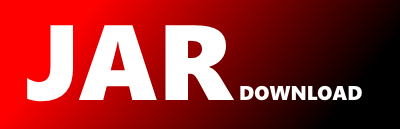
com.oracle.graal.python.lib.PyObjectStrAsObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromLongNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectStrAsObjectNode#str(TruffleString)}
* Activation probability: 0.38500
* With/without class size: 8/0 bytes
* Specialization {@link PyObjectStrAsObjectNode#str(boolean)}
* Activation probability: 0.29500
* With/without class size: 7/0 bytes
* Specialization {@link PyObjectStrAsObjectNode#str(long, FromLongNode)}
* Activation probability: 0.20500
* With/without class size: 8/4 bytes
* Specialization {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Activation probability: 0.11500
* With/without class size: 9/29 bytes
*
*/
@GeneratedBy(PyObjectStrAsObjectNode.class)
@SuppressWarnings("javadoc")
public final class PyObjectStrAsObjectNodeGen extends PyObjectStrAsObjectNode {
private static final StateField STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_0_UPDATER = StateField.create(Str3Data.lookup_(), "str3_state_0_");
private static final StateField STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_1_UPDATER = StateField.create(Str3Data.lookup_(), "str3_state_1_");
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_STR3_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(Str3Data.lookup_(), "str3_getClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getResultClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_STR3_GET_RESULT_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(Str3Data.lookup_(), "str3_getResultClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_STR3_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(Str3Data.lookup_(), "str3_raiseNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectStrAsObjectNode#str(TruffleString)}
* 1: SpecializationActive {@link PyObjectStrAsObjectNode#str(boolean)}
* 2: SpecializationActive {@link PyObjectStrAsObjectNode#str(long, FromLongNode)}
* 3: SpecializationActive {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(long, FromLongNode)}
* Parameter: {@link FromLongNode} fromLongNode
*/
@Child private FromLongNode str2_fromLongNode_;
@Child private Str3Data str3_cache;
private PyObjectStrAsObjectNodeGen() {
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(TruffleString)] || SpecializationActive[PyObjectStrAsObjectNode.str(boolean)] || SpecializationActive[PyObjectStrAsObjectNode.str(long, FromLongNode)] || SpecializationActive[PyObjectStrAsObjectNode.str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(boolean)] */ && arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(long, FromLongNode)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
{
FromLongNode fromLongNode__ = this.str2_fromLongNode_;
if (fromLongNode__ != null) {
return str(arg1Value_, fromLongNode__);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)] */) {
Str3Data s3_ = this.str3_cache;
if (s3_ != null) {
if ((!(PGuards.isTruffleString(arg1Value)))) {
return PyObjectStrAsObjectNode.str((VirtualFrame) frameValue, s3_, arg1Value, INLINED_STR3_GET_CLASS_NODE_, s3_.lookupStr_, s3_.callStr_, INLINED_STR3_GET_RESULT_CLASS_NODE_, s3_.isSubtypeNode_, INLINED_STR3_RAISE_NODE_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectStrAsObjectNode.str(TruffleString)] */;
this.state_0_ = state_0;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectStrAsObjectNode.str(boolean)] */;
this.state_0_ = state_0;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
FromLongNode fromLongNode__ = this.insert((FromLongNode.create()));
Objects.requireNonNull(fromLongNode__, "Specialization 'str(long, FromLongNode)' cache 'fromLongNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.str2_fromLongNode_ = fromLongNode__;
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectStrAsObjectNode.str(long, FromLongNode)] */;
this.state_0_ = state_0;
return str(arg1Value_, fromLongNode__);
}
if ((!(PGuards.isTruffleString(arg1Value)))) {
Str3Data s3_ = this.insert(new Str3Data());
LookupSpecialMethodSlotNode lookupStr__ = s3_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Str)));
Objects.requireNonNull(lookupStr__, "Specialization 'str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)' cache 'lookupStr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.lookupStr_ = lookupStr__;
CallUnaryMethodNode callStr__ = s3_.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callStr__, "Specialization 'str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)' cache 'callStr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.callStr_ = callStr__;
IsSubtypeNode isSubtypeNode__ = s3_.insert((IsSubtypeNode.create()));
Objects.requireNonNull(isSubtypeNode__, "Specialization 'str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)' cache 'isSubtypeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.isSubtypeNode_ = isSubtypeNode__;
VarHandle.storeStoreFence();
this.str3_cache = s3_;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectStrAsObjectNode.str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)] */;
this.state_0_ = state_0;
return PyObjectStrAsObjectNode.str((VirtualFrame) frameValue, s3_, arg1Value, INLINED_STR3_GET_CLASS_NODE_, lookupStr__, callStr__, INLINED_STR3_GET_RESULT_CLASS_NODE_, isSubtypeNode__, INLINED_STR3_RAISE_NODE_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyObjectStrAsObjectNode create() {
return new PyObjectStrAsObjectNodeGen();
}
@NeverDefault
public static PyObjectStrAsObjectNode getUncached() {
return PyObjectStrAsObjectNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#str2_fromLongNode_}
*
- {@link Inlined#str3_cache}
*
*/
@NeverDefault
public static PyObjectStrAsObjectNode inline(@RequiredField(bits = 4, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectStrAsObjectNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectStrAsObjectNode.class)
@DenyReplace
private static final class Inlined extends PyObjectStrAsObjectNode {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectStrAsObjectNode#str(TruffleString)}
* 1: SpecializationActive {@link PyObjectStrAsObjectNode#str(boolean)}
* 2: SpecializationActive {@link PyObjectStrAsObjectNode#str(long, FromLongNode)}
* 3: SpecializationActive {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
*
*/
private final StateField state_0_;
private final ReferenceField str2_fromLongNode_;
private final ReferenceField str3_cache;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode str3_getClassNode_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getResultClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode str3_getResultClassNode_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy str3_raiseNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectStrAsObjectNode.class);
this.state_0_ = target.getState(0, 4);
this.str2_fromLongNode_ = target.getReference(1, FromLongNode.class);
this.str3_cache = target.getReference(2, Str3Data.class);
this.str3_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(Str3Data.lookup_(), "str3_getClassNode__field1_", Node.class)));
this.str3_getResultClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(Str3Data.lookup_(), "str3_getResultClassNode__field1_", Node.class)));
this.str3_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STR3__PY_OBJECT_STR_AS_OBJECT_NODE_STR3_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(Str3Data.lookup_(), "str3_raiseNode__field1_", Node.class)));
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(TruffleString)] || SpecializationActive[PyObjectStrAsObjectNode.str(boolean)] || SpecializationActive[PyObjectStrAsObjectNode.str(long, FromLongNode)] || SpecializationActive[PyObjectStrAsObjectNode.str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(boolean)] */ && arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(long, FromLongNode)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
{
FromLongNode fromLongNode__ = this.str2_fromLongNode_.get(arg0Value);
if (fromLongNode__ != null) {
return str(arg1Value_, fromLongNode__);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectStrAsObjectNode.str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)] */) {
Str3Data s3_ = this.str3_cache.get(arg0Value);
if (s3_ != null) {
if ((!(PGuards.isTruffleString(arg1Value)))) {
return PyObjectStrAsObjectNode.str((VirtualFrame) frameValue, s3_, arg1Value, this.str3_getClassNode_, s3_.lookupStr_, s3_.callStr_, this.str3_getResultClassNode_, s3_.isSubtypeNode_, this.str3_raiseNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectStrAsObjectNode.str(TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectStrAsObjectNode.str(boolean)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
FromLongNode fromLongNode__ = arg0Value.insert((FromLongNode.create()));
Objects.requireNonNull(fromLongNode__, "Specialization 'str(long, FromLongNode)' cache 'fromLongNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.str2_fromLongNode_.set(arg0Value, fromLongNode__);
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectStrAsObjectNode.str(long, FromLongNode)] */;
this.state_0_.set(arg0Value, state_0);
return str(arg1Value_, fromLongNode__);
}
if ((!(PGuards.isTruffleString(arg1Value)))) {
Str3Data s3_ = arg0Value.insert(new Str3Data());
LookupSpecialMethodSlotNode lookupStr__ = s3_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Str)));
Objects.requireNonNull(lookupStr__, "Specialization 'str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)' cache 'lookupStr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.lookupStr_ = lookupStr__;
CallUnaryMethodNode callStr__ = s3_.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callStr__, "Specialization 'str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)' cache 'callStr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.callStr_ = callStr__;
IsSubtypeNode isSubtypeNode__ = s3_.insert((IsSubtypeNode.create()));
Objects.requireNonNull(isSubtypeNode__, "Specialization 'str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)' cache 'isSubtypeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.isSubtypeNode_ = isSubtypeNode__;
VarHandle.storeStoreFence();
this.str3_cache.set(arg0Value, s3_);
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectStrAsObjectNode.str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectStrAsObjectNode.str((VirtualFrame) frameValue, s3_, arg1Value, this.str3_getClassNode_, lookupStr__, callStr__, this.str3_getResultClassNode_, isSubtypeNode__, this.str3_raiseNode_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectStrAsObjectNode.class)
@DenyReplace
private static final class Str3Data extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int str3_state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getResultClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int str3_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node str3_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupStr
*/
@Child LookupSpecialMethodSlotNode lookupStr_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link CallUnaryMethodNode} callStr
*/
@Child CallUnaryMethodNode callStr_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link GetClassNode} getResultClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node str3_getResultClassNode__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link IsSubtypeNode} isSubtypeNode
*/
@Child IsSubtypeNode isSubtypeNode_;
/**
* Source Info:
* Specialization: {@link PyObjectStrAsObjectNode#str(VirtualFrame, Node, Object, GetClassNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, GetClassNode, IsSubtypeNode, Lazy)}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node str3_raiseNode__field1_;
Str3Data() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectStrAsObjectNode.class)
@DenyReplace
private static final class Uncached extends PyObjectStrAsObjectNode {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return PyObjectStrAsObjectNode.str(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
return str(arg1Value_, (FromLongNode.getUncached()));
}
if ((!(PGuards.isTruffleString(arg1Value)))) {
return PyObjectStrAsObjectNode.str((VirtualFrame) frameValue, arg0Value, arg1Value, (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.Str)), (CallUnaryMethodNode.getUncached()), (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()), (Lazy.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy