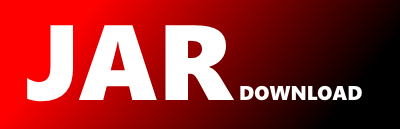
com.oracle.graal.python.lib.PyObjectSetAttrNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.lib;
import com.oracle.graal.python.builtins.objects.type.SpecialMethodSlot;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.call.special.CallBinaryMethodNode;
import com.oracle.graal.python.nodes.call.special.CallTernaryMethodNode;
import com.oracle.graal.python.nodes.call.special.LookupSpecialMethodSlotNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link PyObjectSetAttr#setFixedAttr}
* Activation probability: 0.38500
* With/without class size: 11/4 bytes
* Specialization {@link PyObjectSetAttr#delFixedAttr}
* Activation probability: 0.29500
* With/without class size: 9/4 bytes
* Specialization {@link PyObjectSetAttr#doDynamicAttr}
* Activation probability: 0.20500
* With/without class size: 7/0 bytes
* Specialization {@link PyObjectSetAttr#nameMustBeString}
* Activation probability: 0.11500
* With/without class size: 5/0 bytes
*
*/
@GeneratedBy(PyObjectSetAttr.class)
@SuppressWarnings("javadoc")
public final class PyObjectSetAttrNodeGen extends PyObjectSetAttr {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField SET_FIXED_ATTR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "setFixedAttr_cache", SetFixedAttrData.class);
static final ReferenceField DEL_FIXED_ATTR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "delFixedAttr_cache", DelFixedAttrData.class);
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private static final GetClassNode INLINED_GET_CLASS = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, STATE_0_UPDATER.subUpdater(4, 17), ReferenceField.create(MethodHandles.lookup(), "getClass_field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_RAISE = LazyNodeGen.inline(InlineTarget.create(Lazy.class, STATE_0_UPDATER.subUpdater(21, 1), ReferenceField.create(MethodHandles.lookup(), "raise_field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link PyObjectSetAttr#setFixedAttr}
* 1: SpecializationActive {@link PyObjectSetAttr#doDynamicAttr}
* 2: SpecializationActive {@link PyObjectSetAttr#delFixedAttr}
* 3: SpecializationActive {@link PyObjectSetAttr#nameMustBeString}
* 4-20: InlinedCache
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 21: InlinedCache
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node getClass_field1_;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupSetattr
*/
@Child private LookupSpecialMethodSlotNode lookup;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupGetattr
*/
@Child private LookupSpecialMethodSlotNode lookupGet;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node raise_field1_;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link CallTernaryMethodNode} callSetattr
*/
@Child private CallTernaryMethodNode call;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#delFixedAttr}
* Parameter: {@link LookupSpecialMethodSlotNode} lookupDelattr
*/
@Child private LookupSpecialMethodSlotNode lookupDel;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#delFixedAttr}
* Parameter: {@link CallBinaryMethodNode} callDelattr
*/
@Child private CallBinaryMethodNode callDel;
@UnsafeAccessedField @Child private SetFixedAttrData setFixedAttr_cache;
@UnsafeAccessedField @Child private DelFixedAttrData delFixedAttr_cache;
private PyObjectSetAttrNodeGen() {
}
@Override
public void execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] || SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] || SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] || SpecializationActive[PyObjectSetAttr.nameMustBeString(Node, Object, Object, Object, Lazy)] */) {
if ((state_0 & 0b111) != 0 /* is SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] || SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] || SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */ && arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] */) {
SetFixedAttrData s0_ = this.setFixedAttr_cache;
if (s0_ != null) {
{
LookupSpecialMethodSlotNode lookup_ = this.lookup;
if (lookup_ != null) {
LookupSpecialMethodSlotNode lookupGet_ = this.lookupGet;
if (lookupGet_ != null) {
CallTernaryMethodNode call_ = this.call;
if (call_ != null) {
if ((arg2Value_ == s0_.cachedName_) && (arg3Value != null)) {
PyObjectSetAttr.setFixedAttr(frameValue, this, arg1Value, arg2Value_, arg3Value, s0_.cachedName_, INLINED_GET_CLASS, lookup_, lookupGet_, INLINED_RAISE, call_);
return;
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] */) {
DelFixedAttrData s1_ = this.delFixedAttr_cache;
if (s1_ != null) {
{
LookupSpecialMethodSlotNode lookupDel_ = this.lookupDel;
if (lookupDel_ != null) {
LookupSpecialMethodSlotNode lookupGet_1 = this.lookupGet;
if (lookupGet_1 != null) {
CallBinaryMethodNode callDel_ = this.callDel;
if (callDel_ != null) {
if ((arg2Value_ == s1_.cachedName_) && (arg3Value == null)) {
PyObjectSetAttr.delFixedAttr(frameValue, this, arg1Value, arg2Value_, arg3Value, s1_.cachedName_, INLINED_GET_CLASS, lookupDel_, lookupGet_1, INLINED_RAISE, callDel_);
return;
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */) {
{
LookupSpecialMethodSlotNode lookup_1 = this.lookup;
if (lookup_1 != null) {
LookupSpecialMethodSlotNode lookupDel_1 = this.lookupDel;
if (lookupDel_1 != null) {
LookupSpecialMethodSlotNode lookupGet_2 = this.lookupGet;
if (lookupGet_2 != null) {
CallTernaryMethodNode call_1 = this.call;
if (call_1 != null) {
CallBinaryMethodNode callDel_1 = this.callDel;
if (callDel_1 != null) {
PyObjectSetAttr.doDynamicAttr(frameValue, this, arg1Value, arg2Value_, arg3Value, INLINED_GET_CLASS, lookup_1, lookupDel_1, lookupGet_2, INLINED_RAISE, call_1, callDel_1);
return;
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectSetAttr.nameMustBeString(Node, Object, Object, Object, Lazy)] */) {
if ((!(PGuards.isString(arg2Value)))) {
PyObjectSetAttr.nameMustBeString(this, arg1Value, arg2Value, arg3Value, INLINED_RAISE);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_;
if (arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */) {
while (true) {
int count0_ = 0;
SetFixedAttrData s0_ = SET_FIXED_ATTR_CACHE_UPDATER.getVolatile(this);
SetFixedAttrData s0_original = s0_;
while (s0_ != null) {
{
LookupSpecialMethodSlotNode lookup_ = this.lookup;
if (lookup_ != null) {
LookupSpecialMethodSlotNode lookupGet_ = this.lookupGet;
if (lookupGet_ != null) {
CallTernaryMethodNode call_ = this.call;
if (call_ != null) {
if ((arg2Value_ == s0_.cachedName_) && (arg3Value != null)) {
break;
}
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((arg3Value != null)) {
// assert (arg2Value_ == s0_.cachedName_);
s0_ = this.insert(new SetFixedAttrData());
s0_.cachedName_ = (arg2Value_);
LookupSpecialMethodSlotNode lookup_;
LookupSpecialMethodSlotNode lookup__shared = this.lookup;
if (lookup__shared != null) {
lookup_ = lookup__shared;
} else {
lookup_ = s0_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.SetAttr)));
if (lookup_ == null) {
throw new IllegalStateException("Specialization 'setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)' contains a shared cache with name 'lookupSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookup == null) {
this.lookup = lookup_;
}
LookupSpecialMethodSlotNode lookupGet_;
LookupSpecialMethodSlotNode lookupGet__shared = this.lookupGet;
if (lookupGet__shared != null) {
lookupGet_ = lookupGet__shared;
} else {
lookupGet_ = s0_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
if (lookupGet_ == null) {
throw new IllegalStateException("Specialization 'setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)' contains a shared cache with name 'lookupGetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet == null) {
this.lookupGet = lookupGet_;
}
CallTernaryMethodNode call_;
CallTernaryMethodNode call__shared = this.call;
if (call__shared != null) {
call_ = call__shared;
} else {
call_ = s0_.insert((CallTernaryMethodNode.create()));
if (call_ == null) {
throw new IllegalStateException("Specialization 'setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)' contains a shared cache with name 'callSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.call == null) {
this.call = call_;
}
if (!SET_FIXED_ATTR_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] */;
this.state_0_ = state_0;
}
}
if (s0_ != null) {
PyObjectSetAttr.setFixedAttr(frameValue, this, arg1Value, arg2Value_, arg3Value, s0_.cachedName_, INLINED_GET_CLASS, this.lookup, this.lookupGet, INLINED_RAISE, this.call);
return;
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */) {
while (true) {
int count1_ = 0;
DelFixedAttrData s1_ = DEL_FIXED_ATTR_CACHE_UPDATER.getVolatile(this);
DelFixedAttrData s1_original = s1_;
while (s1_ != null) {
{
LookupSpecialMethodSlotNode lookupDel_ = this.lookupDel;
if (lookupDel_ != null) {
LookupSpecialMethodSlotNode lookupGet_1 = this.lookupGet;
if (lookupGet_1 != null) {
CallBinaryMethodNode callDel_ = this.callDel;
if (callDel_ != null) {
if ((arg2Value_ == s1_.cachedName_) && (arg3Value == null)) {
break;
}
}
}
}
}
count1_++;
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
if ((arg3Value == null)) {
// assert (arg2Value_ == s1_.cachedName_);
s1_ = this.insert(new DelFixedAttrData());
s1_.cachedName_ = (arg2Value_);
LookupSpecialMethodSlotNode lookupDel_;
LookupSpecialMethodSlotNode lookupDel__shared = this.lookupDel;
if (lookupDel__shared != null) {
lookupDel_ = lookupDel__shared;
} else {
lookupDel_ = s1_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.DelAttr)));
if (lookupDel_ == null) {
throw new IllegalStateException("Specialization 'delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)' contains a shared cache with name 'lookupDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupDel == null) {
this.lookupDel = lookupDel_;
}
LookupSpecialMethodSlotNode lookupGet_1;
LookupSpecialMethodSlotNode lookupGet_1_shared = this.lookupGet;
if (lookupGet_1_shared != null) {
lookupGet_1 = lookupGet_1_shared;
} else {
lookupGet_1 = s1_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
if (lookupGet_1 == null) {
throw new IllegalStateException("Specialization 'delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)' contains a shared cache with name 'lookupGetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet == null) {
this.lookupGet = lookupGet_1;
}
CallBinaryMethodNode callDel_;
CallBinaryMethodNode callDel__shared = this.callDel;
if (callDel__shared != null) {
callDel_ = callDel__shared;
} else {
callDel_ = s1_.insert((CallBinaryMethodNode.create()));
if (callDel_ == null) {
throw new IllegalStateException("Specialization 'delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)' contains a shared cache with name 'callDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callDel == null) {
this.callDel = callDel_;
}
if (!DEL_FIXED_ATTR_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] */;
this.state_0_ = state_0;
}
}
if (s1_ != null) {
PyObjectSetAttr.delFixedAttr(frameValue, this, arg1Value, arg2Value_, arg3Value, s1_.cachedName_, INLINED_GET_CLASS, this.lookupDel, this.lookupGet, INLINED_RAISE, this.callDel);
return;
}
break;
}
}
LookupSpecialMethodSlotNode lookup_1;
LookupSpecialMethodSlotNode lookup_1_shared = this.lookup;
if (lookup_1_shared != null) {
lookup_1 = lookup_1_shared;
} else {
lookup_1 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.SetAttr)));
if (lookup_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'lookupSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookup == null) {
VarHandle.storeStoreFence();
this.lookup = lookup_1;
}
LookupSpecialMethodSlotNode lookupDel_1;
LookupSpecialMethodSlotNode lookupDel_1_shared = this.lookupDel;
if (lookupDel_1_shared != null) {
lookupDel_1 = lookupDel_1_shared;
} else {
lookupDel_1 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.DelAttr)));
if (lookupDel_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'lookupDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupDel == null) {
VarHandle.storeStoreFence();
this.lookupDel = lookupDel_1;
}
LookupSpecialMethodSlotNode lookupGet_2;
LookupSpecialMethodSlotNode lookupGet_2_shared = this.lookupGet;
if (lookupGet_2_shared != null) {
lookupGet_2 = lookupGet_2_shared;
} else {
lookupGet_2 = this.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
if (lookupGet_2 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'lookupGetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet == null) {
VarHandle.storeStoreFence();
this.lookupGet = lookupGet_2;
}
CallTernaryMethodNode call_1;
CallTernaryMethodNode call_1_shared = this.call;
if (call_1_shared != null) {
call_1 = call_1_shared;
} else {
call_1 = this.insert((CallTernaryMethodNode.create()));
if (call_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'callSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.call == null) {
VarHandle.storeStoreFence();
this.call = call_1;
}
CallBinaryMethodNode callDel_1;
CallBinaryMethodNode callDel_1_shared = this.callDel;
if (callDel_1_shared != null) {
callDel_1 = callDel_1_shared;
} else {
callDel_1 = this.insert((CallBinaryMethodNode.create()));
if (callDel_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'callDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callDel == null) {
VarHandle.storeStoreFence();
this.callDel = callDel_1;
}
this.setFixedAttr_cache = null;
this.delFixedAttr_cache = null;
state_0 = state_0 & 0xfffffffa /* remove SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)], SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */;
this.state_0_ = state_0;
PyObjectSetAttr.doDynamicAttr(frameValue, this, arg1Value, arg2Value_, arg3Value, INLINED_GET_CLASS, lookup_1, lookupDel_1, lookupGet_2, INLINED_RAISE, call_1, callDel_1);
return;
}
if ((!(PGuards.isString(arg2Value)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectSetAttr.nameMustBeString(Node, Object, Object, Object, Lazy)] */;
this.state_0_ = state_0;
PyObjectSetAttr.nameMustBeString(this, arg1Value, arg2Value, arg3Value, INLINED_RAISE);
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b1111) & ((state_0 & 0b1111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static PyObjectSetAttr create() {
return new PyObjectSetAttrNodeGen();
}
@NeverDefault
public static PyObjectSetAttr getUncached() {
return PyObjectSetAttrNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#getClass_field1_}
*
- {@link Inlined#lookup}
*
- {@link Inlined#lookupGet}
*
- {@link Inlined#raise_field1_}
*
- {@link Inlined#call}
*
- {@link Inlined#lookupDel}
*
- {@link Inlined#callDel}
*
- {@link Inlined#setFixedAttr_cache}
*
- {@link Inlined#delFixedAttr_cache}
*
*/
@NeverDefault
public static PyObjectSetAttr inline(@RequiredField(bits = 22, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new PyObjectSetAttrNodeGen.Inlined(target);
}
@GeneratedBy(PyObjectSetAttr.class)
@DenyReplace
private static final class Inlined extends PyObjectSetAttr {
/**
* State Info:
* 0: SpecializationActive {@link PyObjectSetAttr#setFixedAttr}
* 1: SpecializationActive {@link PyObjectSetAttr#doDynamicAttr}
* 2: SpecializationActive {@link PyObjectSetAttr#delFixedAttr}
* 3: SpecializationActive {@link PyObjectSetAttr#nameMustBeString}
* 4-20: InlinedCache
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
* 21: InlinedCache
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField getClass_field1_;
private final ReferenceField lookup;
private final ReferenceField lookupGet;
private final ReferenceField raise_field1_;
private final ReferenceField call;
private final ReferenceField lookupDel;
private final ReferenceField callDel;
private final ReferenceField setFixedAttr_cache;
private final ReferenceField delFixedAttr_cache;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link GetClassNode} getClass
* Inline method: {@link GetClassNodeGen#inline}
*/
private final GetClassNode getClass;
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link Lazy} raise
* Inline method: {@link LazyNodeGen#inline}
*/
private final Lazy raise;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(PyObjectSetAttr.class);
this.state_0_ = target.getState(0, 22);
this.getClass_field1_ = target.getReference(1, Node.class);
this.lookup = target.getReference(2, LookupSpecialMethodSlotNode.class);
this.lookupGet = target.getReference(3, LookupSpecialMethodSlotNode.class);
this.raise_field1_ = target.getReference(4, Node.class);
this.call = target.getReference(5, CallTernaryMethodNode.class);
this.lookupDel = target.getReference(6, LookupSpecialMethodSlotNode.class);
this.callDel = target.getReference(7, CallBinaryMethodNode.class);
this.setFixedAttr_cache = target.getReference(8, SetFixedAttrData.class);
this.delFixedAttr_cache = target.getReference(9, DelFixedAttrData.class);
this.getClass = GetClassNodeGen.inline(InlineTarget.create(GetClassNode.class, state_0_.subUpdater(4, 17), getClass_field1_));
this.raise = LazyNodeGen.inline(InlineTarget.create(Lazy.class, state_0_.subUpdater(21, 1), raise_field1_));
}
@Override
public void execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] || SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] || SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] || SpecializationActive[PyObjectSetAttr.nameMustBeString(Node, Object, Object, Object, Lazy)] */) {
if ((state_0 & 0b111) != 0 /* is SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] || SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] || SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */ && arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] */) {
SetFixedAttrData s0_ = this.setFixedAttr_cache.get(arg0Value);
if (s0_ != null) {
{
LookupSpecialMethodSlotNode lookup_ = this.lookup.get(arg0Value);
if (lookup_ != null) {
LookupSpecialMethodSlotNode lookupGet_ = this.lookupGet.get(arg0Value);
if (lookupGet_ != null) {
CallTernaryMethodNode call_ = this.call.get(arg0Value);
if (call_ != null) {
if ((arg2Value_ == s0_.cachedName_) && (arg3Value != null)) {
PyObjectSetAttr.setFixedAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, s0_.cachedName_, this.getClass, lookup_, lookupGet_, this.raise, call_);
return;
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] */) {
DelFixedAttrData s1_ = this.delFixedAttr_cache.get(arg0Value);
if (s1_ != null) {
{
LookupSpecialMethodSlotNode lookupDel_ = this.lookupDel.get(arg0Value);
if (lookupDel_ != null) {
LookupSpecialMethodSlotNode lookupGet_1 = this.lookupGet.get(arg0Value);
if (lookupGet_1 != null) {
CallBinaryMethodNode callDel_ = this.callDel.get(arg0Value);
if (callDel_ != null) {
if ((arg2Value_ == s1_.cachedName_) && (arg3Value == null)) {
PyObjectSetAttr.delFixedAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, s1_.cachedName_, this.getClass, lookupDel_, lookupGet_1, this.raise, callDel_);
return;
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */) {
{
LookupSpecialMethodSlotNode lookup_1 = this.lookup.get(arg0Value);
if (lookup_1 != null) {
LookupSpecialMethodSlotNode lookupDel_1 = this.lookupDel.get(arg0Value);
if (lookupDel_1 != null) {
LookupSpecialMethodSlotNode lookupGet_2 = this.lookupGet.get(arg0Value);
if (lookupGet_2 != null) {
CallTernaryMethodNode call_1 = this.call.get(arg0Value);
if (call_1 != null) {
CallBinaryMethodNode callDel_1 = this.callDel.get(arg0Value);
if (callDel_1 != null) {
PyObjectSetAttr.doDynamicAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, this.getClass, lookup_1, lookupDel_1, lookupGet_2, this.raise, call_1, callDel_1);
return;
}
}
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[PyObjectSetAttr.nameMustBeString(Node, Object, Object, Object, Lazy)] */) {
if ((!(PGuards.isString(arg2Value)))) {
PyObjectSetAttr.nameMustBeString(arg0Value, arg1Value, arg2Value, arg3Value, this.raise);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void executeAndSpecialize(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */) {
while (true) {
int count0_ = 0;
SetFixedAttrData s0_ = this.setFixedAttr_cache.getVolatile(arg0Value);
SetFixedAttrData s0_original = s0_;
while (s0_ != null) {
{
LookupSpecialMethodSlotNode lookup_ = this.lookup.get(arg0Value);
if (lookup_ != null) {
LookupSpecialMethodSlotNode lookupGet_ = this.lookupGet.get(arg0Value);
if (lookupGet_ != null) {
CallTernaryMethodNode call_ = this.call.get(arg0Value);
if (call_ != null) {
if ((arg2Value_ == s0_.cachedName_) && (arg3Value != null)) {
break;
}
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((arg3Value != null)) {
// assert (arg2Value_ == s0_.cachedName_);
s0_ = arg0Value.insert(new SetFixedAttrData());
s0_.cachedName_ = (arg2Value_);
LookupSpecialMethodSlotNode lookup_;
LookupSpecialMethodSlotNode lookup__shared = this.lookup.get(arg0Value);
if (lookup__shared != null) {
lookup_ = lookup__shared;
} else {
lookup_ = s0_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.SetAttr)));
if (lookup_ == null) {
throw new IllegalStateException("Specialization 'setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)' contains a shared cache with name 'lookupSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookup.get(arg0Value) == null) {
this.lookup.set(arg0Value, lookup_);
}
LookupSpecialMethodSlotNode lookupGet_;
LookupSpecialMethodSlotNode lookupGet__shared = this.lookupGet.get(arg0Value);
if (lookupGet__shared != null) {
lookupGet_ = lookupGet__shared;
} else {
lookupGet_ = s0_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
if (lookupGet_ == null) {
throw new IllegalStateException("Specialization 'setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)' contains a shared cache with name 'lookupGetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet.get(arg0Value) == null) {
this.lookupGet.set(arg0Value, lookupGet_);
}
CallTernaryMethodNode call_;
CallTernaryMethodNode call__shared = this.call.get(arg0Value);
if (call__shared != null) {
call_ = call__shared;
} else {
call_ = s0_.insert((CallTernaryMethodNode.create()));
if (call_ == null) {
throw new IllegalStateException("Specialization 'setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)' contains a shared cache with name 'callSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.call.get(arg0Value) == null) {
this.call.set(arg0Value, call_);
}
if (!this.setFixedAttr_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
PyObjectSetAttr.setFixedAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, s0_.cachedName_, this.getClass, this.lookup.get(arg0Value), this.lookupGet.get(arg0Value), this.raise, this.call.get(arg0Value));
return;
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */) {
while (true) {
int count1_ = 0;
DelFixedAttrData s1_ = this.delFixedAttr_cache.getVolatile(arg0Value);
DelFixedAttrData s1_original = s1_;
while (s1_ != null) {
{
LookupSpecialMethodSlotNode lookupDel_ = this.lookupDel.get(arg0Value);
if (lookupDel_ != null) {
LookupSpecialMethodSlotNode lookupGet_1 = this.lookupGet.get(arg0Value);
if (lookupGet_1 != null) {
CallBinaryMethodNode callDel_ = this.callDel.get(arg0Value);
if (callDel_ != null) {
if ((arg2Value_ == s1_.cachedName_) && (arg3Value == null)) {
break;
}
}
}
}
}
count1_++;
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
if ((arg3Value == null)) {
// assert (arg2Value_ == s1_.cachedName_);
s1_ = arg0Value.insert(new DelFixedAttrData());
s1_.cachedName_ = (arg2Value_);
LookupSpecialMethodSlotNode lookupDel_;
LookupSpecialMethodSlotNode lookupDel__shared = this.lookupDel.get(arg0Value);
if (lookupDel__shared != null) {
lookupDel_ = lookupDel__shared;
} else {
lookupDel_ = s1_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.DelAttr)));
if (lookupDel_ == null) {
throw new IllegalStateException("Specialization 'delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)' contains a shared cache with name 'lookupDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupDel.get(arg0Value) == null) {
this.lookupDel.set(arg0Value, lookupDel_);
}
LookupSpecialMethodSlotNode lookupGet_1;
LookupSpecialMethodSlotNode lookupGet_1_shared = this.lookupGet.get(arg0Value);
if (lookupGet_1_shared != null) {
lookupGet_1 = lookupGet_1_shared;
} else {
lookupGet_1 = s1_.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
if (lookupGet_1 == null) {
throw new IllegalStateException("Specialization 'delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)' contains a shared cache with name 'lookupGetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet.get(arg0Value) == null) {
this.lookupGet.set(arg0Value, lookupGet_1);
}
CallBinaryMethodNode callDel_;
CallBinaryMethodNode callDel__shared = this.callDel.get(arg0Value);
if (callDel__shared != null) {
callDel_ = callDel__shared;
} else {
callDel_ = s1_.insert((CallBinaryMethodNode.create()));
if (callDel_ == null) {
throw new IllegalStateException("Specialization 'delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)' contains a shared cache with name 'callDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callDel.get(arg0Value) == null) {
this.callDel.set(arg0Value, callDel_);
}
if (!this.delFixedAttr_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s1_ != null) {
PyObjectSetAttr.delFixedAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, s1_.cachedName_, this.getClass, this.lookupDel.get(arg0Value), this.lookupGet.get(arg0Value), this.raise, this.callDel.get(arg0Value));
return;
}
break;
}
}
LookupSpecialMethodSlotNode lookup_1;
LookupSpecialMethodSlotNode lookup_1_shared = this.lookup.get(arg0Value);
if (lookup_1_shared != null) {
lookup_1 = lookup_1_shared;
} else {
lookup_1 = arg0Value.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.SetAttr)));
if (lookup_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'lookupSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookup.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.lookup.set(arg0Value, lookup_1);
}
LookupSpecialMethodSlotNode lookupDel_1;
LookupSpecialMethodSlotNode lookupDel_1_shared = this.lookupDel.get(arg0Value);
if (lookupDel_1_shared != null) {
lookupDel_1 = lookupDel_1_shared;
} else {
lookupDel_1 = arg0Value.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.DelAttr)));
if (lookupDel_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'lookupDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupDel.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.lookupDel.set(arg0Value, lookupDel_1);
}
LookupSpecialMethodSlotNode lookupGet_2;
LookupSpecialMethodSlotNode lookupGet_2_shared = this.lookupGet.get(arg0Value);
if (lookupGet_2_shared != null) {
lookupGet_2 = lookupGet_2_shared;
} else {
lookupGet_2 = arg0Value.insert((LookupSpecialMethodSlotNode.create(SpecialMethodSlot.GetAttribute)));
if (lookupGet_2 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'lookupGetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.lookupGet.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.lookupGet.set(arg0Value, lookupGet_2);
}
CallTernaryMethodNode call_1;
CallTernaryMethodNode call_1_shared = this.call.get(arg0Value);
if (call_1_shared != null) {
call_1 = call_1_shared;
} else {
call_1 = arg0Value.insert((CallTernaryMethodNode.create()));
if (call_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'callSetattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.call.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.call.set(arg0Value, call_1);
}
CallBinaryMethodNode callDel_1;
CallBinaryMethodNode callDel_1_shared = this.callDel.get(arg0Value);
if (callDel_1_shared != null) {
callDel_1 = callDel_1_shared;
} else {
callDel_1 = arg0Value.insert((CallBinaryMethodNode.create()));
if (callDel_1 == null) {
throw new IllegalStateException("Specialization 'doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)' contains a shared cache with name 'callDelattr' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callDel.get(arg0Value) == null) {
VarHandle.storeStoreFence();
this.callDel.set(arg0Value, callDel_1);
}
this.setFixedAttr_cache.set(arg0Value, null);
this.delFixedAttr_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffa /* remove SpecializationActive[PyObjectSetAttr.setFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode)], SpecializationActive[PyObjectSetAttr.delFixedAttr(Frame, Node, Object, TruffleString, Object, TruffleString, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallBinaryMethodNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PyObjectSetAttr.doDynamicAttr(Frame, Node, Object, TruffleString, Object, GetClassNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, LookupSpecialMethodSlotNode, Lazy, CallTernaryMethodNode, CallBinaryMethodNode)] */;
this.state_0_.set(arg0Value, state_0);
PyObjectSetAttr.doDynamicAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, this.getClass, lookup_1, lookupDel_1, lookupGet_2, this.raise, call_1, callDel_1);
return;
}
if ((!(PGuards.isString(arg2Value)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[PyObjectSetAttr.nameMustBeString(Node, Object, Object, Object, Lazy)] */;
this.state_0_.set(arg0Value, state_0);
PyObjectSetAttr.nameMustBeString(arg0Value, arg1Value, arg2Value, arg3Value, this.raise);
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PyObjectSetAttr.class)
@DenyReplace
private static final class SetFixedAttrData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#setFixedAttr}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
SetFixedAttrData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(PyObjectSetAttr.class)
@DenyReplace
private static final class DelFixedAttrData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link PyObjectSetAttr#delFixedAttr}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
DelFixedAttrData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(PyObjectSetAttr.class)
@DenyReplace
private static final class Uncached extends PyObjectSetAttr {
@Override
public void execute(Frame frameValue, Node arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg2Value instanceof TruffleString) {
TruffleString arg2Value_ = (TruffleString) arg2Value;
PyObjectSetAttr.doDynamicAttr(frameValue, arg0Value, arg1Value, arg2Value_, arg3Value, (GetClassNode.getUncached()), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.SetAttr)), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.DelAttr)), (LookupSpecialMethodSlotNode.getUncached(SpecialMethodSlot.GetAttribute)), (Lazy.getUncached()), (CallTernaryMethodNode.getUncached()), (CallBinaryMethodNode.getUncached()));
return;
}
if ((!(PGuards.isString(arg2Value)))) {
PyObjectSetAttr.nameMustBeString(arg0Value, arg1Value, arg2Value, arg3Value, (Lazy.getUncached()));
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy