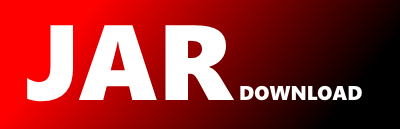
com.oracle.graal.python.nodes.attributes.ReadAttributeFromObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.attributes;
import com.oracle.graal.python.builtins.objects.cext.PythonAbstractNativeObject;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccess.ReadObjectNode;
import com.oracle.graal.python.builtins.objects.cext.structs.CStructAccessFactory.ReadObjectNodeGen;
import com.oracle.graal.python.builtins.objects.common.HashingStorage;
import com.oracle.graal.python.builtins.objects.common.PHashingCollection;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodes.HashingStorageGetItem;
import com.oracle.graal.python.builtins.objects.common.HashingStorageNodesFactory.HashingStorageGetItemNodeGen;
import com.oracle.graal.python.builtins.objects.module.PythonModule;
import com.oracle.graal.python.builtins.objects.object.PythonObject;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.interop.PForeignToPTypeNode;
import com.oracle.graal.python.nodes.object.GetDictIfExistsNode;
import com.oracle.graal.python.nodes.object.GetDictIfExistsNodeGen;
import com.oracle.graal.python.nodes.object.IsForeignObjectNode;
import com.oracle.graal.python.nodes.object.IsForeignObjectNodeGen;
import com.oracle.graal.python.nodes.util.CastToJavaStringNode;
import com.oracle.graal.python.nodes.util.CastToJavaStringNodeGen;
import com.oracle.graal.python.runtime.PythonOptions;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.HiddenKey;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.utilities.TruffleWeakReference;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Activation probability: 0.38500
* With/without class size: 24/33 bytes
* Specialization {@link ReadAttributeFromObjectNode#readHiddenKey}
* Activation probability: 0.29500
* With/without class size: 7/0 bytes
* Specialization {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Activation probability: 0.20500
* With/without class size: 13/26 bytes
* Specialization {@link ReadAttributeFromObjectNode#readForeign}
* Activation probability: 0.11500
* With/without class size: 6/5 bytes
*
*/
@GeneratedBy(ReadAttributeFromObjectNode.class)
@SuppressWarnings("javadoc")
public final class ReadAttributeFromObjectNodeGen extends ReadAttributeFromObjectNode {
private static final StateField READ_FROM_BUILTIN_MODULE_DICT__READ_ATTRIBUTE_FROM_OBJECT_NODE_READ_FROM_BUILTIN_MODULE_DICT_STATE_0_UPDATER = StateField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_state_0_");
private static final StateField READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER = StateField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_state_0_");
private static final StateField STATE_0_ReadAttributeFromObjectNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readFromBuiltinModuleDict_cache", ReadFromBuiltinModuleDictData.class);
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_FROM_BUILTIN_MODULE_DICT__READ_ATTRIBUTE_FROM_OBJECT_NODE_READ_FROM_BUILTIN_MODULE_DICT_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field1_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field2_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field3_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field4_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link InlinedConditionProfile} profileHasDict
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER.subUpdater(2, 8), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field1_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field2_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field3_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field4_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private static final IsForeignObjectNode INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, STATE_0_ReadAttributeFromObjectNode_UPDATER.subUpdater(4, 8)));
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* 1: SpecializationActive {@link ReadAttributeFromObjectNode#readHiddenKey}
* 2: SpecializationActive {@link ReadAttributeFromObjectNode#readObjectAttribute}
* 3: SpecializationActive {@link ReadAttributeFromObjectNode#readForeign}
* 4-11: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readHiddenKey}
* Parameter: {@link ReadAttributeFromDynamicObjectNode} readAttributeFromDynamicObjectNode
*/
@Child private ReadAttributeFromDynamicObjectNode readDynamic;
@UnsafeAccessedField @Child private ReadFromBuiltinModuleDictData readFromBuiltinModuleDict_cache;
@Child private ReadObjectAttributeData readObjectAttribute_cache;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link ReadAttributeFromForeign} read
*/
@Child private ReadAttributeFromForeign readForeign_read_;
private ReadAttributeFromObjectNodeGen() {
}
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] */ && arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
ReadFromBuiltinModuleDictData s0_ = this.readFromBuiltinModuleDict_cache;
if (s0_ != null) {
{
PythonModule cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
PHashingCollection cachedDict__ = (s0_.weakCachedDictGen__.get());
if ((cachedDict__ != null)) {
HashingStorage cachedStorage__ = (s0_.weakCachedStorageGen__.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
Node inliningTarget__ = (s0_);
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, inliningTarget__, cachedObject__, cachedDict__, cachedStorage__, INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_);
}
}
}
}
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] */ && arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
{
ReadAttributeFromDynamicObjectNode readDynamic_ = this.readDynamic;
if (readDynamic_ != null) {
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, readDynamic_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */) {
ReadObjectAttributeData s2_ = this.readObjectAttribute_cache;
if (s2_ != null) {
{
ReadAttributeFromDynamicObjectNode readDynamic_1 = this.readDynamic;
if (readDynamic_1 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__1 = (s2_);
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, inliningTarget__1, INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_, s2_.getDict_, readDynamic_1, INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_);
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
{
ReadAttributeFromForeign read__ = this.readForeign_read_;
if (read__ != null) {
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
Node inliningTarget__2 = (this);
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, inliningTarget__2, INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_, read__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b1111);
try {
{
HashingStorage cachedStorage__ = null;
PHashingCollection cachedDict__ = null;
PythonModule cachedObject__ = null;
Node inliningTarget__ = null;
if (arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
while (true) {
int count0_ = 0;
ReadFromBuiltinModuleDictData s0_ = READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER.getVolatile(this);
ReadFromBuiltinModuleDictData s0_original = s0_;
while (s0_ != null) {
{
cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
cachedDict__ = (s0_.weakCachedDictGen__.get());
if ((cachedDict__ != null)) {
cachedStorage__ = (s0_.weakCachedStorageGen__.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
inliningTarget__ = (s0_);
break;
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference weakCachedObjectGen___ = (new TruffleWeakReference<>(arg0Value_));
cachedObject__ = (weakCachedObjectGen___.get());
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
TruffleWeakReference weakCachedDictGen___ = (new TruffleWeakReference<>(ReadAttributeFromObjectNode.getDict(arg0Value_)));
cachedDict__ = (weakCachedDictGen___.get());
if ((cachedDict__ != null)) {
TruffleWeakReference weakCachedStorageGen___ = (new TruffleWeakReference<>(ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))));
cachedStorage__ = (weakCachedStorageGen___.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
s0_ = this.insert(new ReadFromBuiltinModuleDictData());
s0_.weakCachedStorageGen__ = weakCachedStorageGen___;
s0_.weakCachedDictGen__ = weakCachedDictGen___;
s0_.weakCachedObjectGen__ = weakCachedObjectGen___;
inliningTarget__ = (s0_);
if (!READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] */;
this.state_0_ = state_0;
}
}
}
}
}
if (s0_ != null) {
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, inliningTarget__, cachedObject__, cachedDict__, cachedStorage__, INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_);
}
break;
}
}
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if (arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
ReadAttributeFromDynamicObjectNode readDynamic_;
ReadAttributeFromDynamicObjectNode readDynamic__shared = this.readDynamic;
if (readDynamic__shared != null) {
readDynamic_ = readDynamic__shared;
} else {
readDynamic_ = this.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readDynamic_ == null) {
throw new IllegalStateException("Specialization 'readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)' contains a shared cache with name 'readAttributeFromDynamicObjectNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readDynamic == null) {
VarHandle.storeStoreFence();
this.readDynamic = readDynamic_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, readDynamic_);
}
{
Node inliningTarget__1 = null;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
ReadObjectAttributeData s2_ = this.insert(new ReadObjectAttributeData());
inliningTarget__1 = (s2_);
GetDictIfExistsNode getDict__ = s2_.insert((GetDictIfExistsNodeGen.create()));
Objects.requireNonNull(getDict__, "Specialization 'readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)' cache 'getDict' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.getDict_ = getDict__;
ReadAttributeFromDynamicObjectNode readDynamic_1;
ReadAttributeFromDynamicObjectNode readDynamic_1_shared = this.readDynamic;
if (readDynamic_1_shared != null) {
readDynamic_1 = readDynamic_1_shared;
} else {
readDynamic_1 = s2_.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readDynamic_1 == null) {
throw new IllegalStateException("Specialization 'readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)' contains a shared cache with name 'readAttributeFromDynamicObjectNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readDynamic == null) {
this.readDynamic = readDynamic_1;
}
VarHandle.storeStoreFence();
this.readObjectAttribute_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, inliningTarget__1, INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_, getDict__, readDynamic_1, INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_);
}
}
}
{
Node inliningTarget__2 = null;
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
inliningTarget__2 = (this);
ReadAttributeFromForeign read__ = this.insert((ReadAttributeFromForeignNodeGen.create()));
Objects.requireNonNull(read__, "Specialization 'readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)' cache 'read' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.readForeign_read_ = read__;
state_0 = state_0 | 0b1000 /* add SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, inliningTarget__2, INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_, read__);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
int state_0 = this.state_0_;
int newState_0 = (state_0 & 0b1111);
if (((oldState_0 ^ newState_0) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b1111) & ((state_0 & 0b1111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static ReadAttributeFromObjectNode create() {
return new ReadAttributeFromObjectNodeGen();
}
@GeneratedBy(ReadAttributeFromObjectNode.class)
@DenyReplace
private static final class ReadFromBuiltinModuleDictData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readFromBuiltinModuleDict_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedStorageGen_
*/
@CompilationFinal TruffleWeakReference weakCachedStorageGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedDictGen_
*/
@CompilationFinal TruffleWeakReference weakCachedDictGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedObjectGen_
*/
@CompilationFinal TruffleWeakReference weakCachedObjectGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field5_;
ReadFromBuiltinModuleDictData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectNode.class)
@DenyReplace
private static final class ReadObjectAttributeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link InlinedConditionProfile} profileHasDict
* Inline method: {@link InlinedConditionProfile#inline}
* 2-9: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readObjectAttribute_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child GetDictIfExistsNode getDict_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field5_;
ReadObjectAttributeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
/**
* Debug Info:
* Specialization {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Activation probability: 0.32000
* With/without class size: 20/33 bytes
* Specialization {@link ReadAttributeFromObjectNode#readHiddenKey}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Activation probability: 0.20000
* With/without class size: 12/26 bytes
* Specialization {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Activation probability: 0.14000
* With/without class size: 10/25 bytes
* Specialization {@link ReadAttributeFromObjectNode#readForeign}
* Activation probability: 0.08000
* With/without class size: 5/5 bytes
*
*/
@GeneratedBy(ReadAttributeFromObjectNotTypeNode.class)
@SuppressWarnings("javadoc")
protected static final class ReadAttributeFromObjectNotTypeNodeGen extends ReadAttributeFromObjectNotTypeNode {
private static final StateField READ_FROM_BUILTIN_MODULE_DICT__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_FROM_BUILTIN_MODULE_DICT_STATE_0_UPDATER = StateField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_state_0_");
private static final StateField READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER = StateField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_state_0_");
private static final StateField READ_NATIVE_OBJECT__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_NATIVE_OBJECT_STATE_0_UPDATER = StateField.create(ReadNativeObjectData.lookup_(), "readNativeObject_state_0_");
private static final StateField STATE_0_ReadAttributeFromObjectNotTypeNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readFromBuiltinModuleDict_cache", ReadFromBuiltinModuleDictData.class);
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_FROM_BUILTIN_MODULE_DICT__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_FROM_BUILTIN_MODULE_DICT_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field1_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field2_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field3_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field4_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link InlinedConditionProfile} profileHasDict
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER.subUpdater(2, 8), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field1_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field2_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field3_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field4_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_NATIVE_OBJECT_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_NATIVE_OBJECT__READ_ATTRIBUTE_FROM_OBJECT_NOT_TYPE_NODE_READ_NATIVE_OBJECT_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(ReadNativeObjectData.lookup_(), "readNativeObject_getItem__field1_", Node.class), ReferenceField.create(ReadNativeObjectData.lookup_(), "readNativeObject_getItem__field2_", Node.class), ReferenceField.create(ReadNativeObjectData.lookup_(), "readNativeObject_getItem__field3_", Node.class), ReferenceField.create(ReadNativeObjectData.lookup_(), "readNativeObject_getItem__field4_", Node.class), ReferenceField.create(ReadNativeObjectData.lookup_(), "readNativeObject_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private static final IsForeignObjectNode INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, STATE_0_ReadAttributeFromObjectNotTypeNode_UPDATER.subUpdater(5, 8)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* 1: SpecializationActive {@link ReadAttributeFromObjectNode#readHiddenKey}
* 2: SpecializationActive {@link ReadAttributeFromObjectNode#readObjectAttribute}
* 3: SpecializationActive {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* 4: SpecializationActive {@link ReadAttributeFromObjectNode#readForeign}
* 5-12: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readHiddenKey}
* Parameter: {@link ReadAttributeFromDynamicObjectNode} readAttributeFromDynamicObjectNode
*/
@Child private ReadAttributeFromDynamicObjectNode readDynamic;
@UnsafeAccessedField @Child private ReadFromBuiltinModuleDictData readFromBuiltinModuleDict_cache;
@Child private ReadObjectAttributeData readObjectAttribute_cache;
@Child private ReadNativeObjectData readNativeObject_cache;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link ReadAttributeFromForeign} read
*/
@Child private ReadAttributeFromForeign readForeign_read_;
private ReadAttributeFromObjectNotTypeNodeGen() {
}
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectNotTypeNode.readNativeObject(PythonAbstractNativeObject, Object, Node, GetDictIfExistsNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] */ && arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
ReadFromBuiltinModuleDictData s0_ = this.readFromBuiltinModuleDict_cache;
if (s0_ != null) {
{
PythonModule cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
PHashingCollection cachedDict__ = (s0_.weakCachedDictGen__.get());
if ((cachedDict__ != null)) {
HashingStorage cachedStorage__ = (s0_.weakCachedStorageGen__.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
Node inliningTarget__ = (s0_);
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, inliningTarget__, cachedObject__, cachedDict__, cachedStorage__, INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_);
}
}
}
}
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] */ && arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
{
ReadAttributeFromDynamicObjectNode readDynamic_ = this.readDynamic;
if (readDynamic_ != null) {
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, readDynamic_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */) {
ReadObjectAttributeData s2_ = this.readObjectAttribute_cache;
if (s2_ != null) {
{
ReadAttributeFromDynamicObjectNode readDynamic_1 = this.readDynamic;
if (readDynamic_1 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__1 = (s2_);
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, inliningTarget__1, INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_, s2_.getDict_, readDynamic_1, INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_);
}
}
}
}
}
}
if ((state_0 & 0b11000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectNotTypeNode.readNativeObject(PythonAbstractNativeObject, Object, Node, GetDictIfExistsNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectNotTypeNode.readNativeObject(PythonAbstractNativeObject, Object, Node, GetDictIfExistsNode, HashingStorageGetItem)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
ReadNativeObjectData s3_ = this.readNativeObject_cache;
if (s3_ != null) {
{
Node inliningTarget__2 = (s3_);
return ReadAttributeFromObjectNotTypeNode.readNativeObject(arg0Value_, arg1Value, inliningTarget__2, s3_.getDict_, INLINED_READ_NATIVE_OBJECT_GET_ITEM_);
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
{
ReadAttributeFromForeign read__ = this.readForeign_read_;
if (read__ != null) {
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
Node inliningTarget__3 = (this);
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, inliningTarget__3, INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_, read__);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b11111);
try {
{
HashingStorage cachedStorage__ = null;
PHashingCollection cachedDict__ = null;
PythonModule cachedObject__ = null;
Node inliningTarget__ = null;
if (arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
while (true) {
int count0_ = 0;
ReadFromBuiltinModuleDictData s0_ = READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER.getVolatile(this);
ReadFromBuiltinModuleDictData s0_original = s0_;
while (s0_ != null) {
{
cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
cachedDict__ = (s0_.weakCachedDictGen__.get());
if ((cachedDict__ != null)) {
cachedStorage__ = (s0_.weakCachedStorageGen__.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
inliningTarget__ = (s0_);
break;
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference weakCachedObjectGen___ = (new TruffleWeakReference<>(arg0Value_));
cachedObject__ = (weakCachedObjectGen___.get());
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
TruffleWeakReference weakCachedDictGen___ = (new TruffleWeakReference<>(ReadAttributeFromObjectNode.getDict(arg0Value_)));
cachedDict__ = (weakCachedDictGen___.get());
if ((cachedDict__ != null)) {
TruffleWeakReference weakCachedStorageGen___ = (new TruffleWeakReference<>(ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))));
cachedStorage__ = (weakCachedStorageGen___.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
s0_ = this.insert(new ReadFromBuiltinModuleDictData());
s0_.weakCachedStorageGen__ = weakCachedStorageGen___;
s0_.weakCachedDictGen__ = weakCachedDictGen___;
s0_.weakCachedObjectGen__ = weakCachedObjectGen___;
inliningTarget__ = (s0_);
if (!READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] */;
this.state_0_ = state_0;
}
}
}
}
}
if (s0_ != null) {
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, inliningTarget__, cachedObject__, cachedDict__, cachedStorage__, INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_);
}
break;
}
}
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if (arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
ReadAttributeFromDynamicObjectNode readDynamic_;
ReadAttributeFromDynamicObjectNode readDynamic__shared = this.readDynamic;
if (readDynamic__shared != null) {
readDynamic_ = readDynamic__shared;
} else {
readDynamic_ = this.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readDynamic_ == null) {
throw new IllegalStateException("Specialization 'readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)' contains a shared cache with name 'readAttributeFromDynamicObjectNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readDynamic == null) {
VarHandle.storeStoreFence();
this.readDynamic = readDynamic_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, readDynamic_);
}
{
Node inliningTarget__1 = null;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
ReadObjectAttributeData s2_ = this.insert(new ReadObjectAttributeData());
inliningTarget__1 = (s2_);
GetDictIfExistsNode getDict__ = s2_.insert((GetDictIfExistsNodeGen.create()));
Objects.requireNonNull(getDict__, "Specialization 'readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)' cache 'getDict' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.getDict_ = getDict__;
ReadAttributeFromDynamicObjectNode readDynamic_1;
ReadAttributeFromDynamicObjectNode readDynamic_1_shared = this.readDynamic;
if (readDynamic_1_shared != null) {
readDynamic_1 = readDynamic_1_shared;
} else {
readDynamic_1 = s2_.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readDynamic_1 == null) {
throw new IllegalStateException("Specialization 'readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)' contains a shared cache with name 'readAttributeFromDynamicObjectNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readDynamic == null) {
this.readDynamic = readDynamic_1;
}
VarHandle.storeStoreFence();
this.readObjectAttribute_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, inliningTarget__1, INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_, getDict__, readDynamic_1, INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_);
}
}
}
{
Node inliningTarget__2 = null;
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
ReadNativeObjectData s3_ = this.insert(new ReadNativeObjectData());
inliningTarget__2 = (s3_);
GetDictIfExistsNode getDict__1 = s3_.insert((GetDictIfExistsNodeGen.create()));
Objects.requireNonNull(getDict__1, "Specialization 'readNativeObject(PythonAbstractNativeObject, Object, Node, GetDictIfExistsNode, HashingStorageGetItem)' cache 'getDict' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.getDict_ = getDict__1;
VarHandle.storeStoreFence();
this.readNativeObject_cache = s3_;
state_0 = state_0 | 0b1000 /* add SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectNotTypeNode.readNativeObject(PythonAbstractNativeObject, Object, Node, GetDictIfExistsNode, HashingStorageGetItem)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNotTypeNode.readNativeObject(arg0Value_, arg1Value, inliningTarget__2, getDict__1, INLINED_READ_NATIVE_OBJECT_GET_ITEM_);
}
}
{
Node inliningTarget__3 = null;
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
inliningTarget__3 = (this);
ReadAttributeFromForeign read__ = this.insert((ReadAttributeFromForeignNodeGen.create()));
Objects.requireNonNull(read__, "Specialization 'readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)' cache 'read' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.readForeign_read_ = read__;
state_0 = state_0 | 0b10000 /* add SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, inliningTarget__3, INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_, read__);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
int state_0 = this.state_0_;
int newState_0 = (state_0 & 0b11111);
if (((oldState_0 ^ newState_0) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11111) & ((state_0 & 0b11111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static ReadAttributeFromObjectNotTypeNode create() {
return new ReadAttributeFromObjectNotTypeNodeGen();
}
@NeverDefault
public static ReadAttributeFromObjectNotTypeNode getUncached() {
return ReadAttributeFromObjectNotTypeNodeGen.UNCACHED;
}
@GeneratedBy(ReadAttributeFromObjectNotTypeNode.class)
@DenyReplace
private static final class ReadFromBuiltinModuleDictData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readFromBuiltinModuleDict_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedStorageGen_
*/
@CompilationFinal TruffleWeakReference weakCachedStorageGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedDictGen_
*/
@CompilationFinal TruffleWeakReference weakCachedDictGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedObjectGen_
*/
@CompilationFinal TruffleWeakReference weakCachedObjectGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field5_;
ReadFromBuiltinModuleDictData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectNotTypeNode.class)
@DenyReplace
private static final class ReadObjectAttributeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link InlinedConditionProfile} profileHasDict
* Inline method: {@link InlinedConditionProfile#inline}
* 2-9: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readObjectAttribute_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child GetDictIfExistsNode getDict_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field5_;
ReadObjectAttributeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectNotTypeNode.class)
@DenyReplace
private static final class ReadNativeObjectData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readNativeObject_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child GetDictIfExistsNode getDict_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeObject_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeObject_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeObject_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeObject_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNotTypeNode#readNativeObject}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeObject_getItem__field5_;
ReadNativeObjectData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectNotTypeNode.class)
@DenyReplace
private static final class Uncached extends ReadAttributeFromObjectNotTypeNode {
@TruffleBoundary
@Override
public Object execute(Object arg0Value, Object arg1Value) {
if (arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if ((isSingleContext()) && ((arg0Value_) != null)) {
// assert ((arg0Value_) == arg0Value_);
if (((ReadAttributeFromObjectNode.getDict(arg0Value_)) != null) && ((ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))) != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, (ReadAttributeFromObjectNode.getDict(arg0Value_))) == (ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))))) {
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, (this), (arg0Value_), (ReadAttributeFromObjectNode.getDict(arg0Value_)), (ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))), (HashingStorageGetItemNodeGen.getUncached()));
}
}
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if (arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, (ReadAttributeFromDynamicObjectNode.getUncached()));
}
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, (this), (InlinedConditionProfile.getUncached()), (GetDictIfExistsNode.getUncached()), (ReadAttributeFromDynamicObjectNode.getUncached()), (HashingStorageGetItemNodeGen.getUncached()));
}
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
return ReadAttributeFromObjectNotTypeNode.readNativeObject(arg0Value_, arg1Value, (this), (GetDictIfExistsNode.getUncached()), (HashingStorageGetItemNodeGen.getUncached()));
}
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, (this), (IsForeignObjectNodeGen.getUncached()), (ReadAttributeFromForeignNodeGen.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Activation probability: 0.32000
* With/without class size: 20/33 bytes
* Specialization {@link ReadAttributeFromObjectNode#readHiddenKey}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Activation probability: 0.20000
* With/without class size: 12/26 bytes
* Specialization {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Activation probability: 0.14000
* With/without class size: 10/25 bytes
* Specialization {@link ReadAttributeFromObjectNode#readForeign}
* Activation probability: 0.08000
* With/without class size: 5/5 bytes
*
*/
@GeneratedBy(ReadAttributeFromObjectTpDictNode.class)
@SuppressWarnings("javadoc")
protected static final class ReadAttributeFromObjectTpDictNodeGen extends ReadAttributeFromObjectTpDictNode {
private static final StateField READ_FROM_BUILTIN_MODULE_DICT__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_FROM_BUILTIN_MODULE_DICT_STATE_0_UPDATER = StateField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_state_0_");
private static final StateField READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER = StateField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_state_0_");
private static final StateField READ_NATIVE_CLASS__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_NATIVE_CLASS_STATE_0_UPDATER = StateField.create(ReadNativeClassData.lookup_(), "readNativeClass_state_0_");
private static final StateField STATE_0_ReadAttributeFromObjectTpDictNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "readFromBuiltinModuleDict_cache", ReadFromBuiltinModuleDictData.class);
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_FROM_BUILTIN_MODULE_DICT__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_FROM_BUILTIN_MODULE_DICT_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field1_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field2_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field3_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field4_", Node.class), ReferenceField.create(ReadFromBuiltinModuleDictData.lookup_(), "readFromBuiltinModuleDict_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link InlinedConditionProfile} profileHasDict
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_OBJECT_ATTRIBUTE__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_OBJECT_ATTRIBUTE_STATE_0_UPDATER.subUpdater(2, 8), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field1_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field2_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field3_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field4_", Node.class), ReferenceField.create(ReadObjectAttributeData.lookup_(), "readObjectAttribute_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*/
private static final HashingStorageGetItem INLINED_READ_NATIVE_CLASS_GET_ITEM_ = HashingStorageGetItemNodeGen.inline(InlineTarget.create(HashingStorageGetItem.class, READ_NATIVE_CLASS__READ_ATTRIBUTE_FROM_OBJECT_TP_DICT_NODE_READ_NATIVE_CLASS_STATE_0_UPDATER.subUpdater(0, 8), ReferenceField.create(ReadNativeClassData.lookup_(), "readNativeClass_getItem__field1_", Node.class), ReferenceField.create(ReadNativeClassData.lookup_(), "readNativeClass_getItem__field2_", Node.class), ReferenceField.create(ReadNativeClassData.lookup_(), "readNativeClass_getItem__field3_", Node.class), ReferenceField.create(ReadNativeClassData.lookup_(), "readNativeClass_getItem__field4_", Node.class), ReferenceField.create(ReadNativeClassData.lookup_(), "readNativeClass_getItem__field5_", Node.class)));
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*/
private static final IsForeignObjectNode INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_ = IsForeignObjectNodeGen.inline(InlineTarget.create(IsForeignObjectNode.class, STATE_0_ReadAttributeFromObjectTpDictNode_UPDATER.subUpdater(5, 8)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* 1: SpecializationActive {@link ReadAttributeFromObjectNode#readHiddenKey}
* 2: SpecializationActive {@link ReadAttributeFromObjectNode#readObjectAttribute}
* 3: SpecializationActive {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* 4: SpecializationActive {@link ReadAttributeFromObjectNode#readForeign}
* 5-12: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link IsForeignObjectNode} isForeignObjectNode
* Inline method: {@link IsForeignObjectNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readHiddenKey}
* Parameter: {@link ReadAttributeFromDynamicObjectNode} readAttributeFromDynamicObjectNode
*/
@Child private ReadAttributeFromDynamicObjectNode readDynamic;
@UnsafeAccessedField @Child private ReadFromBuiltinModuleDictData readFromBuiltinModuleDict_cache;
@Child private ReadObjectAttributeData readObjectAttribute_cache;
@Child private ReadNativeClassData readNativeClass_cache;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readForeign}
* Parameter: {@link ReadAttributeFromForeign} read
*/
@Child private ReadAttributeFromForeign readForeign_read_;
private ReadAttributeFromObjectTpDictNodeGen() {
}
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectTpDictNode.readNativeClass(PythonAbstractNativeObject, Object, Node, ReadObjectNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] */ && arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
ReadFromBuiltinModuleDictData s0_ = this.readFromBuiltinModuleDict_cache;
if (s0_ != null) {
{
PythonModule cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
PHashingCollection cachedDict__ = (s0_.weakCachedDictGen__.get());
if ((cachedDict__ != null)) {
HashingStorage cachedStorage__ = (s0_.weakCachedStorageGen__.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
Node inliningTarget__ = (s0_);
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, inliningTarget__, cachedObject__, cachedDict__, cachedStorage__, INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_);
}
}
}
}
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] || SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */ && arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] */ && arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
{
ReadAttributeFromDynamicObjectNode readDynamic_ = this.readDynamic;
if (readDynamic_ != null) {
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, readDynamic_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */) {
ReadObjectAttributeData s2_ = this.readObjectAttribute_cache;
if (s2_ != null) {
{
ReadAttributeFromDynamicObjectNode readDynamic_1 = this.readDynamic;
if (readDynamic_1 != null) {
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
Node inliningTarget__1 = (s2_);
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, inliningTarget__1, INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_, s2_.getDict_, readDynamic_1, INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_);
}
}
}
}
}
}
if ((state_0 & 0b11000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectTpDictNode.readNativeClass(PythonAbstractNativeObject, Object, Node, ReadObjectNode, HashingStorageGetItem)] || SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectTpDictNode.readNativeClass(PythonAbstractNativeObject, Object, Node, ReadObjectNode, HashingStorageGetItem)] */ && arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
ReadNativeClassData s3_ = this.readNativeClass_cache;
if (s3_ != null) {
{
Node inliningTarget__2 = (s3_);
return ReadAttributeFromObjectTpDictNode.readNativeClass(arg0Value_, arg1Value, inliningTarget__2, s3_.getNativeDict_, INLINED_READ_NATIVE_CLASS_GET_ITEM_);
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */) {
{
ReadAttributeFromForeign read__ = this.readForeign_read_;
if (read__ != null) {
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
Node inliningTarget__3 = (this);
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, inliningTarget__3, INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_, read__);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b11111);
try {
{
HashingStorage cachedStorage__ = null;
PHashingCollection cachedDict__ = null;
PythonModule cachedObject__ = null;
Node inliningTarget__ = null;
if (arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
while (true) {
int count0_ = 0;
ReadFromBuiltinModuleDictData s0_ = READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER.getVolatile(this);
ReadFromBuiltinModuleDictData s0_original = s0_;
while (s0_ != null) {
{
cachedObject__ = (s0_.weakCachedObjectGen__.get());
assert DSLSupport.assertIdempotence((isSingleContext()));
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
cachedDict__ = (s0_.weakCachedDictGen__.get());
if ((cachedDict__ != null)) {
cachedStorage__ = (s0_.weakCachedStorageGen__.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
inliningTarget__ = (s0_);
break;
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((isSingleContext())) {
TruffleWeakReference weakCachedObjectGen___ = (new TruffleWeakReference<>(arg0Value_));
cachedObject__ = (weakCachedObjectGen___.get());
if ((cachedObject__ != null) && (cachedObject__ == arg0Value_)) {
TruffleWeakReference weakCachedDictGen___ = (new TruffleWeakReference<>(ReadAttributeFromObjectNode.getDict(arg0Value_)));
cachedDict__ = (weakCachedDictGen___.get());
if ((cachedDict__ != null)) {
TruffleWeakReference weakCachedStorageGen___ = (new TruffleWeakReference<>(ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))));
cachedStorage__ = (weakCachedStorageGen___.get());
if ((cachedStorage__ != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, cachedDict__) == cachedStorage__)) {
s0_ = this.insert(new ReadFromBuiltinModuleDictData());
s0_.weakCachedStorageGen__ = weakCachedStorageGen___;
s0_.weakCachedDictGen__ = weakCachedDictGen___;
s0_.weakCachedObjectGen__ = weakCachedObjectGen___;
inliningTarget__ = (s0_);
if (!READ_FROM_BUILTIN_MODULE_DICT_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[ReadAttributeFromObjectNode.readFromBuiltinModuleDict(PythonModule, TruffleString, Node, PythonModule, PHashingCollection, HashingStorage, HashingStorageGetItem)] */;
this.state_0_ = state_0;
}
}
}
}
}
if (s0_ != null) {
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, inliningTarget__, cachedObject__, cachedDict__, cachedStorage__, INLINED_READ_FROM_BUILTIN_MODULE_DICT_GET_ITEM_);
}
break;
}
}
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if (arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
ReadAttributeFromDynamicObjectNode readDynamic_;
ReadAttributeFromDynamicObjectNode readDynamic__shared = this.readDynamic;
if (readDynamic__shared != null) {
readDynamic_ = readDynamic__shared;
} else {
readDynamic_ = this.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readDynamic_ == null) {
throw new IllegalStateException("Specialization 'readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)' contains a shared cache with name 'readAttributeFromDynamicObjectNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readDynamic == null) {
VarHandle.storeStoreFence();
this.readDynamic = readDynamic_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[ReadAttributeFromObjectNode.readHiddenKey(PythonObject, HiddenKey, ReadAttributeFromDynamicObjectNode)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, readDynamic_);
}
{
Node inliningTarget__1 = null;
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
ReadObjectAttributeData s2_ = this.insert(new ReadObjectAttributeData());
inliningTarget__1 = (s2_);
GetDictIfExistsNode getDict__ = s2_.insert((GetDictIfExistsNodeGen.create()));
Objects.requireNonNull(getDict__, "Specialization 'readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)' cache 'getDict' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s2_.getDict_ = getDict__;
ReadAttributeFromDynamicObjectNode readDynamic_1;
ReadAttributeFromDynamicObjectNode readDynamic_1_shared = this.readDynamic;
if (readDynamic_1_shared != null) {
readDynamic_1 = readDynamic_1_shared;
} else {
readDynamic_1 = s2_.insert((ReadAttributeFromDynamicObjectNode.create()));
if (readDynamic_1 == null) {
throw new IllegalStateException("Specialization 'readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)' contains a shared cache with name 'readAttributeFromDynamicObjectNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readDynamic == null) {
this.readDynamic = readDynamic_1;
}
VarHandle.storeStoreFence();
this.readObjectAttribute_cache = s2_;
state_0 = state_0 | 0b100 /* add SpecializationActive[ReadAttributeFromObjectNode.readObjectAttribute(PythonObject, Object, Node, InlinedConditionProfile, GetDictIfExistsNode, ReadAttributeFromDynamicObjectNode, HashingStorageGetItem)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, inliningTarget__1, INLINED_READ_OBJECT_ATTRIBUTE_PROFILE_HAS_DICT_, getDict__, readDynamic_1, INLINED_READ_OBJECT_ATTRIBUTE_GET_ITEM_);
}
}
}
{
Node inliningTarget__2 = null;
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
ReadNativeClassData s3_ = this.insert(new ReadNativeClassData());
inliningTarget__2 = (s3_);
ReadObjectNode getNativeDict__ = s3_.insert((ReadObjectNodeGen.create()));
Objects.requireNonNull(getNativeDict__, "Specialization 'readNativeClass(PythonAbstractNativeObject, Object, Node, ReadObjectNode, HashingStorageGetItem)' cache 'getNativeDict' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s3_.getNativeDict_ = getNativeDict__;
VarHandle.storeStoreFence();
this.readNativeClass_cache = s3_;
state_0 = state_0 | 0b1000 /* add SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromObjectTpDictNode.readNativeClass(PythonAbstractNativeObject, Object, Node, ReadObjectNode, HashingStorageGetItem)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectTpDictNode.readNativeClass(arg0Value_, arg1Value, inliningTarget__2, getNativeDict__, INLINED_READ_NATIVE_CLASS_GET_ITEM_);
}
}
{
Node inliningTarget__3 = null;
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
inliningTarget__3 = (this);
ReadAttributeFromForeign read__ = this.insert((ReadAttributeFromForeignNodeGen.create()));
Objects.requireNonNull(read__, "Specialization 'readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)' cache 'read' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.readForeign_read_ = read__;
state_0 = state_0 | 0b10000 /* add SpecializationActive[ReadAttributeFromObjectNode.readForeign(Object, Object, Node, IsForeignObjectNode, ReadAttributeFromForeign)] */;
this.state_0_ = state_0;
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, inliningTarget__3, INLINED_READ_FOREIGN_IS_FOREIGN_OBJECT_NODE_, read__);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
int state_0 = this.state_0_;
int newState_0 = (state_0 & 0b11111);
if (((oldState_0 ^ newState_0) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11111) & ((state_0 & 0b11111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static ReadAttributeFromObjectTpDictNode create() {
return new ReadAttributeFromObjectTpDictNodeGen();
}
@NeverDefault
public static ReadAttributeFromObjectTpDictNode getUncached() {
return ReadAttributeFromObjectTpDictNodeGen.UNCACHED;
}
@GeneratedBy(ReadAttributeFromObjectTpDictNode.class)
@DenyReplace
private static final class ReadFromBuiltinModuleDictData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readFromBuiltinModuleDict_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedStorageGen_
*/
@CompilationFinal TruffleWeakReference weakCachedStorageGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedDictGen_
*/
@CompilationFinal TruffleWeakReference weakCachedDictGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link TruffleWeakReference} weakCachedObjectGen_
*/
@CompilationFinal TruffleWeakReference weakCachedObjectGen__;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readFromBuiltinModuleDict}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readFromBuiltinModuleDict_getItem__field5_;
ReadFromBuiltinModuleDictData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectTpDictNode.class)
@DenyReplace
private static final class ReadObjectAttributeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link InlinedConditionProfile} profileHasDict
* Inline method: {@link InlinedConditionProfile#inline}
* 2-9: InlinedCache
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readObjectAttribute_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link GetDictIfExistsNode} getDict
*/
@Child GetDictIfExistsNode getDict_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectNode#readObjectAttribute}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readObjectAttribute_getItem__field5_;
ReadObjectAttributeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectTpDictNode.class)
@DenyReplace
private static final class ReadNativeClassData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-7: InlinedCache
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readNativeClass_state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link ReadObjectNode} getNativeDict
*/
@Child ReadObjectNode getNativeDict_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeClass_getItem__field1_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeClass_getItem__field2_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeClass_getItem__field3_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeClass_getItem__field4_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromObjectTpDictNode#readNativeClass}
* Parameter: {@link HashingStorageGetItem} getItem
* Inline method: {@link HashingStorageGetItemNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readNativeClass_getItem__field5_;
ReadNativeClassData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadAttributeFromObjectTpDictNode.class)
@DenyReplace
private static final class Uncached extends ReadAttributeFromObjectTpDictNode {
@TruffleBoundary
@Override
public Object execute(Object arg0Value, Object arg1Value) {
if (arg0Value instanceof PythonModule) {
PythonModule arg0Value_ = (PythonModule) arg0Value;
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if ((isSingleContext()) && ((arg0Value_) != null)) {
// assert ((arg0Value_) == arg0Value_);
if (((ReadAttributeFromObjectNode.getDict(arg0Value_)) != null) && ((ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))) != null) && (ReadAttributeFromObjectNode.getStorage(arg0Value_, (ReadAttributeFromObjectNode.getDict(arg0Value_))) == (ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))))) {
return ReadAttributeFromObjectNode.readFromBuiltinModuleDict(arg0Value_, arg1Value_, (this), (arg0Value_), (ReadAttributeFromObjectNode.getDict(arg0Value_)), (ReadAttributeFromObjectNode.getStorage(arg0Value_, ReadAttributeFromObjectNode.getDict(arg0Value_))), (HashingStorageGetItemNodeGen.getUncached()));
}
}
}
}
if (arg0Value instanceof PythonObject) {
PythonObject arg0Value_ = (PythonObject) arg0Value;
if (arg1Value instanceof HiddenKey) {
HiddenKey arg1Value_ = (HiddenKey) arg1Value;
return ReadAttributeFromObjectNode.readHiddenKey(arg0Value_, arg1Value_, (ReadAttributeFromDynamicObjectNode.getUncached()));
}
if ((!(ObjectAttributeNode.isHiddenKey(arg1Value)))) {
return ReadAttributeFromObjectNode.readObjectAttribute(arg0Value_, arg1Value, (this), (InlinedConditionProfile.getUncached()), (GetDictIfExistsNode.getUncached()), (ReadAttributeFromDynamicObjectNode.getUncached()), (HashingStorageGetItemNodeGen.getUncached()));
}
}
if (arg0Value instanceof PythonAbstractNativeObject) {
PythonAbstractNativeObject arg0Value_ = (PythonAbstractNativeObject) arg0Value;
return ReadAttributeFromObjectTpDictNode.readNativeClass(arg0Value_, arg1Value, (this), (ReadObjectNode.getUncached()), (HashingStorageGetItemNodeGen.getUncached()));
}
if ((!(PGuards.isPythonObject(arg0Value))) && (!(PGuards.isNativeObject(arg0Value)))) {
return ReadAttributeFromObjectNode.readForeign(arg0Value, arg1Value, (this), (IsForeignObjectNodeGen.getUncached()), (ReadAttributeFromForeignNodeGen.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link ReadAttributeFromForeign#read}
* Activation probability: 1.00000
* With/without class size: 32/12 bytes
*
*/
@GeneratedBy(ReadAttributeFromForeign.class)
@SuppressWarnings("javadoc")
protected static final class ReadAttributeFromForeignNodeGen extends ReadAttributeFromForeign {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link ReadAttributeFromForeign#read}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromForeign#read}
* Parameter: {@link CastToJavaStringNode} castNode
*/
@Child private CastToJavaStringNode castNode_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromForeign#read}
* Parameter: {@link PForeignToPTypeNode} fromForeign
*/
@Child private PForeignToPTypeNode fromForeign_;
/**
* Source Info:
* Specialization: {@link ReadAttributeFromForeign#read}
* Parameter: {@link InteropLibrary} read
*/
@Child private InteropLibrary read_;
private ReadAttributeFromForeignNodeGen() {
}
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromForeign.read(Object, Object, CastToJavaStringNode, PForeignToPTypeNode, InteropLibrary)] */) {
{
CastToJavaStringNode castNode__ = this.castNode_;
if (castNode__ != null) {
PForeignToPTypeNode fromForeign__ = this.fromForeign_;
if (fromForeign__ != null) {
InteropLibrary read__ = this.read_;
if (read__ != null) {
return ReadAttributeFromForeign.read(arg0Value, arg1Value, castNode__, fromForeign__, read__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
CastToJavaStringNode castNode__ = this.insert((CastToJavaStringNodeGen.create()));
Objects.requireNonNull(castNode__, "Specialization 'read(Object, Object, CastToJavaStringNode, PForeignToPTypeNode, InteropLibrary)' cache 'castNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.castNode_ = castNode__;
PForeignToPTypeNode fromForeign__ = this.insert((PForeignToPTypeNode.create()));
Objects.requireNonNull(fromForeign__, "Specialization 'read(Object, Object, CastToJavaStringNode, PForeignToPTypeNode, InteropLibrary)' cache 'fromForeign' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.fromForeign_ = fromForeign__;
InteropLibrary read__ = this.insert((INTEROP_LIBRARY_.createDispatched(PythonOptions.getAttributeAccessInlineCacheMaxDepth())));
Objects.requireNonNull(read__, "Specialization 'read(Object, Object, CastToJavaStringNode, PForeignToPTypeNode, InteropLibrary)' cache 'read' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
VarHandle.storeStoreFence();
this.read_ = read__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReadAttributeFromObjectNode.ReadAttributeFromForeign.read(Object, Object, CastToJavaStringNode, PForeignToPTypeNode, InteropLibrary)] */;
this.state_0_ = state_0;
return ReadAttributeFromForeign.read(arg0Value, arg1Value, castNode__, fromForeign__, read__);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
@NeverDefault
public static ReadAttributeFromForeign create() {
return new ReadAttributeFromForeignNodeGen();
}
@NeverDefault
public static ReadAttributeFromForeign getUncached() {
return ReadAttributeFromForeignNodeGen.UNCACHED;
}
@GeneratedBy(ReadAttributeFromForeign.class)
@DenyReplace
private static final class Uncached extends ReadAttributeFromForeign {
@TruffleBoundary
@Override
public Object execute(Object arg0Value, Object arg1Value) {
return ReadAttributeFromForeign.read(arg0Value, arg1Value, (CastToJavaStringNode.getUncached()), (PForeignToPTypeNode.getUncached()), (INTEROP_LIBRARY_.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy