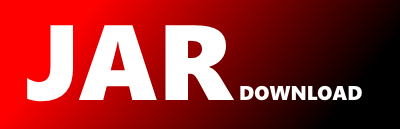
com.oracle.graal.python.nodes.frame.ReadNameNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.frame;
import com.oracle.graal.python.nodes.frame.ReadGlobalOrBuiltinNode.Lazy;
import com.oracle.graal.python.nodes.frame.ReadGlobalOrBuiltinNodeGen.LazyNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link ReadNameNode#readFromLocals}
* Activation probability: 0.65000
* With/without class size: 17/4 bytes
* Specialization {@link ReadNameNode#readFromLocalsDict}
* Activation probability: 0.35000
* With/without class size: 15/13 bytes
*
*/
@GeneratedBy(ReadNameNode.class)
@SuppressWarnings("javadoc")
public final class ReadNameNodeGen extends ReadNameNode {
private static final StateField STATE_0_ReadNameNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link Lazy} readGlobalOrBuiltinNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_READ_FROM_LOCALS_DICT_READ_GLOBAL_OR_BUILTIN_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STATE_0_ReadNameNode_UPDATER.subUpdater(2, 1), ReferenceField.create(MethodHandles.lookup(), "readFromLocalsDict_readGlobalOrBuiltinNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link ReadFromLocalsNode} readFromLocals
* Inline method: {@link ReadFromLocalsNodeGen#inline}
*/
private static final ReadFromLocalsNode INLINED_READ_FROM_LOCALS_DICT_READ_FROM_LOCALS_ = ReadFromLocalsNodeGen.inline(InlineTarget.create(ReadFromLocalsNode.class, STATE_0_ReadNameNode_UPDATER.subUpdater(3, 3), ReferenceField.create(MethodHandles.lookup(), "readFromLocalsDict_readFromLocals__field1_", Node.class), ReferenceField.create(MethodHandles.lookup(), "readFromLocalsDict_readFromLocals__field2_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link ReadNameNode#readFromLocals}
* 1: SpecializationActive {@link ReadNameNode#readFromLocalsDict}
* 2: InlinedCache
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link Lazy} readGlobalOrBuiltinNode
* Inline method: {@link LazyNodeGen#inline}
* 3-5: InlinedCache
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link ReadFromLocalsNode} readFromLocals
* Inline method: {@link ReadFromLocalsNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link ReadNameNode#readFromLocals}
* Parameter: {@link ReadGlobalOrBuiltinNode} readGlobalNode
*/
@Child private ReadGlobalOrBuiltinNode readFromLocals_readGlobalNode_;
/**
* Source Info:
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link Lazy} readGlobalOrBuiltinNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromLocalsDict_readGlobalOrBuiltinNode__field1_;
/**
* Source Info:
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link ReadFromLocalsNode} readFromLocals
* Inline method: {@link ReadFromLocalsNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromLocalsDict_readFromLocals__field1_;
/**
* Source Info:
* Specialization: {@link ReadNameNode#readFromLocalsDict}
* Parameter: {@link ReadFromLocalsNode} readFromLocals
* Inline method: {@link ReadFromLocalsNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromLocalsDict_readFromLocals__field2_;
private ReadNameNodeGen() {
}
@Override
public Object executeImpl(VirtualFrame frameValue, TruffleString arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[ReadNameNode.readFromLocals(VirtualFrame, TruffleString, ReadGlobalOrBuiltinNode)] || SpecializationActive[ReadNameNode.readFromLocalsDict(VirtualFrame, TruffleString, Node, Lazy, ReadFromLocalsNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReadNameNode.readFromLocals(VirtualFrame, TruffleString, ReadGlobalOrBuiltinNode)] */) {
{
ReadGlobalOrBuiltinNode readGlobalNode__ = this.readFromLocals_readGlobalNode_;
if (readGlobalNode__ != null) {
if ((!(hasLocals(frameValue)))) {
return ReadNameNode.readFromLocals(frameValue, arg0Value, readGlobalNode__);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReadNameNode.readFromLocalsDict(VirtualFrame, TruffleString, Node, Lazy, ReadFromLocalsNode)] */) {
if ((hasLocals(frameValue))) {
Node inliningTarget__ = (this);
return ReadNameNode.readFromLocalsDict(frameValue, arg0Value, inliningTarget__, INLINED_READ_FROM_LOCALS_DICT_READ_GLOBAL_OR_BUILTIN_NODE_, INLINED_READ_FROM_LOCALS_DICT_READ_FROM_LOCALS_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value);
}
private Object executeAndSpecialize(VirtualFrame frameValue, TruffleString arg0Value) {
int state_0 = this.state_0_;
if ((!(hasLocals(frameValue)))) {
ReadGlobalOrBuiltinNode readGlobalNode__ = this.insert((ReadGlobalOrBuiltinNode.create()));
Objects.requireNonNull(readGlobalNode__, "Specialization 'readFromLocals(VirtualFrame, TruffleString, ReadGlobalOrBuiltinNode)' cache 'readGlobalNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.readFromLocals_readGlobalNode_ = readGlobalNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReadNameNode.readFromLocals(VirtualFrame, TruffleString, ReadGlobalOrBuiltinNode)] */;
this.state_0_ = state_0;
return ReadNameNode.readFromLocals(frameValue, arg0Value, readGlobalNode__);
}
{
Node inliningTarget__ = null;
if ((hasLocals(frameValue))) {
inliningTarget__ = (this);
state_0 = state_0 | 0b10 /* add SpecializationActive[ReadNameNode.readFromLocalsDict(VirtualFrame, TruffleString, Node, Lazy, ReadFromLocalsNode)] */;
this.state_0_ = state_0;
return ReadNameNode.readFromLocalsDict(frameValue, arg0Value, inliningTarget__, INLINED_READ_FROM_LOCALS_DICT_READ_GLOBAL_OR_BUILTIN_NODE_, INLINED_READ_FROM_LOCALS_DICT_READ_FROM_LOCALS_);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null}, arg0Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11) & ((state_0 & 0b11) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static ReadNameNode create() {
return new ReadNameNodeGen();
}
@NeverDefault
public static ReadNameNode getUncached() {
return ReadNameNodeGen.UNCACHED;
}
@GeneratedBy(ReadNameNode.class)
@DenyReplace
private static final class Uncached extends ReadNameNode {
@Override
public Object executeImpl(VirtualFrame frameValue, TruffleString arg0Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if ((!(hasLocals(frameValue)))) {
return ReadNameNode.readFromLocals(frameValue, arg0Value, (ReadGlobalOrBuiltinNode.getUncached()));
}
if ((hasLocals(frameValue))) {
return ReadNameNode.readFromLocalsDict(frameValue, arg0Value, (this), (LazyNodeGen.getUncached()), (ReadFromLocalsNode.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null}, arg0Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy