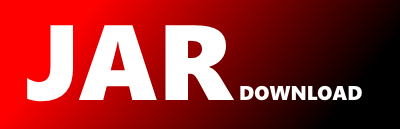
com.oracle.graal.python.runtime.PythonContextFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.graal.python.runtime.PythonContext.GetThreadStateNode;
import com.oracle.graal.python.runtime.PythonContext.PythonThreadState;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
@GeneratedBy(PythonContext.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PythonContextFactory {
/**
* Debug Info:
* Specialization {@link GetThreadStateNode#doNoShutdown}
* Activation probability: 0.38500
* With/without class size: 8/0 bytes
* Specialization {@link GetThreadStateNode#doGeneric}
* Activation probability: 0.29500
* With/without class size: 7/0 bytes
* Specialization {@link GetThreadStateNode#doNoShutdownWithContext}
* Activation probability: 0.20500
* With/without class size: 6/0 bytes
* Specialization {@link GetThreadStateNode#doGenericWithContext}
* Activation probability: 0.11500
* With/without class size: 5/0 bytes
*
*/
@GeneratedBy(GetThreadStateNode.class)
@SuppressWarnings("javadoc")
public static final class GetThreadStateNodeGen extends GetThreadStateNode {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link GetThreadStateNode#doNoShutdown}
* 1: SpecializationActive {@link GetThreadStateNode#doGeneric}
* 2: SpecializationActive {@link GetThreadStateNode#doNoShutdownWithContext}
* 3: SpecializationActive {@link GetThreadStateNode#doGenericWithContext}
*
*/
@CompilationFinal private int state_0_;
private GetThreadStateNodeGen() {
}
@Override
public PythonThreadState execute(Node arg0Value, PythonContext arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] || SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] || SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] || SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] */) {
if ((arg1Value == null)) {
PythonThreadState curThreadState__ = (getThreadState());
if ((!(curThreadState__.isShuttingDown()))) {
return GetThreadStateNode.doNoShutdown(arg1Value, curThreadState__);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] */) {
if ((arg1Value == null)) {
return doGeneric(arg1Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] */) {
{
PythonThreadState curThreadState__1 = (getThreadState());
if ((!(curThreadState__1.isShuttingDown()))) {
return GetThreadStateNode.doNoShutdownWithContext(arg1Value, curThreadState__1);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */) {
return doGenericWithContext(arg1Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private PythonThreadState executeAndSpecialize(Node arg0Value, PythonContext arg1Value) {
int state_0 = this.state_0_;
{
PythonThreadState curThreadState__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] */) {
if ((arg1Value == null)) {
curThreadState__ = (getThreadState());
if ((!(curThreadState__.isShuttingDown()))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] */;
this.state_0_ = state_0;
return GetThreadStateNode.doNoShutdown(arg1Value, curThreadState__);
}
}
}
}
if ((arg1Value == null)) {
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] */;
this.state_0_ = state_0;
return doGeneric(arg1Value);
}
{
PythonThreadState curThreadState__1 = null;
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */) {
{
curThreadState__1 = (getThreadState());
if ((!(curThreadState__1.isShuttingDown()))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] */;
this.state_0_ = state_0;
return GetThreadStateNode.doNoShutdownWithContext(arg1Value, curThreadState__1);
}
}
}
}
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */;
this.state_0_ = state_0;
return doGenericWithContext(arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static GetThreadStateNode create() {
return new GetThreadStateNodeGen();
}
@NeverDefault
public static GetThreadStateNode getUncached() {
return GetThreadStateNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static GetThreadStateNode inline(@RequiredField(bits = 4, value = StateField.class) InlineTarget target) {
return new GetThreadStateNodeGen.Inlined(target);
}
@GeneratedBy(GetThreadStateNode.class)
@DenyReplace
private static final class Inlined extends GetThreadStateNode {
/**
* State Info:
* 0: SpecializationActive {@link GetThreadStateNode#doNoShutdown}
* 1: SpecializationActive {@link GetThreadStateNode#doGeneric}
* 2: SpecializationActive {@link GetThreadStateNode#doNoShutdownWithContext}
* 3: SpecializationActive {@link GetThreadStateNode#doGenericWithContext}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(GetThreadStateNode.class);
this.state_0_ = target.getState(0, 4);
}
@Override
public PythonThreadState execute(Node arg0Value, PythonContext arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] || SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] || SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] || SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] */) {
if ((arg1Value == null)) {
PythonThreadState curThreadState__ = (getThreadState());
if ((!(curThreadState__.isShuttingDown()))) {
return GetThreadStateNode.doNoShutdown(arg1Value, curThreadState__);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] */) {
if ((arg1Value == null)) {
return doGeneric(arg1Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] */) {
{
PythonThreadState curThreadState__1 = (getThreadState());
if ((!(curThreadState__1.isShuttingDown()))) {
return GetThreadStateNode.doNoShutdownWithContext(arg1Value, curThreadState__1);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */) {
return doGenericWithContext(arg1Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private PythonThreadState executeAndSpecialize(Node arg0Value, PythonContext arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
{
PythonThreadState curThreadState__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] */) {
if ((arg1Value == null)) {
curThreadState__ = (getThreadState());
if ((!(curThreadState__.isShuttingDown()))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] */;
this.state_0_.set(arg0Value, state_0);
return GetThreadStateNode.doNoShutdown(arg1Value, curThreadState__);
}
}
}
}
if ((arg1Value == null)) {
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdown(PythonContext, PythonThreadState)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PythonContext.GetThreadStateNode.doGeneric(PythonContext)] */;
this.state_0_.set(arg0Value, state_0);
return doGeneric(arg1Value);
}
{
PythonThreadState curThreadState__1 = null;
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */) {
{
curThreadState__1 = (getThreadState());
if ((!(curThreadState__1.isShuttingDown()))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] */;
this.state_0_.set(arg0Value, state_0);
return GetThreadStateNode.doNoShutdownWithContext(arg1Value, curThreadState__1);
}
}
}
}
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[PythonContext.GetThreadStateNode.doNoShutdownWithContext(PythonContext, PythonThreadState)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PythonContext.GetThreadStateNode.doGenericWithContext(PythonContext)] */;
this.state_0_.set(arg0Value, state_0);
return doGenericWithContext(arg1Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(GetThreadStateNode.class)
@DenyReplace
private static final class Uncached extends GetThreadStateNode {
@TruffleBoundary
@Override
public PythonThreadState execute(Node arg0Value, PythonContext arg1Value) {
if ((arg1Value == null)) {
return doGeneric(arg1Value);
}
return doGenericWithContext(arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy