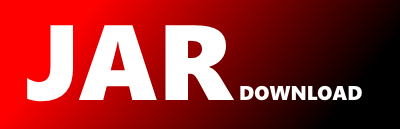
com.oracle.graal.python.lib.PyTupleCheckNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.tuple.PTuple;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyTupleCheckNode#doManaged}
* Activation probability: 0.65000
* With/without class size: 11/0 bytes
* Specialization {@link PyTupleCheckNode#doGeneric}
* Activation probability: 0.35000
* With/without class size: 13/11 bytes
*
*/
@GeneratedBy(PyTupleCheckNode.class)
@SuppressWarnings("javadoc")
public final class PyTupleCheckNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static PyTupleCheckNode getUncached() {
return PyTupleCheckNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#generic_getClassNode__field1_}
*
- {@link Inlined#generic_isSubtypeNode_}
*
*/
@NeverDefault
public static PyTupleCheckNode inline(@RequiredField(bits = 19, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyTupleCheckNodeGen.Inlined(target);
}
@GeneratedBy(PyTupleCheckNode.class)
@DenyReplace
private static final class Inlined extends PyTupleCheckNode {
/**
* State Info:
* 0: SpecializationActive {@link PyTupleCheckNode#doManaged}
* 1: SpecializationActive {@link PyTupleCheckNode#doGeneric}
* 2-18: InlinedCache
* Specialization: {@link PyTupleCheckNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField generic_getClassNode__field1_;
private final ReferenceField generic_isSubtypeNode_;
/**
* Source Info:
* Specialization: {@link PyTupleCheckNode#doGeneric}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode generic_getClassNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyTupleCheckNode.class);
this.state_0_ = target.getState(0, 19);
this.generic_getClassNode__field1_ = target.getReference(1, Node.class);
this.generic_isSubtypeNode_ = target.getReference(2, IsSubtypeNode.class);
this.generic_getClassNode_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, state_0_.subUpdater(2, 17), generic_getClassNode__field1_));
}
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b11) != 0 /* is SpecializationActive[PyTupleCheckNode.doManaged(PTuple)] || SpecializationActive[PyTupleCheckNode.doGeneric(Node, Object, GetClassNode, IsSubtypeNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyTupleCheckNode.doManaged(PTuple)] */ && arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
return PyTupleCheckNode.doManaged(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyTupleCheckNode.doGeneric(Node, Object, GetClassNode, IsSubtypeNode)] */) {
{
IsSubtypeNode isSubtypeNode__ = this.generic_isSubtypeNode_.get(arg0Value);
if (isSubtypeNode__ != null) {
assert InlineSupport.validate(arg0Value, this.state_0_, this.generic_getClassNode__field1_);
return PyTupleCheckNode.doGeneric(arg0Value, arg1Value, this.generic_getClassNode_, isSubtypeNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyTupleCheckNode.doManaged(PTuple)] */;
this.state_0_.set(arg0Value, state_0);
return PyTupleCheckNode.doManaged(arg1Value_);
}
IsSubtypeNode isSubtypeNode__ = arg0Value.insert((IsSubtypeNode.create()));
Objects.requireNonNull(isSubtypeNode__, "Specialization 'doGeneric(Node, Object, GetClassNode, IsSubtypeNode)' cache 'isSubtypeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.generic_isSubtypeNode_.set(arg0Value, isSubtypeNode__);
state_0 = state_0 | 0b10 /* add SpecializationActive[PyTupleCheckNode.doGeneric(Node, Object, GetClassNode, IsSubtypeNode)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.generic_getClassNode__field1_);
return PyTupleCheckNode.doGeneric(arg0Value, arg1Value, this.generic_getClassNode_, isSubtypeNode__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyTupleCheckNode.class)
@DenyReplace
private static final class Uncached extends PyTupleCheckNode {
@TruffleBoundary
@Override
public boolean execute(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof PTuple) {
PTuple arg1Value_ = (PTuple) arg1Value;
return PyTupleCheckNode.doManaged(arg1Value_);
}
return PyTupleCheckNode.doGeneric(arg0Value, arg1Value, (GetClassNode.getUncached()), (IsSubtypeNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy