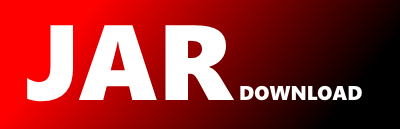
com.oracle.graal.python.nodes.util.BufferToTruffleStringNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.util;
import com.oracle.graal.python.builtins.objects.buffer.PythonBufferAccessLibrary;
import com.oracle.graal.python.builtins.objects.bytes.PBytesLike;
import com.oracle.graal.python.builtins.objects.memoryview.PMemoryView;
import com.oracle.graal.python.builtins.objects.mmap.PMMap;
import com.oracle.graal.python.runtime.PosixSupportLibrary;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromByteArrayNode;
import com.oracle.truffle.api.strings.TruffleString.FromNativePointerNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link BufferToTruffleStringNode#doWithInternalByteArray}
* Activation probability: 0.27381
* With/without class size: 10/4 bytes
* Specialization {@link BufferToTruffleStringNode#doWithInternalByteArray}
* Activation probability: 0.23095
* With/without class size: 6/0 bytes
* Specialization {@link BufferToTruffleStringNode#doNativeBytesLike}
* Activation probability: 0.18810
* With/without class size: 6/0 bytes
* Specialization {@link BufferToTruffleStringNode#doMMap}
* Activation probability: 0.14524
* With/without class size: 8/9 bytes
* Specialization {@link BufferToTruffleStringNode#doMemoryView}
* Activation probability: 0.10238
* With/without class size: 6/4 bytes
* Specialization {@link BufferToTruffleStringNode#doWithInternalOrCopiedByteArray}
* Activation probability: 0.05952
* With/without class size: 5/4 bytes
*
*/
@GeneratedBy(BufferToTruffleStringNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class BufferToTruffleStringNodeGen extends BufferToTruffleStringNode {
private static final StateField M_MAP__BUFFER_TO_TRUFFLE_STRING_NODE_M_MAP_STATE_0_UPDATER = StateField.create(MMapData.lookup_(), "mMap_state_0_");
static final ReferenceField WITH_INTERNAL_BYTE_ARRAY0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "withInternalByteArray0_cache", WithInternalByteArray0Data.class);
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doMMap}
* Parameter: {@link InlinedBranchProfile} unsupportedPosix
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_M_MAP_UNSUPPORTED_POSIX_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, M_MAP__BUFFER_TO_TRUFFLE_STRING_NODE_M_MAP_STATE_0_UPDATER.subUpdater(0, 1)));
private static final LibraryFactory PYTHON_BUFFER_ACCESS_LIBRARY_ = LibraryFactory.resolve(PythonBufferAccessLibrary.class);
private static final LibraryFactory POSIX_SUPPORT_LIBRARY_ = LibraryFactory.resolve(PosixSupportLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link BufferToTruffleStringNode#doWithInternalByteArray}
* 1: SpecializationActive {@link BufferToTruffleStringNode#doWithInternalByteArray}
* 2: SpecializationActive {@link BufferToTruffleStringNode#doNativeBytesLike}
* 3: SpecializationActive {@link BufferToTruffleStringNode#doMMap}
* 4: SpecializationActive {@link BufferToTruffleStringNode#doMemoryView}
* 5: SpecializationActive {@link BufferToTruffleStringNode#doWithInternalOrCopiedByteArray}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doWithInternalByteArray}
* Parameter: {@link FromByteArrayNode} fromByteArrayNode
*/
@Child private FromByteArrayNode fromByteArrayNode;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doNativeBytesLike}
* Parameter: {@link FromNativePointerNode} fromNativePointerNode
*/
@Child private FromNativePointerNode fromNativePointerNode;
@UnsafeAccessedField @Child private WithInternalByteArray0Data withInternalByteArray0_cache;
@Child private MMapData mMap_cache;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doMemoryView}
* Parameter: {@link BufferToTruffleStringNode} bufferToTruffleStringNode
*/
@Child private BufferToTruffleStringNode memoryView_bufferToTruffleStringNode_;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doWithInternalOrCopiedByteArray}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child private PythonBufferAccessLibrary fallback_bufferLib_;
private BufferToTruffleStringNodeGen(boolean allowMemoryView) {
super(allowMemoryView);
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value, int arg1Value) {
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) && ((PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached()).hasInternalByteArray(arg0Value))) {
return false;
}
if (arg0Value instanceof PBytesLike) {
PBytesLike arg0Value_ = (PBytesLike) arg0Value;
if ((BufferToTruffleStringNode.isNativeByteSequenceStorage(arg0Value_.getSequenceStorage()))) {
return false;
}
}
if (!((state_0 & 0b1000) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)] */) && arg0Value instanceof PMMap) {
return false;
}
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doMemoryView(PMemoryView, int, BufferToTruffleStringNode)] */) && arg0Value instanceof PMemoryView && (allowMemoryView)) {
return false;
}
return true;
}
@ExplodeLoop
@Override
public TruffleString execute(Object arg0Value, int arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] || SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] || SpecializationActive[BufferToTruffleStringNode.doNativeBytesLike(PBytesLike, int, FromNativePointerNode)] || SpecializationActive[BufferToTruffleStringNode.doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)] || SpecializationActive[BufferToTruffleStringNode.doMemoryView(PMemoryView, int, BufferToTruffleStringNode)] || SpecializationActive[BufferToTruffleStringNode.doWithInternalOrCopiedByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) {
if ((state_0 & 0b11) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] || SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) {
WithInternalByteArray0Data s0_ = this.withInternalByteArray0_cache;
while (s0_ != null) {
{
FromByteArrayNode fromByteArrayNode_ = this.fromByteArrayNode;
if (fromByteArrayNode_ != null) {
if ((s0_.bufferLib_.accepts(arg0Value)) && (s0_.bufferLib_.hasInternalByteArray(arg0Value))) {
return BufferToTruffleStringNode.doWithInternalByteArray(arg0Value, arg1Value, s0_.bufferLib_, fromByteArrayNode_);
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
FromByteArrayNode fromByteArrayNode_ = this.fromByteArrayNode;
if (fromByteArrayNode_ != null) {
PythonBufferAccessLibrary bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached());
if ((bufferLib__.hasInternalByteArray(arg0Value))) {
return this.withInternalByteArray1Boundary(state_0, arg0Value, arg1Value, fromByteArrayNode_);
}
}
}
} finally {
encapsulating_.set(prev_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doNativeBytesLike(PBytesLike, int, FromNativePointerNode)] */ && arg0Value instanceof PBytesLike) {
PBytesLike arg0Value_ = (PBytesLike) arg0Value;
{
FromNativePointerNode fromNativePointerNode_ = this.fromNativePointerNode;
if (fromNativePointerNode_ != null) {
if ((BufferToTruffleStringNode.isNativeByteSequenceStorage(arg0Value_.getSequenceStorage()))) {
return BufferToTruffleStringNode.doNativeBytesLike(arg0Value_, arg1Value, fromNativePointerNode_);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)] */ && arg0Value instanceof PMMap) {
PMMap arg0Value_ = (PMMap) arg0Value;
MMapData s3_ = this.mMap_cache;
if (s3_ != null) {
{
FromNativePointerNode fromNativePointerNode_1 = this.fromNativePointerNode;
if (fromNativePointerNode_1 != null) {
FromByteArrayNode fromByteArrayNode_1 = this.fromByteArrayNode;
if (fromByteArrayNode_1 != null) {
Node inliningTarget__ = (s3_);
return BufferToTruffleStringNode.doMMap(arg0Value_, arg1Value, inliningTarget__, INLINED_M_MAP_UNSUPPORTED_POSIX_, s3_.posixLib_, s3_.bufferLib_, fromNativePointerNode_1, fromByteArrayNode_1);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doMemoryView(PMemoryView, int, BufferToTruffleStringNode)] */ && arg0Value instanceof PMemoryView) {
PMemoryView arg0Value_ = (PMemoryView) arg0Value;
{
BufferToTruffleStringNode bufferToTruffleStringNode__ = this.memoryView_bufferToTruffleStringNode_;
if (bufferToTruffleStringNode__ != null) {
assert DSLSupport.assertIdempotence((allowMemoryView));
return BufferToTruffleStringNode.doMemoryView(arg0Value_, arg1Value, bufferToTruffleStringNode__);
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[BufferToTruffleStringNode.doWithInternalOrCopiedByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) {
{
PythonBufferAccessLibrary bufferLib__1 = this.fallback_bufferLib_;
if (bufferLib__1 != null) {
FromByteArrayNode fromByteArrayNode_2 = this.fromByteArrayNode;
if (fromByteArrayNode_2 != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return BufferToTruffleStringNode.doWithInternalOrCopiedByteArray(arg0Value, arg1Value, bufferLib__1, fromByteArrayNode_2);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private TruffleString withInternalByteArray1Boundary(int state_0, Object arg0Value, int arg1Value, FromByteArrayNode fromByteArrayNode_) {
{
PythonBufferAccessLibrary bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached());
return BufferToTruffleStringNode.doWithInternalByteArray(arg0Value, arg1Value, bufferLib__, fromByteArrayNode_);
}
}
@SuppressWarnings("unused")
private TruffleString executeAndSpecialize(Object arg0Value, int arg1Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */) {
while (true) {
int count0_ = 0;
WithInternalByteArray0Data s0_ = WITH_INTERNAL_BYTE_ARRAY0_CACHE_UPDATER.getVolatile(this);
WithInternalByteArray0Data s0_original = s0_;
while (s0_ != null) {
{
FromByteArrayNode fromByteArrayNode_ = this.fromByteArrayNode;
if (fromByteArrayNode_ != null) {
if ((s0_.bufferLib_.accepts(arg0Value)) && (s0_.bufferLib_.hasInternalByteArray(arg0Value))) {
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
PythonBufferAccessLibrary bufferLib__ = this.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.create(arg0Value)));
// assert (s0_.bufferLib_.accepts(arg0Value));
if ((bufferLib__.hasInternalByteArray(arg0Value)) && count0_ < (4)) {
s0_ = this.insert(new WithInternalByteArray0Data(s0_original));
Objects.requireNonNull(s0_.insert(bufferLib__), "Specialization 'doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.bufferLib_ = bufferLib__;
FromByteArrayNode fromByteArrayNode_;
FromByteArrayNode fromByteArrayNode__shared = this.fromByteArrayNode;
if (fromByteArrayNode__shared != null) {
fromByteArrayNode_ = fromByteArrayNode__shared;
} else {
fromByteArrayNode_ = s0_.insert((FromByteArrayNode.create()));
if (fromByteArrayNode_ == null) {
throw new IllegalStateException("Specialization 'doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromByteArrayNode == null) {
this.fromByteArrayNode = fromByteArrayNode_;
}
if (!WITH_INTERNAL_BYTE_ARRAY0_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return BufferToTruffleStringNode.doWithInternalByteArray(arg0Value, arg1Value, s0_.bufferLib_, this.fromByteArrayNode);
}
break;
}
}
{
PythonBufferAccessLibrary bufferLib__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
bufferLib__ = (PYTHON_BUFFER_ACCESS_LIBRARY_.getUncached());
if ((bufferLib__.hasInternalByteArray(arg0Value))) {
FromByteArrayNode fromByteArrayNode_;
FromByteArrayNode fromByteArrayNode__shared = this.fromByteArrayNode;
if (fromByteArrayNode__shared != null) {
fromByteArrayNode_ = fromByteArrayNode__shared;
} else {
fromByteArrayNode_ = this.insert((FromByteArrayNode.create()));
if (fromByteArrayNode_ == null) {
throw new IllegalStateException("Specialization 'doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromByteArrayNode == null) {
VarHandle.storeStoreFence();
this.fromByteArrayNode = fromByteArrayNode_;
}
this.withInternalByteArray0_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[BufferToTruffleStringNode.doWithInternalByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */;
this.state_0_ = state_0;
return BufferToTruffleStringNode.doWithInternalByteArray(arg0Value, arg1Value, bufferLib__, fromByteArrayNode_);
}
}
} finally {
encapsulating_.set(prev_);
}
}
}
if (arg0Value instanceof PBytesLike) {
PBytesLike arg0Value_ = (PBytesLike) arg0Value;
if ((BufferToTruffleStringNode.isNativeByteSequenceStorage(arg0Value_.getSequenceStorage()))) {
FromNativePointerNode fromNativePointerNode_;
FromNativePointerNode fromNativePointerNode__shared = this.fromNativePointerNode;
if (fromNativePointerNode__shared != null) {
fromNativePointerNode_ = fromNativePointerNode__shared;
} else {
fromNativePointerNode_ = this.insert((FromNativePointerNode.create()));
if (fromNativePointerNode_ == null) {
throw new IllegalStateException("Specialization 'doNativeBytesLike(PBytesLike, int, FromNativePointerNode)' contains a shared cache with name 'fromNativePointerNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromNativePointerNode == null) {
VarHandle.storeStoreFence();
this.fromNativePointerNode = fromNativePointerNode_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[BufferToTruffleStringNode.doNativeBytesLike(PBytesLike, int, FromNativePointerNode)] */;
this.state_0_ = state_0;
return BufferToTruffleStringNode.doNativeBytesLike(arg0Value_, arg1Value, fromNativePointerNode_);
}
}
{
Node inliningTarget__ = null;
if (arg0Value instanceof PMMap) {
PMMap arg0Value_ = (PMMap) arg0Value;
MMapData s3_ = this.insert(new MMapData());
inliningTarget__ = (s3_);
PosixSupportLibrary posixLib__ = s3_.insert((POSIX_SUPPORT_LIBRARY_.createDispatched(4)));
Objects.requireNonNull(posixLib__, "Specialization 'doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)' cache 'posixLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.posixLib_ = posixLib__;
PythonBufferAccessLibrary bufferLib__2 = s3_.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.createDispatched(1)));
Objects.requireNonNull(bufferLib__2, "Specialization 'doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.bufferLib_ = bufferLib__2;
FromNativePointerNode fromNativePointerNode_1;
FromNativePointerNode fromNativePointerNode_1_shared = this.fromNativePointerNode;
if (fromNativePointerNode_1_shared != null) {
fromNativePointerNode_1 = fromNativePointerNode_1_shared;
} else {
fromNativePointerNode_1 = s3_.insert((FromNativePointerNode.create()));
if (fromNativePointerNode_1 == null) {
throw new IllegalStateException("Specialization 'doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)' contains a shared cache with name 'fromNativePointerNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromNativePointerNode == null) {
this.fromNativePointerNode = fromNativePointerNode_1;
}
FromByteArrayNode fromByteArrayNode_1;
FromByteArrayNode fromByteArrayNode_1_shared = this.fromByteArrayNode;
if (fromByteArrayNode_1_shared != null) {
fromByteArrayNode_1 = fromByteArrayNode_1_shared;
} else {
fromByteArrayNode_1 = s3_.insert((FromByteArrayNode.create()));
if (fromByteArrayNode_1 == null) {
throw new IllegalStateException("Specialization 'doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromByteArrayNode == null) {
this.fromByteArrayNode = fromByteArrayNode_1;
}
VarHandle.storeStoreFence();
this.mMap_cache = s3_;
state_0 = state_0 | 0b1000 /* add SpecializationActive[BufferToTruffleStringNode.doMMap(PMMap, int, Node, InlinedBranchProfile, PosixSupportLibrary, PythonBufferAccessLibrary, FromNativePointerNode, FromByteArrayNode)] */;
this.state_0_ = state_0;
return BufferToTruffleStringNode.doMMap(arg0Value_, arg1Value, inliningTarget__, INLINED_M_MAP_UNSUPPORTED_POSIX_, posixLib__, bufferLib__2, fromNativePointerNode_1, fromByteArrayNode_1);
}
}
if (arg0Value instanceof PMemoryView) {
PMemoryView arg0Value_ = (PMemoryView) arg0Value;
if ((allowMemoryView)) {
BufferToTruffleStringNode bufferToTruffleStringNode__ = this.insert((BufferToTruffleStringNode.create(false)));
Objects.requireNonNull(bufferToTruffleStringNode__, "Specialization 'doMemoryView(PMemoryView, int, BufferToTruffleStringNode)' cache 'bufferToTruffleStringNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.memoryView_bufferToTruffleStringNode_ = bufferToTruffleStringNode__;
state_0 = state_0 | 0b10000 /* add SpecializationActive[BufferToTruffleStringNode.doMemoryView(PMemoryView, int, BufferToTruffleStringNode)] */;
this.state_0_ = state_0;
return BufferToTruffleStringNode.doMemoryView(arg0Value_, arg1Value, bufferToTruffleStringNode__);
}
}
PythonBufferAccessLibrary bufferLib__1 = this.insert((PYTHON_BUFFER_ACCESS_LIBRARY_.createDispatched(5)));
Objects.requireNonNull(bufferLib__1, "Specialization 'doWithInternalOrCopiedByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)' cache 'bufferLib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.fallback_bufferLib_ = bufferLib__1;
FromByteArrayNode fromByteArrayNode_2;
FromByteArrayNode fromByteArrayNode_2_shared = this.fromByteArrayNode;
if (fromByteArrayNode_2_shared != null) {
fromByteArrayNode_2 = fromByteArrayNode_2_shared;
} else {
fromByteArrayNode_2 = this.insert((FromByteArrayNode.create()));
if (fromByteArrayNode_2 == null) {
throw new IllegalStateException("Specialization 'doWithInternalOrCopiedByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromByteArrayNode == null) {
VarHandle.storeStoreFence();
this.fromByteArrayNode = fromByteArrayNode_2;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[BufferToTruffleStringNode.doWithInternalOrCopiedByteArray(Object, int, PythonBufferAccessLibrary, FromByteArrayNode)] */;
this.state_0_ = state_0;
return BufferToTruffleStringNode.doWithInternalOrCopiedByteArray(arg0Value, arg1Value, bufferLib__1, fromByteArrayNode_2);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
WithInternalByteArray0Data s0_ = this.withInternalByteArray0_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static BufferToTruffleStringNode create(boolean allowMemoryView) {
return new BufferToTruffleStringNodeGen(allowMemoryView);
}
@GeneratedBy(BufferToTruffleStringNode.class)
@DenyReplace
private static final class WithInternalByteArray0Data extends Node implements SpecializationDataNode {
@Child WithInternalByteArray0Data next_;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doWithInternalByteArray}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child PythonBufferAccessLibrary bufferLib_;
WithInternalByteArray0Data(WithInternalByteArray0Data next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(BufferToTruffleStringNode.class)
@DenyReplace
private static final class MMapData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link BufferToTruffleStringNode#doMMap}
* Parameter: {@link InlinedBranchProfile} unsupportedPosix
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int mMap_state_0_;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doMMap}
* Parameter: {@link PosixSupportLibrary} posixLib
*/
@Child PosixSupportLibrary posixLib_;
/**
* Source Info:
* Specialization: {@link BufferToTruffleStringNode#doMMap}
* Parameter: {@link PythonBufferAccessLibrary} bufferLib
*/
@Child PythonBufferAccessLibrary bufferLib_;
MMapData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy