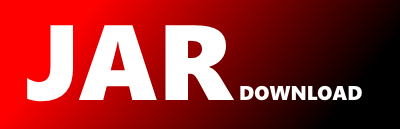
com.oracle.graal.python.nodes.object.GetClassNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.object;
import com.oracle.graal.python.builtins.PythonBuiltinClassType;
import com.oracle.graal.python.builtins.objects.PNone;
import com.oracle.graal.python.builtins.objects.PNotImplemented;
import com.oracle.graal.python.builtins.objects.PythonAbstractObject;
import com.oracle.graal.python.builtins.objects.cell.PCell;
import com.oracle.graal.python.builtins.objects.cext.PythonAbstractNativeObject;
import com.oracle.graal.python.builtins.objects.cext.PythonNativeVoidPtr;
import com.oracle.graal.python.builtins.objects.cext.capi.CExtNodes.GetNativeClassNode;
import com.oracle.graal.python.builtins.objects.cext.capi.CExtNodesFactory.GetNativeClassNodeGen;
import com.oracle.graal.python.builtins.objects.ellipsis.PEllipsis;
import com.oracle.graal.python.builtins.objects.function.PBuiltinFunction;
import com.oracle.graal.python.builtins.objects.function.PFunction;
import com.oracle.graal.python.builtins.objects.object.PythonObject;
import com.oracle.graal.python.builtins.objects.type.PythonBuiltinClass;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.exception.AbstractTruffleException;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.object.Shape;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.utilities.TruffleWeakReference;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link GetClassNode#getBoolean}
* Activation probability: 0.10588
* With/without class size: 5/0 bytes
* Specialization {@link GetClassNode#getInt}
* Activation probability: 0.10000
* With/without class size: 5/0 bytes
* Specialization {@link GetClassNode#getLong}
* Activation probability: 0.09412
* With/without class size: 5/0 bytes
* Specialization {@link GetClassNode#getDouble}
* Activation probability: 0.08824
* With/without class size: 5/0 bytes
* Specialization {@link GetClassNode#getString}
* Activation probability: 0.08235
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getNone}
* Activation probability: 0.07647
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getBuiltinClass}
* Activation probability: 0.07059
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getFunction}
* Activation probability: 0.06471
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getBuiltinFunction}
* Activation probability: 0.05882
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getPythonObjectOrNative}
* Activation probability: 0.05294
* With/without class size: 5/4 bytes
* Specialization {@link GetClassNode#getPBCT}
* Activation probability: 0.04706
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getNotImplemented}
* Activation probability: 0.04118
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getEllipsis}
* Activation probability: 0.03529
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getCell}
* Activation probability: 0.02941
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getNativeVoidPtr}
* Activation probability: 0.02353
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getTruffleException}
* Activation probability: 0.01765
* With/without class size: 4/0 bytes
* Specialization {@link GetClassNode#getForeign}
* Activation probability: 0.01176
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(GetClassNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class GetClassNodeGen extends GetClassNode {
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link GetClassNode#getBoolean}
* 1: SpecializationActive {@link GetClassNode#getInt}
* 2: SpecializationActive {@link GetClassNode#getLong}
* 3: SpecializationActive {@link GetClassNode#getDouble}
* 4: SpecializationActive {@link GetClassNode#getString}
* 5: SpecializationActive {@link GetClassNode#getNone}
* 6: SpecializationActive {@link GetClassNode#getBuiltinClass}
* 7: SpecializationActive {@link GetClassNode#getFunction}
* 8: SpecializationActive {@link GetClassNode#getBuiltinFunction}
* 9: SpecializationActive {@link GetClassNode#getPythonObjectOrNative}
* 10: SpecializationActive {@link GetClassNode#getPBCT}
* 11: SpecializationActive {@link GetClassNode#getNotImplemented}
* 12: SpecializationActive {@link GetClassNode#getEllipsis}
* 13: SpecializationActive {@link GetClassNode#getCell}
* 14: SpecializationActive {@link GetClassNode#getNativeVoidPtr}
* 15: SpecializationActive {@link GetClassNode#getTruffleException}
* 16: SpecializationActive {@link GetClassNode#getForeign}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link GetClassNode#getPythonObjectOrNative}
* Parameter: {@link GetPythonObjectClassNode} getClassNode
*/
@Child private GetPythonObjectClassNode getPythonObjectOrNative_getClassNode_;
private GetClassNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[GetClassNode.getBoolean(Boolean)] */) && arg1Value instanceof Boolean) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[GetClassNode.getInt(Integer)] */) && arg1Value instanceof Integer) {
return false;
}
if (!((state_0 & 0b100) != 0 /* is SpecializationActive[GetClassNode.getLong(Long)] */) && arg1Value instanceof Long) {
return false;
}
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[GetClassNode.getDouble(Double)] */) && arg1Value instanceof Double) {
return false;
}
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[GetClassNode.getString(TruffleString)] */) && arg1Value instanceof TruffleString) {
return false;
}
if (!((state_0 & 0b100000) != 0 /* is SpecializationActive[GetClassNode.getNone(PNone)] */) && arg1Value instanceof PNone) {
return false;
}
if (!((state_0 & 0b1000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] */) && arg1Value instanceof PythonBuiltinClass) {
return false;
}
if (!((state_0 & 0b10000000) != 0 /* is SpecializationActive[GetClassNode.getFunction(PFunction)] */) && arg1Value instanceof PFunction) {
return false;
}
if (!((state_0 & 0b100000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] */) && arg1Value instanceof PBuiltinFunction) {
return false;
}
if (arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
return false;
}
}
if (!((state_0 & 0b10000000000) != 0 /* is SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] */) && arg1Value instanceof PythonBuiltinClassType) {
return false;
}
if (!((state_0 & 0b100000000000) != 0 /* is SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] */) && arg1Value instanceof PNotImplemented) {
return false;
}
if (!((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] */) && arg1Value instanceof PEllipsis) {
return false;
}
if (!((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[GetClassNode.getCell(PCell)] */) && arg1Value instanceof PCell) {
return false;
}
if (!((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] */) && arg1Value instanceof PythonNativeVoidPtr) {
return false;
}
if (!((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] */) && arg1Value instanceof AbstractTruffleException) {
return false;
}
return true;
}
@Override
public Object execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[GetClassNode.getBoolean(Boolean)] || SpecializationActive[GetClassNode.getInt(Integer)] || SpecializationActive[GetClassNode.getLong(Long)] || SpecializationActive[GetClassNode.getDouble(Double)] || SpecializationActive[GetClassNode.getString(TruffleString)] || SpecializationActive[GetClassNode.getNone(PNone)] || SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] || SpecializationActive[GetClassNode.getFunction(PFunction)] || SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] || SpecializationActive[GetClassNode.getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)] || SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] || SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] || SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] || SpecializationActive[GetClassNode.getCell(PCell)] || SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] || SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] || SpecializationActive[GetClassNode.getForeign(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetClassNode.getBoolean(Boolean)] */ && arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return GetClassNode.getBoolean(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetClassNode.getInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return GetClassNode.getInt(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetClassNode.getLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return GetClassNode.getLong(arg1Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GetClassNode.getDouble(Double)] */ && arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return GetClassNode.getDouble(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[GetClassNode.getString(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return GetClassNode.getString(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[GetClassNode.getNone(PNone)] */ && arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
return GetClassNode.getNone(arg1Value_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] */ && arg1Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg1Value_ = (PythonBuiltinClass) arg1Value;
return GetClassNode.getBuiltinClass(arg1Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[GetClassNode.getFunction(PFunction)] */ && arg1Value instanceof PFunction) {
PFunction arg1Value_ = (PFunction) arg1Value;
return GetClassNode.getFunction(arg1Value_);
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] */ && arg1Value instanceof PBuiltinFunction) {
PBuiltinFunction arg1Value_ = (PBuiltinFunction) arg1Value;
return GetClassNode.getBuiltinFunction(arg1Value_);
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[GetClassNode.getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)] */ && arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
{
GetPythonObjectClassNode getClassNode__ = this.getPythonObjectOrNative_getClassNode_;
if (getClassNode__ != null) {
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
return GetClassNode.getPythonObjectOrNative(arg1Value_, getClassNode__);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] */ && arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return GetClassNode.getPBCT(arg1Value_);
}
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] */ && arg1Value instanceof PNotImplemented) {
PNotImplemented arg1Value_ = (PNotImplemented) arg1Value;
return GetClassNode.getNotImplemented(arg1Value_);
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] */ && arg1Value instanceof PEllipsis) {
PEllipsis arg1Value_ = (PEllipsis) arg1Value;
return GetClassNode.getEllipsis(arg1Value_);
}
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[GetClassNode.getCell(PCell)] */ && arg1Value instanceof PCell) {
PCell arg1Value_ = (PCell) arg1Value;
return GetClassNode.getCell(arg1Value_);
}
if ((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] */ && arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
return GetClassNode.getNativeVoidPtr(arg1Value_);
}
if ((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] */ && arg1Value instanceof AbstractTruffleException) {
AbstractTruffleException arg1Value_ = (AbstractTruffleException) arg1Value;
return GetClassNode.getTruffleException(arg1Value_);
}
if ((state_0 & 0x10000) != 0 /* is SpecializationActive[GetClassNode.getForeign(Object)] */) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return GetClassNode.getForeign(arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[GetClassNode.getBoolean(Boolean)] */;
this.state_0_ = state_0;
return GetClassNode.getBoolean(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[GetClassNode.getInt(Integer)] */;
this.state_0_ = state_0;
return GetClassNode.getInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[GetClassNode.getLong(Long)] */;
this.state_0_ = state_0;
return GetClassNode.getLong(arg1Value_);
}
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[GetClassNode.getDouble(Double)] */;
this.state_0_ = state_0;
return GetClassNode.getDouble(arg1Value_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[GetClassNode.getString(TruffleString)] */;
this.state_0_ = state_0;
return GetClassNode.getString(arg1Value_);
}
if (arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[GetClassNode.getNone(PNone)] */;
this.state_0_ = state_0;
return GetClassNode.getNone(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg1Value_ = (PythonBuiltinClass) arg1Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] */;
this.state_0_ = state_0;
return GetClassNode.getBuiltinClass(arg1Value_);
}
if (arg1Value instanceof PFunction) {
PFunction arg1Value_ = (PFunction) arg1Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[GetClassNode.getFunction(PFunction)] */;
this.state_0_ = state_0;
return GetClassNode.getFunction(arg1Value_);
}
if (arg1Value instanceof PBuiltinFunction) {
PBuiltinFunction arg1Value_ = (PBuiltinFunction) arg1Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] */;
this.state_0_ = state_0;
return GetClassNode.getBuiltinFunction(arg1Value_);
}
if (arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
GetPythonObjectClassNode getClassNode__ = this.insert((GetPythonObjectClassNodeGen.create()));
Objects.requireNonNull(getClassNode__, "Specialization 'getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)' cache 'getClassNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.getPythonObjectOrNative_getClassNode_ = getClassNode__;
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[GetClassNode.getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)] */;
this.state_0_ = state_0;
return GetClassNode.getPythonObjectOrNative(arg1Value_, getClassNode__);
}
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] */;
this.state_0_ = state_0;
return GetClassNode.getPBCT(arg1Value_);
}
if (arg1Value instanceof PNotImplemented) {
PNotImplemented arg1Value_ = (PNotImplemented) arg1Value;
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] */;
this.state_0_ = state_0;
return GetClassNode.getNotImplemented(arg1Value_);
}
if (arg1Value instanceof PEllipsis) {
PEllipsis arg1Value_ = (PEllipsis) arg1Value;
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] */;
this.state_0_ = state_0;
return GetClassNode.getEllipsis(arg1Value_);
}
if (arg1Value instanceof PCell) {
PCell arg1Value_ = (PCell) arg1Value;
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[GetClassNode.getCell(PCell)] */;
this.state_0_ = state_0;
return GetClassNode.getCell(arg1Value_);
}
if (arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
state_0 = state_0 | 0b100000000000000 /* add SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] */;
this.state_0_ = state_0;
return GetClassNode.getNativeVoidPtr(arg1Value_);
}
if (arg1Value instanceof AbstractTruffleException) {
AbstractTruffleException arg1Value_ = (AbstractTruffleException) arg1Value;
state_0 = state_0 | 0b1000000000000000 /* add SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] */;
this.state_0_ = state_0;
return GetClassNode.getTruffleException(arg1Value_);
}
state_0 = state_0 | 0x10000 /* add SpecializationActive[GetClassNode.getForeign(Object)] */;
this.state_0_ = state_0;
return GetClassNode.getForeign(arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static GetClassNode create() {
return new GetClassNodeGen();
}
@NeverDefault
public static GetClassNode getUncached() {
return GetClassNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#getPythonObjectOrNative_getClassNode_}
*
*/
@NeverDefault
public static GetClassNode inline(@RequiredField(bits = 17, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new GetClassNodeGen.Inlined(target);
}
@GeneratedBy(GetClassNode.class)
@DenyReplace
private static final class Inlined extends GetClassNode {
/**
* State Info:
* 0: SpecializationActive {@link GetClassNode#getBoolean}
* 1: SpecializationActive {@link GetClassNode#getInt}
* 2: SpecializationActive {@link GetClassNode#getLong}
* 3: SpecializationActive {@link GetClassNode#getDouble}
* 4: SpecializationActive {@link GetClassNode#getString}
* 5: SpecializationActive {@link GetClassNode#getNone}
* 6: SpecializationActive {@link GetClassNode#getBuiltinClass}
* 7: SpecializationActive {@link GetClassNode#getFunction}
* 8: SpecializationActive {@link GetClassNode#getBuiltinFunction}
* 9: SpecializationActive {@link GetClassNode#getPythonObjectOrNative}
* 10: SpecializationActive {@link GetClassNode#getPBCT}
* 11: SpecializationActive {@link GetClassNode#getNotImplemented}
* 12: SpecializationActive {@link GetClassNode#getEllipsis}
* 13: SpecializationActive {@link GetClassNode#getCell}
* 14: SpecializationActive {@link GetClassNode#getNativeVoidPtr}
* 15: SpecializationActive {@link GetClassNode#getTruffleException}
* 16: SpecializationActive {@link GetClassNode#getForeign}
*
*/
private final StateField state_0_;
private final ReferenceField getPythonObjectOrNative_getClassNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(GetClassNode.class);
this.state_0_ = target.getState(0, 17);
this.getPythonObjectOrNative_getClassNode_ = target.getReference(1, GetPythonObjectClassNode.class);
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[GetClassNode.getBoolean(Boolean)] */) && arg1Value instanceof Boolean) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[GetClassNode.getInt(Integer)] */) && arg1Value instanceof Integer) {
return false;
}
if (!((state_0 & 0b100) != 0 /* is SpecializationActive[GetClassNode.getLong(Long)] */) && arg1Value instanceof Long) {
return false;
}
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[GetClassNode.getDouble(Double)] */) && arg1Value instanceof Double) {
return false;
}
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[GetClassNode.getString(TruffleString)] */) && arg1Value instanceof TruffleString) {
return false;
}
if (!((state_0 & 0b100000) != 0 /* is SpecializationActive[GetClassNode.getNone(PNone)] */) && arg1Value instanceof PNone) {
return false;
}
if (!((state_0 & 0b1000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] */) && arg1Value instanceof PythonBuiltinClass) {
return false;
}
if (!((state_0 & 0b10000000) != 0 /* is SpecializationActive[GetClassNode.getFunction(PFunction)] */) && arg1Value instanceof PFunction) {
return false;
}
if (!((state_0 & 0b100000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] */) && arg1Value instanceof PBuiltinFunction) {
return false;
}
if (arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
return false;
}
}
if (!((state_0 & 0b10000000000) != 0 /* is SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] */) && arg1Value instanceof PythonBuiltinClassType) {
return false;
}
if (!((state_0 & 0b100000000000) != 0 /* is SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] */) && arg1Value instanceof PNotImplemented) {
return false;
}
if (!((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] */) && arg1Value instanceof PEllipsis) {
return false;
}
if (!((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[GetClassNode.getCell(PCell)] */) && arg1Value instanceof PCell) {
return false;
}
if (!((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] */) && arg1Value instanceof PythonNativeVoidPtr) {
return false;
}
if (!((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] */) && arg1Value instanceof AbstractTruffleException) {
return false;
}
return true;
}
@Override
public Object execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[GetClassNode.getBoolean(Boolean)] || SpecializationActive[GetClassNode.getInt(Integer)] || SpecializationActive[GetClassNode.getLong(Long)] || SpecializationActive[GetClassNode.getDouble(Double)] || SpecializationActive[GetClassNode.getString(TruffleString)] || SpecializationActive[GetClassNode.getNone(PNone)] || SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] || SpecializationActive[GetClassNode.getFunction(PFunction)] || SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] || SpecializationActive[GetClassNode.getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)] || SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] || SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] || SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] || SpecializationActive[GetClassNode.getCell(PCell)] || SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] || SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] || SpecializationActive[GetClassNode.getForeign(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetClassNode.getBoolean(Boolean)] */ && arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return GetClassNode.getBoolean(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetClassNode.getInt(Integer)] */ && arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return GetClassNode.getInt(arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetClassNode.getLong(Long)] */ && arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return GetClassNode.getLong(arg1Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GetClassNode.getDouble(Double)] */ && arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return GetClassNode.getDouble(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[GetClassNode.getString(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return GetClassNode.getString(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[GetClassNode.getNone(PNone)] */ && arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
return GetClassNode.getNone(arg1Value_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] */ && arg1Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg1Value_ = (PythonBuiltinClass) arg1Value;
return GetClassNode.getBuiltinClass(arg1Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[GetClassNode.getFunction(PFunction)] */ && arg1Value instanceof PFunction) {
PFunction arg1Value_ = (PFunction) arg1Value;
return GetClassNode.getFunction(arg1Value_);
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] */ && arg1Value instanceof PBuiltinFunction) {
PBuiltinFunction arg1Value_ = (PBuiltinFunction) arg1Value;
return GetClassNode.getBuiltinFunction(arg1Value_);
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[GetClassNode.getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)] */ && arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
{
GetPythonObjectClassNode getClassNode__ = this.getPythonObjectOrNative_getClassNode_.get(arg0Value);
if (getClassNode__ != null) {
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
return GetClassNode.getPythonObjectOrNative(arg1Value_, getClassNode__);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] */ && arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return GetClassNode.getPBCT(arg1Value_);
}
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] */ && arg1Value instanceof PNotImplemented) {
PNotImplemented arg1Value_ = (PNotImplemented) arg1Value;
return GetClassNode.getNotImplemented(arg1Value_);
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] */ && arg1Value instanceof PEllipsis) {
PEllipsis arg1Value_ = (PEllipsis) arg1Value;
return GetClassNode.getEllipsis(arg1Value_);
}
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[GetClassNode.getCell(PCell)] */ && arg1Value instanceof PCell) {
PCell arg1Value_ = (PCell) arg1Value;
return GetClassNode.getCell(arg1Value_);
}
if ((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] */ && arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
return GetClassNode.getNativeVoidPtr(arg1Value_);
}
if ((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] */ && arg1Value instanceof AbstractTruffleException) {
AbstractTruffleException arg1Value_ = (AbstractTruffleException) arg1Value;
return GetClassNode.getTruffleException(arg1Value_);
}
if ((state_0 & 0x10000) != 0 /* is SpecializationActive[GetClassNode.getForeign(Object)] */) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return GetClassNode.getForeign(arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[GetClassNode.getBoolean(Boolean)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getBoolean(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[GetClassNode.getInt(Integer)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[GetClassNode.getLong(Long)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getLong(arg1Value_);
}
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[GetClassNode.getDouble(Double)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getDouble(arg1Value_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[GetClassNode.getString(TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getString(arg1Value_);
}
if (arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[GetClassNode.getNone(PNone)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getNone(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg1Value_ = (PythonBuiltinClass) arg1Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[GetClassNode.getBuiltinClass(PythonBuiltinClass)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getBuiltinClass(arg1Value_);
}
if (arg1Value instanceof PFunction) {
PFunction arg1Value_ = (PFunction) arg1Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[GetClassNode.getFunction(PFunction)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getFunction(arg1Value_);
}
if (arg1Value instanceof PBuiltinFunction) {
PBuiltinFunction arg1Value_ = (PBuiltinFunction) arg1Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[GetClassNode.getBuiltinFunction(PBuiltinFunction)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getBuiltinFunction(arg1Value_);
}
if (arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
GetPythonObjectClassNode getClassNode__ = arg0Value.insert((GetPythonObjectClassNodeGen.create()));
Objects.requireNonNull(getClassNode__, "Specialization 'getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)' cache 'getClassNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.getPythonObjectOrNative_getClassNode_.set(arg0Value, getClassNode__);
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[GetClassNode.getPythonObjectOrNative(PythonAbstractObject, GetPythonObjectClassNode)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getPythonObjectOrNative(arg1Value_, getClassNode__);
}
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[GetClassNode.getPBCT(PythonBuiltinClassType)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getPBCT(arg1Value_);
}
if (arg1Value instanceof PNotImplemented) {
PNotImplemented arg1Value_ = (PNotImplemented) arg1Value;
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[GetClassNode.getNotImplemented(PNotImplemented)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getNotImplemented(arg1Value_);
}
if (arg1Value instanceof PEllipsis) {
PEllipsis arg1Value_ = (PEllipsis) arg1Value;
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[GetClassNode.getEllipsis(PEllipsis)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getEllipsis(arg1Value_);
}
if (arg1Value instanceof PCell) {
PCell arg1Value_ = (PCell) arg1Value;
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[GetClassNode.getCell(PCell)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getCell(arg1Value_);
}
if (arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
state_0 = state_0 | 0b100000000000000 /* add SpecializationActive[GetClassNode.getNativeVoidPtr(PythonNativeVoidPtr)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getNativeVoidPtr(arg1Value_);
}
if (arg1Value instanceof AbstractTruffleException) {
AbstractTruffleException arg1Value_ = (AbstractTruffleException) arg1Value;
state_0 = state_0 | 0b1000000000000000 /* add SpecializationActive[GetClassNode.getTruffleException(AbstractTruffleException)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getTruffleException(arg1Value_);
}
state_0 = state_0 | 0x10000 /* add SpecializationActive[GetClassNode.getForeign(Object)] */;
this.state_0_.set(arg0Value, state_0);
return GetClassNode.getForeign(arg1Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(GetClassNode.class)
@DenyReplace
private static final class Uncached extends GetClassNode {
@TruffleBoundary
@Override
public Object execute(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof Boolean) {
Boolean arg1Value_ = (Boolean) arg1Value;
return GetClassNode.getBoolean(arg1Value_);
}
if (arg1Value instanceof Integer) {
Integer arg1Value_ = (Integer) arg1Value;
return GetClassNode.getInt(arg1Value_);
}
if (arg1Value instanceof Long) {
Long arg1Value_ = (Long) arg1Value;
return GetClassNode.getLong(arg1Value_);
}
if (arg1Value instanceof Double) {
Double arg1Value_ = (Double) arg1Value;
return GetClassNode.getDouble(arg1Value_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return GetClassNode.getString(arg1Value_);
}
if (arg1Value instanceof PNone) {
PNone arg1Value_ = (PNone) arg1Value;
return GetClassNode.getNone(arg1Value_);
}
if (arg1Value instanceof PythonBuiltinClass) {
PythonBuiltinClass arg1Value_ = (PythonBuiltinClass) arg1Value;
return GetClassNode.getBuiltinClass(arg1Value_);
}
if (arg1Value instanceof PFunction) {
PFunction arg1Value_ = (PFunction) arg1Value;
return GetClassNode.getFunction(arg1Value_);
}
if (arg1Value instanceof PBuiltinFunction) {
PBuiltinFunction arg1Value_ = (PBuiltinFunction) arg1Value;
return GetClassNode.getBuiltinFunction(arg1Value_);
}
if (arg1Value instanceof PythonAbstractObject) {
PythonAbstractObject arg1Value_ = (PythonAbstractObject) arg1Value;
if ((PGuards.isPythonObject(arg1Value_) || PGuards.isNativeObject(arg1Value_))) {
return GetClassNode.getPythonObjectOrNative(arg1Value_, (GetPythonObjectClassNodeGen.getUncached()));
}
}
if (arg1Value instanceof PythonBuiltinClassType) {
PythonBuiltinClassType arg1Value_ = (PythonBuiltinClassType) arg1Value;
return GetClassNode.getPBCT(arg1Value_);
}
if (arg1Value instanceof PNotImplemented) {
PNotImplemented arg1Value_ = (PNotImplemented) arg1Value;
return GetClassNode.getNotImplemented(arg1Value_);
}
if (arg1Value instanceof PEllipsis) {
PEllipsis arg1Value_ = (PEllipsis) arg1Value;
return GetClassNode.getEllipsis(arg1Value_);
}
if (arg1Value instanceof PCell) {
PCell arg1Value_ = (PCell) arg1Value;
return GetClassNode.getCell(arg1Value_);
}
if (arg1Value instanceof PythonNativeVoidPtr) {
PythonNativeVoidPtr arg1Value_ = (PythonNativeVoidPtr) arg1Value;
return GetClassNode.getNativeVoidPtr(arg1Value_);
}
if (arg1Value instanceof AbstractTruffleException) {
AbstractTruffleException arg1Value_ = (AbstractTruffleException) arg1Value;
return GetClassNode.getTruffleException(arg1Value_);
}
return GetClassNode.getForeign(arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link GetPythonObjectClassNode#getPythonObjectConstantClass}
* Activation probability: 0.38500
* With/without class size: 11/8 bytes
* Specialization {@link GetPythonObjectClassNode#getPythonObject(PythonObject, Object)}
* Activation probability: 0.29500
* With/without class size: 7/0 bytes
* Specialization {@link GetPythonObjectClassNode#getPythonObject(PythonObject, DynamicObjectLibrary)}
* Activation probability: 0.20500
* With/without class size: 8/4 bytes
* Specialization {@link GetPythonObjectClassNode#getNativeObject}
* Activation probability: 0.11500
* With/without class size: 6/4 bytes
*
*/
@GeneratedBy(GetPythonObjectClassNode.class)
@SuppressWarnings("javadoc")
public static final class GetPythonObjectClassNodeGen extends GetPythonObjectClassNode {
static final ReferenceField GET_PYTHON_OBJECT_CONSTANT_CLASS_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getPythonObjectConstantClass_cache", GetPythonObjectConstantClassData.class);
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link GetPythonObjectClassNode#getPythonObjectConstantClass}
* 1: SpecializationActive {@link GetPythonObjectClassNode#getPythonObject(PythonObject, DynamicObjectLibrary)}
* 2: SpecializationActive {@link GetPythonObjectClassNode#getPythonObject(PythonObject, Object)}
* 3: SpecializationActive {@link GetPythonObjectClassNode#getNativeObject}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private GetPythonObjectConstantClassData getPythonObjectConstantClass_cache;
/**
* Source Info:
* Specialization: {@link GetPythonObjectClassNode#getPythonObject(PythonObject, DynamicObjectLibrary)}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child private DynamicObjectLibrary getPythonObject1_dylib_;
/**
* Source Info:
* Specialization: {@link GetPythonObjectClassNode#getNativeObject}
* Parameter: {@link GetNativeClassNode} getNativeClassNode
*/
@Child private GetNativeClassNode getNativeObject_getNativeClassNode_;
private GetPythonObjectClassNodeGen() {
}
@Override
Object executeImpl(Node arg0Value, PythonAbstractObject arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObjectConstantClass(PythonObject, Shape, Object)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, Object)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, DynamicObjectLibrary)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getNativeObject(PythonAbstractNativeObject, GetNativeClassNode)] */) {
if ((state_0 & 0b111) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObjectConstantClass(PythonObject, Shape, Object)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, Object)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, DynamicObjectLibrary)] */ && arg1Value instanceof PythonObject) {
PythonObject arg1Value_ = (PythonObject) arg1Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObjectConstantClass(PythonObject, Shape, Object)] */) {
GetPythonObjectConstantClassData s0_ = this.getPythonObjectConstantClass_cache;
if (s0_ != null) {
{
Object klass__ = (s0_.weakKlassGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((klass__ != null) && (klass__ != null) && (arg1Value_.getShape() == s0_.cachedShape_)) {
assert DSLSupport.assertIdempotence((GetPythonObjectClassNode.hasInitialClass(s0_.cachedShape_)));
return GetPythonObjectClassNode.getPythonObjectConstantClass(arg1Value_, s0_.cachedShape_, klass__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, Object)] */) {
if ((GetPythonObjectClassNode.hasInitialClass(arg1Value_.getShape()))) {
Object klass__1 = (arg1Value_.getInitialPythonClass());
return GetPythonObjectClassNode.getPythonObject(arg1Value_, klass__1);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, DynamicObjectLibrary)] */) {
{
DynamicObjectLibrary dylib__ = this.getPythonObject1_dylib_;
if (dylib__ != null) {
if ((!(GetPythonObjectClassNode.hasInitialClass(arg1Value_.getShape())))) {
return GetPythonObjectClassNode.getPythonObject(arg1Value_, dylib__);
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getNativeObject(PythonAbstractNativeObject, GetNativeClassNode)] */ && arg1Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg1Value_ = (PythonAbstractNativeObject) arg1Value;
{
GetNativeClassNode getNativeClassNode__ = this.getNativeObject_getNativeClassNode_;
if (getNativeClassNode__ != null) {
return GetPythonObjectClassNode.getNativeObject(arg1Value_, getNativeClassNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@Override
public Object execute(Node arg0Value, PythonAbstractNativeObject arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getNativeObject(PythonAbstractNativeObject, GetNativeClassNode)] */) {
{
GetNativeClassNode getNativeClassNode__ = this.getNativeObject_getNativeClassNode_;
if (getNativeClassNode__ != null) {
return GetPythonObjectClassNode.getNativeObject(arg1Value, getNativeClassNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@Override
public Object execute(Node arg0Value, PythonObject arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObjectConstantClass(PythonObject, Shape, Object)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, Object)] || SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, DynamicObjectLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObjectConstantClass(PythonObject, Shape, Object)] */) {
GetPythonObjectConstantClassData s0_ = this.getPythonObjectConstantClass_cache;
if (s0_ != null) {
{
Object klass__ = (s0_.weakKlassGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((klass__ != null) && (klass__ != null) && (arg1Value.getShape() == s0_.cachedShape_)) {
assert DSLSupport.assertIdempotence((GetPythonObjectClassNode.hasInitialClass(s0_.cachedShape_)));
return GetPythonObjectClassNode.getPythonObjectConstantClass(arg1Value, s0_.cachedShape_, klass__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, Object)] */) {
if ((GetPythonObjectClassNode.hasInitialClass(arg1Value.getShape()))) {
Object klass__1 = (arg1Value.getInitialPythonClass());
return GetPythonObjectClassNode.getPythonObject(arg1Value, klass__1);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, DynamicObjectLibrary)] */) {
{
DynamicObjectLibrary dylib__ = this.getPythonObject1_dylib_;
if (dylib__ != null) {
if ((!(GetPythonObjectClassNode.hasInitialClass(arg1Value.getShape())))) {
return GetPythonObjectClassNode.getPythonObject(arg1Value, dylib__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Node arg0Value, PythonAbstractObject arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof PythonObject) {
PythonObject arg1Value_ = (PythonObject) arg1Value;
{
Object klass__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[GetClassNode.GetPythonObjectClassNode.getPythonObject(PythonObject, DynamicObjectLibrary)] */) {
while (true) {
int count0_ = 0;
GetPythonObjectConstantClassData s0_ = GET_PYTHON_OBJECT_CONSTANT_CLASS_CACHE_UPDATER.getVolatile(this);
GetPythonObjectConstantClassData s0_original = s0_;
while (s0_ != null) {
{
klass__ = (s0_.weakKlassGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((klass__ != null) && (klass__ != null) && (arg1Value_.getShape() == s0_.cachedShape_)) {
assert DSLSupport.assertIdempotence((GetPythonObjectClassNode.hasInitialClass(s0_.cachedShape_)));
break;
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy