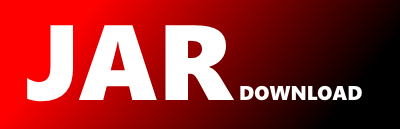
com.oracle.graal.python.lib.PySliceNewNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.PNone;
import com.oracle.graal.python.builtins.objects.ints.PInt;
import com.oracle.graal.python.builtins.objects.slice.PSlice;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link PySliceNew#doInt(int, int, int, PythonObjectFactory)}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link PySliceNew#doInt(PNone, int, PNone, PythonObjectFactory)}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link PySliceNew#doInt(PNone, int, int, PythonObjectFactory)}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link PySliceNew#doLong}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link PySliceNew#doGeneric}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(PySliceNew.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PySliceNewNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PySliceNew getUncached() {
return PySliceNewNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#factory}
*
*/
@NeverDefault
public static PySliceNew inline(@RequiredField(bits = 5, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PySliceNewNodeGen.Inlined(target);
}
@GeneratedBy(PySliceNew.class)
@DenyReplace
private static final class Inlined extends PySliceNew {
/**
* State Info:
* 0: SpecializationActive {@link PySliceNew#doInt(int, int, int, PythonObjectFactory)}
* 1: SpecializationActive {@link PySliceNew#doInt(PNone, int, PNone, PythonObjectFactory)}
* 2: SpecializationActive {@link PySliceNew#doInt(PNone, int, int, PythonObjectFactory)}
* 3: SpecializationActive {@link PySliceNew#doLong}
* 4: SpecializationActive {@link PySliceNew#doGeneric}
*
*/
private final StateField state_0_;
private final ReferenceField factory;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PySliceNew.class);
this.state_0_ = target.getState(0, 5);
this.factory = target.getReference(1, PythonObjectFactory.class);
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
if (arg2Value instanceof Integer) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[PySliceNew.doInt(int, int, int, PythonObjectFactory)] */) && arg1Value instanceof Integer && arg3Value instanceof Integer) {
return false;
}
if (arg1Value instanceof PNone) {
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[PySliceNew.doInt(PNone, int, PNone, PythonObjectFactory)] */) && arg3Value instanceof PNone) {
return false;
}
if (!((state_0 & 0b100) != 0 /* is SpecializationActive[PySliceNew.doInt(PNone, int, int, PythonObjectFactory)] */) && arg3Value instanceof Integer) {
return false;
}
}
}
if (arg1Value instanceof Long && arg2Value instanceof Long && arg3Value instanceof PNone) {
long arg1Value_ = (long) arg1Value;
if ((PInt.isIntRange(arg1Value_))) {
long arg2Value_ = (long) arg2Value;
if ((PInt.isIntRange(arg2Value_))) {
return false;
}
}
}
return true;
}
@Override
public PSlice execute(Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PySliceNew.doInt(int, int, int, PythonObjectFactory)] || SpecializationActive[PySliceNew.doInt(PNone, int, PNone, PythonObjectFactory)] || SpecializationActive[PySliceNew.doInt(PNone, int, int, PythonObjectFactory)] || SpecializationActive[PySliceNew.doLong(long, long, PNone, PythonObjectFactory)] || SpecializationActive[PySliceNew.doGeneric(Object, Object, Object, PythonObjectFactory)] */) {
if ((state_0 & 0b111) != 0 /* is SpecializationActive[PySliceNew.doInt(int, int, int, PythonObjectFactory)] || SpecializationActive[PySliceNew.doInt(PNone, int, PNone, PythonObjectFactory)] || SpecializationActive[PySliceNew.doInt(PNone, int, int, PythonObjectFactory)] */ && arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PySliceNew.doInt(int, int, int, PythonObjectFactory)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
{
PythonObjectFactory factory_ = this.factory.get(arg0Value);
if (factory_ != null) {
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, factory_);
}
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[PySliceNew.doInt(PNone, int, PNone, PythonObjectFactory)] || SpecializationActive[PySliceNew.doInt(PNone, int, int, PythonObjectFactory)] */ && arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PySliceNew.doInt(PNone, int, PNone, PythonObjectFactory)] */ && arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
{
PythonObjectFactory factory_1 = this.factory.get(arg0Value);
if (factory_1 != null) {
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, factory_1);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PySliceNew.doInt(PNone, int, int, PythonObjectFactory)] */ && arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
{
PythonObjectFactory factory_2 = this.factory.get(arg0Value);
if (factory_2 != null) {
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, factory_2);
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PySliceNew.doLong(long, long, PNone, PythonObjectFactory)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
{
PythonObjectFactory factory_3 = this.factory.get(arg0Value);
if (factory_3 != null) {
if ((PInt.isIntRange(arg1Value_)) && (PInt.isIntRange(arg2Value_))) {
return PySliceNew.doLong(arg1Value_, arg2Value_, arg3Value_, factory_3);
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PySliceNew.doGeneric(Object, Object, Object, PythonObjectFactory)] */) {
{
PythonObjectFactory factory_4 = this.factory.get(arg0Value);
if (factory_4 != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value, arg2Value, arg3Value)) {
return PySliceNew.doGeneric(arg1Value, arg2Value, arg3Value, factory_4);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private PSlice executeAndSpecialize(Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory.get(arg0Value);
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = arg0Value.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'doInt(int, int, int, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.factory.set(arg0Value, factory_);
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PySliceNew.doInt(int, int, int, PythonObjectFactory)] */;
this.state_0_.set(arg0Value, state_0);
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, factory_);
}
}
if (arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory.get(arg0Value);
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = arg0Value.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'doInt(PNone, int, PNone, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.factory.set(arg0Value, factory_1);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PySliceNew.doInt(PNone, int, PNone, PythonObjectFactory)] */;
this.state_0_.set(arg0Value, state_0);
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, factory_1);
}
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
PythonObjectFactory factory_2;
PythonObjectFactory factory_2_shared = this.factory.get(arg0Value);
if (factory_2_shared != null) {
factory_2 = factory_2_shared;
} else {
factory_2 = arg0Value.insert((PythonObjectFactory.create()));
if (factory_2 == null) {
throw new IllegalStateException("Specialization 'doInt(PNone, int, int, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.factory.set(arg0Value, factory_2);
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PySliceNew.doInt(PNone, int, int, PythonObjectFactory)] */;
this.state_0_.set(arg0Value, state_0);
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, factory_2);
}
}
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
if ((PInt.isIntRange(arg1Value_)) && (PInt.isIntRange(arg2Value_))) {
PythonObjectFactory factory_3;
PythonObjectFactory factory_3_shared = this.factory.get(arg0Value);
if (factory_3_shared != null) {
factory_3 = factory_3_shared;
} else {
factory_3 = arg0Value.insert((PythonObjectFactory.create()));
if (factory_3 == null) {
throw new IllegalStateException("Specialization 'doLong(long, long, PNone, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.factory.set(arg0Value, factory_3);
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[PySliceNew.doLong(long, long, PNone, PythonObjectFactory)] */;
this.state_0_.set(arg0Value, state_0);
return PySliceNew.doLong(arg1Value_, arg2Value_, arg3Value_, factory_3);
}
}
}
}
PythonObjectFactory factory_4;
PythonObjectFactory factory_4_shared = this.factory.get(arg0Value);
if (factory_4_shared != null) {
factory_4 = factory_4_shared;
} else {
factory_4 = arg0Value.insert((PythonObjectFactory.create()));
if (factory_4 == null) {
throw new IllegalStateException("Specialization 'doGeneric(Object, Object, Object, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.factory.set(arg0Value, factory_4);
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[PySliceNew.doGeneric(Object, Object, Object, PythonObjectFactory)] */;
this.state_0_.set(arg0Value, state_0);
return PySliceNew.doGeneric(arg1Value, arg2Value, arg3Value, factory_4);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PySliceNew.class)
@DenyReplace
private static final class Uncached extends PySliceNew {
@TruffleBoundary
@Override
public PSlice execute(Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
if (arg2Value instanceof Integer) {
int arg2Value_ = (int) arg2Value;
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, (PythonObjectFactory.getUncached()));
}
}
if (arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, (PythonObjectFactory.getUncached()));
}
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
return PySliceNew.doInt(arg1Value_, arg2Value_, arg3Value_, (PythonObjectFactory.getUncached()));
}
}
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
if (arg2Value instanceof Long) {
long arg2Value_ = (long) arg2Value;
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
if ((PInt.isIntRange(arg1Value_)) && (PInt.isIntRange(arg2Value_))) {
return PySliceNew.doLong(arg1Value_, arg2Value_, arg3Value_, (PythonObjectFactory.getUncached()));
}
}
}
}
return PySliceNew.doGeneric(arg1Value, arg2Value, arg3Value, (PythonObjectFactory.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy