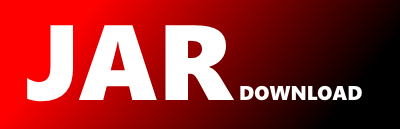
com.oracle.graal.python.nodes.call.CallNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.call;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.function.PBuiltinFunction;
import com.oracle.graal.python.builtins.objects.function.PFunction;
import com.oracle.graal.python.builtins.objects.function.PKeyword;
import com.oracle.graal.python.builtins.objects.method.PBuiltinMethod;
import com.oracle.graal.python.builtins.objects.method.PMethod;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode;
import com.oracle.graal.python.nodes.argument.CreateArgumentsNode;
import com.oracle.graal.python.nodes.argument.CreateArgumentsNodeGen;
import com.oracle.graal.python.nodes.call.special.CallVarargsMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.interop.PForeignToPTypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.runtime.GilNode;
import com.oracle.graal.python.runtime.PythonOptions;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.utilities.TruffleWeakReference;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link CallNode#boundDescriptor}
* Activation probability: 0.12714
* With/without class size: 6/4 bytes
* Specialization {@link CallNode#functionCall}
* Activation probability: 0.11857
* With/without class size: 5/0 bytes
* Specialization {@link CallNode#builtinFunctionCall}
* Activation probability: 0.11000
* With/without class size: 5/0 bytes
* Specialization {@link CallNode#doType}
* Activation probability: 0.10143
* With/without class size: 5/0 bytes
* Specialization {@link CallNode#doPythonClass}
* Activation probability: 0.09286
* With/without class size: 5/0 bytes
* Specialization {@link CallNode#doObjectAndType}
* Activation probability: 0.08429
* With/without class size: 5/0 bytes
* Specialization {@link CallNode#doForeignMethod}
* Activation probability: 0.07571
* With/without class size: 6/13 bytes
* Specialization {@link CallNode#methodCallBuiltinDirect}
* Activation probability: 0.06714
* With/without class size: 4/0 bytes
* Specialization {@link CallNode#methodCallDirect}
* Activation probability: 0.05857
* With/without class size: 4/0 bytes
* Specialization {@link CallNode#builtinMethodCallBuiltinDirectCached}
* Activation probability: 0.05000
* With/without class size: 4/4 bytes
* Specialization {@link CallNode#builtinMethodCallBuiltinDirect}
* Activation probability: 0.04143
* With/without class size: 4/0 bytes
* Specialization {@link CallNode#methodCall}
* Activation probability: 0.03286
* With/without class size: 4/0 bytes
* Specialization {@link CallNode#builtinMethodCall}
* Activation probability: 0.02429
* With/without class size: 4/0 bytes
* Specialization {@link CallNode#doGeneric}
* Activation probability: 0.01571
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(CallNode.class)
@SuppressWarnings("javadoc")
public final class CallNodeGen extends CallNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField FOREIGN_METHOD__CALL_NODE_FOREIGN_METHOD_STATE_0_UPDATER = StateField.create(ForeignMethodData.lookup_(), "foreignMethod_state_0_");
static final ReferenceField BUILTIN_METHOD_CALL_BUILTIN_DIRECT_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "builtinMethodCallBuiltinDirectCached_cache", BuiltinMethodCallBuiltinDirectCachedData.class);
/**
* Source Info:
* Specialization: {@link CallNode#doType}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_CLASS_NODE = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_0_UPDATER.subUpdater(14, 17), ReferenceField.create(MethodHandles.lookup(), "getClassNode_field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link InlinedBranchProfile} keywordsError
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FOREIGN_METHOD_KEYWORDS_ERROR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, FOREIGN_METHOD__CALL_NODE_FOREIGN_METHOD_STATE_0_UPDATER.subUpdater(0, 1)));
/**
* Source Info:
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link InlinedBranchProfile} typeError
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FOREIGN_METHOD_TYPE_ERROR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, FOREIGN_METHOD__CALL_NODE_FOREIGN_METHOD_STATE_0_UPDATER.subUpdater(1, 1)));
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link CallNode#boundDescriptor}
* 1: SpecializationActive {@link CallNode#functionCall}
* 2: SpecializationActive {@link CallNode#builtinFunctionCall}
* 3: SpecializationActive {@link CallNode#doType}
* 4: SpecializationActive {@link CallNode#doPythonClass}
* 5: SpecializationActive {@link CallNode#doObjectAndType}
* 6: SpecializationActive {@link CallNode#doForeignMethod}
* 7: SpecializationActive {@link CallNode#methodCallBuiltinDirect}
* 8: SpecializationActive {@link CallNode#methodCallDirect}
* 9: SpecializationActive {@link CallNode#doGeneric}
* 10: SpecializationActive {@link CallNode#builtinMethodCallBuiltinDirectCached}
* 11: SpecializationActive {@link CallNode#builtinMethodCallBuiltinDirect}
* 12: SpecializationActive {@link CallNode#methodCall}
* 13: SpecializationActive {@link CallNode#builtinMethodCall}
* 14-30: InlinedCache
* Specialization: {@link CallNode#doType}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link CallNode#functionCall}
* Parameter: {@link CallDispatchNode} dispatch
*/
@Child private CallDispatchNode dispatchNode;
/**
* Source Info:
* Specialization: {@link CallNode#functionCall}
* Parameter: {@link CreateArgumentsNode} createArgs
*/
@Child private CreateArgumentsNode argsNode;
/**
* Source Info:
* Specialization: {@link CallNode#doType}
* Parameter: {@link PRaiseNode} raise
*/
@Child private PRaiseNode raise;
/**
* Source Info:
* Specialization: {@link CallNode#doType}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getClassNode_field1_;
/**
* Source Info:
* Specialization: {@link CallNode#doType}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupCall
*/
@Child private LookupSpecialMethodSlotNode lookupCall;
/**
* Source Info:
* Specialization: {@link CallNode#doType}
* Parameter: {@link CallVarargsMethodNode} callCallNode
*/
@Child private CallVarargsMethodNode callCall;
/**
* Source Info:
* Specialization: {@link CallNode#builtinMethodCall}
* Parameter: {@link CallVarargsMethodNode} callCallNode
*/
@Child private CallVarargsMethodNode callVarargs;
/**
* Source Info:
* Specialization: {@link CallNode#boundDescriptor}
* Parameter: {@link CallNode} subNode
*/
@Child private CallNode boundDescriptor_subNode_;
@Child private ForeignMethodData foreignMethod_cache;
@UnsafeAccessedField @CompilationFinal private BuiltinMethodCallBuiltinDirectCachedData builtinMethodCallBuiltinDirectCached_cache;
private CallNodeGen() {
}
@Override
protected Object executeInternal(Frame frameValue, Object arg0Value, Object[] arg1Value, PKeyword[] arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111111111) != 0 /* is SpecializationActive[CallNode.boundDescriptor(VirtualFrame, BoundDescriptor, Object[], PKeyword[], CallNode)] || SpecializationActive[CallNode.functionCall(VirtualFrame, PFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.builtinFunctionCall(VirtualFrame, PBuiltinFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] || SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] || SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] || SpecializationActive[CallNode.doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)] || SpecializationActive[CallNode.methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] || SpecializationActive[CallNode.builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] || SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[CallNode.boundDescriptor(VirtualFrame, BoundDescriptor, Object[], PKeyword[], CallNode)] */ && arg0Value instanceof BoundDescriptor) {
BoundDescriptor arg0Value_ = (BoundDescriptor) arg0Value;
{
CallNode subNode__ = this.boundDescriptor_subNode_;
if (subNode__ != null) {
return CallNode.boundDescriptor((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, subNode__);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CallNode.functionCall(VirtualFrame, PFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */ && arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
{
CallDispatchNode dispatchNode_ = this.dispatchNode;
if (dispatchNode_ != null) {
CreateArgumentsNode argsNode_ = this.argsNode;
if (argsNode_ != null) {
return CallNode.functionCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_, argsNode_);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[CallNode.builtinFunctionCall(VirtualFrame, PBuiltinFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */ && arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
{
CallDispatchNode dispatchNode_1 = this.dispatchNode;
if (dispatchNode_1 != null) {
CreateArgumentsNode argsNode_1 = this.argsNode;
if (argsNode_1 != null) {
return CallNode.builtinFunctionCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_1, argsNode_1);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[CallNode.doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
{
PRaiseNode raise_ = this.raise;
if (raise_ != null) {
LookupSpecialMethodSlotNode lookupCall_ = this.lookupCall;
if (lookupCall_ != null) {
CallVarargsMethodNode callCall_ = this.callCall;
if (callCall_ != null) {
Node inliningTarget__ = (this);
return CallNode.doType((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, inliningTarget__, raise_, INLINED_GET_CLASS_NODE, lookupCall_, callCall_);
}
}
}
}
}
if ((state_0 & 0b110000) != 0 /* is SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] || SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
{
PRaiseNode raise_1 = this.raise;
if (raise_1 != null) {
LookupSpecialMethodSlotNode lookupCall_1 = this.lookupCall;
if (lookupCall_1 != null) {
CallVarargsMethodNode callCall_1 = this.callCall;
if (callCall_1 != null) {
if ((PGuards.isPythonClass(arg0Value))) {
Node inliningTarget__1 = (this);
return CallNode.doPythonClass((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__1, raise_1, INLINED_GET_CLASS_NODE, lookupCall_1, callCall_1);
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
{
PRaiseNode raise_2 = this.raise;
if (raise_2 != null) {
LookupSpecialMethodSlotNode lookupCall_2 = this.lookupCall;
if (lookupCall_2 != null) {
CallVarargsMethodNode callCall_2 = this.callCall;
if (callCall_2 != null) {
if ((!(PGuards.isCallable(arg0Value))) && (!(CallNode.isForeignMethod(arg0Value)))) {
Node inliningTarget__2 = (this);
return CallNode.doObjectAndType((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__2, raise_2, INLINED_GET_CLASS_NODE, lookupCall_2, callCall_2);
}
}
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[CallNode.doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)] */ && arg0Value instanceof ForeignMethod) {
ForeignMethod arg0Value_ = (ForeignMethod) arg0Value;
ForeignMethodData s6_ = this.foreignMethod_cache;
if (s6_ != null) {
{
PRaiseNode raise_3 = this.raise;
if (raise_3 != null) {
Node inliningTarget__3 = (s6_);
return CallNode.doForeignMethod(arg0Value_, arg1Value, arg2Value, inliningTarget__3, raise_3, s6_.fromForeign_, INLINED_FOREIGN_METHOD_KEYWORDS_ERROR_, INLINED_FOREIGN_METHOD_TYPE_ERROR_, s6_.gil_, s6_.interop_);
}
}
}
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[CallNode.methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */ && arg0Value instanceof PMethod) {
PMethod arg0Value_ = (PMethod) arg0Value;
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[CallNode.methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */) {
{
CallDispatchNode dispatchNode_2 = this.dispatchNode;
if (dispatchNode_2 != null) {
CreateArgumentsNode argsNode_2 = this.argsNode;
if (argsNode_2 != null) {
if ((PGuards.isPBuiltinFunction(arg0Value_.getFunction()))) {
return CallNode.methodCallBuiltinDirect((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_2, argsNode_2);
}
}
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[CallNode.methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */) {
{
CallDispatchNode dispatchNode_3 = this.dispatchNode;
if (dispatchNode_3 != null) {
CreateArgumentsNode argsNode_3 = this.argsNode;
if (argsNode_3 != null) {
if ((PGuards.isPFunction(arg0Value_.getFunction()))) {
return CallNode.methodCallDirect((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_3, argsNode_3);
}
}
}
}
}
}
if ((state_0 & 0b110000000000) != 0 /* is SpecializationActive[CallNode.builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)] || SpecializationActive[CallNode.builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */ && arg0Value instanceof PBuiltinMethod) {
PBuiltinMethod arg0Value_ = (PBuiltinMethod) arg0Value;
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[CallNode.builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)] */) {
BuiltinMethodCallBuiltinDirectCachedData s9_ = this.builtinMethodCallBuiltinDirectCached_cache;
if (s9_ != null) {
{
CallDispatchNode dispatchNode_4 = this.dispatchNode;
if (dispatchNode_4 != null) {
CreateArgumentsNode argsNode_4 = this.argsNode;
if (argsNode_4 != null) {
PBuiltinMethod cachedCallable__ = (s9_.weakCachedCallableGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedCallable__ != null) && (arg0Value_ == cachedCallable__) && (PGuards.isPBuiltinFunction(cachedCallable__.getFunction()))) {
return CallNode.builtinMethodCallBuiltinDirectCached((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, cachedCallable__, dispatchNode_4, argsNode_4);
}
}
}
}
}
}
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[CallNode.builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */) {
{
CallDispatchNode dispatchNode_5 = this.dispatchNode;
if (dispatchNode_5 != null) {
CreateArgumentsNode argsNode_5 = this.argsNode;
if (argsNode_5 != null) {
if ((PGuards.isPBuiltinFunction(arg0Value_.getFunction()))) {
return CallNode.builtinMethodCallBuiltinDirect((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_5, argsNode_5);
}
}
}
}
}
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[CallNode.methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PMethod) {
PMethod arg0Value_ = (PMethod) arg0Value;
{
PRaiseNode raise_4 = this.raise;
if (raise_4 != null) {
LookupSpecialMethodSlotNode lookupCall_3 = this.lookupCall;
if (lookupCall_3 != null) {
CallVarargsMethodNode callCall_3 = this.callCall;
if (callCall_3 != null) {
if ((!(PGuards.isFunction(arg0Value_.getFunction())))) {
Node inliningTarget__4 = (this);
return CallNode.methodCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, inliningTarget__4, raise_4, INLINED_GET_CLASS_NODE, lookupCall_3, callCall_3);
}
}
}
}
}
}
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[CallNode.builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PBuiltinMethod) {
PBuiltinMethod arg0Value_ = (PBuiltinMethod) arg0Value;
{
PRaiseNode raise_5 = this.raise;
if (raise_5 != null) {
LookupSpecialMethodSlotNode lookupCall_4 = this.lookupCall;
if (lookupCall_4 != null) {
CallVarargsMethodNode callVarargs_ = this.callVarargs;
if (callVarargs_ != null) {
if ((!(PGuards.isFunction(arg0Value_.getFunction())))) {
Node inliningTarget__5 = (this);
return CallNode.builtinMethodCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, inliningTarget__5, raise_5, INLINED_GET_CLASS_NODE, lookupCall_4, callVarargs_);
}
}
}
}
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
{
CallDispatchNode dispatchNode_6 = this.dispatchNode;
if (dispatchNode_6 != null) {
CreateArgumentsNode argsNode_6 = this.argsNode;
if (argsNode_6 != null) {
PRaiseNode raise_6 = this.raise;
if (raise_6 != null) {
LookupSpecialMethodSlotNode lookupCall_5 = this.lookupCall;
if (lookupCall_5 != null) {
CallVarargsMethodNode callVarargs_1 = this.callVarargs;
if (callVarargs_1 != null) {
if ((!(CallNode.isForeignMethod(arg0Value)))) {
Node inliningTarget__6 = (this);
return CallNode.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__6, dispatchNode_6, argsNode_6, raise_6, INLINED_GET_CLASS_NODE, lookupCall_5, callVarargs_1);
}
}
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Frame frameValue, Object arg0Value, Object[] arg1Value, PKeyword[] arg2Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b11111111111111);
try {
if (arg0Value instanceof BoundDescriptor) {
BoundDescriptor arg0Value_ = (BoundDescriptor) arg0Value;
CallNode subNode__ = this.insert((CallNode.create()));
Objects.requireNonNull(subNode__, "Specialization 'boundDescriptor(VirtualFrame, BoundDescriptor, Object[], PKeyword[], CallNode)' cache 'subNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.boundDescriptor_subNode_ = subNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[CallNode.boundDescriptor(VirtualFrame, BoundDescriptor, Object[], PKeyword[], CallNode)] */;
this.state_0_ = state_0;
return CallNode.boundDescriptor((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, subNode__);
}
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
CallDispatchNode dispatchNode_;
CallDispatchNode dispatchNode__shared = this.dispatchNode;
if (dispatchNode__shared != null) {
dispatchNode_ = dispatchNode__shared;
} else {
dispatchNode_ = this.insert((CallDispatchNode.create()));
if (dispatchNode_ == null) {
throw new IllegalStateException("Specialization 'functionCall(VirtualFrame, PFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
VarHandle.storeStoreFence();
this.dispatchNode = dispatchNode_;
}
CreateArgumentsNode argsNode_;
CreateArgumentsNode argsNode__shared = this.argsNode;
if (argsNode__shared != null) {
argsNode_ = argsNode__shared;
} else {
argsNode_ = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_ == null) {
throw new IllegalStateException("Specialization 'functionCall(VirtualFrame, PFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
VarHandle.storeStoreFence();
this.argsNode = argsNode_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[CallNode.functionCall(VirtualFrame, PFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */;
this.state_0_ = state_0;
return CallNode.functionCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_, argsNode_);
}
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
CallDispatchNode dispatchNode_1;
CallDispatchNode dispatchNode_1_shared = this.dispatchNode;
if (dispatchNode_1_shared != null) {
dispatchNode_1 = dispatchNode_1_shared;
} else {
dispatchNode_1 = this.insert((CallDispatchNode.create()));
if (dispatchNode_1 == null) {
throw new IllegalStateException("Specialization 'builtinFunctionCall(VirtualFrame, PBuiltinFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
VarHandle.storeStoreFence();
this.dispatchNode = dispatchNode_1;
}
CreateArgumentsNode argsNode_1;
CreateArgumentsNode argsNode_1_shared = this.argsNode;
if (argsNode_1_shared != null) {
argsNode_1 = argsNode_1_shared;
} else {
argsNode_1 = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_1 == null) {
throw new IllegalStateException("Specialization 'builtinFunctionCall(VirtualFrame, PBuiltinFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
VarHandle.storeStoreFence();
this.argsNode = argsNode_1;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[CallNode.builtinFunctionCall(VirtualFrame, PBuiltinFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */;
this.state_0_ = state_0;
return CallNode.builtinFunctionCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_1, argsNode_1);
}
{
Node inliningTarget__ = null;
if (((state_0 & 0b1000110000)) == 0 /* is-not SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] && SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] && SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
inliningTarget__ = (this);
PRaiseNode raise_;
PRaiseNode raise__shared = this.raise;
if (raise__shared != null) {
raise_ = raise__shared;
} else {
raise_ = this.insert((PRaiseNode.create()));
if (raise_ == null) {
throw new IllegalStateException("Specialization 'doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
VarHandle.storeStoreFence();
this.raise = raise_;
}
LookupSpecialMethodSlotNode lookupCall_;
LookupSpecialMethodSlotNode lookupCall__shared = this.lookupCall;
if (lookupCall__shared != null) {
lookupCall_ = lookupCall__shared;
} else {
lookupCall_ = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Call)));
if (lookupCall_ == null) {
throw new IllegalStateException("Specialization 'doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'lookupCall' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupCall == null) {
VarHandle.storeStoreFence();
this.lookupCall = lookupCall_;
}
CallVarargsMethodNode callCall_;
CallVarargsMethodNode callCall__shared = this.callCall;
if (callCall__shared != null) {
callCall_ = callCall__shared;
} else {
callCall_ = this.insert((CallVarargsMethodNode.create()));
if (callCall_ == null) {
throw new IllegalStateException("Specialization 'doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'callCallNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callCall == null) {
VarHandle.storeStoreFence();
this.callCall = callCall_;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[CallNode.doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
this.state_0_ = state_0;
return CallNode.doType((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, inliningTarget__, raise_, INLINED_GET_CLASS_NODE, lookupCall_, callCall_);
}
}
{
Node inliningTarget__1 = null;
if (((state_0 & 0b1000100000)) == 0 /* is-not SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] && SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((PGuards.isPythonClass(arg0Value))) {
inliningTarget__1 = (this);
PRaiseNode raise_1;
PRaiseNode raise_1_shared = this.raise;
if (raise_1_shared != null) {
raise_1 = raise_1_shared;
} else {
raise_1 = this.insert((PRaiseNode.create()));
if (raise_1 == null) {
throw new IllegalStateException("Specialization 'doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
VarHandle.storeStoreFence();
this.raise = raise_1;
}
LookupSpecialMethodSlotNode lookupCall_1;
LookupSpecialMethodSlotNode lookupCall_1_shared = this.lookupCall;
if (lookupCall_1_shared != null) {
lookupCall_1 = lookupCall_1_shared;
} else {
lookupCall_1 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Call)));
if (lookupCall_1 == null) {
throw new IllegalStateException("Specialization 'doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'lookupCall' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupCall == null) {
VarHandle.storeStoreFence();
this.lookupCall = lookupCall_1;
}
CallVarargsMethodNode callCall_1;
CallVarargsMethodNode callCall_1_shared = this.callCall;
if (callCall_1_shared != null) {
callCall_1 = callCall_1_shared;
} else {
callCall_1 = this.insert((CallVarargsMethodNode.create()));
if (callCall_1 == null) {
throw new IllegalStateException("Specialization 'doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'callCallNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callCall == null) {
VarHandle.storeStoreFence();
this.callCall = callCall_1;
}
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[CallNode.doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
this.state_0_ = state_0;
return CallNode.doPythonClass((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__1, raise_1, INLINED_GET_CLASS_NODE, lookupCall_1, callCall_1);
}
}
}
{
Node inliningTarget__2 = null;
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((!(PGuards.isCallable(arg0Value))) && (!(CallNode.isForeignMethod(arg0Value)))) {
inliningTarget__2 = (this);
PRaiseNode raise_2;
PRaiseNode raise_2_shared = this.raise;
if (raise_2_shared != null) {
raise_2 = raise_2_shared;
} else {
raise_2 = this.insert((PRaiseNode.create()));
if (raise_2 == null) {
throw new IllegalStateException("Specialization 'doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
VarHandle.storeStoreFence();
this.raise = raise_2;
}
LookupSpecialMethodSlotNode lookupCall_2;
LookupSpecialMethodSlotNode lookupCall_2_shared = this.lookupCall;
if (lookupCall_2_shared != null) {
lookupCall_2 = lookupCall_2_shared;
} else {
lookupCall_2 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Call)));
if (lookupCall_2 == null) {
throw new IllegalStateException("Specialization 'doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'lookupCall' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupCall == null) {
VarHandle.storeStoreFence();
this.lookupCall = lookupCall_2;
}
CallVarargsMethodNode callCall_2;
CallVarargsMethodNode callCall_2_shared = this.callCall;
if (callCall_2_shared != null) {
callCall_2 = callCall_2_shared;
} else {
callCall_2 = this.insert((CallVarargsMethodNode.create()));
if (callCall_2 == null) {
throw new IllegalStateException("Specialization 'doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'callCallNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callCall == null) {
VarHandle.storeStoreFence();
this.callCall = callCall_2;
}
state_0 = state_0 & 0xffffffe7 /* remove SpecializationActive[CallNode.doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)], SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
state_0 = state_0 | 0b100000 /* add SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
this.state_0_ = state_0;
return CallNode.doObjectAndType((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__2, raise_2, INLINED_GET_CLASS_NODE, lookupCall_2, callCall_2);
}
}
}
{
Node inliningTarget__3 = null;
if (arg0Value instanceof ForeignMethod) {
ForeignMethod arg0Value_ = (ForeignMethod) arg0Value;
ForeignMethodData s6_ = this.insert(new ForeignMethodData());
inliningTarget__3 = (s6_);
PRaiseNode raise_3;
PRaiseNode raise_3_shared = this.raise;
if (raise_3_shared != null) {
raise_3 = raise_3_shared;
} else {
raise_3 = s6_.insert((PRaiseNode.create()));
if (raise_3 == null) {
throw new IllegalStateException("Specialization 'doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
this.raise = raise_3;
}
PForeignToPTypeNode fromForeign__ = s6_.insert((PForeignToPTypeNode.create()));
Objects.requireNonNull(fromForeign__, "Specialization 'doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)' cache 'fromForeign' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.fromForeign_ = fromForeign__;
GilNode gil__ = s6_.insert((GilNode.create()));
Objects.requireNonNull(gil__, "Specialization 'doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)' cache 'gil' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.gil_ = gil__;
InteropLibrary interop__ = s6_.insert((INTEROP_LIBRARY_.createDispatched(PythonOptions.getCallSiteInlineCacheMaxDepth())));
Objects.requireNonNull(interop__, "Specialization 'doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)' cache 'interop' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s6_.interop_ = interop__;
VarHandle.storeStoreFence();
this.foreignMethod_cache = s6_;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[CallNode.doForeignMethod(ForeignMethod, Object[], PKeyword[], Node, PRaiseNode, PForeignToPTypeNode, InlinedBranchProfile, InlinedBranchProfile, GilNode, InteropLibrary)] */;
this.state_0_ = state_0;
return CallNode.doForeignMethod(arg0Value_, arg1Value, arg2Value, inliningTarget__3, raise_3, fromForeign__, INLINED_FOREIGN_METHOD_KEYWORDS_ERROR_, INLINED_FOREIGN_METHOD_TYPE_ERROR_, gil__, interop__);
}
}
if (arg0Value instanceof PMethod) {
PMethod arg0Value_ = (PMethod) arg0Value;
if (((state_0 & 0b1100000000)) == 0 /* is-not SpecializationActive[CallNode.methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] && SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((PGuards.isPBuiltinFunction(arg0Value_.getFunction()))) {
CallDispatchNode dispatchNode_2;
CallDispatchNode dispatchNode_2_shared = this.dispatchNode;
if (dispatchNode_2_shared != null) {
dispatchNode_2 = dispatchNode_2_shared;
} else {
dispatchNode_2 = this.insert((CallDispatchNode.create()));
if (dispatchNode_2 == null) {
throw new IllegalStateException("Specialization 'methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
VarHandle.storeStoreFence();
this.dispatchNode = dispatchNode_2;
}
CreateArgumentsNode argsNode_2;
CreateArgumentsNode argsNode_2_shared = this.argsNode;
if (argsNode_2_shared != null) {
argsNode_2 = argsNode_2_shared;
} else {
argsNode_2 = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_2 == null) {
throw new IllegalStateException("Specialization 'methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
VarHandle.storeStoreFence();
this.argsNode = argsNode_2;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[CallNode.methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */;
this.state_0_ = state_0;
return CallNode.methodCallBuiltinDirect((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_2, argsNode_2);
}
}
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((PGuards.isPFunction(arg0Value_.getFunction()))) {
CallDispatchNode dispatchNode_3;
CallDispatchNode dispatchNode_3_shared = this.dispatchNode;
if (dispatchNode_3_shared != null) {
dispatchNode_3 = dispatchNode_3_shared;
} else {
dispatchNode_3 = this.insert((CallDispatchNode.create()));
if (dispatchNode_3 == null) {
throw new IllegalStateException("Specialization 'methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
VarHandle.storeStoreFence();
this.dispatchNode = dispatchNode_3;
}
CreateArgumentsNode argsNode_3;
CreateArgumentsNode argsNode_3_shared = this.argsNode;
if (argsNode_3_shared != null) {
argsNode_3 = argsNode_3_shared;
} else {
argsNode_3 = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_3 == null) {
throw new IllegalStateException("Specialization 'methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
VarHandle.storeStoreFence();
this.argsNode = argsNode_3;
}
state_0 = state_0 & 0xffffff7f /* remove SpecializationActive[CallNode.methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[CallNode.methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */;
this.state_0_ = state_0;
return CallNode.methodCallDirect((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_3, argsNode_3);
}
}
}
if (arg0Value instanceof PBuiltinMethod) {
PBuiltinMethod arg0Value_ = (PBuiltinMethod) arg0Value;
{
PBuiltinMethod cachedCallable__ = null;
if (((state_0 & 0b101000000000)) == 0 /* is-not SpecializationActive[CallNode.builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] && SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
while (true) {
int count9_ = 0;
BuiltinMethodCallBuiltinDirectCachedData s9_ = BUILTIN_METHOD_CALL_BUILTIN_DIRECT_CACHED_CACHE_UPDATER.getVolatile(this);
BuiltinMethodCallBuiltinDirectCachedData s9_original = s9_;
while (s9_ != null) {
{
CallDispatchNode dispatchNode_4 = this.dispatchNode;
if (dispatchNode_4 != null) {
CreateArgumentsNode argsNode_4 = this.argsNode;
if (argsNode_4 != null) {
cachedCallable__ = (s9_.weakCachedCallableGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedCallable__ != null) && (arg0Value_ == cachedCallable__) && (PGuards.isPBuiltinFunction(cachedCallable__.getFunction()))) {
break;
}
}
}
}
count9_++;
s9_ = null;
break;
}
if (s9_ == null && count9_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference weakCachedCallableGen___ = (new TruffleWeakReference<>(arg0Value_));
cachedCallable__ = (weakCachedCallableGen___.get());
if ((cachedCallable__ != null) && (arg0Value_ == cachedCallable__) && (PGuards.isPBuiltinFunction(cachedCallable__.getFunction()))) {
s9_ = new BuiltinMethodCallBuiltinDirectCachedData();
s9_.weakCachedCallableGen__ = weakCachedCallableGen___;
CallDispatchNode dispatchNode_4;
CallDispatchNode dispatchNode_4_shared = this.dispatchNode;
if (dispatchNode_4_shared != null) {
dispatchNode_4 = dispatchNode_4_shared;
} else {
dispatchNode_4 = this.insert((CallDispatchNode.create()));
if (dispatchNode_4 == null) {
throw new IllegalStateException("Specialization 'builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
this.dispatchNode = dispatchNode_4;
}
CreateArgumentsNode argsNode_4;
CreateArgumentsNode argsNode_4_shared = this.argsNode;
if (argsNode_4_shared != null) {
argsNode_4 = argsNode_4_shared;
} else {
argsNode_4 = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_4 == null) {
throw new IllegalStateException("Specialization 'builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
this.argsNode = argsNode_4;
}
if (!BUILTIN_METHOD_CALL_BUILTIN_DIRECT_CACHED_CACHE_UPDATER.compareAndSet(this, s9_original, s9_)) {
continue;
}
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[CallNode.builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)] */;
this.state_0_ = state_0;
}
}
}
if (s9_ != null) {
return CallNode.builtinMethodCallBuiltinDirectCached((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, cachedCallable__, this.dispatchNode, this.argsNode);
}
break;
}
}
}
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */) {
if ((PGuards.isPBuiltinFunction(arg0Value_.getFunction()))) {
CallDispatchNode dispatchNode_5;
CallDispatchNode dispatchNode_5_shared = this.dispatchNode;
if (dispatchNode_5_shared != null) {
dispatchNode_5 = dispatchNode_5_shared;
} else {
dispatchNode_5 = this.insert((CallDispatchNode.create()));
if (dispatchNode_5 == null) {
throw new IllegalStateException("Specialization 'builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
VarHandle.storeStoreFence();
this.dispatchNode = dispatchNode_5;
}
CreateArgumentsNode argsNode_5;
CreateArgumentsNode argsNode_5_shared = this.argsNode;
if (argsNode_5_shared != null) {
argsNode_5 = argsNode_5_shared;
} else {
argsNode_5 = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_5 == null) {
throw new IllegalStateException("Specialization 'builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
VarHandle.storeStoreFence();
this.argsNode = argsNode_5;
}
this.builtinMethodCallBuiltinDirectCached_cache = null;
state_0 = state_0 & 0xfffffbff /* remove SpecializationActive[CallNode.builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)] */;
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[CallNode.builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)] */;
this.state_0_ = state_0;
return CallNode.builtinMethodCallBuiltinDirect((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, dispatchNode_5, argsNode_5);
}
}
}
{
Node inliningTarget__4 = null;
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PMethod) {
PMethod arg0Value_ = (PMethod) arg0Value;
if ((!(PGuards.isFunction(arg0Value_.getFunction())))) {
inliningTarget__4 = (this);
PRaiseNode raise_4;
PRaiseNode raise_4_shared = this.raise;
if (raise_4_shared != null) {
raise_4 = raise_4_shared;
} else {
raise_4 = this.insert((PRaiseNode.create()));
if (raise_4 == null) {
throw new IllegalStateException("Specialization 'methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
VarHandle.storeStoreFence();
this.raise = raise_4;
}
LookupSpecialMethodSlotNode lookupCall_3;
LookupSpecialMethodSlotNode lookupCall_3_shared = this.lookupCall;
if (lookupCall_3_shared != null) {
lookupCall_3 = lookupCall_3_shared;
} else {
lookupCall_3 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Call)));
if (lookupCall_3 == null) {
throw new IllegalStateException("Specialization 'methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'lookupCall' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupCall == null) {
VarHandle.storeStoreFence();
this.lookupCall = lookupCall_3;
}
CallVarargsMethodNode callCall_3;
CallVarargsMethodNode callCall_3_shared = this.callCall;
if (callCall_3_shared != null) {
callCall_3 = callCall_3_shared;
} else {
callCall_3 = this.insert((CallVarargsMethodNode.create()));
if (callCall_3 == null) {
throw new IllegalStateException("Specialization 'methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'callCallNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callCall == null) {
VarHandle.storeStoreFence();
this.callCall = callCall_3;
}
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[CallNode.methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
this.state_0_ = state_0;
return CallNode.methodCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, inliningTarget__4, raise_4, INLINED_GET_CLASS_NODE, lookupCall_3, callCall_3);
}
}
}
{
Node inliningTarget__5 = null;
if (((state_0 & 0b1000000000)) == 0 /* is-not SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */ && arg0Value instanceof PBuiltinMethod) {
PBuiltinMethod arg0Value_ = (PBuiltinMethod) arg0Value;
if ((!(PGuards.isFunction(arg0Value_.getFunction())))) {
inliningTarget__5 = (this);
PRaiseNode raise_5;
PRaiseNode raise_5_shared = this.raise;
if (raise_5_shared != null) {
raise_5 = raise_5_shared;
} else {
raise_5 = this.insert((PRaiseNode.create()));
if (raise_5 == null) {
throw new IllegalStateException("Specialization 'builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
VarHandle.storeStoreFence();
this.raise = raise_5;
}
LookupSpecialMethodSlotNode lookupCall_4;
LookupSpecialMethodSlotNode lookupCall_4_shared = this.lookupCall;
if (lookupCall_4_shared != null) {
lookupCall_4 = lookupCall_4_shared;
} else {
lookupCall_4 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Call)));
if (lookupCall_4 == null) {
throw new IllegalStateException("Specialization 'builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'lookupCall' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupCall == null) {
VarHandle.storeStoreFence();
this.lookupCall = lookupCall_4;
}
CallVarargsMethodNode callVarargs_;
CallVarargsMethodNode callVarargs__shared = this.callVarargs;
if (callVarargs__shared != null) {
callVarargs_ = callVarargs__shared;
} else {
callVarargs_ = this.insert((CallVarargsMethodNode.create()));
if (callVarargs_ == null) {
throw new IllegalStateException("Specialization 'builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'callCallNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callVarargs == null) {
VarHandle.storeStoreFence();
this.callVarargs = callVarargs_;
}
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[CallNode.builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
this.state_0_ = state_0;
return CallNode.builtinMethodCall((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, inliningTarget__5, raise_5, INLINED_GET_CLASS_NODE, lookupCall_4, callVarargs_);
}
}
}
{
Node inliningTarget__6 = null;
if ((!(CallNode.isForeignMethod(arg0Value)))) {
inliningTarget__6 = (this);
CallDispatchNode dispatchNode_6;
CallDispatchNode dispatchNode_6_shared = this.dispatchNode;
if (dispatchNode_6_shared != null) {
dispatchNode_6 = dispatchNode_6_shared;
} else {
dispatchNode_6 = this.insert((CallDispatchNode.create()));
if (dispatchNode_6 == null) {
throw new IllegalStateException("Specialization 'doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'dispatch' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchNode == null) {
VarHandle.storeStoreFence();
this.dispatchNode = dispatchNode_6;
}
CreateArgumentsNode argsNode_6;
CreateArgumentsNode argsNode_6_shared = this.argsNode;
if (argsNode_6_shared != null) {
argsNode_6 = argsNode_6_shared;
} else {
argsNode_6 = this.insert((CreateArgumentsNodeGen.create()));
if (argsNode_6 == null) {
throw new IllegalStateException("Specialization 'doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'createArgs' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.argsNode == null) {
VarHandle.storeStoreFence();
this.argsNode = argsNode_6;
}
PRaiseNode raise_6;
PRaiseNode raise_6_shared = this.raise;
if (raise_6_shared != null) {
raise_6 = raise_6_shared;
} else {
raise_6 = this.insert((PRaiseNode.create()));
if (raise_6 == null) {
throw new IllegalStateException("Specialization 'doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'raise' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.raise == null) {
VarHandle.storeStoreFence();
this.raise = raise_6;
}
LookupSpecialMethodSlotNode lookupCall_5;
LookupSpecialMethodSlotNode lookupCall_5_shared = this.lookupCall;
if (lookupCall_5_shared != null) {
lookupCall_5 = lookupCall_5_shared;
} else {
lookupCall_5 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.Call)));
if (lookupCall_5 == null) {
throw new IllegalStateException("Specialization 'doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'lookupCall' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupCall == null) {
VarHandle.storeStoreFence();
this.lookupCall = lookupCall_5;
}
CallVarargsMethodNode callVarargs_1;
CallVarargsMethodNode callVarargs_1_shared = this.callVarargs;
if (callVarargs_1_shared != null) {
callVarargs_1 = callVarargs_1_shared;
} else {
callVarargs_1 = this.insert((CallVarargsMethodNode.create()));
if (callVarargs_1 == null) {
throw new IllegalStateException("Specialization 'doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)' contains a shared cache with name 'callCallNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callVarargs == null) {
VarHandle.storeStoreFence();
this.callVarargs = callVarargs_1;
}
this.callCall = null;
this.builtinMethodCallBuiltinDirectCached_cache = null;
state_0 = state_0 & 0xffffc241 /* remove SpecializationActive[CallNode.functionCall(VirtualFrame, PFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)], SpecializationActive[CallNode.builtinFunctionCall(VirtualFrame, PBuiltinFunction, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)], SpecializationActive[CallNode.doType(VirtualFrame, PythonBuiltinClassType, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)], SpecializationActive[CallNode.doPythonClass(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)], SpecializationActive[CallNode.doObjectAndType(VirtualFrame, Object, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)], SpecializationActive[CallNode.methodCallBuiltinDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)], SpecializationActive[CallNode.methodCallDirect(VirtualFrame, PMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)], SpecializationActive[CallNode.builtinMethodCallBuiltinDirectCached(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], PBuiltinMethod, CallDispatchNode, CreateArgumentsNode)], SpecializationActive[CallNode.builtinMethodCallBuiltinDirect(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], CallDispatchNode, CreateArgumentsNode)], SpecializationActive[CallNode.methodCall(VirtualFrame, PMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)], SpecializationActive[CallNode.builtinMethodCall(VirtualFrame, PBuiltinMethod, Object[], PKeyword[], Node, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[CallNode.doGeneric(VirtualFrame, Object, Object[], PKeyword[], Node, CallDispatchNode, CreateArgumentsNode, PRaiseNode, GetClassNode, LookupSpecialMethodSlotNode, CallVarargsMethodNode)] */;
this.state_0_ = state_0;
return CallNode.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__6, dispatchNode_6, argsNode_6, raise_6, INLINED_GET_CLASS_NODE, lookupCall_5, callVarargs_1);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
if (((oldState_0 & 0b1000000000) == 0 && (state_0_ & 0b1000000000) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111111111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11111111111111) & ((state_0 & 0b11111111111111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static CallNode create() {
return new CallNodeGen();
}
@NeverDefault
public static CallNode getUncached() {
return CallNodeGen.UNCACHED;
}
@GeneratedBy(CallNode.class)
@DenyReplace
private static final class ForeignMethodData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link InlinedBranchProfile} keywordsError
* Inline method: {@link InlinedBranchProfile#inline}
* 1: InlinedCache
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link InlinedBranchProfile} typeError
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int foreignMethod_state_0_;
/**
* Source Info:
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link PForeignToPTypeNode} fromForeign
*/
@Child PForeignToPTypeNode fromForeign_;
/**
* Source Info:
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link GilNode} gil
*/
@Child GilNode gil_;
/**
* Source Info:
* Specialization: {@link CallNode#doForeignMethod}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
ForeignMethodData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(CallNode.class)
@DenyReplace
private static final class BuiltinMethodCallBuiltinDirectCachedData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link CallNode#builtinMethodCallBuiltinDirectCached}
* Parameter: {@link TruffleWeakReference} weakCachedCallableGen_
*/
@CompilationFinal TruffleWeakReference weakCachedCallableGen__;
BuiltinMethodCallBuiltinDirectCachedData() {
}
}
@GeneratedBy(CallNode.class)
@DenyReplace
private static final class Uncached extends CallNode {
@Override
protected Object executeInternal(Frame frameValue, Object arg0Value, Object[] arg1Value, PKeyword[] arg2Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg0Value instanceof BoundDescriptor) {
BoundDescriptor arg0Value_ = (BoundDescriptor) arg0Value;
return CallNode.boundDescriptor((VirtualFrame) frameValue, arg0Value_, arg1Value, arg2Value, (CallNode.getUncached()));
}
if (arg0Value instanceof ForeignMethod) {
ForeignMethod arg0Value_ = (ForeignMethod) arg0Value;
return CallNode.doForeignMethod(arg0Value_, arg1Value, arg2Value, (this), (PRaiseNode.getUncached()), (PForeignToPTypeNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (GilNode.getUncached()), (INTEROP_LIBRARY_.getUncached()));
}
if ((!(CallNode.isForeignMethod(arg0Value)))) {
return CallNode.doGeneric((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, (this), (CallDispatchNode.getUncached()), (CreateArgumentsNodeGen.getUncached()), (PRaiseNode.getUncached()), (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.Call)), (CallVarargsMethodNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link Lazy#doIt}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(Lazy.class)
@SuppressWarnings("javadoc")
public static final class LazyNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static Lazy getUncached() {
return LazyNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#callNode_}
*
*/
@NeverDefault
public static Lazy inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new LazyNodeGen.Inlined(target);
}
@GeneratedBy(Lazy.class)
@DenyReplace
private static final class Inlined extends Lazy {
/**
* State Info:
* 0: SpecializationActive {@link Lazy#doIt}
*
*/
private final StateField state_0_;
private final ReferenceField callNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(Lazy.class);
this.state_0_ = target.getState(0, 1);
this.callNode_ = target.getReference(1, CallNode.class);
}
@Override
CallNode execute(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[CallNode.Lazy.doIt(CallNode)] */) {
{
CallNode callNode__ = this.callNode_.get(arg0Value);
if (callNode__ != null) {
return Lazy.doIt(callNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private CallNode executeAndSpecialize(Node arg0Value) {
int state_0 = this.state_0_.get(arg0Value);
CallNode callNode__ = arg0Value.insert((CallNode.create()));
Objects.requireNonNull(callNode__, "Specialization 'doIt(CallNode)' cache 'callNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.callNode_.set(arg0Value, callNode__);
state_0 = state_0 | 0b1 /* add SpecializationActive[CallNode.Lazy.doIt(CallNode)] */;
this.state_0_.set(arg0Value, state_0);
return Lazy.doIt(callNode__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(Lazy.class)
@DenyReplace
private static final class Uncached extends Lazy {
@TruffleBoundary
@Override
CallNode execute(Node arg0Value) {
return Lazy.doIt((CallNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy