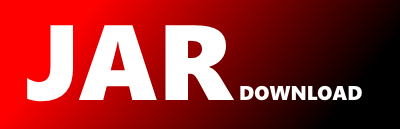
com.oracle.graal.python.nodes.bytecode.SetupAwithNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.bytecode;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PRaiseNode;
import com.oracle.graal.python.nodes.call.special.CallUnaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link SetupAwithNode#setup}
* Activation probability: 1.00000
* With/without class size: 44/23 bytes
*
*/
@GeneratedBy(SetupAwithNode.class)
@SuppressWarnings("javadoc")
public final class SetupAwithNodeGen extends SetupAwithNode {
private static final StateField STATE_0_SetupAwithNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_0_SetupAwithNode_UPDATER.subUpdater(1, 17), ReferenceField.create(MethodHandles.lookup(), "getClassNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link InlinedBranchProfile} error1Profile
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_ERROR1_PROFILE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_SetupAwithNode_UPDATER.subUpdater(18, 1)));
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link InlinedBranchProfile} error2Profile
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_ERROR2_PROFILE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_SetupAwithNode_UPDATER.subUpdater(19, 1)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link SetupAwithNode#setup}
* 1-17: InlinedCache
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* 18: InlinedCache
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link InlinedBranchProfile} error1Profile
* Inline method: {@link InlinedBranchProfile#inline}
* 19: InlinedCache
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link InlinedBranchProfile} error2Profile
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupAEnter
*/
@Child private LookupSpecialMethodSlotNode lookupAEnter_;
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupAExit
*/
@Child private LookupSpecialMethodSlotNode lookupAExit_;
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link CallUnaryMethodNode} callEnter
*/
@Child private CallUnaryMethodNode callEnter_;
/**
* Source Info:
* Specialization: {@link SetupAwithNode#setup}
* Parameter: {@link PRaiseNode} raiseNode
*/
@Child private PRaiseNode raiseNode_;
private SetupAwithNodeGen() {
}
@Override
public int execute(Frame frameValue, int arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SetupAwithNode.setup(VirtualFrame, int, Node, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, InlinedBranchProfile, InlinedBranchProfile, PRaiseNode)] */) {
{
LookupSpecialMethodSlotNode lookupAEnter__ = this.lookupAEnter_;
if (lookupAEnter__ != null) {
LookupSpecialMethodSlotNode lookupAExit__ = this.lookupAExit_;
if (lookupAExit__ != null) {
CallUnaryMethodNode callEnter__ = this.callEnter_;
if (callEnter__ != null) {
PRaiseNode raiseNode__ = this.raiseNode_;
if (raiseNode__ != null) {
Node inliningTarget__ = (this);
return SetupAwithNode.setup((VirtualFrame) frameValue, arg0Value, inliningTarget__, INLINED_GET_CLASS_NODE_, lookupAEnter__, lookupAExit__, callEnter__, INLINED_ERROR1_PROFILE_, INLINED_ERROR2_PROFILE_, raiseNode__);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value);
}
private int executeAndSpecialize(Frame frameValue, int arg0Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
LookupSpecialMethodSlotNode lookupAEnter__ = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.AEnter)));
Objects.requireNonNull(lookupAEnter__, "Specialization 'setup(VirtualFrame, int, Node, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, InlinedBranchProfile, InlinedBranchProfile, PRaiseNode)' cache 'lookupAEnter' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupAEnter_ = lookupAEnter__;
LookupSpecialMethodSlotNode lookupAExit__ = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.AExit)));
Objects.requireNonNull(lookupAExit__, "Specialization 'setup(VirtualFrame, int, Node, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, InlinedBranchProfile, InlinedBranchProfile, PRaiseNode)' cache 'lookupAExit' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupAExit_ = lookupAExit__;
CallUnaryMethodNode callEnter__ = this.insert((CallUnaryMethodNode.create()));
Objects.requireNonNull(callEnter__, "Specialization 'setup(VirtualFrame, int, Node, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, InlinedBranchProfile, InlinedBranchProfile, PRaiseNode)' cache 'callEnter' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callEnter_ = callEnter__;
PRaiseNode raiseNode__ = this.insert((PRaiseNode.create()));
Objects.requireNonNull(raiseNode__, "Specialization 'setup(VirtualFrame, int, Node, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, InlinedBranchProfile, InlinedBranchProfile, PRaiseNode)' cache 'raiseNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.raiseNode_ = raiseNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[SetupAwithNode.setup(VirtualFrame, int, Node, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallUnaryMethodNode, InlinedBranchProfile, InlinedBranchProfile, PRaiseNode)] */;
this.state_0_ = state_0;
return SetupAwithNode.setup((VirtualFrame) frameValue, arg0Value, inliningTarget__, INLINED_GET_CLASS_NODE_, lookupAEnter__, lookupAExit__, callEnter__, INLINED_ERROR1_PROFILE_, INLINED_ERROR2_PROFILE_, raiseNode__);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static SetupAwithNode create() {
return new SetupAwithNodeGen();
}
@NeverDefault
public static SetupAwithNode getUncached() {
return SetupAwithNodeGen.UNCACHED;
}
@GeneratedBy(SetupAwithNode.class)
@DenyReplace
private static final class Uncached extends SetupAwithNode {
@Override
public int execute(Frame frameValue, int arg0Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return SetupAwithNode.setup((VirtualFrame) frameValue, arg0Value, (this), (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.AEnter)), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.AExit)), (CallUnaryMethodNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (PRaiseNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy