoreFence();
this.generic_cache.set(arg0Value, s3_);
this.list_getItemNode_.set(arg0Value, null);
this.tuple_getItemNode_.set(arg0Value, null);
this.dict_getItemNode_.set(arg0Value, null);
state_0 = state_0 & 0xfffffff2 /* remove SpecializationActive[PyObjectGetItem.doList(VirtualFrame, PList, Object, GetItemNode)], SpecializationActive[PyObjectGetItem.doTuple(VirtualFrame, PTuple, Object, GetItemNode)], SpecializationActive[PyObjectGetItem.doDict(VirtualFrame, PDict, Object, GetItemNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectGetItem.doGeneric(VirtualFrame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, LazyPyObjectGetItemClass, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectGetItem.doGeneric((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, this.generic_getClassNode_, lookupGetItem__, callGetItem__, getItemClass__, this.generic_raise_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectGetItem.class)
@DenyReplace
private static final class GenericData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int generic_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node generic_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupGetItem
*/
@Child LookupSpecialMethodSlotNode lookupGetItem_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link CallBinaryMethodNode} callGetItem
*/
@Child CallBinaryMethodNode callGetItem_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link LazyPyObjectGetItemClass} getItemClass
*/
@Child LazyPyObjectGetItemClass getItemClass_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItem#doGeneric}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node generic_raise__field1_;
GenericData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectGetItem.class)
@DenyReplace
private static final class Uncached extends PyObjectGetItem {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return PyObjectGetItem.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.GetItem)), (CallBinaryMethodNode.getUncached()), (LazyPyObjectGetItemClassNodeGen.getUncached()), (Lazy.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link LazyPyObjectGetItemClass#doIt}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(LazyPyObjectGetItemClass.class)
@SuppressWarnings("javadoc")
static final class LazyPyObjectGetItemClassNodeGen extends LazyPyObjectGetItemClass {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link LazyPyObjectGetItemClass#doIt}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link LazyPyObjectGetItemClass#doIt}
* Parameter: {@link PyObjectGetItemClass} node
*/
@Child private PyObjectGetItemClass node_;
private LazyPyObjectGetItemClassNodeGen() {
}
@Override
PyObjectGetItemClass execute(Node frameValue) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyObjectGetItem.LazyPyObjectGetItemClass.doIt(PyObjectGetItemClass)] */) {
{
PyObjectGetItemClass node__ = this.node_;
if (node__ != null) {
return LazyPyObjectGetItemClass.doIt(node__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize();
}
private PyObjectGetItemClass executeAndSpecialize() {
int state_0 = this.state_0_;
PyObjectGetItemClass node__ = this.insert((PyObjectGetItemClassNodeGen.create()));
Objects.requireNonNull(node__, "Specialization 'doIt(PyObjectGetItemClass)' cache 'node' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.node_ = node__;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectGetItem.LazyPyObjectGetItemClass.doIt(PyObjectGetItemClass)] */;
this.state_0_ = state_0;
return LazyPyObjectGetItemClass.doIt(node__);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static LazyPyObjectGetItemClass create() {
return new LazyPyObjectGetItemClassNodeGen();
}
@NeverDefault
public static LazyPyObjectGetItemClass getUncached() {
return LazyPyObjectGetItemClassNodeGen.UNCACHED;
}
@GeneratedBy(LazyPyObjectGetItemClass.class)
@DenyReplace
private static final class Uncached extends LazyPyObjectGetItemClass {
@TruffleBoundary
@Override
PyObjectGetItemClass execute(Node frameValue) {
return LazyPyObjectGetItemClass.doIt((PyObjectGetItemClassNodeGen.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link PyObjectGetItemClass#doGeneric}
* Activation probability: 1.00000
* With/without class size: 76/54 bytes
*
*/
@GeneratedBy(PyObjectGetItemClass.class)
@SuppressWarnings("javadoc")
static final class PyObjectGetItemClassNodeGen extends PyObjectGetItemClass {
private static final StateField STATE_0_PyObjectGetItemClass_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
*/
private static final IsTypeNode INLINED_IS_TYPE_NODE_ = IsTypeNodeGen.inline(InlineTarget.create(IsTypeNode.class, STATE_0_PyObjectGetItemClass_UPDATER.subUpdater(1, 5), ReferenceField.create(MethodHandles.lookup(), "isTypeNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
*/
private static final PyObjectLookupAttr INLINED_LOOKUP_CLASS_GET_ITEM_ = PyObjectLookupAttrNodeGen.inline(InlineTarget.create(PyObjectLookupAttr.class, STATE_0_PyObjectGetItemClass_UPDATER.subUpdater(6, 5), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field3_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field4_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field5_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field6_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field7_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field8_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field9_", Node.class), ReferenceField.create(MethodHandles.lookup(), "lookupClassGetItem__field10_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link InlineIsBuiltinClassProfile} isBuiltinClassProfile
* Inline method: {@link InlineIsBuiltinClassProfileNodeGen#inline}
*/
private static final InlineIsBuiltinClassProfile INLINED_IS_BUILTIN_CLASS_PROFILE_ = InlineIsBuiltinClassProfileNodeGen.inline(InlineTarget.create(InlineIsBuiltinClassProfile.class, STATE_0_PyObjectGetItemClass_UPDATER.subUpdater(11, 3)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectGetItemClass#doGeneric}
* 1-5: InlinedCache
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
* 6-10: InlinedCache
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* 11-13: InlinedCache
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link InlineIsBuiltinClassProfile} isBuiltinClassProfile
* Inline method: {@link InlineIsBuiltinClassProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node isTypeNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field2_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field3_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field4_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field5_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field6
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field6_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field7
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field7_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field8
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field8_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field9
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field9_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PyObjectLookupAttr} lookupClassGetItem
* Inline method: {@link PyObjectLookupAttrNodeGen#inline}
* Inline field: {@link Node} field10
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupClassGetItem__field10_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory_;
/**
* Source Info:
* Specialization: {@link PyObjectGetItemClass#doGeneric}
* Parameter: {@link CallNode} callClassGetItem
*/
@Child private CallNode callClassGetItem_;
private PyObjectGetItemClassNodeGen() {
}
@Override
public Object execute(Frame frameValue, Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectGetItem.PyObjectGetItemClass.doGeneric(VirtualFrame, Object, Object, Node, IsTypeNode, PyObjectLookupAttr, InlineIsBuiltinClassProfile, PythonObjectFactory, CallNode)] */) {
{
PythonObjectFactory factory__ = this.factory_;
if (factory__ != null) {
CallNode callClassGetItem__ = this.callClassGetItem_;
if (callClassGetItem__ != null) {
Node inliningTarget__ = (this);
return PyObjectGetItemClass.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, inliningTarget__, INLINED_IS_TYPE_NODE_, INLINED_LOOKUP_CLASS_GET_ITEM_, INLINED_IS_BUILTIN_CLASS_PROFILE_, factory__, callClassGetItem__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
PythonObjectFactory factory__ = this.insert((PythonObjectFactory.create()));
Objects.requireNonNull(factory__, "Specialization 'doGeneric(VirtualFrame, Object, Object, Node, IsTypeNode, PyObjectLookupAttr, InlineIsBuiltinClassProfile, PythonObjectFactory, CallNode)' cache 'factory' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.factory_ = factory__;
CallNode callClassGetItem__ = this.insert((CallNode.create()));
Objects.requireNonNull(callClassGetItem__, "Specialization 'doGeneric(VirtualFrame, Object, Object, Node, IsTypeNode, PyObjectLookupAttr, InlineIsBuiltinClassProfile, PythonObjectFactory, CallNode)' cache 'callClassGetItem' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callClassGetItem_ = callClassGetItem__;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectGetItem.PyObjectGetItemClass.doGeneric(VirtualFrame, Object, Object, Node, IsTypeNode, PyObjectLookupAttr, InlineIsBuiltinClassProfile, PythonObjectFactory, CallNode)] */;
this.state_0_ = state_0;
return PyObjectGetItemClass.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, inliningTarget__, INLINED_IS_TYPE_NODE_, INLINED_LOOKUP_CLASS_GET_ITEM_, INLINED_IS_BUILTIN_CLASS_PROFILE_, factory__, callClassGetItem__);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static PyObjectGetItemClass create() {
return new PyObjectGetItemClassNodeGen();
}
@NeverDefault
public static PyObjectGetItemClass getUncached() {
return PyObjectGetItemClassNodeGen.UNCACHED;
}
@GeneratedBy(PyObjectGetItemClass.class)
@DenyReplace
private static final class Uncached extends PyObjectGetItemClass {
@Override
public Object execute(Frame frameValue, Object arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return PyObjectGetItemClass.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, (this), (IsTypeNodeGen.getUncached()), (PyObjectLookupAttr.getUncached()), (InlineIsBuiltinClassProfile.getUncached()), (PythonObjectFactory.getUncached()), (CallNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy