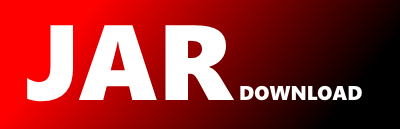
com.oracle.graal.python.lib.PyUnicodeFromEncodedObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.buffer.PythonBufferAccessLibrary;
import com.oracle.graal.python.builtins.objects.buffer.PythonBufferAcquireLibrary;
import com.oracle.graal.python.builtins.objects.bytes.PBytes;
import com.oracle.graal.python.builtins.objects.str.PString;
import com.oracle.graal.python.nodes.IndirectCallData;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromByteArrayNode;
import com.oracle.truffle.api.strings.TruffleString.SwitchEncodingNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyUnicodeFromEncodedObject#doBytes}
* Activation probability: 0.32000
* With/without class size: 14/13 bytes
* Specialization {@link PyUnicodeFromEncodedObject#doString}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link PyUnicodeFromEncodedObject#doPString}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link PyUnicodeFromEncodedObject#doBuffer}
* Activation probability: 0.14000
* With/without class size: 11/33 bytes
* Specialization {@link PyUnicodeFromEncodedObject#doBuffer}
* Activation probability: 0.08000
* With/without class size: 7/25 bytes
*
*/
@GeneratedBy(PyUnicodeFromEncodedObject.class)
@SuppressWarnings("javadoc")
public final class PyUnicodeFromEncodedObjectNodeGen {
private static final StateField BUFFER0__PY_UNICODE_FROM_ENCODED_OBJECT_BUFFER0_STATE_0_UPDATER = StateField.create(Buffer0Data.lookup_(), "buffer0_state_0_");
private static final StateField BUFFER1__PY_UNICODE_FROM_ENCODED_OBJECT_BUFFER1_STATE_0_UPDATER = StateField.create(Buffer1Data.lookup_(), "buffer1_state_0_");
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory PYTHON_BUFFER_ACQUIRE_LIBRARY_ = LibraryFactory.resolve(PythonBufferAcquireLibrary.class);
private static final LibraryFactory PYTHON_BUFFER_ACCESS_LIBRARY_ = LibraryFactory.resolve(PythonBufferAccessLibrary.class);
@NeverDefault
public static PyUnicodeFromEncodedObject getUncached() {
return PyUnicodeFromEncodedObjectNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#raiseNode}
*
- {@link Inlined#bytes_decode__field1_}
*
- {@link Inlined#bytes_decode__field2_}
*
- {@link Inlined#bytes_decode__field3_}
*
- {@link Inlined#buffer0_cache}
*
- {@link Inlined#buffer1_cache}
*
*/
@NeverDefault
public static PyUnicodeFromEncodedObject inline(@RequiredField(bits = 10, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyUnicodeFromEncodedObjectNodeGen.Inlined(target);
}
@GeneratedBy(PyUnicodeFromEncodedObject.class)
@DenyReplace
private static final class Inlined extends PyUnicodeFromEncodedObject {
/**
* State Info:
* 0: SpecializationActive {@link PyUnicodeFromEncodedObject#doBytes}
* 1: SpecializationActive {@link PyUnicodeFromEncodedObject#doString}
* 2: SpecializationActive {@link PyUnicodeFromEncodedObject#doPString}
* 3: SpecializationActive {@link PyUnicodeFromEncodedObject#doBuffer}
* 4: SpecializationActive {@link PyUnicodeFromEncodedObject#doBuffer}
* 5-6: InlinedCache
* Specialization: {@link PyUnicodeFromEncodedObject#doBytes}
* Parameter: {@link InlinedConditionProfile} emptyStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 7-9: InlinedCache
* Specialization: {@link PyUnicodeFromEncodedObject#doBytes}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField raiseNode;
private final ReferenceField bytes_decode__field1_;
private final ReferenceField bytes_decode__field2_;
private final ReferenceField bytes_decode__field3_;
private final ReferenceField buffer0_cache;
private final ReferenceField buffer1_cache;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBytes}
* Parameter: {@link InlinedConditionProfile} emptyStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile bytes_emptyStringProfile_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBytes}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
*/
private final PyUnicodeDecode bytes_decode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link InlinedConditionProfile} emptyStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile buffer0_emptyStringProfile_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
*/
private final PyUnicodeDecode buffer0_decode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link InlinedConditionProfile} emptyStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile buffer1_emptyStringProfile_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
*/
private final PyUnicodeDecode buffer1_decode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyUnicodeFromEncodedObject.class);
this.state_0_ = target.getState(0, 10);
this.raiseNode = target.getReference(1, PRaiseNode.class);
this.bytes_decode__field1_ = target.getReference(2, Node.class);
this.bytes_decode__field2_ = target.getReference(3, Node.class);
this.bytes_decode__field3_ = target.getReference(4, Node.class);
this.buffer0_cache = target.getReference(5, Buffer0Data.class);
this.buffer1_cache = target.getReference(6, Buffer1Data.class);
this.bytes_emptyStringProfile_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, state_0_.subUpdater(5, 2)));
this.bytes_decode_ = PyUnicodeDecodeNodeGen.inline(InlineTarget.create(PyUnicodeDecode.class, state_0_.subUpdater(7, 3), bytes_decode__field1_, bytes_decode__field2_, bytes_decode__field3_));
this.buffer0_emptyStringProfile_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUFFER0__PY_UNICODE_FROM_ENCODED_OBJECT_BUFFER0_STATE_0_UPDATER.subUpdater(0, 2)));
this.buffer0_decode_ = PyUnicodeDecodeNodeGen.inline(InlineTarget.create(PyUnicodeDecode.class, BUFFER0__PY_UNICODE_FROM_ENCODED_OBJECT_BUFFER0_STATE_0_UPDATER.subUpdater(2, 3), ReferenceField.create(Buffer0Data.lookup_(), "buffer0_decode__field1_", Node.class), ReferenceField.create(Buffer0Data.lookup_(), "buffer0_decode__field2_", Node.class), ReferenceField.create(Buffer0Data.lookup_(), "buffer0_decode__field3_", Node.class)));
this.buffer1_emptyStringProfile_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUFFER1__PY_UNICODE_FROM_ENCODED_OBJECT_BUFFER1_STATE_0_UPDATER.subUpdater(0, 2)));
this.buffer1_decode_ = PyUnicodeDecodeNodeGen.inline(InlineTarget.create(PyUnicodeDecode.class, BUFFER1__PY_UNICODE_FROM_ENCODED_OBJECT_BUFFER1_STATE_0_UPDATER.subUpdater(2, 3), ReferenceField.create(Buffer1Data.lookup_(), "buffer1_decode__field1_", Node.class), ReferenceField.create(Buffer1Data.lookup_(), "buffer1_decode__field2_", Node.class), ReferenceField.create(Buffer1Data.lookup_(), "buffer1_decode__field3_", Node.class)));
}
@ExplodeLoop
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doBytes(VirtualFrame, Node, PBytes, Object, Object, InlinedConditionProfile, PyUnicodeDecode)] || SpecializationActive[PyUnicodeFromEncodedObject.doString(VirtualFrame, TruffleString, Object, Object, PRaiseNode)] || SpecializationActive[PyUnicodeFromEncodedObject.doPString(VirtualFrame, PString, Object, Object, PRaiseNode)] || SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] || SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doBytes(VirtualFrame, Node, PBytes, Object, Object, InlinedConditionProfile, PyUnicodeDecode)] */ && arg1Value instanceof PBytes) {
PBytes arg1Value_ = (PBytes) arg1Value;
assert InlineSupport.validate(arg0Value, this.state_0_, this.state_0_, this.bytes_decode__field1_, this.bytes_decode__field2_, this.bytes_decode__field3_);
return PyUnicodeFromEncodedObject.doBytes((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, this.bytes_emptyStringProfile_, this.bytes_decode_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doString(VirtualFrame, TruffleString, Object, Object, PRaiseNode)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
{
PRaiseNode raiseNode_ = this.raiseNode.get(arg0Value);
if (raiseNode_ != null) {
return PyUnicodeFromEncodedObject.doString((VirtualFrame) frameValue, arg1Value_, arg2Value, arg3Value, raiseNode_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doPString(VirtualFrame, PString, Object, Object, PRaiseNode)] */ && arg1Value instanceof PString) {
PString arg1Value_ = (PString) arg1Value;
{
PRaiseNode raiseNode_1 = this.raiseNode.get(arg0Value);
if (raiseNode_1 != null) {
return PyUnicodeFromEncodedObject.doPString((VirtualFrame) frameValue, arg1Value_, arg2Value, arg3Value, raiseNode_1);
}
}
}
if ((state_0 & 0b11000) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] || SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */) {
Buffer0Data s3_ = this.buffer0_cache.get(arg0Value);
while (s3_ != null) {
if ((s3_.bufferAcquireLib_.accepts(arg1Value)) && (s3_.bufferLib_.accepts(arg1Value)) && (!(PGuards.isPBytes(arg1Value))) && (!(PGuards.isString(arg1Value)))) {
return PyUnicodeFromEncodedObject.doBuffer((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, arg3Value, s3_.indirectCallNode_, this.buffer0_emptyStringProfile_, s3_.bufferAcquireLib_, this.buffer0_decode_, s3_.bufferLib_, s3_.fromByteArrayNode_, s3_.switchEncodingNode_);
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */) {
Buffer1Data s4_ = this.buffer1_cache.get(arg0Value);
if (s4_ != null) {
if ((!(PGuards.isPBytes(arg1Value))) && (!(PGuards.isString(arg1Value)))) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(arg0Value);
try {
{
PythonBufferAcquireLibrary bufferAcquireLib__ = (PYTHON_BUFFER_ACQUIRE_LIBRARY_.getUncached());
PythonBufferAccessLibrary bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached());
return PyUnicodeFromEncodedObject.doBuffer((VirtualFrame) frameValue, s4_, arg1Value, arg2Value, arg3Value, s4_.indirectCallNode_, this.buffer1_emptyStringProfile_, bufferAcquireLib__, this.buffer1_decode_, bufferLib__, s4_.fromByteArrayNode_, s4_.switchEncodingNode_);
}
} finally {
encapsulating_.set(prev_);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof PBytes) {
PBytes arg1Value_ = (PBytes) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[PyUnicodeFromEncodedObject.doBytes(VirtualFrame, Node, PBytes, Object, Object, InlinedConditionProfile, PyUnicodeDecode)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.state_0_, this.bytes_decode__field1_, this.bytes_decode__field2_, this.bytes_decode__field3_);
return PyUnicodeFromEncodedObject.doBytes((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, this.bytes_emptyStringProfile_, this.bytes_decode_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
PRaiseNode raiseNode_;
PRaiseNode raiseNode__shared = this.raiseNode.get(arg0Value);
if (raiseNode__shared != null) {
raiseNode_ = raiseNode__shared;
} else {
raiseNode_ = arg0Value.insert((PRaiseNode.create()));
if (raiseNode_ == null) {
throw new IllegalStateException("Specialization 'doString(VirtualFrame, TruffleString, Object, Object, PRaiseNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.raiseNode.set(arg0Value, raiseNode_);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PyUnicodeFromEncodedObject.doString(VirtualFrame, TruffleString, Object, Object, PRaiseNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyUnicodeFromEncodedObject.doString((VirtualFrame) frameValue, arg1Value_, arg2Value, arg3Value, raiseNode_);
}
if (arg1Value instanceof PString) {
PString arg1Value_ = (PString) arg1Value;
PRaiseNode raiseNode_1;
PRaiseNode raiseNode_1_shared = this.raiseNode.get(arg0Value);
if (raiseNode_1_shared != null) {
raiseNode_1 = raiseNode_1_shared;
} else {
raiseNode_1 = arg0Value.insert((PRaiseNode.create()));
if (raiseNode_1 == null) {
throw new IllegalStateException("Specialization 'doPString(VirtualFrame, PString, Object, Object, PRaiseNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.raiseNode.set(arg0Value, raiseNode_1);
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyUnicodeFromEncodedObject.doPString(VirtualFrame, PString, Object, Object, PRaiseNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyUnicodeFromEncodedObject.doPString((VirtualFrame) frameValue, arg1Value_, arg2Value, arg3Value, raiseNode_1);
}
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */) {
while (true) {
int count3_ = 0;
Buffer0Data s3_ = this.buffer0_cache.getVolatile(arg0Value);
Buffer0Data s3_original = s3_;
while (s3_ != null) {
if ((s3_.bufferAcquireLib_.accepts(arg1Value)) && (s3_.bufferLib_.accepts(arg1Value)) && (!(PGuards.isPBytes(arg1Value))) && (!(PGuards.isString(arg1Value)))) {
break;
}
count3_++;
s3_ = s3_.next_;
}
if (s3_ == null) {
if ((!(PGuards.isPBytes(arg1Value))) && (!(PGuards.isString(arg1Value))) && count3_ < (3)) {
// assert (s3_.bufferAcquireLib_.accepts(arg1Value));
// assert (s3_.bufferLib_.accepts(arg1Value));
s3_ = arg0Value.insert(new Buffer0Data(s3_original));
s3_.indirectCallNode_ = s3_.insert((IndirectCallData.create()));
PythonBufferAcquireLibrary bufferAcquireLib__ = s3_.insert((PYTHON_BUFFER_ACQUIRE_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(bufferAcquireLib__, "Specialization 'doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)' cache 'bufferAcquireLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.bufferAcquireLib_ = bufferAcquireLib__;
PythonBufferAccessLibrary bufferLib__ = s3_.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(bufferLib__, "Specialization 'doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.bufferLib_ = bufferLib__;
FromByteArrayNode fromByteArrayNode__ = s3_.insert((FromByteArrayNode.create()));
Objects.requireNonNull(fromByteArrayNode__, "Specialization 'doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)' cache 'fromByteArrayNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.fromByteArrayNode_ = fromByteArrayNode__;
SwitchEncodingNode switchEncodingNode__ = s3_.insert((SwitchEncodingNode.create()));
Objects.requireNonNull(switchEncodingNode__, "Specialization 'doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)' cache 'switchEncodingNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.switchEncodingNode_ = switchEncodingNode__;
if (!this.buffer0_cache.compareAndSet(arg0Value, s3_original, s3_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s3_ != null) {
return PyUnicodeFromEncodedObject.doBuffer((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, arg3Value, s3_.indirectCallNode_, this.buffer0_emptyStringProfile_, s3_.bufferAcquireLib_, this.buffer0_decode_, s3_.bufferLib_, s3_.fromByteArrayNode_, s3_.switchEncodingNode_);
}
break;
}
}
{
PythonBufferAccessLibrary bufferLib__ = null;
PythonBufferAcquireLibrary bufferAcquireLib__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(arg0Value);
try {
if ((!(PGuards.isPBytes(arg1Value))) && (!(PGuards.isString(arg1Value)))) {
Buffer1Data s4_ = arg0Value.insert(new Buffer1Data());
s4_.indirectCallNode_ = s4_.insert((IndirectCallData.create()));
bufferAcquireLib__ = (PYTHON_BUFFER_ACQUIRE_LIBRARY_.getUncached());
bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached());
FromByteArrayNode fromByteArrayNode__ = s4_.insert((FromByteArrayNode.create()));
Objects.requireNonNull(fromByteArrayNode__, "Specialization 'doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)' cache 'fromByteArrayNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.fromByteArrayNode_ = fromByteArrayNode__;
SwitchEncodingNode switchEncodingNode__ = s4_.insert((SwitchEncodingNode.create()));
Objects.requireNonNull(switchEncodingNode__, "Specialization 'doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)' cache 'switchEncodingNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.switchEncodingNode_ = switchEncodingNode__;
VarHandle.storeStoreFence();
this.buffer1_cache.set(arg0Value, s4_);
this.buffer0_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyUnicodeFromEncodedObject.doBuffer(VirtualFrame, Node, Object, Object, Object, IndirectCallData, InlinedConditionProfile, PythonBufferAcquireLibrary, PyUnicodeDecode, PythonBufferAccessLibrary, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyUnicodeFromEncodedObject.doBuffer((VirtualFrame) frameValue, s4_, arg1Value, arg2Value, arg3Value, s4_.indirectCallNode_, this.buffer1_emptyStringProfile_, bufferAcquireLib__, this.buffer1_decode_, bufferLib__, fromByteArrayNode__, switchEncodingNode__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyUnicodeFromEncodedObject.class)
@DenyReplace
private static final class Buffer0Data extends Node implements SpecializationDataNode {
@Child Buffer0Data next_;
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link InlinedConditionProfile} emptyStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 2-4: InlinedCache
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int buffer0_state_0_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link IndirectCallData} indirectCallNode
*/
@Child IndirectCallData indirectCallNode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PythonBufferAcquireLibrary} bufferAcquireLib
*/
@Child PythonBufferAcquireLibrary bufferAcquireLib_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node buffer0_decode__field1_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node buffer0_decode__field2_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node buffer0_decode__field3_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child PythonBufferAccessLibrary bufferLib_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link FromByteArrayNode} fromByteArrayNode
*/
@Child FromByteArrayNode fromByteArrayNode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link SwitchEncodingNode} switchEncodingNode
*/
@Child SwitchEncodingNode switchEncodingNode_;
Buffer0Data(Buffer0Data next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyUnicodeFromEncodedObject.class)
@DenyReplace
private static final class Buffer1Data extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link InlinedConditionProfile} emptyStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 2-4: InlinedCache
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int buffer1_state_0_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link IndirectCallData} indirectCallNode
*/
@Child IndirectCallData indirectCallNode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node buffer1_decode__field1_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node buffer1_decode__field2_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link PyUnicodeDecode} decode
* Inline method: {@link PyUnicodeDecodeNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node buffer1_decode__field3_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link FromByteArrayNode} fromByteArrayNode
*/
@Child FromByteArrayNode fromByteArrayNode_;
/**
* Source Info:
* Specialization: {@link PyUnicodeFromEncodedObject#doBuffer}
* Parameter: {@link SwitchEncodingNode} switchEncodingNode
*/
@Child SwitchEncodingNode switchEncodingNode_;
Buffer1Data() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyUnicodeFromEncodedObject.class)
@DenyReplace
private static final class Uncached extends PyUnicodeFromEncodedObject {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof PBytes) {
PBytes arg1Value_ = (PBytes) arg1Value;
return PyUnicodeFromEncodedObject.doBytes((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, (InlinedConditionProfile.getUncached()), (PyUnicodeDecodeNodeGen.getUncached()));
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return PyUnicodeFromEncodedObject.doString((VirtualFrame) frameValue, arg1Value_, arg2Value, arg3Value, (PRaiseNode.getUncached()));
}
if (arg1Value instanceof PString) {
PString arg1Value_ = (PString) arg1Value;
return PyUnicodeFromEncodedObject.doPString((VirtualFrame) frameValue, arg1Value_, arg2Value, arg3Value, (PRaiseNode.getUncached()));
}
if ((!(PGuards.isPBytes(arg1Value))) && (!(PGuards.isString(arg1Value)))) {
return PyUnicodeFromEncodedObject.doBuffer((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, (IndirectCallData.getUncached()), (InlinedConditionProfile.getUncached()), (PYTHON_BUFFER_ACQUIRE_LIBRARY_.getUncached(arg1Value)), (PyUnicodeDecodeNodeGen.getUncached()), (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached(arg1Value)), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy