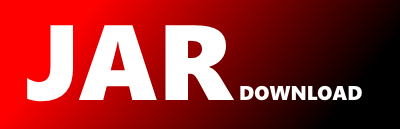
com.oracle.graal.python.nodes.exception.ValidExceptionNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.exception;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.type.PythonBuiltinClass;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.IsTypeNode;
import com.oracle.graal.python.builtins.objects.type.TypeNodesFactory.IsTypeNodeGen;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link ValidExceptionNode#isPythonExceptionTypeCached}
* Activation probability: 0.32000
* With/without class size: 10/5 bytes
* Specialization {@link ValidExceptionNode#isPythonExceptionClassCached}
* Activation probability: 0.26000
* With/without class size: 9/5 bytes
* Specialization {@link ValidExceptionNode#isPythonException}
* Activation probability: 0.20000
* With/without class size: 9/9 bytes
* Specialization {@link ValidExceptionNode#isJavaException}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link ValidExceptionNode#isAnException}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(ValidExceptionNode.class)
@SuppressWarnings("javadoc")
public final class ValidExceptionNodeGen extends ValidExceptionNode {
private static final StateField IS_PYTHON_EXCEPTION__VALID_EXCEPTION_NODE_IS_PYTHON_EXCEPTION_STATE_0_UPDATER = StateField.create(IsPythonExceptionData.lookup_(), "isPythonException_state_0_");
static final ReferenceField IS_PYTHON_EXCEPTION_TYPE_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "isPythonExceptionTypeCached_cache", IsPythonExceptionTypeCachedData.class);
static final ReferenceField IS_PYTHON_EXCEPTION_CLASS_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "isPythonExceptionClassCached_cache", IsPythonExceptionClassCachedData.class);
static final ReferenceField IS_PYTHON_EXCEPTION_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "isPythonException_cache", IsPythonExceptionData.class);
/**
* Source Info:
* Specialization: {@link ValidExceptionNode#isPythonException}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
*/
private static final IsTypeNode INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_ = IsTypeNodeGen.inline(InlineTarget.create(IsTypeNode.class, IS_PYTHON_EXCEPTION__VALID_EXCEPTION_NODE_IS_PYTHON_EXCEPTION_STATE_0_UPDATER.subUpdater(2, 5), ReferenceField.create(IsPythonExceptionData.lookup_(), "isPythonException_isTypeNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
@CompilationFinal(dimensions = 1) private static final PythonBuiltinClassType[] PYTHON_BUILTIN_CLASS_TYPE_VALUES = DSLSupport.lookupEnumConstants(PythonBuiltinClassType.class);
/**
* State Info:
* 0: SpecializationActive {@link ValidExceptionNode#isPythonExceptionTypeCached}
* 1: SpecializationActive {@link ValidExceptionNode#isPythonException}
* 2: SpecializationActive {@link ValidExceptionNode#isPythonExceptionClassCached}
* 3: SpecializationActive {@link ValidExceptionNode#isJavaException}
* 4: SpecializationActive {@link ValidExceptionNode#isAnException}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private IsPythonExceptionTypeCachedData isPythonExceptionTypeCached_cache;
@UnsafeAccessedField @CompilationFinal private IsPythonExceptionClassCachedData isPythonExceptionClassCached_cache;
@UnsafeAccessedField @Child private IsPythonExceptionData isPythonException_cache;
private ValidExceptionNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value) {
{
IsPythonExceptionData s2_ = this.isPythonException_cache;
if ((s2_ == null || ((s2_.isPythonException_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode), guardIndex=0] */ || (INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_.execute((s2_), arg0Value)))) {
return false;
}
}
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[ValidExceptionNode.isJavaException(VirtualFrame, Object)] */) && (emulateJython()) && (isHostObject(arg0Value))) {
return false;
}
return true;
}
@ExplodeLoop
@Override
public boolean execute(Frame frameValue, Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[ValidExceptionNode.isPythonExceptionTypeCached(PythonBuiltinClassType, PythonBuiltinClassType, boolean)] || SpecializationActive[ValidExceptionNode.isPythonExceptionClassCached(PythonBuiltinClass, PythonBuiltinClassType, boolean)] || SpecializationActive[ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)] || SpecializationActive[ValidExceptionNode.isJavaException(VirtualFrame, Object)] || SpecializationActive[ValidExceptionNode.isAnException(VirtualFrame, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ValidExceptionNode.isPythonExceptionTypeCached(PythonBuiltinClassType, PythonBuiltinClassType, boolean)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
IsPythonExceptionTypeCachedData s0_ = this.isPythonExceptionTypeCached_cache;
while (s0_ != null) {
if ((decodePythonBuiltinClassType((s0_.isPythonExceptionTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 1) == arg0Value_)) {
return ValidExceptionNode.isPythonExceptionTypeCached(arg0Value_, decodePythonBuiltinClassType((s0_.isPythonExceptionTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 1), s0_.isExceptionType_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ValidExceptionNode.isPythonExceptionClassCached(PythonBuiltinClass, PythonBuiltinClassType, boolean)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
IsPythonExceptionClassCachedData s1_ = this.isPythonExceptionClassCached_cache;
while (s1_ != null) {
if ((decodePythonBuiltinClassType((s1_.isPythonExceptionClassCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionClassCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2) == arg0Value_.getType())) {
return ValidExceptionNode.isPythonExceptionClassCached(arg0Value_, decodePythonBuiltinClassType((s1_.isPythonExceptionClassCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionClassCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2), s1_.isExceptionType_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b11010) != 0 /* is SpecializationActive[ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)] || SpecializationActive[ValidExceptionNode.isJavaException(VirtualFrame, Object)] || SpecializationActive[ValidExceptionNode.isAnException(VirtualFrame, Object)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)] */) {
IsPythonExceptionData s2_ = this.isPythonException_cache;
if (s2_ != null) {
if ((s2_.isPythonException_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
Node inliningTarget__ = (s2_);
if ((INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_.execute(inliningTarget__, arg0Value))) {
return ValidExceptionNode.isPythonException((VirtualFrame) frameValue, arg0Value, inliningTarget__, INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_, s2_.isSubtype_);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ValidExceptionNode.isJavaException(VirtualFrame, Object)] */) {
assert DSLSupport.assertIdempotence((emulateJython()));
if ((isHostObject(arg0Value))) {
return isJavaException((VirtualFrame) frameValue, arg0Value);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ValidExceptionNode.isAnException(VirtualFrame, Object)] */) {
if (fallbackGuard_(state_0, arg0Value)) {
return ValidExceptionNode.isAnException((VirtualFrame) frameValue, arg0Value);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value);
}
private boolean executeAndSpecialize(Frame frameValue, Object arg0Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)] */ && arg0Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg0Value_ = (PythonBuiltinClassType) arg0Value;
while (true) {
int count0_ = 0;
IsPythonExceptionTypeCachedData s0_ = IS_PYTHON_EXCEPTION_TYPE_CACHED_CACHE_UPDATER.getVolatile(this);
IsPythonExceptionTypeCachedData s0_original = s0_;
while (s0_ != null) {
if ((decodePythonBuiltinClassType((s0_.isPythonExceptionTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 1) == arg0Value_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (decodePythonBuiltinClassType((s0_.isPythonExceptionTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 1) == arg0Value_);
if (count0_ < (3)) {
s0_ = new IsPythonExceptionTypeCachedData(s0_original);
s0_.isPythonExceptionTypeCached_state_0_ = (s0_.isPythonExceptionTypeCached_state_0_ | (((arg0Value_).ordinal() + 1) << 0) /* set-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)] */);
s0_.isExceptionType_ = (ValidExceptionNode.isPythonExceptionType(arg0Value_));
if (!IS_PYTHON_EXCEPTION_TYPE_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[ValidExceptionNode.isPythonExceptionTypeCached(PythonBuiltinClassType, PythonBuiltinClassType, boolean)] */;
this.state_0_ = state_0;
}
}
if (s0_ != null) {
return ValidExceptionNode.isPythonExceptionTypeCached(arg0Value_, decodePythonBuiltinClassType((s0_.isPythonExceptionTypeCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)] */) - 1), s0_.isExceptionType_);
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)] */ && arg0Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg0Value_ = (PythonBuiltinClass) arg0Value;
while (true) {
int count1_ = 0;
IsPythonExceptionClassCachedData s1_ = IS_PYTHON_EXCEPTION_CLASS_CACHED_CACHE_UPDATER.getVolatile(this);
IsPythonExceptionClassCachedData s1_original = s1_;
while (s1_ != null) {
if ((decodePythonBuiltinClassType((s1_.isPythonExceptionClassCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionClassCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2) == arg0Value_.getType())) {
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
PythonBuiltinClassType cachedType__ = (arg0Value_.getType());
if ((cachedType__ == arg0Value_.getType()) && count1_ < (3)) {
s1_ = new IsPythonExceptionClassCachedData(s1_original);
s1_.isPythonExceptionClassCached_state_0_ = (s1_.isPythonExceptionClassCached_state_0_ | ((encodePythonBuiltinClassType(cachedType__) + 2) << 0) /* set-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionClassCached(..., PythonBuiltinClassType cachedType, ...)] */);
s1_.isExceptionType_ = (ValidExceptionNode.isPythonExceptionType(cachedType__));
if (!IS_PYTHON_EXCEPTION_CLASS_CACHED_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[ValidExceptionNode.isPythonExceptionClassCached(PythonBuiltinClass, PythonBuiltinClassType, boolean)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return ValidExceptionNode.isPythonExceptionClassCached(arg0Value_, decodePythonBuiltinClassType((s1_.isPythonExceptionClassCached_state_0_ >>> 0 /* get-int EncodedEnum[cache=ValidExceptionNode.isPythonExceptionClassCached(..., PythonBuiltinClassType cachedType, ...)] */) - 2), s1_.isExceptionType_);
}
break;
}
}
{
Node inliningTarget__ = null;
while (true) {
int count2_ = 0;
IsPythonExceptionData s2_ = IS_PYTHON_EXCEPTION_CACHE_UPDATER.getVolatile(this);
IsPythonExceptionData s2_original = s2_;
while (s2_ != null) {
if ((s2_.isPythonException_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
inliningTarget__ = (s2_);
if ((INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_.execute(inliningTarget__, arg0Value))) {
break;
}
}
if ((s2_.isPythonException_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count2_++;
}
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
{
s2_ = this.insert(new IsPythonExceptionData());
inliningTarget__ = (s2_);
if (((s2_.isPythonException_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode), guardIndex=0] */) {
s2_.isPythonException_state_0_ = s2_.isPythonException_state_0_ | 0b1 /* add GuardActive[specialization=ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode), guardIndex=0] */;
if (!IS_PYTHON_EXCEPTION_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
s2_original = s2_;
s2_ = this.insert(new IsPythonExceptionData(s2_));
}
if ((INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_.execute(inliningTarget__, arg0Value))) {
IsSubtypeNode isSubtype__ = s2_.insert((IsSubtypeNode.create()));
Objects.requireNonNull(isSubtype__, "Specialization 'isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)' cache 'isSubtype' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.isSubtype_ = isSubtype__;
s2_.isPythonException_state_0_ = s2_.isPythonException_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!IS_PYTHON_EXCEPTION_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
this.isPythonExceptionTypeCached_cache = null;
this.isPythonExceptionClassCached_cache = null;
state_0 = state_0 & 0xfffffffa /* remove SpecializationActive[ValidExceptionNode.isPythonExceptionTypeCached(PythonBuiltinClassType, PythonBuiltinClassType, boolean)], SpecializationActive[ValidExceptionNode.isPythonExceptionClassCached(PythonBuiltinClass, PythonBuiltinClassType, boolean)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[ValidExceptionNode.isPythonException(VirtualFrame, Object, Node, IsTypeNode, IsSubtypeNode)] */;
this.state_0_ = state_0;
} else {
s2_ = null;
}
}
}
if (s2_ != null) {
return ValidExceptionNode.isPythonException((VirtualFrame) frameValue, arg0Value, inliningTarget__, INLINED_IS_PYTHON_EXCEPTION_IS_TYPE_NODE_, s2_.isSubtype_);
}
break;
}
}
if ((emulateJython()) && (isHostObject(arg0Value))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[ValidExceptionNode.isJavaException(VirtualFrame, Object)] */;
this.state_0_ = state_0;
return isJavaException((VirtualFrame) frameValue, arg0Value);
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[ValidExceptionNode.isAnException(VirtualFrame, Object)] */;
this.state_0_ = state_0;
return ValidExceptionNode.isAnException((VirtualFrame) frameValue, arg0Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
IsPythonExceptionTypeCachedData s0_ = this.isPythonExceptionTypeCached_cache;
IsPythonExceptionClassCachedData s1_ = this.isPythonExceptionClassCached_cache;
if ((s0_ == null || s0_.next_ == null) && (s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static ValidExceptionNode create() {
return new ValidExceptionNodeGen();
}
@NeverDefault
public static ValidExceptionNode getUncached() {
return ValidExceptionNodeGen.UNCACHED;
}
private static PythonBuiltinClassType decodePythonBuiltinClassType(int state) {
if (state >= 0) {
return PYTHON_BUILTIN_CLASS_TYPE_VALUES[state];
} else {
return null;
}
}
private static int encodePythonBuiltinClassType(PythonBuiltinClassType e) {
if (e != null) {
return e.ordinal();
} else {
return -1;
}
}
@GeneratedBy(ValidExceptionNode.class)
@DenyReplace
private static final class IsPythonExceptionTypeCachedData implements SpecializationDataNode {
@CompilationFinal final IsPythonExceptionTypeCachedData next_;
/**
* State Info:
* 0-8: EncodedEnum[cache=ValidExceptionNode.isPythonExceptionTypeCached(..., PythonBuiltinClassType cachedType, ...)]
*
*/
@CompilationFinal private int isPythonExceptionTypeCached_state_0_;
/**
* Source Info:
* Specialization: {@link ValidExceptionNode#isPythonExceptionTypeCached}
* Parameter: boolean isExceptionType
*/
@CompilationFinal boolean isExceptionType_;
IsPythonExceptionTypeCachedData(IsPythonExceptionTypeCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(ValidExceptionNode.class)
@DenyReplace
private static final class IsPythonExceptionClassCachedData implements SpecializationDataNode {
@CompilationFinal final IsPythonExceptionClassCachedData next_;
/**
* State Info:
* 0-8: EncodedEnum[cache=ValidExceptionNode.isPythonExceptionClassCached(..., PythonBuiltinClassType cachedType, ...)]
*
*/
@CompilationFinal private int isPythonExceptionClassCached_state_0_;
/**
* Source Info:
* Specialization: {@link ValidExceptionNode#isPythonExceptionClassCached}
* Parameter: boolean isExceptionType
*/
@CompilationFinal boolean isExceptionType_;
IsPythonExceptionClassCachedData(IsPythonExceptionClassCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(ValidExceptionNode.class)
@DenyReplace
private static final class IsPythonExceptionData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: GuardActive[guardIndex=0] {@link ValidExceptionNode#isPythonException}
* 1: SpecializationCachesInitialized {@link ValidExceptionNode#isPythonException}
* 2-6: InlinedCache
* Specialization: {@link ValidExceptionNode#isPythonException}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int isPythonException_state_0_;
/**
* Source Info:
* Specialization: {@link ValidExceptionNode#isPythonException}
* Parameter: {@link IsTypeNode} isTypeNode
* Inline method: {@link IsTypeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node isPythonException_isTypeNode__field1_;
/**
* Source Info:
* Specialization: {@link ValidExceptionNode#isPythonException}
* Parameter: {@link IsSubtypeNode} isSubtype
*/
@Child IsSubtypeNode isSubtype_;
IsPythonExceptionData() {
}
IsPythonExceptionData(IsPythonExceptionData delegate) {
this.isPythonException_state_0_ = delegate.isPythonException_state_0_;
this.isPythonException_isTypeNode__field1_ = delegate.isPythonException_isTypeNode__field1_;
this.isSubtype_ = delegate.isSubtype_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ValidExceptionNode.class)
@DenyReplace
private static final class Uncached extends ValidExceptionNode {
@Override
public boolean execute(Frame frameValue, Object arg0Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (((IsTypeNodeGen.getUncached()).execute((this), arg0Value))) {
return ValidExceptionNode.isPythonException((VirtualFrame) frameValue, arg0Value, (this), (IsTypeNodeGen.getUncached()), (IsSubtypeNode.getUncached()));
}
if ((emulateJython()) && (isHostObject(arg0Value))) {
return isJavaException((VirtualFrame) frameValue, arg0Value);
}
return ValidExceptionNode.isAnException((VirtualFrame) frameValue, arg0Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy