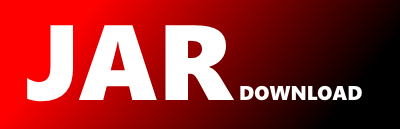
com.oracle.graal.python.nodes.bytecode.MatchKeysNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.bytecode;
import com.oracle.graal.python.lib.PyObjectCallMethodObjArgs;
import com.oracle.graal.python.lib.PyObjectCallMethodObjArgsNodeGen;
import com.oracle.graal.python.lib.PyObjectRichCompareBool.EqNode;
import com.oracle.graal.python.lib.PyObjectRichCompareBoolFactory.EqNodeGen;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link MatchKeysNode#matchCached}
* Activation probability: 0.48333
* With/without class size: 13/4 bytes
* Specialization {@link MatchKeysNode#match}
* Activation probability: 0.33333
* With/without class size: 9/0 bytes
* Specialization {@link MatchKeysNode#matchNoKeys}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(MatchKeysNode.class)
@SuppressWarnings("javadoc")
public final class MatchKeysNodeGen extends MatchKeysNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField STATE_1_UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
static final ReferenceField MATCH_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "matchCached_cache", MatchCachedData.class);
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link EqNode} compareNode
* Inline method: {@link EqNodeGen#inline}
*/
private static final EqNode INLINED_COMPARE_NODE = EqNodeGen.inline(InlineTarget.create(EqNode.class, STATE_0_UPDATER.subUpdater(3, 25), ReferenceField.create(MethodHandles.lookup(), "compareNode_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "compareNode_field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
*/
private static final PyObjectCallMethodObjArgs INLINED_CALL_METHOD = PyObjectCallMethodObjArgsNodeGen.inline(InlineTarget.create(PyObjectCallMethodObjArgs.class, STATE_1_UPDATER.subUpdater(0, 10), ReferenceField.create(MethodHandles.lookup(), "callMethod_field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field2_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field3_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field4_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field5_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field6_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field7_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field8_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field9_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field10_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field11_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field12_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field13_", Node.class), ReferenceField.create(MethodHandles.lookup(), "callMethod_field14_", Node.class)));
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_RAISE = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STATE_0_UPDATER.subUpdater(28, 1), ReferenceField.create(MethodHandles.lookup(), "raise_field1_", Node.class)));
/**
* State Info:
* 0: SpecializationActive {@link MatchKeysNode#matchCached}
* 1: SpecializationActive {@link MatchKeysNode#match}
* 2: SpecializationActive {@link MatchKeysNode#matchNoKeys}
* 3-27: InlinedCache
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link EqNode} compareNode
* Inline method: {@link EqNodeGen#inline}
* 28: InlinedCache
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* State Info:
* 0-9: InlinedCache
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_1_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link EqNode} compareNode
* Inline method: {@link EqNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node compareNode_field1_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link EqNode} compareNode
* Inline method: {@link EqNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node compareNode_field2_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field1_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field2_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field3_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field4_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field5_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field6
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field6_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field7
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field7_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field8
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field8_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field9
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field9_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field10
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field10_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field11
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field11_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field12
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field12_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field13
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field13_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethod
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field14
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node callMethod_field14_;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory;
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node raise_field1_;
@UnsafeAccessedField @Child private MatchCachedData matchCached_cache;
private MatchKeysNodeGen() {
}
@Override
public Object execute(Frame frameValue, Object arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[MatchKeysNode.matchCached(VirtualFrame, Object, Object[], Node, int, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] || SpecializationActive[MatchKeysNode.match(VirtualFrame, Object, Object[], Node, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] || SpecializationActive[MatchKeysNode.matchNoKeys(Object, Object[], PythonObjectFactory)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[MatchKeysNode.matchCached(VirtualFrame, Object, Object[], Node, int, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] */) {
MatchCachedData s0_ = this.matchCached_cache;
if (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
if ((arg1Value.length == s0_.keysLen_)) {
Node inliningTarget__ = (this);
assert DSLSupport.assertIdempotence((s0_.keysLen_ > 0));
assert DSLSupport.assertIdempotence((s0_.keysLen_ <= 32));
return MatchKeysNode.matchCached((VirtualFrame) frameValue, arg0Value, arg1Value, inliningTarget__, s0_.keysLen_, INLINED_COMPARE_NODE, INLINED_CALL_METHOD, factory_, INLINED_RAISE);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[MatchKeysNode.match(VirtualFrame, Object, Object[], Node, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] */) {
{
PythonObjectFactory factory_1 = this.factory;
if (factory_1 != null) {
if ((arg1Value.length > 0)) {
Node inliningTarget__1 = (this);
return MatchKeysNode.match((VirtualFrame) frameValue, arg0Value, arg1Value, inliningTarget__1, INLINED_COMPARE_NODE, INLINED_CALL_METHOD, factory_1, INLINED_RAISE);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[MatchKeysNode.matchNoKeys(Object, Object[], PythonObjectFactory)] */) {
{
PythonObjectFactory factory_2 = this.factory;
if (factory_2 != null) {
if ((arg1Value.length == 0)) {
return MatchKeysNode.matchNoKeys(arg0Value, arg1Value, factory_2);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Object arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[MatchKeysNode.match(VirtualFrame, Object, Object[], Node, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] */) {
while (true) {
int count0_ = 0;
MatchCachedData s0_ = MATCH_CACHED_CACHE_UPDATER.getVolatile(this);
MatchCachedData s0_original = s0_;
while (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
if ((arg1Value.length == s0_.keysLen_)) {
assert DSLSupport.assertIdempotence((s0_.keysLen_ > 0));
assert DSLSupport.assertIdempotence((s0_.keysLen_ <= 32));
inliningTarget__ = (this);
break;
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
int keysLen__ = (arg1Value.length);
// assert (arg1Value.length == s0_.keysLen_);
if ((keysLen__ > 0) && (keysLen__ <= 32)) {
s0_ = this.insert(new MatchCachedData());
inliningTarget__ = (this);
s0_.keysLen_ = keysLen__;
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory;
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = s0_.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'matchCached(VirtualFrame, Object, Object[], Node, int, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_;
}
if (!MATCH_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[MatchKeysNode.matchCached(VirtualFrame, Object, Object[], Node, int, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return MatchKeysNode.matchCached((VirtualFrame) frameValue, arg0Value, arg1Value, inliningTarget__, s0_.keysLen_, INLINED_COMPARE_NODE, INLINED_CALL_METHOD, this.factory, INLINED_RAISE);
}
break;
}
}
}
{
Node inliningTarget__1 = null;
if ((arg1Value.length > 0)) {
inliningTarget__1 = (this);
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory;
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = this.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'match(VirtualFrame, Object, Object[], Node, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_1;
}
this.matchCached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[MatchKeysNode.matchCached(VirtualFrame, Object, Object[], Node, int, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[MatchKeysNode.match(VirtualFrame, Object, Object[], Node, EqNode, PyObjectCallMethodObjArgs, PythonObjectFactory, Lazy)] */;
this.state_0_ = state_0;
return MatchKeysNode.match((VirtualFrame) frameValue, arg0Value, arg1Value, inliningTarget__1, INLINED_COMPARE_NODE, INLINED_CALL_METHOD, factory_1, INLINED_RAISE);
}
}
if ((arg1Value.length == 0)) {
PythonObjectFactory factory_2;
PythonObjectFactory factory_2_shared = this.factory;
if (factory_2_shared != null) {
factory_2 = factory_2_shared;
} else {
factory_2 = this.insert((PythonObjectFactory.create()));
if (factory_2 == null) {
throw new IllegalStateException("Specialization 'matchNoKeys(Object, Object[], PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_2;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[MatchKeysNode.matchNoKeys(Object, Object[], PythonObjectFactory)] */;
this.state_0_ = state_0;
return MatchKeysNode.matchNoKeys(arg0Value, arg1Value, factory_2);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
int counter = 0;
counter += Integer.bitCount((state_0 & 0b111));
if (counter == 1) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static MatchKeysNode create() {
return new MatchKeysNodeGen();
}
@GeneratedBy(MatchKeysNode.class)
@DenyReplace
private static final class MatchCachedData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link MatchKeysNode#matchCached}
* Parameter: int keysLen
*/
@CompilationFinal int keysLen_;
MatchCachedData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy