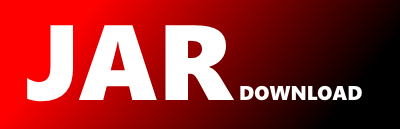
com.oracle.graal.python.lib.PyUnicodeFSDecoderNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.buffer.PythonBufferAccessLibrary;
import com.oracle.graal.python.builtins.objects.bytes.PBytes;
import com.oracle.graal.python.builtins.objects.str.PString;
import com.oracle.graal.python.nodes.util.CastToTruffleStringNode;
import com.oracle.graal.python.nodes.util.CastToTruffleStringNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.ByteIndexOfCodePointNode;
import com.oracle.truffle.api.strings.TruffleString.FromByteArrayNode;
import com.oracle.truffle.api.strings.TruffleString.SwitchEncodingNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyUnicodeFSDecoderNode#doString}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link PyUnicodeFSDecoderNode#doPString}
* Activation probability: 0.26000
* With/without class size: 12/13 bytes
* Specialization {@link PyUnicodeFSDecoderNode#doBytes}
* Activation probability: 0.20000
* With/without class size: 9/12 bytes
* Specialization {@link PyUnicodeFSDecoderNode#doBytes}
* Activation probability: 0.14000
* With/without class size: 7/8 bytes
* Specialization {@link PyUnicodeFSDecoderNode#doPathLike}
* Activation probability: 0.08000
* With/without class size: 6/9 bytes
*
*/
@GeneratedBy(PyUnicodeFSDecoderNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyUnicodeFSDecoderNodeGen extends PyUnicodeFSDecoderNode {
private static final StateField P_STRING__PY_UNICODE_F_S_DECODER_NODE_P_STRING_STATE_0_UPDATER = StateField.create(PStringData.lookup_(), "pString_state_0_");
private static final StateField FALLBACK__PY_UNICODE_F_S_DECODER_NODE_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
static final ReferenceField BYTES0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "bytes0_cache", Bytes0Data.class);
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPString}
* Parameter: {@link CastToTruffleStringNode} cast
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_P_STRING_CAST_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, P_STRING__PY_UNICODE_F_S_DECODER_NODE_P_STRING_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(PStringData.lookup_(), "pString_cast__field1_", Node.class), ReferenceField.create(PStringData.lookup_(), "pString_cast__field2_", Node.class), ReferenceField.create(PStringData.lookup_(), "pString_cast__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPathLike}
* Parameter: {@link PyOSFSPathNode} fspathNode
* Inline method: {@link PyOSFSPathNodeGen#inline}
*/
private static final PyOSFSPathNode INLINED_FALLBACK_FSPATH_NODE_ = PyOSFSPathNodeGen.inline(InlineTarget.create(PyOSFSPathNode.class, FALLBACK__PY_UNICODE_F_S_DECODER_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(0, 4), ReferenceField.create(FallbackData.lookup_(), "fallback_fspathNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory PYTHON_BUFFER_ACCESS_LIBRARY_ = LibraryFactory.resolve(PythonBufferAccessLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link PyUnicodeFSDecoderNode#doString}
* 1: SpecializationActive {@link PyUnicodeFSDecoderNode#doPString}
* 2: SpecializationActive {@link PyUnicodeFSDecoderNode#doBytes}
* 3: SpecializationActive {@link PyUnicodeFSDecoderNode#doBytes}
* 4: SpecializationActive {@link PyUnicodeFSDecoderNode#doPathLike}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doString}
* Parameter: {@link ByteIndexOfCodePointNode} byteIndexOfCodePointNode
*/
@Child private ByteIndexOfCodePointNode byteIndexOfCP;
@Child private PStringData pString_cache;
@UnsafeAccessedField @Child private Bytes0Data bytes0_cache;
@Child private Bytes1Data bytes1_cache;
@Child private FallbackData fallback_cache;
private PyUnicodeFSDecoderNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doString(TruffleString, ByteIndexOfCodePointNode)] */) && arg0Value instanceof TruffleString) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doPString(PString, Node, CastToTruffleStringNode, ByteIndexOfCodePointNode)] */) && arg0Value instanceof PString) {
return false;
}
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */) && arg0Value instanceof PBytes) {
return false;
}
return true;
}
@Override
public TruffleString execute(Frame frameValue, Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doString(TruffleString, ByteIndexOfCodePointNode)] || SpecializationActive[PyUnicodeFSDecoderNode.doPString(PString, Node, CastToTruffleStringNode, ByteIndexOfCodePointNode)] || SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] || SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] || SpecializationActive[PyUnicodeFSDecoderNode.doPathLike(VirtualFrame, Object, Node, PyOSFSPathNode, PyUnicodeFSDecoderNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doString(TruffleString, ByteIndexOfCodePointNode)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
{
ByteIndexOfCodePointNode byteIndexOfCP_ = this.byteIndexOfCP;
if (byteIndexOfCP_ != null) {
return doString(arg0Value_, byteIndexOfCP_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doPString(PString, Node, CastToTruffleStringNode, ByteIndexOfCodePointNode)] */ && arg0Value instanceof PString) {
PString arg0Value_ = (PString) arg0Value;
PStringData s1_ = this.pString_cache;
if (s1_ != null) {
{
ByteIndexOfCodePointNode byteIndexOfCP_1 = this.byteIndexOfCP;
if (byteIndexOfCP_1 != null) {
Node inliningTarget__ = (s1_);
return doPString(arg0Value_, inliningTarget__, INLINED_P_STRING_CAST_, byteIndexOfCP_1);
}
}
}
}
if ((state_0 & 0b1100) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] || SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */ && arg0Value instanceof PBytes) {
PBytes arg0Value_ = (PBytes) arg0Value;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */) {
Bytes0Data s2_ = this.bytes0_cache;
if (s2_ != null) {
{
ByteIndexOfCodePointNode byteIndexOfCP_2 = this.byteIndexOfCP;
if (byteIndexOfCP_2 != null) {
if ((s2_.bufferLib_.accepts(arg0Value_))) {
return doBytes(arg0Value_, s2_.bufferLib_, s2_.fromByteArrayNode_, s2_.switchEncodingNode_, byteIndexOfCP_2);
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */) {
Bytes1Data s3_ = this.bytes1_cache;
if (s3_ != null) {
{
ByteIndexOfCodePointNode byteIndexOfCP_2 = this.byteIndexOfCP;
if (byteIndexOfCP_2 != null) {
return this.bytes1Boundary(state_0, s3_, arg0Value_, byteIndexOfCP_2);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyUnicodeFSDecoderNode.doPathLike(VirtualFrame, Object, Node, PyOSFSPathNode, PyUnicodeFSDecoderNode)] */) {
FallbackData s4_ = this.fallback_cache;
if (s4_ != null) {
{
Node inliningTarget__1 = (s4_);
if (fallbackGuard_(state_0, arg0Value)) {
return doPathLike((VirtualFrame) frameValue, arg0Value, inliningTarget__1, INLINED_FALLBACK_FSPATH_NODE_, s4_.recursive_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private TruffleString bytes1Boundary(int state_0, Bytes1Data s3_, PBytes arg0Value_, ByteIndexOfCodePointNode byteIndexOfCP_2) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
PythonBufferAccessLibrary bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached(arg0Value_));
return doBytes(arg0Value_, bufferLib__, s3_.fromByteArrayNode_, s3_.switchEncodingNode_, byteIndexOfCP_2);
}
} finally {
encapsulating_.set(prev_);
}
}
private TruffleString executeAndSpecialize(Frame frameValue, Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
ByteIndexOfCodePointNode byteIndexOfCP_;
ByteIndexOfCodePointNode byteIndexOfCP__shared = this.byteIndexOfCP;
if (byteIndexOfCP__shared != null) {
byteIndexOfCP_ = byteIndexOfCP__shared;
} else {
byteIndexOfCP_ = this.insert((ByteIndexOfCodePointNode.create()));
if (byteIndexOfCP_ == null) {
throw new IllegalStateException("Specialization 'doString(TruffleString, ByteIndexOfCodePointNode)' contains a shared cache with name 'byteIndexOfCodePointNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.byteIndexOfCP == null) {
VarHandle.storeStoreFence();
this.byteIndexOfCP = byteIndexOfCP_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyUnicodeFSDecoderNode.doString(TruffleString, ByteIndexOfCodePointNode)] */;
this.state_0_ = state_0;
return doString(arg0Value_, byteIndexOfCP_);
}
{
Node inliningTarget__ = null;
if (arg0Value instanceof PString) {
PString arg0Value_ = (PString) arg0Value;
PStringData s1_ = this.insert(new PStringData());
inliningTarget__ = (s1_);
ByteIndexOfCodePointNode byteIndexOfCP_1;
ByteIndexOfCodePointNode byteIndexOfCP_1_shared = this.byteIndexOfCP;
if (byteIndexOfCP_1_shared != null) {
byteIndexOfCP_1 = byteIndexOfCP_1_shared;
} else {
byteIndexOfCP_1 = s1_.insert((ByteIndexOfCodePointNode.create()));
if (byteIndexOfCP_1 == null) {
throw new IllegalStateException("Specialization 'doPString(PString, Node, CastToTruffleStringNode, ByteIndexOfCodePointNode)' contains a shared cache with name 'byteIndexOfCodePointNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.byteIndexOfCP == null) {
this.byteIndexOfCP = byteIndexOfCP_1;
}
VarHandle.storeStoreFence();
this.pString_cache = s1_;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyUnicodeFSDecoderNode.doPString(PString, Node, CastToTruffleStringNode, ByteIndexOfCodePointNode)] */;
this.state_0_ = state_0;
return doPString(arg0Value_, inliningTarget__, INLINED_P_STRING_CAST_, byteIndexOfCP_1);
}
}
if (arg0Value instanceof PBytes) {
PBytes arg0Value_ = (PBytes) arg0Value;
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */) {
while (true) {
int count2_ = 0;
Bytes0Data s2_ = BYTES0_CACHE_UPDATER.getVolatile(this);
Bytes0Data s2_original = s2_;
while (s2_ != null) {
{
ByteIndexOfCodePointNode byteIndexOfCP_2 = this.byteIndexOfCP;
if (byteIndexOfCP_2 != null) {
if ((s2_.bufferLib_.accepts(arg0Value_))) {
break;
}
}
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
// assert (s2_.bufferLib_.accepts(arg0Value_));
s2_ = this.insert(new Bytes0Data());
PythonBufferAccessLibrary bufferLib__ = s2_.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.create(arg0Value_)));
Objects.requireNonNull(bufferLib__, "Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.bufferLib_ = bufferLib__;
FromByteArrayNode fromByteArrayNode__ = s2_.insert((FromByteArrayNode.create()));
Objects.requireNonNull(fromByteArrayNode__, "Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' cache 'fromByteArrayNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.fromByteArrayNode_ = fromByteArrayNode__;
SwitchEncodingNode switchEncodingNode__ = s2_.insert((SwitchEncodingNode.create()));
Objects.requireNonNull(switchEncodingNode__, "Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' cache 'switchEncodingNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.switchEncodingNode_ = switchEncodingNode__;
ByteIndexOfCodePointNode byteIndexOfCP_2;
ByteIndexOfCodePointNode byteIndexOfCP_2_shared = this.byteIndexOfCP;
if (byteIndexOfCP_2_shared != null) {
byteIndexOfCP_2 = byteIndexOfCP_2_shared;
} else {
byteIndexOfCP_2 = s2_.insert((ByteIndexOfCodePointNode.create()));
if (byteIndexOfCP_2 == null) {
throw new IllegalStateException("Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' contains a shared cache with name 'byteIndexOfCodePointNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.byteIndexOfCP == null) {
this.byteIndexOfCP = byteIndexOfCP_2;
}
if (!BYTES0_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */;
this.state_0_ = state_0;
}
if (s2_ != null) {
return doBytes(arg0Value_, s2_.bufferLib_, s2_.fromByteArrayNode_, s2_.switchEncodingNode_, this.byteIndexOfCP);
}
break;
}
}
{
PythonBufferAccessLibrary bufferLib__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
Bytes1Data s3_ = this.insert(new Bytes1Data());
bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached(arg0Value_));
FromByteArrayNode fromByteArrayNode__ = s3_.insert((FromByteArrayNode.create()));
Objects.requireNonNull(fromByteArrayNode__, "Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' cache 'fromByteArrayNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.fromByteArrayNode_ = fromByteArrayNode__;
SwitchEncodingNode switchEncodingNode__ = s3_.insert((SwitchEncodingNode.create()));
Objects.requireNonNull(switchEncodingNode__, "Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' cache 'switchEncodingNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.switchEncodingNode_ = switchEncodingNode__;
ByteIndexOfCodePointNode byteIndexOfCP_2;
ByteIndexOfCodePointNode byteIndexOfCP_2_shared = this.byteIndexOfCP;
if (byteIndexOfCP_2_shared != null) {
byteIndexOfCP_2 = byteIndexOfCP_2_shared;
} else {
byteIndexOfCP_2 = s3_.insert((ByteIndexOfCodePointNode.create()));
if (byteIndexOfCP_2 == null) {
throw new IllegalStateException("Specialization 'doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)' contains a shared cache with name 'byteIndexOfCodePointNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.byteIndexOfCP == null) {
this.byteIndexOfCP = byteIndexOfCP_2;
}
VarHandle.storeStoreFence();
this.bytes1_cache = s3_;
this.bytes0_cache = null;
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyUnicodeFSDecoderNode.doBytes(PBytes, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode, ByteIndexOfCodePointNode)] */;
this.state_0_ = state_0;
return doBytes(arg0Value_, bufferLib__, fromByteArrayNode__, switchEncodingNode__, byteIndexOfCP_2);
} finally {
encapsulating_.set(prev_);
}
}
}
}
{
Node inliningTarget__1 = null;
FallbackData s4_ = this.insert(new FallbackData());
inliningTarget__1 = (s4_);
PyUnicodeFSDecoderNode recursive__ = s4_.insert((PyUnicodeFSDecoderNodeGen.create()));
Objects.requireNonNull(recursive__, "Specialization 'doPathLike(VirtualFrame, Object, Node, PyOSFSPathNode, PyUnicodeFSDecoderNode)' cache 'recursive' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.recursive_ = recursive__;
VarHandle.storeStoreFence();
this.fallback_cache = s4_;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyUnicodeFSDecoderNode.doPathLike(VirtualFrame, Object, Node, PyOSFSPathNode, PyUnicodeFSDecoderNode)] */;
this.state_0_ = state_0;
return doPathLike((VirtualFrame) frameValue, arg0Value, inliningTarget__1, INLINED_FALLBACK_FSPATH_NODE_, recursive__);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyUnicodeFSDecoderNode create() {
return new PyUnicodeFSDecoderNodeGen();
}
@NeverDefault
public static PyUnicodeFSDecoderNode getUncached() {
return PyUnicodeFSDecoderNodeGen.UNCACHED;
}
@GeneratedBy(PyUnicodeFSDecoderNode.class)
@DenyReplace
private static final class PStringData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link PyUnicodeFSDecoderNode#doPString}
* Parameter: {@link CastToTruffleStringNode} cast
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int pString_state_0_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPString}
* Parameter: {@link CastToTruffleStringNode} cast
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pString_cast__field1_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPString}
* Parameter: {@link CastToTruffleStringNode} cast
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pString_cast__field2_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPString}
* Parameter: {@link CastToTruffleStringNode} cast
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node pString_cast__field3_;
PStringData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyUnicodeFSDecoderNode.class)
@DenyReplace
private static final class Bytes0Data extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doBytes}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child PythonBufferAccessLibrary bufferLib_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doBytes}
* Parameter: {@link FromByteArrayNode} fromByteArrayNode
*/
@Child FromByteArrayNode fromByteArrayNode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doBytes}
* Parameter: {@link SwitchEncodingNode} switchEncodingNode
*/
@Child SwitchEncodingNode switchEncodingNode_;
Bytes0Data() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(PyUnicodeFSDecoderNode.class)
@DenyReplace
private static final class Bytes1Data extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doBytes}
* Parameter: {@link FromByteArrayNode} fromByteArrayNode
*/
@Child FromByteArrayNode fromByteArrayNode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doBytes}
* Parameter: {@link SwitchEncodingNode} switchEncodingNode
*/
@Child SwitchEncodingNode switchEncodingNode_;
Bytes1Data() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(PyUnicodeFSDecoderNode.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-3: InlinedCache
* Specialization: {@link PyUnicodeFSDecoderNode#doPathLike}
* Parameter: {@link PyOSFSPathNode} fspathNode
* Inline method: {@link PyOSFSPathNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPathLike}
* Parameter: {@link PyOSFSPathNode} fspathNode
* Inline method: {@link PyOSFSPathNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_fspathNode__field1_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFSDecoderNode#doPathLike}
* Parameter: {@link PyUnicodeFSDecoderNode} recursive
*/
@Child PyUnicodeFSDecoderNode recursive_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyUnicodeFSDecoderNode.class)
@DenyReplace
private static final class Uncached extends PyUnicodeFSDecoderNode {
@Override
public TruffleString execute(Frame frameValue, Object arg0Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
return doString(arg0Value_, (ByteIndexOfCodePointNode.getUncached()));
}
if (arg0Value instanceof PString) {
PString arg0Value_ = (PString) arg0Value;
return doPString(arg0Value_, (this), (CastToTruffleStringNode.getUncached()), (ByteIndexOfCodePointNode.getUncached()));
}
if (arg0Value instanceof PBytes) {
PBytes arg0Value_ = (PBytes) arg0Value;
return doBytes(arg0Value_, (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached(arg0Value_)), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()), (ByteIndexOfCodePointNode.getUncached()));
}
return doPathLike((VirtualFrame) frameValue, arg0Value, (this), (PyOSFSPathNodeGen.getUncached()), (PyUnicodeFSDecoderNodeGen.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy