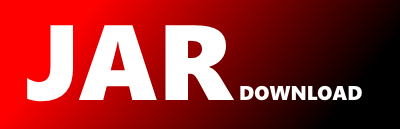
com.oracle.graal.python.lib.PySequenceIterSearchNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.lib.PyObjectRichCompareBool.EqNode;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.nodes.object.BuiltinClassProfiles.IsBuiltinObjectProfile;
import com.oracle.graal.python.nodes.object.BuiltinClassProfilesFactory.IsBuiltinObjectProfileNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.IntField;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedIntValueProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PySequenceIterSearchNode#search}
* Activation probability: 1.00000
* With/without class size: 76/55 bytes
*
*/
@GeneratedBy(PySequenceIterSearchNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PySequenceIterSearchNodeGen extends PySequenceIterSearchNode {
private static final StateField STATE_0_PySequenceIterSearchNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField STATE_1_PySequenceIterSearchNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
private static final StateField STATE_2_PySequenceIterSearchNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_2_");
private static final StateField STATE_3_PySequenceIterSearchNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_3_");
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
*/
private static final PyObjectGetIter INLINED_GET_ITER_ = PyObjectGetIterNodeGen.inline(InlineTarget.create(PyObjectGetIter.class, STATE_0_PySequenceIterSearchNode_UPDATER.subUpdater(1, 2), ReferenceField.create(MethodHandles.lookup(), "getIter__field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "getIter__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noIterProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_NO_ITER_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, STATE_0_PySequenceIterSearchNode_UPDATER.subUpdater(3, 20), ReferenceField.create(MethodHandles.lookup(), "noIterProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STATE_0_PySequenceIterSearchNode_UPDATER.subUpdater(23, 1), ReferenceField.create(MethodHandles.lookup(), "raiseNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link GetClassNode} getIterClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_ITER_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_1_PySequenceIterSearchNode_UPDATER.subUpdater(0, 17), ReferenceField.create(MethodHandles.lookup(), "getIterClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noNextProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_NO_NEXT_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, STATE_2_PySequenceIterSearchNode_UPDATER.subUpdater(0, 20), ReferenceField.create(MethodHandles.lookup(), "noNextProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_STOP_ITERATION_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, STATE_3_PySequenceIterSearchNode_UPDATER.subUpdater(0, 20), ReferenceField.create(MethodHandles.lookup(), "stopIterationProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link InlinedIntValueProfile} opProfile
* Inline method: {@link InlinedIntValueProfile#inline}
*/
private static final InlinedIntValueProfile INLINED_OP_PROFILE_ = InlinedIntValueProfile.inline(InlineTarget.create(InlinedIntValueProfile.class, STATE_0_PySequenceIterSearchNode_UPDATER.subUpdater(24, 2), IntField.create(MethodHandles.lookup(), "opProfile__field1_")));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PySequenceIterSearchNode#search}
* 1-2: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* 3-22: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noIterProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* 23: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* 24-25: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link InlinedIntValueProfile} opProfile
* Inline method: {@link InlinedIntValueProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link GetClassNode} getIterClass
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_1_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noNextProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_2_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_3_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getIter__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getIter__field2_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noIterProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node noIterProfile__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node raiseNode__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link GetClassNode} getIterClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getIterClass__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupIternext
*/
@Child private LookupSpecialMethodSlotNode lookupIternext_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noNextProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node noNextProfile__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link CallUnaryMethodNode} callNext
*/
@Child private CallUnaryMethodNode callNext_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link EqNode} eqNode
*/
@Child private EqNode eqNode_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node stopIterationProfile__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link InlinedIntValueProfile} opProfile
* Inline method: {@link InlinedIntValueProfile#inline}
* Inline field: int field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private int opProfile__field1_;
private PySequenceIterSearchNodeGen() {
}
@Override
public int execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PySequenceIterSearchNode.search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)] */) {
{
LookupSpecialMethodSlotNode lookupIternext__ = this.lookupIternext_;
if (lookupIternext__ != null) {
CallUnaryMethodNode callNext__ = this.callNext_;
if (callNext__ != null) {
EqNode eqNode__ = this.eqNode_;
if (eqNode__ != null) {
return PySequenceIterSearchNode.search(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_ITER_, INLINED_NO_ITER_PROFILE_, INLINED_RAISE_NODE_, INLINED_GET_ITER_CLASS_, lookupIternext__, INLINED_NO_NEXT_PROFILE_, callNext__, eqNode__, INLINED_STOP_ITERATION_PROFILE_, INLINED_OP_PROFILE_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private int executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_;
LookupSpecialMethodSlotNode lookupIternext__ = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Next)));
Objects.requireNonNull(lookupIternext__, "Specialization 'search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)' cache 'lookupIternext' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupIternext_ = lookupIternext__;
CallUnaryMethodNode callNext__ = this.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callNext__, "Specialization 'search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)' cache 'callNext' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callNext_ = callNext__;
EqNode eqNode__ = this.insert((EqNode.create()));
Objects.requireNonNull(eqNode__, "Specialization 'search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)' cache 'eqNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.eqNode_ = eqNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[PySequenceIterSearchNode.search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)] */;
this.state_0_ = state_0;
return PySequenceIterSearchNode.search(frameValue, this, arg1Value, arg2Value, arg3Value, INLINED_GET_ITER_, INLINED_NO_ITER_PROFILE_, INLINED_RAISE_NODE_, INLINED_GET_ITER_CLASS_, lookupIternext__, INLINED_NO_NEXT_PROFILE_, callNext__, eqNode__, INLINED_STOP_ITERATION_PROFILE_, INLINED_OP_PROFILE_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static PySequenceIterSearchNode create() {
return new PySequenceIterSearchNodeGen();
}
@NeverDefault
public static PySequenceIterSearchNode getUncached() {
return PySequenceIterSearchNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#state_1_}
*
- {@link Inlined#state_2_}
*
- {@link Inlined#state_3_}
*
- {@link Inlined#getIter__field1_}
*
- {@link Inlined#getIter__field2_}
*
- {@link Inlined#noIterProfile__field1_}
*
- {@link Inlined#raiseNode__field1_}
*
- {@link Inlined#getIterClass__field1_}
*
- {@link Inlined#lookupIternext_}
*
- {@link Inlined#noNextProfile__field1_}
*
- {@link Inlined#callNext_}
*
- {@link Inlined#eqNode_}
*
- {@link Inlined#stopIterationProfile__field1_}
*
- {@link Inlined#opProfile__field1_}
*
*/
@NeverDefault
public static PySequenceIterSearchNode inline(@RequiredField(bits = 26, value = StateField.class)@RequiredField(bits = 17, value = StateField.class)@RequiredField(bits = 20, value = StateField.class)@RequiredField(bits = 20, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(IntField.class) InlineTarget target) {
return new PySequenceIterSearchNodeGen.Inlined(target);
}
@GeneratedBy(PySequenceIterSearchNode.class)
@DenyReplace
private static final class Inlined extends PySequenceIterSearchNode {
/**
* State Info:
* 0: SpecializationActive {@link PySequenceIterSearchNode#search}
* 1-2: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* 3-22: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noIterProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* 23: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* 24-25: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link InlinedIntValueProfile} opProfile
* Inline method: {@link InlinedIntValueProfile#inline}
*
*/
private final StateField state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link GetClassNode} getIterClass
* Inline method: {@link GetClassNodeGen#inline}
*
*/
private final StateField state_1_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noNextProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
private final StateField state_2_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
private final StateField state_3_;
private final ReferenceField getIter__field1_;
private final ReferenceField getIter__field2_;
private final ReferenceField noIterProfile__field1_;
private final ReferenceField raiseNode__field1_;
private final ReferenceField getIterClass__field1_;
private final ReferenceField lookupIternext_;
private final ReferenceField noNextProfile__field1_;
private final ReferenceField callNext_;
private final ReferenceField eqNode_;
private final ReferenceField stopIterationProfile__field1_;
private final IntField opProfile__field1_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
*/
private final PyObjectGetIter getIter_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noIterProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile noIterProfile_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy raiseNode_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link GetClassNode} getIterClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getIterClass_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} noNextProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile noNextProfile_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile stopIterationProfile_;
/**
* Source Info:
* Specialization: {@link PySequenceIterSearchNode#search}
* Parameter: {@link InlinedIntValueProfile} opProfile
* Inline method: {@link InlinedIntValueProfile#inline}
*/
private final InlinedIntValueProfile opProfile_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PySequenceIterSearchNode.class);
this.state_0_ = target.getState(0, 26);
this.state_1_ = target.getState(1, 17);
this.state_2_ = target.getState(2, 20);
this.state_3_ = target.getState(3, 20);
this.getIter__field1_ = target.getReference(4, Node.class);
this.getIter__field2_ = target.getReference(5, Node.class);
this.noIterProfile__field1_ = target.getReference(6, Node.class);
this.raiseNode__field1_ = target.getReference(7, Node.class);
this.getIterClass__field1_ = target.getReference(8, Node.class);
this.lookupIternext_ = target.getReference(9, LookupSpecialMethodSlotNode.class);
this.noNextProfile__field1_ = target.getReference(10, Node.class);
this.callNext_ = target.getReference(11, CallUnaryMethodNode.class);
this.eqNode_ = target.getReference(12, EqNode.class);
this.stopIterationProfile__field1_ = target.getReference(13, Node.class);
this.opProfile__field1_ = target.getPrimitive(14, IntField.class);
this.getIter_ = PyObjectGetIterNodeGen.inline(InlineTarget.create(PyObjectGetIter.class, state_0_.subUpdater(1, 2), getIter__field1_, getIter__field2_));
this.noIterProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, state_0_.subUpdater(3, 20), noIterProfile__field1_));
this.raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, state_0_.subUpdater(23, 1), raiseNode__field1_));
this.getIterClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, state_1_.subUpdater(0, 17), getIterClass__field1_));
this.noNextProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, state_2_.subUpdater(0, 20), noNextProfile__field1_));
this.stopIterationProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, state_3_.subUpdater(0, 20), stopIterationProfile__field1_));
this.opProfile_ = InlinedIntValueProfile.inline(InlineTarget.create(InlinedIntValueProfile.class, state_0_.subUpdater(24, 2), opProfile__field1_));
}
@Override
public int execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PySequenceIterSearchNode.search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)] */) {
{
LookupSpecialMethodSlotNode lookupIternext__ = this.lookupIternext_.get(arg0Value);
if (lookupIternext__ != null) {
CallUnaryMethodNode callNext__ = this.callNext_.get(arg0Value);
if (callNext__ != null) {
EqNode eqNode__ = this.eqNode_.get(arg0Value);
if (eqNode__ != null) {
assert InlineSupport.validate(arg0Value, this.state_0_, this.getIter__field1_, this.getIter__field2_, this.state_0_, this.noIterProfile__field1_, this.state_0_, this.raiseNode__field1_, this.state_1_, this.getIterClass__field1_, this.state_2_, this.noNextProfile__field1_, this.state_3_, this.stopIterationProfile__field1_, this.state_0_, this.opProfile__field1_);
return PySequenceIterSearchNode.search(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getIter_, this.noIterProfile_, this.raiseNode_, this.getIterClass_, lookupIternext__, this.noNextProfile_, callNext__, eqNode__, this.stopIterationProfile_, this.opProfile_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private int executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
LookupSpecialMethodSlotNode lookupIternext__ = arg0Value.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Next)));
Objects.requireNonNull(lookupIternext__, "Specialization 'search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)' cache 'lookupIternext' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupIternext_.set(arg0Value, lookupIternext__);
CallUnaryMethodNode callNext__ = arg0Value.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callNext__, "Specialization 'search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)' cache 'callNext' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callNext_.set(arg0Value, callNext__);
EqNode eqNode__ = arg0Value.insert((EqNode.create()));
Objects.requireNonNull(eqNode__, "Specialization 'search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)' cache 'eqNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.eqNode_.set(arg0Value, eqNode__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PySequenceIterSearchNode.search(Frame, Node, Object, Object, int, PyObjectGetIter, IsBuiltinObjectProfile, Lazy, GetClassNode, LookupSpecialMethodSlotNode, IsBuiltinObjectProfile, CallUnaryMethodNode, EqNode, IsBuiltinObjectProfile, InlinedIntValueProfile)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.getIter__field1_, this.getIter__field2_, this.state_0_, this.noIterProfile__field1_, this.state_0_, this.raiseNode__field1_, this.state_1_, this.getIterClass__field1_, this.state_2_, this.noNextProfile__field1_, this.state_3_, this.stopIterationProfile__field1_, this.state_0_, this.opProfile__field1_);
return PySequenceIterSearchNode.search(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, this.getIter_, this.noIterProfile_, this.raiseNode_, this.getIterClass_, lookupIternext__, this.noNextProfile_, callNext__, eqNode__, this.stopIterationProfile_, this.opProfile_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PySequenceIterSearchNode.class)
@DenyReplace
private static final class Uncached extends PySequenceIterSearchNode {
@Override
public int execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return PySequenceIterSearchNode.search(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, (PyObjectGetIter.getUncached()), (IsBuiltinObjectProfile.getUncached()), (Lazy.getUncached()), (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.Next)), (IsBuiltinObjectProfile.getUncached()), (CallUnaryMethodNode.getUncached()), (EqNode.getUncached()), (IsBuiltinObjectProfile.getUncached()), (InlinedIntValueProfile.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link LazyPySequenceIterSeachNode#doIt}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(LazyPySequenceIterSeachNode.class)
@SuppressWarnings("javadoc")
public static final class LazyPySequenceIterSeachNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static LazyPySequenceIterSeachNode getUncached() {
return LazyPySequenceIterSeachNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#node_}
*
*/
@NeverDefault
public static LazyPySequenceIterSeachNode inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new LazyPySequenceIterSeachNodeGen.Inlined(target);
}
@GeneratedBy(LazyPySequenceIterSeachNode.class)
@DenyReplace
private static final class Inlined extends LazyPySequenceIterSeachNode {
/**
* State Info:
* 0: SpecializationActive {@link LazyPySequenceIterSeachNode#doIt}
*
*/
private final StateField state_0_;
private final ReferenceField node_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(LazyPySequenceIterSeachNode.class);
this.state_0_ = target.getState(0, 1);
this.node_ = target.getReference(1, PySequenceIterSearchNode.class);
}
@Override
protected PySequenceIterSearchNode execute(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PySequenceIterSearchNode.LazyPySequenceIterSeachNode.doIt(PySequenceIterSearchNode)] */) {
{
PySequenceIterSearchNode node__ = this.node_.get(arg0Value);
if (node__ != null) {
return LazyPySequenceIterSeachNode.doIt(node__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private PySequenceIterSearchNode executeAndSpecialize(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
PySequenceIterSearchNode node__ = arg0Value.insert((PySequenceIterSearchNodeGen.create()));
Objects.requireNonNull(node__, "Specialization 'doIt(PySequenceIterSearchNode)' cache 'node' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.node_.set(arg0Value, node__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PySequenceIterSearchNode.LazyPySequenceIterSeachNode.doIt(PySequenceIterSearchNode)] */;
this.state_0_.set(arg0Value, state_0);
return LazyPySequenceIterSeachNode.doIt(node__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(LazyPySequenceIterSeachNode.class)
@DenyReplace
private static final class Uncached extends LazyPySequenceIterSeachNode {
@TruffleBoundary
@Override
protected PySequenceIterSearchNode execute(Node arg0Value) {
return LazyPySequenceIterSeachNode.doIt((PySequenceIterSearchNodeGen.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy