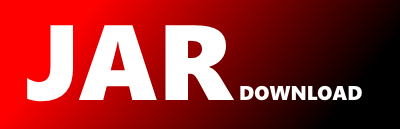
com.oracle.graal.python.lib.PyObjectLookupAttrNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.attributes.LookupAttributeInMRONode;
import com.oracle.graal.python.nodes.attributes.LookupInheritedSlotNode;
import com.oracle.graal.python.nodes.attributes.ReadAttributeFromObjectNode;
import com.oracle.graal.python.nodes.attributes.LookupAttributeInMRONode.Dynamic;
import com.oracle.graal.python.nodes.call.CallNode;
import com.oracle.graal.python.nodes.call.special.CallBinaryMethodNode;
import com.oracle.graal.python.nodes.call.special.CallTernaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.graal.python.nodes.object.BuiltinClassProfiles.IsBuiltinObjectProfile;
import com.oracle.graal.python.nodes.object.BuiltinClassProfilesFactory.IsBuiltinObjectProfileNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.CodePointAtIndexNode;
import com.oracle.truffle.api.strings.TruffleString.CodePointLengthNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link PyObjectLookupAttr#doBuiltinObject}
* Activation probability: 0.32000
* With/without class size: 15/15 bytes
* Specialization {@link PyObjectLookupAttr#doBuiltinModule}
* Activation probability: 0.26000
* With/without class size: 16/29 bytes
* Specialization {@link PyObjectLookupAttr#doBuiltinTypeType}
* Activation probability: 0.20000
* With/without class size: 11/18 bytes
* Specialization {@link PyObjectLookupAttr#doBuiltinType}
* Activation probability: 0.14000
* With/without class size: 10/26 bytes
* Specialization {@link PyObjectLookupAttr#getDynamicAttr}
* Activation probability: 0.08000
* With/without class size: 7/29 bytes
*
*/
@GeneratedBy(PyObjectLookupAttr.class)
@SuppressWarnings({"javadoc", "unused"})
public final class PyObjectLookupAttrNodeGen extends PyObjectLookupAttr {
private static final StateField BUILTIN_OBJECT__PY_OBJECT_LOOKUP_ATTR_BUILTIN_OBJECT_STATE_0_UPDATER = StateField.create(BuiltinObjectData.lookup_(), "builtinObject_state_0_");
private static final StateField BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_0_UPDATER = StateField.create(BuiltinModuleData.lookup_(), "builtinModule_state_0_");
private static final StateField BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_1_UPDATER = StateField.create(BuiltinModuleData.lookup_(), "builtinModule_state_1_");
private static final StateField BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER = StateField.create(BuiltinTypeTypeData.lookup_(), "builtinTypeType_state_0_");
private static final StateField BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_1_UPDATER = StateField.create(BuiltinTypeTypeData.lookup_(), "builtinTypeType_state_1_");
private static final StateField BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER = StateField.create(BuiltinTypeData.lookup_(), "builtinType_state_0_");
private static final StateField BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_1_UPDATER = StateField.create(BuiltinTypeData.lookup_(), "builtinType_state_1_");
private static final StateField GET_DYNAMIC_ATTR__PY_OBJECT_LOOKUP_ATTR_GET_DYNAMIC_ATTR_STATE_0_UPDATER = StateField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_state_0_");
private static final StateField GET_DYNAMIC_ATTR__PY_OBJECT_LOOKUP_ATTR_GET_DYNAMIC_ATTR_STATE_1_UPDATER = StateField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_state_1_");
static final ReferenceField BUILTIN_OBJECT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "builtinObject_cache", BuiltinObjectData.class);
static final ReferenceField BUILTIN_MODULE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "builtinModule_cache", BuiltinModuleData.class);
static final ReferenceField BUILTIN_TYPE_TYPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "builtinTypeType_cache", BuiltinTypeTypeData.class);
static final ReferenceField BUILTIN_TYPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "builtinType_cache", BuiltinTypeData.class);
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_BUILTIN_OBJECT_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_OBJECT__PY_OBJECT_LOOKUP_ATTR_BUILTIN_OBJECT_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinObjectData.lookup_(), "builtinObject_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_BUILTIN_MODULE_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinModuleData.lookup_(), "builtinModule_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_BUILTIN_MODULE_ERROR_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(BuiltinModuleData.lookup_(), "builtinModule_errorProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link InlinedConditionProfile} noValueFound
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_BUILTIN_MODULE_NO_VALUE_FOUND_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_0_UPDATER.subUpdater(17, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_BUILTIN_TYPE_TYPE_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinTypeTypeData.lookup_(), "builtinTypeType_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link InlinedConditionProfile} valueFound
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_BUILTIN_TYPE_TYPE_VALUE_FOUND_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER.subUpdater(17, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link InlinedConditionProfile} noGetMethod
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_BUILTIN_TYPE_TYPE_NO_GET_METHOD_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER.subUpdater(19, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_BUILTIN_TYPE_TYPE_ERROR_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(BuiltinTypeTypeData.lookup_(), "builtinTypeType_errorProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_BUILTIN_TYPE_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinTypeData.lookup_(), "builtinType_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link InlinedConditionProfile} valueFound
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_BUILTIN_TYPE_VALUE_FOUND_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER.subUpdater(17, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link InlinedConditionProfile} noGetMethod
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_BUILTIN_TYPE_NO_GET_METHOD_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER.subUpdater(19, 2)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_BUILTIN_TYPE_ERROR_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(BuiltinTypeData.lookup_(), "builtinType_errorProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_DYNAMIC_ATTR_GET_CLASS_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_DYNAMIC_ATTR__PY_OBJECT_LOOKUP_ATTR_GET_DYNAMIC_ATTR_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_getClass__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_GET_DYNAMIC_ATTR_ERROR_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, GET_DYNAMIC_ATTR__PY_OBJECT_LOOKUP_ATTR_GET_DYNAMIC_ATTR_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_errorProfile__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectLookupAttr#doBuiltinObject}
* 1: SpecializationActive {@link PyObjectLookupAttr#getDynamicAttr}
* 2: SpecializationActive {@link PyObjectLookupAttr#doBuiltinModule}
* 3: SpecializationActive {@link PyObjectLookupAttr#doBuiltinTypeType}
* 4: SpecializationActive {@link PyObjectLookupAttr#doBuiltinType}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link ReadAttributeFromObjectNode} readNode
*/
@Child private ReadAttributeFromObjectNode readNode;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link LookupInheritedSlotNode} lookupValueGet
*/
@Child private LookupInheritedSlotNode lookupValueGet;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link CallTernaryMethodNode} invokeValueGet
*/
@Child private CallTernaryMethodNode invokeValueGet;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link CodePointLengthNode} codePointLengthNode
*/
@Child private CodePointLengthNode codePointLengthNode;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link CodePointAtIndexNode} codePointAtIndexNode
*/
@Child private CodePointAtIndexNode codePointAtIndexNode;
@UnsafeAccessedField @Child private BuiltinObjectData builtinObject_cache;
@UnsafeAccessedField @Child private BuiltinModuleData builtinModule_cache;
@UnsafeAccessedField @Child private BuiltinTypeTypeData builtinTypeType_cache;
@UnsafeAccessedField @Child private BuiltinTypeData builtinType_cache;
@Child private GetDynamicAttrData getDynamicAttr_cache;
private PyObjectLookupAttrNodeGen() {
}
@ExplodeLoop
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] || SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
if ((state_0 & 0b11101) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */ && arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] */) {
BuiltinObjectData s0_ = this.builtinObject_cache;
while (s0_ != null) {
{
ReadAttributeFromObjectNode readNode_ = this.readNode;
if (readNode_ != null) {
Object type__ = (INLINED_BUILTIN_OBJECT_GET_CLASS_.execute(s0_, arg1Value));
if ((PyObjectLookupAttr.isObjectGetAttribute(type__)) && (PyObjectLookupAttr.hasNoGetAttr(type__)) && (arg2Value_ == s0_.cachedName_)) {
Object descr__ = (s0_.lookupName_.execute(type__));
if ((PGuards.isNoValue(descr__))) {
return PyObjectLookupAttr.doBuiltinObject((VirtualFrame) frameValue, s0_, arg1Value, arg2Value_, s0_.cachedName_, INLINED_BUILTIN_OBJECT_GET_CLASS_, type__, s0_.lookupName_, descr__, readNode_);
}
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] */) {
BuiltinModuleData s1_ = this.builtinModule_cache;
if (s1_ != null) {
{
ReadAttributeFromObjectNode readNode_1 = this.readNode;
if (readNode_1 != null) {
Object type__1 = (INLINED_BUILTIN_MODULE_GET_CLASS_.execute(s1_, arg1Value));
if ((PyObjectLookupAttr.isModuleGetAttribute(type__1)) && (PyObjectLookupAttr.hasNoGetAttr(type__1)) && (arg2Value_ == s1_.cachedName_)) {
Object descr__1 = (s1_.lookupName_.execute(type__1));
if ((PGuards.isNoValue(descr__1))) {
return PyObjectLookupAttr.doBuiltinModule((VirtualFrame) frameValue, s1_, arg1Value, arg2Value_, s1_.cachedName_, INLINED_BUILTIN_MODULE_GET_CLASS_, type__1, s1_.lookupName_, descr__1, readNode_1, s1_.readGetattr_, INLINED_BUILTIN_MODULE_ERROR_PROFILE_, INLINED_BUILTIN_MODULE_NO_VALUE_FOUND_, s1_.callGetattr_);
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
BuiltinTypeTypeData s2_ = this.builtinTypeType_cache;
if (s2_ != null) {
{
LookupInheritedSlotNode lookupValueGet_ = this.lookupValueGet;
if (lookupValueGet_ != null) {
CallTernaryMethodNode invokeValueGet_ = this.invokeValueGet;
if (invokeValueGet_ != null) {
CodePointLengthNode codePointLengthNode_ = this.codePointLengthNode;
if (codePointLengthNode_ != null) {
CodePointAtIndexNode codePointAtIndexNode_ = this.codePointAtIndexNode;
if (codePointAtIndexNode_ != null) {
Object type__2 = (INLINED_BUILTIN_TYPE_TYPE_GET_CLASS_.execute(s2_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__2)) && (PyObjectLookupAttr.isBuiltinTypeType(type__2)) && (!(PyObjectLookupAttr.isTypeSlot(arg2Value_, codePointLengthNode_, codePointAtIndexNode_)))) {
return PyObjectLookupAttr.doBuiltinTypeType((VirtualFrame) frameValue, s2_, arg1Value, arg2Value_, INLINED_BUILTIN_TYPE_TYPE_GET_CLASS_, type__2, s2_.readNode_, INLINED_BUILTIN_TYPE_TYPE_VALUE_FOUND_, lookupValueGet_, INLINED_BUILTIN_TYPE_TYPE_NO_GET_METHOD_, invokeValueGet_, INLINED_BUILTIN_TYPE_TYPE_ERROR_PROFILE_, codePointLengthNode_, codePointAtIndexNode_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */) {
BuiltinTypeData s3_ = this.builtinType_cache;
if (s3_ != null) {
{
LookupInheritedSlotNode lookupValueGet_1 = this.lookupValueGet;
if (lookupValueGet_1 != null) {
CallTernaryMethodNode invokeValueGet_1 = this.invokeValueGet;
if (invokeValueGet_1 != null) {
Object type__3 = (INLINED_BUILTIN_TYPE_GET_CLASS_.execute(s3_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__3)) && (PyObjectLookupAttr.hasNoGetAttr(type__3)) && (arg2Value_ == s3_.cachedName_)) {
Object metaClassDescr__ = (s3_.lookupInMetaclassHierachy_.execute(type__3));
if ((PGuards.isNoValue(metaClassDescr__))) {
return PyObjectLookupAttr.doBuiltinType((VirtualFrame) frameValue, s3_, arg1Value, arg2Value_, s3_.cachedName_, INLINED_BUILTIN_TYPE_GET_CLASS_, type__3, s3_.lookupInMetaclassHierachy_, metaClassDescr__, s3_.readNode_, INLINED_BUILTIN_TYPE_VALUE_FOUND_, lookupValueGet_1, INLINED_BUILTIN_TYPE_NO_GET_METHOD_, invokeValueGet_1, INLINED_BUILTIN_TYPE_ERROR_PROFILE_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
GetDynamicAttrData s4_ = this.getDynamicAttr_cache;
if (s4_ != null) {
{
CodePointLengthNode codePointLengthNode_1 = this.codePointLengthNode;
if (codePointLengthNode_1 != null) {
CodePointAtIndexNode codePointAtIndexNode_1 = this.codePointAtIndexNode;
if (codePointAtIndexNode_1 != null) {
return PyObjectLookupAttr.getDynamicAttr(frameValue, s4_, arg1Value, arg2Value, INLINED_GET_DYNAMIC_ATTR_GET_CLASS_, s4_.lookupGetattribute_, s4_.lookupGetattr_, s4_.callGetattribute_, s4_.callGetattr_, INLINED_GET_DYNAMIC_ATTR_ERROR_PROFILE_, codePointLengthNode_1, codePointAtIndexNode_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
{
Object descr__ = null;
Object type__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count0_ = 0;
BuiltinObjectData s0_ = BUILTIN_OBJECT_CACHE_UPDATER.getVolatile(this);
BuiltinObjectData s0_original = s0_;
while (s0_ != null) {
{
ReadAttributeFromObjectNode readNode_ = this.readNode;
if (readNode_ != null) {
type__ = (INLINED_BUILTIN_OBJECT_GET_CLASS_.execute(s0_, arg1Value));
if ((PyObjectLookupAttr.isObjectGetAttribute(type__)) && (PyObjectLookupAttr.hasNoGetAttr(type__)) && (arg2Value_ == s0_.cachedName_)) {
descr__ = (s0_.lookupName_.execute(type__));
if ((PGuards.isNoValue(descr__))) {
break;
}
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
s0_ = this.insert(new BuiltinObjectData(s0_original));
type__ = (INLINED_BUILTIN_OBJECT_GET_CLASS_.execute(s0_, arg1Value));
if ((PyObjectLookupAttr.isObjectGetAttribute(type__)) && (PyObjectLookupAttr.hasNoGetAttr(type__))) {
LookupAttributeInMRONode lookupName__ = s0_.insert((LookupAttributeInMRONode.create(arg2Value_)));
descr__ = (lookupName__.execute(type__));
// assert (arg2Value_ == s0_.cachedName_);
if ((PGuards.isNoValue(descr__)) && count0_ < (3)) {
s0_.cachedName_ = (arg2Value_);
Objects.requireNonNull(s0_.insert(lookupName__), "Specialization 'doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)' cache 'lookupName' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.lookupName_ = lookupName__;
ReadAttributeFromObjectNode readNode_;
ReadAttributeFromObjectNode readNode__shared = this.readNode;
if (readNode__shared != null) {
readNode_ = readNode__shared;
} else {
readNode_ = s0_.insert((ReadAttributeFromObjectNode.create()));
if (readNode_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)' contains a shared cache with name 'readNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readNode == null) {
this.readNode = readNode_;
}
if (!BUILTIN_OBJECT_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] */;
this.state_0_ = state_0;
} else {
s0_ = null;
}
} else {
s0_ = null;
}
}
}
if (s0_ != null) {
return PyObjectLookupAttr.doBuiltinObject((VirtualFrame) frameValue, s0_, arg1Value, arg2Value_, s0_.cachedName_, INLINED_BUILTIN_OBJECT_GET_CLASS_, type__, s0_.lookupName_, descr__, this.readNode);
}
break;
}
}
}
{
Object descr__1 = null;
Object type__1 = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count1_ = 0;
BuiltinModuleData s1_ = BUILTIN_MODULE_CACHE_UPDATER.getVolatile(this);
BuiltinModuleData s1_original = s1_;
while (s1_ != null) {
{
ReadAttributeFromObjectNode readNode_1 = this.readNode;
if (readNode_1 != null) {
type__1 = (INLINED_BUILTIN_MODULE_GET_CLASS_.execute(s1_, arg1Value));
if ((PyObjectLookupAttr.isModuleGetAttribute(type__1)) && (PyObjectLookupAttr.hasNoGetAttr(type__1)) && (arg2Value_ == s1_.cachedName_)) {
descr__1 = (s1_.lookupName_.execute(type__1));
if ((PGuards.isNoValue(descr__1))) {
break;
}
}
}
}
count1_++;
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
{
s1_ = this.insert(new BuiltinModuleData());
type__1 = (INLINED_BUILTIN_MODULE_GET_CLASS_.execute(s1_, arg1Value));
if ((PyObjectLookupAttr.isModuleGetAttribute(type__1)) && (PyObjectLookupAttr.hasNoGetAttr(type__1))) {
LookupAttributeInMRONode lookupName__1 = s1_.insert((LookupAttributeInMRONode.create(arg2Value_)));
descr__1 = (lookupName__1.execute(type__1));
// assert (arg2Value_ == s1_.cachedName_);
if ((PGuards.isNoValue(descr__1))) {
s1_.cachedName_ = (arg2Value_);
Objects.requireNonNull(s1_.insert(lookupName__1), "Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' cache 'lookupName' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.lookupName_ = lookupName__1;
ReadAttributeFromObjectNode readNode_1;
ReadAttributeFromObjectNode readNode_1_shared = this.readNode;
if (readNode_1_shared != null) {
readNode_1 = readNode_1_shared;
} else {
readNode_1 = s1_.insert((ReadAttributeFromObjectNode.create()));
if (readNode_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' contains a shared cache with name 'readNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readNode == null) {
this.readNode = readNode_1;
}
ReadAttributeFromObjectNode readGetattr__ = s1_.insert((ReadAttributeFromObjectNode.create()));
Objects.requireNonNull(readGetattr__, "Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' cache 'readGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.readGetattr_ = readGetattr__;
CallNode callGetattr__ = s1_.insert((CallNode.create()));
Objects.requireNonNull(callGetattr__, "Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' cache 'callGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.callGetattr_ = callGetattr__;
if (!BUILTIN_MODULE_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] */;
this.state_0_ = state_0;
} else {
s1_ = null;
}
} else {
s1_ = null;
}
}
}
if (s1_ != null) {
return PyObjectLookupAttr.doBuiltinModule((VirtualFrame) frameValue, s1_, arg1Value, arg2Value_, s1_.cachedName_, INLINED_BUILTIN_MODULE_GET_CLASS_, type__1, s1_.lookupName_, descr__1, this.readNode, s1_.readGetattr_, INLINED_BUILTIN_MODULE_ERROR_PROFILE_, INLINED_BUILTIN_MODULE_NO_VALUE_FOUND_, s1_.callGetattr_);
}
break;
}
}
}
{
Object type__2 = null;
if (((state_0 & 0b10010)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] && SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count2_ = 0;
BuiltinTypeTypeData s2_ = BUILTIN_TYPE_TYPE_CACHE_UPDATER.getVolatile(this);
BuiltinTypeTypeData s2_original = s2_;
while (s2_ != null) {
{
LookupInheritedSlotNode lookupValueGet_ = this.lookupValueGet;
if (lookupValueGet_ != null) {
CallTernaryMethodNode invokeValueGet_ = this.invokeValueGet;
if (invokeValueGet_ != null) {
CodePointLengthNode codePointLengthNode_ = this.codePointLengthNode;
if (codePointLengthNode_ != null) {
CodePointAtIndexNode codePointAtIndexNode_ = this.codePointAtIndexNode;
if (codePointAtIndexNode_ != null) {
type__2 = (INLINED_BUILTIN_TYPE_TYPE_GET_CLASS_.execute(s2_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__2)) && (PyObjectLookupAttr.isBuiltinTypeType(type__2)) && (!(PyObjectLookupAttr.isTypeSlot(arg2Value_, codePointLengthNode_, codePointAtIndexNode_)))) {
break;
}
}
}
}
}
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
{
s2_ = this.insert(new BuiltinTypeTypeData());
type__2 = (INLINED_BUILTIN_TYPE_TYPE_GET_CLASS_.execute(s2_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__2)) && (PyObjectLookupAttr.isBuiltinTypeType(type__2))) {
CodePointLengthNode codePointLengthNode_;
CodePointLengthNode codePointLengthNode__shared = this.codePointLengthNode;
if (codePointLengthNode__shared != null) {
codePointLengthNode_ = codePointLengthNode__shared;
} else {
codePointLengthNode_ = s2_.insert((CodePointLengthNode.create()));
if (codePointLengthNode_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
CodePointAtIndexNode codePointAtIndexNode_;
CodePointAtIndexNode codePointAtIndexNode__shared = this.codePointAtIndexNode;
if (codePointAtIndexNode__shared != null) {
codePointAtIndexNode_ = codePointAtIndexNode__shared;
} else {
codePointAtIndexNode_ = s2_.insert((CodePointAtIndexNode.create()));
if (codePointAtIndexNode_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((!(PyObjectLookupAttr.isTypeSlot(arg2Value_, codePointLengthNode_, codePointAtIndexNode_)))) {
Dynamic readNode__ = s2_.insert((Dynamic.create()));
Objects.requireNonNull(readNode__, "Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'readNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.readNode_ = readNode__;
LookupInheritedSlotNode lookupValueGet_;
LookupInheritedSlotNode lookupValueGet__shared = this.lookupValueGet;
if (lookupValueGet__shared != null) {
lookupValueGet_ = lookupValueGet__shared;
} else {
lookupValueGet_ = s2_.insert((LookupInheritedSlotNode.create(SpecialMethodSlot.Get)));
if (lookupValueGet_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'lookupValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupValueGet == null) {
this.lookupValueGet = lookupValueGet_;
}
CallTernaryMethodNode invokeValueGet_;
CallTernaryMethodNode invokeValueGet__shared = this.invokeValueGet;
if (invokeValueGet__shared != null) {
invokeValueGet_ = invokeValueGet__shared;
} else {
invokeValueGet_ = s2_.insert((CallTernaryMethodNode.create()));
if (invokeValueGet_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'invokeValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invokeValueGet == null) {
this.invokeValueGet = invokeValueGet_;
}
if (this.codePointLengthNode == null) {
this.codePointLengthNode = codePointLengthNode_;
}
if (this.codePointAtIndexNode == null) {
this.codePointAtIndexNode = codePointAtIndexNode_;
}
if (!BUILTIN_TYPE_TYPE_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
} else {
s2_ = null;
}
} else {
s2_ = null;
}
}
}
if (s2_ != null) {
return PyObjectLookupAttr.doBuiltinTypeType((VirtualFrame) frameValue, s2_, arg1Value, arg2Value_, INLINED_BUILTIN_TYPE_TYPE_GET_CLASS_, type__2, s2_.readNode_, INLINED_BUILTIN_TYPE_TYPE_VALUE_FOUND_, this.lookupValueGet, INLINED_BUILTIN_TYPE_TYPE_NO_GET_METHOD_, this.invokeValueGet, INLINED_BUILTIN_TYPE_TYPE_ERROR_PROFILE_, this.codePointLengthNode, this.codePointAtIndexNode);
}
break;
}
}
}
{
Object metaClassDescr__ = null;
Object type__3 = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count3_ = 0;
BuiltinTypeData s3_ = BUILTIN_TYPE_CACHE_UPDATER.getVolatile(this);
BuiltinTypeData s3_original = s3_;
while (s3_ != null) {
{
LookupInheritedSlotNode lookupValueGet_1 = this.lookupValueGet;
if (lookupValueGet_1 != null) {
CallTernaryMethodNode invokeValueGet_1 = this.invokeValueGet;
if (invokeValueGet_1 != null) {
type__3 = (INLINED_BUILTIN_TYPE_GET_CLASS_.execute(s3_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__3)) && (PyObjectLookupAttr.hasNoGetAttr(type__3)) && (arg2Value_ == s3_.cachedName_)) {
metaClassDescr__ = (s3_.lookupInMetaclassHierachy_.execute(type__3));
if ((PGuards.isNoValue(metaClassDescr__))) {
break;
}
}
}
}
}
count3_++;
s3_ = null;
break;
}
if (s3_ == null && count3_ < 1) {
{
s3_ = this.insert(new BuiltinTypeData());
type__3 = (INLINED_BUILTIN_TYPE_GET_CLASS_.execute(s3_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__3)) && (PyObjectLookupAttr.hasNoGetAttr(type__3))) {
LookupAttributeInMRONode lookupInMetaclassHierachy__ = s3_.insert((LookupAttributeInMRONode.create(arg2Value_)));
metaClassDescr__ = (lookupInMetaclassHierachy__.execute(type__3));
// assert (arg2Value_ == s3_.cachedName_);
if ((PGuards.isNoValue(metaClassDescr__))) {
s3_.cachedName_ = (arg2Value_);
Objects.requireNonNull(s3_.insert(lookupInMetaclassHierachy__), "Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' cache 'lookupInMetaclassHierachy' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.lookupInMetaclassHierachy_ = lookupInMetaclassHierachy__;
LookupAttributeInMRONode readNode__1 = s3_.insert((LookupAttributeInMRONode.create(arg2Value_)));
Objects.requireNonNull(readNode__1, "Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' cache 'readNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.readNode_ = readNode__1;
LookupInheritedSlotNode lookupValueGet_1;
LookupInheritedSlotNode lookupValueGet_1_shared = this.lookupValueGet;
if (lookupValueGet_1_shared != null) {
lookupValueGet_1 = lookupValueGet_1_shared;
} else {
lookupValueGet_1 = s3_.insert((LookupInheritedSlotNode.create(SpecialMethodSlot.Get)));
if (lookupValueGet_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' contains a shared cache with name 'lookupValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupValueGet == null) {
this.lookupValueGet = lookupValueGet_1;
}
CallTernaryMethodNode invokeValueGet_1;
CallTernaryMethodNode invokeValueGet_1_shared = this.invokeValueGet;
if (invokeValueGet_1_shared != null) {
invokeValueGet_1 = invokeValueGet_1_shared;
} else {
invokeValueGet_1 = s3_.insert((CallTernaryMethodNode.create()));
if (invokeValueGet_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' contains a shared cache with name 'invokeValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invokeValueGet == null) {
this.invokeValueGet = invokeValueGet_1;
}
if (!BUILTIN_TYPE_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
this.builtinTypeType_cache = null;
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */;
this.state_0_ = state_0;
} else {
s3_ = null;
}
} else {
s3_ = null;
}
}
}
if (s3_ != null) {
return PyObjectLookupAttr.doBuiltinType((VirtualFrame) frameValue, s3_, arg1Value, arg2Value_, s3_.cachedName_, INLINED_BUILTIN_TYPE_GET_CLASS_, type__3, s3_.lookupInMetaclassHierachy_, metaClassDescr__, s3_.readNode_, INLINED_BUILTIN_TYPE_VALUE_FOUND_, this.lookupValueGet, INLINED_BUILTIN_TYPE_NO_GET_METHOD_, this.invokeValueGet, INLINED_BUILTIN_TYPE_ERROR_PROFILE_);
}
break;
}
}
}
}
GetDynamicAttrData s4_ = this.insert(new GetDynamicAttrData());
LookupSpecialMethodSlotNode lookupGetattribute__ = s4_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
Objects.requireNonNull(lookupGetattribute__, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'lookupGetattribute' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.lookupGetattribute_ = lookupGetattribute__;
LookupSpecialMethodSlotNode lookupGetattr__ = s4_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttr)));
Objects.requireNonNull(lookupGetattr__, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'lookupGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.lookupGetattr_ = lookupGetattr__;
CallBinaryMethodNode callGetattribute__ = s4_.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callGetattribute__, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'callGetattribute' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callGetattribute_ = callGetattribute__;
CallBinaryMethodNode callGetattr__1 = s4_.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callGetattr__1, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'callGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callGetattr_ = callGetattr__1;
CodePointLengthNode codePointLengthNode_1;
CodePointLengthNode codePointLengthNode_1_shared = this.codePointLengthNode;
if (codePointLengthNode_1_shared != null) {
codePointLengthNode_1 = codePointLengthNode_1_shared;
} else {
codePointLengthNode_1 = s4_.insert((CodePointLengthNode.create()));
if (codePointLengthNode_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.codePointLengthNode == null) {
this.codePointLengthNode = codePointLengthNode_1;
}
CodePointAtIndexNode codePointAtIndexNode_1;
CodePointAtIndexNode codePointAtIndexNode_1_shared = this.codePointAtIndexNode;
if (codePointAtIndexNode_1_shared != null) {
codePointAtIndexNode_1 = codePointAtIndexNode_1_shared;
} else {
codePointAtIndexNode_1 = s4_.insert((CodePointAtIndexNode.create()));
if (codePointAtIndexNode_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.codePointAtIndexNode == null) {
this.codePointAtIndexNode = codePointAtIndexNode_1;
}
VarHandle.storeStoreFence();
this.getDynamicAttr_cache = s4_;
this.builtinObject_cache = null;
this.builtinModule_cache = null;
this.builtinTypeType_cache = null;
this.builtinType_cache = null;
state_0 = state_0 & 0xffffffe2 /* remove SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)], SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)], SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)], SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_ = state_0;
return PyObjectLookupAttr.getDynamicAttr(frameValue, s4_, arg1Value, arg2Value, INLINED_GET_DYNAMIC_ATTR_GET_CLASS_, lookupGetattribute__, lookupGetattr__, callGetattribute__, callGetattr__1, INLINED_GET_DYNAMIC_ATTR_ERROR_PROFILE_, codePointLengthNode_1, codePointAtIndexNode_1);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
BuiltinObjectData s0_ = this.builtinObject_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyObjectLookupAttr create() {
return new PyObjectLookupAttrNodeGen();
}
@NeverDefault
public static PyObjectLookupAttr getUncached() {
return PyObjectLookupAttrNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#readNode}
*
- {@link Inlined#lookupValueGet}
*
- {@link Inlined#invokeValueGet}
*
- {@link Inlined#codePointLengthNode}
*
- {@link Inlined#codePointAtIndexNode}
*
- {@link Inlined#builtinObject_cache}
*
- {@link Inlined#builtinModule_cache}
*
- {@link Inlined#builtinTypeType_cache}
*
- {@link Inlined#builtinType_cache}
*
- {@link Inlined#getDynamicAttr_cache}
*
*/
@NeverDefault
public static PyObjectLookupAttr inline(@RequiredField(bits = 5, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectLookupAttrNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class Inlined extends PyObjectLookupAttr {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectLookupAttr#doBuiltinObject}
* 1: SpecializationActive {@link PyObjectLookupAttr#getDynamicAttr}
* 2: SpecializationActive {@link PyObjectLookupAttr#doBuiltinModule}
* 3: SpecializationActive {@link PyObjectLookupAttr#doBuiltinTypeType}
* 4: SpecializationActive {@link PyObjectLookupAttr#doBuiltinType}
*
*/
private final StateField state_0_;
private final ReferenceField readNode;
private final ReferenceField lookupValueGet;
private final ReferenceField invokeValueGet;
private final ReferenceField codePointLengthNode;
private final ReferenceField codePointAtIndexNode;
private final ReferenceField builtinObject_cache;
private final ReferenceField builtinModule_cache;
private final ReferenceField builtinTypeType_cache;
private final ReferenceField builtinType_cache;
private final ReferenceField getDynamicAttr_cache;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode builtinObject_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode builtinModule_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile builtinModule_errorProfile_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link InlinedConditionProfile} noValueFound
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile builtinModule_noValueFound_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode builtinTypeType_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link InlinedConditionProfile} valueFound
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile builtinTypeType_valueFound_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link InlinedConditionProfile} noGetMethod
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile builtinTypeType_noGetMethod_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile builtinTypeType_errorProfile_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode builtinType_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link InlinedConditionProfile} valueFound
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile builtinType_valueFound_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link InlinedConditionProfile} noGetMethod
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile builtinType_noGetMethod_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile builtinType_errorProfile_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getDynamicAttr_getClass_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private final IsBuiltinObjectProfile getDynamicAttr_errorProfile_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectLookupAttr.class);
this.state_0_ = target.getState(0, 5);
this.readNode = target.getReference(1, ReadAttributeFromObjectNode.class);
this.lookupValueGet = target.getReference(2, LookupInheritedSlotNode.class);
this.invokeValueGet = target.getReference(3, CallTernaryMethodNode.class);
this.codePointLengthNode = target.getReference(4, CodePointLengthNode.class);
this.codePointAtIndexNode = target.getReference(5, CodePointAtIndexNode.class);
this.builtinObject_cache = target.getReference(6, BuiltinObjectData.class);
this.builtinModule_cache = target.getReference(7, BuiltinModuleData.class);
this.builtinTypeType_cache = target.getReference(8, BuiltinTypeTypeData.class);
this.builtinType_cache = target.getReference(9, BuiltinTypeData.class);
this.getDynamicAttr_cache = target.getReference(10, GetDynamicAttrData.class);
this.builtinObject_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_OBJECT__PY_OBJECT_LOOKUP_ATTR_BUILTIN_OBJECT_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinObjectData.lookup_(), "builtinObject_getClass__field1_", Node.class)));
this.builtinModule_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinModuleData.lookup_(), "builtinModule_getClass__field1_", Node.class)));
this.builtinModule_errorProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(BuiltinModuleData.lookup_(), "builtinModule_errorProfile__field1_", Node.class)));
this.builtinModule_noValueFound_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_MODULE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_MODULE_STATE_0_UPDATER.subUpdater(17, 2)));
this.builtinTypeType_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinTypeTypeData.lookup_(), "builtinTypeType_getClass__field1_", Node.class)));
this.builtinTypeType_valueFound_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER.subUpdater(17, 2)));
this.builtinTypeType_noGetMethod_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_0_UPDATER.subUpdater(19, 2)));
this.builtinTypeType_errorProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, BUILTIN_TYPE_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_TYPE_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(BuiltinTypeTypeData.lookup_(), "builtinTypeType_errorProfile__field1_", Node.class)));
this.builtinType_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(BuiltinTypeData.lookup_(), "builtinType_getClass__field1_", Node.class)));
this.builtinType_valueFound_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER.subUpdater(17, 2)));
this.builtinType_noGetMethod_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_0_UPDATER.subUpdater(19, 2)));
this.builtinType_errorProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, BUILTIN_TYPE__PY_OBJECT_LOOKUP_ATTR_BUILTIN_TYPE_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(BuiltinTypeData.lookup_(), "builtinType_errorProfile__field1_", Node.class)));
this.getDynamicAttr_getClass_ = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, GET_DYNAMIC_ATTR__PY_OBJECT_LOOKUP_ATTR_GET_DYNAMIC_ATTR_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_getClass__field1_", Node.class)));
this.getDynamicAttr_errorProfile_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, GET_DYNAMIC_ATTR__PY_OBJECT_LOOKUP_ATTR_GET_DYNAMIC_ATTR_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(GetDynamicAttrData.lookup_(), "getDynamicAttr_errorProfile__field1_", Node.class)));
}
@ExplodeLoop
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] || SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
if ((state_0 & 0b11101) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] || SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */ && arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] */) {
BuiltinObjectData s0_ = this.builtinObject_cache.get(arg0Value);
while (s0_ != null) {
{
ReadAttributeFromObjectNode readNode_ = this.readNode.get(arg0Value);
if (readNode_ != null) {
Object type__ = (this.builtinObject_getClass_.execute(s0_, arg1Value));
if ((PyObjectLookupAttr.isObjectGetAttribute(type__)) && (PyObjectLookupAttr.hasNoGetAttr(type__)) && (arg2Value_ == s0_.cachedName_)) {
Object descr__ = (s0_.lookupName_.execute(type__));
if ((PGuards.isNoValue(descr__))) {
return PyObjectLookupAttr.doBuiltinObject((VirtualFrame) frameValue, s0_, arg1Value, arg2Value_, s0_.cachedName_, this.builtinObject_getClass_, type__, s0_.lookupName_, descr__, readNode_);
}
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] */) {
BuiltinModuleData s1_ = this.builtinModule_cache.get(arg0Value);
if (s1_ != null) {
{
ReadAttributeFromObjectNode readNode_1 = this.readNode.get(arg0Value);
if (readNode_1 != null) {
Object type__1 = (this.builtinModule_getClass_.execute(s1_, arg1Value));
if ((PyObjectLookupAttr.isModuleGetAttribute(type__1)) && (PyObjectLookupAttr.hasNoGetAttr(type__1)) && (arg2Value_ == s1_.cachedName_)) {
Object descr__1 = (s1_.lookupName_.execute(type__1));
if ((PGuards.isNoValue(descr__1))) {
return PyObjectLookupAttr.doBuiltinModule((VirtualFrame) frameValue, s1_, arg1Value, arg2Value_, s1_.cachedName_, this.builtinModule_getClass_, type__1, s1_.lookupName_, descr__1, readNode_1, s1_.readGetattr_, this.builtinModule_errorProfile_, this.builtinModule_noValueFound_, s1_.callGetattr_);
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
BuiltinTypeTypeData s2_ = this.builtinTypeType_cache.get(arg0Value);
if (s2_ != null) {
{
LookupInheritedSlotNode lookupValueGet_ = this.lookupValueGet.get(arg0Value);
if (lookupValueGet_ != null) {
CallTernaryMethodNode invokeValueGet_ = this.invokeValueGet.get(arg0Value);
if (invokeValueGet_ != null) {
CodePointLengthNode codePointLengthNode_ = this.codePointLengthNode.get(arg0Value);
if (codePointLengthNode_ != null) {
CodePointAtIndexNode codePointAtIndexNode_ = this.codePointAtIndexNode.get(arg0Value);
if (codePointAtIndexNode_ != null) {
Object type__2 = (this.builtinTypeType_getClass_.execute(s2_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__2)) && (PyObjectLookupAttr.isBuiltinTypeType(type__2)) && (!(PyObjectLookupAttr.isTypeSlot(arg2Value_, codePointLengthNode_, codePointAtIndexNode_)))) {
return PyObjectLookupAttr.doBuiltinTypeType((VirtualFrame) frameValue, s2_, arg1Value, arg2Value_, this.builtinTypeType_getClass_, type__2, s2_.readNode_, this.builtinTypeType_valueFound_, lookupValueGet_, this.builtinTypeType_noGetMethod_, invokeValueGet_, this.builtinTypeType_errorProfile_, codePointLengthNode_, codePointAtIndexNode_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */) {
BuiltinTypeData s3_ = this.builtinType_cache.get(arg0Value);
if (s3_ != null) {
{
LookupInheritedSlotNode lookupValueGet_1 = this.lookupValueGet.get(arg0Value);
if (lookupValueGet_1 != null) {
CallTernaryMethodNode invokeValueGet_1 = this.invokeValueGet.get(arg0Value);
if (invokeValueGet_1 != null) {
Object type__3 = (this.builtinType_getClass_.execute(s3_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__3)) && (PyObjectLookupAttr.hasNoGetAttr(type__3)) && (arg2Value_ == s3_.cachedName_)) {
Object metaClassDescr__ = (s3_.lookupInMetaclassHierachy_.execute(type__3));
if ((PGuards.isNoValue(metaClassDescr__))) {
return PyObjectLookupAttr.doBuiltinType((VirtualFrame) frameValue, s3_, arg1Value, arg2Value_, s3_.cachedName_, this.builtinType_getClass_, type__3, s3_.lookupInMetaclassHierachy_, metaClassDescr__, s3_.readNode_, this.builtinType_valueFound_, lookupValueGet_1, this.builtinType_noGetMethod_, invokeValueGet_1, this.builtinType_errorProfile_);
}
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
GetDynamicAttrData s4_ = this.getDynamicAttr_cache.get(arg0Value);
if (s4_ != null) {
{
CodePointLengthNode codePointLengthNode_1 = this.codePointLengthNode.get(arg0Value);
if (codePointLengthNode_1 != null) {
CodePointAtIndexNode codePointAtIndexNode_1 = this.codePointAtIndexNode.get(arg0Value);
if (codePointAtIndexNode_1 != null) {
return PyObjectLookupAttr.getDynamicAttr(frameValue, s4_, arg1Value, arg2Value, this.getDynamicAttr_getClass_, s4_.lookupGetattribute_, s4_.lookupGetattr_, s4_.callGetattribute_, s4_.callGetattr_, this.getDynamicAttr_errorProfile_, codePointLengthNode_1, codePointAtIndexNode_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value);
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
{
Object descr__ = null;
Object type__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count0_ = 0;
BuiltinObjectData s0_ = this.builtinObject_cache.getVolatile(arg0Value);
BuiltinObjectData s0_original = s0_;
while (s0_ != null) {
{
ReadAttributeFromObjectNode readNode_ = this.readNode.get(arg0Value);
if (readNode_ != null) {
type__ = (this.builtinObject_getClass_.execute(s0_, arg1Value));
if ((PyObjectLookupAttr.isObjectGetAttribute(type__)) && (PyObjectLookupAttr.hasNoGetAttr(type__)) && (arg2Value_ == s0_.cachedName_)) {
descr__ = (s0_.lookupName_.execute(type__));
if ((PGuards.isNoValue(descr__))) {
break;
}
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
s0_ = arg0Value.insert(new BuiltinObjectData(s0_original));
type__ = (this.builtinObject_getClass_.execute(s0_, arg1Value));
if ((PyObjectLookupAttr.isObjectGetAttribute(type__)) && (PyObjectLookupAttr.hasNoGetAttr(type__))) {
LookupAttributeInMRONode lookupName__ = s0_.insert((LookupAttributeInMRONode.create(arg2Value_)));
descr__ = (lookupName__.execute(type__));
// assert (arg2Value_ == s0_.cachedName_);
if ((PGuards.isNoValue(descr__)) && count0_ < (3)) {
s0_.cachedName_ = (arg2Value_);
Objects.requireNonNull(s0_.insert(lookupName__), "Specialization 'doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)' cache 'lookupName' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.lookupName_ = lookupName__;
ReadAttributeFromObjectNode readNode_;
ReadAttributeFromObjectNode readNode__shared = this.readNode.get(arg0Value);
if (readNode__shared != null) {
readNode_ = readNode__shared;
} else {
readNode_ = s0_.insert((ReadAttributeFromObjectNode.create()));
if (readNode_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)' contains a shared cache with name 'readNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readNode.get(arg0Value) == null) {
this.readNode.set(arg0Value, readNode_);
}
if (!this.builtinObject_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s0_ = null;
}
} else {
s0_ = null;
}
}
}
if (s0_ != null) {
return PyObjectLookupAttr.doBuiltinObject((VirtualFrame) frameValue, s0_, arg1Value, arg2Value_, s0_.cachedName_, this.builtinObject_getClass_, type__, s0_.lookupName_, descr__, this.readNode.get(arg0Value));
}
break;
}
}
}
{
Object descr__1 = null;
Object type__1 = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count1_ = 0;
BuiltinModuleData s1_ = this.builtinModule_cache.getVolatile(arg0Value);
BuiltinModuleData s1_original = s1_;
while (s1_ != null) {
{
ReadAttributeFromObjectNode readNode_1 = this.readNode.get(arg0Value);
if (readNode_1 != null) {
type__1 = (this.builtinModule_getClass_.execute(s1_, arg1Value));
if ((PyObjectLookupAttr.isModuleGetAttribute(type__1)) && (PyObjectLookupAttr.hasNoGetAttr(type__1)) && (arg2Value_ == s1_.cachedName_)) {
descr__1 = (s1_.lookupName_.execute(type__1));
if ((PGuards.isNoValue(descr__1))) {
break;
}
}
}
}
count1_++;
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
{
s1_ = arg0Value.insert(new BuiltinModuleData());
type__1 = (this.builtinModule_getClass_.execute(s1_, arg1Value));
if ((PyObjectLookupAttr.isModuleGetAttribute(type__1)) && (PyObjectLookupAttr.hasNoGetAttr(type__1))) {
LookupAttributeInMRONode lookupName__1 = s1_.insert((LookupAttributeInMRONode.create(arg2Value_)));
descr__1 = (lookupName__1.execute(type__1));
// assert (arg2Value_ == s1_.cachedName_);
if ((PGuards.isNoValue(descr__1))) {
s1_.cachedName_ = (arg2Value_);
Objects.requireNonNull(s1_.insert(lookupName__1), "Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' cache 'lookupName' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.lookupName_ = lookupName__1;
ReadAttributeFromObjectNode readNode_1;
ReadAttributeFromObjectNode readNode_1_shared = this.readNode.get(arg0Value);
if (readNode_1_shared != null) {
readNode_1 = readNode_1_shared;
} else {
readNode_1 = s1_.insert((ReadAttributeFromObjectNode.create()));
if (readNode_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' contains a shared cache with name 'readNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readNode.get(arg0Value) == null) {
this.readNode.set(arg0Value, readNode_1);
}
ReadAttributeFromObjectNode readGetattr__ = s1_.insert((ReadAttributeFromObjectNode.create()));
Objects.requireNonNull(readGetattr__, "Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' cache 'readGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.readGetattr_ = readGetattr__;
CallNode callGetattr__ = s1_.insert((CallNode.create()));
Objects.requireNonNull(callGetattr__, "Specialization 'doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)' cache 'callGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.callGetattr_ = callGetattr__;
if (!this.builtinModule_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s1_ = null;
}
} else {
s1_ = null;
}
}
}
if (s1_ != null) {
return PyObjectLookupAttr.doBuiltinModule((VirtualFrame) frameValue, s1_, arg1Value, arg2Value_, s1_.cachedName_, this.builtinModule_getClass_, type__1, s1_.lookupName_, descr__1, this.readNode.get(arg0Value), s1_.readGetattr_, this.builtinModule_errorProfile_, this.builtinModule_noValueFound_, s1_.callGetattr_);
}
break;
}
}
}
{
Object type__2 = null;
if (((state_0 & 0b10010)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] && SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count2_ = 0;
BuiltinTypeTypeData s2_ = this.builtinTypeType_cache.getVolatile(arg0Value);
BuiltinTypeTypeData s2_original = s2_;
while (s2_ != null) {
{
LookupInheritedSlotNode lookupValueGet_ = this.lookupValueGet.get(arg0Value);
if (lookupValueGet_ != null) {
CallTernaryMethodNode invokeValueGet_ = this.invokeValueGet.get(arg0Value);
if (invokeValueGet_ != null) {
CodePointLengthNode codePointLengthNode_ = this.codePointLengthNode.get(arg0Value);
if (codePointLengthNode_ != null) {
CodePointAtIndexNode codePointAtIndexNode_ = this.codePointAtIndexNode.get(arg0Value);
if (codePointAtIndexNode_ != null) {
type__2 = (this.builtinTypeType_getClass_.execute(s2_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__2)) && (PyObjectLookupAttr.isBuiltinTypeType(type__2)) && (!(PyObjectLookupAttr.isTypeSlot(arg2Value_, codePointLengthNode_, codePointAtIndexNode_)))) {
break;
}
}
}
}
}
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
{
s2_ = arg0Value.insert(new BuiltinTypeTypeData());
type__2 = (this.builtinTypeType_getClass_.execute(s2_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__2)) && (PyObjectLookupAttr.isBuiltinTypeType(type__2))) {
CodePointLengthNode codePointLengthNode_;
CodePointLengthNode codePointLengthNode__shared = this.codePointLengthNode.get(arg0Value);
if (codePointLengthNode__shared != null) {
codePointLengthNode_ = codePointLengthNode__shared;
} else {
codePointLengthNode_ = s2_.insert((CodePointLengthNode.create()));
if (codePointLengthNode_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
CodePointAtIndexNode codePointAtIndexNode_;
CodePointAtIndexNode codePointAtIndexNode__shared = this.codePointAtIndexNode.get(arg0Value);
if (codePointAtIndexNode__shared != null) {
codePointAtIndexNode_ = codePointAtIndexNode__shared;
} else {
codePointAtIndexNode_ = s2_.insert((CodePointAtIndexNode.create()));
if (codePointAtIndexNode_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((!(PyObjectLookupAttr.isTypeSlot(arg2Value_, codePointLengthNode_, codePointAtIndexNode_)))) {
Dynamic readNode__ = s2_.insert((Dynamic.create()));
Objects.requireNonNull(readNode__, "Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'readNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.readNode_ = readNode__;
LookupInheritedSlotNode lookupValueGet_;
LookupInheritedSlotNode lookupValueGet__shared = this.lookupValueGet.get(arg0Value);
if (lookupValueGet__shared != null) {
lookupValueGet_ = lookupValueGet__shared;
} else {
lookupValueGet_ = s2_.insert((LookupInheritedSlotNode.create(SpecialMethodSlot.Get)));
if (lookupValueGet_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'lookupValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupValueGet.get(arg0Value) == null) {
this.lookupValueGet.set(arg0Value, lookupValueGet_);
}
CallTernaryMethodNode invokeValueGet_;
CallTernaryMethodNode invokeValueGet__shared = this.invokeValueGet.get(arg0Value);
if (invokeValueGet__shared != null) {
invokeValueGet_ = invokeValueGet__shared;
} else {
invokeValueGet_ = s2_.insert((CallTernaryMethodNode.create()));
if (invokeValueGet_ == null) {
throw new IllegalStateException("Specialization 'doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'invokeValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invokeValueGet.get(arg0Value) == null) {
this.invokeValueGet.set(arg0Value, invokeValueGet_);
}
if (this.codePointLengthNode.get(arg0Value) == null) {
this.codePointLengthNode.set(arg0Value, codePointLengthNode_);
}
if (this.codePointAtIndexNode.get(arg0Value) == null) {
this.codePointAtIndexNode.set(arg0Value, codePointAtIndexNode_);
}
if (!this.builtinTypeType_cache.compareAndSet(arg0Value, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s2_ = null;
}
} else {
s2_ = null;
}
}
}
if (s2_ != null) {
return PyObjectLookupAttr.doBuiltinTypeType((VirtualFrame) frameValue, s2_, arg1Value, arg2Value_, this.builtinTypeType_getClass_, type__2, s2_.readNode_, this.builtinTypeType_valueFound_, this.lookupValueGet.get(arg0Value), this.builtinTypeType_noGetMethod_, this.invokeValueGet.get(arg0Value), this.builtinTypeType_errorProfile_, this.codePointLengthNode.get(arg0Value), this.codePointAtIndexNode.get(arg0Value));
}
break;
}
}
}
{
Object metaClassDescr__ = null;
Object type__3 = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */) {
while (true) {
int count3_ = 0;
BuiltinTypeData s3_ = this.builtinType_cache.getVolatile(arg0Value);
BuiltinTypeData s3_original = s3_;
while (s3_ != null) {
{
LookupInheritedSlotNode lookupValueGet_1 = this.lookupValueGet.get(arg0Value);
if (lookupValueGet_1 != null) {
CallTernaryMethodNode invokeValueGet_1 = this.invokeValueGet.get(arg0Value);
if (invokeValueGet_1 != null) {
type__3 = (this.builtinType_getClass_.execute(s3_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__3)) && (PyObjectLookupAttr.hasNoGetAttr(type__3)) && (arg2Value_ == s3_.cachedName_)) {
metaClassDescr__ = (s3_.lookupInMetaclassHierachy_.execute(type__3));
if ((PGuards.isNoValue(metaClassDescr__))) {
break;
}
}
}
}
}
count3_++;
s3_ = null;
break;
}
if (s3_ == null && count3_ < 1) {
{
s3_ = arg0Value.insert(new BuiltinTypeData());
type__3 = (this.builtinType_getClass_.execute(s3_, arg1Value));
if ((PyObjectLookupAttr.isTypeGetAttribute(type__3)) && (PyObjectLookupAttr.hasNoGetAttr(type__3))) {
LookupAttributeInMRONode lookupInMetaclassHierachy__ = s3_.insert((LookupAttributeInMRONode.create(arg2Value_)));
metaClassDescr__ = (lookupInMetaclassHierachy__.execute(type__3));
// assert (arg2Value_ == s3_.cachedName_);
if ((PGuards.isNoValue(metaClassDescr__))) {
s3_.cachedName_ = (arg2Value_);
Objects.requireNonNull(s3_.insert(lookupInMetaclassHierachy__), "Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' cache 'lookupInMetaclassHierachy' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.lookupInMetaclassHierachy_ = lookupInMetaclassHierachy__;
LookupAttributeInMRONode readNode__1 = s3_.insert((LookupAttributeInMRONode.create(arg2Value_)));
Objects.requireNonNull(readNode__1, "Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' cache 'readNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.readNode_ = readNode__1;
LookupInheritedSlotNode lookupValueGet_1;
LookupInheritedSlotNode lookupValueGet_1_shared = this.lookupValueGet.get(arg0Value);
if (lookupValueGet_1_shared != null) {
lookupValueGet_1 = lookupValueGet_1_shared;
} else {
lookupValueGet_1 = s3_.insert((LookupInheritedSlotNode.create(SpecialMethodSlot.Get)));
if (lookupValueGet_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' contains a shared cache with name 'lookupValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupValueGet.get(arg0Value) == null) {
this.lookupValueGet.set(arg0Value, lookupValueGet_1);
}
CallTernaryMethodNode invokeValueGet_1;
CallTernaryMethodNode invokeValueGet_1_shared = this.invokeValueGet.get(arg0Value);
if (invokeValueGet_1_shared != null) {
invokeValueGet_1 = invokeValueGet_1_shared;
} else {
invokeValueGet_1 = s3_.insert((CallTernaryMethodNode.create()));
if (invokeValueGet_1 == null) {
throw new IllegalStateException("Specialization 'doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)' contains a shared cache with name 'invokeValueGet' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invokeValueGet.get(arg0Value) == null) {
this.invokeValueGet.set(arg0Value, invokeValueGet_1);
}
if (!this.builtinType_cache.compareAndSet(arg0Value, s3_original, s3_)) {
continue;
}
this.builtinTypeType_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffff7 /* remove SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */;
this.state_0_.set(arg0Value, state_0);
} else {
s3_ = null;
}
} else {
s3_ = null;
}
}
}
if (s3_ != null) {
return PyObjectLookupAttr.doBuiltinType((VirtualFrame) frameValue, s3_, arg1Value, arg2Value_, s3_.cachedName_, this.builtinType_getClass_, type__3, s3_.lookupInMetaclassHierachy_, metaClassDescr__, s3_.readNode_, this.builtinType_valueFound_, this.lookupValueGet.get(arg0Value), this.builtinType_noGetMethod_, this.invokeValueGet.get(arg0Value), this.builtinType_errorProfile_);
}
break;
}
}
}
}
GetDynamicAttrData s4_ = arg0Value.insert(new GetDynamicAttrData());
LookupSpecialMethodSlotNode lookupGetattribute__ = s4_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
Objects.requireNonNull(lookupGetattribute__, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'lookupGetattribute' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.lookupGetattribute_ = lookupGetattribute__;
LookupSpecialMethodSlotNode lookupGetattr__ = s4_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttr)));
Objects.requireNonNull(lookupGetattr__, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'lookupGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.lookupGetattr_ = lookupGetattr__;
CallBinaryMethodNode callGetattribute__ = s4_.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callGetattribute__, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'callGetattribute' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callGetattribute_ = callGetattribute__;
CallBinaryMethodNode callGetattr__1 = s4_.insert((CallBinaryMethodNode.create()));
Objects.requireNonNull(callGetattr__1, "Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' cache 'callGetattr' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s4_.callGetattr_ = callGetattr__1;
CodePointLengthNode codePointLengthNode_1;
CodePointLengthNode codePointLengthNode_1_shared = this.codePointLengthNode.get(arg0Value);
if (codePointLengthNode_1_shared != null) {
codePointLengthNode_1 = codePointLengthNode_1_shared;
} else {
codePointLengthNode_1 = s4_.insert((CodePointLengthNode.create()));
if (codePointLengthNode_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointLengthNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.codePointLengthNode.get(arg0Value) == null) {
this.codePointLengthNode.set(arg0Value, codePointLengthNode_1);
}
CodePointAtIndexNode codePointAtIndexNode_1;
CodePointAtIndexNode codePointAtIndexNode_1_shared = this.codePointAtIndexNode.get(arg0Value);
if (codePointAtIndexNode_1_shared != null) {
codePointAtIndexNode_1 = codePointAtIndexNode_1_shared;
} else {
codePointAtIndexNode_1 = s4_.insert((CodePointAtIndexNode.create()));
if (codePointAtIndexNode_1 == null) {
throw new IllegalStateException("Specialization 'getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)' contains a shared cache with name 'codePointAtIndexNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.codePointAtIndexNode.get(arg0Value) == null) {
this.codePointAtIndexNode.set(arg0Value, codePointAtIndexNode_1);
}
VarHandle.storeStoreFence();
this.getDynamicAttr_cache.set(arg0Value, s4_);
this.builtinObject_cache.set(arg0Value, null);
this.builtinModule_cache.set(arg0Value, null);
this.builtinTypeType_cache.set(arg0Value, null);
this.builtinType_cache.set(arg0Value, null);
state_0 = state_0 & 0xffffffe2 /* remove SpecializationActive[PyObjectLookupAttr.doBuiltinObject(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode)], SpecializationActive[PyObjectLookupAttr.doBuiltinModule(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, ReadAttributeFromObjectNode, ReadAttributeFromObjectNode, IsBuiltinObjectProfile, InlinedConditionProfile, CallNode)], SpecializationActive[PyObjectLookupAttr.doBuiltinTypeType(VirtualFrame, Node, Object, TruffleString, GetClassNode, Object, Dynamic, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)], SpecializationActive[PyObjectLookupAttr.doBuiltinType(VirtualFrame, Node, Object, TruffleString, TruffleString, GetClassNode, Object, LookupAttributeInMRONode, Object, LookupAttributeInMRONode, InlinedConditionProfile, LookupInheritedSlotNode, InlinedConditionProfile, CallTernaryMethodNode, IsBuiltinObjectProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectLookupAttr.getDynamicAttr(Frame, Node, Object, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, CallBinaryMethodNode, CallBinaryMethodNode, IsBuiltinObjectProfile, CodePointLengthNode, CodePointAtIndexNode)] */;
this.state_0_.set(arg0Value, state_0);
return PyObjectLookupAttr.getDynamicAttr(frameValue, s4_, arg1Value, arg2Value, this.getDynamicAttr_getClass_, lookupGetattribute__, lookupGetattr__, callGetattribute__, callGetattr__1, this.getDynamicAttr_errorProfile_, codePointLengthNode_1, codePointAtIndexNode_1);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class BuiltinObjectData extends Node implements SpecializationDataNode {
@Child BuiltinObjectData next_;
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinObject_state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinObject_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinObject}
* Parameter: {@link LookupAttributeInMRONode} lookupName
*/
@Child LookupAttributeInMRONode lookupName_;
BuiltinObjectData(BuiltinObjectData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class BuiltinModuleData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 17-18: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link InlinedConditionProfile} noValueFound
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinModule_state_0_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinModule_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinModule_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link LookupAttributeInMRONode} lookupName
*/
@Child LookupAttributeInMRONode lookupName_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link ReadAttributeFromObjectNode} readGetattr
*/
@Child ReadAttributeFromObjectNode readGetattr_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinModule_errorProfile__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinModule}
* Parameter: {@link CallNode} callGetattr
*/
@Child CallNode callGetattr_;
BuiltinModuleData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class BuiltinTypeTypeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 17-18: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link InlinedConditionProfile} valueFound
* Inline method: {@link InlinedConditionProfile#inline}
* 19-20: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link InlinedConditionProfile} noGetMethod
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinTypeType_state_0_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinTypeType_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinTypeType_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link Dynamic} readNode
*/
@Child Dynamic readNode_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinTypeType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinTypeType_errorProfile__field1_;
BuiltinTypeTypeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class BuiltinTypeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 17-18: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link InlinedConditionProfile} valueFound
* Inline method: {@link InlinedConditionProfile#inline}
* 19-20: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link InlinedConditionProfile} noGetMethod
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinType_state_0_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int builtinType_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinType_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link LookupAttributeInMRONode} lookupInMetaclassHierachy
*/
@Child LookupAttributeInMRONode lookupInMetaclassHierachy_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link LookupAttributeInMRONode} readNode
*/
@Child LookupAttributeInMRONode readNode_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#doBuiltinType}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node builtinType_errorProfile__field1_;
BuiltinTypeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class GetDynamicAttrData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getDynamicAttr_state_0_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getDynamicAttr_state_1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getDynamicAttr_getClass__field1_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupGetattribute
*/
@Child LookupSpecialMethodSlotNode lookupGetattribute_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupGetattr
*/
@Child LookupSpecialMethodSlotNode lookupGetattr_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link CallBinaryMethodNode} callGetattribute
*/
@Child CallBinaryMethodNode callGetattribute_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link CallBinaryMethodNode} callGetattr
*/
@Child CallBinaryMethodNode callGetattr_;
/**
* Source Info:
* Specialization: {@link PyObjectLookupAttr#getDynamicAttr}
* Parameter: {@link IsBuiltinObjectProfile} errorProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getDynamicAttr_errorProfile__field1_;
GetDynamicAttrData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PyObjectLookupAttr.class)
@DenyReplace
private static final class Uncached extends PyObjectLookupAttr {
@Override
public Object execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return PyObjectLookupAttr.getDynamicAttr(frameValue, arg0Value, arg1Value, arg2Value, (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.GetAttribute)), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.GetAttr)), (CallBinaryMethodNode.getUncached()), (CallBinaryMethodNode.getUncached()), (IsBuiltinObjectProfile.getUncached()), (CodePointLengthNode.getUncached()), (CodePointAtIndexNode.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy