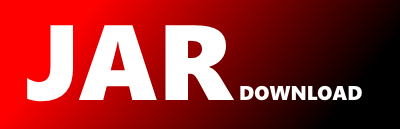
com.oracle.graal.python.nodes.attributes.LookupAttributeInMRONodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.attributes;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.type.MroShape;
import com.oracle.graal.python.builtins.objects.type.PythonClass;
import com.oracle.graal.python.builtins.objects.type.MroShape.MroShapeLookupResult;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.GetMroStorageNode;
import com.oracle.graal.python.builtins.objects.type.TypeNodesFactory.GetMroStorageNodeGen;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.runtime.PythonOptions;
import com.oracle.graal.python.runtime.sequence.storage.MroSequenceStorage;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link LookupAttributeInMRONode#lookupPBCTCached}
* Activation probability: 0.19111
* With/without class size: 8/8 bytes
* Specialization {@link LookupAttributeInMRONode#lookupPBCTCachedMulti}
* Activation probability: 0.17111
* With/without class size: 8/8 bytes
* Specialization {@link LookupAttributeInMRONode#lookupPBCTCachedOwner}
* Activation probability: 0.15111
* With/without class size: 7/8 bytes
* Specialization {@link LookupAttributeInMRONode#lookupPBCTGeneric}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link LookupAttributeInMRONode#lookupConstantMROCached}
* Activation probability: 0.11111
* With/without class size: 6/8 bytes
* Specialization {@link LookupAttributeInMRONode#lookupConstantMROShape}
* Activation probability: 0.09111
* With/without class size: 6/8 bytes
* Specialization {@link LookupAttributeInMRONode#lookupConstantMRO}
* Activation probability: 0.07111
* With/without class size: 7/24 bytes
* Specialization {@link LookupAttributeInMRONode#lookupCachedLen}
* Activation probability: 0.05111
* With/without class size: 5/12 bytes
* Specialization {@link LookupAttributeInMRONode#lookupGeneric}
* Activation probability: 0.03111
* With/without class size: 4/8 bytes
*
*/
@GeneratedBy(LookupAttributeInMRONode.class)
@SuppressWarnings("javadoc")
public final class LookupAttributeInMRONodeGen extends LookupAttributeInMRONode {
static final ReferenceField LOOKUP_P_B_C_T_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupPBCTCached_cache", LookupPBCTCachedData.class);
static final ReferenceField LOOKUP_P_B_C_T_CACHED_MULTI_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupPBCTCachedMulti_cache", LookupPBCTCachedMultiData.class);
static final ReferenceField LOOKUP_P_B_C_T_CACHED_OWNER_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupPBCTCachedOwner_cache", LookupPBCTCachedOwnerData.class);
static final ReferenceField LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMROCached_cache", LookupConstantMROCachedData.class);
static final ReferenceField LOOKUP_CONSTANT_M_R_O_SHAPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMROShape_cache", LookupConstantMROShapeData.class);
static final ReferenceField LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMRO_cache", LookupConstantMROData.class);
static final ReferenceField LOOKUP_CACHED_LEN_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupCachedLen_cache", LookupCachedLenData.class);
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
@CompilationFinal(dimensions = 1) private static final PythonBuiltinClassType[] PYTHON_BUILTIN_CLASS_TYPE_VALUES = DSLSupport.lookupEnumConstants(PythonBuiltinClassType.class);
/**
* State Info:
* 0: SpecializationActive {@link LookupAttributeInMRONode#lookupPBCTCached}
* 1: SpecializationActive {@link LookupAttributeInMRONode#lookupPBCTCachedOwner}
* 2: SpecializationActive {@link LookupAttributeInMRONode#lookupPBCTGeneric}
* 3: SpecializationActive {@link LookupAttributeInMRONode#lookupPBCTCachedMulti}
* 4: SpecializationActive {@link LookupAttributeInMRONode#lookupConstantMROCached}
* 5: SpecializationActive {@link LookupAttributeInMRONode#lookupCachedLen}
* 6: SpecializationActive {@link LookupAttributeInMRONode#lookupGeneric}
* 7: SpecializationActive {@link LookupAttributeInMRONode#lookupConstantMROShape}
* 8: SpecializationActive {@link LookupAttributeInMRONode#lookupConstantMRO}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupPBCTCachedOwner}
* Parameter: {@link ReadAttributeFromDynamicObjectNode} readAttrNode
*/
@Child private ReadAttributeFromDynamicObjectNode readAttrNode;
@UnsafeAccessedField @CompilationFinal private LookupPBCTCachedData lookupPBCTCached_cache;
@UnsafeAccessedField @CompilationFinal private LookupPBCTCachedMultiData lookupPBCTCachedMulti_cache;
@UnsafeAccessedField @CompilationFinal private LookupPBCTCachedOwnerData lookupPBCTCachedOwner_cache;
@UnsafeAccessedField @CompilationFinal private LookupConstantMROCachedData lookupConstantMROCached_cache;
@UnsafeAccessedField @Child private LookupConstantMROShapeData lookupConstantMROShape_cache;
@UnsafeAccessedField @Child private LookupConstantMROData lookupConstantMRO_cache;
@UnsafeAccessedField @Child private LookupCachedLenData lookupCachedLen_cache;
@Child private LookupGenericData lookupGeneric_cache;
private LookupAttributeInMRONodeGen(TruffleString key, boolean skipNonStaticBases) {
super(key, skipNonStaticBases);
}
@ExplodeLoop
@Override
public Object execute(Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupPBCTCached(PythonBuiltinClassType, PythonBuiltinClassType, Object)] || SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedMulti(PythonBuiltinClassType, PythonBuiltinClassType, Object)] || SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[LookupAttributeInMRONode.lookupConstantMROCached(Object, Object, AttributeAssumptionPair)] || SpecializationActive[LookupAttributeInMRONode.lookupConstantMROShape(PythonClass, MroShape, MroShapeLookupResult)] || SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] || SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] || SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */) {
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupPBCTCached(PythonBuiltinClassType, PythonBuiltinClassType, Object)] || SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedMulti(PythonBuiltinClassType, PythonBuiltinClassType, Object)] || SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupPBCTCached(PythonBuiltinClassType, PythonBuiltinClassType, Object)] */) {
assert DSLSupport.assertIdempotence((isSingleContext()));
LookupPBCTCachedData s0_ = this.lookupPBCTCached_cache;
while (s0_ != null) {
if ((arg0Value_ == decodePythonBuiltinClassType((s0_.lookupPBCTCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1))) {
return LookupAttributeInMRONode.lookupPBCTCached(arg0Value_, decodePythonBuiltinClassType((s0_.lookupPBCTCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), s0_.cachedValue_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedMulti(PythonBuiltinClassType, PythonBuiltinClassType, Object)] */) {
LookupPBCTCachedMultiData s1_ = this.lookupPBCTCachedMulti_cache;
while (s1_ != null) {
if ((arg0Value_ == decodePythonBuiltinClassType((s1_.lookupPBCTCachedMulti_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1))) {
assert DSLSupport.assertIdempotence((LookupAttributeInMRONode.canCache(s1_.cachedValue_)));
return LookupAttributeInMRONode.lookupPBCTCachedMulti(arg0Value_, decodePythonBuiltinClassType((s1_.lookupPBCTCachedMulti_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), s1_.cachedValue_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */) {
LookupPBCTCachedOwnerData s2_ = this.lookupPBCTCachedOwner_cache;
while (s2_ != null) {
{
ReadAttributeFromDynamicObjectNode readAttrNode_ = this.readAttrNode;
if (readAttrNode_ != null) {
if ((arg0Value_ == decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0b111111111) >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1))) {
return lookupPBCTCachedOwner(arg0Value_, decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0b111111111) >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0x3fe00) >>> 9 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType ownerKlass, ...)] */) - 2), readAttrNode_);
}
}
}
s2_ = s2_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */) {
{
ReadAttributeFromDynamicObjectNode readAttrNode_1 = this.readAttrNode;
if (readAttrNode_1 != null) {
return lookupPBCTGeneric(arg0Value_, readAttrNode_1);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupConstantMROCached(Object, Object, AttributeAssumptionPair)] */) {
assert DSLSupport.assertIdempotence((isSingleContext()));
LookupConstantMROCachedData s4_ = this.lookupConstantMROCached_cache;
while (s4_ != null) {
if (!Assumption.isValidAssumption((s4_.cachedAttrInMROInfo_.assumption))) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeLookupConstantMROCached_(s4_);
return executeAndSpecialize(arg0Value);
}
if ((isSameType(s4_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s4_.cachedAttrInMROInfo_ != null));
return LookupAttributeInMRONode.lookupConstantMROCached(arg0Value, s4_.cachedKlass_, s4_.cachedAttrInMROInfo_);
}
s4_ = s4_.next_;
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupConstantMROShape(PythonClass, MroShape, MroShapeLookupResult)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
assert DSLSupport.assertIdempotence((!(isSingleContext())));
LookupConstantMROShapeData s5_ = this.lookupConstantMROShape_cache;
while (s5_ != null) {
assert DSLSupport.assertIdempotence((s5_.cachedMroShape_ != null));
if ((arg0Value_.getMroShape() == s5_.cachedMroShape_)) {
return lookupConstantMROShape(arg0Value_, s5_.cachedMroShape_, s5_.lookupResult_);
}
s5_ = s5_.next_;
}
}
if ((state_0 & 0b101100000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] || SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] || SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] */) {
assert DSLSupport.assertIdempotence((isSingleContext()));
LookupConstantMROData s6_ = this.lookupConstantMRO_cache;
while (s6_ != null) {
if (!Assumption.isValidAssumption((s6_.lookupStable_))) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeLookupConstantMRO_(s6_);
return executeAndSpecialize(arg0Value);
}
if ((isSameType(s6_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s6_.mroLength_ < 32));
return lookupConstantMRO(arg0Value, s6_.cachedKlass_, s6_.mro_, s6_.lookupStable_, s6_.mroLength_, s6_.dylib_, s6_.readAttrNodes_);
}
s6_ = s6_.next_;
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] */) {
LookupCachedLenData s7_ = this.lookupCachedLen_cache;
while (s7_ != null) {
{
MroSequenceStorage mro__ = (getMro(arg0Value));
int mroLength__ = (mro__.length());
if ((mroLength__ == s7_.cachedMroLength_)) {
assert DSLSupport.assertIdempotence((s7_.cachedMroLength_ < 32));
return lookupCachedLen(arg0Value, mro__, mroLength__, s7_.cachedMroLength_, s7_.dylib_, s7_.readAttrNodes_);
}
}
s7_ = s7_.next_;
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */) {
LookupGenericData s8_ = this.lookupGeneric_cache;
if (s8_ != null) {
return lookupGeneric(arg0Value, s8_.dylib_, s8_.readAttrNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
int oldState_0 = state_0;
try {
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
if (((state_0 & 0b110)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] && SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */ && (isSingleContext())) {
while (true) {
int count0_ = 0;
LookupPBCTCachedData s0_ = LOOKUP_P_B_C_T_CACHED_CACHE_UPDATER.getVolatile(this);
LookupPBCTCachedData s0_original = s0_;
while (s0_ != null) {
if ((arg0Value_ == decodePythonBuiltinClassType((s0_.lookupPBCTCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg0Value_ == decodePythonBuiltinClassType((s0_.lookupPBCTCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1));
if (count0_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s0_ = new LookupPBCTCachedData(s0_original);
s0_.lookupPBCTCached_state_0_ = (s0_.lookupPBCTCached_state_0_ | (((arg0Value_).ordinal() + 1) << 0) /* set-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */);
s0_.cachedValue_ = (LookupAttributeInMRONode.findAttr(getContext(), decodePythonBuiltinClassType((s0_.lookupPBCTCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), key));
if (!LOOKUP_P_B_C_T_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[LookupAttributeInMRONode.lookupPBCTCached(PythonBuiltinClassType, PythonBuiltinClassType, Object)] */;
this.state_0_ = state_0;
}
}
if (s0_ != null) {
return LookupAttributeInMRONode.lookupPBCTCached(arg0Value_, decodePythonBuiltinClassType((s0_.lookupPBCTCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), s0_.cachedValue_);
}
break;
}
}
if (((state_0 & 0b110)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] && SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */) {
while (true) {
int count1_ = 0;
LookupPBCTCachedMultiData s1_ = LOOKUP_P_B_C_T_CACHED_MULTI_CACHE_UPDATER.getVolatile(this);
LookupPBCTCachedMultiData s1_original = s1_;
while (s1_ != null) {
if ((arg0Value_ == decodePythonBuiltinClassType((s1_.lookupPBCTCachedMulti_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1))) {
assert DSLSupport.assertIdempotence((LookupAttributeInMRONode.canCache(s1_.cachedValue_)));
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
PythonBuiltinClassType cachedKlass__ = (arg0Value_);
Object cachedValue__ = (LookupAttributeInMRONode.findAttr(getContext(), cachedKlass__, key));
// assert (arg0Value_ == decodePythonBuiltinClassType((s1_.lookupPBCTCachedMulti_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1));
if ((LookupAttributeInMRONode.canCache(cachedValue__)) && count1_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s1_ = new LookupPBCTCachedMultiData(s1_original);
s1_.lookupPBCTCachedMulti_state_0_ = (s1_.lookupPBCTCachedMulti_state_0_ | ((cachedKlass__.ordinal() + 1) << 0) /* set-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)] */);
s1_.cachedValue_ = cachedValue__;
if (!LOOKUP_P_B_C_T_CACHED_MULTI_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedMulti(PythonBuiltinClassType, PythonBuiltinClassType, Object)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return LookupAttributeInMRONode.lookupPBCTCachedMulti(arg0Value_, decodePythonBuiltinClassType((s1_.lookupPBCTCachedMulti_state_0_ >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), s1_.cachedValue_);
}
break;
}
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */) {
while (true) {
int count2_ = 0;
LookupPBCTCachedOwnerData s2_ = LOOKUP_P_B_C_T_CACHED_OWNER_CACHE_UPDATER.getVolatile(this);
LookupPBCTCachedOwnerData s2_original = s2_;
while (s2_ != null) {
{
ReadAttributeFromDynamicObjectNode readAttrNode_ = this.readAttrNode;
if (readAttrNode_ != null) {
if ((arg0Value_ == decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0b111111111) >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1))) {
break;
}
}
}
count2_++;
s2_ = s2_.next_;
}
if (s2_ == null) {
// assert (arg0Value_ == decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0b111111111) >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1));
if (count2_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s2_ = new LookupPBCTCachedOwnerData(s2_original);
s2_.lookupPBCTCachedOwner_state_0_ = (s2_.lookupPBCTCachedOwner_state_0_ | (((arg0Value_).ordinal() + 1) << 0) /* set-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */);
s2_.lookupPBCTCachedOwner_state_0_ = (s2_.lookupPBCTCachedOwner_state_0_ | ((encodePythonBuiltinClassType((LookupAttributeInMRONode.findOwnerInMro(getContext(), decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0b111111111) >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), key))) + 2) << 9) /* set-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType ownerKlass, ...)] */);
ReadAttributeFromDynamicObjectNode readAttrNode_;
ReadAttributeFromDynamicObjectNode readAttrNode__shared = this.readAttrNode;
if (readAttrNode__shared != null) {
readAttrNode_ = readAttrNode__shared;
} else {
readAttrNode_ = this.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readAttrNode_ == null) {
throw new IllegalStateException("Specialization 'lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)' contains a shared cache with name 'readAttrNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readAttrNode == null) {
this.readAttrNode = readAttrNode_;
}
if (!LOOKUP_P_B_C_T_CACHED_OWNER_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
this.lookupPBCTCached_cache = null;
this.lookupPBCTCachedMulti_cache = null;
state_0 = state_0 & 0xfffffff6 /* remove SpecializationActive[LookupAttributeInMRONode.lookupPBCTCached(PythonBuiltinClassType, PythonBuiltinClassType, Object)], SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedMulti(PythonBuiltinClassType, PythonBuiltinClassType, Object)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */;
this.state_0_ = state_0;
}
}
if (s2_ != null) {
return lookupPBCTCachedOwner(arg0Value_, decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0b111111111) >>> 0 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)] */) - 1), decodePythonBuiltinClassType(((s2_.lookupPBCTCachedOwner_state_0_ & 0x3fe00) >>> 9 /* get-int EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType ownerKlass, ...)] */) - 2), this.readAttrNode);
}
break;
}
}
ReadAttributeFromDynamicObjectNode readAttrNode_1;
ReadAttributeFromDynamicObjectNode readAttrNode_1_shared = this.readAttrNode;
if (readAttrNode_1_shared != null) {
readAttrNode_1 = readAttrNode_1_shared;
} else {
readAttrNode_1 = this.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readAttrNode_1 == null) {
throw new IllegalStateException("Specialization 'lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)' contains a shared cache with name 'readAttrNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readAttrNode == null) {
VarHandle.storeStoreFence();
this.readAttrNode = readAttrNode_1;
}
this.lookupPBCTCached_cache = null;
this.lookupPBCTCachedMulti_cache = null;
this.lookupPBCTCachedOwner_cache = null;
state_0 = state_0 & 0xfffffff4 /* remove SpecializationActive[LookupAttributeInMRONode.lookupPBCTCached(PythonBuiltinClassType, PythonBuiltinClassType, Object)], SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedMulti(PythonBuiltinClassType, PythonBuiltinClassType, Object)], SpecializationActive[LookupAttributeInMRONode.lookupPBCTCachedOwner(PythonBuiltinClassType, PythonBuiltinClassType, PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[LookupAttributeInMRONode.lookupPBCTGeneric(PythonBuiltinClassType, ReadAttributeFromDynamicObjectNode)] */;
this.state_0_ = state_0;
return lookupPBCTGeneric(arg0Value_, readAttrNode_1);
}
if (((state_0 & 0b1100000)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] && SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */ && (isSingleContext())) {
while (true) {
int count4_ = 0;
LookupConstantMROCachedData s4_ = LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER.getVolatile(this);
LookupConstantMROCachedData s4_original = s4_;
while (s4_ != null) {
if ((isSameType(s4_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s4_.cachedAttrInMROInfo_ != null));
if (Assumption.isValidAssumption((s4_.cachedAttrInMROInfo_.assumption))) {
break;
}
}
count4_++;
s4_ = s4_.next_;
}
if (s4_ == null) {
{
Object cachedKlass__1 = (arg0Value);
if ((isSameType(cachedKlass__1, arg0Value))) {
AttributeAssumptionPair cachedAttrInMROInfo__ = (findAttrAndAssumptionInMRO(cachedKlass__1));
if ((cachedAttrInMROInfo__ != null)) {
Assumption assumption0 = (cachedAttrInMROInfo__.assumption);
if (Assumption.isValidAssumption(assumption0)) {
if (count4_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s4_ = new LookupConstantMROCachedData(s4_original);
s4_.cachedKlass_ = cachedKlass__1;
s4_.cachedAttrInMROInfo_ = cachedAttrInMROInfo__;
if (!LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER.compareAndSet(this, s4_original, s4_)) {
continue;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[LookupAttributeInMRONode.lookupConstantMROCached(Object, Object, AttributeAssumptionPair)] */;
this.state_0_ = state_0;
}
}
}
}
}
}
if (s4_ != null) {
return LookupAttributeInMRONode.lookupConstantMROCached(arg0Value, s4_.cachedKlass_, s4_.cachedAttrInMROInfo_);
}
break;
}
}
if (((state_0 & 0b101100000)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] && SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] && SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(isSingleContext()))) {
while (true) {
int count5_ = 0;
LookupConstantMROShapeData s5_ = LOOKUP_CONSTANT_M_R_O_SHAPE_CACHE_UPDATER.getVolatile(this);
LookupConstantMROShapeData s5_original = s5_;
while (s5_ != null) {
assert DSLSupport.assertIdempotence((s5_.cachedMroShape_ != null));
if ((arg0Value_.getMroShape() == s5_.cachedMroShape_)) {
break;
}
count5_++;
s5_ = s5_.next_;
}
if (s5_ == null) {
{
MroShape cachedMroShape__ = (arg0Value_.getMroShape());
if ((cachedMroShape__ != null) && (arg0Value_.getMroShape() == cachedMroShape__) && count5_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s5_ = this.insert(new LookupConstantMROShapeData(s5_original));
s5_.cachedMroShape_ = cachedMroShape__;
s5_.lookupResult_ = s5_.insert((lookupInMroShape(cachedMroShape__, arg0Value_)));
if (!LOOKUP_CONSTANT_M_R_O_SHAPE_CACHE_UPDATER.compareAndSet(this, s5_original, s5_)) {
continue;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[LookupAttributeInMRONode.lookupConstantMROShape(PythonClass, MroShape, MroShapeLookupResult)] */;
this.state_0_ = state_0;
}
}
}
if (s5_ != null) {
return lookupConstantMROShape(arg0Value_, s5_.cachedMroShape_, s5_.lookupResult_);
}
break;
}
}
}
if (((state_0 & 0b1100000)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] && SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */ && (isSingleContext())) {
while (true) {
int count6_ = 0;
LookupConstantMROData s6_ = LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.getVolatile(this);
LookupConstantMROData s6_original = s6_;
while (s6_ != null) {
if ((isSameType(s6_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s6_.mroLength_ < 32));
if (Assumption.isValidAssumption((s6_.lookupStable_))) {
break;
}
}
count6_++;
s6_ = s6_.next_;
}
if (s6_ == null) {
{
Object cachedKlass__2 = (arg0Value);
if ((isSameType(cachedKlass__2, arg0Value))) {
MroSequenceStorage mro__1 = (getMro(cachedKlass__2));
int mroLength__1 = (mro__1.length());
if ((mroLength__1 < 32)) {
Assumption lookupStable__ = (mro__1.getLookupStableAssumption());
Assumption assumption0 = (lookupStable__);
if (Assumption.isValidAssumption(assumption0)) {
if (count6_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s6_ = this.insert(new LookupConstantMROData(s6_original));
s6_.cachedKlass_ = cachedKlass__2;
s6_.mro_ = mro__1;
s6_.lookupStable_ = lookupStable__;
s6_.mroLength_ = mroLength__1;
DynamicObjectLibrary dylib__ = s6_.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(dylib__, "Specialization 'lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])' cache 'dylib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.dylib_ = dylib__;
ReadAttributeFromObjectNode[] readAttrNodes__ = s6_.insert((LookupAttributeInMRONode.create(mroLength__1)));
Objects.requireNonNull(readAttrNodes__, "Specialization 'lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])' cache 'readAttrNodes' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.readAttrNodes_ = readAttrNodes__;
if (!LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.compareAndSet(this, s6_original, s6_)) {
continue;
}
this.lookupConstantMROShape_cache = null;
state_0 = state_0 & 0xffffff7f /* remove SpecializationActive[LookupAttributeInMRONode.lookupConstantMROShape(PythonClass, MroShape, MroShapeLookupResult)] */;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] */;
this.state_0_ = state_0;
}
}
}
}
}
}
if (s6_ != null) {
return lookupConstantMRO(arg0Value, s6_.cachedKlass_, s6_.mro_, s6_.lookupStable_, s6_.mroLength_, s6_.dylib_, s6_.readAttrNodes_);
}
break;
}
}
{
int mroLength__ = 0;
MroSequenceStorage mro__ = null;
if (((state_0 & 0b1000000)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */) {
while (true) {
int count7_ = 0;
LookupCachedLenData s7_ = LOOKUP_CACHED_LEN_CACHE_UPDATER.getVolatile(this);
LookupCachedLenData s7_original = s7_;
while (s7_ != null) {
{
mro__ = (getMro(arg0Value));
mroLength__ = (mro__.length());
if ((mroLength__ == s7_.cachedMroLength_)) {
assert DSLSupport.assertIdempotence((s7_.cachedMroLength_ < 32));
break;
}
}
count7_++;
s7_ = s7_.next_;
}
if (s7_ == null) {
{
mro__ = (getMro(arg0Value));
mroLength__ = (mro__.length());
int cachedMroLength__ = (mro__.length());
if ((mroLength__ == cachedMroLength__) && (cachedMroLength__ < 32) && count7_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s7_ = this.insert(new LookupCachedLenData(s7_original));
s7_.cachedMroLength_ = cachedMroLength__;
DynamicObjectLibrary dylib__1 = s7_.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(dylib__1, "Specialization 'lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])' cache 'dylib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s7_.dylib_ = dylib__1;
ReadAttributeFromObjectNode[] readAttrNodes__1 = s7_.insert((LookupAttributeInMRONode.create(cachedMroLength__)));
Objects.requireNonNull(readAttrNodes__1, "Specialization 'lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])' cache 'readAttrNodes' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s7_.readAttrNodes_ = readAttrNodes__1;
if (!LOOKUP_CACHED_LEN_CACHE_UPDATER.compareAndSet(this, s7_original, s7_)) {
continue;
}
this.lookupConstantMROCached_cache = null;
this.lookupConstantMROShape_cache = null;
this.lookupConstantMRO_cache = null;
state_0 = state_0 & 0xfffffe6f /* remove SpecializationActive[LookupAttributeInMRONode.lookupConstantMROCached(Object, Object, AttributeAssumptionPair)], SpecializationActive[LookupAttributeInMRONode.lookupConstantMROShape(PythonClass, MroShape, MroShapeLookupResult)], SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] */;
state_0 = state_0 | 0b100000 /* add SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] */;
this.state_0_ = state_0;
}
}
}
if (s7_ != null) {
return lookupCachedLen(arg0Value, mro__, mroLength__, s7_.cachedMroLength_, s7_.dylib_, s7_.readAttrNodes_);
}
break;
}
}
}
LookupGenericData s8_ = this.insert(new LookupGenericData());
DynamicObjectLibrary dylib__2 = s8_.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(dylib__2, "Specialization 'lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)' cache 'dylib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s8_.dylib_ = dylib__2;
ReadAttributeFromObjectNode readAttrNode__ = s8_.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readAttrNode__, "Specialization 'lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)' cache 'readAttrNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s8_.readAttrNode_ = readAttrNode__;
VarHandle.storeStoreFence();
this.lookupGeneric_cache = s8_;
this.lookupConstantMROCached_cache = null;
this.lookupConstantMROShape_cache = null;
this.lookupConstantMRO_cache = null;
this.lookupCachedLen_cache = null;
state_0 = state_0 & 0xfffffe4f /* remove SpecializationActive[LookupAttributeInMRONode.lookupConstantMROCached(Object, Object, AttributeAssumptionPair)], SpecializationActive[LookupAttributeInMRONode.lookupConstantMROShape(PythonClass, MroShape, MroShapeLookupResult)], SpecializationActive[LookupAttributeInMRONode.lookupConstantMRO(Object, Object, MroSequenceStorage, Assumption, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])], SpecializationActive[LookupAttributeInMRONode.lookupCachedLen(Object, MroSequenceStorage, int, int, DynamicObjectLibrary, ReadAttributeFromObjectNode[])] */;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[LookupAttributeInMRONode.lookupGeneric(Object, DynamicObjectLibrary, ReadAttributeFromObjectNode)] */;
this.state_0_ = state_0;
return lookupGeneric(arg0Value, dylib__2, readAttrNode__);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
if (((oldState_0 & 0b1000000) == 0 && (state_0_ & 0b1000000) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
LookupPBCTCachedData s0_ = this.lookupPBCTCached_cache;
LookupPBCTCachedMultiData s1_ = this.lookupPBCTCachedMulti_cache;
LookupPBCTCachedOwnerData s2_ = this.lookupPBCTCachedOwner_cache;
LookupConstantMROCachedData s4_ = this.lookupConstantMROCached_cache;
LookupConstantMROShapeData s5_ = this.lookupConstantMROShape_cache;
LookupConstantMROData s6_ = this.lookupConstantMRO_cache;
LookupCachedLenData s7_ = this.lookupCachedLen_cache;
if ((s0_ == null || s0_.next_ == null) && (s1_ == null || s1_.next_ == null) && (s2_ == null || s2_.next_ == null) && (s4_ == null || s4_.next_ == null) && (s5_ == null || s5_.next_ == null) && (s6_ == null || s6_.next_ == null) && (s7_ == null || s7_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
void removeLookupConstantMROCached_(LookupConstantMROCachedData s4_) {
while (true) {
LookupConstantMROCachedData cur = this.lookupConstantMROCached_cache;
LookupConstantMROCachedData original = cur;
LookupConstantMROCachedData update = null;
while (cur != null) {
if (cur == s4_) {
if (cur == original) {
update = cur.next_;
} else {
update = original.remove(s4_);
}
break;
}
cur = cur.next_;
}
if (cur != null && !LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER.compareAndSet(this, original, update)) {
continue;
}
break;
}
}
void removeLookupConstantMRO_(LookupConstantMROData s6_) {
while (true) {
LookupConstantMROData cur = this.lookupConstantMRO_cache;
LookupConstantMROData original = cur;
LookupConstantMROData update = null;
while (cur != null) {
if (cur == s6_) {
if (cur == original) {
update = cur.next_;
} else {
update = original.remove(this, s6_);
}
break;
}
cur = cur.next_;
}
if (cur != null && !LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.compareAndSet(this, original, update)) {
continue;
}
break;
}
}
@NeverDefault
public static LookupAttributeInMRONode create(TruffleString key, boolean skipNonStaticBases) {
return new LookupAttributeInMRONodeGen(key, skipNonStaticBases);
}
private static PythonBuiltinClassType decodePythonBuiltinClassType(int state) {
if (state >= 0) {
return PYTHON_BUILTIN_CLASS_TYPE_VALUES[state];
} else {
return null;
}
}
private static int encodePythonBuiltinClassType(PythonBuiltinClassType e) {
if (e != null) {
return e.ordinal();
} else {
return -1;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupPBCTCachedData implements SpecializationDataNode {
@CompilationFinal final LookupPBCTCachedData next_;
/**
* State Info:
* 0-8: EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCached(..., PythonBuiltinClassType cachedKlass, ...)]
*
*/
@CompilationFinal private int lookupPBCTCached_state_0_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupPBCTCached}
* Parameter: {@link Object} cachedValue
*/
@CompilationFinal Object cachedValue_;
LookupPBCTCachedData(LookupPBCTCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupPBCTCachedMultiData implements SpecializationDataNode {
@CompilationFinal final LookupPBCTCachedMultiData next_;
/**
* State Info:
* 0-8: EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedMulti(..., PythonBuiltinClassType cachedKlass, ...)]
*
*/
@CompilationFinal private int lookupPBCTCachedMulti_state_0_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupPBCTCachedMulti}
* Parameter: {@link Object} cachedValue
*/
@CompilationFinal Object cachedValue_;
LookupPBCTCachedMultiData(LookupPBCTCachedMultiData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupPBCTCachedOwnerData implements SpecializationDataNode {
@CompilationFinal final LookupPBCTCachedOwnerData next_;
/**
* State Info:
* 0-8: EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType cachedKlass, ...)]
* 9-17: EncodedEnum[cache=LookupAttributeInMRONode.lookupPBCTCachedOwner(..., PythonBuiltinClassType ownerKlass, ...)]
*
*/
@CompilationFinal private int lookupPBCTCachedOwner_state_0_;
LookupPBCTCachedOwnerData(LookupPBCTCachedOwnerData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupConstantMROCachedData implements SpecializationDataNode {
@CompilationFinal final LookupConstantMROCachedData next_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMROCached}
* Parameter: {@link Object} cachedKlass
*/
@CompilationFinal Object cachedKlass_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMROCached}
* Parameter: {@link AttributeAssumptionPair} cachedAttrInMROInfo
*/
@CompilationFinal AttributeAssumptionPair cachedAttrInMROInfo_;
LookupConstantMROCachedData(LookupConstantMROCachedData next_) {
this.next_ = next_;
}
LookupConstantMROCachedData remove(LookupConstantMROCachedData search) {
LookupConstantMROCachedData newNext = this.next_;
if (newNext != null) {
if (search == newNext) {
newNext = newNext.next_;
} else {
newNext = newNext.remove(search);
}
}
LookupConstantMROCachedData copy = new LookupConstantMROCachedData(newNext);
copy.cachedKlass_ = this.cachedKlass_;
copy.cachedAttrInMROInfo_ = this.cachedAttrInMROInfo_;
return copy;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupConstantMROShapeData extends Node implements SpecializationDataNode {
@Child LookupConstantMROShapeData next_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMROShape}
* Parameter: {@link MroShape} cachedMroShape
*/
@CompilationFinal MroShape cachedMroShape_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMROShape}
* Parameter: {@link MroShapeLookupResult} lookupResult
*/
@Child MroShapeLookupResult lookupResult_;
LookupConstantMROShapeData(LookupConstantMROShapeData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupConstantMROData extends Node implements SpecializationDataNode {
@Child LookupConstantMROData next_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMRO}
* Parameter: {@link Object} cachedKlass
*/
@CompilationFinal Object cachedKlass_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMRO}
* Parameter: {@link MroSequenceStorage} mro
*/
@CompilationFinal MroSequenceStorage mro_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMRO}
* Parameter: {@link Assumption} lookupStable
*/
@CompilationFinal Assumption lookupStable_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMRO}
* Parameter: int mroLength
*/
@CompilationFinal int mroLength_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMRO}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child DynamicObjectLibrary dylib_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupConstantMRO}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNodes
*/
@Children ReadAttributeFromObjectNode[] readAttrNodes_;
LookupConstantMROData(LookupConstantMROData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
LookupConstantMROData remove(Node parent, LookupConstantMROData search) {
LookupConstantMROData newNext = this.next_;
if (newNext != null) {
if (search == newNext) {
newNext = newNext.next_;
} else {
newNext = newNext.remove(this, search);
}
}
LookupConstantMROData copy = parent.insert(new LookupConstantMROData(newNext));
copy.cachedKlass_ = this.cachedKlass_;
copy.mro_ = this.mro_;
copy.lookupStable_ = this.lookupStable_;
copy.mroLength_ = this.mroLength_;
copy.dylib_ = copy.insert(this.dylib_);
copy.readAttrNodes_ = copy.insert(this.readAttrNodes_);
return copy;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupCachedLenData extends Node implements SpecializationDataNode {
@Child LookupCachedLenData next_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupCachedLen}
* Parameter: int cachedMroLength
*/
@CompilationFinal int cachedMroLength_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupCachedLen}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child DynamicObjectLibrary dylib_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupCachedLen}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNodes
*/
@Children ReadAttributeFromObjectNode[] readAttrNodes_;
LookupCachedLenData(LookupCachedLenData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(LookupAttributeInMRONode.class)
@DenyReplace
private static final class LookupGenericData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupGeneric}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child DynamicObjectLibrary dylib_;
/**
* Source Info:
* Specialization: {@link LookupAttributeInMRONode#lookupGeneric}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNode
*/
@Child ReadAttributeFromObjectNode readAttrNode_;
LookupGenericData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
/**
* Debug Info:
* Specialization {@link Dynamic#lookupConstantMRO}
* Activation probability: 0.48333
* With/without class size: 19/12 bytes
* Specialization {@link Dynamic#lookupInBuiltinType}
* Activation probability: 0.33333
* With/without class size: 10/4 bytes
* Specialization {@link Dynamic#lookupGeneric}
* Activation probability: 0.18333
* With/without class size: 9/9 bytes
*
*/
@GeneratedBy(Dynamic.class)
@SuppressWarnings("javadoc")
public static final class DynamicNodeGen extends Dynamic {
private static final StateField STATE_0_Dynamic_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMRO_cache", LookupConstantMROData.class);
/**
* Source Info:
* Specialization: {@link Dynamic#lookupGeneric}
* Parameter: {@link GetMroStorageNode} getMroNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*/
private static final GetMroStorageNode INLINED_LOOKUP_GENERIC_GET_MRO_NODE_ = GetMroStorageNodeGen.inline(InlineTarget.create(GetMroStorageNode.class, STATE_0_Dynamic_UPDATER.subUpdater(3, 8), ReferenceField.create(MethodHandles.lookup(), "lookupGeneric_getMroNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link Dynamic#lookupConstantMRO}
* 1: SpecializationActive {@link Dynamic#lookupInBuiltinType}
* 2: SpecializationActive {@link Dynamic#lookupGeneric}
* 3-10: InlinedCache
* Specialization: {@link Dynamic#lookupGeneric}
* Parameter: {@link GetMroStorageNode} getMroNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
@UnsafeAccessedField @Child private LookupConstantMROData lookupConstantMRO_cache;
/**
* Source Info:
* Specialization: {@link Dynamic#lookupInBuiltinType}
* Parameter: {@link ReadAttributeFromDynamicObjectNode} readAttrNode
*/
@Child private ReadAttributeFromDynamicObjectNode lookupInBuiltinType_readAttrNode_;
/**
* Source Info:
* Specialization: {@link Dynamic#lookupGeneric}
* Parameter: {@link GetMroStorageNode} getMroNode
* Inline method: {@link GetMroStorageNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupGeneric_getMroNode__field1_;
/**
* Source Info:
* Specialization: {@link Dynamic#lookupGeneric}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNode
*/
@Child private ReadAttributeFromObjectNode lookupGeneric_readAttrNode_;
private DynamicNodeGen() {
}
@ExplodeLoop
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)] || SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupInBuiltinType(PythonBuiltinClassType, Object, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupGeneric(Object, Object, Node, GetMroStorageNode, ReadAttributeFromObjectNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
LookupConstantMROData s0_ = this.lookupConstantMRO_cache;
while (s0_ != null) {
if ((PGuards.stringEquals(arg1Value_, s0_.cachedKey_, s0_.equalNode_))) {
return Dynamic.lookupConstantMRO(arg0Value, arg1Value_, s0_.cachedKey_, s0_.equalNode_, s0_.lookup_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupInBuiltinType(PythonBuiltinClassType, Object, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupGeneric(Object, Object, Node, GetMroStorageNode, ReadAttributeFromObjectNode)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupInBuiltinType(PythonBuiltinClassType, Object, ReadAttributeFromDynamicObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
ReadAttributeFromDynamicObjectNode readAttrNode__ = this.lookupInBuiltinType_readAttrNode_;
if (readAttrNode__ != null) {
return lookupInBuiltinType(arg0Value_, arg1Value, readAttrNode__);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupGeneric(Object, Object, Node, GetMroStorageNode, ReadAttributeFromObjectNode)] */) {
{
ReadAttributeFromObjectNode readAttrNode__1 = this.lookupGeneric_readAttrNode_;
if (readAttrNode__1 != null) {
Node inliningTarget__ = (this);
return Dynamic.lookupGeneric(arg0Value, arg1Value, inliningTarget__, INLINED_LOOKUP_GENERIC_GET_MRO_NODE_, readAttrNode__1);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b110)) == 0 /* is-not SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupInBuiltinType(PythonBuiltinClassType, Object, ReadAttributeFromDynamicObjectNode)] && SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupGeneric(Object, Object, Node, GetMroStorageNode, ReadAttributeFromObjectNode)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
while (true) {
int count0_ = 0;
LookupConstantMROData s0_ = LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.getVolatile(this);
LookupConstantMROData s0_original = s0_;
while (s0_ != null) {
if ((PGuards.stringEquals(arg1Value_, s0_.cachedKey_, s0_.equalNode_))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
TruffleString cachedKey__ = (arg1Value_);
EqualNode equalNode__ = this.insert((EqualNode.create()));
if ((PGuards.stringEquals(arg1Value_, cachedKey__, equalNode__)) && count0_ < (2)) {
s0_ = this.insert(new LookupConstantMROData(s0_original));
s0_.cachedKey_ = cachedKey__;
Objects.requireNonNull(s0_.insert(equalNode__), "Specialization 'lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)' cache 'equalNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.equalNode_ = equalNode__;
LookupAttributeInMRONode lookup__ = s0_.insert((LookupAttributeInMRONode.create(arg1Value_)));
Objects.requireNonNull(lookup__, "Specialization 'lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)' cache 'lookup' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.lookup_ = lookup__;
if (!LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return Dynamic.lookupConstantMRO(arg0Value, arg1Value_, s0_.cachedKey_, s0_.equalNode_, s0_.lookup_);
}
break;
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
ReadAttributeFromDynamicObjectNode readAttrNode__ = this.insert((ReadAttributeFromDynamicObjectNode.create()));
Objects.requireNonNull(readAttrNode__, "Specialization 'lookupInBuiltinType(PythonBuiltinClassType, Object, ReadAttributeFromDynamicObjectNode)' cache 'readAttrNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupInBuiltinType_readAttrNode_ = readAttrNode__;
this.lookupConstantMRO_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupInBuiltinType(PythonBuiltinClassType, Object, ReadAttributeFromDynamicObjectNode)] */;
this.state_0_ = state_0;
return lookupInBuiltinType(arg0Value_, arg1Value, readAttrNode__);
}
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
ReadAttributeFromObjectNode readAttrNode__1 = this.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readAttrNode__1, "Specialization 'lookupGeneric(Object, Object, Node, GetMroStorageNode, ReadAttributeFromObjectNode)' cache 'readAttrNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupGeneric_readAttrNode_ = readAttrNode__1;
this.lookupConstantMRO_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupConstantMRO(Object, TruffleString, TruffleString, EqualNode, LookupAttributeInMRONode)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[LookupAttributeInMRONode.Dynamic.lookupGeneric(Object, Object, Node, GetMroStorageNode, ReadAttributeFromObjectNode)] */;
this.state_0_ = state_0;
return Dynamic.lookupGeneric(arg0Value, arg1Value, inliningTarget__, INLINED_LOOKUP_GENERIC_GET_MRO_NODE_, readAttrNode__1);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b111) & ((state_0 & 0b111) - 1)) == 0 /* is-single */) {
LookupConstantMROData s0_ = this.lookupConstantMRO_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static Dynamic create() {
return new DynamicNodeGen();
}
@NeverDefault
public static Dynamic getUncached() {
return DynamicNodeGen.UNCACHED;
}
@GeneratedBy(Dynamic.class)
@DenyReplace
private static final class LookupConstantMROData extends Node implements SpecializationDataNode {
@Child LookupConstantMROData next_;
/**
* Source Info:
* Specialization: {@link Dynamic#lookupConstantMRO}
* Parameter: {@link TruffleString} cachedKey
*/
@CompilationFinal TruffleString cachedKey_;
/**
* Source Info:
* Specialization: {@link Dynamic#lookupConstantMRO}
* Parameter: {@link EqualNode} equalNode
*/
@Child EqualNode equalNode_;
/**
* Source Info:
* Specialization: {@link Dynamic#lookupConstantMRO}
* Parameter: {@link LookupAttributeInMRONode} lookup
*/
@Child LookupAttributeInMRONode lookup_;
LookupConstantMROData(LookupConstantMROData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(Dynamic.class)
@DenyReplace
private static final class Uncached extends Dynamic {
@TruffleBoundary
@Override
public Object execute(Object arg0Value, Object arg1Value) {
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
return lookupInBuiltinType(arg0Value_, arg1Value, (ReadAttributeFromDynamicObjectNode.getUncached()));
}
return Dynamic.lookupGeneric(arg0Value, arg1Value, (this), (GetMroStorageNode.getUncached()), (ReadAttributeFromObjectNode.getUncachedForceType()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy