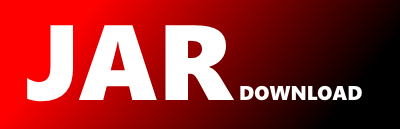
com.oracle.graal.python.nodes.call.GenericInvokeNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.call;
import com.oracle.graal.python.builtins.objects.function.PBuiltinFunction;
import com.oracle.graal.python.builtins.objects.function.PFunction;
import com.oracle.graal.python.runtime.ExecutionContext.CallContext;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.RootCallTarget;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.IndirectCallNode;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link GenericInvokeNode#invokeFunctionWithFrame}
* Activation probability: 0.27381
* With/without class size: 8/0 bytes
* Specialization {@link GenericInvokeNode#invokeBuiltinWithFrame}
* Activation probability: 0.23095
* With/without class size: 7/0 bytes
* Specialization {@link GenericInvokeNode#invokeCallTargetWithFrame}
* Activation probability: 0.18810
* With/without class size: 7/0 bytes
* Specialization {@link GenericInvokeNode#invokeFunction}
* Activation probability: 0.14524
* With/without class size: 6/0 bytes
* Specialization {@link GenericInvokeNode#invokeBuiltin}
* Activation probability: 0.10238
* With/without class size: 5/0 bytes
* Specialization {@link GenericInvokeNode#invokeCallTarget}
* Activation probability: 0.05952
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(GenericInvokeNode.class)
@SuppressWarnings("javadoc")
public final class GenericInvokeNodeGen extends GenericInvokeNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link GenericInvokeNode#invokeFunctionWithFrame}
* Parameter: {@link InlinedConditionProfile} isGeneratorFunctionProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_GENERATOR_FUNCTION_PROFILE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_UPDATER.subUpdater(6, 2)));
/**
* Source Info:
* Specialization: {@link GenericInvokeNode#invokeFunction}
* Parameter: {@link InlinedConditionProfile} isNullFrameProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_NULL_FRAME_PROFILE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_UPDATER.subUpdater(8, 2)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link GenericInvokeNode#invokeFunctionWithFrame}
* 1: SpecializationActive {@link GenericInvokeNode#invokeFunction}
* 2: SpecializationActive {@link GenericInvokeNode#invokeBuiltinWithFrame}
* 3: SpecializationActive {@link GenericInvokeNode#invokeBuiltin}
* 4: SpecializationActive {@link GenericInvokeNode#invokeCallTargetWithFrame}
* 5: SpecializationActive {@link GenericInvokeNode#invokeCallTarget}
* 6-7: InlinedCache
* Specialization: {@link GenericInvokeNode#invokeFunctionWithFrame}
* Parameter: {@link InlinedConditionProfile} isGeneratorFunctionProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 8-9: InlinedCache
* Specialization: {@link GenericInvokeNode#invokeFunction}
* Parameter: {@link InlinedConditionProfile} isNullFrameProfile
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link GenericInvokeNode#invokeFunctionWithFrame}
* Parameter: {@link IndirectCallNode} callNode
*/
@Child private IndirectCallNode callNode;
/**
* Source Info:
* Specialization: {@link GenericInvokeNode#invokeFunctionWithFrame}
* Parameter: {@link CallContext} callContext
*/
@Child private CallContext callContext;
private GenericInvokeNodeGen() {
}
@Override
protected Object executeInternal(Frame frameValue, Object arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111) != 0 /* is SpecializationActive[GenericInvokeNode.invokeFunctionWithFrame(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] || SpecializationActive[GenericInvokeNode.invokeBuiltinWithFrame(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] || SpecializationActive[GenericInvokeNode.invokeCallTargetWithFrame(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] || SpecializationActive[GenericInvokeNode.invokeFunction(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] || SpecializationActive[GenericInvokeNode.invokeBuiltin(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] || SpecializationActive[GenericInvokeNode.invokeCallTarget(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GenericInvokeNode.invokeFunctionWithFrame(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */ && arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
{
IndirectCallNode callNode_ = this.callNode;
if (callNode_ != null) {
CallContext callContext_ = this.callContext;
if (callContext_ != null) {
if ((frameValue != null)) {
Node inliningTarget__ = (this);
return invokeFunctionWithFrame(frameValue, arg0Value_, arg1Value, inliningTarget__, callNode_, callContext_, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GenericInvokeNode.invokeBuiltinWithFrame(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */ && arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
{
IndirectCallNode callNode_1 = this.callNode;
if (callNode_1 != null) {
CallContext callContext_1 = this.callContext;
if (callContext_1 != null) {
if ((frameValue != null)) {
Node inliningTarget__1 = (this);
return invokeBuiltinWithFrame(frameValue, arg0Value_, arg1Value, inliningTarget__1, callNode_1, callContext_1, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[GenericInvokeNode.invokeCallTargetWithFrame(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */ && arg0Value instanceof RootCallTarget) {
RootCallTarget arg0Value_ = (RootCallTarget) arg0Value;
{
IndirectCallNode callNode_2 = this.callNode;
if (callNode_2 != null) {
CallContext callContext_2 = this.callContext;
if (callContext_2 != null) {
if ((frameValue != null)) {
Node inliningTarget__2 = (this);
return invokeCallTargetWithFrame(frameValue, arg0Value_, arg1Value, inliningTarget__2, callNode_2, callContext_2, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GenericInvokeNode.invokeFunction(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */ && arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
{
IndirectCallNode callNode_3 = this.callNode;
if (callNode_3 != null) {
CallContext callContext_3 = this.callContext;
if (callContext_3 != null) {
Node inliningTarget__3 = (this);
return invokeFunction(frameValue, arg0Value_, arg1Value, inliningTarget__3, callNode_3, callContext_3, INLINED_IS_NULL_FRAME_PROFILE, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GenericInvokeNode.invokeBuiltin(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */ && arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
{
IndirectCallNode callNode_4 = this.callNode;
if (callNode_4 != null) {
CallContext callContext_4 = this.callContext;
if (callContext_4 != null) {
Node inliningTarget__4 = (this);
return invokeBuiltin(frameValue, arg0Value_, arg1Value, inliningTarget__4, callNode_4, callContext_4, INLINED_IS_NULL_FRAME_PROFILE, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[GenericInvokeNode.invokeCallTarget(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */ && arg0Value instanceof RootCallTarget) {
RootCallTarget arg0Value_ = (RootCallTarget) arg0Value;
{
IndirectCallNode callNode_5 = this.callNode;
if (callNode_5 != null) {
CallContext callContext_5 = this.callContext;
if (callContext_5 != null) {
Node inliningTarget__5 = (this);
return invokeCallTarget(frameValue, arg0Value_, arg1Value, inliningTarget__5, callNode_5, callContext_5, INLINED_IS_NULL_FRAME_PROFILE, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value);
}
private Object executeAndSpecialize(Frame frameValue, Object arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[GenericInvokeNode.invokeFunction(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */ && arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
if ((frameValue != null)) {
inliningTarget__ = (this);
IndirectCallNode callNode_;
IndirectCallNode callNode__shared = this.callNode;
if (callNode__shared != null) {
callNode_ = callNode__shared;
} else {
callNode_ = this.insert((IndirectCallNode.create()));
if (callNode_ == null) {
throw new IllegalStateException("Specialization 'invokeFunctionWithFrame(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_;
}
CallContext callContext_;
CallContext callContext__shared = this.callContext;
if (callContext__shared != null) {
callContext_ = callContext__shared;
} else {
callContext_ = this.insert((CallContext.create()));
if (callContext_ == null) {
throw new IllegalStateException("Specialization 'invokeFunctionWithFrame(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)' contains a shared cache with name 'callContext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callContext == null) {
VarHandle.storeStoreFence();
this.callContext = callContext_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[GenericInvokeNode.invokeFunctionWithFrame(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return invokeFunctionWithFrame(frameValue, arg0Value_, arg1Value, inliningTarget__, callNode_, callContext_, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
{
Node inliningTarget__1 = null;
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[GenericInvokeNode.invokeBuiltin(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */ && arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
if ((frameValue != null)) {
inliningTarget__1 = (this);
IndirectCallNode callNode_1;
IndirectCallNode callNode_1_shared = this.callNode;
if (callNode_1_shared != null) {
callNode_1 = callNode_1_shared;
} else {
callNode_1 = this.insert((IndirectCallNode.create()));
if (callNode_1 == null) {
throw new IllegalStateException("Specialization 'invokeBuiltinWithFrame(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_1;
}
CallContext callContext_1;
CallContext callContext_1_shared = this.callContext;
if (callContext_1_shared != null) {
callContext_1 = callContext_1_shared;
} else {
callContext_1 = this.insert((CallContext.create()));
if (callContext_1 == null) {
throw new IllegalStateException("Specialization 'invokeBuiltinWithFrame(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)' contains a shared cache with name 'callContext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callContext == null) {
VarHandle.storeStoreFence();
this.callContext = callContext_1;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[GenericInvokeNode.invokeBuiltinWithFrame(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return invokeBuiltinWithFrame(frameValue, arg0Value_, arg1Value, inliningTarget__1, callNode_1, callContext_1, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
{
Node inliningTarget__2 = null;
if (((state_0 & 0b100000)) == 0 /* is-not SpecializationActive[GenericInvokeNode.invokeCallTarget(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */ && arg0Value instanceof RootCallTarget) {
RootCallTarget arg0Value_ = (RootCallTarget) arg0Value;
if ((frameValue != null)) {
inliningTarget__2 = (this);
IndirectCallNode callNode_2;
IndirectCallNode callNode_2_shared = this.callNode;
if (callNode_2_shared != null) {
callNode_2 = callNode_2_shared;
} else {
callNode_2 = this.insert((IndirectCallNode.create()));
if (callNode_2 == null) {
throw new IllegalStateException("Specialization 'invokeCallTargetWithFrame(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_2;
}
CallContext callContext_2;
CallContext callContext_2_shared = this.callContext;
if (callContext_2_shared != null) {
callContext_2 = callContext_2_shared;
} else {
callContext_2 = this.insert((CallContext.create()));
if (callContext_2 == null) {
throw new IllegalStateException("Specialization 'invokeCallTargetWithFrame(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)' contains a shared cache with name 'callContext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callContext == null) {
VarHandle.storeStoreFence();
this.callContext = callContext_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[GenericInvokeNode.invokeCallTargetWithFrame(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return invokeCallTargetWithFrame(frameValue, arg0Value_, arg1Value, inliningTarget__2, callNode_2, callContext_2, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
}
{
Node inliningTarget__3 = null;
if (arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
inliningTarget__3 = (this);
IndirectCallNode callNode_3;
IndirectCallNode callNode_3_shared = this.callNode;
if (callNode_3_shared != null) {
callNode_3 = callNode_3_shared;
} else {
callNode_3 = this.insert((IndirectCallNode.create()));
if (callNode_3 == null) {
throw new IllegalStateException("Specialization 'invokeFunction(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_3;
}
CallContext callContext_3;
CallContext callContext_3_shared = this.callContext;
if (callContext_3_shared != null) {
callContext_3 = callContext_3_shared;
} else {
callContext_3 = this.insert((CallContext.create()));
if (callContext_3 == null) {
throw new IllegalStateException("Specialization 'invokeFunction(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)' contains a shared cache with name 'callContext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callContext == null) {
VarHandle.storeStoreFence();
this.callContext = callContext_3;
}
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[GenericInvokeNode.invokeFunctionWithFrame(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[GenericInvokeNode.invokeFunction(Frame, PFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return invokeFunction(frameValue, arg0Value_, arg1Value, inliningTarget__3, callNode_3, callContext_3, INLINED_IS_NULL_FRAME_PROFILE, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
{
Node inliningTarget__4 = null;
if (arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
inliningTarget__4 = (this);
IndirectCallNode callNode_4;
IndirectCallNode callNode_4_shared = this.callNode;
if (callNode_4_shared != null) {
callNode_4 = callNode_4_shared;
} else {
callNode_4 = this.insert((IndirectCallNode.create()));
if (callNode_4 == null) {
throw new IllegalStateException("Specialization 'invokeBuiltin(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_4;
}
CallContext callContext_4;
CallContext callContext_4_shared = this.callContext;
if (callContext_4_shared != null) {
callContext_4 = callContext_4_shared;
} else {
callContext_4 = this.insert((CallContext.create()));
if (callContext_4 == null) {
throw new IllegalStateException("Specialization 'invokeBuiltin(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)' contains a shared cache with name 'callContext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callContext == null) {
VarHandle.storeStoreFence();
this.callContext = callContext_4;
}
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[GenericInvokeNode.invokeBuiltinWithFrame(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[GenericInvokeNode.invokeBuiltin(Frame, PBuiltinFunction, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return invokeBuiltin(frameValue, arg0Value_, arg1Value, inliningTarget__4, callNode_4, callContext_4, INLINED_IS_NULL_FRAME_PROFILE, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
{
Node inliningTarget__5 = null;
if (arg0Value instanceof RootCallTarget) {
RootCallTarget arg0Value_ = (RootCallTarget) arg0Value;
inliningTarget__5 = (this);
IndirectCallNode callNode_5;
IndirectCallNode callNode_5_shared = this.callNode;
if (callNode_5_shared != null) {
callNode_5 = callNode_5_shared;
} else {
callNode_5 = this.insert((IndirectCallNode.create()));
if (callNode_5 == null) {
throw new IllegalStateException("Specialization 'invokeCallTarget(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)' contains a shared cache with name 'callNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_5;
}
CallContext callContext_5;
CallContext callContext_5_shared = this.callContext;
if (callContext_5_shared != null) {
callContext_5 = callContext_5_shared;
} else {
callContext_5 = this.insert((CallContext.create()));
if (callContext_5 == null) {
throw new IllegalStateException("Specialization 'invokeCallTarget(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)' contains a shared cache with name 'callContext' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callContext == null) {
VarHandle.storeStoreFence();
this.callContext = callContext_5;
}
state_0 = state_0 & 0xffffffef /* remove SpecializationActive[GenericInvokeNode.invokeCallTargetWithFrame(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile)] */;
state_0 = state_0 | 0b100000 /* add SpecializationActive[GenericInvokeNode.invokeCallTarget(Frame, RootCallTarget, Object[], Node, IndirectCallNode, CallContext, InlinedConditionProfile, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return invokeCallTarget(frameValue, arg0Value_, arg1Value, inliningTarget__5, callNode_5, callContext_5, INLINED_IS_NULL_FRAME_PROFILE, INLINED_IS_GENERATOR_FUNCTION_PROFILE);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b111111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b111111) & ((state_0 & 0b111111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static GenericInvokeNode create() {
return new GenericInvokeNodeGen();
}
@NeverDefault
public static GenericInvokeNode getUncached() {
return GenericInvokeNodeGen.UNCACHED;
}
@GeneratedBy(GenericInvokeNode.class)
@DenyReplace
private static final class Uncached extends GenericInvokeNode {
@Override
protected Object executeInternal(Frame frameValue, Object arg0Value, Object[] arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg0Value instanceof PFunction) {
PFunction arg0Value_ = (PFunction) arg0Value;
return invokeFunction(frameValue, arg0Value_, arg1Value, (this), (IndirectCallNode.getUncached()), (CallContext.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()));
}
if (arg0Value instanceof PBuiltinFunction) {
PBuiltinFunction arg0Value_ = (PBuiltinFunction) arg0Value;
return invokeBuiltin(frameValue, arg0Value_, arg1Value, (this), (IndirectCallNode.getUncached()), (CallContext.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()));
}
if (arg0Value instanceof RootCallTarget) {
RootCallTarget arg0Value_ = (RootCallTarget) arg0Value;
return invokeCallTarget(frameValue, arg0Value_, arg1Value, (this), (IndirectCallNode.getUncached()), (CallContext.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy