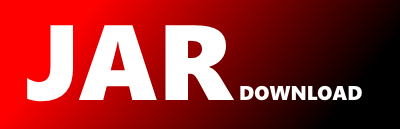
com.oracle.graal.python.lib.PyObjectGetMethodNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.attributes.LookupAttributeInMRONode;
import com.oracle.graal.python.nodes.attributes.LookupCallableSlotInMRONode;
import com.oracle.graal.python.nodes.attributes.ReadAttributeFromObjectNode;
import com.oracle.graal.python.nodes.attributes.LookupAttributeInMRONode.Dynamic;
import com.oracle.graal.python.nodes.call.special.CallTernaryMethodNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.nodes.object.IsForeignObjectNode;
import com.oracle.graal.python.nodes.object.IsForeignObjectNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.ToJavaStringNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectGetMethod#getFixedAttr}
* Activation probability: 0.32000
* With/without class size: 18/25 bytes
* Specialization {@link PyObjectGetMethod#getDynamicAttr}
* Activation probability: 0.26000
* With/without class size: 14/21 bytes
* Specialization {@link PyObjectGetMethod#getForeignMethod}
* Activation probability: 0.20000
* With/without class size: 11/18 bytes
* Specialization {@link PyObjectGetMethod#getForeignMethod}
* Activation probability: 0.14000
* With/without class size: 8/14 bytes
* Specialization {@link PyObjectGetMethod#getGenericAttr}
* Activation probability: 0.08000
* With/without class size: 6/9 bytes
*
*/
@GeneratedBy(PyObjectGetMethod.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyObjectGetMethodNodeGen extends PyObjectGetMethod {
private static final StateField GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER = StateField.create(GetFixedAttrData.lookup_(), "getFixedAttr_state_0_");
private static final StateField GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_1_UPDATER = StateField.create(GetFixedAttrData.lookup_(), "getFixedAttr_state_1_");
private static final StateField GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_0_UPDATER = StateField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_state_0_");
private static final StateField GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_1_UPDATER = StateField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_state_1_");
private static final StateField GET_FOREIGN_METHOD0__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD0_STATE_0_UPDATER = StateField.create(GetForeignMethod0Data.lookup_(), "getForeignMethod0_state_0_");
private static final StateField GET_FOREIGN_METHOD1__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD1_STATE_0_UPDATER = StateField.create(GetForeignMethod1Data.lookup_(), "getForeignMethod1_state_0_");
private static final StateField FALLBACK__PY_OBJECT_GET_METHOD_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
static final ReferenceField GET_FIXED_ATTR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getFixedAttr_cache", GetFixedAttrData.class);
static final ReferenceField GET_DYNAMIC_ATTR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getDynamicAttr_cache", GetDynamicAttrData.class);
static final ReferenceField GET_FOREIGN_METHOD0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getForeignMethod0_cache", GetForeignMethod0Data.class);
static final ReferenceField GET_FOREIGN_METHOD1_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "getForeignMethod1_cache", GetForeignMethod1Data.class);
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_FIXED_ATTR_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GetFixedAttrData.lookup_(), "getFixedAttr_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_FIXED_ATTR_GET_DESCR_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(GetFixedAttrData.lookup_(), "getFixedAttr_getDescrClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_GET_FIXED_ATTR_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(GetFixedAttrData.lookup_(), "getFixedAttr_raiseNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} hasDescr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_GET_FIXED_ATTR_HAS_DESCR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(18, 1)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnDataDescr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_GET_FIXED_ATTR_RETURN_DATA_DESCR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(19, 1)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnAttr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_GET_FIXED_ATTR_RETURN_ATTR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(20, 1)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnUnboundMethod
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_GET_FIXED_ATTR_RETURN_UNBOUND_METHOD_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(21, 1)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnBoundDescr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_GET_FIXED_ATTR_RETURN_BOUND_DESCR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(22, 1)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_DYNAMIC_ATTR_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_0_UPDATER.subUpdater(2, 17), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_DYNAMIC_ATTR_GET_DESCR_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_getDescrClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_GET_DYNAMIC_ATTR_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_0_UPDATER.subUpdater(19, 1), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_raiseNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private static final IsForeignObjectNode INLINED_GET_FOREIGN_METHOD0_IS_FOREIGN_OBJECT_NODE_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, GET_FOREIGN_METHOD0__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD0_STATE_0_UPDATER.subUpdater(0, 8)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*/
private static final PyObjectGetAttr INLINED_GET_FOREIGN_METHOD0_GET_ATTR_ = PyObjectGetAttrNodeGen.inline(InlineTarget.create(PyObjectGetAttr.class, GET_FOREIGN_METHOD0__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD0_STATE_0_UPDATER.subUpdater(8, 2), ReferenceField.create(GetForeignMethod0Data.lookup_(), "getForeignMethod0_getAttr__field1_", Node.class), ReferenceField.create(GetForeignMethod0Data.lookup_(), "getForeignMethod0_getAttr__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private static final IsForeignObjectNode INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, GET_FOREIGN_METHOD1__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD1_STATE_0_UPDATER.subUpdater(2, 8)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*/
private static final PyObjectGetAttr INLINED_GET_FOREIGN_METHOD1_GET_ATTR_ = PyObjectGetAttrNodeGen.inline(InlineTarget.create(PyObjectGetAttr.class, GET_FOREIGN_METHOD1__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD1_STATE_0_UPDATER.subUpdater(10, 2), ReferenceField.create(GetForeignMethod1Data.lookup_(), "getForeignMethod1_getAttr__field1_", Node.class), ReferenceField.create(GetForeignMethod1Data.lookup_(), "getForeignMethod1_getAttr__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getGenericAttr}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*/
private static final PyObjectGetAttr INLINED_FALLBACK_GET_ATTR_ = PyObjectGetAttrNodeGen.inline(InlineTarget.create(PyObjectGetAttr.class, FALLBACK__PY_OBJECT_GET_METHOD_FALLBACK_STATE_0_UPDATER.subUpdater(0, 2), ReferenceField.create(FallbackData.lookup_(), "fallback_getAttr__field1_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_getAttr__field2_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link PyObjectGetMethod#getFixedAttr}
* 1: SpecializationActive {@link PyObjectGetMethod#getDynamicAttr}
* 2: SpecializationActive {@link PyObjectGetMethod#getForeignMethod}
* 3: SpecializationActive {@link PyObjectGetMethod#getForeignMethod}
* 4: SpecializationActive {@link PyObjectGetMethod#getGenericAttr}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link LookupCallableSlotInMRONode} lookupGet
*/
@Child private LookupCallableSlotInMRONode lookupGet;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link LookupCallableSlotInMRONode} lookupSet
*/
@Child private LookupCallableSlotInMRONode lookupSet;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link CallTernaryMethodNode} callGet
*/
@Child private CallTernaryMethodNode callGet;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link ReadAttributeFromObjectNode} readAttr
*/
@Child private ReadAttributeFromObjectNode readAttr;
@UnsafeAccessedField @Child private GetFixedAttrData getFixedAttr_cache;
@UnsafeAccessedField @Child private GetDynamicAttrData getDynamicAttr_cache;
@UnsafeAccessedField @Child private GetForeignMethod0Data getForeignMethod0_cache;
@UnsafeAccessedField @Child private GetForeignMethod1Data getForeignMethod1_cache;
@Child private FallbackData fallback_cache;
private PyObjectGetMethodNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
{
GetDynamicAttrData s1_ = this.getDynamicAttr_cache;
if ((s1_ == null || ((s1_.getDynamicAttr_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy), guardIndex=0] */ || (PyObjectGetMethod.isObjectGetAttribute((INLINED_GET_DYNAMIC_ATTR_GET_CLASS_.execute(s1_, arg1Value)))))) {
return false;
}
}
{
GetForeignMethod1Data s3_ = this.getForeignMethod1_cache;
if ((s3_ == null || ((s3_.getForeignMethod1_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr), guardIndex=0] */ || (INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_.execute(s3_, arg1Value)))) {
return false;
}
}
return true;
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] || SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] || SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] || SpecializationActive[PyObjectGetMethod.getGenericAttr(Frame, Node, Object, TruffleString, PyObjectGetAttr)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */) {
GetFixedAttrData s0_ = this.getFixedAttr_cache;
if (s0_ != null) {
{
LookupCallableSlotInMRONode lookupGet_ = this.lookupGet;
if (lookupGet_ != null) {
LookupCallableSlotInMRONode lookupSet_ = this.lookupSet;
if (lookupSet_ != null) {
CallTernaryMethodNode callGet_ = this.callGet;
if (callGet_ != null) {
ReadAttributeFromObjectNode readAttr_ = this.readAttr;
if (readAttr_ != null) {
Object lazyClass__ = (INLINED_GET_FIXED_ATTR_GET_CLASS_.execute(s0_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__)) && (arg2Value == s0_.cachedName_)) {
return PyObjectGetMethod.getFixedAttr((VirtualFrame) frameValue, s0_, arg1Value, arg2Value, INLINED_GET_FIXED_ATTR_GET_CLASS_, lazyClass__, s0_.cachedName_, s0_.lookupNode_, INLINED_GET_FIXED_ATTR_GET_DESCR_CLASS_, lookupGet_, lookupSet_, callGet_, readAttr_, INLINED_GET_FIXED_ATTR_RAISE_NODE_, INLINED_GET_FIXED_ATTR_HAS_DESCR_, INLINED_GET_FIXED_ATTR_RETURN_DATA_DESCR_, INLINED_GET_FIXED_ATTR_RETURN_ATTR_, INLINED_GET_FIXED_ATTR_RETURN_UNBOUND_METHOD_, INLINED_GET_FIXED_ATTR_RETURN_BOUND_DESCR_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] */) {
GetDynamicAttrData s1_ = this.getDynamicAttr_cache;
if (s1_ != null) {
if ((s1_.getDynamicAttr_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
LookupCallableSlotInMRONode lookupGet_1 = this.lookupGet;
if (lookupGet_1 != null) {
LookupCallableSlotInMRONode lookupSet_1 = this.lookupSet;
if (lookupSet_1 != null) {
CallTernaryMethodNode callGet_1 = this.callGet;
if (callGet_1 != null) {
ReadAttributeFromObjectNode readAttr_1 = this.readAttr;
if (readAttr_1 != null) {
Object lazyClass__1 = (INLINED_GET_DYNAMIC_ATTR_GET_CLASS_.execute(s1_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__1))) {
return PyObjectGetMethod.getDynamicAttr(frameValue, s1_, arg1Value, arg2Value, INLINED_GET_DYNAMIC_ATTR_GET_CLASS_, lazyClass__1, s1_.lookupNode_, INLINED_GET_DYNAMIC_ATTR_GET_DESCR_CLASS_, lookupGet_1, lookupSet_1, callGet_1, readAttr_1, INLINED_GET_DYNAMIC_ATTR_RAISE_NODE_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */) {
GetForeignMethod0Data s2_ = this.getForeignMethod0_cache;
if (s2_ != null) {
if ((s2_.lib_.accepts(arg1Value)) && (INLINED_GET_FOREIGN_METHOD0_IS_FOREIGN_OBJECT_NODE_.execute(s2_, arg1Value))) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s2_, arg1Value, arg2Value, INLINED_GET_FOREIGN_METHOD0_IS_FOREIGN_OBJECT_NODE_, s2_.toJavaString_, s2_.lib_, INLINED_GET_FOREIGN_METHOD0_GET_ATTR_);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */) {
GetForeignMethod1Data s3_ = this.getForeignMethod1_cache;
if (s3_ != null) {
if ((s3_.getForeignMethod1_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */ && (INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_.execute(s3_, arg1Value))) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary lib__ = (INTEROP_LIBRARY_.getUncached());
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_, s3_.toJavaString_, lib__, INLINED_GET_FOREIGN_METHOD1_GET_ATTR_);
}
} finally {
encapsulating_.set(prev_);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyObjectGetMethod.getGenericAttr(Frame, Node, Object, TruffleString, PyObjectGetAttr)] */) {
FallbackData s4_ = this.fallback_cache;
if (s4_ != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value, arg2Value)) {
return PyObjectGetMethod.getGenericAttr(frameValue, s4_, arg1Value, arg2Value, INLINED_FALLBACK_GET_ATTR_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
int state_0 = this.state_0_;
{
Object lazyClass__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] */) {
while (true) {
int count0_ = 0;
GetFixedAttrData s0_ = GET_FIXED_ATTR_CACHE_UPDATER.getVolatile(this);
GetFixedAttrData s0_original = s0_;
while (s0_ != null) {
{
LookupCallableSlotInMRONode lookupGet_ = this.lookupGet;
if (lookupGet_ != null) {
LookupCallableSlotInMRONode lookupSet_ = this.lookupSet;
if (lookupSet_ != null) {
CallTernaryMethodNode callGet_ = this.callGet;
if (callGet_ != null) {
ReadAttributeFromObjectNode readAttr_ = this.readAttr;
if (readAttr_ != null) {
lazyClass__ = (INLINED_GET_FIXED_ATTR_GET_CLASS_.execute(s0_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__)) && (arg2Value == s0_.cachedName_)) {
break;
}
}
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
s0_ = this.insert(new GetFixedAttrData());
lazyClass__ = (INLINED_GET_FIXED_ATTR_GET_CLASS_.execute(s0_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__))) {
// assert (arg2Value == s0_.cachedName_);
s0_.cachedName_ = (arg2Value);
LookupAttributeInMRONode lookupNode__ = s0_.insert((LookupAttributeInMRONode.create(arg2Value)));
Objects.requireNonNull(lookupNode__, "Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' cache 'lookupNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.lookupNode_ = lookupNode__;
LookupCallableSlotInMRONode lookupGet_;
LookupCallableSlotInMRONode lookupGet__shared = this.lookupGet;
if (lookupGet__shared != null) {
lookupGet_ = lookupGet__shared;
} else {
lookupGet_ = s0_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Get)));
if (lookupGet_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'lookupGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet == null) {
this.lookupGet = lookupGet_;
}
LookupCallableSlotInMRONode lookupSet_;
LookupCallableSlotInMRONode lookupSet__shared = this.lookupSet;
if (lookupSet__shared != null) {
lookupSet_ = lookupSet__shared;
} else {
lookupSet_ = s0_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Set)));
if (lookupSet_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'lookupSet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupSet == null) {
this.lookupSet = lookupSet_;
}
CallTernaryMethodNode callGet_;
CallTernaryMethodNode callGet__shared = this.callGet;
if (callGet__shared != null) {
callGet_ = callGet__shared;
} else {
callGet_ = s0_.insert((CallTernaryMethodNode.create()));
if (callGet_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'callGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callGet == null) {
this.callGet = callGet_;
}
ReadAttributeFromObjectNode readAttr_;
ReadAttributeFromObjectNode readAttr__shared = this.readAttr;
if (readAttr__shared != null) {
readAttr_ = readAttr__shared;
} else {
readAttr_ = s0_.insert((ReadAttributeFromObjectNode.create()));
if (readAttr_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'readAttr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readAttr == null) {
this.readAttr = readAttr_;
}
if (!GET_FIXED_ATTR_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_ = state_0;
} else {
s0_ = null;
}
}
}
if (s0_ != null) {
return PyObjectGetMethod.getFixedAttr((VirtualFrame) frameValue, s0_, arg1Value, arg2Value, INLINED_GET_FIXED_ATTR_GET_CLASS_, lazyClass__, s0_.cachedName_, s0_.lookupNode_, INLINED_GET_FIXED_ATTR_GET_DESCR_CLASS_, this.lookupGet, this.lookupSet, this.callGet, this.readAttr, INLINED_GET_FIXED_ATTR_RAISE_NODE_, INLINED_GET_FIXED_ATTR_HAS_DESCR_, INLINED_GET_FIXED_ATTR_RETURN_DATA_DESCR_, INLINED_GET_FIXED_ATTR_RETURN_ATTR_, INLINED_GET_FIXED_ATTR_RETURN_UNBOUND_METHOD_, INLINED_GET_FIXED_ATTR_RETURN_BOUND_DESCR_);
}
break;
}
}
}
{
Object lazyClass__1 = null;
while (true) {
int count1_ = 0;
GetDynamicAttrData s1_ = GET_DYNAMIC_ATTR_CACHE_UPDATER.getVolatile(this);
GetDynamicAttrData s1_original = s1_;
while (s1_ != null) {
if ((s1_.getDynamicAttr_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
LookupCallableSlotInMRONode lookupGet_1 = this.lookupGet;
if (lookupGet_1 != null) {
LookupCallableSlotInMRONode lookupSet_1 = this.lookupSet;
if (lookupSet_1 != null) {
CallTernaryMethodNode callGet_1 = this.callGet;
if (callGet_1 != null) {
ReadAttributeFromObjectNode readAttr_1 = this.readAttr;
if (readAttr_1 != null) {
lazyClass__1 = (INLINED_GET_DYNAMIC_ATTR_GET_CLASS_.execute(s1_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__1))) {
break;
}
}
}
}
}
}
if ((s1_.getDynamicAttr_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count1_++;
}
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
{
s1_ = this.insert(new GetDynamicAttrData());
lazyClass__1 = (INLINED_GET_DYNAMIC_ATTR_GET_CLASS_.execute(s1_, arg1Value));
if (((s1_.getDynamicAttr_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy), guardIndex=0] */) {
s1_.getDynamicAttr_state_0_ = s1_.getDynamicAttr_state_0_ | 0b1 /* add GuardActive[specialization=PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy), guardIndex=0] */;
if (!GET_DYNAMIC_ATTR_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
s1_original = s1_;
s1_ = this.insert(new GetDynamicAttrData(s1_));
}
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__1))) {
Dynamic lookupNode__1 = s1_.insert((Dynamic.create()));
Objects.requireNonNull(lookupNode__1, "Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' cache 'lookupNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.lookupNode_ = lookupNode__1;
LookupCallableSlotInMRONode lookupGet_1;
LookupCallableSlotInMRONode lookupGet_1_shared = this.lookupGet;
if (lookupGet_1_shared != null) {
lookupGet_1 = lookupGet_1_shared;
} else {
lookupGet_1 = s1_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Get)));
if (lookupGet_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'lookupGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet == null) {
this.lookupGet = lookupGet_1;
}
LookupCallableSlotInMRONode lookupSet_1;
LookupCallableSlotInMRONode lookupSet_1_shared = this.lookupSet;
if (lookupSet_1_shared != null) {
lookupSet_1 = lookupSet_1_shared;
} else {
lookupSet_1 = s1_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Set)));
if (lookupSet_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'lookupSet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupSet == null) {
this.lookupSet = lookupSet_1;
}
CallTernaryMethodNode callGet_1;
CallTernaryMethodNode callGet_1_shared = this.callGet;
if (callGet_1_shared != null) {
callGet_1 = callGet_1_shared;
} else {
callGet_1 = s1_.insert((CallTernaryMethodNode.create()));
if (callGet_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'callGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callGet == null) {
this.callGet = callGet_1;
}
ReadAttributeFromObjectNode readAttr_1;
ReadAttributeFromObjectNode readAttr_1_shared = this.readAttr;
if (readAttr_1_shared != null) {
readAttr_1 = readAttr_1_shared;
} else {
readAttr_1 = s1_.insert((ReadAttributeFromObjectNode.create()));
if (readAttr_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'readAttr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readAttr == null) {
this.readAttr = readAttr_1;
}
s1_.getDynamicAttr_state_0_ = s1_.getDynamicAttr_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!GET_DYNAMIC_ATTR_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
this.getFixedAttr_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] */;
this.state_0_ = state_0;
} else {
s1_ = null;
}
}
}
if (s1_ != null) {
return PyObjectGetMethod.getDynamicAttr(frameValue, s1_, arg1Value, arg2Value, INLINED_GET_DYNAMIC_ATTR_GET_CLASS_, lazyClass__1, s1_.lookupNode_, INLINED_GET_DYNAMIC_ATTR_GET_DESCR_CLASS_, this.lookupGet, this.lookupSet, this.callGet, this.readAttr, INLINED_GET_DYNAMIC_ATTR_RAISE_NODE_);
}
break;
}
}
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */) {
while (true) {
int count2_ = 0;
GetForeignMethod0Data s2_ = GET_FOREIGN_METHOD0_CACHE_UPDATER.getVolatile(this);
GetForeignMethod0Data s2_original = s2_;
while (s2_ != null) {
if ((s2_.lib_.accepts(arg1Value)) && (INLINED_GET_FOREIGN_METHOD0_IS_FOREIGN_OBJECT_NODE_.execute(s2_, arg1Value))) {
break;
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
// assert (s2_.lib_.accepts(arg1Value));
{
s2_ = this.insert(new GetForeignMethod0Data());
if ((INLINED_GET_FOREIGN_METHOD0_IS_FOREIGN_OBJECT_NODE_.execute(s2_, arg1Value))) {
ToJavaStringNode toJavaString__ = s2_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaString__, "Specialization 'getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)' cache 'toJavaString' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.toJavaString_ = toJavaString__;
InteropLibrary lib__ = s2_.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(lib__, "Specialization 'getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)' cache 'lib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.lib_ = lib__;
if (!GET_FOREIGN_METHOD0_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */;
this.state_0_ = state_0;
} else {
s2_ = null;
}
}
}
if (s2_ != null) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s2_, arg1Value, arg2Value, INLINED_GET_FOREIGN_METHOD0_IS_FOREIGN_OBJECT_NODE_, s2_.toJavaString_, s2_.lib_, INLINED_GET_FOREIGN_METHOD0_GET_ATTR_);
}
break;
}
}
{
InteropLibrary lib__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
while (true) {
int count3_ = 0;
GetForeignMethod1Data s3_ = GET_FOREIGN_METHOD1_CACHE_UPDATER.getVolatile(this);
GetForeignMethod1Data s3_original = s3_;
while (s3_ != null) {
if ((s3_.getForeignMethod1_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */ && (INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_.execute(s3_, arg1Value))) {
lib__ = (INTEROP_LIBRARY_.getUncached());
break;
}
if ((s3_.getForeignMethod1_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count3_++;
}
s3_ = null;
break;
}
if (s3_ == null && count3_ < 1) {
{
s3_ = this.insert(new GetForeignMethod1Data());
if (((s3_.getForeignMethod1_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr), guardIndex=0] */) {
s3_.getForeignMethod1_state_0_ = s3_.getForeignMethod1_state_0_ | 0b1 /* add GuardActive[specialization=PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr), guardIndex=0] */;
if (!GET_FOREIGN_METHOD1_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
s3_original = s3_;
s3_ = this.insert(new GetForeignMethod1Data(s3_));
}
if ((INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_.execute(s3_, arg1Value))) {
ToJavaStringNode toJavaString__ = s3_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaString__, "Specialization 'getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)' cache 'toJavaString' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.toJavaString_ = toJavaString__;
lib__ = (INTEROP_LIBRARY_.getUncached());
s3_.getForeignMethod1_state_0_ = s3_.getForeignMethod1_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!GET_FOREIGN_METHOD1_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
this.getForeignMethod0_cache = null;
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */;
this.state_0_ = state_0;
} else {
s3_ = null;
}
}
}
if (s3_ != null) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, INLINED_GET_FOREIGN_METHOD1_IS_FOREIGN_OBJECT_NODE_, s3_.toJavaString_, lib__, INLINED_GET_FOREIGN_METHOD1_GET_ATTR_);
}
break;
}
} finally {
encapsulating_.set(prev_);
}
}
}
FallbackData s4_ = this.insert(new FallbackData());
VarHandle.storeStoreFence();
this.fallback_cache = s4_;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyObjectGetMethod.getGenericAttr(Frame, Node, Object, TruffleString, PyObjectGetAttr)] */;
this.state_0_ = state_0;
return PyObjectGetMethod.getGenericAttr(frameValue, s4_, arg1Value, arg2Value, INLINED_FALLBACK_GET_ATTR_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyObjectGetMethod create() {
return new PyObjectGetMethodNodeGen();
}
@NeverDefault
public static PyObjectGetMethod getUncached() {
return PyObjectGetMethodNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#lookupGet}
*
- {@link Inlined#lookupSet}
*
- {@link Inlined#callGet}
*
- {@link Inlined#readAttr}
*
- {@link Inlined#getFixedAttr_cache}
*
- {@link Inlined#getDynamicAttr_cache}
*
- {@link Inlined#getForeignMethod0_cache}
*
- {@link Inlined#getForeignMethod1_cache}
*
- {@link Inlined#fallback_cache}
*
*/
@NeverDefault
public static PyObjectGetMethod inline(@RequiredField(bits = 5, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectGetMethodNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class Inlined extends PyObjectGetMethod {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectGetMethod#getFixedAttr}
* 1: SpecializationActive {@link PyObjectGetMethod#getDynamicAttr}
* 2: SpecializationActive {@link PyObjectGetMethod#getForeignMethod}
* 3: SpecializationActive {@link PyObjectGetMethod#getForeignMethod}
* 4: SpecializationActive {@link PyObjectGetMethod#getGenericAttr}
*
*/
private final StateField state_0_;
private final ReferenceField lookupGet;
private final ReferenceField lookupSet;
private final ReferenceField callGet;
private final ReferenceField readAttr;
private final ReferenceField getFixedAttr_cache;
private final ReferenceField getDynamicAttr_cache;
private final ReferenceField getForeignMethod0_cache;
private final ReferenceField getForeignMethod1_cache;
private final ReferenceField fallback_cache;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getFixedAttr_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getFixedAttr_getDescrClass_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy getFixedAttr_raiseNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} hasDescr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile getFixedAttr_hasDescr_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnDataDescr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile getFixedAttr_returnDataDescr_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnAttr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile getFixedAttr_returnAttr_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnUnboundMethod
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile getFixedAttr_returnUnboundMethod_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnBoundDescr
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile getFixedAttr_returnBoundDescr_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getDynamicAttr_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getDynamicAttr_getDescrClass_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy getDynamicAttr_raiseNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private final IsForeignObjectNode getForeignMethod0_isForeignObjectNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*/
private final PyObjectGetAttr getForeignMethod0_getAttr_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private final IsForeignObjectNode getForeignMethod1_isForeignObjectNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*/
private final PyObjectGetAttr getForeignMethod1_getAttr_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getGenericAttr}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*/
private final PyObjectGetAttr fallback_getAttr_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectGetMethod.class);
this.state_0_ = target.getState(0, 5);
this.lookupGet = target.getReference(1, LookupCallableSlotInMRONode.class);
this.lookupSet = target.getReference(2, LookupCallableSlotInMRONode.class);
this.callGet = target.getReference(3, CallTernaryMethodNode.class);
this.readAttr = target.getReference(4, ReadAttributeFromObjectNode.class);
this.getFixedAttr_cache = target.getReference(5, GetFixedAttrData.class);
this.getDynamicAttr_cache = target.getReference(6, GetDynamicAttrData.class);
this.getForeignMethod0_cache = target.getReference(7, GetForeignMethod0Data.class);
this.getForeignMethod1_cache = target.getReference(8, GetForeignMethod1Data.class);
this.fallback_cache = target.getReference(9, FallbackData.class);
this.getFixedAttr_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GetFixedAttrData.lookup_(), "getFixedAttr_getClass__field1_", Node.class)));
this.getFixedAttr_getDescrClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(GetFixedAttrData.lookup_(), "getFixedAttr_getDescrClass__field1_", Node.class)));
this.getFixedAttr_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(GetFixedAttrData.lookup_(), "getFixedAttr_raiseNode__field1_", Node.class)));
this.getFixedAttr_hasDescr_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(18, 1)));
this.getFixedAttr_returnDataDescr_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(19, 1)));
this.getFixedAttr_returnAttr_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(20, 1)));
this.getFixedAttr_returnUnboundMethod_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(21, 1)));
this.getFixedAttr_returnBoundDescr_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, GET_FIXED_ATTR__PY_OBJECT_GET_METHOD_GET_FIXED_ATTR_STATE_0_UPDATER.subUpdater(22, 1)));
this.getDynamicAttr_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_0_UPDATER.subUpdater(2, 17), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_getClass__field1_", Node.class)));
this.getDynamicAttr_getDescrClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_1_UPDATER.subUpdater(0, 17), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_getDescrClass__field1_", Node.class)));
this.getDynamicAttr_raiseNode_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, GET_DYNAMIC_ATTR__PY_OBJECT_GET_METHOD_GET_DYNAMIC_ATTR_STATE_0_UPDATER.subUpdater(19, 1), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_raiseNode__field1_", Node.class)));
this.getForeignMethod0_isForeignObjectNode_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, GET_FOREIGN_METHOD0__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD0_STATE_0_UPDATER.subUpdater(0, 8)));
this.getForeignMethod0_getAttr_ = PyObjectGetAttrNodeGen.inline(InlineTarget.create(PyObjectGetAttr.class, GET_FOREIGN_METHOD0__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD0_STATE_0_UPDATER.subUpdater(8, 2), ReferenceField.create(GetForeignMethod0Data.lookup_(), "getForeignMethod0_getAttr__field1_", Node.class), ReferenceField.create(GetForeignMethod0Data.lookup_(), "getForeignMethod0_getAttr__field2_", Node.class)));
this.getForeignMethod1_isForeignObjectNode_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, GET_FOREIGN_METHOD1__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD1_STATE_0_UPDATER.subUpdater(2, 8)));
this.getForeignMethod1_getAttr_ = PyObjectGetAttrNodeGen.inline(InlineTarget.create(PyObjectGetAttr.class, GET_FOREIGN_METHOD1__PY_OBJECT_GET_METHOD_GET_FOREIGN_METHOD1_STATE_0_UPDATER.subUpdater(10, 2), ReferenceField.create(GetForeignMethod1Data.lookup_(), "getForeignMethod1_getAttr__field1_", Node.class), ReferenceField.create(GetForeignMethod1Data.lookup_(), "getForeignMethod1_getAttr__field2_", Node.class)));
this.fallback_getAttr_ = PyObjectGetAttrNodeGen.inline(InlineTarget.create(PyObjectGetAttr.class, FALLBACK__PY_OBJECT_GET_METHOD_FALLBACK_STATE_0_UPDATER.subUpdater(0, 2), ReferenceField.create(FallbackData.lookup_(), "fallback_getAttr__field1_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_getAttr__field2_", Node.class)));
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
{
GetDynamicAttrData s1_ = this.getDynamicAttr_cache.get(arg0Value);
if ((s1_ == null || ((s1_.getDynamicAttr_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy), guardIndex=0] */ || (PyObjectGetMethod.isObjectGetAttribute((this.getDynamicAttr_getClass_.execute(s1_, arg1Value)))))) {
return false;
}
}
{
GetForeignMethod1Data s3_ = this.getForeignMethod1_cache.get(arg0Value);
if ((s3_ == null || ((s3_.getForeignMethod1_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr), guardIndex=0] */ || (this.getForeignMethod1_isForeignObjectNode_.execute(s3_, arg1Value)))) {
return false;
}
}
return true;
}
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] || SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] || SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] || SpecializationActive[PyObjectGetMethod.getGenericAttr(Frame, Node, Object, TruffleString, PyObjectGetAttr)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */) {
GetFixedAttrData s0_ = this.getFixedAttr_cache.get(arg0Value);
if (s0_ != null) {
{
LookupCallableSlotInMRONode lookupGet_ = this.lookupGet.get(arg0Value);
if (lookupGet_ != null) {
LookupCallableSlotInMRONode lookupSet_ = this.lookupSet.get(arg0Value);
if (lookupSet_ != null) {
CallTernaryMethodNode callGet_ = this.callGet.get(arg0Value);
if (callGet_ != null) {
ReadAttributeFromObjectNode readAttr_ = this.readAttr.get(arg0Value);
if (readAttr_ != null) {
Object lazyClass__ = (this.getFixedAttr_getClass_.execute(s0_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__)) && (arg2Value == s0_.cachedName_)) {
return PyObjectGetMethod.getFixedAttr((VirtualFrame) frameValue, s0_, arg1Value, arg2Value, this.getFixedAttr_getClass_, lazyClass__, s0_.cachedName_, s0_.lookupNode_, this.getFixedAttr_getDescrClass_, lookupGet_, lookupSet_, callGet_, readAttr_, this.getFixedAttr_raiseNode_, this.getFixedAttr_hasDescr_, this.getFixedAttr_returnDataDescr_, this.getFixedAttr_returnAttr_, this.getFixedAttr_returnUnboundMethod_, this.getFixedAttr_returnBoundDescr_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] */) {
GetDynamicAttrData s1_ = this.getDynamicAttr_cache.get(arg0Value);
if (s1_ != null) {
if ((s1_.getDynamicAttr_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
LookupCallableSlotInMRONode lookupGet_1 = this.lookupGet.get(arg0Value);
if (lookupGet_1 != null) {
LookupCallableSlotInMRONode lookupSet_1 = this.lookupSet.get(arg0Value);
if (lookupSet_1 != null) {
CallTernaryMethodNode callGet_1 = this.callGet.get(arg0Value);
if (callGet_1 != null) {
ReadAttributeFromObjectNode readAttr_1 = this.readAttr.get(arg0Value);
if (readAttr_1 != null) {
Object lazyClass__1 = (this.getDynamicAttr_getClass_.execute(s1_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__1))) {
return PyObjectGetMethod.getDynamicAttr(frameValue, s1_, arg1Value, arg2Value, this.getDynamicAttr_getClass_, lazyClass__1, s1_.lookupNode_, this.getDynamicAttr_getDescrClass_, lookupGet_1, lookupSet_1, callGet_1, readAttr_1, this.getDynamicAttr_raiseNode_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */) {
GetForeignMethod0Data s2_ = this.getForeignMethod0_cache.get(arg0Value);
if (s2_ != null) {
if ((s2_.lib_.accepts(arg1Value)) && (this.getForeignMethod0_isForeignObjectNode_.execute(s2_, arg1Value))) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s2_, arg1Value, arg2Value, this.getForeignMethod0_isForeignObjectNode_, s2_.toJavaString_, s2_.lib_, this.getForeignMethod0_getAttr_);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */) {
GetForeignMethod1Data s3_ = this.getForeignMethod1_cache.get(arg0Value);
if (s3_ != null) {
if ((s3_.getForeignMethod1_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */ && (this.getForeignMethod1_isForeignObjectNode_.execute(s3_, arg1Value))) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(arg0Value);
try {
{
InteropLibrary lib__ = (INTEROP_LIBRARY_.getUncached());
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, this.getForeignMethod1_isForeignObjectNode_, s3_.toJavaString_, lib__, this.getForeignMethod1_getAttr_);
}
} finally {
encapsulating_.set(prev_);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyObjectGetMethod.getGenericAttr(Frame, Node, Object, TruffleString, PyObjectGetAttr)] */) {
FallbackData s4_ = this.fallback_cache.get(arg0Value);
if (s4_ != null) {
if (fallbackGuard_(state_0, arg0Value, arg1Value, arg2Value)) {
return PyObjectGetMethod.getGenericAttr(frameValue, s4_, arg1Value, arg2Value, this.fallback_getAttr_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
{
Object lazyClass__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] */) {
while (true) {
int count0_ = 0;
GetFixedAttrData s0_ = this.getFixedAttr_cache.getVolatile(arg0Value);
GetFixedAttrData s0_original = s0_;
while (s0_ != null) {
{
LookupCallableSlotInMRONode lookupGet_ = this.lookupGet.get(arg0Value);
if (lookupGet_ != null) {
LookupCallableSlotInMRONode lookupSet_ = this.lookupSet.get(arg0Value);
if (lookupSet_ != null) {
CallTernaryMethodNode callGet_ = this.callGet.get(arg0Value);
if (callGet_ != null) {
ReadAttributeFromObjectNode readAttr_ = this.readAttr.get(arg0Value);
if (readAttr_ != null) {
lazyClass__ = (this.getFixedAttr_getClass_.execute(s0_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__)) && (arg2Value == s0_.cachedName_)) {
break;
}
}
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
s0_ = arg0Value.insert(new GetFixedAttrData());
lazyClass__ = (this.getFixedAttr_getClass_.execute(s0_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__))) {
// assert (arg2Value == s0_.cachedName_);
s0_.cachedName_ = (arg2Value);
LookupAttributeInMRONode lookupNode__ = s0_.insert((LookupAttributeInMRONode.create(arg2Value)));
Objects.requireNonNull(lookupNode__, "Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' cache 'lookupNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.lookupNode_ = lookupNode__;
LookupCallableSlotInMRONode lookupGet_;
LookupCallableSlotInMRONode lookupGet__shared = this.lookupGet.get(arg0Value);
if (lookupGet__shared != null) {
lookupGet_ = lookupGet__shared;
} else {
lookupGet_ = s0_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Get)));
if (lookupGet_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'lookupGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet.get(arg0Value) == null) {
this.lookupGet.set(arg0Value, lookupGet_);
}
LookupCallableSlotInMRONode lookupSet_;
LookupCallableSlotInMRONode lookupSet__shared = this.lookupSet.get(arg0Value);
if (lookupSet__shared != null) {
lookupSet_ = lookupSet__shared;
} else {
lookupSet_ = s0_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Set)));
if (lookupSet_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'lookupSet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupSet.get(arg0Value) == null) {
this.lookupSet.set(arg0Value, lookupSet_);
}
CallTernaryMethodNode callGet_;
CallTernaryMethodNode callGet__shared = this.callGet.get(arg0Value);
if (callGet__shared != null) {
callGet_ = callGet__shared;
} else {
callGet_ = s0_.insert((CallTernaryMethodNode.create()));
if (callGet_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'callGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callGet.get(arg0Value) == null) {
this.callGet.set(arg0Value, callGet_);
}
ReadAttributeFromObjectNode readAttr_;
ReadAttributeFromObjectNode readAttr__shared = this.readAttr.get(arg0Value);
if (readAttr__shared != null) {
readAttr_ = readAttr__shared;
} else {
readAttr_ = s0_.insert((ReadAttributeFromObjectNode.create()));
if (readAttr_ == null) {
throw new IllegalStateException("Specialization 'getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)' contains a shared cache with name 'readAttr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readAttr.get(arg0Value) == null) {
this.readAttr.set(arg0Value, readAttr_);
}
if (!this.getFixedAttr_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s0_ = null;
}
}
}
if (s0_ != null) {
return PyObjectGetMethod.getFixedAttr((VirtualFrame) frameValue, s0_, arg1Value, arg2Value, this.getFixedAttr_getClass_, lazyClass__, s0_.cachedName_, s0_.lookupNode_, this.getFixedAttr_getDescrClass_, this.lookupGet.get(arg0Value), this.lookupSet.get(arg0Value), this.callGet.get(arg0Value), this.readAttr.get(arg0Value), this.getFixedAttr_raiseNode_, this.getFixedAttr_hasDescr_, this.getFixedAttr_returnDataDescr_, this.getFixedAttr_returnAttr_, this.getFixedAttr_returnUnboundMethod_, this.getFixedAttr_returnBoundDescr_);
}
break;
}
}
}
{
Object lazyClass__1 = null;
while (true) {
int count1_ = 0;
GetDynamicAttrData s1_ = this.getDynamicAttr_cache.getVolatile(arg0Value);
GetDynamicAttrData s1_original = s1_;
while (s1_ != null) {
if ((s1_.getDynamicAttr_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
LookupCallableSlotInMRONode lookupGet_1 = this.lookupGet.get(arg0Value);
if (lookupGet_1 != null) {
LookupCallableSlotInMRONode lookupSet_1 = this.lookupSet.get(arg0Value);
if (lookupSet_1 != null) {
CallTernaryMethodNode callGet_1 = this.callGet.get(arg0Value);
if (callGet_1 != null) {
ReadAttributeFromObjectNode readAttr_1 = this.readAttr.get(arg0Value);
if (readAttr_1 != null) {
lazyClass__1 = (this.getDynamicAttr_getClass_.execute(s1_, arg1Value));
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__1))) {
break;
}
}
}
}
}
}
if ((s1_.getDynamicAttr_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count1_++;
}
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
{
s1_ = arg0Value.insert(new GetDynamicAttrData());
lazyClass__1 = (this.getDynamicAttr_getClass_.execute(s1_, arg1Value));
if (((s1_.getDynamicAttr_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy), guardIndex=0] */) {
s1_.getDynamicAttr_state_0_ = s1_.getDynamicAttr_state_0_ | 0b1 /* add GuardActive[specialization=PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy), guardIndex=0] */;
if (!this.getDynamicAttr_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
s1_original = s1_;
s1_ = arg0Value.insert(new GetDynamicAttrData(s1_));
}
if ((PyObjectGetMethod.isObjectGetAttribute(lazyClass__1))) {
Dynamic lookupNode__1 = s1_.insert((Dynamic.create()));
Objects.requireNonNull(lookupNode__1, "Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' cache 'lookupNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.lookupNode_ = lookupNode__1;
LookupCallableSlotInMRONode lookupGet_1;
LookupCallableSlotInMRONode lookupGet_1_shared = this.lookupGet.get(arg0Value);
if (lookupGet_1_shared != null) {
lookupGet_1 = lookupGet_1_shared;
} else {
lookupGet_1 = s1_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Get)));
if (lookupGet_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'lookupGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet.get(arg0Value) == null) {
this.lookupGet.set(arg0Value, lookupGet_1);
}
LookupCallableSlotInMRONode lookupSet_1;
LookupCallableSlotInMRONode lookupSet_1_shared = this.lookupSet.get(arg0Value);
if (lookupSet_1_shared != null) {
lookupSet_1 = lookupSet_1_shared;
} else {
lookupSet_1 = s1_.insert((LookupCallableSlotInMRONode.create(SpecialMethodSlot.Set)));
if (lookupSet_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'lookupSet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupSet.get(arg0Value) == null) {
this.lookupSet.set(arg0Value, lookupSet_1);
}
CallTernaryMethodNode callGet_1;
CallTernaryMethodNode callGet_1_shared = this.callGet.get(arg0Value);
if (callGet_1_shared != null) {
callGet_1 = callGet_1_shared;
} else {
callGet_1 = s1_.insert((CallTernaryMethodNode.create()));
if (callGet_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'callGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callGet.get(arg0Value) == null) {
this.callGet.set(arg0Value, callGet_1);
}
ReadAttributeFromObjectNode readAttr_1;
ReadAttributeFromObjectNode readAttr_1_shared = this.readAttr.get(arg0Value);
if (readAttr_1_shared != null) {
readAttr_1 = readAttr_1_shared;
} else {
readAttr_1 = s1_.insert((ReadAttributeFromObjectNode.create()));
if (readAttr_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)' contains a shared cache with name 'readAttr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readAttr.get(arg0Value) == null) {
this.readAttr.set(arg0Value, readAttr_1);
}
s1_.getDynamicAttr_state_0_ = s1_.getDynamicAttr_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!this.getDynamicAttr_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
this.getFixedAttr_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PyObjectGetMethod.getFixedAttr(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, TruffleString, LookupAttributeInMRONode, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectGetMethod.getDynamicAttr(Frame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, GetClassNode, LookupCallableSlotInMRONode, LookupCallableSlotInMRONode, CallTernaryMethodNode, ReadAttributeFromObjectNode, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s1_ = null;
}
}
}
if (s1_ != null) {
return PyObjectGetMethod.getDynamicAttr(frameValue, s1_, arg1Value, arg2Value, this.getDynamicAttr_getClass_, lazyClass__1, s1_.lookupNode_, this.getDynamicAttr_getDescrClass_, this.lookupGet.get(arg0Value), this.lookupSet.get(arg0Value), this.callGet.get(arg0Value), this.readAttr.get(arg0Value), this.getDynamicAttr_raiseNode_);
}
break;
}
}
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */) {
while (true) {
int count2_ = 0;
GetForeignMethod0Data s2_ = this.getForeignMethod0_cache.getVolatile(arg0Value);
GetForeignMethod0Data s2_original = s2_;
while (s2_ != null) {
if ((s2_.lib_.accepts(arg1Value)) && (this.getForeignMethod0_isForeignObjectNode_.execute(s2_, arg1Value))) {
break;
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
// assert (s2_.lib_.accepts(arg1Value));
{
s2_ = arg0Value.insert(new GetForeignMethod0Data());
if ((this.getForeignMethod0_isForeignObjectNode_.execute(s2_, arg1Value))) {
ToJavaStringNode toJavaString__ = s2_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaString__, "Specialization 'getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)' cache 'toJavaString' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.toJavaString_ = toJavaString__;
InteropLibrary lib__ = s2_.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(lib__, "Specialization 'getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)' cache 'lib' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.lib_ = lib__;
if (!this.getForeignMethod0_cache.compareAndSet(arg0Value, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s2_ = null;
}
}
}
if (s2_ != null) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s2_, arg1Value, arg2Value, this.getForeignMethod0_isForeignObjectNode_, s2_.toJavaString_, s2_.lib_, this.getForeignMethod0_getAttr_);
}
break;
}
}
{
InteropLibrary lib__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(arg0Value);
try {
while (true) {
int count3_ = 0;
GetForeignMethod1Data s3_ = this.getForeignMethod1_cache.getVolatile(arg0Value);
GetForeignMethod1Data s3_original = s3_;
while (s3_ != null) {
if ((s3_.getForeignMethod1_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */ && (this.getForeignMethod1_isForeignObjectNode_.execute(s3_, arg1Value))) {
lib__ = (INTEROP_LIBRARY_.getUncached());
break;
}
if ((s3_.getForeignMethod1_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count3_++;
}
s3_ = null;
break;
}
if (s3_ == null && count3_ < 1) {
{
s3_ = arg0Value.insert(new GetForeignMethod1Data());
if (((s3_.getForeignMethod1_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr), guardIndex=0] */) {
s3_.getForeignMethod1_state_0_ = s3_.getForeignMethod1_state_0_ | 0b1 /* add GuardActive[specialization=PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr), guardIndex=0] */;
if (!this.getForeignMethod1_cache.compareAndSet(arg0Value, s3_original, s3_)) {
continue;
}
s3_original = s3_;
s3_ = arg0Value.insert(new GetForeignMethod1Data(s3_));
}
if ((this.getForeignMethod1_isForeignObjectNode_.execute(s3_, arg1Value))) {
ToJavaStringNode toJavaString__ = s3_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaString__, "Specialization 'getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)' cache 'toJavaString' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.toJavaString_ = toJavaString__;
lib__ = (INTEROP_LIBRARY_.getUncached());
s3_.getForeignMethod1_state_0_ = s3_.getForeignMethod1_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!this.getForeignMethod1_cache.compareAndSet(arg0Value, s3_original, s3_)) {
continue;
}
this.getForeignMethod0_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectGetMethod.getForeignMethod(VirtualFrame, Node, Object, TruffleString, IsForeignObjectNode, ToJavaStringNode, InteropLibrary, PyObjectGetAttr)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s3_ = null;
}
}
}
if (s3_ != null) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, s3_, arg1Value, arg2Value, this.getForeignMethod1_isForeignObjectNode_, s3_.toJavaString_, lib__, this.getForeignMethod1_getAttr_);
}
break;
}
} finally {
encapsulating_.set(prev_);
}
}
}
FallbackData s4_ = arg0Value.insert(new FallbackData());
VarHandle.storeStoreFence();
this.fallback_cache.set(arg0Value, s4_);
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyObjectGetMethod.getGenericAttr(Frame, Node, Object, TruffleString, PyObjectGetAttr)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectGetMethod.getGenericAttr(frameValue, s4_, arg1Value, arg2Value, this.fallback_getAttr_);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class GetFixedAttrData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* 18: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} hasDescr
* Inline method: {@link InlinedBranchProfile#inline}
* 19: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnDataDescr
* Inline method: {@link InlinedBranchProfile#inline}
* 20: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnAttr
* Inline method: {@link InlinedBranchProfile#inline}
* 21: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnUnboundMethod
* Inline method: {@link InlinedBranchProfile#inline}
* 22: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link InlinedBranchProfile} returnBoundDescr
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getFixedAttr_state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getFixedAttr_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getFixedAttr_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link LookupAttributeInMRONode} lookupNode
*/
@Child LookupAttributeInMRONode lookupNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getFixedAttr_getDescrClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getFixedAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getFixedAttr_raiseNode__field1_;
GetFixedAttrData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class GetDynamicAttrData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: GuardActive[guardIndex=0] {@link PyObjectGetMethod#getDynamicAttr}
* 1: SpecializationCachesInitialized {@link PyObjectGetMethod#getDynamicAttr}
* 2-18: InlinedCache
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 19: InlinedCache
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getDynamicAttr_state_0_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getDynamicAttr_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getDynamicAttr_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link Dynamic} lookupNode
*/
@Child Dynamic lookupNode_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link GetClassNode} getDescrClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getDynamicAttr_getDescrClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getDynamicAttr}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getDynamicAttr_raiseNode__field1_;
GetDynamicAttrData() {
}
GetDynamicAttrData(GetDynamicAttrData delegate) {
this.getDynamicAttr_state_0_ = delegate.getDynamicAttr_state_0_;
this.getDynamicAttr_state_1_ = delegate.getDynamicAttr_state_1_;
this.getDynamicAttr_getClass__field1_ = delegate.getDynamicAttr_getClass__field1_;
this.lookupNode_ = delegate.lookupNode_;
this.getDynamicAttr_getDescrClass__field1_ = delegate.getDynamicAttr_getDescrClass__field1_;
this.getDynamicAttr_raiseNode__field1_ = delegate.getDynamicAttr_raiseNode__field1_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class GetForeignMethod0Data extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
* 8-9: InlinedCache
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getForeignMethod0_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link ToJavaStringNode} toJavaString
*/
@Child ToJavaStringNode toJavaString_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link InteropLibrary} lib
*/
@Child InteropLibrary lib_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getForeignMethod0_getAttr__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getForeignMethod0_getAttr__field2_;
GetForeignMethod0Data() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class GetForeignMethod1Data extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: GuardActive[guardIndex=0] {@link PyObjectGetMethod#getForeignMethod}
* 1: SpecializationCachesInitialized {@link PyObjectGetMethod#getForeignMethod}
* 2-9: InlinedCache
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
* 10-11: InlinedCache
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getForeignMethod1_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link ToJavaStringNode} toJavaString
*/
@Child ToJavaStringNode toJavaString_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getForeignMethod1_getAttr__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getForeignMethod}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getForeignMethod1_getAttr__field2_;
GetForeignMethod1Data() {
}
GetForeignMethod1Data(GetForeignMethod1Data delegate) {
this.getForeignMethod1_state_0_ = delegate.getForeignMethod1_state_0_;
this.toJavaString_ = delegate.toJavaString_;
this.getForeignMethod1_getAttr__field1_ = delegate.getForeignMethod1_getAttr__field1_;
this.getForeignMethod1_getAttr__field2_ = delegate.getForeignMethod1_getAttr__field2_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link PyObjectGetMethod#getGenericAttr}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getGenericAttr}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getAttr__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectGetMethod#getGenericAttr}
* Parameter: {@link PyObjectGetAttr} getAttr
* Inline method: {@link PyObjectGetAttrNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getAttr__field2_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectGetMethod.class)
@DenyReplace
private static final class Uncached extends PyObjectGetMethod {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, TruffleString arg2Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if ((PyObjectGetMethod.isObjectGetAttribute(((GetClassNode.getUncached()).execute(arg0Value, arg1Value))))) {
return PyObjectGetMethod.getDynamicAttr(frameValue, arg0Value, arg1Value, arg2Value, (GetClassNode.getUncached()), ((GetClassNode.getUncached()).execute(arg0Value, arg1Value)), (Dynamic.getUncached()), (GetClassNode.getUncached()), (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.Get)), (LookupCallableSlotInMRONode.getUncached(SpecialMethodSlot.Set)), (CallTernaryMethodNode.getUncached()), (ReadAttributeFromObjectNode.getUncached()), (Lazy.getUncached()));
}
if (((IsForeignObjectNodeGen.getUncached()).execute(arg0Value, arg1Value))) {
return PyObjectGetMethod.getForeignMethod((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, (IsForeignObjectNodeGen.getUncached()), (ToJavaStringNode.getUncached()), (INTEROP_LIBRARY_.getUncached(arg1Value)), (PyObjectGetAttr.getUncached()));
}
return PyObjectGetMethod.getGenericAttr(frameValue, arg0Value, arg1Value, arg2Value, (PyObjectGetAttr.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy