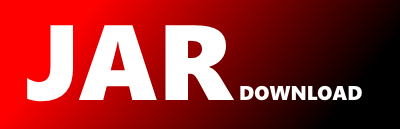
com.oracle.graal.python.nodes.bytecode.CopyDictWithoutKeysNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.bytecode;
import com.oracle.graal.python.builtins.objects.dict.PDict;
import com.oracle.graal.python.builtins.objects.dict.DictBuiltins.DelItemNode;
import com.oracle.graal.python.builtins.objects.dict.DictBuiltinsFactory.DelItemNodeFactory;
import com.oracle.graal.python.builtins.objects.dict.DictNodes.UpdateNode;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)}
* Activation probability: 0.65000
* With/without class size: 14/4 bytes
* Specialization {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(CopyDictWithoutKeysNode.class)
@SuppressWarnings("javadoc")
public final class CopyDictWithoutKeysNodeGen extends CopyDictWithoutKeysNode {
static final ReferenceField COPY0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "copy0_cache", Copy0Data.class);
/**
* State Info:
* 0: SpecializationActive {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)}
* 1: SpecializationActive {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory;
/**
* Source Info:
* Specialization: {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)}
* Parameter: {@link UpdateNode} updateNode
*/
@Child private UpdateNode updateNode;
/**
* Source Info:
* Specialization: {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)}
* Parameter: {@link DelItemNode} delItemNode
*/
@Child private DelItemNode delItemNode;
@UnsafeAccessedField @CompilationFinal private Copy0Data copy0_cache;
private CopyDictWithoutKeysNodeGen() {
}
@Override
public PDict execute(Frame frameValue, Object arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[CopyDictWithoutKeysNode.copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)] || SpecializationActive[CopyDictWithoutKeysNode.copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[CopyDictWithoutKeysNode.copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)] */) {
Copy0Data s0_ = this.copy0_cache;
if (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
UpdateNode updateNode_ = this.updateNode;
if (updateNode_ != null) {
DelItemNode delItemNode_ = this.delItemNode;
if (delItemNode_ != null) {
if ((arg1Value.length == s0_.keysLength_)) {
assert DSLSupport.assertIdempotence((s0_.keysLength_ <= 32));
return CopyDictWithoutKeysNode.copy((VirtualFrame) frameValue, arg0Value, arg1Value, s0_.keysLength_, factory_, updateNode_, delItemNode_);
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CopyDictWithoutKeysNode.copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)] */) {
{
PythonObjectFactory factory_1 = this.factory;
if (factory_1 != null) {
UpdateNode updateNode_1 = this.updateNode;
if (updateNode_1 != null) {
DelItemNode delItemNode_1 = this.delItemNode;
if (delItemNode_1 != null) {
if ((arg1Value.length > 32)) {
return CopyDictWithoutKeysNode.copy((VirtualFrame) frameValue, arg0Value, arg1Value, factory_1, updateNode_1, delItemNode_1);
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private PDict executeAndSpecialize(Frame frameValue, Object arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
while (true) {
int count0_ = 0;
Copy0Data s0_ = COPY0_CACHE_UPDATER.getVolatile(this);
Copy0Data s0_original = s0_;
while (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
UpdateNode updateNode_ = this.updateNode;
if (updateNode_ != null) {
DelItemNode delItemNode_ = this.delItemNode;
if (delItemNode_ != null) {
if ((arg1Value.length == s0_.keysLength_)) {
assert DSLSupport.assertIdempotence((s0_.keysLength_ <= 32));
break;
}
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
int keysLength__ = (arg1Value.length);
// assert (arg1Value.length == s0_.keysLength_);
if ((keysLength__ <= 32)) {
s0_ = new Copy0Data();
s0_.keysLength_ = keysLength__;
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory;
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = this.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_;
}
UpdateNode updateNode_;
UpdateNode updateNode__shared = this.updateNode;
if (updateNode__shared != null) {
updateNode_ = updateNode__shared;
} else {
updateNode_ = this.insert((UpdateNode.create()));
if (updateNode_ == null) {
throw new IllegalStateException("Specialization 'copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)' contains a shared cache with name 'updateNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.updateNode == null) {
this.updateNode = updateNode_;
}
DelItemNode delItemNode_;
DelItemNode delItemNode__shared = this.delItemNode;
if (delItemNode__shared != null) {
delItemNode_ = delItemNode__shared;
} else {
delItemNode_ = this.insert((DelItemNodeFactory.create()));
if (delItemNode_ == null) {
throw new IllegalStateException("Specialization 'copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)' contains a shared cache with name 'delItemNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.delItemNode == null) {
this.delItemNode = delItemNode_;
}
if (!COPY0_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[CopyDictWithoutKeysNode.copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return CopyDictWithoutKeysNode.copy((VirtualFrame) frameValue, arg0Value, arg1Value, s0_.keysLength_, this.factory, this.updateNode, this.delItemNode);
}
break;
}
if ((arg1Value.length > 32)) {
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory;
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = this.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_1;
}
UpdateNode updateNode_1;
UpdateNode updateNode_1_shared = this.updateNode;
if (updateNode_1_shared != null) {
updateNode_1 = updateNode_1_shared;
} else {
updateNode_1 = this.insert((UpdateNode.create()));
if (updateNode_1 == null) {
throw new IllegalStateException("Specialization 'copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)' contains a shared cache with name 'updateNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.updateNode == null) {
VarHandle.storeStoreFence();
this.updateNode = updateNode_1;
}
DelItemNode delItemNode_1;
DelItemNode delItemNode_1_shared = this.delItemNode;
if (delItemNode_1_shared != null) {
delItemNode_1 = delItemNode_1_shared;
} else {
delItemNode_1 = this.insert((DelItemNodeFactory.create()));
if (delItemNode_1 == null) {
throw new IllegalStateException("Specialization 'copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)' contains a shared cache with name 'delItemNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.delItemNode == null) {
VarHandle.storeStoreFence();
this.delItemNode = delItemNode_1;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[CopyDictWithoutKeysNode.copy(VirtualFrame, Object, Object[], PythonObjectFactory, UpdateNode, DelItemNode)] */;
this.state_0_ = state_0;
return CopyDictWithoutKeysNode.copy((VirtualFrame) frameValue, arg0Value, arg1Value, factory_1, updateNode_1, delItemNode_1);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static CopyDictWithoutKeysNode create() {
return new CopyDictWithoutKeysNodeGen();
}
@GeneratedBy(CopyDictWithoutKeysNode.class)
@DenyReplace
private static final class Copy0Data implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link CopyDictWithoutKeysNode#copy(VirtualFrame, Object, Object[], int, PythonObjectFactory, UpdateNode, DelItemNode)}
* Parameter: int keysLength
*/
@CompilationFinal int keysLength_;
Copy0Data() {
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy