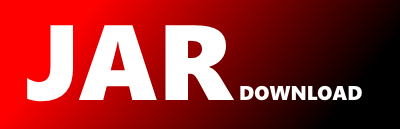
com.oracle.graal.python.nodes.PConstructAndRaiseNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.function.PKeyword;
import com.oracle.graal.python.nodes.call.special.CallVarargsMethodNode;
import com.oracle.graal.python.runtime.exception.PException;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PConstructAndRaiseNode#constructAndRaiseNoFormatString}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link PConstructAndRaiseNode#constructAndRaiseNoArgs}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link PConstructAndRaiseNode#constructAndRaise}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(PConstructAndRaiseNode.class)
@SuppressWarnings("javadoc")
public final class PConstructAndRaiseNodeGen extends PConstructAndRaiseNode {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PConstructAndRaiseNode#constructAndRaiseNoFormatString}
* 1: SpecializationActive {@link PConstructAndRaiseNode#constructAndRaiseNoArgs}
* 2: SpecializationActive {@link PConstructAndRaiseNode#constructAndRaise}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PConstructAndRaiseNode#constructAndRaiseNoFormatString}
* Parameter: {@link CallVarargsMethodNode} callNode
*/
@Child private CallVarargsMethodNode callNode;
/**
* Source Info:
* Specialization: {@link PConstructAndRaiseNode#constructAndRaiseNoFormatString}
* Parameter: {@link FromJavaStringNode} fromJavaStringNode
*/
@Child private FromJavaStringNode js2ts;
private PConstructAndRaiseNodeGen() {
}
@Override
public PException execute(Frame frameValue, PythonBuiltinClassType arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value, Object[] arg4Value, PKeyword[] arg5Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PConstructAndRaiseNode.constructAndRaiseNoFormatString(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] || SpecializationActive[PConstructAndRaiseNode.constructAndRaiseNoArgs(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] || SpecializationActive[PConstructAndRaiseNode.constructAndRaise(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PConstructAndRaiseNode.constructAndRaiseNoFormatString(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */) {
{
CallVarargsMethodNode callNode_ = this.callNode;
if (callNode_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
if ((arg2Value == null) && (arg3Value == null)) {
return constructAndRaiseNoFormatString((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, callNode_, js2ts_);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PConstructAndRaiseNode.constructAndRaiseNoArgs(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */) {
{
CallVarargsMethodNode callNode_1 = this.callNode;
if (callNode_1 != null) {
FromJavaStringNode js2ts_1 = this.js2ts;
if (js2ts_1 != null) {
if ((arg2Value != null) && (arg4Value == null)) {
return constructAndRaiseNoArgs((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, callNode_1, js2ts_1);
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PConstructAndRaiseNode.constructAndRaise(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */) {
{
CallVarargsMethodNode callNode_2 = this.callNode;
if (callNode_2 != null) {
FromJavaStringNode js2ts_2 = this.js2ts;
if (js2ts_2 != null) {
if ((arg2Value != null) && (arg4Value != null)) {
return constructAndRaise((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, callNode_2, js2ts_2);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private PException executeAndSpecialize(Frame frameValue, PythonBuiltinClassType arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value, Object[] arg4Value, PKeyword[] arg5Value) {
int state_0 = this.state_0_;
if ((arg2Value == null) && (arg3Value == null)) {
CallVarargsMethodNode callNode_;
CallVarargsMethodNode callNode__shared = this.callNode;
if (callNode__shared != null) {
callNode_ = callNode__shared;
} else {
callNode_ = this.insert((CallVarargsMethodNode.create()));
if (callNode_ == null) {
throw new IllegalStateException("Specialization 'constructAndRaiseNoFormatString(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'constructAndRaiseNoFormatString(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PConstructAndRaiseNode.constructAndRaiseNoFormatString(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return constructAndRaiseNoFormatString((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, callNode_, js2ts_);
}
if ((arg2Value != null) && (arg4Value == null)) {
CallVarargsMethodNode callNode_1;
CallVarargsMethodNode callNode_1_shared = this.callNode;
if (callNode_1_shared != null) {
callNode_1 = callNode_1_shared;
} else {
callNode_1 = this.insert((CallVarargsMethodNode.create()));
if (callNode_1 == null) {
throw new IllegalStateException("Specialization 'constructAndRaiseNoArgs(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_1;
}
FromJavaStringNode js2ts_1;
FromJavaStringNode js2ts_1_shared = this.js2ts;
if (js2ts_1_shared != null) {
js2ts_1 = js2ts_1_shared;
} else {
js2ts_1 = this.insert((FromJavaStringNode.create()));
if (js2ts_1 == null) {
throw new IllegalStateException("Specialization 'constructAndRaiseNoArgs(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_1;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PConstructAndRaiseNode.constructAndRaiseNoArgs(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return constructAndRaiseNoArgs((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, callNode_1, js2ts_1);
}
if ((arg2Value != null) && (arg4Value != null)) {
CallVarargsMethodNode callNode_2;
CallVarargsMethodNode callNode_2_shared = this.callNode;
if (callNode_2_shared != null) {
callNode_2 = callNode_2_shared;
} else {
callNode_2 = this.insert((CallVarargsMethodNode.create()));
if (callNode_2 == null) {
throw new IllegalStateException("Specialization 'constructAndRaise(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_2;
}
FromJavaStringNode js2ts_2;
FromJavaStringNode js2ts_2_shared = this.js2ts;
if (js2ts_2_shared != null) {
js2ts_2 = js2ts_2_shared;
} else {
js2ts_2 = this.insert((FromJavaStringNode.create()));
if (js2ts_2 == null) {
throw new IllegalStateException("Specialization 'constructAndRaise(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_2;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PConstructAndRaiseNode.constructAndRaise(VirtualFrame, PythonBuiltinClassType, Object, TruffleString, Object[], Object[], PKeyword[], CallVarargsMethodNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return constructAndRaise((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, callNode_2, js2ts_2);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PConstructAndRaiseNode create() {
return new PConstructAndRaiseNodeGen();
}
@NeverDefault
public static PConstructAndRaiseNode getUncached() {
return PConstructAndRaiseNodeGen.UNCACHED;
}
@GeneratedBy(PConstructAndRaiseNode.class)
@DenyReplace
private static final class Uncached extends PConstructAndRaiseNode {
@Override
public PException execute(Frame frameValue, PythonBuiltinClassType arg0Value, Object arg1Value, TruffleString arg2Value, Object[] arg3Value, Object[] arg4Value, PKeyword[] arg5Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if ((arg2Value == null) && (arg3Value == null)) {
return constructAndRaiseNoFormatString((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (CallVarargsMethodNode.getUncached()), (FromJavaStringNode.getUncached()));
}
if ((arg2Value != null) && (arg4Value == null)) {
return constructAndRaiseNoArgs((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (CallVarargsMethodNode.getUncached()), (FromJavaStringNode.getUncached()));
}
if ((arg2Value != null) && (arg4Value != null)) {
return constructAndRaise((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (CallVarargsMethodNode.getUncached()), (FromJavaStringNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link Lazy#doIt}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(Lazy.class)
@SuppressWarnings("javadoc")
public static final class LazyNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static Lazy getUncached() {
return LazyNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#node_}
*
*/
@NeverDefault
public static Lazy inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new LazyNodeGen.Inlined(target);
}
@GeneratedBy(Lazy.class)
@DenyReplace
private static final class Inlined extends Lazy {
/**
* State Info:
* 0: SpecializationActive {@link Lazy#doIt}
*
*/
private final StateField state_0_;
private final ReferenceField node_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(Lazy.class);
this.state_0_ = target.getState(0, 1);
this.node_ = target.getReference(1, PConstructAndRaiseNode.class);
}
@Override
PConstructAndRaiseNode execute(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PConstructAndRaiseNode.Lazy.doIt(PConstructAndRaiseNode)] */) {
{
PConstructAndRaiseNode node__ = this.node_.get(arg0Value);
if (node__ != null) {
return Lazy.doIt(node__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private PConstructAndRaiseNode executeAndSpecialize(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
PConstructAndRaiseNode node__ = arg0Value.insert((PConstructAndRaiseNode.create()));
Objects.requireNonNull(node__, "Specialization 'doIt(PConstructAndRaiseNode)' cache 'node' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.node_.set(arg0Value, node__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PConstructAndRaiseNode.Lazy.doIt(PConstructAndRaiseNode)] */;
this.state_0_.set(arg0Value, state_0);
return Lazy.doIt(node__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(Lazy.class)
@DenyReplace
private static final class Uncached extends Lazy {
@TruffleBoundary
@Override
PConstructAndRaiseNode execute(Node arg0Value) {
return Lazy.doIt((PConstructAndRaiseNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy