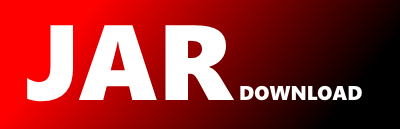
com.oracle.graal.python.nodes.expression.LookupAndCallInplaceNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.expression;
import com.oracle.graal.python.nodes.attributes.LookupInMROBaseNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link LookupAndCallInplaceNode#doBinary}
* Activation probability: 1.00000
* With/without class size: 32/11 bytes
*
*/
@GeneratedBy(LookupAndCallInplaceNode.class)
@SuppressWarnings("javadoc")
public final class LookupAndCallInplaceNodeGen extends LookupAndCallInplaceNode {
private static final StateField STATE_0_LookupAndCallInplaceNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link LookupAndCallInplaceNode#doBinary}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_CLASS_NODE_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_0_LookupAndCallInplaceNode_UPDATER.subUpdater(1, 17), ReferenceField.create(MethodHandles.lookup(), "getClassNode__field1_", Node.class)));
/**
* State Info:
* 0: SpecializationActive {@link LookupAndCallInplaceNode#doBinary}
* 1-17: InlinedCache
* Specialization: {@link LookupAndCallInplaceNode#doBinary}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link LookupAndCallInplaceNode#doBinary}
* Parameter: {@link GetClassNode} getClassNode
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link LookupAndCallInplaceNode#doBinary}
* Parameter: {@link LookupInMROBaseNode} lookupInplace
*/
@Child private LookupInMROBaseNode lookupInplace_;
private LookupAndCallInplaceNodeGen(InplaceArithmetic arithmetic) {
super(arithmetic);
}
@Override
public Object executeTernary(VirtualFrame frameValue, Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[LookupAndCallInplaceNode.doBinary(VirtualFrame, Object, Object, Object, Node, GetClassNode, LookupInMROBaseNode)] */) {
{
LookupInMROBaseNode lookupInplace__ = this.lookupInplace_;
if (lookupInplace__ != null) {
Node inliningTarget__ = (this);
return doBinary(frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__, INLINED_GET_CLASS_NODE_, lookupInplace__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(VirtualFrame frameValue, Object arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
LookupInMROBaseNode lookupInplace__ = this.insert((createInplaceLookup()));
Objects.requireNonNull(lookupInplace__, "Specialization 'doBinary(VirtualFrame, Object, Object, Object, Node, GetClassNode, LookupInMROBaseNode)' cache 'lookupInplace' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.lookupInplace_ = lookupInplace__;
state_0 = state_0 | 0b1 /* add SpecializationActive[LookupAndCallInplaceNode.doBinary(VirtualFrame, Object, Object, Object, Node, GetClassNode, LookupInMROBaseNode)] */;
this.state_0_ = state_0;
return doBinary(frameValue, arg0Value, arg1Value, arg2Value, inliningTarget__, INLINED_GET_CLASS_NODE_, lookupInplace__);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static LookupAndCallInplaceNode create(InplaceArithmetic arithmetic) {
return new LookupAndCallInplaceNodeGen(arithmetic);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy