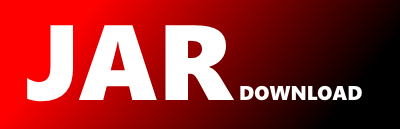
com.oracle.graal.python.nodes.object.GetDictIfExistsNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.object;
import com.oracle.graal.python.builtins.objects.cext.PythonAbstractNativeObject;
import com.oracle.graal.python.builtins.objects.cext.capi.CExtNodes.PCallCapiFunction;
import com.oracle.graal.python.builtins.objects.cext.capi.transitions.CApiTransitions.NativeToPythonNode;
import com.oracle.graal.python.builtins.objects.cext.capi.transitions.CApiTransitions.PythonToNativeNode;
import com.oracle.graal.python.builtins.objects.cext.capi.transitions.CApiTransitionsFactory.NativeToPythonNodeGen;
import com.oracle.graal.python.builtins.objects.cext.capi.transitions.CApiTransitionsFactory.PythonToNativeNodeGen;
import com.oracle.graal.python.builtins.objects.dict.PDict;
import com.oracle.graal.python.builtins.objects.object.PythonObject;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.object.Shape;
import com.oracle.truffle.api.utilities.TruffleWeakReference;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link GetDictIfExistsNode#getNoDictCachedShape}
* Activation probability: 0.27381
* With/without class size: 8/4 bytes
* Specialization {@link GetDictIfExistsNode#getNoDict}
* Activation probability: 0.23095
* With/without class size: 6/0 bytes
* Specialization {@link GetDictIfExistsNode#getConstant}
* Activation probability: 0.18810
* With/without class size: 7/8 bytes
* Specialization {@link GetDictIfExistsNode#doPythonObject}
* Activation probability: 0.14524
* With/without class size: 6/4 bytes
* Specialization {@link GetDictIfExistsNode#doNativeObject}
* Activation probability: 0.10238
* With/without class size: 6/12 bytes
* Specialization {@link GetDictIfExistsNode#doOther}
* Activation probability: 0.05952
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(GetDictIfExistsNode.class)
@SuppressWarnings("javadoc")
public final class GetDictIfExistsNodeGen extends GetDictIfExistsNode {
static final ReferenceField GET_NO_DICT_CACHED_SHAPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getNoDictCachedShape_cache", GetNoDictCachedShapeData.class);
static final ReferenceField GET_CONSTANT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getConstant_cache", GetConstantData.class);
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link GetDictIfExistsNode#getNoDictCachedShape}
* 1: SpecializationActive {@link GetDictIfExistsNode#getNoDict}
* 2: SpecializationActive {@link GetDictIfExistsNode#getConstant}
* 3: SpecializationActive {@link GetDictIfExistsNode#doPythonObject}
* 4: SpecializationActive {@link GetDictIfExistsNode#doNativeObject}
* 5: SpecializationActive {@link GetDictIfExistsNode#doOther}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private GetNoDictCachedShapeData getNoDictCachedShape_cache;
@UnsafeAccessedField @CompilationFinal private GetConstantData getConstant_cache;
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#doPythonObject}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child private DynamicObjectLibrary pythonObject_dylib_;
@Child private NativeObjectData nativeObject_cache;
private GetDictIfExistsNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value) {
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */) && arg0Value instanceof PythonObject) {
return false;
}
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)] */) && arg0Value instanceof PythonAbstractNativeObject) {
return false;
}
return true;
}
@Override
public PDict execute(Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] || SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] || SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] || SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] || SpecializationActive[GetDictIfExistsNode.doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)] || SpecializationActive[GetDictIfExistsNode.doOther(Object)] */) {
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] || SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] || SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] || SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] */) {
GetNoDictCachedShapeData s0_ = this.getNoDictCachedShape_cache;
if (s0_ != null) {
if ((arg0Value_.getShape() == s0_.cachedShape_)) {
assert DSLSupport.assertIdempotence((GetDictIfExistsNode.hasNoDict(s0_.cachedShape_)));
return GetDictIfExistsNode.getNoDictCachedShape(arg0Value_, s0_.cachedShape_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] */) {
if ((GetDictIfExistsNode.hasNoDict(arg0Value_.getShape()))) {
return GetDictIfExistsNode.getNoDict(arg0Value_);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] */) {
GetConstantData s2_ = this.getConstant_cache;
if (s2_ != null) {
{
PythonObject cached__ = (s2_.weakCachedGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cached__ != null) && (arg0Value_ == cached__) && (dictIsConstant(cached__))) {
PDict dict__ = (s2_.weakDictGen__.get());
if ((dict__ != null) && (dict__ != null)) {
return GetDictIfExistsNode.getConstant(arg0Value_, cached__, dict__);
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */) {
{
DynamicObjectLibrary dylib__ = this.pythonObject_dylib_;
if (dylib__ != null) {
return GetDictIfExistsNode.doPythonObject(arg0Value_, dylib__);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
NativeObjectData s4_ = this.nativeObject_cache;
if (s4_ != null) {
return doNativeObject(arg0Value_, s4_.toSulong_, s4_.toJava_, s4_.callGetDictNode_);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doOther(Object)] */) {
if (fallbackGuard_(state_0, arg0Value)) {
return GetDictIfExistsNode.doOther(arg0Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
@Override
public PDict execute(PythonObject arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b101111) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] || SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] || SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] || SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] || SpecializationActive[GetDictIfExistsNode.doOther(Object)] */) {
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] || SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] || SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] || SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] */) {
GetNoDictCachedShapeData s0_ = this.getNoDictCachedShape_cache;
if (s0_ != null) {
if ((arg0Value.getShape() == s0_.cachedShape_)) {
assert DSLSupport.assertIdempotence((GetDictIfExistsNode.hasNoDict(s0_.cachedShape_)));
return GetDictIfExistsNode.getNoDictCachedShape(arg0Value, s0_.cachedShape_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] */) {
if ((GetDictIfExistsNode.hasNoDict(arg0Value.getShape()))) {
return GetDictIfExistsNode.getNoDict(arg0Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] */) {
GetConstantData s2_ = this.getConstant_cache;
if (s2_ != null) {
{
PythonObject cached__ = (s2_.weakCachedGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cached__ != null) && (arg0Value == cached__) && (dictIsConstant(cached__))) {
PDict dict__ = (s2_.weakDictGen__.get());
if ((dict__ != null) && (dict__ != null)) {
return GetDictIfExistsNode.getConstant(arg0Value, cached__, dict__);
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */) {
{
DynamicObjectLibrary dylib__ = this.pythonObject_dylib_;
if (dylib__ != null) {
return GetDictIfExistsNode.doPythonObject(arg0Value, dylib__);
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[GetDictIfExistsNode.doOther(Object)] */) {
if (fallbackGuard_(state_0, arg0Value)) {
return GetDictIfExistsNode.doOther(arg0Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
@SuppressWarnings("unused")
private PDict executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] */) {
while (true) {
int count0_ = 0;
GetNoDictCachedShapeData s0_ = GET_NO_DICT_CACHED_SHAPE_CACHE_UPDATER.getVolatile(this);
GetNoDictCachedShapeData s0_original = s0_;
while (s0_ != null) {
if ((arg0Value_.getShape() == s0_.cachedShape_)) {
assert DSLSupport.assertIdempotence((GetDictIfExistsNode.hasNoDict(s0_.cachedShape_)));
break;
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
Shape cachedShape__ = (arg0Value_.getShape());
if ((arg0Value_.getShape() == cachedShape__) && (GetDictIfExistsNode.hasNoDict(cachedShape__))) {
s0_ = new GetNoDictCachedShapeData();
Objects.requireNonNull(cachedShape__, "Specialization 'getNoDictCachedShape(PythonObject, Shape)' cache 'cachedShape' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.cachedShape_ = cachedShape__;
if (!GET_NO_DICT_CACHED_SHAPE_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return GetDictIfExistsNode.getNoDictCachedShape(arg0Value_, s0_.cachedShape_);
}
break;
}
}
if ((GetDictIfExistsNode.hasNoDict(arg0Value_.getShape()))) {
this.getNoDictCachedShape_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[GetDictIfExistsNode.getNoDictCachedShape(PythonObject, Shape)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[GetDictIfExistsNode.getNoDict(PythonObject)] */;
this.state_0_ = state_0;
return GetDictIfExistsNode.getNoDict(arg0Value_);
}
{
PDict dict__ = null;
PythonObject cached__ = null;
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */) {
while (true) {
int count2_ = 0;
GetConstantData s2_ = GET_CONSTANT_CACHE_UPDATER.getVolatile(this);
GetConstantData s2_original = s2_;
while (s2_ != null) {
{
cached__ = (s2_.weakCachedGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cached__ != null) && (arg0Value_ == cached__) && (dictIsConstant(cached__))) {
dict__ = (s2_.weakDictGen__.get());
if ((dict__ != null) && (dict__ != null)) {
break;
}
}
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference weakCachedGen___ = (new TruffleWeakReference<>(arg0Value_));
cached__ = (weakCachedGen___.get());
if ((cached__ != null) && (arg0Value_ == cached__) && (dictIsConstant(cached__))) {
TruffleWeakReference weakDictGen___ = (new TruffleWeakReference<>(getDictUncached(arg0Value_)));
dict__ = (weakDictGen___.get());
if ((dict__ != null) && (dict__ != null)) {
s2_ = new GetConstantData();
s2_.weakDictGen__ = weakDictGen___;
s2_.weakCachedGen__ = weakCachedGen___;
if (!GET_CONSTANT_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] */;
this.state_0_ = state_0;
}
}
}
}
if (s2_ != null) {
return GetDictIfExistsNode.getConstant(arg0Value_, cached__, dict__);
}
break;
}
}
}
DynamicObjectLibrary dylib__ = this.insert((DYNAMIC_OBJECT_LIBRARY_.createDispatched(4)));
Objects.requireNonNull(dylib__, "Specialization 'doPythonObject(PythonObject, DynamicObjectLibrary)' cache 'dylib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.pythonObject_dylib_ = dylib__;
this.getConstant_cache = null;
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[GetDictIfExistsNode.getConstant(PythonObject, PythonObject, PDict)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[GetDictIfExistsNode.doPythonObject(PythonObject, DynamicObjectLibrary)] */;
this.state_0_ = state_0;
return GetDictIfExistsNode.doPythonObject(arg0Value_, dylib__);
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
NativeObjectData s4_ = this.insert(new NativeObjectData());
PythonToNativeNode toSulong__ = s4_.insert((PythonToNativeNodeGen.create()));
Objects.requireNonNull(toSulong__, "Specialization 'doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)' cache 'toSulong' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.toSulong_ = toSulong__;
NativeToPythonNode toJava__ = s4_.insert((NativeToPythonNodeGen.create()));
Objects.requireNonNull(toJava__, "Specialization 'doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)' cache 'toJava' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.toJava_ = toJava__;
PCallCapiFunction callGetDictNode__ = s4_.insert((PCallCapiFunction.create()));
Objects.requireNonNull(callGetDictNode__, "Specialization 'doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)' cache 'callGetDictNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callGetDictNode_ = callGetDictNode__;
VarHandle.storeStoreFence();
this.nativeObject_cache = s4_;
state_0 = state_0 | 0b10000 /* add SpecializationActive[GetDictIfExistsNode.doNativeObject(PythonAbstractNativeObject, PythonToNativeNode, NativeToPythonNode, PCallCapiFunction)] */;
this.state_0_ = state_0;
return doNativeObject(arg0Value_, toSulong__, toJava__, callGetDictNode__);
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[GetDictIfExistsNode.doOther(Object)] */;
this.state_0_ = state_0;
return GetDictIfExistsNode.doOther(arg0Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static GetDictIfExistsNode create() {
return new GetDictIfExistsNodeGen();
}
@NeverDefault
public static GetDictIfExistsNode getUncached() {
return GetDictIfExistsNodeGen.UNCACHED;
}
@GeneratedBy(GetDictIfExistsNode.class)
@DenyReplace
private static final class GetNoDictCachedShapeData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#getNoDictCachedShape}
* Parameter: {@link Shape} cachedShape
*/
@CompilationFinal Shape cachedShape_;
GetNoDictCachedShapeData() {
}
}
@GeneratedBy(GetDictIfExistsNode.class)
@DenyReplace
private static final class GetConstantData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#getConstant}
* Parameter: {@link TruffleWeakReference} weakDictGen_
*/
@CompilationFinal TruffleWeakReference weakDictGen__;
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#getConstant}
* Parameter: {@link TruffleWeakReference} weakCachedGen_
*/
@CompilationFinal TruffleWeakReference weakCachedGen__;
GetConstantData() {
}
}
@GeneratedBy(GetDictIfExistsNode.class)
@DenyReplace
private static final class NativeObjectData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#doNativeObject}
* Parameter: {@link PythonToNativeNode} toSulong
*/
@Child PythonToNativeNode toSulong_;
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#doNativeObject}
* Parameter: {@link NativeToPythonNode} toJava
*/
@Child NativeToPythonNode toJava_;
/**
* Source Info:
* Specialization: {@link GetDictIfExistsNode#doNativeObject}
* Parameter: {@link PCallCapiFunction} callGetDictNode
*/
@Child PCallCapiFunction callGetDictNode_;
NativeObjectData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(GetDictIfExistsNode.class)
@DenyReplace
private static final class Uncached extends GetDictIfExistsNode {
@TruffleBoundary
@Override
public PDict execute(Object arg0Value) {
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((GetDictIfExistsNode.hasNoDict(arg0Value_.getShape()))) {
return GetDictIfExistsNode.getNoDict(arg0Value_);
}
return GetDictIfExistsNode.doPythonObject(arg0Value_, (DYNAMIC_OBJECT_LIBRARY_.getUncached()));
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
return doNativeObject(arg0Value_, (PythonToNativeNodeGen.getUncached()), (NativeToPythonNodeGen.getUncached()), (PCallCapiFunction.getUncached()));
}
return GetDictIfExistsNode.doOther(arg0Value);
}
@TruffleBoundary
@Override
public PDict execute(PythonObject arg0Value) {
if ((GetDictIfExistsNode.hasNoDict(arg0Value.getShape()))) {
return GetDictIfExistsNode.getNoDict(arg0Value);
}
return GetDictIfExistsNode.doPythonObject(arg0Value, (DYNAMIC_OBJECT_LIBRARY_.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy