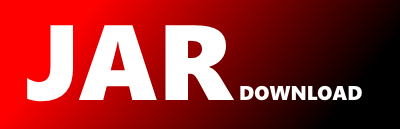
com.oracle.graal.python.nodes.util.CastToJavaIntLossyNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.util;
import com.oracle.graal.python.builtins.objects.ints.PInt;
import com.oracle.graal.python.nodes.truffle.PythonArithmeticTypesGen;
import com.oracle.graal.python.util.OverflowException;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
/**
* Debug Info:
* Specialization {@link CastToJavaIntNode#toInt}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link CastToJavaIntLossyNode#toInt}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link CastToJavaIntLossyNode#toIntPInt}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link CastToJavaIntLossyNode#toIntOverflow}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link CastToJavaIntNode#doUnsupported}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(CastToJavaIntLossyNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class CastToJavaIntLossyNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static CastToJavaIntLossyNode getUncached() {
return CastToJavaIntLossyNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static CastToJavaIntLossyNode inline(@RequiredField(bits = 11, value = StateField.class) InlineTarget target) {
return new CastToJavaIntLossyNodeGen.Inlined(target);
}
@GeneratedBy(CastToJavaIntLossyNode.class)
@DenyReplace
private static final class Inlined extends CastToJavaIntLossyNode {
/**
* State Info:
* 0: SpecializationActive {@link CastToJavaIntNode#toInt}
* 1: SpecializationActive {@link CastToJavaIntLossyNode#toInt}
* 2: SpecializationActive {@link CastToJavaIntLossyNode#toIntPInt}
* 3: SpecializationExcluded {@link CastToJavaIntLossyNode#toIntPInt}
* 4: SpecializationActive {@link CastToJavaIntLossyNode#toIntOverflow}
* 5: SpecializationActive {@link CastToJavaIntNode#doUnsupported}
* 6-7: ImplicitCast[type=int, index=1]
* 8-10: ImplicitCast[type=long, index=1]
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(CastToJavaIntLossyNode.class);
this.state_0_ = target.getState(0, 11);
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value) {
if (PythonArithmeticTypesGen.isImplicitLong(arg1Value)) {
return false;
}
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toIntOverflow(PInt)] */) && arg1Value instanceof PInt) {
return false;
}
return true;
}
@Override
public int execute(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b110111) != 0 /* is SpecializationActive[CastToJavaIntNode.toInt(int)] || SpecializationActive[CastToJavaIntLossyNode.toInt(long)] || SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] || SpecializationActive[CastToJavaIntLossyNode.toIntOverflow(PInt)] || SpecializationActive[CastToJavaIntNode.doUnsupported(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[CastToJavaIntNode.toInt(int)] */ && PythonArithmeticTypesGen.isImplicitInteger((state_0 & 0b11000000) >>> 6 /* get-int ImplicitCast[type=int, index=1] */, arg1Value)) {
int arg1Value_ = PythonArithmeticTypesGen.asImplicitInteger((state_0 & 0b11000000) >>> 6 /* get-int ImplicitCast[type=int, index=1] */, arg1Value);
return CastToJavaIntNode.toInt(arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toInt(long)] */ && PythonArithmeticTypesGen.isImplicitLong((state_0 & 0b11100000000) >>> 8 /* get-int ImplicitCast[type=long, index=1] */, arg1Value)) {
long arg1Value_ = PythonArithmeticTypesGen.asImplicitLong((state_0 & 0b11100000000) >>> 8 /* get-int ImplicitCast[type=long, index=1] */, arg1Value);
return CastToJavaIntLossyNode.toInt(arg1Value_);
}
if ((state_0 & 0b10100) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] || SpecializationActive[CastToJavaIntLossyNode.toIntOverflow(PInt)] */ && arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] */) {
try {
return CastToJavaIntLossyNode.toIntPInt(arg1Value_);
} catch (OverflowException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_.get(arg0Value);
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] */;
state_0 = state_0 | 0b1000 /* add SpecializationExcluded */;
this.state_0_.set(arg0Value, state_0);
return executeAndSpecialize(arg0Value, arg1Value_);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toIntOverflow(PInt)] */) {
return CastToJavaIntLossyNode.toIntOverflow(arg1Value_);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[CastToJavaIntNode.doUnsupported(Object)] */) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return CastToJavaIntNode.doUnsupported(arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@Override
public int execute(Node arg0Value, long arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b100010) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toInt(long)] || SpecializationActive[CastToJavaIntNode.doUnsupported(Object)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CastToJavaIntLossyNode.toInt(long)] */ && PythonArithmeticTypesGen.isImplicitLong((state_0 & 0b11100000000) >>> 8 /* get-int ImplicitCast[type=long, index=1] */, arg1Value)) {
long arg1Value_ = PythonArithmeticTypesGen.asImplicitLong((state_0 & 0b11100000000) >>> 8 /* get-int ImplicitCast[type=long, index=1] */, arg1Value);
return CastToJavaIntLossyNode.toInt(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[CastToJavaIntNode.doUnsupported(Object)] */) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return CastToJavaIntNode.doUnsupported(arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private int executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
{
int intCast1;
if ((intCast1 = PythonArithmeticTypesGen.specializeImplicitInteger(arg1Value)) != 0) {
int arg1Value_ = PythonArithmeticTypesGen.asImplicitInteger(intCast1, arg1Value);
state_0 = (state_0 | (intCast1 << 6) /* set-int ImplicitCast[type=int, index=1] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[CastToJavaIntNode.toInt(int)] */;
this.state_0_.set(arg0Value, state_0);
return CastToJavaIntNode.toInt(arg1Value_);
}
}
{
int longCast1;
if ((longCast1 = PythonArithmeticTypesGen.specializeImplicitLong(arg1Value)) != 0) {
long arg1Value_ = PythonArithmeticTypesGen.asImplicitLong(longCast1, arg1Value);
state_0 = (state_0 | (longCast1 << 8) /* set-int ImplicitCast[type=long, index=1] */);
state_0 = state_0 | 0b10 /* add SpecializationActive[CastToJavaIntLossyNode.toInt(long)] */;
this.state_0_.set(arg0Value, state_0);
return CastToJavaIntLossyNode.toInt(arg1Value_);
}
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[CastToJavaIntLossyNode.toIntOverflow(PInt)] */ && ((state_0 & 0b1000)) == 0 /* is-not SpecializationExcluded */) {
state_0 = state_0 | 0b100 /* add SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] */;
this.state_0_.set(arg0Value, state_0);
try {
return CastToJavaIntLossyNode.toIntPInt(arg1Value_);
} catch (OverflowException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_.get(arg0Value);
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] */;
state_0 = state_0 | 0b1000 /* add SpecializationExcluded */;
this.state_0_.set(arg0Value, state_0);
return executeAndSpecialize(arg0Value, arg1Value_);
}
}
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[CastToJavaIntLossyNode.toIntPInt(PInt)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[CastToJavaIntLossyNode.toIntOverflow(PInt)] */;
this.state_0_.set(arg0Value, state_0);
return CastToJavaIntLossyNode.toIntOverflow(arg1Value_);
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[CastToJavaIntNode.doUnsupported(Object)] */;
this.state_0_.set(arg0Value, state_0);
return CastToJavaIntNode.doUnsupported(arg1Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(CastToJavaIntLossyNode.class)
@DenyReplace
private static final class Uncached extends CastToJavaIntLossyNode {
@TruffleBoundary
@Override
public int execute(Node arg0Value, Object arg1Value) {
if (PythonArithmeticTypesGen.isImplicitInteger(arg1Value)) {
int arg1Value_ = PythonArithmeticTypesGen.asImplicitInteger(arg1Value);
return CastToJavaIntNode.toInt(arg1Value_);
}
if (PythonArithmeticTypesGen.isImplicitLong(arg1Value)) {
long arg1Value_ = PythonArithmeticTypesGen.asImplicitLong(arg1Value);
return CastToJavaIntLossyNode.toInt(arg1Value_);
}
if (arg1Value instanceof PInt) {
PInt arg1Value_ = (PInt) arg1Value;
return CastToJavaIntLossyNode.toIntOverflow(arg1Value_);
}
return CastToJavaIntNode.doUnsupported(arg1Value);
}
@TruffleBoundary
@Override
public int execute(Node arg0Value, long arg1Value) {
if (PythonArithmeticTypesGen.isImplicitLong(arg1Value)) {
long arg1Value_ = PythonArithmeticTypesGen.asImplicitLong(arg1Value);
return CastToJavaIntLossyNode.toInt(arg1Value_);
}
return CastToJavaIntNode.doUnsupported(arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy