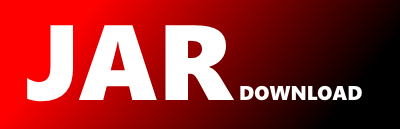
com.oracle.graal.python.nodes.attributes.ReadAttributeFromDynamicObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.attributes;
import com.oracle.graal.python.nodes.util.CastToTruffleStringNode;
import com.oracle.graal.python.nodes.util.CastToTruffleStringNodeGen;
import com.oracle.graal.python.runtime.PythonOptions;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.object.HiddenKey;
import com.oracle.truffle.api.object.Location;
import com.oracle.truffle.api.object.Shape;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.utilities.TruffleWeakReference;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link ReadAttributeFromDynamicObjectNode#readFinalAttr}
* Activation probability: 0.23929
* With/without class size: 12/24 bytes
* Specialization {@link ReadAttributeFromDynamicObjectNode#readFinalPrimitiveAttr}
* Activation probability: 0.20714
* With/without class size: 11/24 bytes
* Specialization {@link ReadAttributeFromDynamicObjectNode#readDirect}
* Activation probability: 0.17500
* With/without class size: 8/4 bytes
* Specialization {@link ReadAttributeFromDynamicObjectNode#readDirectHidden}
* Activation probability: 0.14286
* With/without class size: 7/4 bytes
* Specialization {@link ReadAttributeFromDynamicObjectNode#readDirectHidden}
* Activation probability: 0.11071
* With/without class size: 5/0 bytes
* Specialization {@link ReadAttributeFromDynamicObjectNode#read}
* Activation probability: 0.07857
* With/without class size: 7/17 bytes
* Specialization {@link ReadAttributeFromDynamicObjectNode#read}
* Activation probability: 0.04643
* With/without class size: 5/13 bytes
*
*/
@GeneratedBy(ReadAttributeFromDynamicObjectNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class ReadAttributeFromDynamicObjectNodeGen extends ReadAttributeFromDynamicObjectNode {
private static final StateField READ0__READ_ATTRIBUTE_FROM_DYNAMIC_OBJECT_NODE_READ0_STATE_0_UPDATER = StateField.create(Read0Data.lookup_(), "read0_state_0_");
private static final StateField READ1__READ_ATTRIBUTE_FROM_DYNAMIC_OBJECT_NODE_READ1_STATE_0_UPDATER = StateField.create(Read1Data.lookup_(), "read1_state_0_");
static final ReferenceField READ_FINAL_ATTR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readFinalAttr_cache", ReadFinalAttrData.class);
static final ReferenceField READ_FINAL_PRIMITIVE_ATTR_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readFinalPrimitiveAttr_cache", ReadFinalPrimitiveAttrData.class);
static final ReferenceField READ_DIRECT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readDirect_cache", ReadDirectData.class);
static final ReferenceField READ_DIRECT_HIDDEN0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readDirectHidden0_cache", ReadDirectHidden0Data.class);
static final ReferenceField READ0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "read0_cache", Read0Data.class);
/**
* Source Info:
* Specialization: {@link ReadAttributeFromDynamicObjectNode#read}
* Parameter: {@link CastToTruffleStringNode} castNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_READ0_CAST_NODE_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, READ0__READ_ATTRIBUTE_FROM_DYNAMIC_OBJECT_NODE_READ0_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(Read0Data.lookup_(), "read0_castNode__field1_", Node.class), ReferenceField.create(Read0Data.lookup_(), "read0_castNode__field2_", Node.class), ReferenceField.create(Read0Data.lookup_(), "read0_castNode__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromDynamicObjectNode#read}
* Parameter: {@link CastToTruffleStringNode} castNode
* Inline method: {@link CastToTruffleStringNodeGen#inline}
*/
private static final CastToTruffleStringNode INLINED_READ1_CAST_NODE_ = CastToTruffleStringNodeGen.inline(InlineTarget.create(CastToTruffleStringNode.class, READ1__READ_ATTRIBUTE_FROM_DYNAMIC_OBJECT_NODE_READ1_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(Read1Data.lookup_(), "read1_castNode__field1_", Node.class), ReferenceField.create(Read1Data.lookup_(), "read1_castNode__field2_", Node.class), ReferenceField.create(Read1Data.lookup_(), "read1_castNode__field3_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#readFinalAttr}
* 1: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#readDirect}
* 2: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#read}
* 3: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#read}
* 4: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#readFinalPrimitiveAttr}
* 5: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#readDirectHidden}
* 6: SpecializationActive {@link ReadAttributeFromDynamicObjectNode#readDirectHidden}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private ReadFinalAttrData readFinalAttr_cache;
@UnsafeAccessedField @CompilationFinal private ReadFinalPrimitiveAttrData readFinalPrimitiveAttr_cache;
@UnsafeAccessedField @Child private ReadDirectData readDirect_cache;
@UnsafeAccessedField @Child private ReadDirectHidden0Data readDirectHidden0_cache;
@UnsafeAccessedField @Child private Read0Data read0_cache;
@Child private Read1Data read1_cache;
private ReadAttributeFromDynamicObjectNodeGen() {
}
@ExplodeLoop
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */ && arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((state_0 & 0b10011) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] */) {
ReadFinalAttrData s0_ = this.readFinalAttr_cache;
if (s0_ != null) {
if (!Assumption.isValidAssumption(s0_.assumption0_) || !Assumption.isValidAssumption(s0_.assumption1_)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeReadFinalAttr_(s0_);
return executeAndSpecialize(arg0Value_, arg1Value_);
}
{
DynamicObject cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (arg0Value_ == cachedObject__) && (ReadAttributeFromDynamicObjectNode.isLongLivedObject(cachedObject__)) && (arg1Value_ == s0_.cachedKey_) && (arg0Value_.getShape() == s0_.cachedShape_) && (ReadAttributeFromDynamicObjectNode.locationIsAssumedFinal(s0_.loc_))) {
Object value__ = (s0_.weakValueGen__.get());
if ((value__ != null) && (!(ReadAttributeFromDynamicObjectNode.isPrimitive(value__)))) {
return ReadAttributeFromDynamicObjectNode.readFinalAttr(arg0Value_, arg1Value_, s0_.cachedKey_, cachedObject__, s0_.cachedShape_, s0_.loc_, s0_.propertyAssumption_, value__);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] */) {
ReadFinalPrimitiveAttrData s1_ = this.readFinalPrimitiveAttr_cache;
if (s1_ != null) {
if (!Assumption.isValidAssumption(s1_.assumption0_) || !Assumption.isValidAssumption(s1_.assumption1_)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeReadFinalPrimitiveAttr_(s1_);
return executeAndSpecialize(arg0Value_, arg1Value_);
}
{
DynamicObject cachedObject__1 = (s1_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__1 != null) && (arg0Value_ == cachedObject__1) && (ReadAttributeFromDynamicObjectNode.isLongLivedObject(cachedObject__1)) && (arg1Value_ == s1_.cachedKey_) && (arg0Value_.getShape() == s1_.cachedShape_) && (ReadAttributeFromDynamicObjectNode.locationIsAssumedFinal(s1_.loc_))) {
assert DSLSupport.assertIdempotence((ReadAttributeFromDynamicObjectNode.isPrimitive(s1_.value_)));
return ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(arg0Value_, arg1Value_, s1_.cachedKey_, cachedObject__1, s1_.cachedShape_, s1_.loc_, s1_.propertyAssumption_, s1_.value_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] */) {
ReadDirectData s2_ = this.readDirect_cache;
while (s2_ != null) {
if ((s2_.dylib_.accepts(arg0Value_))) {
return ReadAttributeFromDynamicObjectNode.readDirect(arg0Value_, arg1Value_, s2_.dylib_);
}
s2_ = s2_.next_;
}
}
}
if ((state_0 & 0b1101100) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] */) {
ReadDirectHidden0Data s3_ = this.readDirectHidden0_cache;
while (s3_ != null) {
if ((s3_.dylib_.accepts(arg0Value_)) && (ObjectAttributeNode.isHiddenKey(arg1Value))) {
return ReadAttributeFromDynamicObjectNode.readDirectHidden(arg0Value_, arg1Value, s3_.dylib_);
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] */) {
if ((ObjectAttributeNode.isHiddenKey(arg1Value))) {
return this.readDirectHidden1Boundary(state_0, arg0Value_, arg1Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
Read0Data s5_ = this.read0_cache;
while (s5_ != null) {
if ((s5_.dylib_.accepts(arg0Value_)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__ = (s5_);
return ReadAttributeFromDynamicObjectNode.read(arg0Value_, arg1Value, inliningTarget__, INLINED_READ0_CAST_NODE_, s5_.dylib_);
}
s5_ = s5_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
Read1Data s6_ = this.read1_cache;
if (s6_ != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return this.read1Boundary(state_0, s6_, arg0Value_, arg1Value);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object readDirectHidden1Boundary(int state_0, DynamicObject arg0Value_, Object arg1Value) {
{
DynamicObjectLibrary dylib__ = (DYNAMIC_OBJECT_LIBRARY_.getUncached(arg0Value_));
return ReadAttributeFromDynamicObjectNode.readDirectHidden(arg0Value_, arg1Value, dylib__);
}
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object read1Boundary(int state_0, Read1Data s6_, DynamicObject arg0Value_, Object arg1Value) {
{
Node inliningTarget__ = (s6_);
DynamicObjectLibrary dylib__1 = (DYNAMIC_OBJECT_LIBRARY_.getUncached(arg0Value_));
return ReadAttributeFromDynamicObjectNode.read(arg0Value_, arg1Value, inliningTarget__, INLINED_READ1_CAST_NODE_, dylib__1);
}
}
@ExplodeLoop
@Override
public Object execute(Object arg0Value, HiddenKey arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1101100) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */ && arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] */) {
ReadDirectHidden0Data s3_ = this.readDirectHidden0_cache;
while (s3_ != null) {
if ((s3_.dylib_.accepts(arg0Value_)) && (ObjectAttributeNode.isHiddenKey(arg1Value))) {
return ReadAttributeFromDynamicObjectNode.readDirectHidden(arg0Value_, arg1Value, s3_.dylib_);
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] */) {
if ((ObjectAttributeNode.isHiddenKey(arg1Value))) {
return this.readDirectHidden1Boundary0(state_0, arg0Value_, arg1Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
Read0Data s5_ = this.read0_cache;
while (s5_ != null) {
if ((s5_.dylib_.accepts(arg0Value_)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__ = (s5_);
return ReadAttributeFromDynamicObjectNode.read(arg0Value_, arg1Value, inliningTarget__, INLINED_READ0_CAST_NODE_, s5_.dylib_);
}
s5_ = s5_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
Read1Data s6_ = this.read1_cache;
if (s6_ != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return this.read1Boundary1(state_0, s6_, arg0Value_, arg1Value);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object readDirectHidden1Boundary0(int state_0, DynamicObject arg0Value_, HiddenKey arg1Value) {
{
DynamicObjectLibrary dylib__ = (DYNAMIC_OBJECT_LIBRARY_.getUncached(arg0Value_));
return ReadAttributeFromDynamicObjectNode.readDirectHidden(arg0Value_, arg1Value, dylib__);
}
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object read1Boundary1(int state_0, Read1Data s6_, DynamicObject arg0Value_, HiddenKey arg1Value) {
{
Node inliningTarget__ = (s6_);
DynamicObjectLibrary dylib__1 = (DYNAMIC_OBJECT_LIBRARY_.getUncached(arg0Value_));
return ReadAttributeFromDynamicObjectNode.read(arg0Value_, arg1Value, inliningTarget__, INLINED_READ1_CAST_NODE_, dylib__1);
}
}
@ExplodeLoop
@Override
public Object execute(Object arg0Value, TruffleString arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */ && arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if ((state_0 & 0b10011) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] */) {
ReadFinalAttrData s0_ = this.readFinalAttr_cache;
if (s0_ != null) {
if (!Assumption.isValidAssumption(s0_.assumption0_) || !Assumption.isValidAssumption(s0_.assumption1_)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeReadFinalAttr_(s0_);
return executeAndSpecialize(arg0Value_, arg1Value);
}
{
DynamicObject cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (arg0Value_ == cachedObject__) && (ReadAttributeFromDynamicObjectNode.isLongLivedObject(cachedObject__)) && (arg1Value == s0_.cachedKey_) && (arg0Value_.getShape() == s0_.cachedShape_) && (ReadAttributeFromDynamicObjectNode.locationIsAssumedFinal(s0_.loc_))) {
Object value__ = (s0_.weakValueGen__.get());
if ((value__ != null) && (!(ReadAttributeFromDynamicObjectNode.isPrimitive(value__)))) {
return ReadAttributeFromDynamicObjectNode.readFinalAttr(arg0Value_, arg1Value, s0_.cachedKey_, cachedObject__, s0_.cachedShape_, s0_.loc_, s0_.propertyAssumption_, value__);
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(DynamicObject, TruffleString, TruffleString, DynamicObject, Shape, Location, Assumption, Object)] */) {
ReadFinalPrimitiveAttrData s1_ = this.readFinalPrimitiveAttr_cache;
if (s1_ != null) {
if (!Assumption.isValidAssumption(s1_.assumption0_) || !Assumption.isValidAssumption(s1_.assumption1_)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeReadFinalPrimitiveAttr_(s1_);
return executeAndSpecialize(arg0Value_, arg1Value);
}
{
DynamicObject cachedObject__1 = (s1_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__1 != null) && (arg0Value_ == cachedObject__1) && (ReadAttributeFromDynamicObjectNode.isLongLivedObject(cachedObject__1)) && (arg1Value == s1_.cachedKey_) && (arg0Value_.getShape() == s1_.cachedShape_) && (ReadAttributeFromDynamicObjectNode.locationIsAssumedFinal(s1_.loc_))) {
assert DSLSupport.assertIdempotence((ReadAttributeFromDynamicObjectNode.isPrimitive(s1_.value_)));
return ReadAttributeFromDynamicObjectNode.readFinalPrimitiveAttr(arg0Value_, arg1Value, s1_.cachedKey_, cachedObject__1, s1_.cachedShape_, s1_.loc_, s1_.propertyAssumption_, s1_.value_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] */) {
ReadDirectData s2_ = this.readDirect_cache;
while (s2_ != null) {
if ((s2_.dylib_.accepts(arg0Value_))) {
return ReadAttributeFromDynamicObjectNode.readDirect(arg0Value_, arg1Value, s2_.dylib_);
}
s2_ = s2_.next_;
}
}
}
if ((state_0 & 0b1101100) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] || SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] */) {
ReadDirectHidden0Data s3_ = this.readDirectHidden0_cache;
while (s3_ != null) {
if ((s3_.dylib_.accepts(arg0Value_)) && (ObjectAttributeNode.isHiddenKey(arg1Value))) {
return ReadAttributeFromDynamicObjectNode.readDirectHidden(arg0Value_, arg1Value, s3_.dylib_);
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirectHidden(DynamicObject, Object, DynamicObjectLibrary)] */) {
if ((ObjectAttributeNode.isHiddenKey(arg1Value))) {
return this.readDirectHidden1Boundary2(state_0, arg0Value_, arg1Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
Read0Data s5_ = this.read0_cache;
while (s5_ != null) {
if ((s5_.dylib_.accepts(arg0Value_)) && (!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__ = (s5_);
return ReadAttributeFromDynamicObjectNode.read(arg0Value_, arg1Value, inliningTarget__, INLINED_READ0_CAST_NODE_, s5_.dylib_);
}
s5_ = s5_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
Read1Data s6_ = this.read1_cache;
if (s6_ != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return this.read1Boundary3(state_0, s6_, arg0Value_, arg1Value);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object readDirectHidden1Boundary2(int state_0, DynamicObject arg0Value_, TruffleString arg1Value) {
{
DynamicObjectLibrary dylib__ = (DYNAMIC_OBJECT_LIBRARY_.getUncached(arg0Value_));
return ReadAttributeFromDynamicObjectNode.readDirectHidden(arg0Value_, arg1Value, dylib__);
}
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object read1Boundary3(int state_0, Read1Data s6_, DynamicObject arg0Value_, TruffleString arg1Value) {
{
Node inliningTarget__ = (s6_);
DynamicObjectLibrary dylib__1 = (DYNAMIC_OBJECT_LIBRARY_.getUncached(arg0Value_));
return ReadAttributeFromDynamicObjectNode.read(arg0Value_, arg1Value, inliningTarget__, INLINED_READ1_CAST_NODE_, dylib__1);
}
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof DynamicObject) {
DynamicObject arg0Value_ = (DynamicObject) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
{
Object value__ = null;
DynamicObject cachedObject__ = null;
if (((state_0 & 0b1110)) == 0 /* is-not SpecializationActive[ReadAttributeFromDynamicObjectNode.readDirect(DynamicObject, TruffleString, DynamicObjectLibrary)] && SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] && SpecializationActive[ReadAttributeFromDynamicObjectNode.read(DynamicObject, Object, Node, CastToTruffleStringNode, DynamicObjectLibrary)] */) {
while (true) {
int count0_ = 0;
ReadFinalAttrData s0_ = READ_FINAL_ATTR_CACHE_UPDATER.getVolatile(this);
ReadFinalAttrData s0_original = s0_;
while (s0_ != null) {
{
cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (arg0Value_ == cachedObject__) && (ReadAttributeFromDynamicObjectNode.isLongLivedObject(cachedObject__)) && (arg1Value_ == s0_.cachedKey_) && (arg0Value_.getShape() == s0_.cachedShape_) && (ReadAttributeFromDynamicObjectNode.locationIsAssumedFinal(s0_.loc_))) {
value__ = (s0_.weakValueGen__.get());
if ((value__ != null) && (!(ReadAttributeFromDynamicObjectNode.isPrimitive(value__))) && Assumption.isValidAssumption(s0_.assumption0_) && Assumption.isValidAssumption(s0_.assumption1_)) {
break;
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference weakCachedObjectGen___ = (new TruffleWeakReference<>(arg0Value_));
cachedObject__ = (weakCachedObjectGen___.get());
if ((cachedObject__ != null) && (arg0Value_ == cachedObject__) && (ReadAttributeFromDynamicObjectNode.isLongLivedObject(cachedObject__))) {
Shape cachedShape__ = (arg0Value_.getShape());
// assert (arg1Value_ == s0_.cachedKey_);
if ((arg0Value_.getShape() == cachedShape__)) {
TruffleString cachedKey__ = (arg1Value_);
Location loc__ = (ObjectAttributeNode.getLocationOrNull(cachedShape__.getProperty(cachedKey__)));
if ((ReadAttributeFromDynamicObjectNode.locationIsAssumedFinal(loc__))) {
TruffleWeakReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy