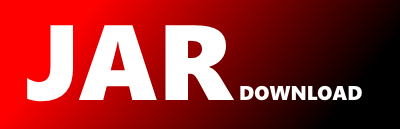
com.oracle.graal.python.nodes.attributes.LookupNativeSlotNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.attributes;
import com.oracle.graal.python.builtins.objects.cext.capi.SlotMethodDef;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccess.ReadPointerNode;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccessFactory.ReadPointerNodeGen;
import com.oracle.graal.python.builtins.objects.type.MroShape;
import com.oracle.graal.python.builtins.objects.type.PythonClass;
import com.oracle.graal.python.builtins.objects.type.PythonManagedClass;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.builtins.objects.type.MroShape.MroShapeLookupResult;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.GetMroStorageNode;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.IsSameTypeNode;
import com.oracle.graal.python.builtins.objects.type.TypeNodesFactory.GetMroStorageNodeGen;
import com.oracle.graal.python.builtins.objects.type.TypeNodesFactory.IsSameTypeNodeGen;
import com.oracle.graal.python.nodes.attributes.LookupAttributeInMRONode.AttributeAssumptionPair;
import com.oracle.graal.python.runtime.PythonOptions;
import com.oracle.graal.python.runtime.sequence.storage.MroSequenceStorage;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link LookupNativeSlotNode#lookupConstantMROCached}
* Activation probability: 0.32000
* With/without class size: 15/13 bytes
* Specialization {@link LookupNativeSlotNode#lookupConstantMROShape}
* Activation probability: 0.26000
* With/without class size: 13/13 bytes
* Specialization {@link LookupNativeSlotNode#lookupConstantMRO}
* Activation probability: 0.20000
* With/without class size: 14/29 bytes
* Specialization {@link LookupNativeSlotNode#lookupCachedLen}
* Activation probability: 0.14000
* With/without class size: 9/17 bytes
* Specialization {@link LookupNativeSlotNode#lookupGeneric}
* Activation probability: 0.08000
* With/without class size: 6/13 bytes
*
*/
@GeneratedBy(LookupNativeSlotNode.class)
@SuppressWarnings("javadoc")
public final class LookupNativeSlotNodeGen extends LookupNativeSlotNode {
private static final StateField LOOKUP_CONSTANT_M_R_O_CACHED__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CONSTANT_M_R_O_CACHED_STATE_0_UPDATER = StateField.create(LookupConstantMROCachedData.lookup_(), "lookupConstantMROCached_state_0_");
private static final StateField LOOKUP_CONSTANT_M_R_O_SHAPE__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CONSTANT_M_R_O_SHAPE_STATE_0_UPDATER = StateField.create(LookupConstantMROShapeData.lookup_(), "lookupConstantMROShape_state_0_");
private static final StateField LOOKUP_CONSTANT_M_R_O__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CONSTANT_M_R_O_STATE_0_UPDATER = StateField.create(LookupConstantMROData.lookup_(), "lookupConstantMRO_state_0_");
private static final StateField LOOKUP_CACHED_LEN__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CACHED_LEN_STATE_0_UPDATER = StateField.create(LookupCachedLenData.lookup_(), "lookupCachedLen_state_0_");
private static final StateField LOOKUP_GENERIC__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_GENERIC_STATE_0_UPDATER = StateField.create(LookupGenericData.lookup_(), "lookupGeneric_state_0_");
static final ReferenceField LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMROCached_cache", LookupConstantMROCachedData.class);
static final ReferenceField LOOKUP_CONSTANT_M_R_O_SHAPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMROShape_cache", LookupConstantMROShapeData.class);
static final ReferenceField LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupConstantMRO_cache", LookupConstantMROData.class);
static final ReferenceField LOOKUP_CACHED_LEN_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "lookupCachedLen_cache", LookupCachedLenData.class);
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROCached}
* Parameter: {@link IsSameTypeNode} isSameTypeNode
* Inline method: {@link IsSameTypeNodeGen#inline}
*/
private static final IsSameTypeNode INLINED_LOOKUP_CONSTANT_M_R_O_CACHED_IS_SAME_TYPE_NODE_ = IsSameTypeNodeGen.inline(InlineTarget.create(IsSameTypeNode.class, LOOKUP_CONSTANT_M_R_O_CACHED__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CONSTANT_M_R_O_CACHED_STATE_0_UPDATER.subUpdater(0, 6), ReferenceField.create(LookupConstantMROCachedData.lookup_(), "lookupConstantMROCached_isSameTypeNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROShape}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*/
private static final GetMroStorageNode INLINED_LOOKUP_CONSTANT_M_R_O_SHAPE_GET_MRO_STORAGE_NODE_ = GetMroStorageNodeGen.inline(InlineTarget.create(GetMroStorageNode.class, LOOKUP_CONSTANT_M_R_O_SHAPE__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CONSTANT_M_R_O_SHAPE_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(LookupConstantMROShapeData.lookup_(), "lookupConstantMROShape_getMroStorageNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link IsSameTypeNode} isSameTypeNode
* Inline method: {@link IsSameTypeNodeGen#inline}
*/
private static final IsSameTypeNode INLINED_LOOKUP_CONSTANT_M_R_O_IS_SAME_TYPE_NODE_ = IsSameTypeNodeGen.inline(InlineTarget.create(IsSameTypeNode.class, LOOKUP_CONSTANT_M_R_O__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CONSTANT_M_R_O_STATE_0_UPDATER.subUpdater(0, 6), ReferenceField.create(LookupConstantMROData.lookup_(), "lookupConstantMRO_isSameTypeNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupCachedLen}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*/
private static final GetMroStorageNode INLINED_LOOKUP_CACHED_LEN_GET_MRO_STORAGE_NODE_ = GetMroStorageNodeGen.inline(InlineTarget.create(GetMroStorageNode.class, LOOKUP_CACHED_LEN__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_CACHED_LEN_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(LookupCachedLenData.lookup_(), "lookupCachedLen_getMroStorageNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupGeneric}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*/
private static final GetMroStorageNode INLINED_LOOKUP_GENERIC_GET_MRO_STORAGE_NODE_ = GetMroStorageNodeGen.inline(InlineTarget.create(GetMroStorageNode.class, LOOKUP_GENERIC__LOOKUP_NATIVE_SLOT_NODE_LOOKUP_GENERIC_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(LookupGenericData.lookup_(), "lookupGeneric_getMroStorageNode__field1_", Node.class)));
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link LookupNativeSlotNode#lookupConstantMROCached}
* 1: SpecializationActive {@link LookupNativeSlotNode#lookupCachedLen}
* 2: SpecializationActive {@link LookupNativeSlotNode#lookupGeneric}
* 3: SpecializationActive {@link LookupNativeSlotNode#lookupConstantMROShape}
* 4: SpecializationActive {@link LookupNativeSlotNode#lookupConstantMRO}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link ReadPointerNode} readPointerNode
*/
@Child private ReadPointerNode readPointerNode;
@UnsafeAccessedField @Child private LookupConstantMROCachedData lookupConstantMROCached_cache;
@UnsafeAccessedField @Child private LookupConstantMROShapeData lookupConstantMROShape_cache;
@UnsafeAccessedField @Child private LookupConstantMROData lookupConstantMRO_cache;
@UnsafeAccessedField @Child private LookupCachedLenData lookupCachedLen_cache;
@Child private LookupGenericData lookupGeneric_cache;
private LookupNativeSlotNodeGen(SlotMethodDef slot) {
super(slot);
}
@ExplodeLoop
@Override
public Object execute(PythonManagedClass arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupConstantMROCached(PythonManagedClass, Node, IsSameTypeNode, Object, AttributeAssumptionPair)] || SpecializationActive[LookupNativeSlotNode.lookupConstantMROShape(PythonClass, Node, GetMroStorageNode, MroShape, MroShapeLookupResult)] || SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] || SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] || SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupConstantMROCached(PythonManagedClass, Node, IsSameTypeNode, Object, AttributeAssumptionPair)] */) {
assert DSLSupport.assertIdempotence((isSingleContext()));
LookupConstantMROCachedData s0_ = this.lookupConstantMROCached_cache;
while (s0_ != null) {
if (!Assumption.isValidAssumption((s0_.cachedAttrInMROInfo_.assumption))) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeLookupConstantMROCached_(s0_);
return executeAndSpecialize(arg0Value);
}
{
Node inliningTarget__ = (s0_);
if ((INLINED_LOOKUP_CONSTANT_M_R_O_CACHED_IS_SAME_TYPE_NODE_.execute(inliningTarget__, s0_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s0_.cachedAttrInMROInfo_ != null));
return LookupNativeSlotNode.lookupConstantMROCached(arg0Value, inliningTarget__, INLINED_LOOKUP_CONSTANT_M_R_O_CACHED_IS_SAME_TYPE_NODE_, s0_.cachedKlass_, s0_.cachedAttrInMROInfo_);
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupConstantMROShape(PythonClass, Node, GetMroStorageNode, MroShape, MroShapeLookupResult)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
assert DSLSupport.assertIdempotence((!(isSingleContext())));
LookupConstantMROShapeData s1_ = this.lookupConstantMROShape_cache;
while (s1_ != null) {
assert DSLSupport.assertIdempotence((s1_.cachedMroShape_ != null));
if ((arg0Value_.getMroShape() == s1_.cachedMroShape_)) {
Node inliningTarget__1 = (s1_);
return lookupConstantMROShape(arg0Value_, inliningTarget__1, INLINED_LOOKUP_CONSTANT_M_R_O_SHAPE_GET_MRO_STORAGE_NODE_, s1_.cachedMroShape_, s1_.lookupResult_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b10110) != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] || SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] || SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */) {
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] */) {
assert DSLSupport.assertIdempotence((isSingleContext()));
LookupConstantMROData s2_ = this.lookupConstantMRO_cache;
while (s2_ != null) {
if (!Assumption.isValidAssumption((s2_.lookupStable_))) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeLookupConstantMRO_(s2_);
return executeAndSpecialize(arg0Value);
}
{
ReadPointerNode readPointerNode_ = this.readPointerNode;
if (readPointerNode_ != null) {
Node inliningTarget__2 = (s2_);
if ((INLINED_LOOKUP_CONSTANT_M_R_O_IS_SAME_TYPE_NODE_.execute(inliningTarget__2, s2_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s2_.mroLength_ < 32));
return lookupConstantMRO(arg0Value, inliningTarget__2, INLINED_LOOKUP_CONSTANT_M_R_O_IS_SAME_TYPE_NODE_, s2_.cachedKlass_, s2_.mro_, s2_.lookupStable_, s2_.mroLength_, s2_.readAttrNodes_, readPointerNode_, s2_.interopLibrary_);
}
}
}
s2_ = s2_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] */) {
LookupCachedLenData s3_ = this.lookupCachedLen_cache;
while (s3_ != null) {
{
ReadPointerNode readPointerNode_1 = this.readPointerNode;
if (readPointerNode_1 != null) {
Node inliningTarget__3 = (s3_);
MroSequenceStorage mro__ = (INLINED_LOOKUP_CACHED_LEN_GET_MRO_STORAGE_NODE_.execute(inliningTarget__3, arg0Value));
int mroLength__ = (mro__.length());
if ((mroLength__ == s3_.cachedMroLength_)) {
assert DSLSupport.assertIdempotence((s3_.cachedMroLength_ < 32));
return lookupCachedLen(arg0Value, inliningTarget__3, INLINED_LOOKUP_CACHED_LEN_GET_MRO_STORAGE_NODE_, mro__, mroLength__, s3_.cachedMroLength_, s3_.readAttrNodes_, readPointerNode_1, s3_.interopLibrary_);
}
}
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */) {
LookupGenericData s4_ = this.lookupGeneric_cache;
if (s4_ != null) {
{
ReadPointerNode readPointerNode_2 = this.readPointerNode;
if (readPointerNode_2 != null) {
Node inliningTarget__4 = (s4_);
return lookupGeneric(arg0Value, inliningTarget__4, INLINED_LOOKUP_GENERIC_GET_MRO_STORAGE_NODE_, s4_.readAttrNode_, readPointerNode_2, s4_.interopLibrary_);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(PythonManagedClass arg0Value) {
int state_0 = this.state_0_;
int oldState_0 = state_0;
try {
{
Node inliningTarget__ = null;
if (((state_0 & 0b110)) == 0 /* is-not SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] && SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */ && (isSingleContext())) {
while (true) {
int count0_ = 0;
LookupConstantMROCachedData s0_ = LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER.getVolatile(this);
LookupConstantMROCachedData s0_original = s0_;
while (s0_ != null) {
{
inliningTarget__ = (s0_);
if ((INLINED_LOOKUP_CONSTANT_M_R_O_CACHED_IS_SAME_TYPE_NODE_.execute(inliningTarget__, s0_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s0_.cachedAttrInMROInfo_ != null));
if (Assumption.isValidAssumption((s0_.cachedAttrInMROInfo_.assumption))) {
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
s0_ = this.insert(new LookupConstantMROCachedData(s0_original));
inliningTarget__ = (s0_);
Object cachedKlass__ = (arg0Value);
if ((INLINED_LOOKUP_CONSTANT_M_R_O_CACHED_IS_SAME_TYPE_NODE_.execute(inliningTarget__, cachedKlass__, arg0Value))) {
AttributeAssumptionPair cachedAttrInMROInfo__ = (findAttrAndAssumptionInMRO(cachedKlass__));
if ((cachedAttrInMROInfo__ != null)) {
Assumption assumption0 = (cachedAttrInMROInfo__.assumption);
if (Assumption.isValidAssumption(assumption0)) {
if (count0_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s0_.cachedKlass_ = cachedKlass__;
s0_.cachedAttrInMROInfo_ = cachedAttrInMROInfo__;
if (!LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[LookupNativeSlotNode.lookupConstantMROCached(PythonManagedClass, Node, IsSameTypeNode, Object, AttributeAssumptionPair)] */;
this.state_0_ = state_0;
} else {
s0_ = null;
}
} else {
s0_ = null;
}
} else {
s0_ = null;
}
} else {
s0_ = null;
}
}
}
if (s0_ != null) {
return LookupNativeSlotNode.lookupConstantMROCached(arg0Value, inliningTarget__, INLINED_LOOKUP_CONSTANT_M_R_O_CACHED_IS_SAME_TYPE_NODE_, s0_.cachedKlass_, s0_.cachedAttrInMROInfo_);
}
break;
}
}
}
{
Node inliningTarget__1 = null;
if (((state_0 & 0b10110)) == 0 /* is-not SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] && SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] && SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(isSingleContext()))) {
while (true) {
int count1_ = 0;
LookupConstantMROShapeData s1_ = LOOKUP_CONSTANT_M_R_O_SHAPE_CACHE_UPDATER.getVolatile(this);
LookupConstantMROShapeData s1_original = s1_;
while (s1_ != null) {
assert DSLSupport.assertIdempotence((s1_.cachedMroShape_ != null));
if ((arg0Value_.getMroShape() == s1_.cachedMroShape_)) {
inliningTarget__1 = (s1_);
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
MroShape cachedMroShape__ = (arg0Value_.getMroShape());
if ((cachedMroShape__ != null) && (arg0Value_.getMroShape() == cachedMroShape__) && count1_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s1_ = this.insert(new LookupConstantMROShapeData(s1_original));
inliningTarget__1 = (s1_);
s1_.cachedMroShape_ = cachedMroShape__;
s1_.lookupResult_ = s1_.insert((lookupInMroShape(cachedMroShape__, arg0Value_)));
if (!LOOKUP_CONSTANT_M_R_O_SHAPE_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[LookupNativeSlotNode.lookupConstantMROShape(PythonClass, Node, GetMroStorageNode, MroShape, MroShapeLookupResult)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return lookupConstantMROShape(arg0Value_, inliningTarget__1, INLINED_LOOKUP_CONSTANT_M_R_O_SHAPE_GET_MRO_STORAGE_NODE_, s1_.cachedMroShape_, s1_.lookupResult_);
}
break;
}
}
}
}
{
Node inliningTarget__2 = null;
if (((state_0 & 0b110)) == 0 /* is-not SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] && SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */ && (isSingleContext())) {
while (true) {
int count2_ = 0;
LookupConstantMROData s2_ = LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.getVolatile(this);
LookupConstantMROData s2_original = s2_;
while (s2_ != null) {
{
ReadPointerNode readPointerNode_ = this.readPointerNode;
if (readPointerNode_ != null) {
inliningTarget__2 = (s2_);
if ((INLINED_LOOKUP_CONSTANT_M_R_O_IS_SAME_TYPE_NODE_.execute(inliningTarget__2, s2_.cachedKlass_, arg0Value))) {
assert DSLSupport.assertIdempotence((s2_.mroLength_ < 32));
if (Assumption.isValidAssumption((s2_.lookupStable_))) {
break;
}
}
}
}
count2_++;
s2_ = s2_.next_;
}
if (s2_ == null) {
{
s2_ = this.insert(new LookupConstantMROData(s2_original));
inliningTarget__2 = (s2_);
Object cachedKlass__1 = (arg0Value);
if ((INLINED_LOOKUP_CONSTANT_M_R_O_IS_SAME_TYPE_NODE_.execute(inliningTarget__2, cachedKlass__1, arg0Value))) {
MroSequenceStorage mro__1 = (LookupNativeSlotNode.getMroStorageUncached(cachedKlass__1));
int mroLength__1 = (mro__1.length());
if ((mroLength__1 < 32)) {
Assumption lookupStable__ = (mro__1.getLookupStableAssumption());
Assumption assumption0 = (lookupStable__);
if (Assumption.isValidAssumption(assumption0)) {
if (count2_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s2_.cachedKlass_ = cachedKlass__1;
s2_.mro_ = mro__1;
s2_.lookupStable_ = lookupStable__;
s2_.mroLength_ = mroLength__1;
ReadAttributeFromObjectNode[] readAttrNodes__ = s2_.insert((LookupAttributeInMRONode.create(mroLength__1)));
Objects.requireNonNull(readAttrNodes__, "Specialization 'lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)' cache 'readAttrNodes' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.readAttrNodes_ = readAttrNodes__;
ReadPointerNode readPointerNode_;
ReadPointerNode readPointerNode__shared = this.readPointerNode;
if (readPointerNode__shared != null) {
readPointerNode_ = readPointerNode__shared;
} else {
readPointerNode_ = s2_.insert((ReadPointerNodeGen.create()));
if (readPointerNode_ == null) {
throw new IllegalStateException("Specialization 'lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)' contains a shared cache with name 'readPointerNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readPointerNode == null) {
this.readPointerNode = readPointerNode_;
}
InteropLibrary interopLibrary__ = s2_.insert((INTEROP_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(interopLibrary__, "Specialization 'lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)' cache 'interopLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.interopLibrary_ = interopLibrary__;
if (!LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
this.lookupConstantMROShape_cache = null;
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[LookupNativeSlotNode.lookupConstantMROShape(PythonClass, Node, GetMroStorageNode, MroShape, MroShapeLookupResult)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] */;
this.state_0_ = state_0;
} else {
s2_ = null;
}
} else {
s2_ = null;
}
} else {
s2_ = null;
}
} else {
s2_ = null;
}
}
}
if (s2_ != null) {
return lookupConstantMRO(arg0Value, inliningTarget__2, INLINED_LOOKUP_CONSTANT_M_R_O_IS_SAME_TYPE_NODE_, s2_.cachedKlass_, s2_.mro_, s2_.lookupStable_, s2_.mroLength_, s2_.readAttrNodes_, this.readPointerNode, s2_.interopLibrary_);
}
break;
}
}
}
{
int mroLength__ = 0;
MroSequenceStorage mro__ = null;
Node inliningTarget__3 = null;
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */) {
while (true) {
int count3_ = 0;
LookupCachedLenData s3_ = LOOKUP_CACHED_LEN_CACHE_UPDATER.getVolatile(this);
LookupCachedLenData s3_original = s3_;
while (s3_ != null) {
{
ReadPointerNode readPointerNode_1 = this.readPointerNode;
if (readPointerNode_1 != null) {
inliningTarget__3 = (s3_);
mro__ = (INLINED_LOOKUP_CACHED_LEN_GET_MRO_STORAGE_NODE_.execute(inliningTarget__3, arg0Value));
mroLength__ = (mro__.length());
if ((mroLength__ == s3_.cachedMroLength_)) {
assert DSLSupport.assertIdempotence((s3_.cachedMroLength_ < 32));
break;
}
}
}
count3_++;
s3_ = s3_.next_;
}
if (s3_ == null) {
{
s3_ = this.insert(new LookupCachedLenData(s3_original));
inliningTarget__3 = (s3_);
mro__ = (INLINED_LOOKUP_CACHED_LEN_GET_MRO_STORAGE_NODE_.execute(inliningTarget__3, arg0Value));
mroLength__ = (mro__.length());
int cachedMroLength__ = (mro__.length());
if ((mroLength__ == cachedMroLength__) && (cachedMroLength__ < 32) && count3_ < (PythonOptions.getAttributeAccessInlineCacheMaxDepth())) {
s3_.cachedMroLength_ = cachedMroLength__;
ReadAttributeFromObjectNode[] readAttrNodes__1 = s3_.insert((LookupAttributeInMRONode.create(cachedMroLength__)));
Objects.requireNonNull(readAttrNodes__1, "Specialization 'lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)' cache 'readAttrNodes' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.readAttrNodes_ = readAttrNodes__1;
ReadPointerNode readPointerNode_1;
ReadPointerNode readPointerNode_1_shared = this.readPointerNode;
if (readPointerNode_1_shared != null) {
readPointerNode_1 = readPointerNode_1_shared;
} else {
readPointerNode_1 = s3_.insert((ReadPointerNodeGen.create()));
if (readPointerNode_1 == null) {
throw new IllegalStateException("Specialization 'lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)' contains a shared cache with name 'readPointerNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readPointerNode == null) {
this.readPointerNode = readPointerNode_1;
}
InteropLibrary interopLibrary__1 = s3_.insert((INTEROP_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(interopLibrary__1, "Specialization 'lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)' cache 'interopLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.interopLibrary_ = interopLibrary__1;
if (!LOOKUP_CACHED_LEN_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
this.lookupConstantMROCached_cache = null;
this.lookupConstantMROShape_cache = null;
this.lookupConstantMRO_cache = null;
state_0 = state_0 & 0xffffffe6 /* remove SpecializationActive[LookupNativeSlotNode.lookupConstantMROCached(PythonManagedClass, Node, IsSameTypeNode, Object, AttributeAssumptionPair)], SpecializationActive[LookupNativeSlotNode.lookupConstantMROShape(PythonClass, Node, GetMroStorageNode, MroShape, MroShapeLookupResult)], SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] */;
this.state_0_ = state_0;
} else {
s3_ = null;
}
}
}
if (s3_ != null) {
return lookupCachedLen(arg0Value, inliningTarget__3, INLINED_LOOKUP_CACHED_LEN_GET_MRO_STORAGE_NODE_, mro__, mroLength__, s3_.cachedMroLength_, s3_.readAttrNodes_, this.readPointerNode, s3_.interopLibrary_);
}
break;
}
}
}
{
Node inliningTarget__4 = null;
LookupGenericData s4_ = this.insert(new LookupGenericData());
inliningTarget__4 = (s4_);
ReadAttributeFromObjectNode readAttrNode__ = s4_.insert((ReadAttributeFromObjectNode.createForceType()));
Objects.requireNonNull(readAttrNode__, "Specialization 'lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)' cache 'readAttrNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.readAttrNode_ = readAttrNode__;
ReadPointerNode readPointerNode_2;
ReadPointerNode readPointerNode_2_shared = this.readPointerNode;
if (readPointerNode_2_shared != null) {
readPointerNode_2 = readPointerNode_2_shared;
} else {
readPointerNode_2 = s4_.insert((ReadPointerNodeGen.create()));
if (readPointerNode_2 == null) {
throw new IllegalStateException("Specialization 'lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)' contains a shared cache with name 'readPointerNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readPointerNode == null) {
this.readPointerNode = readPointerNode_2;
}
InteropLibrary interopLibrary__2 = s4_.insert((INTEROP_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(interopLibrary__2, "Specialization 'lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)' cache 'interopLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.interopLibrary_ = interopLibrary__2;
VarHandle.storeStoreFence();
this.lookupGeneric_cache = s4_;
this.lookupConstantMROCached_cache = null;
this.lookupConstantMROShape_cache = null;
this.lookupConstantMRO_cache = null;
this.lookupCachedLen_cache = null;
state_0 = state_0 & 0xffffffe4 /* remove SpecializationActive[LookupNativeSlotNode.lookupConstantMROCached(PythonManagedClass, Node, IsSameTypeNode, Object, AttributeAssumptionPair)], SpecializationActive[LookupNativeSlotNode.lookupConstantMROShape(PythonClass, Node, GetMroStorageNode, MroShape, MroShapeLookupResult)], SpecializationActive[LookupNativeSlotNode.lookupConstantMRO(PythonManagedClass, Node, IsSameTypeNode, Object, MroSequenceStorage, Assumption, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)], SpecializationActive[LookupNativeSlotNode.lookupCachedLen(PythonManagedClass, Node, GetMroStorageNode, MroSequenceStorage, int, int, ReadAttributeFromObjectNode[], ReadPointerNode, InteropLibrary)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[LookupNativeSlotNode.lookupGeneric(PythonManagedClass, Node, GetMroStorageNode, ReadAttributeFromObjectNode, ReadPointerNode, InteropLibrary)] */;
this.state_0_ = state_0;
return lookupGeneric(arg0Value, inliningTarget__4, INLINED_LOOKUP_GENERIC_GET_MRO_STORAGE_NODE_, readAttrNode__, readPointerNode_2, interopLibrary__2);
}
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
if (((oldState_0 & 0b100) == 0 && (state_0_ & 0b100) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
LookupConstantMROCachedData s0_ = this.lookupConstantMROCached_cache;
LookupConstantMROShapeData s1_ = this.lookupConstantMROShape_cache;
LookupConstantMROData s2_ = this.lookupConstantMRO_cache;
LookupCachedLenData s3_ = this.lookupCachedLen_cache;
if ((s0_ == null || s0_.next_ == null) && (s1_ == null || s1_.next_ == null) && (s2_ == null || s2_.next_ == null) && (s3_ == null || s3_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
void removeLookupConstantMROCached_(LookupConstantMROCachedData s0_) {
while (true) {
LookupConstantMROCachedData cur = this.lookupConstantMROCached_cache;
LookupConstantMROCachedData original = cur;
LookupConstantMROCachedData update = null;
while (cur != null) {
if (cur == s0_) {
if (cur == original) {
update = cur.next_;
} else {
update = original.remove(this, s0_);
}
break;
}
cur = cur.next_;
}
if (cur != null && !LOOKUP_CONSTANT_M_R_O_CACHED_CACHE_UPDATER.compareAndSet(this, original, update)) {
continue;
}
break;
}
}
void removeLookupConstantMRO_(LookupConstantMROData s2_) {
while (true) {
LookupConstantMROData cur = this.lookupConstantMRO_cache;
LookupConstantMROData original = cur;
LookupConstantMROData update = null;
while (cur != null) {
if (cur == s2_) {
if (cur == original) {
update = cur.next_;
} else {
update = original.remove(this, s2_);
}
break;
}
cur = cur.next_;
}
if (cur != null && !LOOKUP_CONSTANT_M_R_O_CACHE_UPDATER.compareAndSet(this, original, update)) {
continue;
}
break;
}
}
@NeverDefault
public static LookupNativeSlotNode create(SlotMethodDef slot) {
return new LookupNativeSlotNodeGen(slot);
}
@GeneratedBy(LookupNativeSlotNode.class)
@DenyReplace
private static final class LookupConstantMROCachedData extends Node implements SpecializationDataNode {
@Child LookupConstantMROCachedData next_;
/**
* State Info:
* 0-5: InlinedCache
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROCached}
* Parameter: {@link IsSameTypeNode} isSameTypeNode
* Inline method: {@link IsSameTypeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int lookupConstantMROCached_state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROCached}
* Parameter: {@link IsSameTypeNode} isSameTypeNode
* Inline method: {@link IsSameTypeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupConstantMROCached_isSameTypeNode__field1_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROCached}
* Parameter: {@link Object} cachedKlass
*/
@CompilationFinal Object cachedKlass_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROCached}
* Parameter: {@link AttributeAssumptionPair} cachedAttrInMROInfo
*/
@CompilationFinal AttributeAssumptionPair cachedAttrInMROInfo_;
LookupConstantMROCachedData(LookupConstantMROCachedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
LookupConstantMROCachedData remove(Node parent, LookupConstantMROCachedData search) {
LookupConstantMROCachedData newNext = this.next_;
if (newNext != null) {
if (search == newNext) {
newNext = newNext.next_;
} else {
newNext = newNext.remove(this, search);
}
}
LookupConstantMROCachedData copy = parent.insert(new LookupConstantMROCachedData(newNext));
copy.lookupConstantMROCached_isSameTypeNode__field1_ = copy.insert(this.lookupConstantMROCached_isSameTypeNode__field1_);
copy.cachedKlass_ = this.cachedKlass_;
copy.cachedAttrInMROInfo_ = this.cachedAttrInMROInfo_;
return copy;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(LookupNativeSlotNode.class)
@DenyReplace
private static final class LookupConstantMROShapeData extends Node implements SpecializationDataNode {
@Child LookupConstantMROShapeData next_;
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROShape}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int lookupConstantMROShape_state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROShape}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupConstantMROShape_getMroStorageNode__field1_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROShape}
* Parameter: {@link MroShape} cachedMroShape
*/
@CompilationFinal MroShape cachedMroShape_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMROShape}
* Parameter: {@link MroShapeLookupResult} lookupResult
*/
@Child MroShapeLookupResult lookupResult_;
LookupConstantMROShapeData(LookupConstantMROShapeData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(LookupNativeSlotNode.class)
@DenyReplace
private static final class LookupConstantMROData extends Node implements SpecializationDataNode {
@Child LookupConstantMROData next_;
/**
* State Info:
* 0-5: InlinedCache
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link IsSameTypeNode} isSameTypeNode
* Inline method: {@link IsSameTypeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int lookupConstantMRO_state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link IsSameTypeNode} isSameTypeNode
* Inline method: {@link IsSameTypeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupConstantMRO_isSameTypeNode__field1_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link Object} cachedKlass
*/
@CompilationFinal Object cachedKlass_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link MroSequenceStorage} mro
*/
@CompilationFinal MroSequenceStorage mro_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link Assumption} lookupStable
*/
@CompilationFinal Assumption lookupStable_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: int mroLength
*/
@CompilationFinal int mroLength_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNodes
*/
@Children ReadAttributeFromObjectNode[] readAttrNodes_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupConstantMRO}
* Parameter: {@link InteropLibrary} interopLibrary
*/
@Child InteropLibrary interopLibrary_;
LookupConstantMROData(LookupConstantMROData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
LookupConstantMROData remove(Node parent, LookupConstantMROData search) {
LookupConstantMROData newNext = this.next_;
if (newNext != null) {
if (search == newNext) {
newNext = newNext.next_;
} else {
newNext = newNext.remove(this, search);
}
}
LookupConstantMROData copy = parent.insert(new LookupConstantMROData(newNext));
copy.lookupConstantMRO_isSameTypeNode__field1_ = copy.insert(this.lookupConstantMRO_isSameTypeNode__field1_);
copy.cachedKlass_ = this.cachedKlass_;
copy.mro_ = this.mro_;
copy.lookupStable_ = this.lookupStable_;
copy.mroLength_ = this.mroLength_;
copy.readAttrNodes_ = copy.insert(this.readAttrNodes_);
copy.interopLibrary_ = copy.insert(this.interopLibrary_);
return copy;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(LookupNativeSlotNode.class)
@DenyReplace
private static final class LookupCachedLenData extends Node implements SpecializationDataNode {
@Child LookupCachedLenData next_;
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link LookupNativeSlotNode#lookupCachedLen}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int lookupCachedLen_state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupCachedLen}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupCachedLen_getMroStorageNode__field1_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupCachedLen}
* Parameter: int cachedMroLength
*/
@CompilationFinal int cachedMroLength_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupCachedLen}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNodes
*/
@Children ReadAttributeFromObjectNode[] readAttrNodes_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupCachedLen}
* Parameter: {@link InteropLibrary} interopLibrary
*/
@Child InteropLibrary interopLibrary_;
LookupCachedLenData(LookupCachedLenData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(LookupNativeSlotNode.class)
@DenyReplace
private static final class LookupGenericData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link LookupNativeSlotNode#lookupGeneric}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int lookupGeneric_state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupGeneric}
* Parameter: {@link GetMroStorageNode} getMroStorageNode
* Inline method: {@link GetMroStorageNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node lookupGeneric_getMroStorageNode__field1_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupGeneric}
* Parameter: {@link ReadAttributeFromObjectNode} readAttrNode
*/
@Child ReadAttributeFromObjectNode readAttrNode_;
/**
* Source Info:
* Specialization: {@link LookupNativeSlotNode#lookupGeneric}
* Parameter: {@link InteropLibrary} interopLibrary
*/
@Child InteropLibrary interopLibrary_;
LookupGenericData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
/**
* Debug Info:
* Specialization {@link LookupNativeGetattroSlotNode#get}
* Activation probability: 1.00000
* With/without class size: 32/12 bytes
*
*/
@GeneratedBy(LookupNativeGetattroSlotNode.class)
@SuppressWarnings("javadoc")
public static final class LookupNativeGetattroSlotNodeGen extends LookupNativeGetattroSlotNode {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link LookupNativeGetattroSlotNode#get}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link LookupNativeGetattroSlotNode#get}
* Parameter: {@link LookupCallableSlotInMRONode} lookupGetattr
*/
@Child private LookupCallableSlotInMRONode lookupGetattr_;
/**
* Source Info:
* Specialization: {@link LookupNativeGetattroSlotNode#get}
* Parameter: {@link LookupCallableSlotInMRONode} lookupGetattribute
*/
@Child private LookupCallableSlotInMRONode lookupGetattribute_;
/**
* Source Info:
* Specialization: {@link LookupNativeGetattroSlotNode#get}
* Parameter: {@link LookupNativeSlotNode} lookupNativeGetattro
*/
@Child private LookupNativeSlotNode lookupNativeGetattro_;
private LookupNativeGetattroSlotNodeGen() {
}
@Override
public Object execute(PythonManagedClass arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[LookupNativeSlotNode.LookupNativeGetattroSlotNode.get(PythonManagedClass, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, LookupNativeSlotNode)] */) {
{
LookupCallableSlotInMRONode lookupGetattr__ = this.lookupGetattr_;
if (lookupGetattr__ != null) {
LookupCallableSlotInMRONode lookupGetattribute__ = this.lookupGetattribute_;
if (lookupGetattribute__ != null) {
LookupNativeSlotNode lookupNativeGetattro__ = this.lookupNativeGetattro_;
if (lookupNativeGetattro__ != null) {
return get(arg0Value, lookupGetattr__, lookupGetattribute__, lookupNativeGetattro__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(PythonManagedClass arg0Value) {
int state_0 = this.state_0_;
LookupCallableSlotInMRONode lookupGetattr__ = this.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.GetAttr)));
Objects.requireNonNull(lookupGetattr__, "Specialization 'get(PythonManagedClass, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, LookupNativeSlotNode)' cache 'lookupGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupGetattr_ = lookupGetattr__;
LookupCallableSlotInMRONode lookupGetattribute__ = this.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.GetAttribute)));
Objects.requireNonNull(lookupGetattribute__, "Specialization 'get(PythonManagedClass, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, LookupNativeSlotNode)' cache 'lookupGetattribute' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupGetattribute_ = lookupGetattribute__;
LookupNativeSlotNode lookupNativeGetattro__ = this.insert((LookupNativeSlotNodeGen.create(SlotMethodDef.TP_GETATTRO)));
Objects.requireNonNull(lookupNativeGetattro__, "Specialization 'get(PythonManagedClass, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, LookupNativeSlotNode)' cache 'lookupNativeGetattro' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupNativeGetattro_ = lookupNativeGetattro__;
state_0 = state_0 | 0b1 /* add SpecializationActive[LookupNativeSlotNode.LookupNativeGetattroSlotNode.get(PythonManagedClass, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, LookupNativeSlotNode)] */;
this.state_0_ = state_0;
return get(arg0Value, lookupGetattr__, lookupGetattribute__, lookupNativeGetattro__);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static LookupNativeGetattroSlotNode create() {
return new LookupNativeGetattroSlotNodeGen();
}
@NeverDefault
public static LookupNativeGetattroSlotNode getUncached() {
return LookupNativeGetattroSlotNodeGen.UNCACHED;
}
@GeneratedBy(LookupNativeGetattroSlotNode.class)
@DenyReplace
private static final class Uncached extends LookupNativeGetattroSlotNode {
@TruffleBoundary
@Override
public Object execute(PythonManagedClass arg0Value) {
return get(arg0Value, (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.GetAttr)), (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.GetAttribute)), (LookupNativeSlotNode.getUncached(SlotMethodDef.TP_GETATTRO)));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy