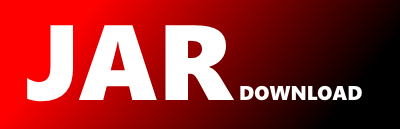
com.oracle.graal.python.nodes.bytecode.UnpackExNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.bytecode;
import com.oracle.graal.python.builtins.objects.common.SequenceNodes.GetSequenceStorageNode;
import com.oracle.graal.python.builtins.objects.common.SequenceNodesFactory.GetSequenceStorageNodeGen;
import com.oracle.graal.python.builtins.objects.common.SequenceStorageNodes.GetItemScalarNode;
import com.oracle.graal.python.builtins.objects.common.SequenceStorageNodes.GetItemSliceNode;
import com.oracle.graal.python.builtins.objects.common.SequenceStorageNodesFactory.GetItemScalarNodeGen;
import com.oracle.graal.python.lib.GetNextNode;
import com.oracle.graal.python.lib.PyObjectGetIter;
import com.oracle.graal.python.lib.PyObjectGetIterNodeGen;
import com.oracle.graal.python.nodes.PGuards;
import com.oracle.graal.python.nodes.PRaiseNode.Lazy;
import com.oracle.graal.python.nodes.PRaiseNodeGen.LazyNodeGen;
import com.oracle.graal.python.nodes.builtins.ListNodes.ConstructListNode;
import com.oracle.graal.python.nodes.object.BuiltinClassProfiles.IsBuiltinObjectProfile;
import com.oracle.graal.python.nodes.object.BuiltinClassProfilesFactory.IsBuiltinObjectProfileNodeGen;
import com.oracle.graal.python.nodes.object.GetClassNode.GetPythonObjectClassNode;
import com.oracle.graal.python.nodes.object.GetClassNodeGen.GetPythonObjectClassNodeGen;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.graal.python.runtime.sequence.PSequence;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link UnpackExNode#doUnpackSequence}
* Activation probability: 0.65000
* With/without class size: 35/30 bytes
* Specialization {@link UnpackExNode#doUnpackIterable}
* Activation probability: 0.35000
* With/without class size: 25/43 bytes
*
*/
@GeneratedBy(UnpackExNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class UnpackExNodeGen extends UnpackExNode {
private static final StateField UNPACK_SEQUENCE__UNPACK_EX_NODE_UNPACK_SEQUENCE_STATE_0_UPDATER = StateField.create(UnpackSequenceData.lookup_(), "unpackSequence_state_0_");
private static final StateField FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_0_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_0_");
private static final StateField FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_1_UPDATER = StateField.create(FallbackData.lookup_(), "fallback_state_1_");
static final ReferenceField UNPACK_SEQUENCE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "unpackSequence_cache", UnpackSequenceData.class);
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetPythonObjectClassNode} getClassNode
* Inline method: {@link GetPythonObjectClassNodeGen#inline}
*/
private static final GetPythonObjectClassNode INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_ = GetPythonObjectClassNodeGen.inline(InlineTarget.create(GetPythonObjectClassNode.class, UNPACK_SEQUENCE__UNPACK_EX_NODE_UNPACK_SEQUENCE_STATE_0_UPDATER.subUpdater(2, 4), ReferenceField.create(UnpackSequenceData.lookup_(), "unpackSequence_getClassNode__field1_", Object.class), ReferenceField.create(UnpackSequenceData.lookup_(), "unpackSequence_getClassNode__field2_", Node.class), ReferenceField.create(UnpackSequenceData.lookup_(), "unpackSequence_getClassNode__field3_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
*/
private static final GetSequenceStorageNode INLINED_UNPACK_SEQUENCE_GET_SEQUENCE_STORAGE_NODE_ = GetSequenceStorageNodeGen.inline(InlineTarget.create(GetSequenceStorageNode.class, UNPACK_SEQUENCE__UNPACK_EX_NODE_UNPACK_SEQUENCE_STATE_0_UPDATER.subUpdater(6, 3), ReferenceField.create(UnpackSequenceData.lookup_(), "unpackSequence_getSequenceStorageNode__field1_", Object.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetItemScalarNode} getItemNode
* Inline method: {@link GetItemScalarNodeGen#inline}
*/
private static final GetItemScalarNode INLINED_UNPACK_SEQUENCE_GET_ITEM_NODE_ = GetItemScalarNodeGen.inline(InlineTarget.create(GetItemScalarNode.class, UNPACK_SEQUENCE__UNPACK_EX_NODE_UNPACK_SEQUENCE_STATE_0_UPDATER.subUpdater(9, 8), ReferenceField.create(UnpackSequenceData.lookup_(), "unpackSequence_getItemNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_UNPACK_SEQUENCE_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, UNPACK_SEQUENCE__UNPACK_EX_NODE_UNPACK_SEQUENCE_STATE_0_UPDATER.subUpdater(17, 1), ReferenceField.create(UnpackSequenceData.lookup_(), "unpackSequence_raiseNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
*/
private static final PyObjectGetIter INLINED_FALLBACK_GET_ITER_ = PyObjectGetIterNodeGen.inline(InlineTarget.create(PyObjectGetIter.class, FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(0, 2), ReferenceField.create(FallbackData.lookup_(), "fallback_getIter__field1_", Node.class), ReferenceField.create(FallbackData.lookup_(), "fallback_getIter__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link IsBuiltinObjectProfile} notIterableProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_FALLBACK_NOT_ITERABLE_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(2, 20), ReferenceField.create(FallbackData.lookup_(), "fallback_notIterableProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*/
private static final IsBuiltinObjectProfile INLINED_FALLBACK_STOP_ITERATION_PROFILE_ = IsBuiltinObjectProfileNodeGen.inline(InlineTarget.create(IsBuiltinObjectProfile.class, FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_1_UPDATER.subUpdater(0, 20), ReferenceField.create(FallbackData.lookup_(), "fallback_stopIterationProfile__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link GetItemScalarNode} getItemNode
* Inline method: {@link GetItemScalarNodeGen#inline}
*/
private static final GetItemScalarNode INLINED_FALLBACK_GET_ITEM_NODE_ = GetItemScalarNodeGen.inline(InlineTarget.create(GetItemScalarNode.class, FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(22, 8), ReferenceField.create(FallbackData.lookup_(), "fallback_getItemNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*/
private static final Lazy INLINED_FALLBACK_RAISE_NODE_ = LazyNodeGen.inline(InlineTarget.create(Lazy.class, FALLBACK__UNPACK_EX_NODE_FALLBACK_STATE_0_UPDATER.subUpdater(30, 1), ReferenceField.create(FallbackData.lookup_(), "fallback_raiseNode__field1_", Node.class)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link UnpackExNode#doUnpackSequence}
* 1: SpecializationActive {@link UnpackExNode#doUnpackIterable}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory;
@UnsafeAccessedField @Child private UnpackSequenceData unpackSequence_cache;
@Child private FallbackData fallback_cache;
private UnpackExNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, int arg0Value, Object arg1Value, int arg2Value, int arg3Value) {
if (arg1Value instanceof PSequence) {
UnpackSequenceData s0_ = this.unpackSequence_cache;
PSequence arg1Value_ = (PSequence) arg1Value;
if ((s0_ == null || ((s0_.unpackSequence_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy), guardIndex=0] */ || (PGuards.cannotBeOverridden(arg1Value_, (s0_), INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_))) && (s0_ == null || ((s0_.unpackSequence_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy), guardIndex=0] */ || (!(PGuards.isPString(arg1Value_))))) {
return false;
}
}
return true;
}
@Override
public int execute(Frame frameValue, int arg0Value, Object arg1Value, int arg2Value, int arg3Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)] || SpecializationActive[UnpackExNode.doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)] */ && arg1Value instanceof PSequence) {
PSequence arg1Value_ = (PSequence) arg1Value;
UnpackSequenceData s0_ = this.unpackSequence_cache;
if (s0_ != null) {
if ((s0_.unpackSequence_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
Node inliningTarget__ = (s0_);
if ((PGuards.cannotBeOverridden(arg1Value_, inliningTarget__, INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_)) && (!(PGuards.isPString(arg1Value_)))) {
return UnpackExNode.doUnpackSequence((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, inliningTarget__, INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_, INLINED_UNPACK_SEQUENCE_GET_SEQUENCE_STORAGE_NODE_, INLINED_UNPACK_SEQUENCE_GET_ITEM_NODE_, s0_.getItemSliceNode_, factory_, INLINED_UNPACK_SEQUENCE_RAISE_NODE_);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[UnpackExNode.doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)] */) {
FallbackData s1_ = this.fallback_cache;
if (s1_ != null) {
{
PythonObjectFactory factory_1 = this.factory;
if (factory_1 != null) {
Node inliningTarget__1 = (s1_);
if (fallbackGuard_(state_0, arg0Value, arg1Value, arg2Value, arg3Value)) {
return UnpackExNode.doUnpackIterable((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, inliningTarget__1, INLINED_FALLBACK_GET_ITER_, s1_.getNextNode_, INLINED_FALLBACK_NOT_ITERABLE_PROFILE_, INLINED_FALLBACK_STOP_ITERATION_PROFILE_, s1_.constructListNode_, INLINED_FALLBACK_GET_ITEM_NODE_, s1_.getItemSliceNode_, factory_1, INLINED_FALLBACK_RAISE_NODE_);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(frameValue, arg0Value, arg1Value, arg2Value, arg3Value);
}
private int executeAndSpecialize(Frame frameValue, int arg0Value, Object arg1Value, int arg2Value, int arg3Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
if (arg1Value instanceof PSequence) {
PSequence arg1Value_ = (PSequence) arg1Value;
while (true) {
int count0_ = 0;
UnpackSequenceData s0_ = UNPACK_SEQUENCE_CACHE_UPDATER.getVolatile(this);
UnpackSequenceData s0_original = s0_;
while (s0_ != null) {
if ((s0_.unpackSequence_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
inliningTarget__ = (s0_);
if ((PGuards.cannotBeOverridden(arg1Value_, inliningTarget__, INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_)) && (!(PGuards.isPString(arg1Value_)))) {
break;
}
}
}
if ((s0_.unpackSequence_state_0_ & 0b10) != 0 /* is SpecializationCachesInitialized */) {
count0_++;
}
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
s0_ = this.insert(new UnpackSequenceData());
inliningTarget__ = (s0_);
if (((s0_.unpackSequence_state_0_ & 0b1)) == 0 /* is-not GuardActive[specialization=UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy), guardIndex=0] */) {
s0_.unpackSequence_state_0_ = s0_.unpackSequence_state_0_ | 0b1 /* add GuardActive[specialization=UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy), guardIndex=0] */;
if (!UNPACK_SEQUENCE_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
s0_original = s0_;
s0_ = this.insert(new UnpackSequenceData(s0_));
}
if ((PGuards.cannotBeOverridden(arg1Value_, inliningTarget__, INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_)) && (!(PGuards.isPString(arg1Value_)))) {
GetItemSliceNode getItemSliceNode__ = s0_.insert((GetItemSliceNode.create()));
Objects.requireNonNull(getItemSliceNode__, "Specialization 'doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)' cache 'getItemSliceNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.getItemSliceNode_ = getItemSliceNode__;
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory;
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = s0_.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_;
}
s0_.unpackSequence_state_0_ = s0_.unpackSequence_state_0_ | 0b10 /* add SpecializationCachesInitialized */;
if (!UNPACK_SEQUENCE_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[UnpackExNode.doUnpackSequence(VirtualFrame, int, PSequence, int, int, Node, GetPythonObjectClassNode, GetSequenceStorageNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)] */;
this.state_0_ = state_0;
} else {
s0_ = null;
}
}
}
if (s0_ != null) {
return UnpackExNode.doUnpackSequence((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, inliningTarget__, INLINED_UNPACK_SEQUENCE_GET_CLASS_NODE_, INLINED_UNPACK_SEQUENCE_GET_SEQUENCE_STORAGE_NODE_, INLINED_UNPACK_SEQUENCE_GET_ITEM_NODE_, s0_.getItemSliceNode_, this.factory, INLINED_UNPACK_SEQUENCE_RAISE_NODE_);
}
break;
}
}
}
{
Node inliningTarget__1 = null;
FallbackData s1_ = this.insert(new FallbackData());
inliningTarget__1 = (s1_);
GetNextNode getNextNode__ = s1_.insert((GetNextNode.create()));
Objects.requireNonNull(getNextNode__, "Specialization 'doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)' cache 'getNextNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.getNextNode_ = getNextNode__;
ConstructListNode constructListNode__ = s1_.insert((ConstructListNode.create()));
Objects.requireNonNull(constructListNode__, "Specialization 'doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)' cache 'constructListNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.constructListNode_ = constructListNode__;
GetItemSliceNode getItemSliceNode__1 = s1_.insert((GetItemSliceNode.create()));
Objects.requireNonNull(getItemSliceNode__1, "Specialization 'doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)' cache 'getItemSliceNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s1_.getItemSliceNode_ = getItemSliceNode__1;
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory;
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = s1_.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_1;
}
VarHandle.storeStoreFence();
this.fallback_cache = s1_;
state_0 = state_0 | 0b10 /* add SpecializationActive[UnpackExNode.doUnpackIterable(VirtualFrame, int, Object, int, int, Node, PyObjectGetIter, GetNextNode, IsBuiltinObjectProfile, IsBuiltinObjectProfile, ConstructListNode, GetItemScalarNode, GetItemSliceNode, PythonObjectFactory, Lazy)] */;
this.state_0_ = state_0;
return UnpackExNode.doUnpackIterable((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, inliningTarget__1, INLINED_FALLBACK_GET_ITER_, getNextNode__, INLINED_FALLBACK_NOT_ITERABLE_PROFILE_, INLINED_FALLBACK_STOP_ITERATION_PROFILE_, constructListNode__, INLINED_FALLBACK_GET_ITEM_NODE_, getItemSliceNode__1, factory_1, INLINED_FALLBACK_RAISE_NODE_);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static UnpackExNode create() {
return new UnpackExNodeGen();
}
@NeverDefault
public static UnpackExNode getUncached() {
return UnpackExNodeGen.UNCACHED;
}
@GeneratedBy(UnpackExNode.class)
@DenyReplace
private static final class UnpackSequenceData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0: GuardActive[guardIndex=0] {@link UnpackExNode#doUnpackSequence}
* 1: SpecializationCachesInitialized {@link UnpackExNode#doUnpackSequence}
* 2-5: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetPythonObjectClassNode} getClassNode
* Inline method: {@link GetPythonObjectClassNodeGen#inline}
* 6-8: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
* 9-16: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetItemScalarNode} getItemNode
* Inline method: {@link GetItemScalarNodeGen#inline}
* 17: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int unpackSequence_state_0_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetPythonObjectClassNode} getClassNode
* Inline method: {@link GetPythonObjectClassNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object unpackSequence_getClassNode__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetPythonObjectClassNode} getClassNode
* Inline method: {@link GetPythonObjectClassNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node unpackSequence_getClassNode__field2_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetPythonObjectClassNode} getClassNode
* Inline method: {@link GetPythonObjectClassNodeGen#inline}
* Inline field: {@link Node} field3
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node unpackSequence_getClassNode__field3_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object unpackSequence_getSequenceStorageNode__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetItemScalarNode} getItemNode
* Inline method: {@link GetItemScalarNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node unpackSequence_getItemNode__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link GetItemSliceNode} getItemSliceNode
*/
@Child GetItemSliceNode getItemSliceNode_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackSequence}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node unpackSequence_raiseNode__field1_;
UnpackSequenceData() {
}
UnpackSequenceData(UnpackSequenceData delegate) {
this.unpackSequence_state_0_ = delegate.unpackSequence_state_0_;
this.unpackSequence_getClassNode__field1_ = delegate.unpackSequence_getClassNode__field1_;
this.unpackSequence_getClassNode__field2_ = delegate.unpackSequence_getClassNode__field2_;
this.unpackSequence_getClassNode__field3_ = delegate.unpackSequence_getClassNode__field3_;
this.unpackSequence_getSequenceStorageNode__field1_ = delegate.unpackSequence_getSequenceStorageNode__field1_;
this.unpackSequence_getItemNode__field1_ = delegate.unpackSequence_getItemNode__field1_;
this.getItemSliceNode_ = delegate.getItemSliceNode_;
this.unpackSequence_raiseNode__field1_ = delegate.unpackSequence_raiseNode__field1_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(UnpackExNode.class)
@DenyReplace
private static final class FallbackData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* 2-21: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link IsBuiltinObjectProfile} notIterableProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* 22-29: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link GetItemScalarNode} getItemNode
* Inline method: {@link GetItemScalarNodeGen#inline}
* 30: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_0_;
/**
* State Info:
* 0-19: InlinedCache
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int fallback_state_1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getIter__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link PyObjectGetIter} getIter
* Inline method: {@link PyObjectGetIterNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getIter__field2_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link GetNextNode} getNextNode
*/
@Child GetNextNode getNextNode_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link IsBuiltinObjectProfile} notIterableProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_notIterableProfile__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link IsBuiltinObjectProfile} stopIterationProfile
* Inline method: {@link IsBuiltinObjectProfileNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_stopIterationProfile__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link ConstructListNode} constructListNode
*/
@Child ConstructListNode constructListNode_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link GetItemScalarNode} getItemNode
* Inline method: {@link GetItemScalarNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_getItemNode__field1_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link GetItemSliceNode} getItemSliceNode
*/
@Child GetItemSliceNode getItemSliceNode_;
/**
* Source Info:
* Specialization: {@link UnpackExNode#doUnpackIterable}
* Parameter: {@link Lazy} raiseNode
* Inline method: {@link LazyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node fallback_raiseNode__field1_;
FallbackData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(UnpackExNode.class)
@DenyReplace
private static final class Uncached extends UnpackExNode {
@Override
public int execute(Frame frameValue, int arg0Value, Object arg1Value, int arg2Value, int arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if (arg1Value instanceof PSequence) {
PSequence arg1Value_ = (PSequence) arg1Value;
if ((PGuards.cannotBeOverridden(arg1Value_, (this), (GetPythonObjectClassNodeGen.getUncached()))) && (!(PGuards.isPString(arg1Value_)))) {
return UnpackExNode.doUnpackSequence((VirtualFrame) frameValue, arg0Value, arg1Value_, arg2Value, arg3Value, (this), (GetPythonObjectClassNodeGen.getUncached()), (GetSequenceStorageNode.getUncached()), (GetItemScalarNodeGen.getUncached()), (GetItemSliceNode.getUncached()), (PythonObjectFactory.getUncached()), (Lazy.getUncached()));
}
}
return UnpackExNode.doUnpackIterable((VirtualFrame) frameValue, arg0Value, arg1Value, arg2Value, arg3Value, (this), (PyObjectGetIter.getUncached()), (GetNextNode.getUncached()), (IsBuiltinObjectProfile.getUncached()), (IsBuiltinObjectProfile.getUncached()), (ConstructListNode.getUncached()), (GetItemScalarNodeGen.getUncached()), (GetItemSliceNode.getUncached()), (PythonObjectFactory.getUncached()), (Lazy.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy