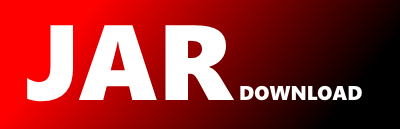
com.oracle.graal.python.nodes.attributes.WriteAttributeToObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.attributes;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.cext.PythonAbstractNativeObject;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccess.ReadObjectNode;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccessFactory.ReadObjectNodeGen;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodes.HashingStorageSetItem;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodesFactory.HashingStorageSetItemNodeGen;
import com.oracle.graal.python.builtins.objects.dict.PDict;
import com.oracle.graal.python.builtins.objects.object.PythonObject;
import com.oracle.graal.python.builtins.objects.type.PythonBuiltinClass;
import com.oracle.graal.python.builtins.objects.type.PythonClass;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.IsTypeNode;
import com.oracle.graal.python.builtins.objects.type.TypeNodesFactory.IsTypeNodeGen;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode;
import com.oracle.graal.python.nodes.object.GetDictIfExistsNode;
import com.oracle.graal.python.nodes.object.GetDictIfExistsNodeGen;
import com.oracle.graal.python.nodes.util.CastToTruffleStringNode;
import com.oracle.graal.python.nodes.util.CastToTruffleStringNodeGen;
import com.oracle.graal.python.runtime.PythonOptions;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.object.HiddenKey;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.CodePointAtIndexNode;
import com.oracle.truffle.api.strings.TruffleString.CodePointLengthNode;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Activation probability: 0.23929
* With/without class size: 8/4 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Activation probability: 0.20714
* With/without class size: 7/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Activation probability: 0.17500
* With/without class size: 9/14 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictNoType}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictBuiltinType}
* Activation probability: 0.11071
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictClass}
* Activation probability: 0.07857
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#doPBCT}
* Activation probability: 0.04643
* With/without class size: 4/4 bytes
*
*/
@GeneratedBy(WriteAttributeToObjectNode.class)
@SuppressWarnings("javadoc")
public final class WriteAttributeToObjectNodeGen extends WriteAttributeToObjectNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER = StateField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_state_0_");
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_CAST_TO_STR = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, STATE_0_UPDATER.subUpdater(7, 8), ReferenceField.create(MethodHandles.lookup(), "castToStr_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "castToStr_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "castToStr_field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CALL_ATTR_UPDATE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(15, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_UPDATE_STORAGE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(16, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*/
private static final HashingStorageSetItem INLINED_SET_HASHING_STORAGE_ITEM = HashingStorageSetItemNodeGen.inline(InlineTarget.create(HashingStorageSetItem.class, STATE_0_UPDATER.subUpdater(17, 6), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field3_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field4_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field1_", Node.class), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field2_", Node.class), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(8, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} updateFlags
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(9, 1)));
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* 1: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* 2: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* 3: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictNoType}
* 4: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictBuiltinType}
* 5: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictClass}
* 6: SpecializationActive {@link WriteAttributeToObjectNode#doPBCT}
* 7-14: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 15: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
* 16: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
* 17-22: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child private GetDictIfExistsNode getDict;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child private DynamicObjectLibrary dylib;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CodePointLengthNode} codePointLengthNode
*/
@Child private CodePointLengthNode cpLen;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CodePointAtIndexNode} codePointAtIndexNode
*/
@Child private CodePointAtIndexNode cpAtIndex;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field4_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field5_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Parameter: {@link WriteAttributeToDynamicObjectNode} writeNode
*/
@Child private WriteAttributeToDynamicObjectNode writeToDynamicStorageNoType_writeNode_;
@Child private WriteToDynamicStoragePythonClassData writeToDynamicStoragePythonClass_cache;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#doPBCT}
* Parameter: {@link WriteAttributeToObjectNode} recursive
*/
@Child private WriteAttributeToObjectNode pBCT_recursive_;
private WriteAttributeToObjectNodeGen() {
}
@Override
public boolean execute(Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_ = this.getDict;
if (getDict_ != null) {
WriteAttributeToDynamicObjectNode writeNode__ = this.writeToDynamicStorageNoType_writeNode_;
if (writeNode__ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_1 = this.getDict;
if (getDict_1 != null) {
DynamicObjectLibrary dylib_ = this.dylib;
if (dylib_ != null) {
CodePointLengthNode cpLen_ = this.cpLen;
if (cpLen_ != null) {
CodePointAtIndexNode cpAtIndex_ = this.cpAtIndex;
if (cpAtIndex_ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_1.execute(arg0Value_) == null)) {
Node inliningTarget__ = (this);
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
WriteToDynamicStoragePythonClassData s2_ = this.writeToDynamicStoragePythonClass_cache;
if (s2_ != null) {
{
GetDictIfExistsNode getDict_2 = this.getDict;
if (getDict_2 != null) {
DynamicObjectLibrary dylib_1 = this.dylib;
if (dylib_1 != null) {
CodePointLengthNode cpLen_1 = this.cpLen;
if (cpLen_1 != null) {
CodePointAtIndexNode cpAtIndex_1 = this.cpAtIndex;
if (cpAtIndex_1 != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_2.execute(arg0Value_) == null)) {
Node inliningTarget__1 = (s2_);
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_3 = this.getDict;
if (getDict_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
Node inliningTarget__2 = (this);
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_4 = this.getDict;
if (getDict_4 != null) {
CodePointLengthNode cpLen_2 = this.cpLen;
if (cpLen_2 != null) {
CodePointAtIndexNode cpAtIndex_2 = this.cpAtIndex;
if (cpAtIndex_2 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
Node inliningTarget__3 = (this);
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
{
GetDictIfExistsNode getDict_5 = this.getDict;
if (getDict_5 != null) {
CodePointLengthNode cpLen_3 = this.cpLen;
if (cpLen_3 != null) {
CodePointAtIndexNode cpAtIndex_3 = this.cpAtIndex;
if (cpAtIndex_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
Node inliningTarget__4 = (this);
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
WriteAttributeToObjectNode recursive__ = this.pBCT_recursive_;
if (recursive__ != null) {
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
@Override
public boolean execute(Object arg0Value, HiddenKey arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_ = this.getDict;
if (getDict_ != null) {
WriteAttributeToDynamicObjectNode writeNode__ = this.writeToDynamicStorageNoType_writeNode_;
if (writeNode__ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_1 = this.getDict;
if (getDict_1 != null) {
DynamicObjectLibrary dylib_ = this.dylib;
if (dylib_ != null) {
CodePointLengthNode cpLen_ = this.cpLen;
if (cpLen_ != null) {
CodePointAtIndexNode cpAtIndex_ = this.cpAtIndex;
if (cpAtIndex_ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_1.execute(arg0Value_) == null)) {
Node inliningTarget__ = (this);
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
WriteToDynamicStoragePythonClassData s2_ = this.writeToDynamicStoragePythonClass_cache;
if (s2_ != null) {
{
GetDictIfExistsNode getDict_2 = this.getDict;
if (getDict_2 != null) {
DynamicObjectLibrary dylib_1 = this.dylib;
if (dylib_1 != null) {
CodePointLengthNode cpLen_1 = this.cpLen;
if (cpLen_1 != null) {
CodePointAtIndexNode cpAtIndex_1 = this.cpAtIndex;
if (cpAtIndex_1 != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_2.execute(arg0Value_) == null)) {
Node inliningTarget__1 = (s2_);
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_3 = this.getDict;
if (getDict_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
Node inliningTarget__2 = (this);
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_4 = this.getDict;
if (getDict_4 != null) {
CodePointLengthNode cpLen_2 = this.cpLen;
if (cpLen_2 != null) {
CodePointAtIndexNode cpAtIndex_2 = this.cpAtIndex;
if (cpAtIndex_2 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
Node inliningTarget__3 = (this);
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
{
GetDictIfExistsNode getDict_5 = this.getDict;
if (getDict_5 != null) {
CodePointLengthNode cpLen_3 = this.cpLen;
if (cpLen_3 != null) {
CodePointAtIndexNode cpAtIndex_3 = this.cpAtIndex;
if (cpAtIndex_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
Node inliningTarget__4 = (this);
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
WriteAttributeToObjectNode recursive__ = this.pBCT_recursive_;
if (recursive__ != null) {
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private boolean executeAndSpecialize(Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value))) {
GetDictIfExistsNode getDict_;
GetDictIfExistsNode getDict__shared = this.getDict;
if (getDict__shared != null) {
getDict_ = getDict__shared;
} else {
getDict_ = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_;
}
WriteAttributeToDynamicObjectNode writeNode__ = this.insert((WriteAttributeToDynamicObjectNode.create()));
Objects.requireNonNull(writeNode__, "Specialization 'writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)' cache 'writeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.writeToDynamicStorageNoType_writeNode_ = writeNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
{
Node inliningTarget__ = null;
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_1;
GetDictIfExistsNode getDict_1_shared = this.getDict;
if (getDict_1_shared != null) {
getDict_1 = getDict_1_shared;
} else {
getDict_1 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((getDict_1.execute(arg0Value_) == null)) {
inliningTarget__ = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_1;
}
DynamicObjectLibrary dylib_;
DynamicObjectLibrary dylib__shared = this.dylib;
if (dylib__shared != null) {
dylib_ = dylib__shared;
} else {
dylib_ = this.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
if (dylib_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'dylib' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dylib == null) {
VarHandle.storeStoreFence();
this.dylib = dylib_;
}
CodePointLengthNode cpLen_;
CodePointLengthNode cpLen__shared = this.cpLen;
if (cpLen__shared != null) {
cpLen_ = cpLen__shared;
} else {
cpLen_ = this.insert((CodePointLengthNode.create()));
if (cpLen_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_;
}
CodePointAtIndexNode cpAtIndex_;
CodePointAtIndexNode cpAtIndex__shared = this.cpAtIndex;
if (cpAtIndex__shared != null) {
cpAtIndex_ = cpAtIndex__shared;
} else {
cpAtIndex_ = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
{
Node inliningTarget__1 = null;
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_2;
GetDictIfExistsNode getDict_2_shared = this.getDict;
if (getDict_2_shared != null) {
getDict_2 = getDict_2_shared;
} else {
getDict_2 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_2 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((getDict_2.execute(arg0Value_) == null)) {
WriteToDynamicStoragePythonClassData s2_ = this.insert(new WriteToDynamicStoragePythonClassData());
inliningTarget__1 = (s2_);
if (this.getDict == null) {
this.getDict = getDict_2;
}
DynamicObjectLibrary dylib_1;
DynamicObjectLibrary dylib_1_shared = this.dylib;
if (dylib_1_shared != null) {
dylib_1 = dylib_1_shared;
} else {
dylib_1 = s2_.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
if (dylib_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'dylib' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dylib == null) {
this.dylib = dylib_1;
}
CodePointLengthNode cpLen_1;
CodePointLengthNode cpLen_1_shared = this.cpLen;
if (cpLen_1_shared != null) {
cpLen_1 = cpLen_1_shared;
} else {
cpLen_1 = s2_.insert((CodePointLengthNode.create()));
if (cpLen_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
this.cpLen = cpLen_1;
}
CodePointAtIndexNode cpAtIndex_1;
CodePointAtIndexNode cpAtIndex_1_shared = this.cpAtIndex;
if (cpAtIndex_1_shared != null) {
cpAtIndex_1 = cpAtIndex_1_shared;
} else {
cpAtIndex_1 = s2_.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
this.cpAtIndex = cpAtIndex_1;
}
VarHandle.storeStoreFence();
this.writeToDynamicStoragePythonClass_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
{
PDict dict__ = null;
Node inliningTarget__2 = null;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_3;
GetDictIfExistsNode getDict_3_shared = this.getDict;
if (getDict_3_shared != null) {
getDict_3 = getDict_3_shared;
} else {
getDict_3 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
inliningTarget__2 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_3;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
{
PDict dict__1 = null;
Node inliningTarget__3 = null;
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_4;
GetDictIfExistsNode getDict_4_shared = this.getDict;
if (getDict_4_shared != null) {
getDict_4 = getDict_4_shared;
} else {
getDict_4 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_4 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
inliningTarget__3 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_4;
}
CodePointLengthNode cpLen_2;
CodePointLengthNode cpLen_2_shared = this.cpLen;
if (cpLen_2_shared != null) {
cpLen_2 = cpLen_2_shared;
} else {
cpLen_2 = this.insert((CodePointLengthNode.create()));
if (cpLen_2 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_2;
}
CodePointAtIndexNode cpAtIndex_2;
CodePointAtIndexNode cpAtIndex_2_shared = this.cpAtIndex;
if (cpAtIndex_2_shared != null) {
cpAtIndex_2 = cpAtIndex_2_shared;
} else {
cpAtIndex_2 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_2 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
{
PDict dict__2 = null;
Node inliningTarget__4 = null;
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_5;
GetDictIfExistsNode getDict_5_shared = this.getDict;
if (getDict_5_shared != null) {
getDict_5 = getDict_5_shared;
} else {
getDict_5 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_5 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
inliningTarget__4 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_5;
}
CodePointLengthNode cpLen_3;
CodePointLengthNode cpLen_3_shared = this.cpLen;
if (cpLen_3_shared != null) {
cpLen_3 = cpLen_3_shared;
} else {
cpLen_3 = this.insert((CodePointLengthNode.create()));
if (cpLen_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_3;
}
CodePointAtIndexNode cpAtIndex_3;
CodePointAtIndexNode cpAtIndex_3_shared = this.cpAtIndex;
if (cpAtIndex_3_shared != null) {
cpAtIndex_3 = cpAtIndex_3_shared;
} else {
cpAtIndex_3 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_3;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
WriteAttributeToObjectNode recursive__ = this.insert((WriteAttributeToObjectNode.create()));
Objects.requireNonNull(recursive__, "Specialization 'doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)' cache 'recursive' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.pBCT_recursive_ = recursive__;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b1111111) & ((state_0 & 0b1111111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static WriteAttributeToObjectNode create() {
return new WriteAttributeToObjectNodeGen();
}
@GeneratedBy(WriteAttributeToObjectNode.class)
@DenyReplace
private static final class WriteToDynamicStoragePythonClassData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 8: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
* 9: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} updateFlags
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int writeToDynamicStoragePythonClass_state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field3_;
WriteToDynamicStoragePythonClassData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
/**
* Debug Info:
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Activation probability: 0.19111
* With/without class size: 7/4 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Activation probability: 0.15111
* With/without class size: 8/14 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictNoType}
* Activation probability: 0.13111
* With/without class size: 6/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictBuiltinType}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictClass}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#doPBCT}
* Activation probability: 0.07111
* With/without class size: 5/4 bytes
* Specialization {@link WriteAttributeToObjectNotTypeNode#writeNativeObject}
* Activation probability: 0.05111
* With/without class size: 4/0 bytes
* Specialization {@link WriteAttributeToObjectNotTypeNode#doError}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(WriteAttributeToObjectNotTypeNode.class)
@SuppressWarnings("javadoc")
protected static final class WriteAttributeToObjectNotTypeNodeGen extends WriteAttributeToObjectNotTypeNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NOT_TYPE_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER = StateField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_state_0_");
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_CAST_TO_STR = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, STATE_0_UPDATER.subUpdater(9, 8), ReferenceField.create(MethodHandles.lookup(), "castToStr_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "castToStr_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "castToStr_field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CALL_ATTR_UPDATE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(17, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_UPDATE_STORAGE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(18, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*/
private static final HashingStorageSetItem INLINED_SET_HASHING_STORAGE_ITEM = HashingStorageSetItemNodeGen.inline(InlineTarget.create(HashingStorageSetItem.class, STATE_0_UPDATER.subUpdater(19, 6), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field3_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field4_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NOT_TYPE_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field1_", Node.class), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field2_", Node.class), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NOT_TYPE_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(8, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} updateFlags
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_NOT_TYPE_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(9, 1)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* 1: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* 2: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* 3: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictNoType}
* 4: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictBuiltinType}
* 5: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictClass}
* 6: SpecializationActive {@link WriteAttributeToObjectNode#doPBCT}
* 7: SpecializationActive {@link WriteAttributeToObjectNotTypeNode#writeNativeObject}
* 8: SpecializationActive {@link WriteAttributeToObjectNotTypeNode#doError}
* 9-16: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
* 18: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
* 19-24: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child private GetDictIfExistsNode getDict;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child private DynamicObjectLibrary dylib;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CodePointLengthNode} codePointLengthNode
*/
@Child private CodePointLengthNode cpLen;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CodePointAtIndexNode} codePointAtIndexNode
*/
@Child private CodePointAtIndexNode cpAtIndex;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field4_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field5_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNotTypeNode#writeNativeObject}
* Parameter: {@link PRaiseNode} raiseNode
*/
@Child private PRaiseNode raiseNode;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Parameter: {@link WriteAttributeToDynamicObjectNode} writeNode
*/
@Child private WriteAttributeToDynamicObjectNode writeToDynamicStorageNoType_writeNode_;
@Child private WriteToDynamicStoragePythonClassData writeToDynamicStoragePythonClass_cache;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#doPBCT}
* Parameter: {@link WriteAttributeToObjectNode} recursive
*/
@Child private WriteAttributeToObjectNode pBCT_recursive_;
private WriteAttributeToObjectNotTypeNodeGen() {
}
@Override
public boolean execute(Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_ = this.getDict;
if (getDict_ != null) {
WriteAttributeToDynamicObjectNode writeNode__ = this.writeToDynamicStorageNoType_writeNode_;
if (writeNode__ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_1 = this.getDict;
if (getDict_1 != null) {
DynamicObjectLibrary dylib_ = this.dylib;
if (dylib_ != null) {
CodePointLengthNode cpLen_ = this.cpLen;
if (cpLen_ != null) {
CodePointAtIndexNode cpAtIndex_ = this.cpAtIndex;
if (cpAtIndex_ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_1.execute(arg0Value_) == null)) {
Node inliningTarget__ = (this);
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
WriteToDynamicStoragePythonClassData s2_ = this.writeToDynamicStoragePythonClass_cache;
if (s2_ != null) {
{
GetDictIfExistsNode getDict_2 = this.getDict;
if (getDict_2 != null) {
DynamicObjectLibrary dylib_1 = this.dylib;
if (dylib_1 != null) {
CodePointLengthNode cpLen_1 = this.cpLen;
if (cpLen_1 != null) {
CodePointAtIndexNode cpAtIndex_1 = this.cpAtIndex;
if (cpAtIndex_1 != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_2.execute(arg0Value_) == null)) {
Node inliningTarget__1 = (s2_);
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_3 = this.getDict;
if (getDict_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
Node inliningTarget__2 = (this);
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_4 = this.getDict;
if (getDict_4 != null) {
CodePointLengthNode cpLen_2 = this.cpLen;
if (cpLen_2 != null) {
CodePointAtIndexNode cpAtIndex_2 = this.cpAtIndex;
if (cpAtIndex_2 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
Node inliningTarget__3 = (this);
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
{
GetDictIfExistsNode getDict_5 = this.getDict;
if (getDict_5 != null) {
CodePointLengthNode cpLen_3 = this.cpLen;
if (cpLen_3 != null) {
CodePointAtIndexNode cpAtIndex_3 = this.cpAtIndex;
if (cpAtIndex_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
Node inliningTarget__4 = (this);
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
WriteAttributeToObjectNode recursive__ = this.pBCT_recursive_;
if (recursive__ != null) {
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
{
GetDictIfExistsNode getDict_6 = this.getDict;
if (getDict_6 != null) {
PRaiseNode raiseNode_ = this.raiseNode;
if (raiseNode_ != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__5 = (this);
return WriteAttributeToObjectNotTypeNode.writeNativeObject(arg0Value_, arg1Value, arg2Value, inliningTarget__5, getDict_6, INLINED_SET_HASHING_STORAGE_ITEM, INLINED_UPDATE_STORAGE, raiseNode_);
}
}
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
{
GetDictIfExistsNode getDict_7 = this.getDict;
if (getDict_7 != null) {
PRaiseNode raiseNode_1 = this.raiseNode;
if (raiseNode_1 != null) {
if ((WriteAttributeToObjectNode.isErrorCase(getDict_7, arg0Value, arg1Value))) {
return WriteAttributeToObjectNotTypeNode.doError(arg0Value, arg1Value, arg2Value, getDict_7, raiseNode_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
@Override
public boolean execute(Object arg0Value, HiddenKey arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_ = this.getDict;
if (getDict_ != null) {
WriteAttributeToDynamicObjectNode writeNode__ = this.writeToDynamicStorageNoType_writeNode_;
if (writeNode__ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_1 = this.getDict;
if (getDict_1 != null) {
DynamicObjectLibrary dylib_ = this.dylib;
if (dylib_ != null) {
CodePointLengthNode cpLen_ = this.cpLen;
if (cpLen_ != null) {
CodePointAtIndexNode cpAtIndex_ = this.cpAtIndex;
if (cpAtIndex_ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_1.execute(arg0Value_) == null)) {
Node inliningTarget__ = (this);
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
WriteToDynamicStoragePythonClassData s2_ = this.writeToDynamicStoragePythonClass_cache;
if (s2_ != null) {
{
GetDictIfExistsNode getDict_2 = this.getDict;
if (getDict_2 != null) {
DynamicObjectLibrary dylib_1 = this.dylib;
if (dylib_1 != null) {
CodePointLengthNode cpLen_1 = this.cpLen;
if (cpLen_1 != null) {
CodePointAtIndexNode cpAtIndex_1 = this.cpAtIndex;
if (cpAtIndex_1 != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_2.execute(arg0Value_) == null)) {
Node inliningTarget__1 = (s2_);
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_3 = this.getDict;
if (getDict_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
Node inliningTarget__2 = (this);
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_4 = this.getDict;
if (getDict_4 != null) {
CodePointLengthNode cpLen_2 = this.cpLen;
if (cpLen_2 != null) {
CodePointAtIndexNode cpAtIndex_2 = this.cpAtIndex;
if (cpAtIndex_2 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
Node inliningTarget__3 = (this);
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
{
GetDictIfExistsNode getDict_5 = this.getDict;
if (getDict_5 != null) {
CodePointLengthNode cpLen_3 = this.cpLen;
if (cpLen_3 != null) {
CodePointAtIndexNode cpAtIndex_3 = this.cpAtIndex;
if (cpAtIndex_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
Node inliningTarget__4 = (this);
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
WriteAttributeToObjectNode recursive__ = this.pBCT_recursive_;
if (recursive__ != null) {
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
{
GetDictIfExistsNode getDict_6 = this.getDict;
if (getDict_6 != null) {
PRaiseNode raiseNode_ = this.raiseNode;
if (raiseNode_ != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__5 = (this);
return WriteAttributeToObjectNotTypeNode.writeNativeObject(arg0Value_, arg1Value, arg2Value, inliningTarget__5, getDict_6, INLINED_SET_HASHING_STORAGE_ITEM, INLINED_UPDATE_STORAGE, raiseNode_);
}
}
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
{
GetDictIfExistsNode getDict_7 = this.getDict;
if (getDict_7 != null) {
PRaiseNode raiseNode_1 = this.raiseNode;
if (raiseNode_1 != null) {
if ((WriteAttributeToObjectNode.isErrorCase(getDict_7, arg0Value, arg1Value))) {
return WriteAttributeToObjectNotTypeNode.doError(arg0Value, arg1Value, arg2Value, getDict_7, raiseNode_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private boolean executeAndSpecialize(Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value))) {
GetDictIfExistsNode getDict_;
GetDictIfExistsNode getDict__shared = this.getDict;
if (getDict__shared != null) {
getDict_ = getDict__shared;
} else {
getDict_ = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_;
}
WriteAttributeToDynamicObjectNode writeNode__ = this.insert((WriteAttributeToDynamicObjectNode.create()));
Objects.requireNonNull(writeNode__, "Specialization 'writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)' cache 'writeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.writeToDynamicStorageNoType_writeNode_ = writeNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
{
Node inliningTarget__ = null;
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_1;
GetDictIfExistsNode getDict_1_shared = this.getDict;
if (getDict_1_shared != null) {
getDict_1 = getDict_1_shared;
} else {
getDict_1 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((getDict_1.execute(arg0Value_) == null)) {
inliningTarget__ = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_1;
}
DynamicObjectLibrary dylib_;
DynamicObjectLibrary dylib__shared = this.dylib;
if (dylib__shared != null) {
dylib_ = dylib__shared;
} else {
dylib_ = this.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
if (dylib_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'dylib' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dylib == null) {
VarHandle.storeStoreFence();
this.dylib = dylib_;
}
CodePointLengthNode cpLen_;
CodePointLengthNode cpLen__shared = this.cpLen;
if (cpLen__shared != null) {
cpLen_ = cpLen__shared;
} else {
cpLen_ = this.insert((CodePointLengthNode.create()));
if (cpLen_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_;
}
CodePointAtIndexNode cpAtIndex_;
CodePointAtIndexNode cpAtIndex__shared = this.cpAtIndex;
if (cpAtIndex__shared != null) {
cpAtIndex_ = cpAtIndex__shared;
} else {
cpAtIndex_ = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
{
Node inliningTarget__1 = null;
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_2;
GetDictIfExistsNode getDict_2_shared = this.getDict;
if (getDict_2_shared != null) {
getDict_2 = getDict_2_shared;
} else {
getDict_2 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_2 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((getDict_2.execute(arg0Value_) == null)) {
WriteToDynamicStoragePythonClassData s2_ = this.insert(new WriteToDynamicStoragePythonClassData());
inliningTarget__1 = (s2_);
if (this.getDict == null) {
this.getDict = getDict_2;
}
DynamicObjectLibrary dylib_1;
DynamicObjectLibrary dylib_1_shared = this.dylib;
if (dylib_1_shared != null) {
dylib_1 = dylib_1_shared;
} else {
dylib_1 = s2_.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
if (dylib_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'dylib' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dylib == null) {
this.dylib = dylib_1;
}
CodePointLengthNode cpLen_1;
CodePointLengthNode cpLen_1_shared = this.cpLen;
if (cpLen_1_shared != null) {
cpLen_1 = cpLen_1_shared;
} else {
cpLen_1 = s2_.insert((CodePointLengthNode.create()));
if (cpLen_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
this.cpLen = cpLen_1;
}
CodePointAtIndexNode cpAtIndex_1;
CodePointAtIndexNode cpAtIndex_1_shared = this.cpAtIndex;
if (cpAtIndex_1_shared != null) {
cpAtIndex_1 = cpAtIndex_1_shared;
} else {
cpAtIndex_1 = s2_.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
this.cpAtIndex = cpAtIndex_1;
}
VarHandle.storeStoreFence();
this.writeToDynamicStoragePythonClass_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
{
PDict dict__ = null;
Node inliningTarget__2 = null;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_3;
GetDictIfExistsNode getDict_3_shared = this.getDict;
if (getDict_3_shared != null) {
getDict_3 = getDict_3_shared;
} else {
getDict_3 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
inliningTarget__2 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_3;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
{
PDict dict__1 = null;
Node inliningTarget__3 = null;
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_4;
GetDictIfExistsNode getDict_4_shared = this.getDict;
if (getDict_4_shared != null) {
getDict_4 = getDict_4_shared;
} else {
getDict_4 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_4 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
inliningTarget__3 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_4;
}
CodePointLengthNode cpLen_2;
CodePointLengthNode cpLen_2_shared = this.cpLen;
if (cpLen_2_shared != null) {
cpLen_2 = cpLen_2_shared;
} else {
cpLen_2 = this.insert((CodePointLengthNode.create()));
if (cpLen_2 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_2;
}
CodePointAtIndexNode cpAtIndex_2;
CodePointAtIndexNode cpAtIndex_2_shared = this.cpAtIndex;
if (cpAtIndex_2_shared != null) {
cpAtIndex_2 = cpAtIndex_2_shared;
} else {
cpAtIndex_2 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_2 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
{
PDict dict__2 = null;
Node inliningTarget__4 = null;
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_5;
GetDictIfExistsNode getDict_5_shared = this.getDict;
if (getDict_5_shared != null) {
getDict_5 = getDict_5_shared;
} else {
getDict_5 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_5 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
inliningTarget__4 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_5;
}
CodePointLengthNode cpLen_3;
CodePointLengthNode cpLen_3_shared = this.cpLen;
if (cpLen_3_shared != null) {
cpLen_3 = cpLen_3_shared;
} else {
cpLen_3 = this.insert((CodePointLengthNode.create()));
if (cpLen_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_3;
}
CodePointAtIndexNode cpAtIndex_3;
CodePointAtIndexNode cpAtIndex_3_shared = this.cpAtIndex;
if (cpAtIndex_3_shared != null) {
cpAtIndex_3 = cpAtIndex_3_shared;
} else {
cpAtIndex_3 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_3;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
WriteAttributeToObjectNode recursive__ = this.insert((WriteAttributeToObjectNode.create()));
Objects.requireNonNull(recursive__, "Specialization 'doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)' cache 'recursive' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.pBCT_recursive_ = recursive__;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
{
Node inliningTarget__5 = null;
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
inliningTarget__5 = (this);
GetDictIfExistsNode getDict_6;
GetDictIfExistsNode getDict_6_shared = this.getDict;
if (getDict_6_shared != null) {
getDict_6 = getDict_6_shared;
} else {
getDict_6 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_6 == null) {
throw new IllegalStateException("Specialization 'writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_6;
}
PRaiseNode raiseNode_;
PRaiseNode raiseNode__shared = this.raiseNode;
if (raiseNode__shared != null) {
raiseNode_ = raiseNode__shared;
} else {
raiseNode_ = this.insert((PRaiseNode.create()));
if (raiseNode_ == null) {
throw new IllegalStateException("Specialization 'writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode == null) {
VarHandle.storeStoreFence();
this.raiseNode = raiseNode_;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.writeNativeObject(PythonAbstractNativeObject, Object, Object, Node, GetDictIfExistsNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNotTypeNode.writeNativeObject(arg0Value_, arg1Value, arg2Value, inliningTarget__5, getDict_6, INLINED_SET_HASHING_STORAGE_ITEM, INLINED_UPDATE_STORAGE, raiseNode_);
}
}
}
{
GetDictIfExistsNode getDict_7;
GetDictIfExistsNode getDict_7_shared = this.getDict;
if (getDict_7_shared != null) {
getDict_7 = getDict_7_shared;
} else {
getDict_7 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_7 == null) {
throw new IllegalStateException("Specialization 'doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((WriteAttributeToObjectNode.isErrorCase(getDict_7, arg0Value, arg1Value))) {
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_7;
}
PRaiseNode raiseNode_1;
PRaiseNode raiseNode_1_shared = this.raiseNode;
if (raiseNode_1_shared != null) {
raiseNode_1 = raiseNode_1_shared;
} else {
raiseNode_1 = this.insert((PRaiseNode.create()));
if (raiseNode_1 == null) {
throw new IllegalStateException("Specialization 'doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode == null) {
VarHandle.storeStoreFence();
this.raiseNode = raiseNode_1;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectNotTypeNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNotTypeNode.doError(arg0Value, arg1Value, arg2Value, getDict_7, raiseNode_1);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b111111111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b111111111) & ((state_0 & 0b111111111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static WriteAttributeToObjectNotTypeNode create() {
return new WriteAttributeToObjectNotTypeNodeGen();
}
@NeverDefault
public static WriteAttributeToObjectNotTypeNode getUncached() {
return WriteAttributeToObjectNotTypeNodeGen.UNCACHED;
}
@GeneratedBy(WriteAttributeToObjectNotTypeNode.class)
@DenyReplace
private static final class WriteToDynamicStoragePythonClassData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 8: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
* 9: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} updateFlags
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int writeToDynamicStoragePythonClass_state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field3_;
WriteToDynamicStoragePythonClassData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(WriteAttributeToObjectNotTypeNode.class)
@DenyReplace
private static final class Uncached extends WriteAttributeToObjectNotTypeNode {
@TruffleBoundary
@Override
public boolean execute(Object arg0Value, Object arg1Value, Object arg2Value) {
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, (GetDictIfExistsNode.getUncached())))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (WriteAttributeToDynamicObjectNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, (WriteAttributeToObjectNode.getUncached()));
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return WriteAttributeToObjectNotTypeNode.writeNativeObject(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (InlinedBranchProfile.getUncached()), (PRaiseNode.getUncached()));
}
}
if ((WriteAttributeToObjectNode.isErrorCase((GetDictIfExistsNode.getUncached()), arg0Value, arg1Value))) {
return WriteAttributeToObjectNotTypeNode.doError(arg0Value, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (PRaiseNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@TruffleBoundary
@Override
public boolean execute(Object arg0Value, HiddenKey arg1Value, Object arg2Value) {
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, (GetDictIfExistsNode.getUncached())))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (WriteAttributeToDynamicObjectNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, (WriteAttributeToObjectNode.getUncached()));
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return WriteAttributeToObjectNotTypeNode.writeNativeObject(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (InlinedBranchProfile.getUncached()), (PRaiseNode.getUncached()));
}
}
if ((WriteAttributeToObjectNode.isErrorCase((GetDictIfExistsNode.getUncached()), arg0Value, arg1Value))) {
return WriteAttributeToObjectNotTypeNode.doError(arg0Value, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (PRaiseNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Activation probability: 0.17364
* With/without class size: 7/4 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Activation probability: 0.15727
* With/without class size: 6/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Activation probability: 0.14091
* With/without class size: 8/14 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictNoType}
* Activation probability: 0.12455
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictBuiltinType}
* Activation probability: 0.10818
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#writeToDictClass}
* Activation probability: 0.09182
* With/without class size: 5/0 bytes
* Specialization {@link WriteAttributeToObjectNode#doPBCT}
* Activation probability: 0.07545
* With/without class size: 5/4 bytes
* Specialization {@link WriteAttributeToObjectTpDictNode#writeNativeClassSimple}
* Activation probability: 0.05909
* With/without class size: 4/0 bytes
* Specialization {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Activation probability: 0.04273
* With/without class size: 6/43 bytes
* Specialization {@link WriteAttributeToObjectTpDictNode#doError}
* Activation probability: 0.02636
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(WriteAttributeToObjectTpDictNode.class)
@SuppressWarnings("javadoc")
protected static final class WriteAttributeToObjectTpDictNodeGen extends WriteAttributeToObjectTpDictNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER = StateField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_state_0_");
private static final StateField WRITE_NATIVE_CLASS_GENERIC__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_NATIVE_CLASS_GENERIC_STATE_0_UPDATER = StateField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_state_0_");
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_CAST_TO_STR = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, STATE_0_UPDATER.subUpdater(10, 8), ReferenceField.create(MethodHandles.lookup(), "castToStr_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "castToStr_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "castToStr_field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CALL_ATTR_UPDATE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(18, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_UPDATE_STORAGE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(19, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*/
private static final HashingStorageSetItem INLINED_SET_HASHING_STORAGE_ITEM = HashingStorageSetItemNodeGen.inline(InlineTarget.create(HashingStorageSetItem.class, STATE_0_UPDATER.subUpdater(20, 6), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field3_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field4_", Node.class), ReferenceField.create(MethodHandles.lookup(), "setHashingStorageItem_field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field1_", Node.class), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field2_", Node.class), ReferenceField.create(WriteToDynamicStoragePythonClassData.lookup_(), "writeToDynamicStoragePythonClass_castToStrNode__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(8, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} updateFlags
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_STATE_0_UPDATER.subUpdater(9, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*/
private static final HashingStorageSetItem INLINED_WRITE_NATIVE_CLASS_GENERIC_SET_HASHING_STORAGE_ITEM_ = HashingStorageSetItemNodeGen.inline(InlineTarget.create(HashingStorageSetItem.class, WRITE_NATIVE_CLASS_GENERIC__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_NATIVE_CLASS_GENERIC_STATE_0_UPDATER.subUpdater(0, 6), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_setHashingStorageItem__field1_", Node.class), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_setHashingStorageItem__field2_", Node.class), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_setHashingStorageItem__field3_", Node.class), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_setHashingStorageItem__field4_", Node.class), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_setHashingStorageItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_NATIVE_CLASS_GENERIC_UPDATE_STORAGE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_NATIVE_CLASS_GENERIC__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_NATIVE_CLASS_GENERIC_STATE_0_UPDATER.subUpdater(6, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link InlinedBranchProfile} canBeSpecialSlot
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_WRITE_NATIVE_CLASS_GENERIC_CAN_BE_SPECIAL_SLOT_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, WRITE_NATIVE_CLASS_GENERIC__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_NATIVE_CLASS_GENERIC_STATE_0_UPDATER.subUpdater(7, 1)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link CastToTruffleStringNode} castKeyNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_WRITE_NATIVE_CLASS_GENERIC_CAST_KEY_NODE_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, WRITE_NATIVE_CLASS_GENERIC__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_NATIVE_CLASS_GENERIC_STATE_0_UPDATER.subUpdater(8, 8), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_castKeyNode__field1_", Node.class), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_castKeyNode__field2_", Node.class), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_castKeyNode__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
*/
private static final IsTypeNode INLINED_WRITE_NATIVE_CLASS_GENERIC_IS_TYPE_NODE_ = IsTypeNodeGen.inline(InlineTarget.create(IsTypeNode.class, WRITE_NATIVE_CLASS_GENERIC__WRITE_ATTRIBUTE_TO_OBJECT_TP_DICT_NODE_WRITE_NATIVE_CLASS_GENERIC_STATE_0_UPDATER.subUpdater(16, 5), ReferenceField.create(WriteNativeClassGenericData.lookup_(), "writeNativeClassGeneric_isTypeNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* 1: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* 2: SpecializationActive {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* 3: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictNoType}
* 4: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictBuiltinType}
* 5: SpecializationActive {@link WriteAttributeToObjectNode#writeToDictClass}
* 6: SpecializationActive {@link WriteAttributeToObjectNode#doPBCT}
* 7: SpecializationActive {@link WriteAttributeToObjectTpDictNode#writeNativeClassSimple}
* 8: SpecializationActive {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* 9: SpecializationActive {@link WriteAttributeToObjectTpDictNode#doError}
* 10-17: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 18: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
* 19: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
* 20-25: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child private GetDictIfExistsNode getDict;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node castToStr_field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child private DynamicObjectLibrary dylib;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CodePointLengthNode} codePointLengthNode
*/
@Child private CodePointLengthNode cpLen;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageBuiltinType}
* Parameter: {@link CodePointAtIndexNode} codePointAtIndexNode
*/
@Child private CodePointAtIndexNode cpAtIndex;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field4_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDictNoType}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setHashingStorageItem_field5_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassSimple}
* Parameter: {@link ReadObjectNode} getNativeDict
*/
@Child private ReadObjectNode getNativeDict;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassSimple}
* Parameter: {@link PRaiseNode} raiseNode
*/
@Child private PRaiseNode raiseNode;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStorageNoType}
* Parameter: {@link WriteAttributeToDynamicObjectNode} writeNode
*/
@Child private WriteAttributeToDynamicObjectNode writeToDynamicStorageNoType_writeNode_;
@Child private WriteToDynamicStoragePythonClassData writeToDynamicStoragePythonClass_cache;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#doPBCT}
* Parameter: {@link WriteAttributeToObjectNode} recursive
*/
@Child private WriteAttributeToObjectNode pBCT_recursive_;
@Child private WriteNativeClassGenericData writeNativeClassGeneric_cache;
private WriteAttributeToObjectTpDictNodeGen() {
}
@Override
public boolean execute(Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
if ((state_0 & 0b1111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_ = this.getDict;
if (getDict_ != null) {
WriteAttributeToDynamicObjectNode writeNode__ = this.writeToDynamicStorageNoType_writeNode_;
if (writeNode__ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_1 = this.getDict;
if (getDict_1 != null) {
DynamicObjectLibrary dylib_ = this.dylib;
if (dylib_ != null) {
CodePointLengthNode cpLen_ = this.cpLen;
if (cpLen_ != null) {
CodePointAtIndexNode cpAtIndex_ = this.cpAtIndex;
if (cpAtIndex_ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_1.execute(arg0Value_) == null)) {
Node inliningTarget__ = (this);
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
WriteToDynamicStoragePythonClassData s2_ = this.writeToDynamicStoragePythonClass_cache;
if (s2_ != null) {
{
GetDictIfExistsNode getDict_2 = this.getDict;
if (getDict_2 != null) {
DynamicObjectLibrary dylib_1 = this.dylib;
if (dylib_1 != null) {
CodePointLengthNode cpLen_1 = this.cpLen;
if (cpLen_1 != null) {
CodePointAtIndexNode cpAtIndex_1 = this.cpAtIndex;
if (cpAtIndex_1 != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_2.execute(arg0Value_) == null)) {
Node inliningTarget__1 = (s2_);
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_3 = this.getDict;
if (getDict_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
Node inliningTarget__2 = (this);
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_4 = this.getDict;
if (getDict_4 != null) {
CodePointLengthNode cpLen_2 = this.cpLen;
if (cpLen_2 != null) {
CodePointAtIndexNode cpAtIndex_2 = this.cpAtIndex;
if (cpAtIndex_2 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
Node inliningTarget__3 = (this);
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
{
GetDictIfExistsNode getDict_5 = this.getDict;
if (getDict_5 != null) {
CodePointLengthNode cpLen_3 = this.cpLen;
if (cpLen_3 != null) {
CodePointAtIndexNode cpAtIndex_3 = this.cpAtIndex;
if (cpAtIndex_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
Node inliningTarget__4 = (this);
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
WriteAttributeToObjectNode recursive__ = this.pBCT_recursive_;
if (recursive__ != null) {
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
}
}
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
{
ReadObjectNode getNativeDict_ = this.getNativeDict;
if (getNativeDict_ != null) {
PRaiseNode raiseNode_ = this.raiseNode;
if (raiseNode_ != null) {
CodePointLengthNode cpLen_4 = this.cpLen;
if (cpLen_4 != null) {
CodePointAtIndexNode cpAtIndex_4 = this.cpAtIndex;
if (cpAtIndex_4 != null) {
if ((!(SpecialMethodSlot.canBeSpecial(arg1Value_, cpLen_4, cpAtIndex_4)))) {
Node inliningTarget__5 = (this);
return WriteAttributeToObjectTpDictNode.writeNativeClassSimple(arg0Value_, arg1Value_, arg2Value, inliningTarget__5, getNativeDict_, INLINED_SET_HASHING_STORAGE_ITEM, INLINED_UPDATE_STORAGE, raiseNode_, cpLen_4, cpAtIndex_4);
}
}
}
}
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] */) {
WriteNativeClassGenericData s8_ = this.writeNativeClassGeneric_cache;
if (s8_ != null) {
{
ReadObjectNode getNativeDict_1 = this.getNativeDict;
if (getNativeDict_1 != null) {
PRaiseNode raiseNode_1 = this.raiseNode;
if (raiseNode_1 != null) {
CodePointLengthNode cpLen_5 = this.cpLen;
if (cpLen_5 != null) {
CodePointAtIndexNode cpAtIndex_5 = this.cpAtIndex;
if (cpAtIndex_5 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__6 = (s8_);
return WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(arg0Value_, arg1Value, arg2Value, inliningTarget__6, getNativeDict_1, INLINED_WRITE_NATIVE_CLASS_GENERIC_SET_HASHING_STORAGE_ITEM_, INLINED_WRITE_NATIVE_CLASS_GENERIC_UPDATE_STORAGE_, INLINED_WRITE_NATIVE_CLASS_GENERIC_CAN_BE_SPECIAL_SLOT_, INLINED_WRITE_NATIVE_CLASS_GENERIC_CAST_KEY_NODE_, INLINED_WRITE_NATIVE_CLASS_GENERIC_IS_TYPE_NODE_, raiseNode_1, cpLen_5, cpAtIndex_5, s8_.equalNode_);
}
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
{
GetDictIfExistsNode getDict_6 = this.getDict;
if (getDict_6 != null) {
PRaiseNode raiseNode_2 = this.raiseNode;
if (raiseNode_2 != null) {
if ((WriteAttributeToObjectNode.isErrorCase(getDict_6, arg0Value, arg1Value))) {
return WriteAttributeToObjectTpDictNode.doError(arg0Value, arg1Value, arg2Value, getDict_6, raiseNode_2);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
@Override
public boolean execute(Object arg0Value, HiddenKey arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1101111111) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] || SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_ = this.getDict;
if (getDict_ != null) {
WriteAttributeToDynamicObjectNode writeNode__ = this.writeToDynamicStorageNoType_writeNode_;
if (writeNode__ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_1 = this.getDict;
if (getDict_1 != null) {
DynamicObjectLibrary dylib_ = this.dylib;
if (dylib_ != null) {
CodePointLengthNode cpLen_ = this.cpLen;
if (cpLen_ != null) {
CodePointAtIndexNode cpAtIndex_ = this.cpAtIndex;
if (cpAtIndex_ != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_1.execute(arg0Value_) == null)) {
Node inliningTarget__ = (this);
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
WriteToDynamicStoragePythonClassData s2_ = this.writeToDynamicStoragePythonClass_cache;
if (s2_ != null) {
{
GetDictIfExistsNode getDict_2 = this.getDict;
if (getDict_2 != null) {
DynamicObjectLibrary dylib_1 = this.dylib;
if (dylib_1 != null) {
CodePointLengthNode cpLen_1 = this.cpLen;
if (cpLen_1 != null) {
CodePointAtIndexNode cpAtIndex_1 = this.cpAtIndex;
if (cpAtIndex_1 != null) {
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (getDict_2.execute(arg0Value_) == null)) {
Node inliningTarget__1 = (s2_);
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
{
GetDictIfExistsNode getDict_3 = this.getDict;
if (getDict_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
Node inliningTarget__2 = (this);
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
{
GetDictIfExistsNode getDict_4 = this.getDict;
if (getDict_4 != null) {
CodePointLengthNode cpLen_2 = this.cpLen;
if (cpLen_2 != null) {
CodePointAtIndexNode cpAtIndex_2 = this.cpAtIndex;
if (cpAtIndex_2 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
Node inliningTarget__3 = (this);
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */ && arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
{
GetDictIfExistsNode getDict_5 = this.getDict;
if (getDict_5 != null) {
CodePointLengthNode cpLen_3 = this.cpLen;
if (cpLen_3 != null) {
CodePointAtIndexNode cpAtIndex_3 = this.cpAtIndex;
if (cpAtIndex_3 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
PDict dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
Node inliningTarget__4 = (this);
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
WriteAttributeToObjectNode recursive__ = this.pBCT_recursive_;
if (recursive__ != null) {
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
WriteNativeClassGenericData s8_ = this.writeNativeClassGeneric_cache;
if (s8_ != null) {
{
ReadObjectNode getNativeDict_1 = this.getNativeDict;
if (getNativeDict_1 != null) {
PRaiseNode raiseNode_1 = this.raiseNode;
if (raiseNode_1 != null) {
CodePointLengthNode cpLen_5 = this.cpLen;
if (cpLen_5 != null) {
CodePointAtIndexNode cpAtIndex_5 = this.cpAtIndex;
if (cpAtIndex_5 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__6 = (s8_);
return WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(arg0Value_, arg1Value, arg2Value, inliningTarget__6, getNativeDict_1, INLINED_WRITE_NATIVE_CLASS_GENERIC_SET_HASHING_STORAGE_ITEM_, INLINED_WRITE_NATIVE_CLASS_GENERIC_UPDATE_STORAGE_, INLINED_WRITE_NATIVE_CLASS_GENERIC_CAN_BE_SPECIAL_SLOT_, INLINED_WRITE_NATIVE_CLASS_GENERIC_CAST_KEY_NODE_, INLINED_WRITE_NATIVE_CLASS_GENERIC_IS_TYPE_NODE_, raiseNode_1, cpLen_5, cpAtIndex_5, s8_.equalNode_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */) {
{
GetDictIfExistsNode getDict_6 = this.getDict;
if (getDict_6 != null) {
PRaiseNode raiseNode_2 = this.raiseNode;
if (raiseNode_2 != null) {
if ((WriteAttributeToObjectNode.isErrorCase(getDict_6, arg0Value, arg1Value))) {
return WriteAttributeToObjectTpDictNode.doError(arg0Value, arg1Value, arg2Value, getDict_6, raiseNode_2);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private boolean executeAndSpecialize(Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value))) {
GetDictIfExistsNode getDict_;
GetDictIfExistsNode getDict__shared = this.getDict;
if (getDict__shared != null) {
getDict_ = getDict__shared;
} else {
getDict_ = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, getDict_))) {
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_;
}
WriteAttributeToDynamicObjectNode writeNode__ = this.insert((WriteAttributeToDynamicObjectNode.create()));
Objects.requireNonNull(writeNode__, "Specialization 'writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)' cache 'writeNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.writeToDynamicStorageNoType_writeNode_ = writeNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageNoType(PythonObject, Object, Object, GetDictIfExistsNode, WriteAttributeToDynamicObjectNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, getDict_, writeNode__);
}
}
}
{
Node inliningTarget__ = null;
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_1;
GetDictIfExistsNode getDict_1_shared = this.getDict;
if (getDict_1_shared != null) {
getDict_1 = getDict_1_shared;
} else {
getDict_1 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((getDict_1.execute(arg0Value_) == null)) {
inliningTarget__ = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_1;
}
DynamicObjectLibrary dylib_;
DynamicObjectLibrary dylib__shared = this.dylib;
if (dylib__shared != null) {
dylib_ = dylib__shared;
} else {
dylib_ = this.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
if (dylib_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'dylib' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dylib == null) {
VarHandle.storeStoreFence();
this.dylib = dylib_;
}
CodePointLengthNode cpLen_;
CodePointLengthNode cpLen__shared = this.cpLen;
if (cpLen__shared != null) {
cpLen_ = cpLen__shared;
} else {
cpLen_ = this.insert((CodePointLengthNode.create()));
if (cpLen_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_;
}
CodePointAtIndexNode cpAtIndex_;
CodePointAtIndexNode cpAtIndex__shared = this.cpAtIndex;
if (cpAtIndex__shared != null) {
cpAtIndex_ = cpAtIndex__shared;
} else {
cpAtIndex_ = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_ == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStorageBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__, getDict_1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, dylib_, cpLen_, cpAtIndex_);
}
}
}
}
{
Node inliningTarget__1 = null;
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_2;
GetDictIfExistsNode getDict_2_shared = this.getDict;
if (getDict_2_shared != null) {
getDict_2 = getDict_2_shared;
} else {
getDict_2 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_2 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((getDict_2.execute(arg0Value_) == null)) {
WriteToDynamicStoragePythonClassData s2_ = this.insert(new WriteToDynamicStoragePythonClassData());
inliningTarget__1 = (s2_);
if (this.getDict == null) {
this.getDict = getDict_2;
}
DynamicObjectLibrary dylib_1;
DynamicObjectLibrary dylib_1_shared = this.dylib;
if (dylib_1_shared != null) {
dylib_1 = dylib_1_shared;
} else {
dylib_1 = s2_.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
if (dylib_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'dylib' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dylib == null) {
this.dylib = dylib_1;
}
CodePointLengthNode cpLen_1;
CodePointLengthNode cpLen_1_shared = this.cpLen;
if (cpLen_1_shared != null) {
cpLen_1 = cpLen_1_shared;
} else {
cpLen_1 = s2_.insert((CodePointLengthNode.create()));
if (cpLen_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
this.cpLen = cpLen_1;
}
CodePointAtIndexNode cpAtIndex_1;
CodePointAtIndexNode cpAtIndex_1_shared = this.cpAtIndex;
if (cpAtIndex_1_shared != null) {
cpAtIndex_1 = cpAtIndex_1_shared;
} else {
cpAtIndex_1 = s2_.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_1 == null) {
throw new IllegalStateException("Specialization 'writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
this.cpAtIndex = cpAtIndex_1;
}
VarHandle.storeStoreFence();
this.writeToDynamicStoragePythonClass_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, DynamicObjectLibrary, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, inliningTarget__1, getDict_2, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CAST_TO_STR_NODE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_CALL_ATTR_UPDATE_, INLINED_WRITE_TO_DYNAMIC_STORAGE_PYTHON_CLASS_UPDATE_FLAGS_, dylib_1, cpLen_1, cpAtIndex_1);
}
}
}
}
{
PDict dict__ = null;
Node inliningTarget__2 = null;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_3;
GetDictIfExistsNode getDict_3_shared = this.getDict;
if (getDict_3_shared != null) {
getDict_3 = getDict_3_shared;
} else {
getDict_3 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__ = (getDict_3.execute(arg0Value_));
if ((dict__ != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
inliningTarget__2 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_3;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictNoType(PythonObject, Object, Object, Node, GetDictIfExistsNode, PDict, InlinedBranchProfile, HashingStorageSetItem)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, inliningTarget__2, getDict_3, dict__, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM);
}
}
}
}
{
PDict dict__1 = null;
Node inliningTarget__3 = null;
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_4;
GetDictIfExistsNode getDict_4_shared = this.getDict;
if (getDict_4_shared != null) {
getDict_4 = getDict_4_shared;
} else {
getDict_4 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_4 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__1 = (getDict_4.execute(arg0Value_));
if ((dict__1 != null)) {
inliningTarget__3 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_4;
}
CodePointLengthNode cpLen_2;
CodePointLengthNode cpLen_2_shared = this.cpLen;
if (cpLen_2_shared != null) {
cpLen_2 = cpLen_2_shared;
} else {
cpLen_2 = this.insert((CodePointLengthNode.create()));
if (cpLen_2 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_2;
}
CodePointAtIndexNode cpAtIndex_2;
CodePointAtIndexNode cpAtIndex_2_shared = this.cpAtIndex;
if (cpAtIndex_2_shared != null) {
cpAtIndex_2 = cpAtIndex_2_shared;
} else {
cpAtIndex_2 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_2 == null) {
throw new IllegalStateException("Specialization 'writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictBuiltinType(PythonBuiltinClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, inliningTarget__3, getDict_4, dict__1, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_2, cpAtIndex_2);
}
}
}
}
{
PDict dict__2 = null;
Node inliningTarget__4 = null;
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
GetDictIfExistsNode getDict_5;
GetDictIfExistsNode getDict_5_shared = this.getDict;
if (getDict_5_shared != null) {
getDict_5 = getDict_5_shared;
} else {
getDict_5 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_5 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
dict__2 = (getDict_5.execute(arg0Value_));
if ((dict__2 != null)) {
inliningTarget__4 = (this);
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_5;
}
CodePointLengthNode cpLen_3;
CodePointLengthNode cpLen_3_shared = this.cpLen;
if (cpLen_3_shared != null) {
cpLen_3 = cpLen_3_shared;
} else {
cpLen_3 = this.insert((CodePointLengthNode.create()));
if (cpLen_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_3;
}
CodePointAtIndexNode cpAtIndex_3;
CodePointAtIndexNode cpAtIndex_3_shared = this.cpAtIndex;
if (cpAtIndex_3_shared != null) {
cpAtIndex_3 = cpAtIndex_3_shared;
} else {
cpAtIndex_3 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_3 == null) {
throw new IllegalStateException("Specialization 'writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_3;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[WriteAttributeToObjectNode.writeToDictClass(PythonClass, Object, Object, Node, GetDictIfExistsNode, PDict, CastToTruffleStringNode, InlinedBranchProfile, InlinedBranchProfile, HashingStorageSetItem, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, inliningTarget__4, getDict_5, dict__2, INLINED_CAST_TO_STR, INLINED_CALL_ATTR_UPDATE, INLINED_UPDATE_STORAGE, INLINED_SET_HASHING_STORAGE_ITEM, cpLen_3, cpAtIndex_3);
}
}
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
WriteAttributeToObjectNode recursive__ = this.insert((WriteAttributeToObjectNode.create()));
Objects.requireNonNull(recursive__, "Specialization 'doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)' cache 'recursive' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.pBCT_recursive_ = recursive__;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[WriteAttributeToObjectNode.doPBCT(PythonBuiltinClassType, Object, Object, WriteAttributeToObjectNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, recursive__);
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
{
Node inliningTarget__5 = null;
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
{
CodePointLengthNode cpLen_4;
CodePointLengthNode cpLen_4_shared = this.cpLen;
if (cpLen_4_shared != null) {
cpLen_4 = cpLen_4_shared;
} else {
cpLen_4 = this.insert((CodePointLengthNode.create()));
if (cpLen_4 == null) {
throw new IllegalStateException("Specialization 'writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
CodePointAtIndexNode cpAtIndex_4;
CodePointAtIndexNode cpAtIndex_4_shared = this.cpAtIndex;
if (cpAtIndex_4_shared != null) {
cpAtIndex_4 = cpAtIndex_4_shared;
} else {
cpAtIndex_4 = this.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_4 == null) {
throw new IllegalStateException("Specialization 'writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((!(SpecialMethodSlot.canBeSpecial(arg1Value_, cpLen_4, cpAtIndex_4)))) {
inliningTarget__5 = (this);
ReadObjectNode getNativeDict_;
ReadObjectNode getNativeDict__shared = this.getNativeDict;
if (getNativeDict__shared != null) {
getNativeDict_ = getNativeDict__shared;
} else {
getNativeDict_ = this.insert((ReadObjectNodeGen.create()));
if (getNativeDict_ == null) {
throw new IllegalStateException("Specialization 'writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'getNativeDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getNativeDict == null) {
VarHandle.storeStoreFence();
this.getNativeDict = getNativeDict_;
}
PRaiseNode raiseNode_;
PRaiseNode raiseNode__shared = this.raiseNode;
if (raiseNode__shared != null) {
raiseNode_ = raiseNode__shared;
} else {
raiseNode_ = this.insert((PRaiseNode.create()));
if (raiseNode_ == null) {
throw new IllegalStateException("Specialization 'writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode == null) {
VarHandle.storeStoreFence();
this.raiseNode = raiseNode_;
}
if (this.cpLen == null) {
VarHandle.storeStoreFence();
this.cpLen = cpLen_4;
}
if (this.cpAtIndex == null) {
VarHandle.storeStoreFence();
this.cpAtIndex = cpAtIndex_4;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectTpDictNode.writeNativeClassSimple(arg0Value_, arg1Value_, arg2Value, inliningTarget__5, getNativeDict_, INLINED_SET_HASHING_STORAGE_ITEM, INLINED_UPDATE_STORAGE, raiseNode_, cpLen_4, cpAtIndex_4);
}
}
}
}
{
Node inliningTarget__6 = null;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
WriteNativeClassGenericData s8_ = this.insert(new WriteNativeClassGenericData());
inliningTarget__6 = (s8_);
ReadObjectNode getNativeDict_1;
ReadObjectNode getNativeDict_1_shared = this.getNativeDict;
if (getNativeDict_1_shared != null) {
getNativeDict_1 = getNativeDict_1_shared;
} else {
getNativeDict_1 = s8_.insert((ReadObjectNodeGen.create()));
if (getNativeDict_1 == null) {
throw new IllegalStateException("Specialization 'writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)' contains a shared cache with name 'getNativeDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getNativeDict == null) {
this.getNativeDict = getNativeDict_1;
}
PRaiseNode raiseNode_1;
PRaiseNode raiseNode_1_shared = this.raiseNode;
if (raiseNode_1_shared != null) {
raiseNode_1 = raiseNode_1_shared;
} else {
raiseNode_1 = s8_.insert((PRaiseNode.create()));
if (raiseNode_1 == null) {
throw new IllegalStateException("Specialization 'writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode == null) {
this.raiseNode = raiseNode_1;
}
CodePointLengthNode cpLen_5;
CodePointLengthNode cpLen_5_shared = this.cpLen;
if (cpLen_5_shared != null) {
cpLen_5 = cpLen_5_shared;
} else {
cpLen_5 = s8_.insert((CodePointLengthNode.create()));
if (cpLen_5 == null) {
throw new IllegalStateException("Specialization 'writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpLen == null) {
this.cpLen = cpLen_5;
}
CodePointAtIndexNode cpAtIndex_5;
CodePointAtIndexNode cpAtIndex_5_shared = this.cpAtIndex;
if (cpAtIndex_5_shared != null) {
cpAtIndex_5 = cpAtIndex_5_shared;
} else {
cpAtIndex_5 = s8_.insert((CodePointAtIndexNode.create()));
if (cpAtIndex_5 == null) {
throw new IllegalStateException("Specialization 'writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.cpAtIndex == null) {
this.cpAtIndex = cpAtIndex_5;
}
EqualNode equalNode__ = s8_.insert((EqualNode.create()));
Objects.requireNonNull(equalNode__, "Specialization 'writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)' cache 'equalNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s8_.equalNode_ = equalNode__;
VarHandle.storeStoreFence();
this.writeNativeClassGeneric_cache = s8_;
state_0 = state_0 & 0xffffff7f /* remove SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassSimple(PythonAbstractNativeObject, TruffleString, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode)] */;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(PythonAbstractNativeObject, Object, Object, Node, ReadObjectNode, HashingStorageSetItem, InlinedBranchProfile, InlinedBranchProfile, CastToTruffleStringNode, IsTypeNode, PRaiseNode, CodePointLengthNode, CodePointAtIndexNode, EqualNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(arg0Value_, arg1Value, arg2Value, inliningTarget__6, getNativeDict_1, INLINED_WRITE_NATIVE_CLASS_GENERIC_SET_HASHING_STORAGE_ITEM_, INLINED_WRITE_NATIVE_CLASS_GENERIC_UPDATE_STORAGE_, INLINED_WRITE_NATIVE_CLASS_GENERIC_CAN_BE_SPECIAL_SLOT_, INLINED_WRITE_NATIVE_CLASS_GENERIC_CAST_KEY_NODE_, INLINED_WRITE_NATIVE_CLASS_GENERIC_IS_TYPE_NODE_, raiseNode_1, cpLen_5, cpAtIndex_5, equalNode__);
}
}
}
{
GetDictIfExistsNode getDict_6;
GetDictIfExistsNode getDict_6_shared = this.getDict;
if (getDict_6_shared != null) {
getDict_6 = getDict_6_shared;
} else {
getDict_6 = this.insert((GetDictIfExistsNodeGen.create()));
if (getDict_6 == null) {
throw new IllegalStateException("Specialization 'doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)' contains a shared cache with name 'getDict' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((WriteAttributeToObjectNode.isErrorCase(getDict_6, arg0Value, arg1Value))) {
if (this.getDict == null) {
VarHandle.storeStoreFence();
this.getDict = getDict_6;
}
PRaiseNode raiseNode_2;
PRaiseNode raiseNode_2_shared = this.raiseNode;
if (raiseNode_2_shared != null) {
raiseNode_2 = raiseNode_2_shared;
} else {
raiseNode_2 = this.insert((PRaiseNode.create()));
if (raiseNode_2 == null) {
throw new IllegalStateException("Specialization 'doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)' contains a shared cache with name 'raiseNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raiseNode == null) {
VarHandle.storeStoreFence();
this.raiseNode = raiseNode_2;
}
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[WriteAttributeToObjectNode.WriteAttributeToObjectTpDictNode.doError(Object, Object, Object, GetDictIfExistsNode, PRaiseNode)] */;
this.state_0_ = state_0;
return WriteAttributeToObjectTpDictNode.doError(arg0Value, arg1Value, arg2Value, getDict_6, raiseNode_2);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b1111111111) & ((state_0 & 0b1111111111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static WriteAttributeToObjectTpDictNode create() {
return new WriteAttributeToObjectTpDictNodeGen();
}
@NeverDefault
public static WriteAttributeToObjectTpDictNode getUncached() {
return WriteAttributeToObjectTpDictNodeGen.UNCACHED;
}
@GeneratedBy(WriteAttributeToObjectTpDictNode.class)
@DenyReplace
private static final class WriteToDynamicStoragePythonClassData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 8: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} callAttrUpdate
* Inline method: {@link InlinedBranchProfile#inline}
* 9: InlinedCache
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link InlinedBranchProfile} updateFlags
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int writeToDynamicStoragePythonClass_state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectNode#writeToDynamicStoragePythonClass}
* Parameter: {@link CastToTruffleStringNode} castToStrNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeToDynamicStoragePythonClass_castToStrNode__field3_;
WriteToDynamicStoragePythonClassData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(WriteAttributeToObjectTpDictNode.class)
@DenyReplace
private static final class WriteNativeClassGenericData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-5: InlinedCache
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* 6: InlinedCache
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link InlinedBranchProfile} updateStorage
* Inline method: {@link InlinedBranchProfile#inline}
* 7: InlinedCache
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link InlinedBranchProfile} canBeSpecialSlot
* Inline method: {@link InlinedBranchProfile#inline}
* 8-15: InlinedCache
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link CastToTruffleStringNode} castKeyNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* 16-20: InlinedCache
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int writeNativeClassGeneric_state_0_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_setHashingStorageItem__field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_setHashingStorageItem__field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_setHashingStorageItem__field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_setHashingStorageItem__field4_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link HashingStorageSetItem} setHashingStorageItem
* Inline method: {@link HashingStorageSetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_setHashingStorageItem__field5_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link CastToTruffleStringNode} castKeyNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_castKeyNode__field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link CastToTruffleStringNode} castKeyNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_castKeyNode__field2_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link CastToTruffleStringNode} castKeyNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_castKeyNode__field3_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node writeNativeClassGeneric_isTypeNode__field1_;
/**
* Source Info:
* Specialization: {@link WriteAttributeToObjectTpDictNode#writeNativeClassGeneric}
* Parameter: {@link EqualNode} equalNode
*/
@Child EqualNode equalNode_;
WriteNativeClassGenericData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(WriteAttributeToObjectTpDictNode.class)
@DenyReplace
private static final class Uncached extends WriteAttributeToObjectTpDictNode {
@TruffleBoundary
@Override
public boolean execute(Object arg0Value, Object arg1Value, Object arg2Value) {
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, (GetDictIfExistsNode.getUncached())))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (WriteAttributeToDynamicObjectNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, (WriteAttributeToObjectNode.getUncached()));
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(arg0Value_, arg1Value, arg2Value, (this), (ReadObjectNode.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (CastToTruffleStringNode.getUncached()), (IsTypeNodeGen.getUncached()), (PRaiseNode.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()), (EqualNode.getUncached()));
}
}
if ((WriteAttributeToObjectNode.isErrorCase((GetDictIfExistsNode.getUncached()), arg0Value, arg1Value))) {
return WriteAttributeToObjectTpDictNode.doError(arg0Value, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (PRaiseNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@TruffleBoundary
@Override
public boolean execute(Object arg0Value, HiddenKey arg1Value, Object arg2Value) {
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (WriteAttributeToObjectNode.writeToDynamicStorageNoTypeGuard(arg0Value_, arg1Value, (GetDictIfExistsNode.getUncached())))) {
return WriteAttributeToObjectNode.writeToDynamicStorageNoType(arg0Value_, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (WriteAttributeToDynamicObjectNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return writeToDynamicStorageBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((WriteAttributeToObjectNode.isAttrWritable(arg0Value_, arg1Value)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value))) && ((GetDictIfExistsNode.getUncached()).execute(arg0Value_) == null)) {
return WriteAttributeToObjectNode.writeToDynamicStoragePythonClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (DYNAMIC_OBJECT_LIBRARY_.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null) && (!(PGuards.isManagedClass(arg0Value_)))) {
return WriteAttributeToObjectNode.writeToDictNoType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return writeToDictBuiltinType(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonClass) {
PythonClass arg0Value_ = (PythonClass) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value))) && (((GetDictIfExistsNode.getUncached()).execute(arg0Value_)) != null)) {
return WriteAttributeToObjectNode.writeToDictClass(arg0Value_, arg1Value, arg2Value, (this), (GetDictIfExistsNode.getUncached()), ((GetDictIfExistsNode.getUncached()).execute(arg0Value_)), (CastToTruffleStringNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
}
if (arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
return WriteAttributeToObjectNode.doPBCT(arg0Value_, arg1Value, arg2Value, (WriteAttributeToObjectNode.getUncached()));
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return WriteAttributeToObjectTpDictNode.writeNativeClassGeneric(arg0Value_, arg1Value, arg2Value, (this), (ReadObjectNode.getUncached()), (HashingStorageSetItemNodeGen.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (CastToTruffleStringNode.getUncached()), (IsTypeNodeGen.getUncached()), (PRaiseNode.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()), (EqualNode.getUncached()));
}
}
if ((WriteAttributeToObjectNode.isErrorCase((GetDictIfExistsNode.getUncached()), arg0Value, arg1Value))) {
return WriteAttributeToObjectTpDictNode.doError(arg0Value, arg1Value, arg2Value, (GetDictIfExistsNode.getUncached()), (PRaiseNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy