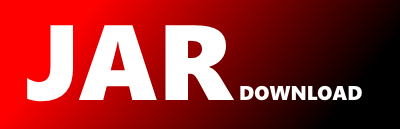
com.oracle.graal.python.lib.PyObjectReprAsObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.object.ObjectNodes.DefaultObjectReprNode;
import com.oracle.graal.python.builtins.objects.object.ObjectNodesFactory.DefaultObjectReprNodeGen;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectReprAsObjectNode#repr}
* Activation probability: 1.00000
* With/without class size: 44/23 bytes
*
*/
@GeneratedBy(PyObjectReprAsObjectNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyObjectReprAsObjectNodeGen extends PyObjectReprAsObjectNode {
private static final StateField STATE_0_PyObjectReprAsObjectNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_0_PyObjectReprAsObjectNode_UPDATER.subUpdater(1, 17), ReferenceField.create(MethodHandles.lookup(), "getClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isString
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_STRING_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_PyObjectReprAsObjectNode_UPDATER.subUpdater(18, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isPString
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_P_STRING_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_PyObjectReprAsObjectNode_UPDATER.subUpdater(20, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STATE_0_PyObjectReprAsObjectNode_UPDATER.subUpdater(22, 1), ReferenceField.create(MethodHandles.lookup(), "raiseNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectReprAsObjectNode#repr}
* 1-17: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 18-19: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isString
* Inline method: {@link InlinedConditionProfile#inline}
* 20-21: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isPString
* Inline method: {@link InlinedConditionProfile#inline}
* 22: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupRepr
*/
@Child private LookupSpecialMethodSlotNode lookupRepr_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link CallUnaryMethodNode} callRepr
*/
@Child private CallUnaryMethodNode callRepr_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link DefaultObjectReprNode} defaultRepr
*/
@Child private DefaultObjectReprNode defaultRepr_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node raiseNode__field1_;
private PyObjectReprAsObjectNodeGen() {
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectReprAsObjectNode.repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)] */) {
{
LookupSpecialMethodSlotNode lookupRepr__ = this.lookupRepr_;
if (lookupRepr__ != null) {
CallUnaryMethodNode callRepr__ = this.callRepr_;
if (callRepr__ != null) {
DefaultObjectReprNode defaultRepr__ = this.defaultRepr_;
if (defaultRepr__ != null) {
return PyObjectReprAsObjectNode.repr((VirtualFrame) frameValue, this, arg1Value, INLINED_GET_CLASS_NODE_, lookupRepr__, callRepr__, defaultRepr__, INLINED_IS_STRING_, INLINED_IS_P_STRING_, INLINED_RAISE_NODE_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
LookupSpecialMethodSlotNode lookupRepr__ = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Repr)));
Objects.requireNonNull(lookupRepr__, "Specialization 'repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)' cache 'lookupRepr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupRepr_ = lookupRepr__;
CallUnaryMethodNode callRepr__ = this.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callRepr__, "Specialization 'repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)' cache 'callRepr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callRepr_ = callRepr__;
DefaultObjectReprNode defaultRepr__ = this.insert((DefaultObjectReprNode.create()));
Objects.requireNonNull(defaultRepr__, "Specialization 'repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)' cache 'defaultRepr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.defaultRepr_ = defaultRepr__;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectReprAsObjectNode.repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)] */;
this.state_0_ = state_0;
return PyObjectReprAsObjectNode.repr((VirtualFrame) frameValue, this, arg1Value, INLINED_GET_CLASS_NODE_, lookupRepr__, callRepr__, defaultRepr__, INLINED_IS_STRING_, INLINED_IS_P_STRING_, INLINED_RAISE_NODE_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static PyObjectReprAsObjectNode create() {
return new PyObjectReprAsObjectNodeGen();
}
@NeverDefault
public static PyObjectReprAsObjectNode getUncached() {
return PyObjectReprAsObjectNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#getClassNode__field1_}
*
- {@link Inlined#lookupRepr_}
*
- {@link Inlined#callRepr_}
*
- {@link Inlined#defaultRepr_}
*
- {@link Inlined#raiseNode__field1_}
*
*/
@NeverDefault
public static PyObjectReprAsObjectNode inline(@RequiredField(bits = 23, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectReprAsObjectNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectReprAsObjectNode.class)
@DenyReplace
private static final class Inlined extends PyObjectReprAsObjectNode {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectReprAsObjectNode#repr}
* 1-17: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 18-19: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isString
* Inline method: {@link InlinedConditionProfile#inline}
* 20-21: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isPString
* Inline method: {@link InlinedConditionProfile#inline}
* 22: InlinedCache
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField getClassNode__field1_;
private final ReferenceField lookupRepr_;
private final ReferenceField callRepr_;
private final ReferenceField defaultRepr_;
private final ReferenceField raiseNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getClassNode_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isString
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile isString_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link InlinedConditionProfile} isPString
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile isPString_;
/**
* Source Info:
* Specialization: {@link PyObjectReprAsObjectNode#repr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy raiseNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectReprAsObjectNode.class);
this.state_0_ = target.getState(0, 23);
this.getClassNode__field1_ = target.getReference(1, Node.class);
this.lookupRepr_ = target.getReference(2, LookupSpecialMethodSlotNode.class);
this.callRepr_ = target.getReference(3, CallUnaryMethodNode.class);
this.defaultRepr_ = target.getReference(4, DefaultObjectReprNode.class);
this.raiseNode__field1_ = target.getReference(5, Node.class);
this.getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, state_0_.subUpdater(1, 17), getClassNode__field1_));
this.isString_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, state_0_.subUpdater(18, 2)));
this.isPString_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, state_0_.subUpdater(20, 2)));
this.raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, state_0_.subUpdater(22, 1), raiseNode__field1_));
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectReprAsObjectNode.repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)] */) {
{
LookupSpecialMethodSlotNode lookupRepr__ = this.lookupRepr_.get(arg0Value);
if (lookupRepr__ != null) {
CallUnaryMethodNode callRepr__ = this.callRepr_.get(arg0Value);
if (callRepr__ != null) {
DefaultObjectReprNode defaultRepr__ = this.defaultRepr_.get(arg0Value);
if (defaultRepr__ != null) {
assert InlineSupport.validate(arg0Value, this.state_0_, this.getClassNode__field1_, this.state_0_, this.state_0_, this.state_0_, this.raiseNode__field1_);
return PyObjectReprAsObjectNode.repr((VirtualFrame) frameValue, arg0Value, arg1Value, this.getClassNode_, lookupRepr__, callRepr__, defaultRepr__, this.isString_, this.isPString_, this.raiseNode_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
LookupSpecialMethodSlotNode lookupRepr__ = arg0Value.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Repr)));
Objects.requireNonNull(lookupRepr__, "Specialization 'repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)' cache 'lookupRepr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupRepr_.set(arg0Value, lookupRepr__);
CallUnaryMethodNode callRepr__ = arg0Value.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callRepr__, "Specialization 'repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)' cache 'callRepr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callRepr_.set(arg0Value, callRepr__);
DefaultObjectReprNode defaultRepr__ = arg0Value.insert((DefaultObjectReprNode.create()));
Objects.requireNonNull(defaultRepr__, "Specialization 'repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)' cache 'defaultRepr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.defaultRepr_.set(arg0Value, defaultRepr__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectReprAsObjectNode.repr(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, DefaultObjectReprNode, InlinedConditionProfile, InlinedConditionProfile, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.getClassNode__field1_, this.state_0_, this.state_0_, this.state_0_, this.raiseNode__field1_);
return PyObjectReprAsObjectNode.repr((VirtualFrame) frameValue, arg0Value, arg1Value, this.getClassNode_, lookupRepr__, callRepr__, defaultRepr__, this.isString_, this.isPString_, this.raiseNode_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectReprAsObjectNode.class)
@DenyReplace
private static final class Uncached extends PyObjectReprAsObjectNode {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return PyObjectReprAsObjectNode.repr((VirtualFrame) frameValue, arg0Value, arg1Value, (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.Repr)), (CallUnaryMethodNode.getUncached()), (DefaultObjectReprNodeGen.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()), (Lazy.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy