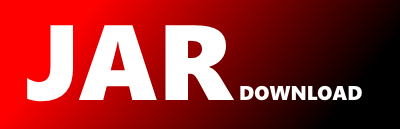
com.oracle.graal.python.nodes.bytecode.SendNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.bytecode;
import com.oracle.graal.python.builtins.objects.PNone;
import com.oracle.graal.python.builtins.objects.exception.StopIterationBuiltins.StopIterationValueNode;
import com.oracle.graal.python.builtins.objects.exception.StopIterationBuiltinsFactory.StopIterationValueNodeFactory;
import com.oracle.graal.python.builtins.objects.generator.PGenerator;
import com.oracle.graal.python.builtins.objects.generator.CommonGeneratorBuiltinsFactory.SendNodeFactory;
import com.oracle.graal.python.lib.GetNextNode;
import com.oracle.graal.python.lib.PyIterCheckNode;
import com.oracle.graal.python.lib.PyIterCheckNodeGen;
import com.oracle.graal.python.lib.PyObjectCallMethodObjArgs;
import com.oracle.graal.python.lib.PyObjectCallMethodObjArgsNodeGen;
import com.oracle.graal.python.nodes.object.BuiltinClassProfiles.IsBuiltinObjectProfile;
import com.oracle.graal.python.nodes.object.BuiltinClassProfilesFactory.IsBuiltinObjectProfileNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* Activation probability: 0.48333
* With/without class size: 19/15 bytes
* Specialization {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Activation probability: 0.33333
* With/without class size: 20/30 bytes
* Specialization {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Activation probability: 0.18333
* With/without class size: 19/68 bytes
*
*/
@GeneratedBy(com.oracle.graal.python.nodes.bytecode.SendNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class SendNodeGen extends com.oracle.graal.python.nodes.bytecode.SendNode {
private static final StateField STATE_0_SendNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField ITERATOR__SEND_NODE_ITERATOR_STATE_0_UPDATER = StateField.create(IteratorData.lookup_(), "iterator_state_0_");
private static final StateField ITERATOR__SEND_NODE_ITERATOR_STATE_1_UPDATER = StateField.create(IteratorData.lookup_(), "iterator_state_1_");
private static final StateField FALLBACK__SEND_NODE_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
static final ReferenceField ITERATOR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "iterator_cache", IteratorData.class);
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_GENERATOR_STOP_ITERATION_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, STATE_0_SendNode_UPDATER.subUpdater(3, 20), ReferenceField.create(MethodHandles.lookup(), "generator_stopIterationProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link PyIterCheckNode} iterCheck
* Inline method: {@link PyIterCheckNodeGen#inline}
*/
private static final PyIterCheckNode INLINED_ITERATOR_ITER_CHECK_ = PyIterCheckNodeGen.inline(InlineTarget.create(PyIterCheckNode.class, ITERATOR__SEND_NODE_ITERATOR_STATE_0_UPDATER.subUpdater(2, 25), ReferenceField.create(IteratorData.lookup_(), "iterator_iterCheck__field1_", Node.class), ReferenceField.create(IteratorData.lookup_(), "iterator_iterCheck__field2_", Node.class), ReferenceField.create(IteratorData.lookup_(), "iterator_iterCheck__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_ITERATOR_STOP_ITERATION_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, ITERATOR__SEND_NODE_ITERATOR_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(IteratorData.lookup_(), "iterator_stopIterationProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
*/
private static final PyObjectCallMethodObjArgs INLINED_FALLBACK_CALL_METHOD_NODE_ = PyObjectCallMethodObjArgsNodeGen.inline(InlineTarget.create(PyObjectCallMethodObjArgs.class, FALLBACK__SEND_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(0, 10), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field1_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field2_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field3_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field4_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field5_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field6_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field7_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field8_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field9_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field10_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field11_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field12_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field13_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_callMethodNode__field14_", Node.class)));
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_FALLBACK_STOP_ITERATION_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, FALLBACK__SEND_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(10, 20), ReferenceField.create(FallbackData.lookup_(), "fallback_stopIterationProfile__field1_", Node.class)));
/**
* State Info:
* 0: SpecializationActive {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* 1: SpecializationActive {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* 2: SpecializationActive {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* 3-22: InlinedCache
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* Parameter: {@link com.oracle.graal.python.builtins.objects.generator.CommonGeneratorBuiltins.SendNode} sendNode
*/
@Child private com.oracle.graal.python.builtins.objects.generator.CommonGeneratorBuiltins.SendNode generator_sendNode_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node generator_stopIterationProfile__field1_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doGenerator}
* Parameter: {@link StopIterationValueNode} getValue
*/
@Child private StopIterationValueNode generator_getValue_;
@UnsafeAccessedField @Child private IteratorData iterator_cache;
@Child private FallbackData fallback_cache;
private SendNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, int arg0Value, Object arg1Value, Object arg2Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[SendNode.doGenerator(VirtualFrame, int, PGenerator, Object, Node, SendNode, IsBuiltinObjectProfile, StopIterationValueNode)] */) && arg1Value instanceof PGenerator) {
return false;
}
if (arg2Value instanceof PNone) {
IteratorData s1_ = this.iterator_cache;
if ((s1_ == null || ((s1_.iterator_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode), guardIndex=0] */ || (INLINED_ITERATOR_ITER_CHECK_.execute((s1_), arg1Value)))) {
return false;
}
}
return true;
}
@Override
public boolean execute(VirtualFrame frameValue, int arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[SendNode.doGenerator(VirtualFrame, int, PGenerator, Object, Node, SendNode, IsBuiltinObjectProfile, StopIterationValueNode)] || SpecializationActive[SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode)] || SpecializationActive[SendNode.doOther(VirtualFrame, int, Object, Object, Node, PyObjectCallMethodObjArgs, IsBuiltinObjectProfile, StopIterationValueNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SendNode.doGenerator(VirtualFrame, int, PGenerator, Object, Node, SendNode, IsBuiltinObjectProfile, StopIterationValueNode)] */ && arg1Value instanceof PGenerator) {
PGenerator arg1Value_ = (PGenerator) arg1Value;
{
com.oracle.graal.python.builtins.objects.generator.CommonGeneratorBuiltins.SendNode sendNode__ = this.generator_sendNode_;
if (sendNode__ != null) {
StopIterationValueNode getValue__ = this.generator_getValue_;
if (getValue__ != null) {
Node inliningTarget__ = (this);
return com.oracle.graal.python.nodes.bytecode.SendNode.doGenerator(frameValue, arg0Value, arg1Value_, arg2Value, inliningTarget__, sendNode__, INLINED_GENERATOR_STOP_ITERATION_PROFILE_, getValue__);
}
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode)] || SpecializationActive[SendNode.doOther(VirtualFrame, int, Object, Object, Node, PyObjectCallMethodObjArgs, IsBuiltinObjectProfile, StopIterationValueNode)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode)] */ && arg2Value instanceof PNone) {
PNone arg2Value_ = (PNone) arg2Value;
IteratorData s1_ = this.iterator_cache;
if (s1_ != null) {
if ((s1_.iterator_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
Node inliningTarget__1 = (s1_);
if ((INLINED_ITERATOR_ITER_CHECK_.execute(inliningTarget__1, arg1Value))) {
return com.oracle.graal.python.nodes.bytecode.SendNode.doIterator(frameValue, arg0Value, arg1Value, arg2Value_, inliningTarget__1, INLINED_ITERATOR_ITER_CHECK_, s1_.getNextNode_, INLINED_ITERATOR_STOP_ITERATION_PROFILE_, s1_.getValue_);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SendNode.doOther(VirtualFrame, int, Object, Object, Node, PyObjectCallMethodObjArgs, IsBuiltinObjectProfile, StopIterationValueNode)] */) {
FallbackData s2_ = this.fallback_cache;
if (s2_ != null) {
{
Node inliningTarget__2 = (s2_);
if (fallbackGuard_(state_0, arg0Value, arg1Value, arg2Value)) {
return com.oracle.graal.python.nodes.bytecode.SendNode.doOther(frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__2, INLINED_FALLBACK_CALL_METHOD_NODE_, INLINED_FALLBACK_STOP_ITERATION_PROFILE_, s2_.getValue_);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private boolean executeAndSpecialize(VirtualFrame frameValue, int arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
if (arg1Value instanceof PGenerator) {
PGenerator arg1Value_ = (PGenerator) arg1Value;
inliningTarget__ = (this);
com.oracle.graal.python.builtins.objects.generator.CommonGeneratorBuiltins.SendNode sendNode__ = this.insert((SendNodeFactory.create()));
Objects.requireNonNull(sendNode__, "Specialization 'doGenerator(VirtualFrame, int, PGenerator, Object, Node, SendNode, IsBuiltinObjectProfile, StopIterationValueNode)' cache 'sendNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.generator_sendNode_ = sendNode__;
StopIterationValueNode getValue__ = this.insert((StopIterationValueNodeFactory.create()));
Objects.requireNonNull(getValue__, "Specialization 'doGenerator(VirtualFrame, int, PGenerator, Object, Node, SendNode, IsBuiltinObjectProfile, StopIterationValueNode)' cache 'getValue' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.generator_getValue_ = getValue__;
state_0 = state_0 | 0b1 /* add SpecializationActive[SendNode.doGenerator(VirtualFrame, int, PGenerator, Object, Node, SendNode, IsBuiltinObjectProfile, StopIterationValueNode)] */;
this.state_0_ = state_0;
return com.oracle.graal.python.nodes.bytecode.SendNode.doGenerator(frameValue, arg0Value, arg1Value_, arg2Value, inliningTarget__, sendNode__, INLINED_GENERATOR_STOP_ITERATION_PROFILE_, getValue__);
}
}
{
Node inliningTarget__1 = null;
if (arg2Value instanceof PNone) {
PNone arg2Value_ = (PNone) arg2Value;
while (true) {
int count1_ = 0;
IteratorData s1_ = ITERATOR_CACHE_UPDATER.getVolatile(this);
IteratorData s1_original = s1_;
while (s1_ != null) {
if ((s1_.iterator_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
inliningTarget__1 = (s1_);
if ((INLINED_ITERATOR_ITER_CHECK_.execute(inliningTarget__1, arg1Value))) {
break;
}
}
if ((s1_.iterator_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count1_++;
}
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
{
s1_ = this.insert(new IteratorData());
inliningTarget__1 = (s1_);
if (((s1_.iterator_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode), guardIndex=0] */) {
s1_.iterator_state_0_ = s1_.iterator_state_0_ | 0b1 /* add GuardActive[specialization=SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode), guardIndex=0] */;
if (!ITERATOR_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
s1_original = s1_;
s1_ = this.insert(new IteratorData(s1_));
}
if ((INLINED_ITERATOR_ITER_CHECK_.execute(inliningTarget__1, arg1Value))) {
GetNextNode getNextNode__ = s1_.insert((GetNextNode.create()));
Objects.requireNonNull(getNextNode__, "Specialization 'doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode)' cache 'getNextNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.getNextNode_ = getNextNode__;
StopIterationValueNode getValue__1 = s1_.insert((StopIterationValueNodeFactory.create()));
Objects.requireNonNull(getValue__1, "Specialization 'doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode)' cache 'getValue' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.getValue_ = getValue__1;
s1_.iterator_state_0_ = s1_.iterator_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!ITERATOR_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[SendNode.doIterator(VirtualFrame, int, Object, PNone, Node, PyIterCheckNode, GetNextNode, IsBuiltinObjectProfile, StopIterationValueNode)] */;
this.state_0_ = state_0;
} else {
s1_ = null;
}
}
}
if (s1_ != null) {
return com.oracle.graal.python.nodes.bytecode.SendNode.doIterator(frameValue, arg0Value, arg1Value, arg2Value_, inliningTarget__1, INLINED_ITERATOR_ITER_CHECK_, s1_.getNextNode_, INLINED_ITERATOR_STOP_ITERATION_PROFILE_, s1_.getValue_);
}
break;
}
}
}
{
Node inliningTarget__2 = null;
FallbackData s2_ = this.insert(new FallbackData());
inliningTarget__2 = (s2_);
StopIterationValueNode getValue__2 = s2_.insert((StopIterationValueNodeFactory.create()));
Objects.requireNonNull(getValue__2, "Specialization 'doOther(VirtualFrame, int, Object, Object, Node, PyObjectCallMethodObjArgs, IsBuiltinObjectProfile, StopIterationValueNode)' cache 'getValue' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.getValue_ = getValue__2;
VarHandle.storeStoreFence();
this.fallback_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[SendNode.doOther(VirtualFrame, int, Object, Object, Node, PyObjectCallMethodObjArgs, IsBuiltinObjectProfile, StopIterationValueNode)] */;
this.state_0_ = state_0;
return com.oracle.graal.python.nodes.bytecode.SendNode.doOther(frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__2, INLINED_FALLBACK_CALL_METHOD_NODE_, INLINED_FALLBACK_STOP_ITERATION_PROFILE_, getValue__2);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b111) & ((state_0 & 0b111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static com.oracle.graal.python.nodes.bytecode.SendNode create() {
return new SendNodeGen();
}
@GeneratedBy(com.oracle.graal.python.nodes.bytecode.SendNode.class)
@DenyReplace
private static final class IteratorData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: GuardActive[guardIndex=0] {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* 1: SpecializationCachesInitialized {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* 2-26: InlinedCache
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link PyIterCheckNode} iterCheck
* Inline method: {@link PyIterCheckNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int iterator_state_0_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int iterator_state_1_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link PyIterCheckNode} iterCheck
* Inline method: {@link PyIterCheckNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node iterator_iterCheck__field1_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link PyIterCheckNode} iterCheck
* Inline method: {@link PyIterCheckNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node iterator_iterCheck__field2_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link PyIterCheckNode} iterCheck
* Inline method: {@link PyIterCheckNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node iterator_iterCheck__field3_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link GetNextNode} getNextNode
*/
@Child GetNextNode getNextNode_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node iterator_stopIterationProfile__field1_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doIterator}
* Parameter: {@link StopIterationValueNode} getValue
*/
@Child StopIterationValueNode getValue_;
IteratorData() {
}
IteratorData(IteratorData delegate) {
this.iterator_state_0_ = delegate.iterator_state_0_;
this.iterator_state_1_ = delegate.iterator_state_1_;
this.iterator_iterCheck__field1_ = delegate.iterator_iterCheck__field1_;
this.iterator_iterCheck__field2_ = delegate.iterator_iterCheck__field2_;
this.iterator_iterCheck__field3_ = delegate.iterator_iterCheck__field3_;
this.getNextNode_ = delegate.getNextNode_;
this.iterator_stopIterationProfile__field1_ = delegate.iterator_stopIterationProfile__field1_;
this.getValue_ = delegate.getValue_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(com.oracle.graal.python.nodes.bytecode.SendNode.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-9: InlinedCache
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* 10-29: InlinedCache
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field1_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field2_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field3_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field4_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field5_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field6
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field6_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field7
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field7_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field8
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field8_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field9
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field9_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field10
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field10_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field11
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field11_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field12
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field12_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field13
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field13_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link PyObjectCallMethodObjArgs} callMethodNode
* Inline method: {@link PyObjectCallMethodObjArgsNodeGen#inline}
* Inline field: {@link Node} field14
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_callMethodNode__field14_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_stopIterationProfile__field1_;
/**
* Source Info:
* Specialization: {@link com.oracle.graal.python.nodes.bytecode.SendNode#doOther}
* Parameter: {@link StopIterationValueNode} getValue
*/
@Child StopIterationValueNode getValue_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy