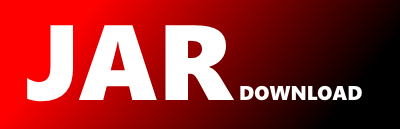
com.oracle.graal.python.nodes.PRaiseNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.PNone;
import com.oracle.graal.python.builtins.objects.exception.PBaseException;
import com.oracle.graal.python.runtime.exception.PException;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PRaiseNode#doPythonBuiltinTypeCached}
* Activation probability: 0.32000
* With/without class size: 10/4 bytes
* Specialization {@link PRaiseNode#doPythonBuiltinType}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link PRaiseNode#doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link PRaiseNode#doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link PRaiseNode#doBuiltinTypeWithCause}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(PRaiseNode.class)
@SuppressWarnings("javadoc")
public final class PRaiseNodeGen extends PRaiseNode {
static final ReferenceField PYTHON_BUILTIN_TYPE_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "pythonBuiltinTypeCached_cache", PythonBuiltinTypeCachedData.class);
private static final Uncached UNCACHED = new Uncached();
@CompilationFinal(dimensions = 1) private static final PythonBuiltinClassType[] PYTHON_BUILTIN_CLASS_TYPE_VALUES = DSLSupport.lookupEnumConstants(PythonBuiltinClassType.class);
/**
* State Info:
* 0: SpecializationActive {@link PRaiseNode#doPythonBuiltinTypeCached}
* 1: SpecializationActive {@link PRaiseNode#doPythonBuiltinType}
* 2: SpecializationActive {@link PRaiseNode#doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)}
* 3: SpecializationActive {@link PRaiseNode#doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)}
* 4: SpecializationActive {@link PRaiseNode#doBuiltinTypeWithCause}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PRaiseNode#doPythonBuiltinTypeCached}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory;
/**
* Source Info:
* Specialization: {@link PRaiseNode#doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)}
* Parameter: {@link FromJavaStringNode} fromJavaStringNode
*/
@Child private FromJavaStringNode js2ts;
@UnsafeAccessedField @CompilationFinal private PythonBuiltinTypeCachedData pythonBuiltinTypeCached_cache;
private PRaiseNodeGen() {
}
@ExplodeLoop
@Override
public PException execute(Node arg0Value, PythonBuiltinClassType arg1Value, Object[] arg2Value, Object arg3Value, Object arg4Value, Object[] arg5Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PRaiseNode.doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)] || SpecializationActive[PRaiseNode.doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)] || SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)] || SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] || SpecializationActive[PRaiseNode.doBuiltinTypeWithCause(Node, PythonBuiltinClassType, Object[], PBaseException, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] */) {
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[PRaiseNode.doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)] || SpecializationActive[PRaiseNode.doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)] || SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)] || SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] */ && arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[PRaiseNode.doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)] || SpecializationActive[PRaiseNode.doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)] || SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)] */ && arg4Value instanceof PNone) {
PNone arg4Value_ = (PNone) arg4Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PRaiseNode.doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)] */ && (PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length == 0)) {
PythonBuiltinTypeCachedData s0_ = this.pythonBuiltinTypeCached_cache;
while (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
if ((arg1Value == decodePythonBuiltinClassType((s0_.pythonBuiltinTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2))) {
return PRaiseNode.doPythonBuiltinTypeCached(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, decodePythonBuiltinClassType((s0_.pythonBuiltinTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2), factory_);
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PRaiseNode.doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)] */) {
{
PythonObjectFactory factory_1 = this.factory;
if (factory_1 != null) {
if ((PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length == 0)) {
return PRaiseNode.doPythonBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_1);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)] */) {
{
PythonObjectFactory factory_2 = this.factory;
if (factory_2 != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
if ((PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length > 0)) {
return PRaiseNode.doBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_2, js2ts_);
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] */ && arg4Value instanceof TruffleString) {
TruffleString arg4Value_ = (TruffleString) arg4Value;
{
PythonObjectFactory factory_3 = this.factory;
if (factory_3 != null) {
FromJavaStringNode js2ts_1 = this.js2ts;
if (js2ts_1 != null) {
if ((PGuards.isNoValue(arg3Value_))) {
return PRaiseNode.doBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_3, js2ts_1);
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PRaiseNode.doBuiltinTypeWithCause(Node, PythonBuiltinClassType, Object[], PBaseException, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] */ && arg3Value instanceof PBaseException) {
PBaseException arg3Value_ = (PBaseException) arg3Value;
if (arg4Value instanceof TruffleString) {
TruffleString arg4Value_ = (TruffleString) arg4Value;
{
PythonObjectFactory factory_4 = this.factory;
if (factory_4 != null) {
FromJavaStringNode js2ts_2 = this.js2ts;
if (js2ts_2 != null) {
if ((!(PGuards.isNoValue(arg3Value_)))) {
return PRaiseNode.doBuiltinTypeWithCause(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_4, js2ts_2);
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private PException executeAndSpecialize(Node arg0Value, PythonBuiltinClassType arg1Value, Object[] arg2Value, Object arg3Value, Object arg4Value, Object[] arg5Value) {
int state_0 = this.state_0_;
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
if (arg4Value instanceof PNone) {
PNone arg4Value_ = (PNone) arg4Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PRaiseNode.doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)] */ && (PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length == 0)) {
while (true) {
int count0_ = 0;
PythonBuiltinTypeCachedData s0_ = PYTHON_BUILTIN_TYPE_CACHED_CACHE_UPDATER.getVolatile(this);
PythonBuiltinTypeCachedData s0_original = s0_;
while (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
if ((arg1Value == decodePythonBuiltinClassType((s0_.pythonBuiltinTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2))) {
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg1Value == decodePythonBuiltinClassType((s0_.pythonBuiltinTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2));
if (count0_ < (8)) {
s0_ = new PythonBuiltinTypeCachedData(s0_original);
s0_.pythonBuiltinTypeCached_state_0_ = (s0_.pythonBuiltinTypeCached_state_0_ | ((encodePythonBuiltinClassType((arg1Value)) + 2) << 0) /* set-int EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)] */);
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory;
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = this.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_;
}
if (!PYTHON_BUILTIN_TYPE_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PRaiseNode.doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)] */;
this.state_0_ = state_0;
}
}
if (s0_ != null) {
return PRaiseNode.doPythonBuiltinTypeCached(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, decodePythonBuiltinClassType((s0_.pythonBuiltinTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2), this.factory);
}
break;
}
}
if ((PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length == 0)) {
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory;
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = this.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_1;
}
this.pythonBuiltinTypeCached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PRaiseNode.doPythonBuiltinTypeCached(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonBuiltinClassType, PythonObjectFactory)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PRaiseNode.doPythonBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory)] */;
this.state_0_ = state_0;
return PRaiseNode.doPythonBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_1);
}
if ((PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length > 0)) {
PythonObjectFactory factory_2;
PythonObjectFactory factory_2_shared = this.factory;
if (factory_2_shared != null) {
factory_2 = factory_2_shared;
} else {
factory_2 = this.insert((PythonObjectFactory.create()));
if (factory_2 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_2;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, PNone, Object[], PythonObjectFactory, FromJavaStringNode)] */;
this.state_0_ = state_0;
return PRaiseNode.doBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_2, js2ts_);
}
}
if (arg4Value instanceof TruffleString) {
TruffleString arg4Value_ = (TruffleString) arg4Value;
if ((PGuards.isNoValue(arg3Value_))) {
PythonObjectFactory factory_3;
PythonObjectFactory factory_3_shared = this.factory;
if (factory_3_shared != null) {
factory_3 = factory_3_shared;
} else {
factory_3 = this.insert((PythonObjectFactory.create()));
if (factory_3 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_3;
}
FromJavaStringNode js2ts_1;
FromJavaStringNode js2ts_1_shared = this.js2ts;
if (js2ts_1_shared != null) {
js2ts_1 = js2ts_1_shared;
} else {
js2ts_1 = this.insert((FromJavaStringNode.create()));
if (js2ts_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_1;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[PRaiseNode.doBuiltinType(Node, PythonBuiltinClassType, Object[], PNone, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] */;
this.state_0_ = state_0;
return PRaiseNode.doBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_3, js2ts_1);
}
}
}
if (arg3Value instanceof PBaseException) {
PBaseException arg3Value_ = (PBaseException) arg3Value;
if (arg4Value instanceof TruffleString) {
TruffleString arg4Value_ = (TruffleString) arg4Value;
if ((!(PGuards.isNoValue(arg3Value_)))) {
PythonObjectFactory factory_4;
PythonObjectFactory factory_4_shared = this.factory;
if (factory_4_shared != null) {
factory_4 = factory_4_shared;
} else {
factory_4 = this.insert((PythonObjectFactory.create()));
if (factory_4 == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeWithCause(Node, PythonBuiltinClassType, Object[], PBaseException, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_4;
}
FromJavaStringNode js2ts_2;
FromJavaStringNode js2ts_2_shared = this.js2ts;
if (js2ts_2_shared != null) {
js2ts_2 = js2ts_2_shared;
} else {
js2ts_2 = this.insert((FromJavaStringNode.create()));
if (js2ts_2 == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeWithCause(Node, PythonBuiltinClassType, Object[], PBaseException, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[PRaiseNode.doBuiltinTypeWithCause(Node, PythonBuiltinClassType, Object[], PBaseException, TruffleString, Object[], PythonObjectFactory, FromJavaStringNode)] */;
this.state_0_ = state_0;
return PRaiseNode.doBuiltinTypeWithCause(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, factory_4, js2ts_2);
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
PythonBuiltinTypeCachedData s0_ = this.pythonBuiltinTypeCached_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PRaiseNode create() {
return new PRaiseNodeGen();
}
@NeverDefault
public static PRaiseNode getUncached() {
return PRaiseNodeGen.UNCACHED;
}
private static PythonBuiltinClassType decodePythonBuiltinClassType(int state) {
if (state >= 0) {
return PYTHON_BUILTIN_CLASS_TYPE_VALUES[state];
} else {
return null;
}
}
private static int encodePythonBuiltinClassType(PythonBuiltinClassType e) {
if (e != null) {
return e.ordinal();
} else {
return -1;
}
}
@GeneratedBy(PRaiseNode.class)
@DenyReplace
private static final class PythonBuiltinTypeCachedData implements SpecializationDataNode {
@CompilationFinal final PythonBuiltinTypeCachedData next_;
/**
* State Info:
* 0-8: EncodedEnum[cache=PRaiseNode.doPythonBuiltinTypeCached(..., PythonBuiltinClassType cachedType, ...)]
*
*/
@CompilationFinal private int pythonBuiltinTypeCached_state_0_;
PythonBuiltinTypeCachedData(PythonBuiltinTypeCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(PRaiseNode.class)
@DenyReplace
private static final class Uncached extends PRaiseNode {
@TruffleBoundary
@Override
public PException execute(Node arg0Value, PythonBuiltinClassType arg1Value, Object[] arg2Value, Object arg3Value, Object arg4Value, Object[] arg5Value) {
if (arg3Value instanceof PNone) {
PNone arg3Value_ = (PNone) arg3Value;
if (arg4Value instanceof PNone) {
PNone arg4Value_ = (PNone) arg4Value;
if ((PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length == 0)) {
return PRaiseNode.doPythonBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, (PythonObjectFactory.getUncached()));
}
if ((PGuards.isNoValue(arg3Value_)) && (PGuards.isNoValue(arg4Value_)) && (arg5Value.length > 0)) {
return PRaiseNode.doBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, (PythonObjectFactory.getUncached()), (FromJavaStringNode.getUncached()));
}
}
if (arg4Value instanceof TruffleString) {
TruffleString arg4Value_ = (TruffleString) arg4Value;
if ((PGuards.isNoValue(arg3Value_))) {
return PRaiseNode.doBuiltinType(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, (PythonObjectFactory.getUncached()), (FromJavaStringNode.getUncached()));
}
}
}
if (arg3Value instanceof PBaseException) {
PBaseException arg3Value_ = (PBaseException) arg3Value;
if (arg4Value instanceof TruffleString) {
TruffleString arg4Value_ = (TruffleString) arg4Value;
if ((!(PGuards.isNoValue(arg3Value_)))) {
return PRaiseNode.doBuiltinTypeWithCause(arg0Value, arg1Value, arg2Value, arg3Value_, arg4Value_, arg5Value, (PythonObjectFactory.getUncached()), (FromJavaStringNode.getUncached()));
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link Lazy#doIt}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(Lazy.class)
@SuppressWarnings("javadoc")
public static final class LazyNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static Lazy getUncached() {
return LazyNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#node_}
*
*/
@NeverDefault
public static Lazy inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new LazyNodeGen.Inlined(target);
}
@GeneratedBy(Lazy.class)
@DenyReplace
private static final class Inlined extends Lazy {
/**
* State Info:
* 0: SpecializationActive {@link Lazy#doIt}
*
*/
private final StateField state_0_;
private final ReferenceField node_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(Lazy.class);
this.state_0_ = target.getState(0, 1);
this.node_ = target.getReference(1, PRaiseNode.class);
}
@Override
PRaiseNode execute(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PRaiseNode.Lazy.doIt(PRaiseNode)] */) {
{
PRaiseNode node__ = this.node_.get(arg0Value);
if (node__ != null) {
return Lazy.doIt(node__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private PRaiseNode executeAndSpecialize(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
PRaiseNode node__ = arg0Value.insert((PRaiseNode.create()));
Objects.requireNonNull(node__, "Specialization 'doIt(PRaiseNode)' cache 'node' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.node_.set(arg0Value, node__);
state_0 = state_0 | 0b1 /* add SpecializationActive[PRaiseNode.Lazy.doIt(PRaiseNode)] */;
this.state_0_.set(arg0Value, state_0);
return Lazy.doIt(node__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(Lazy.class)
@DenyReplace
private static final class Uncached extends Lazy {
@TruffleBoundary
@Override
PRaiseNode execute(Node arg0Value) {
return Lazy.doIt((PRaiseNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy