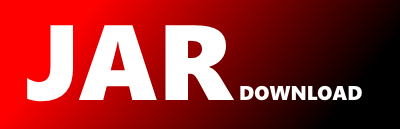
com.oracle.graal.python.nodes.bytecode.GetSendValueNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.bytecode;
import com.oracle.graal.python.builtins.objects.generator.ThrowData;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.NodeCost;
/**
* Debug Info:
* Specialization {@link GetSendValueNode#doNext}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link GetSendValueNode#doThrow}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link GetSendValueNode#doSend}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(GetSendValueNode.class)
@SuppressWarnings("javadoc")
public final class GetSendValueNodeGen extends GetSendValueNode {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link GetSendValueNode#doNext}
* 1: SpecializationActive {@link GetSendValueNode#doThrow}
* 2: SpecializationActive {@link GetSendValueNode#doSend}
*
*/
@CompilationFinal private int state_0_;
private GetSendValueNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[GetSendValueNode.doNext(Object)] */) && (arg0Value == null)) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[GetSendValueNode.doThrow(ThrowData)] */) && arg0Value instanceof ThrowData) {
return false;
}
return true;
}
@Override
public Object execute(Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[GetSendValueNode.doNext(Object)] || SpecializationActive[GetSendValueNode.doThrow(ThrowData)] || SpecializationActive[GetSendValueNode.doSend(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetSendValueNode.doNext(Object)] */) {
if ((arg0Value == null)) {
return doNext(arg0Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetSendValueNode.doThrow(ThrowData)] */ && arg0Value instanceof ThrowData) {
ThrowData arg0Value_ = (ThrowData) arg0Value;
return doThrow(arg0Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetSendValueNode.doSend(Object)] */) {
if (fallbackGuard_(state_0, arg0Value)) {
return doSend(arg0Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if ((arg0Value == null)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[GetSendValueNode.doNext(Object)] */;
this.state_0_ = state_0;
return doNext(arg0Value);
}
if (arg0Value instanceof ThrowData) {
ThrowData arg0Value_ = (ThrowData) arg0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[GetSendValueNode.doThrow(ThrowData)] */;
this.state_0_ = state_0;
return doThrow(arg0Value_);
}
state_0 = state_0 | 0b100 /* add SpecializationActive[GetSendValueNode.doSend(Object)] */;
this.state_0_ = state_0;
return doSend(arg0Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static GetSendValueNode create() {
return new GetSendValueNodeGen();
}
@NeverDefault
public static GetSendValueNode getUncached() {
return GetSendValueNodeGen.UNCACHED;
}
@GeneratedBy(GetSendValueNode.class)
@DenyReplace
private static final class Uncached extends GetSendValueNode {
@TruffleBoundary
@Override
public Object execute(Object arg0Value) {
if ((arg0Value == null)) {
return doNext(arg0Value);
}
if (arg0Value instanceof ThrowData) {
ThrowData arg0Value_ = (ThrowData) arg0Value;
return doThrow(arg0Value_);
}
return doSend(arg0Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy