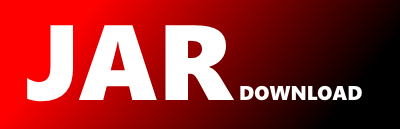
com.oracle.graal.python.util.CharsetMappingFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.util;
import com.oracle.graal.python.util.CharsetMapping.NormalizeEncodingNameNode;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.CreateCodePointIteratorNode;
import com.oracle.truffle.api.strings.TruffleStringBuilder.AppendCodePointNode;
import com.oracle.truffle.api.strings.TruffleStringBuilder.ToStringNode;
import com.oracle.truffle.api.strings.TruffleStringIterator.NextNode;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(CharsetMapping.class)
@SuppressWarnings("javadoc")
public final class CharsetMappingFactory {
/**
* Debug Info:
* Specialization {@link NormalizeEncodingNameNode#normalize}
* Activation probability: 1.00000
* With/without class size: 36/16 bytes
*
*/
@GeneratedBy(NormalizeEncodingNameNode.class)
@SuppressWarnings("javadoc")
public static final class NormalizeEncodingNameNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static NormalizeEncodingNameNode getUncached() {
return NormalizeEncodingNameNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#createCodePointIteratorNode_}
*
- {@link Inlined#nextNode_}
*
- {@link Inlined#appendCodePointNode_}
*
- {@link Inlined#toStringNode_}
*
*/
@NeverDefault
public static NormalizeEncodingNameNode inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new NormalizeEncodingNameNodeGen.Inlined(target);
}
@GeneratedBy(NormalizeEncodingNameNode.class)
@DenyReplace
private static final class Inlined extends NormalizeEncodingNameNode {
/**
* State Info:
* 0: SpecializationActive {@link NormalizeEncodingNameNode#normalize}
*
*/
private final StateField state_0_;
private final ReferenceField createCodePointIteratorNode_;
private final ReferenceField nextNode_;
private final ReferenceField appendCodePointNode_;
private final ReferenceField toStringNode_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(NormalizeEncodingNameNode.class);
this.state_0_ = target.getState(0, 1);
this.createCodePointIteratorNode_ = target.getReference(1, CreateCodePointIteratorNode.class);
this.nextNode_ = target.getReference(2, NextNode.class);
this.appendCodePointNode_ = target.getReference(3, AppendCodePointNode.class);
this.toStringNode_ = target.getReference(4, ToStringNode.class);
}
@Override
public TruffleString execute(Node arg0Value, TruffleString arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[CharsetMapping.NormalizeEncodingNameNode.normalize(TruffleString, CreateCodePointIteratorNode, NextNode, AppendCodePointNode, ToStringNode)] */) {
{
CreateCodePointIteratorNode createCodePointIteratorNode__ = this.createCodePointIteratorNode_.get(arg0Value);
if (createCodePointIteratorNode__ != null) {
NextNode nextNode__ = this.nextNode_.get(arg0Value);
if (nextNode__ != null) {
AppendCodePointNode appendCodePointNode__ = this.appendCodePointNode_.get(arg0Value);
if (appendCodePointNode__ != null) {
ToStringNode toStringNode__ = this.toStringNode_.get(arg0Value);
if (toStringNode__ != null) {
return NormalizeEncodingNameNode.normalize(arg1Value, createCodePointIteratorNode__, nextNode__, appendCodePointNode__, toStringNode__);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private TruffleString executeAndSpecialize(Node arg0Value, TruffleString arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
CreateCodePointIteratorNode createCodePointIteratorNode__ = arg0Value.insert((CreateCodePointIteratorNode.create()));
Objects.requireNonNull(createCodePointIteratorNode__, "Specialization 'normalize(TruffleString, CreateCodePointIteratorNode, NextNode, AppendCodePointNode, ToStringNode)' cache 'createCodePointIteratorNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.createCodePointIteratorNode_.set(arg0Value, createCodePointIteratorNode__);
NextNode nextNode__ = arg0Value.insert((NextNode.create()));
Objects.requireNonNull(nextNode__, "Specialization 'normalize(TruffleString, CreateCodePointIteratorNode, NextNode, AppendCodePointNode, ToStringNode)' cache 'nextNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.nextNode_.set(arg0Value, nextNode__);
AppendCodePointNode appendCodePointNode__ = arg0Value.insert((AppendCodePointNode.create()));
Objects.requireNonNull(appendCodePointNode__, "Specialization 'normalize(TruffleString, CreateCodePointIteratorNode, NextNode, AppendCodePointNode, ToStringNode)' cache 'appendCodePointNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.appendCodePointNode_.set(arg0Value, appendCodePointNode__);
ToStringNode toStringNode__ = arg0Value.insert((ToStringNode.create()));
Objects.requireNonNull(toStringNode__, "Specialization 'normalize(TruffleString, CreateCodePointIteratorNode, NextNode, AppendCodePointNode, ToStringNode)' cache 'toStringNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.toStringNode_.set(arg0Value, toStringNode__);
state_0 = state_0 | 0b1 /* add SpecializationActive[CharsetMapping.NormalizeEncodingNameNode.normalize(TruffleString, CreateCodePointIteratorNode, NextNode, AppendCodePointNode, ToStringNode)] */;
this.state_0_.set(arg0Value, state_0);
return NormalizeEncodingNameNode.normalize(arg1Value, createCodePointIteratorNode__, nextNode__, appendCodePointNode__, toStringNode__);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(NormalizeEncodingNameNode.class)
@DenyReplace
private static final class Uncached extends NormalizeEncodingNameNode {
@TruffleBoundary
@Override
public TruffleString execute(Node arg0Value, TruffleString arg1Value) {
return NormalizeEncodingNameNode.normalize(arg1Value, (CreateCodePointIteratorNode.getUncached()), (NextNode.getUncached()), (AppendCodePointNode.getUncached()), (ToStringNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy