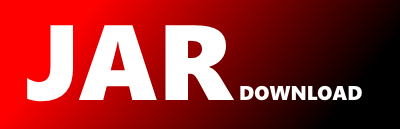
com.oracle.graal.python.runtime.AddrInfoCursorImplGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.graal.python.runtime.NFIPosixSupport.AddrInfoCursorImpl;
import com.oracle.graal.python.runtime.NFIPosixSupport.InvokeNativeFunction;
import com.oracle.graal.python.runtime.PosixSupportLibrary.AddrInfoCursor;
import com.oracle.graal.python.runtime.PosixSupportLibrary.AddrInfoCursorLibrary;
import com.oracle.graal.python.runtime.PosixSupportLibrary.UniversalSockAddr;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.VarHandle;
@GeneratedBy(AddrInfoCursorImpl.class)
@SuppressWarnings("javadoc")
final class AddrInfoCursorImplGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(AddrInfoCursorImpl.class, new AddrInfoCursorLibraryExports());
}
private AddrInfoCursorImplGen() {
}
@GeneratedBy(AddrInfoCursorImpl.class)
protected static class AddrInfoCursorLibraryExports extends LibraryExport {
private AddrInfoCursorLibraryExports() {
super(AddrInfoCursorLibrary.class, AddrInfoCursorImpl.class, false, false, 0);
}
@Override
protected AddrInfoCursorLibrary createUncached(Object receiver) {
assert receiver instanceof AddrInfoCursorImpl;
AddrInfoCursorLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected AddrInfoCursorLibrary createCached(Object receiver) {
assert receiver instanceof AddrInfoCursorImpl;
return new Cached(receiver);
}
@GeneratedBy(AddrInfoCursorImpl.class)
protected static class Cached extends AddrInfoCursorLibrary {
private final Class extends AddrInfoCursorImpl> receiverClass_;
/**
* State Info:
* 0: SpecializationActive {@link AddrInfoCursorImpl#release(AddrInfoCursorImpl, InvokeNativeFunction)}
* 1: SpecializationActive {@link AddrInfoCursorImpl#next(AddrInfoCursorImpl, InvokeNativeFunction)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link AddrInfoCursorImpl#release}
* Parameter: {@link InvokeNativeFunction} invokeNode
*/
@Child private InvokeNativeFunction invoke;
protected Cached(Object receiver) {
AddrInfoCursorImpl castReceiver = ((AddrInfoCursorImpl) receiver) ;
this.receiverClass_ = castReceiver.getClass();
}
@Override
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
/**
* Debug Info:
* Specialization {@link AddrInfoCursorImpl#release(AddrInfoCursorImpl, InvokeNativeFunction)}
* Activation probability: 0.50000
* With/without class size: 10/0 bytes
*
*/
@Override
public void release(AddrInfoCursor arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
AddrInfoCursorImpl arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[AddrInfoCursorImpl.release(AddrInfoCursorImpl, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.release(invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
releaseNode_AndSpecialize(arg0Value);
return;
}
private void releaseNode_AndSpecialize(AddrInfoCursorImpl arg0Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'release(AddrInfoCursorImpl, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[AddrInfoCursorImpl.release(AddrInfoCursorImpl, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.release(invoke_);
return;
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
/**
* Debug Info:
* Specialization {@link AddrInfoCursorImpl#next(AddrInfoCursorImpl, InvokeNativeFunction)}
* Activation probability: 0.50000
* With/without class size: 10/0 bytes
*
*/
@Override
public boolean next(AddrInfoCursor arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
AddrInfoCursorImpl arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[AddrInfoCursorImpl.next(AddrInfoCursorImpl, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.next(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return nextNode_AndSpecialize(arg0Value);
}
private boolean nextNode_AndSpecialize(AddrInfoCursorImpl arg0Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'next(AddrInfoCursorImpl, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[AddrInfoCursorImpl.next(AddrInfoCursorImpl, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.next(invoke_);
}
@Override
public int getFlags(AddrInfoCursor receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getFlags();
}
@Override
public int getFamily(AddrInfoCursor receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getFamily();
}
@Override
public int getSockType(AddrInfoCursor receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getSockType();
}
@Override
public int getProtocol(AddrInfoCursor receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getProtocol();
}
@Override
public Object getCanonName(AddrInfoCursor receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getCanonName();
}
@Override
public UniversalSockAddr getSockAddr(AddrInfoCursor receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getSockAddr();
}
}
@GeneratedBy(AddrInfoCursorImpl.class)
protected static class Uncached extends AddrInfoCursorLibrary {
private final Class extends AddrInfoCursorImpl> receiverClass_;
protected Uncached(Object receiver) {
this.receiverClass_ = ((AddrInfoCursorImpl) receiver).getClass();
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
@Override
public final boolean isAdoptable() {
return false;
}
@Override
public final NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public void release(AddrInfoCursor arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
AddrInfoCursorImpl arg0Value = ((AddrInfoCursorImpl) arg0Value_);
arg0Value.release((InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean next(AddrInfoCursor arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
AddrInfoCursorImpl arg0Value = ((AddrInfoCursorImpl) arg0Value_);
return arg0Value.next((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int getFlags(AddrInfoCursor receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((AddrInfoCursorImpl) receiver) .getFlags();
}
@TruffleBoundary
@Override
public int getFamily(AddrInfoCursor receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((AddrInfoCursorImpl) receiver) .getFamily();
}
@TruffleBoundary
@Override
public int getSockType(AddrInfoCursor receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((AddrInfoCursorImpl) receiver) .getSockType();
}
@TruffleBoundary
@Override
public int getProtocol(AddrInfoCursor receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((AddrInfoCursorImpl) receiver) .getProtocol();
}
@TruffleBoundary
@Override
public Object getCanonName(AddrInfoCursor receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((AddrInfoCursorImpl) receiver) .getCanonName();
}
@TruffleBoundary
@Override
public UniversalSockAddr getSockAddr(AddrInfoCursor receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((AddrInfoCursorImpl) receiver) .getSockAddr();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy