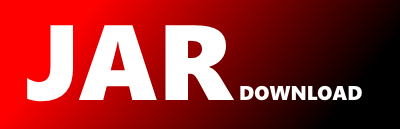
com.oracle.graal.python.runtime.UniversalSockAddrImplGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.graal.python.runtime.NFIPosixSupport.InvokeNativeFunction;
import com.oracle.graal.python.runtime.NFIPosixSupport.UniversalSockAddrImpl;
import com.oracle.graal.python.runtime.PosixSupportLibrary.Inet4SockAddr;
import com.oracle.graal.python.runtime.PosixSupportLibrary.Inet6SockAddr;
import com.oracle.graal.python.runtime.PosixSupportLibrary.UniversalSockAddr;
import com.oracle.graal.python.runtime.PosixSupportLibrary.UniversalSockAddrLibrary;
import com.oracle.graal.python.runtime.PosixSupportLibrary.UnixSockAddr;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.VarHandle;
@GeneratedBy(UniversalSockAddrImpl.class)
@SuppressWarnings("javadoc")
final class UniversalSockAddrImplGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(UniversalSockAddrImpl.class, new UniversalSockAddrLibraryExports());
}
private UniversalSockAddrImplGen() {
}
@GeneratedBy(UniversalSockAddrImpl.class)
protected static class UniversalSockAddrLibraryExports extends LibraryExport {
private UniversalSockAddrLibraryExports() {
super(UniversalSockAddrLibrary.class, UniversalSockAddrImpl.class, false, false, 0);
}
@Override
protected UniversalSockAddrLibrary createUncached(Object receiver) {
assert receiver instanceof UniversalSockAddrImpl;
UniversalSockAddrLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected UniversalSockAddrLibrary createCached(Object receiver) {
assert receiver instanceof UniversalSockAddrImpl;
return new Cached(receiver);
}
@GeneratedBy(UniversalSockAddrImpl.class)
protected static class Cached extends UniversalSockAddrLibrary {
private final Class extends UniversalSockAddrImpl> receiverClass_;
/**
* State Info:
* 0: SpecializationActive {@link UniversalSockAddrImpl#asInet4SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)}
* 1: SpecializationActive {@link UniversalSockAddrImpl#asInet6SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)}
* 2: SpecializationActive {@link UniversalSockAddrImpl#asUnixSockAddr(UniversalSockAddrImpl, InvokeNativeFunction)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link UniversalSockAddrImpl#asInet4SockAddr}
* Parameter: {@link InvokeNativeFunction} invokeNode
*/
@Child private InvokeNativeFunction invoke;
protected Cached(Object receiver) {
UniversalSockAddrImpl castReceiver = ((UniversalSockAddrImpl) receiver) ;
this.receiverClass_ = castReceiver.getClass();
}
@Override
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
@Override
public int getFamily(UniversalSockAddr receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getFamily();
}
/**
* Debug Info:
* Specialization {@link UniversalSockAddrImpl#asInet4SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
*
*/
@Override
public Inet4SockAddr asInet4SockAddr(UniversalSockAddr arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
UniversalSockAddrImpl arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[UniversalSockAddrImpl.asInet4SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.asInet4SockAddr(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asInet4SockAddrNode_AndSpecialize(arg0Value);
}
private Inet4SockAddr asInet4SockAddrNode_AndSpecialize(UniversalSockAddrImpl arg0Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'asInet4SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[UniversalSockAddrImpl.asInet4SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.asInet4SockAddr(invoke_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
/**
* Debug Info:
* Specialization {@link UniversalSockAddrImpl#asInet6SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
*
*/
@Override
public Inet6SockAddr asInet6SockAddr(UniversalSockAddr arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
UniversalSockAddrImpl arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[UniversalSockAddrImpl.asInet6SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.asInet6SockAddr(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asInet6SockAddrNode_AndSpecialize(arg0Value);
}
private Inet6SockAddr asInet6SockAddrNode_AndSpecialize(UniversalSockAddrImpl arg0Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'asInet6SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[UniversalSockAddrImpl.asInet6SockAddr(UniversalSockAddrImpl, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.asInet6SockAddr(invoke_);
}
/**
* Debug Info:
* Specialization {@link UniversalSockAddrImpl#asUnixSockAddr(UniversalSockAddrImpl, InvokeNativeFunction)}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
*
*/
@Override
public UnixSockAddr asUnixSockAddr(UniversalSockAddr arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
UniversalSockAddrImpl arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[UniversalSockAddrImpl.asUnixSockAddr(UniversalSockAddrImpl, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.asUnixSockAddr(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asUnixSockAddrNode_AndSpecialize(arg0Value);
}
private UnixSockAddr asUnixSockAddrNode_AndSpecialize(UniversalSockAddrImpl arg0Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'asUnixSockAddr(UniversalSockAddrImpl, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[UniversalSockAddrImpl.asUnixSockAddr(UniversalSockAddrImpl, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.asUnixSockAddr(invoke_);
}
}
@GeneratedBy(UniversalSockAddrImpl.class)
protected static class Uncached extends UniversalSockAddrLibrary {
private final Class extends UniversalSockAddrImpl> receiverClass_;
protected Uncached(Object receiver) {
this.receiverClass_ = ((UniversalSockAddrImpl) receiver).getClass();
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
@Override
public final boolean isAdoptable() {
return false;
}
@Override
public final NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public int getFamily(UniversalSockAddr receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UniversalSockAddrImpl) receiver) .getFamily();
}
@TruffleBoundary
@Override
public Inet4SockAddr asInet4SockAddr(UniversalSockAddr arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
UniversalSockAddrImpl arg0Value = ((UniversalSockAddrImpl) arg0Value_);
return arg0Value.asInet4SockAddr((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Inet6SockAddr asInet6SockAddr(UniversalSockAddr arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
UniversalSockAddrImpl arg0Value = ((UniversalSockAddrImpl) arg0Value_);
return arg0Value.asInet6SockAddr((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public UnixSockAddr asUnixSockAddr(UniversalSockAddr arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
UniversalSockAddrImpl arg0Value = ((UniversalSockAddrImpl) arg0Value_);
return arg0Value.asUnixSockAddr((InvokeNativeFunction.getUncached()));
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy